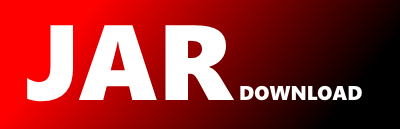
com.amazonaws.services.wafv2.model.CreateRuleGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wafv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wafv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateRuleGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the rule group. You cannot change the name of a rule group after you create it.
*
*/
private String name;
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*/
private String scope;
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you add or
* modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of rules using
* CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*/
private Long capacity;
/**
*
* A description of the rule group that helps with identification.
*
*/
private String description;
/**
*
* The Rule statements used to identify the web requests that you want to manage. Each rule includes one
* top-level statement that WAF uses to identify matching web requests, and parameters that govern how WAF handles
* them.
*
*/
private java.util.List rules;
/**
*
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*
*/
private VisibilityConfig visibilityConfig;
/**
*
* An array of key:value pairs to associate with the resource.
*
*/
private java.util.List tags;
/**
*
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send a
* custom response to the web request. You define these for the rule group, and then use them in the rules that you
* define in the rule group.
*
*
* For information about customizing web requests and responses, see Customizing web
* requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF Developer
* Guide.
*
*/
private java.util.Map customResponseBodies;
/**
*
* The name of the rule group. You cannot change the name of a rule group after you create it.
*
*
* @param name
* The name of the rule group. You cannot change the name of a rule group after you create it.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the rule group. You cannot change the name of a rule group after you create it.
*
*
* @return The name of the rule group. You cannot change the name of a rule group after you create it.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the rule group. You cannot change the name of a rule group after you create it.
*
*
* @param name
* The name of the rule group. You cannot change the name of a rule group after you create it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @see Scope
*/
public void setScope(String scope) {
this.scope = scope;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @return Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @see Scope
*/
public String getScope() {
return this.scope;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public CreateRuleGroupRequest withScope(String scope) {
setScope(scope);
return this;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public CreateRuleGroupRequest withScope(Scope scope) {
this.scope = scope.toString();
return this;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you add or
* modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of rules using
* CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @param capacity
* The web ACL capacity units (WCUs) required for this rule group.
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you
* add or modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of
* rules using CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost
* of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more
* processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage
* when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
*/
public void setCapacity(Long capacity) {
this.capacity = capacity;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you add or
* modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of rules using
* CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @return The web ACL capacity units (WCUs) required for this rule group.
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you
* add or modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of
* rules using CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative
* cost of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use
* more processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU
* usage when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
*/
public Long getCapacity() {
return this.capacity;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you add or
* modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of rules using
* CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @param capacity
* The web ACL capacity units (WCUs) required for this rule group.
*
* When you create your own rule group, you define this, and you cannot change it after creation. When you
* add or modify the rules in a rule group, WAF enforces this limit. You can check the capacity for a set of
* rules using CheckCapacity.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost
* of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more
* processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage
* when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withCapacity(Long capacity) {
setCapacity(capacity);
return this;
}
/**
*
* A description of the rule group that helps with identification.
*
*
* @param description
* A description of the rule group that helps with identification.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the rule group that helps with identification.
*
*
* @return A description of the rule group that helps with identification.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the rule group that helps with identification.
*
*
* @param description
* A description of the rule group that helps with identification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Rule statements used to identify the web requests that you want to manage. Each rule includes one
* top-level statement that WAF uses to identify matching web requests, and parameters that govern how WAF handles
* them.
*
*
* @return The Rule statements used to identify the web requests that you want to manage. Each rule includes
* one top-level statement that WAF uses to identify matching web requests, and parameters that govern how
* WAF handles them.
*/
public java.util.List getRules() {
return rules;
}
/**
*
* The Rule statements used to identify the web requests that you want to manage. Each rule includes one
* top-level statement that WAF uses to identify matching web requests, and parameters that govern how WAF handles
* them.
*
*
* @param rules
* The Rule statements used to identify the web requests that you want to manage. Each rule includes
* one top-level statement that WAF uses to identify matching web requests, and parameters that govern how
* WAF handles them.
*/
public void setRules(java.util.Collection rules) {
if (rules == null) {
this.rules = null;
return;
}
this.rules = new java.util.ArrayList(rules);
}
/**
*
* The Rule statements used to identify the web requests that you want to manage. Each rule includes one
* top-level statement that WAF uses to identify matching web requests, and parameters that govern how WAF handles
* them.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRules(java.util.Collection)} or {@link #withRules(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param rules
* The Rule statements used to identify the web requests that you want to manage. Each rule includes
* one top-level statement that WAF uses to identify matching web requests, and parameters that govern how
* WAF handles them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withRules(Rule... rules) {
if (this.rules == null) {
setRules(new java.util.ArrayList(rules.length));
}
for (Rule ele : rules) {
this.rules.add(ele);
}
return this;
}
/**
*
* The Rule statements used to identify the web requests that you want to manage. Each rule includes one
* top-level statement that WAF uses to identify matching web requests, and parameters that govern how WAF handles
* them.
*
*
* @param rules
* The Rule statements used to identify the web requests that you want to manage. Each rule includes
* one top-level statement that WAF uses to identify matching web requests, and parameters that govern how
* WAF handles them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withRules(java.util.Collection rules) {
setRules(rules);
return this;
}
/**
*
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*
*
* @param visibilityConfig
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*/
public void setVisibilityConfig(VisibilityConfig visibilityConfig) {
this.visibilityConfig = visibilityConfig;
}
/**
*
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*
*
* @return Defines and enables Amazon CloudWatch metrics and web request sample collection.
*/
public VisibilityConfig getVisibilityConfig() {
return this.visibilityConfig;
}
/**
*
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*
*
* @param visibilityConfig
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withVisibilityConfig(VisibilityConfig visibilityConfig) {
setVisibilityConfig(visibilityConfig);
return this;
}
/**
*
* An array of key:value pairs to associate with the resource.
*
*
* @return An array of key:value pairs to associate with the resource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key:value pairs to associate with the resource.
*
*
* @param tags
* An array of key:value pairs to associate with the resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key:value pairs to associate with the resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key:value pairs to associate with the resource.
*
*
* @param tags
* An array of key:value pairs to associate with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send a
* custom response to the web request. You define these for the rule group, and then use them in the rules that you
* define in the rule group.
*
*
* For information about customizing web requests and responses, see Customizing web
* requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF Developer
* Guide.
*
*
* @return A map of custom response keys and content bodies. When you create a rule with a block action, you can
* send a custom response to the web request. You define these for the rule group, and then use them in the
* rules that you define in the rule group.
*
* For information about customizing web requests and responses, see Customizing
* web requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF
* Developer Guide.
*/
public java.util.Map getCustomResponseBodies() {
return customResponseBodies;
}
/**
*
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send a
* custom response to the web request. You define these for the rule group, and then use them in the rules that you
* define in the rule group.
*
*
* For information about customizing web requests and responses, see Customizing web
* requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF Developer
* Guide.
*
*
* @param customResponseBodies
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send
* a custom response to the web request. You define these for the rule group, and then use them in the rules
* that you define in the rule group.
*
* For information about customizing web requests and responses, see Customizing
* web requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF
* Developer Guide.
*/
public void setCustomResponseBodies(java.util.Map customResponseBodies) {
this.customResponseBodies = customResponseBodies;
}
/**
*
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send a
* custom response to the web request. You define these for the rule group, and then use them in the rules that you
* define in the rule group.
*
*
* For information about customizing web requests and responses, see Customizing web
* requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF Developer
* Guide.
*
*
* @param customResponseBodies
* A map of custom response keys and content bodies. When you create a rule with a block action, you can send
* a custom response to the web request. You define these for the rule group, and then use them in the rules
* that you define in the rule group.
*
* For information about customizing web requests and responses, see Customizing
* web requests and responses in WAF in the WAF Developer Guide.
*
*
* For information about the limits on count and size for custom request and response settings, see WAF quotas in the WAF
* Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest withCustomResponseBodies(java.util.Map customResponseBodies) {
setCustomResponseBodies(customResponseBodies);
return this;
}
/**
* Add a single CustomResponseBodies entry
*
* @see CreateRuleGroupRequest#withCustomResponseBodies
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest addCustomResponseBodiesEntry(String key, CustomResponseBody value) {
if (null == this.customResponseBodies) {
this.customResponseBodies = new java.util.HashMap();
}
if (this.customResponseBodies.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.customResponseBodies.put(key, value);
return this;
}
/**
* Removes all the entries added into CustomResponseBodies.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRuleGroupRequest clearCustomResponseBodiesEntries() {
this.customResponseBodies = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getScope() != null)
sb.append("Scope: ").append(getScope()).append(",");
if (getCapacity() != null)
sb.append("Capacity: ").append(getCapacity()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getRules() != null)
sb.append("Rules: ").append(getRules()).append(",");
if (getVisibilityConfig() != null)
sb.append("VisibilityConfig: ").append(getVisibilityConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCustomResponseBodies() != null)
sb.append("CustomResponseBodies: ").append(getCustomResponseBodies());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateRuleGroupRequest == false)
return false;
CreateRuleGroupRequest other = (CreateRuleGroupRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getScope() == null ^ this.getScope() == null)
return false;
if (other.getScope() != null && other.getScope().equals(this.getScope()) == false)
return false;
if (other.getCapacity() == null ^ this.getCapacity() == null)
return false;
if (other.getCapacity() != null && other.getCapacity().equals(this.getCapacity()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getRules() == null ^ this.getRules() == null)
return false;
if (other.getRules() != null && other.getRules().equals(this.getRules()) == false)
return false;
if (other.getVisibilityConfig() == null ^ this.getVisibilityConfig() == null)
return false;
if (other.getVisibilityConfig() != null && other.getVisibilityConfig().equals(this.getVisibilityConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCustomResponseBodies() == null ^ this.getCustomResponseBodies() == null)
return false;
if (other.getCustomResponseBodies() != null && other.getCustomResponseBodies().equals(this.getCustomResponseBodies()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getScope() == null) ? 0 : getScope().hashCode());
hashCode = prime * hashCode + ((getCapacity() == null) ? 0 : getCapacity().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getRules() == null) ? 0 : getRules().hashCode());
hashCode = prime * hashCode + ((getVisibilityConfig() == null) ? 0 : getVisibilityConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCustomResponseBodies() == null) ? 0 : getCustomResponseBodies().hashCode());
return hashCode;
}
@Override
public CreateRuleGroupRequest clone() {
return (CreateRuleGroupRequest) super.clone();
}
}