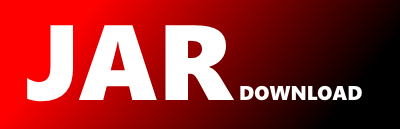
com.amazonaws.services.wellarchitected.AWSWellArchitectedAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-wellarchitected Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.wellarchitected;
import javax.annotation.Generated;
import com.amazonaws.services.wellarchitected.model.*;
/**
* Interface for accessing Well-Architected asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.wellarchitected.AbstractAWSWellArchitectedAsync} instead.
*
*
* AWS Well-Architected Tool
*
* This is the AWS Well-Architected Tool API Reference. The AWS Well-Architected Tool API provides programmatic
* access to the AWS Well-Architected Tool in the AWS Management Console. For information about the AWS
* Well-Architected Tool, see the AWS
* Well-Architected Tool User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSWellArchitectedAsync extends AWSWellArchitected {
/**
*
* Associate a lens to a workload.
*
*
* @param associateLensesRequest
* Input to associate lens reviews.
* @return A Java Future containing the result of the AssociateLenses operation returned by the service.
* @sample AWSWellArchitectedAsync.AssociateLenses
* @see AWS API Documentation
*/
java.util.concurrent.Future associateLensesAsync(AssociateLensesRequest associateLensesRequest);
/**
*
* Associate a lens to a workload.
*
*
* @param associateLensesRequest
* Input to associate lens reviews.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateLenses operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.AssociateLenses
* @see AWS API Documentation
*/
java.util.concurrent.Future associateLensesAsync(AssociateLensesRequest associateLensesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a milestone for an existing workload.
*
*
* @param createMilestoneRequest
* Input for milestone creation.
* @return A Java Future containing the result of the CreateMilestone operation returned by the service.
* @sample AWSWellArchitectedAsync.CreateMilestone
* @see AWS API Documentation
*/
java.util.concurrent.Future createMilestoneAsync(CreateMilestoneRequest createMilestoneRequest);
/**
*
* Create a milestone for an existing workload.
*
*
* @param createMilestoneRequest
* Input for milestone creation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMilestone operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.CreateMilestone
* @see AWS API Documentation
*/
java.util.concurrent.Future createMilestoneAsync(CreateMilestoneRequest createMilestoneRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new workload.
*
*
* The owner of a workload can share the workload with other AWS accounts and IAM users in the same AWS Region. Only
* the owner of a workload can delete it.
*
*
* For more information, see Defining a Workload
* in the AWS Well-Architected Tool User Guide.
*
*
* @param createWorkloadRequest
* Input for workload creation.
* @return A Java Future containing the result of the CreateWorkload operation returned by the service.
* @sample AWSWellArchitectedAsync.CreateWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkloadAsync(CreateWorkloadRequest createWorkloadRequest);
/**
*
* Create a new workload.
*
*
* The owner of a workload can share the workload with other AWS accounts and IAM users in the same AWS Region. Only
* the owner of a workload can delete it.
*
*
* For more information, see Defining a Workload
* in the AWS Well-Architected Tool User Guide.
*
*
* @param createWorkloadRequest
* Input for workload creation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkload operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.CreateWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkloadAsync(CreateWorkloadRequest createWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a workload share.
*
*
* The owner of a workload can share it with other AWS accounts and IAM users in the same AWS Region. Shared access
* to a workload is not removed until the workload invitation is deleted.
*
*
* For more information, see Sharing a Workload
* in the AWS Well-Architected Tool User Guide.
*
*
* @param createWorkloadShareRequest
* Input for Create Workload Share
* @return A Java Future containing the result of the CreateWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsync.CreateWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorkloadShareAsync(CreateWorkloadShareRequest createWorkloadShareRequest);
/**
*
* Create a workload share.
*
*
* The owner of a workload can share it with other AWS accounts and IAM users in the same AWS Region. Shared access
* to a workload is not removed until the workload invitation is deleted.
*
*
* For more information, see Sharing a Workload
* in the AWS Well-Architected Tool User Guide.
*
*
* @param createWorkloadShareRequest
* Input for Create Workload Share
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.CreateWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorkloadShareAsync(CreateWorkloadShareRequest createWorkloadShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an existing workload.
*
*
* @param deleteWorkloadRequest
* Input for workload deletion.
* @return A Java Future containing the result of the DeleteWorkload operation returned by the service.
* @sample AWSWellArchitectedAsync.DeleteWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorkloadAsync(DeleteWorkloadRequest deleteWorkloadRequest);
/**
*
* Delete an existing workload.
*
*
* @param deleteWorkloadRequest
* Input for workload deletion.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkload operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.DeleteWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorkloadAsync(DeleteWorkloadRequest deleteWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a workload share.
*
*
* @param deleteWorkloadShareRequest
* Input for Delete Workload Share
* @return A Java Future containing the result of the DeleteWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsync.DeleteWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkloadShareAsync(DeleteWorkloadShareRequest deleteWorkloadShareRequest);
/**
*
* Delete a workload share.
*
*
* @param deleteWorkloadShareRequest
* Input for Delete Workload Share
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.DeleteWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkloadShareAsync(DeleteWorkloadShareRequest deleteWorkloadShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociate a lens from a workload.
*
*
*
* The AWS Well-Architected Framework lens (wellarchitected
) cannot be removed from a workload.
*
*
*
* @param disassociateLensesRequest
* Input to disassociate lens reviews.
* @return A Java Future containing the result of the DisassociateLenses operation returned by the service.
* @sample AWSWellArchitectedAsync.DisassociateLenses
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateLensesAsync(DisassociateLensesRequest disassociateLensesRequest);
/**
*
* Disassociate a lens from a workload.
*
*
*
* The AWS Well-Architected Framework lens (wellarchitected
) cannot be removed from a workload.
*
*
*
* @param disassociateLensesRequest
* Input to disassociate lens reviews.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateLenses operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.DisassociateLenses
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateLensesAsync(DisassociateLensesRequest disassociateLensesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the answer to a specific question in a workload review.
*
*
* @param getAnswerRequest
* Input to get answer.
* @return A Java Future containing the result of the GetAnswer operation returned by the service.
* @sample AWSWellArchitectedAsync.GetAnswer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAnswerAsync(GetAnswerRequest getAnswerRequest);
/**
*
* Get the answer to a specific question in a workload review.
*
*
* @param getAnswerRequest
* Input to get answer.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAnswer operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetAnswer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAnswerAsync(GetAnswerRequest getAnswerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get lens review.
*
*
* @param getLensReviewRequest
* Input to get lens review.
* @return A Java Future containing the result of the GetLensReview operation returned by the service.
* @sample AWSWellArchitectedAsync.GetLensReview
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getLensReviewAsync(GetLensReviewRequest getLensReviewRequest);
/**
*
* Get lens review.
*
*
* @param getLensReviewRequest
* Input to get lens review.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLensReview operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetLensReview
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getLensReviewAsync(GetLensReviewRequest getLensReviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get lens review report.
*
*
* @param getLensReviewReportRequest
* Input to get lens review report.
* @return A Java Future containing the result of the GetLensReviewReport operation returned by the service.
* @sample AWSWellArchitectedAsync.GetLensReviewReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getLensReviewReportAsync(GetLensReviewReportRequest getLensReviewReportRequest);
/**
*
* Get lens review report.
*
*
* @param getLensReviewReportRequest
* Input to get lens review report.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLensReviewReport operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetLensReviewReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getLensReviewReportAsync(GetLensReviewReportRequest getLensReviewReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get lens version differences.
*
*
* @param getLensVersionDifferenceRequest
* @return A Java Future containing the result of the GetLensVersionDifference operation returned by the service.
* @sample AWSWellArchitectedAsync.GetLensVersionDifference
* @see AWS API Documentation
*/
java.util.concurrent.Future getLensVersionDifferenceAsync(GetLensVersionDifferenceRequest getLensVersionDifferenceRequest);
/**
*
* Get lens version differences.
*
*
* @param getLensVersionDifferenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLensVersionDifference operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetLensVersionDifference
* @see AWS API Documentation
*/
java.util.concurrent.Future getLensVersionDifferenceAsync(GetLensVersionDifferenceRequest getLensVersionDifferenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a milestone for an existing workload.
*
*
* @param getMilestoneRequest
* Input to get a milestone.
* @return A Java Future containing the result of the GetMilestone operation returned by the service.
* @sample AWSWellArchitectedAsync.GetMilestone
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMilestoneAsync(GetMilestoneRequest getMilestoneRequest);
/**
*
* Get a milestone for an existing workload.
*
*
* @param getMilestoneRequest
* Input to get a milestone.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMilestone operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetMilestone
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMilestoneAsync(GetMilestoneRequest getMilestoneRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get an existing workload.
*
*
* @param getWorkloadRequest
* Input to get a workload.
* @return A Java Future containing the result of the GetWorkload operation returned by the service.
* @sample AWSWellArchitectedAsync.GetWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorkloadAsync(GetWorkloadRequest getWorkloadRequest);
/**
*
* Get an existing workload.
*
*
* @param getWorkloadRequest
* Input to get a workload.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetWorkload operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.GetWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorkloadAsync(GetWorkloadRequest getWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List of answers.
*
*
* @param listAnswersRequest
* Input to list answers.
* @return A Java Future containing the result of the ListAnswers operation returned by the service.
* @sample AWSWellArchitectedAsync.ListAnswers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAnswersAsync(ListAnswersRequest listAnswersRequest);
/**
*
* List of answers.
*
*
* @param listAnswersRequest
* Input to list answers.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAnswers operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListAnswers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAnswersAsync(ListAnswersRequest listAnswersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List lens review improvements.
*
*
* @param listLensReviewImprovementsRequest
* Input to list lens review improvements.
* @return A Java Future containing the result of the ListLensReviewImprovements operation returned by the service.
* @sample AWSWellArchitectedAsync.ListLensReviewImprovements
* @see AWS API Documentation
*/
java.util.concurrent.Future listLensReviewImprovementsAsync(
ListLensReviewImprovementsRequest listLensReviewImprovementsRequest);
/**
*
* List lens review improvements.
*
*
* @param listLensReviewImprovementsRequest
* Input to list lens review improvements.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLensReviewImprovements operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListLensReviewImprovements
* @see AWS API Documentation
*/
java.util.concurrent.Future listLensReviewImprovementsAsync(
ListLensReviewImprovementsRequest listLensReviewImprovementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List lens reviews.
*
*
* @param listLensReviewsRequest
* Input to list lens reviews.
* @return A Java Future containing the result of the ListLensReviews operation returned by the service.
* @sample AWSWellArchitectedAsync.ListLensReviews
* @see AWS API Documentation
*/
java.util.concurrent.Future listLensReviewsAsync(ListLensReviewsRequest listLensReviewsRequest);
/**
*
* List lens reviews.
*
*
* @param listLensReviewsRequest
* Input to list lens reviews.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLensReviews operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListLensReviews
* @see AWS API Documentation
*/
java.util.concurrent.Future listLensReviewsAsync(ListLensReviewsRequest listLensReviewsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the available lenses.
*
*
* @param listLensesRequest
* Input to list lenses.
* @return A Java Future containing the result of the ListLenses operation returned by the service.
* @sample AWSWellArchitectedAsync.ListLenses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLensesAsync(ListLensesRequest listLensesRequest);
/**
*
* List the available lenses.
*
*
* @param listLensesRequest
* Input to list lenses.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLenses operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListLenses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLensesAsync(ListLensesRequest listLensesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all milestones for an existing workload.
*
*
* @param listMilestonesRequest
* Input to list all milestones for a workload.
* @return A Java Future containing the result of the ListMilestones operation returned by the service.
* @sample AWSWellArchitectedAsync.ListMilestones
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMilestonesAsync(ListMilestonesRequest listMilestonesRequest);
/**
*
* List all milestones for an existing workload.
*
*
* @param listMilestonesRequest
* Input to list all milestones for a workload.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMilestones operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListMilestones
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMilestonesAsync(ListMilestonesRequest listMilestonesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List lens notifications.
*
*
* @param listNotificationsRequest
* @return A Java Future containing the result of the ListNotifications operation returned by the service.
* @sample AWSWellArchitectedAsync.ListNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future listNotificationsAsync(ListNotificationsRequest listNotificationsRequest);
/**
*
* List lens notifications.
*
*
* @param listNotificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNotifications operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future listNotificationsAsync(ListNotificationsRequest listNotificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the workload invitations.
*
*
* @param listShareInvitationsRequest
* Input for List Share Invitations
* @return A Java Future containing the result of the ListShareInvitations operation returned by the service.
* @sample AWSWellArchitectedAsync.ListShareInvitations
* @see AWS API Documentation
*/
java.util.concurrent.Future listShareInvitationsAsync(ListShareInvitationsRequest listShareInvitationsRequest);
/**
*
* List the workload invitations.
*
*
* @param listShareInvitationsRequest
* Input for List Share Invitations
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListShareInvitations operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListShareInvitations
* @see AWS API Documentation
*/
java.util.concurrent.Future listShareInvitationsAsync(ListShareInvitationsRequest listShareInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSWellArchitectedAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the workload shares associated with the workload.
*
*
* @param listWorkloadSharesRequest
* Input for List Workload Share
* @return A Java Future containing the result of the ListWorkloadShares operation returned by the service.
* @sample AWSWellArchitectedAsync.ListWorkloadShares
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkloadSharesAsync(ListWorkloadSharesRequest listWorkloadSharesRequest);
/**
*
* List the workload shares associated with the workload.
*
*
* @param listWorkloadSharesRequest
* Input for List Workload Share
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkloadShares operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListWorkloadShares
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkloadSharesAsync(ListWorkloadSharesRequest listWorkloadSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List workloads. Paginated.
*
*
* @param listWorkloadsRequest
* Input to list all workloads.
* @return A Java Future containing the result of the ListWorkloads operation returned by the service.
* @sample AWSWellArchitectedAsync.ListWorkloads
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorkloadsAsync(ListWorkloadsRequest listWorkloadsRequest);
/**
*
* List workloads. Paginated.
*
*
* @param listWorkloadsRequest
* Input to list all workloads.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkloads operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.ListWorkloads
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorkloadsAsync(ListWorkloadsRequest listWorkloadsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSWellArchitectedAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds one or more tags to the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes specified tags from a resource.
*
*
* To specify multiple tags, use separate tagKeys parameters, for example:
*
*
* DELETE /tags/WorkloadArn?tagKeys=key1&tagKeys=key2
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSWellArchitectedAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes specified tags from a resource.
*
*
* To specify multiple tags, use separate tagKeys parameters, for example:
*
*
* DELETE /tags/WorkloadArn?tagKeys=key1&tagKeys=key2
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the answer to a specific question in a workload review.
*
*
* @param updateAnswerRequest
* Input to update answer.
* @return A Java Future containing the result of the UpdateAnswer operation returned by the service.
* @sample AWSWellArchitectedAsync.UpdateAnswer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAnswerAsync(UpdateAnswerRequest updateAnswerRequest);
/**
*
* Update the answer to a specific question in a workload review.
*
*
* @param updateAnswerRequest
* Input to update answer.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAnswer operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpdateAnswer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAnswerAsync(UpdateAnswerRequest updateAnswerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update lens review.
*
*
* @param updateLensReviewRequest
* Input for update lens review.
* @return A Java Future containing the result of the UpdateLensReview operation returned by the service.
* @sample AWSWellArchitectedAsync.UpdateLensReview
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLensReviewAsync(UpdateLensReviewRequest updateLensReviewRequest);
/**
*
* Update lens review.
*
*
* @param updateLensReviewRequest
* Input for update lens review.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLensReview operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpdateLensReview
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLensReviewAsync(UpdateLensReviewRequest updateLensReviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update a workload invitation.
*
*
* @param updateShareInvitationRequest
* Input for Update Share Invitation
* @return A Java Future containing the result of the UpdateShareInvitation operation returned by the service.
* @sample AWSWellArchitectedAsync.UpdateShareInvitation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateShareInvitationAsync(UpdateShareInvitationRequest updateShareInvitationRequest);
/**
*
* Update a workload invitation.
*
*
* @param updateShareInvitationRequest
* Input for Update Share Invitation
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateShareInvitation operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpdateShareInvitation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateShareInvitationAsync(UpdateShareInvitationRequest updateShareInvitationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an existing workload.
*
*
* @param updateWorkloadRequest
* Input to update a workload.
* @return A Java Future containing the result of the UpdateWorkload operation returned by the service.
* @sample AWSWellArchitectedAsync.UpdateWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateWorkloadAsync(UpdateWorkloadRequest updateWorkloadRequest);
/**
*
* Update an existing workload.
*
*
* @param updateWorkloadRequest
* Input to update a workload.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkload operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpdateWorkload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateWorkloadAsync(UpdateWorkloadRequest updateWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update a workload share.
*
*
* @param updateWorkloadShareRequest
* Input for Update Workload Share
* @return A Java Future containing the result of the UpdateWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsync.UpdateWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkloadShareAsync(UpdateWorkloadShareRequest updateWorkloadShareRequest);
/**
*
* Update a workload share.
*
*
* @param updateWorkloadShareRequest
* Input for Update Workload Share
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkloadShare operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpdateWorkloadShare
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkloadShareAsync(UpdateWorkloadShareRequest updateWorkloadShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Upgrade lens review.
*
*
* @param upgradeLensReviewRequest
* @return A Java Future containing the result of the UpgradeLensReview operation returned by the service.
* @sample AWSWellArchitectedAsync.UpgradeLensReview
* @see AWS API Documentation
*/
java.util.concurrent.Future upgradeLensReviewAsync(UpgradeLensReviewRequest upgradeLensReviewRequest);
/**
*
* Upgrade lens review.
*
*
* @param upgradeLensReviewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpgradeLensReview operation returned by the service.
* @sample AWSWellArchitectedAsyncHandler.UpgradeLensReview
* @see AWS API Documentation
*/
java.util.concurrent.Future upgradeLensReviewAsync(UpgradeLensReviewRequest upgradeLensReviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}