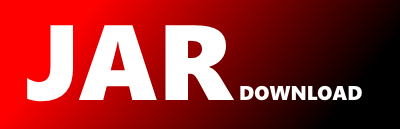
com.amazonaws.services.workspaces.AmazonWorkspacesAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspaces Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspaces;
import javax.annotation.Generated;
import com.amazonaws.services.workspaces.model.*;
/**
* Interface for accessing Amazon WorkSpaces asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.workspaces.AbstractAmazonWorkspacesAsync} instead.
*
*
* Amazon WorkSpaces Service
*
* Amazon WorkSpaces enables you to provision virtual, cloud-based Microsoft Windows desktops for your users.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonWorkspacesAsync extends AmazonWorkspaces {
/**
*
* Associates the specified IP access control group with the specified directory.
*
*
* @param associateIpGroupsRequest
* @return A Java Future containing the result of the AssociateIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsync.AssociateIpGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateIpGroupsAsync(AssociateIpGroupsRequest associateIpGroupsRequest);
/**
*
* Associates the specified IP access control group with the specified directory.
*
*
* @param associateIpGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.AssociateIpGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateIpGroupsAsync(AssociateIpGroupsRequest associateIpGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more rules to the specified IP access control group.
*
*
* This action gives users permission to access their WorkSpaces from the CIDR address ranges specified in the
* rules.
*
*
* @param authorizeIpRulesRequest
* @return A Java Future containing the result of the AuthorizeIpRules operation returned by the service.
* @sample AmazonWorkspacesAsync.AuthorizeIpRules
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeIpRulesAsync(AuthorizeIpRulesRequest authorizeIpRulesRequest);
/**
*
* Adds one or more rules to the specified IP access control group.
*
*
* This action gives users permission to access their WorkSpaces from the CIDR address ranges specified in the
* rules.
*
*
* @param authorizeIpRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeIpRules operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.AuthorizeIpRules
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeIpRulesAsync(AuthorizeIpRulesRequest authorizeIpRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IP access control group.
*
*
* An IP access control group provides you with the ability to control the IP addresses from which users are allowed
* to access their WorkSpaces. To specify the CIDR address ranges, add rules to your IP access control group and
* then associate the group with your directory. You can add rules when you create the group or at any time using
* AuthorizeIpRules.
*
*
* There is a default IP access control group associated with your directory. If you don't associate an IP access
* control group with your directory, the default group is used. The default group includes a default rule that
* allows users to access their WorkSpaces from anywhere. You cannot modify the default IP access control group for
* your directory.
*
*
* @param createIpGroupRequest
* @return A Java Future containing the result of the CreateIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsync.CreateIpGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpGroupAsync(CreateIpGroupRequest createIpGroupRequest);
/**
*
* Creates an IP access control group.
*
*
* An IP access control group provides you with the ability to control the IP addresses from which users are allowed
* to access their WorkSpaces. To specify the CIDR address ranges, add rules to your IP access control group and
* then associate the group with your directory. You can add rules when you create the group or at any time using
* AuthorizeIpRules.
*
*
* There is a default IP access control group associated with your directory. If you don't associate an IP access
* control group with your directory, the default group is used. The default group includes a default rule that
* allows users to access their WorkSpaces from anywhere. You cannot modify the default IP access control group for
* your directory.
*
*
* @param createIpGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.CreateIpGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpGroupAsync(CreateIpGroupRequest createIpGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the specified tags for the specified WorkSpace.
*
*
* @param createTagsRequest
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonWorkspacesAsync.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest);
/**
*
* Creates the specified tags for the specified WorkSpace.
*
*
* @param createTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates one or more WorkSpaces.
*
*
* This operation is asynchronous and returns before the WorkSpaces are created.
*
*
* @param createWorkspacesRequest
* @return A Java Future containing the result of the CreateWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.CreateWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkspacesAsync(CreateWorkspacesRequest createWorkspacesRequest);
/**
*
* Creates one or more WorkSpaces.
*
*
* This operation is asynchronous and returns before the WorkSpaces are created.
*
*
* @param createWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.CreateWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkspacesAsync(CreateWorkspacesRequest createWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified IP access control group.
*
*
* You cannot delete an IP access control group that is associated with a directory.
*
*
* @param deleteIpGroupRequest
* @return A Java Future containing the result of the DeleteIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsync.DeleteIpGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIpGroupAsync(DeleteIpGroupRequest deleteIpGroupRequest);
/**
*
* Deletes the specified IP access control group.
*
*
* You cannot delete an IP access control group that is associated with a directory.
*
*
* @param deleteIpGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DeleteIpGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIpGroupAsync(DeleteIpGroupRequest deleteIpGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified tags from the specified WorkSpace.
*
*
* @param deleteTagsRequest
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonWorkspacesAsync.DeleteTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest);
/**
*
* Deletes the specified tags from the specified WorkSpace.
*
*
* @param deleteTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DeleteTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more of your IP access control groups.
*
*
* @param describeIpGroupsRequest
* @return A Java Future containing the result of the DescribeIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeIpGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIpGroupsAsync(DescribeIpGroupsRequest describeIpGroupsRequest);
/**
*
* Describes one or more of your IP access control groups.
*
*
* @param describeIpGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeIpGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIpGroupsAsync(DescribeIpGroupsRequest describeIpGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified tags for the specified WorkSpace.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest);
/**
*
* Describes the specified tags for the specified WorkSpace.
*
*
* @param describeTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the available WorkSpace bundles.
*
*
* You can filter the results using either bundle ID or owner, but not both.
*
*
* @param describeWorkspaceBundlesRequest
* @return A Java Future containing the result of the DescribeWorkspaceBundles operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaceBundles
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest describeWorkspaceBundlesRequest);
/**
*
* Describes the available WorkSpace bundles.
*
*
* You can filter the results using either bundle ID or owner, but not both.
*
*
* @param describeWorkspaceBundlesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaceBundles operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaceBundles
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest describeWorkspaceBundlesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaceBundles operation.
*
* @see #describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest)
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaceBundles operation with an AsyncHandler.
*
* @see #describeWorkspaceBundlesAsync(DescribeWorkspaceBundlesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspaceBundlesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the available AWS Directory Service directories that are registered with Amazon WorkSpaces.
*
*
* @param describeWorkspaceDirectoriesRequest
* @return A Java Future containing the result of the DescribeWorkspaceDirectories operation returned by the
* service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaceDirectories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
DescribeWorkspaceDirectoriesRequest describeWorkspaceDirectoriesRequest);
/**
*
* Describes the available AWS Directory Service directories that are registered with Amazon WorkSpaces.
*
*
* @param describeWorkspaceDirectoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaceDirectories operation returned by the
* service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaceDirectories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
DescribeWorkspaceDirectoriesRequest describeWorkspaceDirectoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaceDirectories operation.
*
* @see #describeWorkspaceDirectoriesAsync(DescribeWorkspaceDirectoriesRequest)
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaceDirectories operation with an AsyncHandler.
*
* @see #describeWorkspaceDirectoriesAsync(DescribeWorkspaceDirectoriesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspaceDirectoriesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified WorkSpaces.
*
*
* You can filter the results using bundle ID, directory ID, or owner, but you can specify only one filter at a
* time.
*
*
* @param describeWorkspacesRequest
* @return A Java Future containing the result of the DescribeWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.DescribeWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeWorkspacesAsync(DescribeWorkspacesRequest describeWorkspacesRequest);
/**
*
* Describes the specified WorkSpaces.
*
*
* You can filter the results using bundle ID, directory ID, or owner, but you can specify only one filter at a
* time.
*
*
* @param describeWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeWorkspacesAsync(DescribeWorkspacesRequest describeWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeWorkspaces operation.
*
* @see #describeWorkspacesAsync(DescribeWorkspacesRequest)
*/
java.util.concurrent.Future describeWorkspacesAsync();
/**
* Simplified method form for invoking the DescribeWorkspaces operation with an AsyncHandler.
*
* @see #describeWorkspacesAsync(DescribeWorkspacesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeWorkspacesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the connection status of the specified WorkSpaces.
*
*
* @param describeWorkspacesConnectionStatusRequest
* @return A Java Future containing the result of the DescribeWorkspacesConnectionStatus operation returned by the
* service.
* @sample AmazonWorkspacesAsync.DescribeWorkspacesConnectionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspacesConnectionStatusAsync(
DescribeWorkspacesConnectionStatusRequest describeWorkspacesConnectionStatusRequest);
/**
*
* Describes the connection status of the specified WorkSpaces.
*
*
* @param describeWorkspacesConnectionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspacesConnectionStatus operation returned by the
* service.
* @sample AmazonWorkspacesAsyncHandler.DescribeWorkspacesConnectionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspacesConnectionStatusAsync(
DescribeWorkspacesConnectionStatusRequest describeWorkspacesConnectionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified IP access control group from the specified directory.
*
*
* @param disassociateIpGroupsRequest
* @return A Java Future containing the result of the DisassociateIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsync.DisassociateIpGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateIpGroupsAsync(DisassociateIpGroupsRequest disassociateIpGroupsRequest);
/**
*
* Disassociates the specified IP access control group from the specified directory.
*
*
* @param disassociateIpGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateIpGroups operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.DisassociateIpGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateIpGroupsAsync(DisassociateIpGroupsRequest disassociateIpGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified WorkSpace properties.
*
*
* @param modifyWorkspacePropertiesRequest
* @return A Java Future containing the result of the ModifyWorkspaceProperties operation returned by the service.
* @sample AmazonWorkspacesAsync.ModifyWorkspaceProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyWorkspacePropertiesAsync(
ModifyWorkspacePropertiesRequest modifyWorkspacePropertiesRequest);
/**
*
* Modifies the specified WorkSpace properties.
*
*
* @param modifyWorkspacePropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyWorkspaceProperties operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.ModifyWorkspaceProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyWorkspacePropertiesAsync(
ModifyWorkspacePropertiesRequest modifyWorkspacePropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the state of the specified WorkSpace.
*
*
* To maintain a WorkSpace without being interrupted, set the WorkSpace state to ADMIN_MAINTENANCE
.
* WorkSpaces in this state do not respond to requests to reboot, stop, start, or rebuild. An AutoStop WorkSpace in
* this state is not stopped. Users can log into a WorkSpace in the ADMIN_MAINTENANCE
state.
*
*
* @param modifyWorkspaceStateRequest
* @return A Java Future containing the result of the ModifyWorkspaceState operation returned by the service.
* @sample AmazonWorkspacesAsync.ModifyWorkspaceState
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyWorkspaceStateAsync(ModifyWorkspaceStateRequest modifyWorkspaceStateRequest);
/**
*
* Sets the state of the specified WorkSpace.
*
*
* To maintain a WorkSpace without being interrupted, set the WorkSpace state to ADMIN_MAINTENANCE
.
* WorkSpaces in this state do not respond to requests to reboot, stop, start, or rebuild. An AutoStop WorkSpace in
* this state is not stopped. Users can log into a WorkSpace in the ADMIN_MAINTENANCE
state.
*
*
* @param modifyWorkspaceStateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyWorkspaceState operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.ModifyWorkspaceState
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyWorkspaceStateAsync(ModifyWorkspaceStateRequest modifyWorkspaceStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reboots the specified WorkSpaces.
*
*
* You cannot reboot a WorkSpace unless its state is AVAILABLE
or UNHEALTHY
.
*
*
* This operation is asynchronous and returns before the WorkSpaces have rebooted.
*
*
* @param rebootWorkspacesRequest
* @return A Java Future containing the result of the RebootWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.RebootWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebootWorkspacesAsync(RebootWorkspacesRequest rebootWorkspacesRequest);
/**
*
* Reboots the specified WorkSpaces.
*
*
* You cannot reboot a WorkSpace unless its state is AVAILABLE
or UNHEALTHY
.
*
*
* This operation is asynchronous and returns before the WorkSpaces have rebooted.
*
*
* @param rebootWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.RebootWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebootWorkspacesAsync(RebootWorkspacesRequest rebootWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rebuilds the specified WorkSpace.
*
*
* You cannot rebuild a WorkSpace unless its state is AVAILABLE
, ERROR
, or
* UNHEALTHY
.
*
*
* Rebuilding a WorkSpace is a potentially destructive action that can result in the loss of data. For more
* information, see Rebuild a
* WorkSpace.
*
*
* This operation is asynchronous and returns before the WorkSpaces have been completely rebuilt.
*
*
* @param rebuildWorkspacesRequest
* @return A Java Future containing the result of the RebuildWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.RebuildWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebuildWorkspacesAsync(RebuildWorkspacesRequest rebuildWorkspacesRequest);
/**
*
* Rebuilds the specified WorkSpace.
*
*
* You cannot rebuild a WorkSpace unless its state is AVAILABLE
, ERROR
, or
* UNHEALTHY
.
*
*
* Rebuilding a WorkSpace is a potentially destructive action that can result in the loss of data. For more
* information, see Rebuild a
* WorkSpace.
*
*
* This operation is asynchronous and returns before the WorkSpaces have been completely rebuilt.
*
*
* @param rebuildWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebuildWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.RebuildWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebuildWorkspacesAsync(RebuildWorkspacesRequest rebuildWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more rules from the specified IP access control group.
*
*
* @param revokeIpRulesRequest
* @return A Java Future containing the result of the RevokeIpRules operation returned by the service.
* @sample AmazonWorkspacesAsync.RevokeIpRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future revokeIpRulesAsync(RevokeIpRulesRequest revokeIpRulesRequest);
/**
*
* Removes one or more rules from the specified IP access control group.
*
*
* @param revokeIpRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RevokeIpRules operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.RevokeIpRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future revokeIpRulesAsync(RevokeIpRulesRequest revokeIpRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the specified WorkSpaces.
*
*
* You cannot start a WorkSpace unless it has a running mode of AutoStop
and a state of
* STOPPED
.
*
*
* @param startWorkspacesRequest
* @return A Java Future containing the result of the StartWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.StartWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startWorkspacesAsync(StartWorkspacesRequest startWorkspacesRequest);
/**
*
* Starts the specified WorkSpaces.
*
*
* You cannot start a WorkSpace unless it has a running mode of AutoStop
and a state of
* STOPPED
.
*
*
* @param startWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.StartWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startWorkspacesAsync(StartWorkspacesRequest startWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops the specified WorkSpaces.
*
*
* You cannot stop a WorkSpace unless it has a running mode of AutoStop
and a state of
* AVAILABLE
, IMPAIRED
, UNHEALTHY
, or ERROR
.
*
*
* @param stopWorkspacesRequest
* @return A Java Future containing the result of the StopWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.StopWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopWorkspacesAsync(StopWorkspacesRequest stopWorkspacesRequest);
/**
*
* Stops the specified WorkSpaces.
*
*
* You cannot stop a WorkSpace unless it has a running mode of AutoStop
and a state of
* AVAILABLE
, IMPAIRED
, UNHEALTHY
, or ERROR
.
*
*
* @param stopWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.StopWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopWorkspacesAsync(StopWorkspacesRequest stopWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Terminates the specified WorkSpaces.
*
*
* Terminating a WorkSpace is a permanent action and cannot be undone. The user's data is destroyed. If you need to
* archive any user data, contact Amazon Web Services before terminating the WorkSpace.
*
*
* You can terminate a WorkSpace that is in any state except SUSPENDED
.
*
*
* This operation is asynchronous and returns before the WorkSpaces have been completely terminated.
*
*
* @param terminateWorkspacesRequest
* @return A Java Future containing the result of the TerminateWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsync.TerminateWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future terminateWorkspacesAsync(TerminateWorkspacesRequest terminateWorkspacesRequest);
/**
*
* Terminates the specified WorkSpaces.
*
*
* Terminating a WorkSpace is a permanent action and cannot be undone. The user's data is destroyed. If you need to
* archive any user data, contact Amazon Web Services before terminating the WorkSpace.
*
*
* You can terminate a WorkSpace that is in any state except SUSPENDED
.
*
*
* This operation is asynchronous and returns before the WorkSpaces have been completely terminated.
*
*
* @param terminateWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TerminateWorkspaces operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.TerminateWorkspaces
* @see AWS
* API Documentation
*/
java.util.concurrent.Future terminateWorkspacesAsync(TerminateWorkspacesRequest terminateWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Replaces the current rules of the specified IP access control group with the specified rules.
*
*
* @param updateRulesOfIpGroupRequest
* @return A Java Future containing the result of the UpdateRulesOfIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsync.UpdateRulesOfIpGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRulesOfIpGroupAsync(UpdateRulesOfIpGroupRequest updateRulesOfIpGroupRequest);
/**
*
* Replaces the current rules of the specified IP access control group with the specified rules.
*
*
* @param updateRulesOfIpGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRulesOfIpGroup operation returned by the service.
* @sample AmazonWorkspacesAsyncHandler.UpdateRulesOfIpGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRulesOfIpGroupAsync(UpdateRulesOfIpGroupRequest updateRulesOfIpGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}