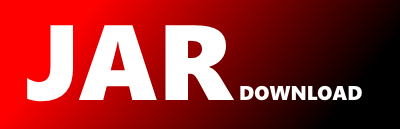
com.amazonaws.services.wafv2.model.GetSampledRequestsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wafv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wafv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetSampledRequestsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*
*/
private String webAclArn;
/**
*
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a sample
* of requests.
*
*/
private String ruleMetricName;
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*/
private String scope;
/**
*
* The start date and time and the end date and time of the range for which you want GetSampledRequests
* to return a sample of requests. You must specify the times in Coordinated Universal Time (UTC) format. UTC format
* includes the special designator, Z
. For example, "2016-09-27T14:50Z"
. You can specify
* any time range in the previous three hours. If you specify a start time that's earlier than three hours ago, WAF
* sets it to three hours ago.
*
*/
private TimeWindow timeWindow;
/**
*
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon Web
* Services resource received during the time range. If your resource received fewer requests than the value of
* MaxItems
, GetSampledRequests
returns information about all of them.
*
*/
private Long maxItems;
/**
*
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*
*
* @param webAclArn
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*/
public void setWebAclArn(String webAclArn) {
this.webAclArn = webAclArn;
}
/**
*
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*
*
* @return The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*/
public String getWebAclArn() {
return this.webAclArn;
}
/**
*
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
*
*
* @param webAclArn
* The Amazon resource name (ARN) of the WebACL
for which you want a sample of requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSampledRequestsRequest withWebAclArn(String webAclArn) {
setWebAclArn(webAclArn);
return this;
}
/**
*
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a sample
* of requests.
*
*
* @param ruleMetricName
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a
* sample of requests.
*/
public void setRuleMetricName(String ruleMetricName) {
this.ruleMetricName = ruleMetricName;
}
/**
*
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a sample
* of requests.
*
*
* @return The metric name assigned to the Rule
or RuleGroup
dimension for which you want
* a sample of requests.
*/
public String getRuleMetricName() {
return this.ruleMetricName;
}
/**
*
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a sample
* of requests.
*
*
* @param ruleMetricName
* The metric name assigned to the Rule
or RuleGroup
dimension for which you want a
* sample of requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSampledRequestsRequest withRuleMetricName(String ruleMetricName) {
setRuleMetricName(ruleMetricName);
return this;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @see Scope
*/
public void setScope(String scope) {
this.scope = scope;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @return Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @see Scope
*/
public String getScope() {
return this.scope;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public GetSampledRequestsRequest withScope(String scope) {
setScope(scope);
return this;
}
/**
*
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL API, an
* Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access instance.
*
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope: --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
*
*
* @param scope
* Specifies whether this is for an Amazon CloudFront distribution or for a regional application. A regional
* application can be an Application Load Balancer (ALB), an Amazon API Gateway REST API, an AppSync GraphQL
* API, an Amazon Cognito user pool, an App Runner service, or an Amazon Web Services Verified Access
* instance.
*
* To work with CloudFront, you must also specify the Region US East (N. Virginia) as follows:
*
*
* -
*
* CLI - Specify the Region when you use the CloudFront scope:
* --scope=CLOUDFRONT --region=us-east-1
.
*
*
* -
*
* API and SDKs - For all calls, use the Region endpoint us-east-1.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public GetSampledRequestsRequest withScope(Scope scope) {
this.scope = scope.toString();
return this;
}
/**
*
* The start date and time and the end date and time of the range for which you want GetSampledRequests
* to return a sample of requests. You must specify the times in Coordinated Universal Time (UTC) format. UTC format
* includes the special designator, Z
. For example, "2016-09-27T14:50Z"
. You can specify
* any time range in the previous three hours. If you specify a start time that's earlier than three hours ago, WAF
* sets it to three hours ago.
*
*
* @param timeWindow
* The start date and time and the end date and time of the range for which you want
* GetSampledRequests
to return a sample of requests. You must specify the times in Coordinated
* Universal Time (UTC) format. UTC format includes the special designator, Z
. For example,
* "2016-09-27T14:50Z"
. You can specify any time range in the previous three hours. If you
* specify a start time that's earlier than three hours ago, WAF sets it to three hours ago.
*/
public void setTimeWindow(TimeWindow timeWindow) {
this.timeWindow = timeWindow;
}
/**
*
* The start date and time and the end date and time of the range for which you want GetSampledRequests
* to return a sample of requests. You must specify the times in Coordinated Universal Time (UTC) format. UTC format
* includes the special designator, Z
. For example, "2016-09-27T14:50Z"
. You can specify
* any time range in the previous three hours. If you specify a start time that's earlier than three hours ago, WAF
* sets it to three hours ago.
*
*
* @return The start date and time and the end date and time of the range for which you want
* GetSampledRequests
to return a sample of requests. You must specify the times in Coordinated
* Universal Time (UTC) format. UTC format includes the special designator, Z
. For example,
* "2016-09-27T14:50Z"
. You can specify any time range in the previous three hours. If you
* specify a start time that's earlier than three hours ago, WAF sets it to three hours ago.
*/
public TimeWindow getTimeWindow() {
return this.timeWindow;
}
/**
*
* The start date and time and the end date and time of the range for which you want GetSampledRequests
* to return a sample of requests. You must specify the times in Coordinated Universal Time (UTC) format. UTC format
* includes the special designator, Z
. For example, "2016-09-27T14:50Z"
. You can specify
* any time range in the previous three hours. If you specify a start time that's earlier than three hours ago, WAF
* sets it to three hours ago.
*
*
* @param timeWindow
* The start date and time and the end date and time of the range for which you want
* GetSampledRequests
to return a sample of requests. You must specify the times in Coordinated
* Universal Time (UTC) format. UTC format includes the special designator, Z
. For example,
* "2016-09-27T14:50Z"
. You can specify any time range in the previous three hours. If you
* specify a start time that's earlier than three hours ago, WAF sets it to three hours ago.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSampledRequestsRequest withTimeWindow(TimeWindow timeWindow) {
setTimeWindow(timeWindow);
return this;
}
/**
*
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon Web
* Services resource received during the time range. If your resource received fewer requests than the value of
* MaxItems
, GetSampledRequests
returns information about all of them.
*
*
* @param maxItems
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon
* Web Services resource received during the time range. If your resource received fewer requests than the
* value of MaxItems
, GetSampledRequests
returns information about all of them.
*/
public void setMaxItems(Long maxItems) {
this.maxItems = maxItems;
}
/**
*
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon Web
* Services resource received during the time range. If your resource received fewer requests than the value of
* MaxItems
, GetSampledRequests
returns information about all of them.
*
*
* @return The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon
* Web Services resource received during the time range. If your resource received fewer requests than the
* value of MaxItems
, GetSampledRequests
returns information about all of them.
*/
public Long getMaxItems() {
return this.maxItems;
}
/**
*
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon Web
* Services resource received during the time range. If your resource received fewer requests than the value of
* MaxItems
, GetSampledRequests
returns information about all of them.
*
*
* @param maxItems
* The number of requests that you want WAF to return from among the first 5,000 requests that your Amazon
* Web Services resource received during the time range. If your resource received fewer requests than the
* value of MaxItems
, GetSampledRequests
returns information about all of them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSampledRequestsRequest withMaxItems(Long maxItems) {
setMaxItems(maxItems);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWebAclArn() != null)
sb.append("WebAclArn: ").append(getWebAclArn()).append(",");
if (getRuleMetricName() != null)
sb.append("RuleMetricName: ").append(getRuleMetricName()).append(",");
if (getScope() != null)
sb.append("Scope: ").append(getScope()).append(",");
if (getTimeWindow() != null)
sb.append("TimeWindow: ").append(getTimeWindow()).append(",");
if (getMaxItems() != null)
sb.append("MaxItems: ").append(getMaxItems());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetSampledRequestsRequest == false)
return false;
GetSampledRequestsRequest other = (GetSampledRequestsRequest) obj;
if (other.getWebAclArn() == null ^ this.getWebAclArn() == null)
return false;
if (other.getWebAclArn() != null && other.getWebAclArn().equals(this.getWebAclArn()) == false)
return false;
if (other.getRuleMetricName() == null ^ this.getRuleMetricName() == null)
return false;
if (other.getRuleMetricName() != null && other.getRuleMetricName().equals(this.getRuleMetricName()) == false)
return false;
if (other.getScope() == null ^ this.getScope() == null)
return false;
if (other.getScope() != null && other.getScope().equals(this.getScope()) == false)
return false;
if (other.getTimeWindow() == null ^ this.getTimeWindow() == null)
return false;
if (other.getTimeWindow() != null && other.getTimeWindow().equals(this.getTimeWindow()) == false)
return false;
if (other.getMaxItems() == null ^ this.getMaxItems() == null)
return false;
if (other.getMaxItems() != null && other.getMaxItems().equals(this.getMaxItems()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWebAclArn() == null) ? 0 : getWebAclArn().hashCode());
hashCode = prime * hashCode + ((getRuleMetricName() == null) ? 0 : getRuleMetricName().hashCode());
hashCode = prime * hashCode + ((getScope() == null) ? 0 : getScope().hashCode());
hashCode = prime * hashCode + ((getTimeWindow() == null) ? 0 : getTimeWindow().hashCode());
hashCode = prime * hashCode + ((getMaxItems() == null) ? 0 : getMaxItems().hashCode());
return hashCode;
}
@Override
public GetSampledRequestsRequest clone() {
return (GetSampledRequestsRequest) super.clone();
}
}