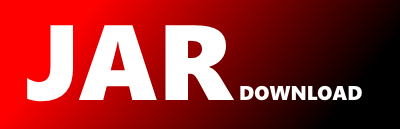
com.amazonaws.services.wafv2.model.DescribeManagedRuleGroupResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wafv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wafv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeManagedRuleGroupResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The managed rule group's version.
*
*/
private String versionName;
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive notifications
* when the managed rule group is modified, such as for new versions and for version expiration. For more
* information, see the Amazon Simple Notification
* Service Developer Guide.
*
*/
private String snsTopicArn;
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*/
private Long capacity;
/** */
private java.util.List rules;
/**
*
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this prefix.
*
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined and
* the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*
*/
private String labelNamespace;
/**
*
* The labels that one or more rules in this rule group add to matching web requests. These labels are defined in
* the RuleLabels
for a Rule.
*
*/
private java.util.List availableLabels;
/**
*
* The labels that one or more rules in this rule group match against in label match statements. These labels are
* defined in a LabelMatchStatement
specification, in the Statement definition of a rule.
*
*/
private java.util.List consumedLabels;
/**
*
* The managed rule group's version.
*
*
* @param versionName
* The managed rule group's version.
*/
public void setVersionName(String versionName) {
this.versionName = versionName;
}
/**
*
* The managed rule group's version.
*
*
* @return The managed rule group's version.
*/
public String getVersionName() {
return this.versionName;
}
/**
*
* The managed rule group's version.
*
*
* @param versionName
* The managed rule group's version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withVersionName(String versionName) {
setVersionName(versionName);
return this;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive notifications
* when the managed rule group is modified, such as for new versions and for version expiration. For more
* information, see the Amazon Simple Notification
* Service Developer Guide.
*
*
* @param snsTopicArn
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive
* notifications when the managed rule group is modified, such as for new versions and for version
* expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide.
*/
public void setSnsTopicArn(String snsTopicArn) {
this.snsTopicArn = snsTopicArn;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive notifications
* when the managed rule group is modified, such as for new versions and for version expiration. For more
* information, see the Amazon Simple Notification
* Service Developer Guide.
*
*
* @return The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive
* notifications when the managed rule group is modified, such as for new versions and for version
* expiration. For more information, see the Amazon Simple Notification Service
* Developer Guide.
*/
public String getSnsTopicArn() {
return this.snsTopicArn;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive notifications
* when the managed rule group is modified, such as for new versions and for version expiration. For more
* information, see the Amazon Simple Notification
* Service Developer Guide.
*
*
* @param snsTopicArn
* The Amazon resource name (ARN) of the Amazon Simple Notification Service SNS topic that's used to provide
* notification of changes to the managed rule group. You can subscribe to the SNS topic to receive
* notifications when the managed rule group is modified, such as for new versions and for version
* expiration. For more information, see the Amazon Simple Notification Service Developer
* Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withSnsTopicArn(String snsTopicArn) {
setSnsTopicArn(snsTopicArn);
return this;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @param capacity
* The web ACL capacity units (WCUs) required for this rule group.
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost
* of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more
* processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage
* when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
*/
public void setCapacity(Long capacity) {
this.capacity = capacity;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @return The web ACL capacity units (WCUs) required for this rule group.
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative
* cost of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use
* more processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU
* usage when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
*/
public Long getCapacity() {
return this.capacity;
}
/**
*
* The web ACL capacity units (WCUs) required for this rule group.
*
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and
* web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule.
* Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule
* group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group.
* For more information, see WAF web ACL capacity
* units (WCU) in the WAF Developer Guide.
*
*
* @param capacity
* The web ACL capacity units (WCUs) required for this rule group.
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule
* groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost
* of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more
* processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage
* when they use a rule group. For more information, see WAF web ACL
* capacity units (WCU) in the WAF Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withCapacity(Long capacity) {
setCapacity(capacity);
return this;
}
/**
*
*
* @return
*/
public java.util.List getRules() {
return rules;
}
/**
*
*
* @param rules
*/
public void setRules(java.util.Collection rules) {
if (rules == null) {
this.rules = null;
return;
}
this.rules = new java.util.ArrayList(rules);
}
/**
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRules(java.util.Collection)} or {@link #withRules(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param rules
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withRules(RuleSummary... rules) {
if (this.rules == null) {
setRules(new java.util.ArrayList(rules.length));
}
for (RuleSummary ele : rules) {
this.rules.add(ele);
}
return this;
}
/**
*
*
* @param rules
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withRules(java.util.Collection rules) {
setRules(rules);
return this;
}
/**
*
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this prefix.
*
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined and
* the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*
*
* @param labelNamespace
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this
* prefix.
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined
* and the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*/
public void setLabelNamespace(String labelNamespace) {
this.labelNamespace = labelNamespace;
}
/**
*
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this prefix.
*
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined and
* the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*
*
* @return The label namespace prefix for this rule group. All labels added by rules in this rule group have this
* prefix.
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A
* fully qualified label is made up of the label namespace from the rule group or web ACL where the rule is
* defined and the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*/
public String getLabelNamespace() {
return this.labelNamespace;
}
/**
*
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this prefix.
*
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined and
* the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
*
*
* @param labelNamespace
* The label namespace prefix for this rule group. All labels added by rules in this rule group have this
* prefix.
*
* -
*
* The syntax for the label namespace prefix for a managed rule group is the following:
*
*
* awswaf:managed:<vendor>:<rule group name>
:
*
*
* -
*
* When a rule with a label matches a web request, WAF adds the fully qualified label to the request. A fully
* qualified label is made up of the label namespace from the rule group or web ACL where the rule is defined
* and the label from the rule, separated by a colon:
*
*
* <label namespace>:<label from rule>
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withLabelNamespace(String labelNamespace) {
setLabelNamespace(labelNamespace);
return this;
}
/**
*
* The labels that one or more rules in this rule group add to matching web requests. These labels are defined in
* the RuleLabels
for a Rule.
*
*
* @return The labels that one or more rules in this rule group add to matching web requests. These labels are
* defined in the RuleLabels
for a Rule.
*/
public java.util.List getAvailableLabels() {
return availableLabels;
}
/**
*
* The labels that one or more rules in this rule group add to matching web requests. These labels are defined in
* the RuleLabels
for a Rule.
*
*
* @param availableLabels
* The labels that one or more rules in this rule group add to matching web requests. These labels are
* defined in the RuleLabels
for a Rule.
*/
public void setAvailableLabels(java.util.Collection availableLabels) {
if (availableLabels == null) {
this.availableLabels = null;
return;
}
this.availableLabels = new java.util.ArrayList(availableLabels);
}
/**
*
* The labels that one or more rules in this rule group add to matching web requests. These labels are defined in
* the RuleLabels
for a Rule.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAvailableLabels(java.util.Collection)} or {@link #withAvailableLabels(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param availableLabels
* The labels that one or more rules in this rule group add to matching web requests. These labels are
* defined in the RuleLabels
for a Rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withAvailableLabels(LabelSummary... availableLabels) {
if (this.availableLabels == null) {
setAvailableLabels(new java.util.ArrayList(availableLabels.length));
}
for (LabelSummary ele : availableLabels) {
this.availableLabels.add(ele);
}
return this;
}
/**
*
* The labels that one or more rules in this rule group add to matching web requests. These labels are defined in
* the RuleLabels
for a Rule.
*
*
* @param availableLabels
* The labels that one or more rules in this rule group add to matching web requests. These labels are
* defined in the RuleLabels
for a Rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withAvailableLabels(java.util.Collection availableLabels) {
setAvailableLabels(availableLabels);
return this;
}
/**
*
* The labels that one or more rules in this rule group match against in label match statements. These labels are
* defined in a LabelMatchStatement
specification, in the Statement definition of a rule.
*
*
* @return The labels that one or more rules in this rule group match against in label match statements. These
* labels are defined in a LabelMatchStatement
specification, in the Statement
* definition of a rule.
*/
public java.util.List getConsumedLabels() {
return consumedLabels;
}
/**
*
* The labels that one or more rules in this rule group match against in label match statements. These labels are
* defined in a LabelMatchStatement
specification, in the Statement definition of a rule.
*
*
* @param consumedLabels
* The labels that one or more rules in this rule group match against in label match statements. These labels
* are defined in a LabelMatchStatement
specification, in the Statement definition of a
* rule.
*/
public void setConsumedLabels(java.util.Collection consumedLabels) {
if (consumedLabels == null) {
this.consumedLabels = null;
return;
}
this.consumedLabels = new java.util.ArrayList(consumedLabels);
}
/**
*
* The labels that one or more rules in this rule group match against in label match statements. These labels are
* defined in a LabelMatchStatement
specification, in the Statement definition of a rule.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setConsumedLabels(java.util.Collection)} or {@link #withConsumedLabels(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param consumedLabels
* The labels that one or more rules in this rule group match against in label match statements. These labels
* are defined in a LabelMatchStatement
specification, in the Statement definition of a
* rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withConsumedLabels(LabelSummary... consumedLabels) {
if (this.consumedLabels == null) {
setConsumedLabels(new java.util.ArrayList(consumedLabels.length));
}
for (LabelSummary ele : consumedLabels) {
this.consumedLabels.add(ele);
}
return this;
}
/**
*
* The labels that one or more rules in this rule group match against in label match statements. These labels are
* defined in a LabelMatchStatement
specification, in the Statement definition of a rule.
*
*
* @param consumedLabels
* The labels that one or more rules in this rule group match against in label match statements. These labels
* are defined in a LabelMatchStatement
specification, in the Statement definition of a
* rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeManagedRuleGroupResult withConsumedLabels(java.util.Collection consumedLabels) {
setConsumedLabels(consumedLabels);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVersionName() != null)
sb.append("VersionName: ").append(getVersionName()).append(",");
if (getSnsTopicArn() != null)
sb.append("SnsTopicArn: ").append(getSnsTopicArn()).append(",");
if (getCapacity() != null)
sb.append("Capacity: ").append(getCapacity()).append(",");
if (getRules() != null)
sb.append("Rules: ").append(getRules()).append(",");
if (getLabelNamespace() != null)
sb.append("LabelNamespace: ").append(getLabelNamespace()).append(",");
if (getAvailableLabels() != null)
sb.append("AvailableLabels: ").append(getAvailableLabels()).append(",");
if (getConsumedLabels() != null)
sb.append("ConsumedLabels: ").append(getConsumedLabels());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeManagedRuleGroupResult == false)
return false;
DescribeManagedRuleGroupResult other = (DescribeManagedRuleGroupResult) obj;
if (other.getVersionName() == null ^ this.getVersionName() == null)
return false;
if (other.getVersionName() != null && other.getVersionName().equals(this.getVersionName()) == false)
return false;
if (other.getSnsTopicArn() == null ^ this.getSnsTopicArn() == null)
return false;
if (other.getSnsTopicArn() != null && other.getSnsTopicArn().equals(this.getSnsTopicArn()) == false)
return false;
if (other.getCapacity() == null ^ this.getCapacity() == null)
return false;
if (other.getCapacity() != null && other.getCapacity().equals(this.getCapacity()) == false)
return false;
if (other.getRules() == null ^ this.getRules() == null)
return false;
if (other.getRules() != null && other.getRules().equals(this.getRules()) == false)
return false;
if (other.getLabelNamespace() == null ^ this.getLabelNamespace() == null)
return false;
if (other.getLabelNamespace() != null && other.getLabelNamespace().equals(this.getLabelNamespace()) == false)
return false;
if (other.getAvailableLabels() == null ^ this.getAvailableLabels() == null)
return false;
if (other.getAvailableLabels() != null && other.getAvailableLabels().equals(this.getAvailableLabels()) == false)
return false;
if (other.getConsumedLabels() == null ^ this.getConsumedLabels() == null)
return false;
if (other.getConsumedLabels() != null && other.getConsumedLabels().equals(this.getConsumedLabels()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVersionName() == null) ? 0 : getVersionName().hashCode());
hashCode = prime * hashCode + ((getSnsTopicArn() == null) ? 0 : getSnsTopicArn().hashCode());
hashCode = prime * hashCode + ((getCapacity() == null) ? 0 : getCapacity().hashCode());
hashCode = prime * hashCode + ((getRules() == null) ? 0 : getRules().hashCode());
hashCode = prime * hashCode + ((getLabelNamespace() == null) ? 0 : getLabelNamespace().hashCode());
hashCode = prime * hashCode + ((getAvailableLabels() == null) ? 0 : getAvailableLabels().hashCode());
hashCode = prime * hashCode + ((getConsumedLabels() == null) ? 0 : getConsumedLabels().hashCode());
return hashCode;
}
@Override
public DescribeManagedRuleGroupResult clone() {
try {
return (DescribeManagedRuleGroupResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}