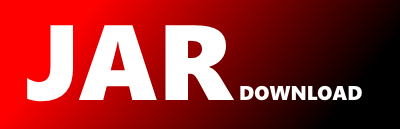
com.amazonaws.services.worklink.AmazonWorkLinkAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-worklink Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.worklink;
import javax.annotation.Generated;
import com.amazonaws.services.worklink.model.*;
/**
* Interface for accessing WorkLink asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.worklink.AbstractAmazonWorkLinkAsync} instead.
*
*
*
* Amazon WorkLink is a cloud-based service that provides secure access to internal websites and web apps from iOS and
* Android phones. In a single step, your users, such as employees, can access internal websites as efficiently as they
* access any other public website. They enter a URL in their web browser, or choose a link to an internal website in an
* email. Amazon WorkLink authenticates the user's access and securely renders authorized internal web content in a
* secure rendering service in the AWS cloud. Amazon WorkLink doesn't download or store any internal web content on
* mobile devices.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonWorkLinkAsync extends AmazonWorkLink {
/**
*
* Specifies a domain to be associated to Amazon WorkLink.
*
*
* @param associateDomainRequest
* @return A Java Future containing the result of the AssociateDomain operation returned by the service.
* @sample AmazonWorkLinkAsync.AssociateDomain
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future associateDomainAsync(AssociateDomainRequest associateDomainRequest);
/**
*
* Specifies a domain to be associated to Amazon WorkLink.
*
*
* @param associateDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateDomain operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.AssociateDomain
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future associateDomainAsync(AssociateDomainRequest associateDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a website authorization provider with a specified fleet. This is used to authorize users against
* associated websites in the company network.
*
*
* @param associateWebsiteAuthorizationProviderRequest
* @return A Java Future containing the result of the AssociateWebsiteAuthorizationProvider operation returned by
* the service.
* @sample AmazonWorkLinkAsync.AssociateWebsiteAuthorizationProvider
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associateWebsiteAuthorizationProviderAsync(
AssociateWebsiteAuthorizationProviderRequest associateWebsiteAuthorizationProviderRequest);
/**
*
* Associates a website authorization provider with a specified fleet. This is used to authorize users against
* associated websites in the company network.
*
*
* @param associateWebsiteAuthorizationProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateWebsiteAuthorizationProvider operation returned by
* the service.
* @sample AmazonWorkLinkAsyncHandler.AssociateWebsiteAuthorizationProvider
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associateWebsiteAuthorizationProviderAsync(
AssociateWebsiteAuthorizationProviderRequest associateWebsiteAuthorizationProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Imports the root certificate of a certificate authority (CA) used to obtain TLS certificates used by associated
* websites within the company network.
*
*
* @param associateWebsiteCertificateAuthorityRequest
* @return A Java Future containing the result of the AssociateWebsiteCertificateAuthority operation returned by the
* service.
* @sample AmazonWorkLinkAsync.AssociateWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associateWebsiteCertificateAuthorityAsync(
AssociateWebsiteCertificateAuthorityRequest associateWebsiteCertificateAuthorityRequest);
/**
*
* Imports the root certificate of a certificate authority (CA) used to obtain TLS certificates used by associated
* websites within the company network.
*
*
* @param associateWebsiteCertificateAuthorityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateWebsiteCertificateAuthority operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.AssociateWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associateWebsiteCertificateAuthorityAsync(
AssociateWebsiteCertificateAuthorityRequest associateWebsiteCertificateAuthorityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a fleet. A fleet consists of resources and the configuration that delivers associated websites to
* authorized users who download and set up the Amazon WorkLink app.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonWorkLinkAsync.CreateFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Creates a fleet. A fleet consists of resources and the configuration that delivers associated websites to
* authorized users who download and set up the Amazon WorkLink app.
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a fleet. Prevents users from accessing previously associated websites.
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonWorkLinkAsync.DeleteFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes a fleet. Prevents users from accessing previously associated websites.
*
*
* @param deleteFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.DeleteFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the configuration for delivering audit streams to the customer account.
*
*
* @param describeAuditStreamConfigurationRequest
* @return A Java Future containing the result of the DescribeAuditStreamConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.DescribeAuditStreamConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAuditStreamConfigurationAsync(
DescribeAuditStreamConfigurationRequest describeAuditStreamConfigurationRequest);
/**
*
* Describes the configuration for delivering audit streams to the customer account.
*
*
* @param describeAuditStreamConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAuditStreamConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.DescribeAuditStreamConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAuditStreamConfigurationAsync(
DescribeAuditStreamConfigurationRequest describeAuditStreamConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the networking configuration to access the internal websites associated with the specified fleet.
*
*
* @param describeCompanyNetworkConfigurationRequest
* @return A Java Future containing the result of the DescribeCompanyNetworkConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.DescribeCompanyNetworkConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeCompanyNetworkConfigurationAsync(
DescribeCompanyNetworkConfigurationRequest describeCompanyNetworkConfigurationRequest);
/**
*
* Describes the networking configuration to access the internal websites associated with the specified fleet.
*
*
* @param describeCompanyNetworkConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCompanyNetworkConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.DescribeCompanyNetworkConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeCompanyNetworkConfigurationAsync(
DescribeCompanyNetworkConfigurationRequest describeCompanyNetworkConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about a user's device.
*
*
* @param describeDeviceRequest
* @return A Java Future containing the result of the DescribeDevice operation returned by the service.
* @sample AmazonWorkLinkAsync.DescribeDevice
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeDeviceAsync(DescribeDeviceRequest describeDeviceRequest);
/**
*
* Provides information about a user's device.
*
*
* @param describeDeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDevice operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.DescribeDevice
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeDeviceAsync(DescribeDeviceRequest describeDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the device policy configuration for the specified fleet.
*
*
* @param describeDevicePolicyConfigurationRequest
* @return A Java Future containing the result of the DescribeDevicePolicyConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.DescribeDevicePolicyConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeDevicePolicyConfigurationAsync(
DescribeDevicePolicyConfigurationRequest describeDevicePolicyConfigurationRequest);
/**
*
* Describes the device policy configuration for the specified fleet.
*
*
* @param describeDevicePolicyConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDevicePolicyConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.DescribeDevicePolicyConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeDevicePolicyConfigurationAsync(
DescribeDevicePolicyConfigurationRequest describeDevicePolicyConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the domain.
*
*
* @param describeDomainRequest
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* @sample AmazonWorkLinkAsync.DescribeDomain
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeDomainAsync(DescribeDomainRequest describeDomainRequest);
/**
*
* Provides information about the domain.
*
*
* @param describeDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.DescribeDomain
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeDomainAsync(DescribeDomainRequest describeDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides basic information for the specified fleet, excluding identity provider, networking, and device
* configuration details.
*
*
* @param describeFleetMetadataRequest
* @return A Java Future containing the result of the DescribeFleetMetadata operation returned by the service.
* @sample AmazonWorkLinkAsync.DescribeFleetMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeFleetMetadataAsync(DescribeFleetMetadataRequest describeFleetMetadataRequest);
/**
*
* Provides basic information for the specified fleet, excluding identity provider, networking, and device
* configuration details.
*
*
* @param describeFleetMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetMetadata operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.DescribeFleetMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeFleetMetadataAsync(DescribeFleetMetadataRequest describeFleetMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the identity provider configuration of the specified fleet.
*
*
* @param describeIdentityProviderConfigurationRequest
* @return A Java Future containing the result of the DescribeIdentityProviderConfiguration operation returned by
* the service.
* @sample AmazonWorkLinkAsync.DescribeIdentityProviderConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeIdentityProviderConfigurationAsync(
DescribeIdentityProviderConfigurationRequest describeIdentityProviderConfigurationRequest);
/**
*
* Describes the identity provider configuration of the specified fleet.
*
*
* @param describeIdentityProviderConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIdentityProviderConfiguration operation returned by
* the service.
* @sample AmazonWorkLinkAsyncHandler.DescribeIdentityProviderConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeIdentityProviderConfigurationAsync(
DescribeIdentityProviderConfigurationRequest describeIdentityProviderConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the certificate authority.
*
*
* @param describeWebsiteCertificateAuthorityRequest
* @return A Java Future containing the result of the DescribeWebsiteCertificateAuthority operation returned by the
* service.
* @sample AmazonWorkLinkAsync.DescribeWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeWebsiteCertificateAuthorityAsync(
DescribeWebsiteCertificateAuthorityRequest describeWebsiteCertificateAuthorityRequest);
/**
*
* Provides information about the certificate authority.
*
*
* @param describeWebsiteCertificateAuthorityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWebsiteCertificateAuthority operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.DescribeWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeWebsiteCertificateAuthorityAsync(
DescribeWebsiteCertificateAuthorityRequest describeWebsiteCertificateAuthorityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a domain from Amazon WorkLink. End users lose the ability to access the domain with Amazon
* WorkLink.
*
*
* @param disassociateDomainRequest
* @return A Java Future containing the result of the DisassociateDomain operation returned by the service.
* @sample AmazonWorkLinkAsync.DisassociateDomain
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateDomainAsync(DisassociateDomainRequest disassociateDomainRequest);
/**
*
* Disassociates a domain from Amazon WorkLink. End users lose the ability to access the domain with Amazon
* WorkLink.
*
*
* @param disassociateDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateDomain operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.DisassociateDomain
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateDomainAsync(DisassociateDomainRequest disassociateDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a website authorization provider from a specified fleet. After the disassociation, users can't load
* any associated websites that require this authorization provider.
*
*
* @param disassociateWebsiteAuthorizationProviderRequest
* @return A Java Future containing the result of the DisassociateWebsiteAuthorizationProvider operation returned by
* the service.
* @sample AmazonWorkLinkAsync.DisassociateWebsiteAuthorizationProvider
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateWebsiteAuthorizationProviderAsync(
DisassociateWebsiteAuthorizationProviderRequest disassociateWebsiteAuthorizationProviderRequest);
/**
*
* Disassociates a website authorization provider from a specified fleet. After the disassociation, users can't load
* any associated websites that require this authorization provider.
*
*
* @param disassociateWebsiteAuthorizationProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateWebsiteAuthorizationProvider operation returned by
* the service.
* @sample AmazonWorkLinkAsyncHandler.DisassociateWebsiteAuthorizationProvider
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateWebsiteAuthorizationProviderAsync(
DisassociateWebsiteAuthorizationProviderRequest disassociateWebsiteAuthorizationProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a certificate authority (CA).
*
*
* @param disassociateWebsiteCertificateAuthorityRequest
* @return A Java Future containing the result of the DisassociateWebsiteCertificateAuthority operation returned by
* the service.
* @sample AmazonWorkLinkAsync.DisassociateWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateWebsiteCertificateAuthorityAsync(
DisassociateWebsiteCertificateAuthorityRequest disassociateWebsiteCertificateAuthorityRequest);
/**
*
* Removes a certificate authority (CA).
*
*
* @param disassociateWebsiteCertificateAuthorityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateWebsiteCertificateAuthority operation returned by
* the service.
* @sample AmazonWorkLinkAsyncHandler.DisassociateWebsiteCertificateAuthority
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociateWebsiteCertificateAuthorityAsync(
DisassociateWebsiteCertificateAuthorityRequest disassociateWebsiteCertificateAuthorityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of devices registered with the specified fleet.
*
*
* @param listDevicesRequest
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AmazonWorkLinkAsync.ListDevices
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest);
/**
*
* Retrieves a list of devices registered with the specified fleet.
*
*
* @param listDevicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.ListDevices
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of domains associated to a specified fleet.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonWorkLinkAsync.ListDomains
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest);
/**
*
* Retrieves a list of domains associated to a specified fleet.
*
*
* @param listDomainsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.ListDomains
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of fleets for the current account and Region.
*
*
* @param listFleetsRequest
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AmazonWorkLinkAsync.ListFleets
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest);
/**
*
* Retrieves a list of fleets for the current account and Region.
*
*
* @param listFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.ListFleets
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonWorkLinkAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves a list of tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of website authorization providers associated with a specified fleet.
*
*
* @param listWebsiteAuthorizationProvidersRequest
* @return A Java Future containing the result of the ListWebsiteAuthorizationProviders operation returned by the
* service.
* @sample AmazonWorkLinkAsync.ListWebsiteAuthorizationProviders
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future listWebsiteAuthorizationProvidersAsync(
ListWebsiteAuthorizationProvidersRequest listWebsiteAuthorizationProvidersRequest);
/**
*
* Retrieves a list of website authorization providers associated with a specified fleet.
*
*
* @param listWebsiteAuthorizationProvidersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWebsiteAuthorizationProviders operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.ListWebsiteAuthorizationProviders
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future listWebsiteAuthorizationProvidersAsync(
ListWebsiteAuthorizationProvidersRequest listWebsiteAuthorizationProvidersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of certificate authorities added for the current account and Region.
*
*
* @param listWebsiteCertificateAuthoritiesRequest
* @return A Java Future containing the result of the ListWebsiteCertificateAuthorities operation returned by the
* service.
* @sample AmazonWorkLinkAsync.ListWebsiteCertificateAuthorities
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future listWebsiteCertificateAuthoritiesAsync(
ListWebsiteCertificateAuthoritiesRequest listWebsiteCertificateAuthoritiesRequest);
/**
*
* Retrieves a list of certificate authorities added for the current account and Region.
*
*
* @param listWebsiteCertificateAuthoritiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWebsiteCertificateAuthorities operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.ListWebsiteCertificateAuthorities
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future listWebsiteCertificateAuthoritiesAsync(
ListWebsiteCertificateAuthoritiesRequest listWebsiteCertificateAuthoritiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Moves a domain to ACTIVE status if it was in the INACTIVE status.
*
*
* @param restoreDomainAccessRequest
* @return A Java Future containing the result of the RestoreDomainAccess operation returned by the service.
* @sample AmazonWorkLinkAsync.RestoreDomainAccess
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future restoreDomainAccessAsync(RestoreDomainAccessRequest restoreDomainAccessRequest);
/**
*
* Moves a domain to ACTIVE status if it was in the INACTIVE status.
*
*
* @param restoreDomainAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestoreDomainAccess operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.RestoreDomainAccess
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future restoreDomainAccessAsync(RestoreDomainAccessRequest restoreDomainAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Moves a domain to INACTIVE status if it was in the ACTIVE status.
*
*
* @param revokeDomainAccessRequest
* @return A Java Future containing the result of the RevokeDomainAccess operation returned by the service.
* @sample AmazonWorkLinkAsync.RevokeDomainAccess
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future revokeDomainAccessAsync(RevokeDomainAccessRequest revokeDomainAccessRequest);
/**
*
* Moves a domain to INACTIVE status if it was in the ACTIVE status.
*
*
* @param revokeDomainAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RevokeDomainAccess operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.RevokeDomainAccess
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future revokeDomainAccessAsync(RevokeDomainAccessRequest revokeDomainAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Signs the user out from all of their devices. The user can sign in again if they have valid credentials.
*
*
* @param signOutUserRequest
* @return A Java Future containing the result of the SignOutUser operation returned by the service.
* @sample AmazonWorkLinkAsync.SignOutUser
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future signOutUserAsync(SignOutUserRequest signOutUserRequest);
/**
*
* Signs the user out from all of their devices. The user can sign in again if they have valid credentials.
*
*
* @param signOutUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SignOutUser operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.SignOutUser
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future signOutUserAsync(SignOutUserRequest signOutUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites one or more tags for the specified resource, such as a fleet. Each tag consists of a key and
* an optional value. If a resource already has a tag with the same key, this operation updates its value.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonWorkLinkAsync.TagResource
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or overwrites one or more tags for the specified resource, such as a fleet. Each tag consists of a key and
* an optional value. If a resource already has a tag with the same key, this operation updates its value.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonWorkLinkAsync.UntagResource
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the audit stream configuration for the fleet.
*
*
* @param updateAuditStreamConfigurationRequest
* @return A Java Future containing the result of the UpdateAuditStreamConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.UpdateAuditStreamConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateAuditStreamConfigurationAsync(
UpdateAuditStreamConfigurationRequest updateAuditStreamConfigurationRequest);
/**
*
* Updates the audit stream configuration for the fleet.
*
*
* @param updateAuditStreamConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAuditStreamConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.UpdateAuditStreamConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateAuditStreamConfigurationAsync(
UpdateAuditStreamConfigurationRequest updateAuditStreamConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the company network configuration for the fleet.
*
*
* @param updateCompanyNetworkConfigurationRequest
* @return A Java Future containing the result of the UpdateCompanyNetworkConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.UpdateCompanyNetworkConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateCompanyNetworkConfigurationAsync(
UpdateCompanyNetworkConfigurationRequest updateCompanyNetworkConfigurationRequest);
/**
*
* Updates the company network configuration for the fleet.
*
*
* @param updateCompanyNetworkConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCompanyNetworkConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.UpdateCompanyNetworkConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateCompanyNetworkConfigurationAsync(
UpdateCompanyNetworkConfigurationRequest updateCompanyNetworkConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the device policy configuration for the fleet.
*
*
* @param updateDevicePolicyConfigurationRequest
* @return A Java Future containing the result of the UpdateDevicePolicyConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.UpdateDevicePolicyConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateDevicePolicyConfigurationAsync(
UpdateDevicePolicyConfigurationRequest updateDevicePolicyConfigurationRequest);
/**
*
* Updates the device policy configuration for the fleet.
*
*
* @param updateDevicePolicyConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDevicePolicyConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.UpdateDevicePolicyConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateDevicePolicyConfigurationAsync(
UpdateDevicePolicyConfigurationRequest updateDevicePolicyConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates domain metadata, such as DisplayName.
*
*
* @param updateDomainMetadataRequest
* @return A Java Future containing the result of the UpdateDomainMetadata operation returned by the service.
* @sample AmazonWorkLinkAsync.UpdateDomainMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future updateDomainMetadataAsync(UpdateDomainMetadataRequest updateDomainMetadataRequest);
/**
*
* Updates domain metadata, such as DisplayName.
*
*
* @param updateDomainMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDomainMetadata operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.UpdateDomainMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future updateDomainMetadataAsync(UpdateDomainMetadataRequest updateDomainMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates fleet metadata, such as DisplayName.
*
*
* @param updateFleetMetadataRequest
* @return A Java Future containing the result of the UpdateFleetMetadata operation returned by the service.
* @sample AmazonWorkLinkAsync.UpdateFleetMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future updateFleetMetadataAsync(UpdateFleetMetadataRequest updateFleetMetadataRequest);
/**
*
* Updates fleet metadata, such as DisplayName.
*
*
* @param updateFleetMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFleetMetadata operation returned by the service.
* @sample AmazonWorkLinkAsyncHandler.UpdateFleetMetadata
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future updateFleetMetadataAsync(UpdateFleetMetadataRequest updateFleetMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the identity provider configuration for the fleet.
*
*
* @param updateIdentityProviderConfigurationRequest
* @return A Java Future containing the result of the UpdateIdentityProviderConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsync.UpdateIdentityProviderConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateIdentityProviderConfigurationAsync(
UpdateIdentityProviderConfigurationRequest updateIdentityProviderConfigurationRequest);
/**
*
* Updates the identity provider configuration for the fleet.
*
*
* @param updateIdentityProviderConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIdentityProviderConfiguration operation returned by the
* service.
* @sample AmazonWorkLinkAsyncHandler.UpdateIdentityProviderConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future updateIdentityProviderConfigurationAsync(
UpdateIdentityProviderConfigurationRequest updateIdentityProviderConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}