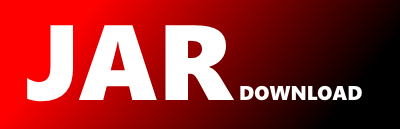
com.amazonaws.services.wafv2.model.ManagedRuleGroupConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wafv2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wafv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Additional information that's used by a managed rule group. Many managed rule groups don't require this.
*
*
* Use the AWSManagedRulesATPRuleSet
configuration object for the account takeover prevention managed rule
* group, to provide information such as the sign-in page of your application and the type of content to accept or
* reject from the client.
*
*
* Use the AWSManagedRulesBotControlRuleSet
configuration object to configure the protection level that you
* want the Bot Control rule group to use.
*
*
* For example specifications, see the examples section of CreateWebACL.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ManagedRuleGroupConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*
*/
@Deprecated
private String loginPath;
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*/
@Deprecated
private String payloadType;
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*/
@Deprecated
private UsernameField usernameField;
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*/
@Deprecated
private PasswordField passwordField;
/**
*
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection level
* that you want to use. For information about using the Bot Control managed rule group, see WAF Bot Control
* rule group and WAF Bot
* Control in the WAF Developer Guide.
*
*/
private AWSManagedRulesBotControlRuleSet aWSManagedRulesBotControlRuleSet;
/**
*
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group. For web
* ACLs that protect CloudFront distributions, use this to also provide the information about how your distribution
* responds to login requests.
*
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud Control
* account takeover prevention (ATP) rule group and WAF Fraud Control account takeover
* prevention (ATP) in the WAF Developer Guide.
*
*/
private AWSManagedRulesATPRuleSet aWSManagedRulesATPRuleSet;
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*
*
* @param loginPath
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*/
@Deprecated
public void setLoginPath(String loginPath) {
this.loginPath = loginPath;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*
*
* @return
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*/
@Deprecated
public String getLoginPath() {
return this.loginPath;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
*
*
* @param loginPath
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ManagedRuleGroupConfig withLoginPath(String loginPath) {
setLoginPath(loginPath);
return this;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param payloadType
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @see PayloadType
*/
@Deprecated
public void setPayloadType(String payloadType) {
this.payloadType = payloadType;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @return
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @see PayloadType
*/
@Deprecated
public String getPayloadType() {
return this.payloadType;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param payloadType
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PayloadType
*/
@Deprecated
public ManagedRuleGroupConfig withPayloadType(String payloadType) {
setPayloadType(payloadType);
return this;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param payloadType
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PayloadType
*/
@Deprecated
public ManagedRuleGroupConfig withPayloadType(PayloadType payloadType) {
this.payloadType = payloadType.toString();
return this;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param usernameField
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*/
@Deprecated
public void setUsernameField(UsernameField usernameField) {
this.usernameField = usernameField;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @return
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*/
@Deprecated
public UsernameField getUsernameField() {
return this.usernameField;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param usernameField
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ManagedRuleGroupConfig withUsernameField(UsernameField usernameField) {
setUsernameField(usernameField);
return this;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param passwordField
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*/
@Deprecated
public void setPasswordField(PasswordField passwordField) {
this.passwordField = passwordField;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @return
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*/
@Deprecated
public PasswordField getPasswordField() {
return this.passwordField;
}
/**
*
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
*
*
* @param passwordField
*
* Instead of this setting, provide your configuration under AWSManagedRulesATPRuleSet
* RequestInspection
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ManagedRuleGroupConfig withPasswordField(PasswordField passwordField) {
setPasswordField(passwordField);
return this;
}
/**
*
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection level
* that you want to use. For information about using the Bot Control managed rule group, see WAF Bot Control
* rule group and WAF Bot
* Control in the WAF Developer Guide.
*
*
* @param aWSManagedRulesBotControlRuleSet
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection
* level that you want to use. For information about using the Bot Control managed rule group, see WAF Bot
* Control rule group and WAF Bot Control in
* the WAF Developer Guide.
*/
public void setAWSManagedRulesBotControlRuleSet(AWSManagedRulesBotControlRuleSet aWSManagedRulesBotControlRuleSet) {
this.aWSManagedRulesBotControlRuleSet = aWSManagedRulesBotControlRuleSet;
}
/**
*
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection level
* that you want to use. For information about using the Bot Control managed rule group, see WAF Bot Control
* rule group and WAF Bot
* Control in the WAF Developer Guide.
*
*
* @return Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection
* level that you want to use. For information about using the Bot Control managed rule group, see WAF Bot
* Control rule group and WAF Bot Control in
* the WAF Developer Guide.
*/
public AWSManagedRulesBotControlRuleSet getAWSManagedRulesBotControlRuleSet() {
return this.aWSManagedRulesBotControlRuleSet;
}
/**
*
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection level
* that you want to use. For information about using the Bot Control managed rule group, see WAF Bot Control
* rule group and WAF Bot
* Control in the WAF Developer Guide.
*
*
* @param aWSManagedRulesBotControlRuleSet
* Additional configuration for using the Bot Control managed rule group. Use this to specify the inspection
* level that you want to use. For information about using the Bot Control managed rule group, see WAF Bot
* Control rule group and WAF Bot Control in
* the WAF Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ManagedRuleGroupConfig withAWSManagedRulesBotControlRuleSet(AWSManagedRulesBotControlRuleSet aWSManagedRulesBotControlRuleSet) {
setAWSManagedRulesBotControlRuleSet(aWSManagedRulesBotControlRuleSet);
return this;
}
/**
*
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group. For web
* ACLs that protect CloudFront distributions, use this to also provide the information about how your distribution
* responds to login requests.
*
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud Control
* account takeover prevention (ATP) rule group and WAF Fraud Control account takeover
* prevention (ATP) in the WAF Developer Guide.
*
*
* @param aWSManagedRulesATPRuleSet
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group.
* For web ACLs that protect CloudFront distributions, use this to also provide the information about how
* your distribution responds to login requests.
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud
* Control account takeover prevention (ATP) rule group and WAF Fraud Control account
* takeover prevention (ATP) in the WAF Developer Guide.
*/
public void setAWSManagedRulesATPRuleSet(AWSManagedRulesATPRuleSet aWSManagedRulesATPRuleSet) {
this.aWSManagedRulesATPRuleSet = aWSManagedRulesATPRuleSet;
}
/**
*
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group. For web
* ACLs that protect CloudFront distributions, use this to also provide the information about how your distribution
* responds to login requests.
*
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud Control
* account takeover prevention (ATP) rule group and WAF Fraud Control account takeover
* prevention (ATP) in the WAF Developer Guide.
*
*
* @return Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group.
* For web ACLs that protect CloudFront distributions, use this to also provide the information about how
* your distribution responds to login requests.
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
* and provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud
* Control account takeover prevention (ATP) rule group and WAF Fraud Control account
* takeover prevention (ATP) in the WAF Developer Guide.
*/
public AWSManagedRulesATPRuleSet getAWSManagedRulesATPRuleSet() {
return this.aWSManagedRulesATPRuleSet;
}
/**
*
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group. For web
* ACLs that protect CloudFront distributions, use this to also provide the information about how your distribution
* responds to login requests.
*
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud Control
* account takeover prevention (ATP) rule group and WAF Fraud Control account takeover
* prevention (ATP) in the WAF Developer Guide.
*
*
* @param aWSManagedRulesATPRuleSet
* Additional configuration for using the account takeover prevention (ATP) managed rule group,
* AWSManagedRulesATPRuleSet
. Use this to provide login request information to the rule group.
* For web ACLs that protect CloudFront distributions, use this to also provide the information about how
* your distribution responds to login requests.
*
* This configuration replaces the individual configuration fields in ManagedRuleGroupConfig
and
* provides additional feature configuration.
*
*
* For information about using the ATP managed rule group, see WAF Fraud
* Control account takeover prevention (ATP) rule group and WAF Fraud Control account
* takeover prevention (ATP) in the WAF Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ManagedRuleGroupConfig withAWSManagedRulesATPRuleSet(AWSManagedRulesATPRuleSet aWSManagedRulesATPRuleSet) {
setAWSManagedRulesATPRuleSet(aWSManagedRulesATPRuleSet);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLoginPath() != null)
sb.append("LoginPath: ").append(getLoginPath()).append(",");
if (getPayloadType() != null)
sb.append("PayloadType: ").append(getPayloadType()).append(",");
if (getUsernameField() != null)
sb.append("UsernameField: ").append(getUsernameField()).append(",");
if (getPasswordField() != null)
sb.append("PasswordField: ").append(getPasswordField()).append(",");
if (getAWSManagedRulesBotControlRuleSet() != null)
sb.append("AWSManagedRulesBotControlRuleSet: ").append(getAWSManagedRulesBotControlRuleSet()).append(",");
if (getAWSManagedRulesATPRuleSet() != null)
sb.append("AWSManagedRulesATPRuleSet: ").append(getAWSManagedRulesATPRuleSet());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ManagedRuleGroupConfig == false)
return false;
ManagedRuleGroupConfig other = (ManagedRuleGroupConfig) obj;
if (other.getLoginPath() == null ^ this.getLoginPath() == null)
return false;
if (other.getLoginPath() != null && other.getLoginPath().equals(this.getLoginPath()) == false)
return false;
if (other.getPayloadType() == null ^ this.getPayloadType() == null)
return false;
if (other.getPayloadType() != null && other.getPayloadType().equals(this.getPayloadType()) == false)
return false;
if (other.getUsernameField() == null ^ this.getUsernameField() == null)
return false;
if (other.getUsernameField() != null && other.getUsernameField().equals(this.getUsernameField()) == false)
return false;
if (other.getPasswordField() == null ^ this.getPasswordField() == null)
return false;
if (other.getPasswordField() != null && other.getPasswordField().equals(this.getPasswordField()) == false)
return false;
if (other.getAWSManagedRulesBotControlRuleSet() == null ^ this.getAWSManagedRulesBotControlRuleSet() == null)
return false;
if (other.getAWSManagedRulesBotControlRuleSet() != null
&& other.getAWSManagedRulesBotControlRuleSet().equals(this.getAWSManagedRulesBotControlRuleSet()) == false)
return false;
if (other.getAWSManagedRulesATPRuleSet() == null ^ this.getAWSManagedRulesATPRuleSet() == null)
return false;
if (other.getAWSManagedRulesATPRuleSet() != null && other.getAWSManagedRulesATPRuleSet().equals(this.getAWSManagedRulesATPRuleSet()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLoginPath() == null) ? 0 : getLoginPath().hashCode());
hashCode = prime * hashCode + ((getPayloadType() == null) ? 0 : getPayloadType().hashCode());
hashCode = prime * hashCode + ((getUsernameField() == null) ? 0 : getUsernameField().hashCode());
hashCode = prime * hashCode + ((getPasswordField() == null) ? 0 : getPasswordField().hashCode());
hashCode = prime * hashCode + ((getAWSManagedRulesBotControlRuleSet() == null) ? 0 : getAWSManagedRulesBotControlRuleSet().hashCode());
hashCode = prime * hashCode + ((getAWSManagedRulesATPRuleSet() == null) ? 0 : getAWSManagedRulesATPRuleSet().hashCode());
return hashCode;
}
@Override
public ManagedRuleGroupConfig clone() {
try {
return (ManagedRuleGroupConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.wafv2.model.transform.ManagedRuleGroupConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}