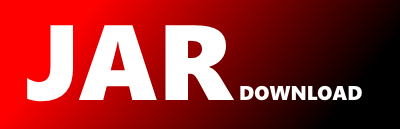
com.amazonaws.services.workspaces.AmazonWorkspacesAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspaces Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.workspaces;
import com.amazonaws.services.workspaces.model.*;
/**
* Interface for accessing Amazon WorkSpaces asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Amazon WorkSpaces Service
*
* This is the Amazon WorkSpaces API Reference. This guide provides
* detailed information about Amazon WorkSpaces operations, data types,
* parameters, and errors.
*
*/
public interface AmazonWorkspacesAsync extends AmazonWorkspaces {
/**
*
* Creates one or more WorkSpaces.
*
*
*
* This operation is asynchronous and returns before the WorkSpaces are
* created.
*
*
*
* @param createWorkspacesRequest
* Contains the inputs for the CreateWorkspaces operation.
* @return A Java Future containing the result of the CreateWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsync.CreateWorkspaces
*/
java.util.concurrent.Future createWorkspacesAsync(
CreateWorkspacesRequest createWorkspacesRequest);
/**
*
* Creates one or more WorkSpaces.
*
*
*
* This operation is asynchronous and returns before the WorkSpaces are
* created.
*
*
*
* @param createWorkspacesRequest
* Contains the inputs for the CreateWorkspaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.CreateWorkspaces
*/
java.util.concurrent.Future createWorkspacesAsync(
CreateWorkspacesRequest createWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the WorkSpace bundles that are available to
* your account in the specified region.
*
*
* You can filter the results with either the BundleIds
* parameter, or the Owner
parameter, but not both.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspaceBundlesRequest
* Contains the inputs for the DescribeWorkspaceBundles
* operation.
* @return A Java Future containing the result of the
* DescribeWorkspaceBundles operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaceBundles
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(
DescribeWorkspaceBundlesRequest describeWorkspaceBundlesRequest);
/**
*
* Obtains information about the WorkSpace bundles that are available to
* your account in the specified region.
*
*
* You can filter the results with either the BundleIds
* parameter, or the Owner
parameter, but not both.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspaceBundlesRequest
* Contains the inputs for the DescribeWorkspaceBundles
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeWorkspaceBundles operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaceBundles
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(
DescribeWorkspaceBundlesRequest describeWorkspaceBundlesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaceBundles
* operation.
*
* @see #describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest)
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaceBundles
* operation with an AsyncHandler.
*
* @see #describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the AWS Directory Service directories in the
* region that are registered with Amazon WorkSpaces and are available to
* your account.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspaceDirectoriesRequest
* Contains the inputs for the DescribeWorkspaceDirectories
* operation.
* @return A Java Future containing the result of the
* DescribeWorkspaceDirectories operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaceDirectories
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
DescribeWorkspaceDirectoriesRequest describeWorkspaceDirectoriesRequest);
/**
*
* Retrieves information about the AWS Directory Service directories in the
* region that are registered with Amazon WorkSpaces and are available to
* your account.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspaceDirectoriesRequest
* Contains the inputs for the DescribeWorkspaceDirectories
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeWorkspaceDirectories operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaceDirectories
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
DescribeWorkspaceDirectoriesRequest describeWorkspaceDirectoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaceDirectories
* operation.
*
* @see #describeWorkspaceDirectoriesAsync(DescribeWorkspaceDirectoriesRequest)
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaceDirectories
* operation with an AsyncHandler.
*
* @see #describeWorkspaceDirectoriesAsync(DescribeWorkspaceDirectoriesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the specified WorkSpaces.
*
*
* Only one of the filter parameters, such as BundleId
,
* DirectoryId
, or WorkspaceIds
, can be specified
* at a time.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspacesRequest
* Contains the inputs for the DescribeWorkspaces operation.
* @return A Java Future containing the result of the DescribeWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaces
*/
java.util.concurrent.Future describeWorkspacesAsync(
DescribeWorkspacesRequest describeWorkspacesRequest);
/**
*
* Obtains information about the specified WorkSpaces.
*
*
* Only one of the filter parameters, such as BundleId
,
* DirectoryId
, or WorkspaceIds
, can be specified
* at a time.
*
*
* This operation supports pagination with the use of the
* NextToken
request and response parameters. If more results
* are available, the NextToken
response member contains a
* token that you pass in the next call to this operation to retrieve the
* next set of items.
*
*
* @param describeWorkspacesRequest
* Contains the inputs for the DescribeWorkspaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaces
*/
java.util.concurrent.Future describeWorkspacesAsync(
DescribeWorkspacesRequest describeWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaces operation.
*
* @see #describeWorkspacesAsync(DescribeWorkspacesRequest)
*/
java.util.concurrent.Future describeWorkspacesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaces operation with
* an AsyncHandler.
*
* @see #describeWorkspacesAsync(DescribeWorkspacesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspacesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reboots the specified WorkSpaces.
*
*
* To be able to reboot a WorkSpace, the WorkSpace must have a State
* of AVAILABLE
, IMPAIRED
, or
* INOPERABLE
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* rebooted.
*
*
*
* @param rebootWorkspacesRequest
* Contains the inputs for the RebootWorkspaces operation.
* @return A Java Future containing the result of the RebootWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsync.RebootWorkspaces
*/
java.util.concurrent.Future rebootWorkspacesAsync(
RebootWorkspacesRequest rebootWorkspacesRequest);
/**
*
* Reboots the specified WorkSpaces.
*
*
* To be able to reboot a WorkSpace, the WorkSpace must have a State
* of AVAILABLE
, IMPAIRED
, or
* INOPERABLE
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* rebooted.
*
*
*
* @param rebootWorkspacesRequest
* Contains the inputs for the RebootWorkspaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.RebootWorkspaces
*/
java.util.concurrent.Future rebootWorkspacesAsync(
RebootWorkspacesRequest rebootWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rebuilds the specified WorkSpaces.
*
*
* Rebuilding a WorkSpace is a potentially destructive action that can
* result in the loss of data. Rebuilding a WorkSpace causes the following
* to occur:
*
*
* - The system is restored to the image of the bundle that the WorkSpace
* is created from. Any applications that have been installed, or system
* settings that have been made since the WorkSpace was created will be
* lost.
* - The data drive (D drive) is re-created from the last automatic
* snapshot taken of the data drive. The current contents of the data drive
* are overwritten. Automatic snapshots of the data drive are taken every 12
* hours, so the snapshot can be as much as 12 hours old.
*
*
* To be able to rebuild a WorkSpace, the WorkSpace must have a State
* of AVAILABLE
or ERROR
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* been completely rebuilt.
*
*
*
* @param rebuildWorkspacesRequest
* Contains the inputs for the RebuildWorkspaces operation.
* @return A Java Future containing the result of the RebuildWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsync.RebuildWorkspaces
*/
java.util.concurrent.Future rebuildWorkspacesAsync(
RebuildWorkspacesRequest rebuildWorkspacesRequest);
/**
*
* Rebuilds the specified WorkSpaces.
*
*
* Rebuilding a WorkSpace is a potentially destructive action that can
* result in the loss of data. Rebuilding a WorkSpace causes the following
* to occur:
*
*
* - The system is restored to the image of the bundle that the WorkSpace
* is created from. Any applications that have been installed, or system
* settings that have been made since the WorkSpace was created will be
* lost.
* - The data drive (D drive) is re-created from the last automatic
* snapshot taken of the data drive. The current contents of the data drive
* are overwritten. Automatic snapshots of the data drive are taken every 12
* hours, so the snapshot can be as much as 12 hours old.
*
*
* To be able to rebuild a WorkSpace, the WorkSpace must have a State
* of AVAILABLE
or ERROR
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* been completely rebuilt.
*
*
*
* @param rebuildWorkspacesRequest
* Contains the inputs for the RebuildWorkspaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebuildWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.RebuildWorkspaces
*/
java.util.concurrent.Future rebuildWorkspacesAsync(
RebuildWorkspacesRequest rebuildWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Terminates the specified WorkSpaces.
*
*
* Terminating a WorkSpace is a permanent action and cannot be undone. The
* user's data is not maintained and will be destroyed. If you need to
* archive any user data, contact Amazon Web Services before terminating the
* WorkSpace.
*
*
* You can terminate a WorkSpace that is in any state except
* SUSPENDED
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* been completely terminated.
*
*
*
* @param terminateWorkspacesRequest
* Contains the inputs for the TerminateWorkspaces operation.
* @return A Java Future containing the result of the TerminateWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsync.TerminateWorkspaces
*/
java.util.concurrent.Future terminateWorkspacesAsync(
TerminateWorkspacesRequest terminateWorkspacesRequest);
/**
*
* Terminates the specified WorkSpaces.
*
*
* Terminating a WorkSpace is a permanent action and cannot be undone. The
* user's data is not maintained and will be destroyed. If you need to
* archive any user data, contact Amazon Web Services before terminating the
* WorkSpace.
*
*
* You can terminate a WorkSpace that is in any state except
* SUSPENDED
.
*
*
*
* This operation is asynchronous and will return before the WorkSpaces have
* been completely terminated.
*
*
*
* @param terminateWorkspacesRequest
* Contains the inputs for the TerminateWorkspaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TerminateWorkspaces
* operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.TerminateWorkspaces
*/
java.util.concurrent.Future terminateWorkspacesAsync(
TerminateWorkspacesRequest terminateWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}