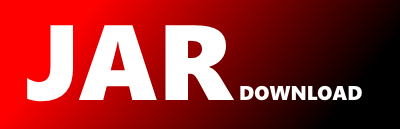
com.amazonaws.services.wafv2.model.TextTransformation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wafv2 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wafv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
*
* This is the latest version of AWS WAF, named AWS WAFV2, released in November, 2019. For information, including
* how to migrate your AWS WAF resources from the prior release, see the AWS WAF Developer Guide.
*
*
*
* Text transformations eliminate some of the unusual formatting that attackers use in web requests in an effort to
* bypass detection.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TextTransformation implements Serializable, Cloneable, StructuredPojo {
/**
*
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS WAF
* processes all transformations, from lowest priority to highest, before inspecting the transformed content. The
* priorities don't need to be consecutive, but they must all be different.
*
*/
private Integer priority;
/**
*
* You can specify the following transformation types:
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using unusual
* formatting to disguise some or all of the command, use this option to perform the following transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters. HTML_ENTITY_DECODE
* performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
*
*/
private String type;
/**
*
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS WAF
* processes all transformations, from lowest priority to highest, before inspecting the transformed content. The
* priorities don't need to be consecutive, but they must all be different.
*
*
* @param priority
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS
* WAF processes all transformations, from lowest priority to highest, before inspecting the transformed
* content. The priorities don't need to be consecutive, but they must all be different.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS WAF
* processes all transformations, from lowest priority to highest, before inspecting the transformed content. The
* priorities don't need to be consecutive, but they must all be different.
*
*
* @return Sets the relative processing order for multiple transformations that are defined for a rule statement.
* AWS WAF processes all transformations, from lowest priority to highest, before inspecting the transformed
* content. The priorities don't need to be consecutive, but they must all be different.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS WAF
* processes all transformations, from lowest priority to highest, before inspecting the transformed content. The
* priorities don't need to be consecutive, but they must all be different.
*
*
* @param priority
* Sets the relative processing order for multiple transformations that are defined for a rule statement. AWS
* WAF processes all transformations, from lowest priority to highest, before inspecting the transformed
* content. The priorities don't need to be consecutive, but they must all be different.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TextTransformation withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* You can specify the following transformation types:
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using unusual
* formatting to disguise some or all of the command, use this option to perform the following transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters. HTML_ENTITY_DECODE
* performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
*
*
* @param type
* You can specify the following transformation types:
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using
* unusual formatting to disguise some or all of the command, use this option to perform the following
* transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters.
* HTML_ENTITY_DECODE
performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
* @see TextTransformationType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* You can specify the following transformation types:
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using unusual
* formatting to disguise some or all of the command, use this option to perform the following transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters. HTML_ENTITY_DECODE
* performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
*
*
* @return You can specify the following transformation types:
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using
* unusual formatting to disguise some or all of the command, use this option to perform the following
* transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters.
* HTML_ENTITY_DECODE
performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
* @see TextTransformationType
*/
public String getType() {
return this.type;
}
/**
*
* You can specify the following transformation types:
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using unusual
* formatting to disguise some or all of the command, use this option to perform the following transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters. HTML_ENTITY_DECODE
* performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
*
*
* @param type
* You can specify the following transformation types:
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using
* unusual formatting to disguise some or all of the command, use this option to perform the following
* transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters.
* HTML_ENTITY_DECODE
performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TextTransformationType
*/
public TextTransformation withType(String type) {
setType(type);
return this;
}
/**
*
* You can specify the following transformation types:
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using unusual
* formatting to disguise some or all of the command, use this option to perform the following transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters. HTML_ENTITY_DECODE
* performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
*
*
* @param type
* You can specify the following transformation types:
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system command line command and using
* unusual formatting to disguise some or all of the command, use this option to perform the following
* transformations:
*
*
* -
*
* Delete the following characters: \ " ' ^
*
*
* -
*
* Delete spaces before the following characters: / (
*
*
* -
*
* Replace the following characters with a space: , ;
*
*
* -
*
* Replace multiple spaces with one space
*
*
* -
*
* Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space character (decimal 32):
*
*
* -
*
* \f, formfeed, decimal 12
*
*
* -
*
* \t, tab, decimal 9
*
*
* -
*
* \n, newline, decimal 10
*
*
* -
*
* \r, carriage return, decimal 13
*
*
* -
*
* \v, vertical tab, decimal 11
*
*
* -
*
* non-breaking space, decimal 160
*
*
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded characters.
* HTML_ENTITY_DECODE
performs the following operations:
*
*
* -
*
* Replaces (ampersand)quot;
with "
*
*
* -
*
* Replaces (ampersand)nbsp;
with a non-breaking space, decimal 160
*
*
* -
*
* Replaces (ampersand)lt;
with a "less than" symbol
*
*
* -
*
* Replaces (ampersand)gt;
with >
*
*
* -
*
* Replaces characters that are represented in hexadecimal format, (ampersand)#xhhhh;
, with the
* corresponding characters
*
*
* -
*
* Replaces characters that are represented in decimal format, (ampersand)#nnnn;
, with the
* corresponding characters
*
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want any text transformations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TextTransformationType
*/
public TextTransformation withType(TextTransformationType type) {
this.type = type.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TextTransformation == false)
return false;
TextTransformation other = (TextTransformation) obj;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
return hashCode;
}
@Override
public TextTransformation clone() {
try {
return (TextTransformation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.wafv2.model.transform.TextTransformationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}