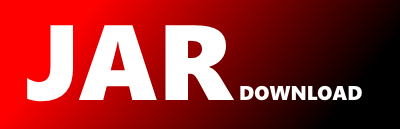
com.amazonaws.services.workspacesweb.AmazonWorkSpacesWebAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb;
import javax.annotation.Generated;
import com.amazonaws.services.workspacesweb.model.*;
/**
* Interface for accessing Amazon WorkSpaces Web asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.workspacesweb.AbstractAmazonWorkSpacesWebAsync} instead.
*
*
*
* WorkSpaces Web is a low cost, fully managed WorkSpace built specifically to facilitate secure, web-based workloads.
* WorkSpaces Web makes it easy for customers to safely provide their employees with access to internal websites and
* SaaS web applications without the administrative burden of appliances or specialized client software. WorkSpaces Web
* provides simple policy tools tailored for user interactions, while offloading common tasks like capacity management,
* scaling, and maintaining browser images.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonWorkSpacesWebAsync extends AmazonWorkSpacesWeb {
/**
*
* Associates a browser settings resource with a web portal.
*
*
* @param associateBrowserSettingsRequest
* @return A Java Future containing the result of the AssociateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.AssociateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateBrowserSettingsAsync(AssociateBrowserSettingsRequest associateBrowserSettingsRequest);
/**
*
* Associates a browser settings resource with a web portal.
*
*
* @param associateBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateBrowserSettingsAsync(AssociateBrowserSettingsRequest associateBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an IP access settings resource with a web portal.
*
*
* @param associateIpAccessSettingsRequest
* @return A Java Future containing the result of the AssociateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.AssociateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIpAccessSettingsAsync(
AssociateIpAccessSettingsRequest associateIpAccessSettingsRequest);
/**
*
* Associates an IP access settings resource with a web portal.
*
*
* @param associateIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIpAccessSettingsAsync(
AssociateIpAccessSettingsRequest associateIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a network settings resource with a web portal.
*
*
* @param associateNetworkSettingsRequest
* @return A Java Future containing the result of the AssociateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.AssociateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateNetworkSettingsAsync(AssociateNetworkSettingsRequest associateNetworkSettingsRequest);
/**
*
* Associates a network settings resource with a web portal.
*
*
* @param associateNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateNetworkSettingsAsync(AssociateNetworkSettingsRequest associateNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a trust store with a web portal.
*
*
* @param associateTrustStoreRequest
* @return A Java Future containing the result of the AssociateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.AssociateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTrustStoreAsync(AssociateTrustStoreRequest associateTrustStoreRequest);
/**
*
* Associates a trust store with a web portal.
*
*
* @param associateTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTrustStoreAsync(AssociateTrustStoreRequest associateTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a user access logging settings resource with a web portal.
*
*
* @param associateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the AssociateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.AssociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateUserAccessLoggingSettingsAsync(
AssociateUserAccessLoggingSettingsRequest associateUserAccessLoggingSettingsRequest);
/**
*
* Associates a user access logging settings resource with a web portal.
*
*
* @param associateUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateUserAccessLoggingSettingsAsync(
AssociateUserAccessLoggingSettingsRequest associateUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a user settings resource with a web portal.
*
*
* @param associateUserSettingsRequest
* @return A Java Future containing the result of the AssociateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.AssociateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateUserSettingsAsync(AssociateUserSettingsRequest associateUserSettingsRequest);
/**
*
* Associates a user settings resource with a web portal.
*
*
* @param associateUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.AssociateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future associateUserSettingsAsync(AssociateUserSettingsRequest associateUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a browser settings resource that can be associated with a web portal. Once associated with a web portal,
* browser settings control how the browser will behave once a user starts a streaming session for the web portal.
*
*
* @param createBrowserSettingsRequest
* @return A Java Future containing the result of the CreateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createBrowserSettingsAsync(CreateBrowserSettingsRequest createBrowserSettingsRequest);
/**
*
* Creates a browser settings resource that can be associated with a web portal. Once associated with a web portal,
* browser settings control how the browser will behave once a user starts a streaming session for the web portal.
*
*
* @param createBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createBrowserSettingsAsync(CreateBrowserSettingsRequest createBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an identity provider resource that is then associated with a web portal.
*
*
* @param createIdentityProviderRequest
* @return A Java Future containing the result of the CreateIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdentityProviderAsync(CreateIdentityProviderRequest createIdentityProviderRequest);
/**
*
* Creates an identity provider resource that is then associated with a web portal.
*
*
* @param createIdentityProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdentityProviderAsync(CreateIdentityProviderRequest createIdentityProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IP access settings resource that can be associated with a web portal.
*
*
* @param createIpAccessSettingsRequest
* @return A Java Future containing the result of the CreateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createIpAccessSettingsAsync(CreateIpAccessSettingsRequest createIpAccessSettingsRequest);
/**
*
* Creates an IP access settings resource that can be associated with a web portal.
*
*
* @param createIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createIpAccessSettingsAsync(CreateIpAccessSettingsRequest createIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a network settings resource that can be associated with a web portal. Once associated with a web portal,
* network settings define how streaming instances will connect with your specified VPC.
*
*
* @param createNetworkSettingsRequest
* @return A Java Future containing the result of the CreateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkSettingsAsync(CreateNetworkSettingsRequest createNetworkSettingsRequest);
/**
*
* Creates a network settings resource that can be associated with a web portal. Once associated with a web portal,
* network settings define how streaming instances will connect with your specified VPC.
*
*
* @param createNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkSettingsAsync(CreateNetworkSettingsRequest createNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a web portal.
*
*
* @param createPortalRequest
* @return A Java Future containing the result of the CreatePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreatePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPortalAsync(CreatePortalRequest createPortalRequest);
/**
*
* Creates a web portal.
*
*
* @param createPortalRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreatePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPortalAsync(CreatePortalRequest createPortalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a trust store that can be associated with a web portal. A trust store contains certificate authority (CA)
* certificates. Once associated with a web portal, the browser in a streaming session will recognize certificates
* that have been issued using any of the CAs in the trust store. If your organization has internal websites that
* use certificates issued by private CAs, you should add the private CA certificate to the trust store.
*
*
* @param createTrustStoreRequest
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrustStoreAsync(CreateTrustStoreRequest createTrustStoreRequest);
/**
*
* Creates a trust store that can be associated with a web portal. A trust store contains certificate authority (CA)
* certificates. Once associated with a web portal, the browser in a streaming session will recognize certificates
* that have been issued using any of the CAs in the trust store. If your organization has internal websites that
* use certificates issued by private CAs, you should add the private CA certificate to the trust store.
*
*
* @param createTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrustStoreAsync(CreateTrustStoreRequest createTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user access logging settings resource that can be associated with a web portal.
*
*
* @param createUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the CreateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.CreateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createUserAccessLoggingSettingsAsync(
CreateUserAccessLoggingSettingsRequest createUserAccessLoggingSettingsRequest);
/**
*
* Creates a user access logging settings resource that can be associated with a web portal.
*
*
* @param createUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createUserAccessLoggingSettingsAsync(
CreateUserAccessLoggingSettingsRequest createUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user settings resource that can be associated with a web portal. Once associated with a web portal,
* user settings control how users can transfer data between a streaming session and the their local devices.
*
*
* @param createUserSettingsRequest
* @return A Java Future containing the result of the CreateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.CreateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createUserSettingsAsync(CreateUserSettingsRequest createUserSettingsRequest);
/**
*
* Creates a user settings resource that can be associated with a web portal. Once associated with a web portal,
* user settings control how users can transfer data between a streaming session and the their local devices.
*
*
* @param createUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.CreateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future createUserSettingsAsync(CreateUserSettingsRequest createUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes browser settings.
*
*
* @param deleteBrowserSettingsRequest
* @return A Java Future containing the result of the DeleteBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBrowserSettingsAsync(DeleteBrowserSettingsRequest deleteBrowserSettingsRequest);
/**
*
* Deletes browser settings.
*
*
* @param deleteBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBrowserSettingsAsync(DeleteBrowserSettingsRequest deleteBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the identity provider.
*
*
* @param deleteIdentityProviderRequest
* @return A Java Future containing the result of the DeleteIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdentityProviderAsync(DeleteIdentityProviderRequest deleteIdentityProviderRequest);
/**
*
* Deletes the identity provider.
*
*
* @param deleteIdentityProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdentityProviderAsync(DeleteIdentityProviderRequest deleteIdentityProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes IP access settings.
*
*
* @param deleteIpAccessSettingsRequest
* @return A Java Future containing the result of the DeleteIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIpAccessSettingsAsync(DeleteIpAccessSettingsRequest deleteIpAccessSettingsRequest);
/**
*
* Deletes IP access settings.
*
*
* @param deleteIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIpAccessSettingsAsync(DeleteIpAccessSettingsRequest deleteIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes network settings.
*
*
* @param deleteNetworkSettingsRequest
* @return A Java Future containing the result of the DeleteNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteNetworkSettingsAsync(DeleteNetworkSettingsRequest deleteNetworkSettingsRequest);
/**
*
* Deletes network settings.
*
*
* @param deleteNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteNetworkSettingsAsync(DeleteNetworkSettingsRequest deleteNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a web portal.
*
*
* @param deletePortalRequest
* @return A Java Future containing the result of the DeletePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeletePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePortalAsync(DeletePortalRequest deletePortalRequest);
/**
*
* Deletes a web portal.
*
*
* @param deletePortalRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeletePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePortalAsync(DeletePortalRequest deletePortalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the trust store.
*
*
* @param deleteTrustStoreRequest
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTrustStoreAsync(DeleteTrustStoreRequest deleteTrustStoreRequest);
/**
*
* Deletes the trust store.
*
*
* @param deleteTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTrustStoreAsync(DeleteTrustStoreRequest deleteTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes user access logging settings.
*
*
* @param deleteUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the DeleteUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.DeleteUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserAccessLoggingSettingsAsync(
DeleteUserAccessLoggingSettingsRequest deleteUserAccessLoggingSettingsRequest);
/**
*
* Deletes user access logging settings.
*
*
* @param deleteUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserAccessLoggingSettingsAsync(
DeleteUserAccessLoggingSettingsRequest deleteUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes user settings.
*
*
* @param deleteUserSettingsRequest
* @return A Java Future containing the result of the DeleteUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DeleteUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserSettingsAsync(DeleteUserSettingsRequest deleteUserSettingsRequest);
/**
*
* Deletes user settings.
*
*
* @param deleteUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DeleteUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserSettingsAsync(DeleteUserSettingsRequest deleteUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates browser settings from a web portal.
*
*
* @param disassociateBrowserSettingsRequest
* @return A Java Future containing the result of the DisassociateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DisassociateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateBrowserSettingsAsync(
DisassociateBrowserSettingsRequest disassociateBrowserSettingsRequest);
/**
*
* Disassociates browser settings from a web portal.
*
*
* @param disassociateBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateBrowserSettingsAsync(
DisassociateBrowserSettingsRequest disassociateBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates IP access settings from a web portal.
*
*
* @param disassociateIpAccessSettingsRequest
* @return A Java Future containing the result of the DisassociateIpAccessSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.DisassociateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateIpAccessSettingsAsync(
DisassociateIpAccessSettingsRequest disassociateIpAccessSettingsRequest);
/**
*
* Disassociates IP access settings from a web portal.
*
*
* @param disassociateIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateIpAccessSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateIpAccessSettingsAsync(
DisassociateIpAccessSettingsRequest disassociateIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates network settings from a web portal.
*
*
* @param disassociateNetworkSettingsRequest
* @return A Java Future containing the result of the DisassociateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DisassociateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateNetworkSettingsAsync(
DisassociateNetworkSettingsRequest disassociateNetworkSettingsRequest);
/**
*
* Disassociates network settings from a web portal.
*
*
* @param disassociateNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateNetworkSettingsAsync(
DisassociateNetworkSettingsRequest disassociateNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a trust store from a web portal.
*
*
* @param disassociateTrustStoreRequest
* @return A Java Future containing the result of the DisassociateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DisassociateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTrustStoreAsync(DisassociateTrustStoreRequest disassociateTrustStoreRequest);
/**
*
* Disassociates a trust store from a web portal.
*
*
* @param disassociateTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTrustStoreAsync(DisassociateTrustStoreRequest disassociateTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates user access logging settings from a web portal.
*
*
* @param disassociateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the DisassociateUserAccessLoggingSettings operation returned by
* the service.
* @sample AmazonWorkSpacesWebAsync.DisassociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateUserAccessLoggingSettingsAsync(
DisassociateUserAccessLoggingSettingsRequest disassociateUserAccessLoggingSettingsRequest);
/**
*
* Disassociates user access logging settings from a web portal.
*
*
* @param disassociateUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateUserAccessLoggingSettings operation returned by
* the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateUserAccessLoggingSettingsAsync(
DisassociateUserAccessLoggingSettingsRequest disassociateUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates user settings from a web portal.
*
*
* @param disassociateUserSettingsRequest
* @return A Java Future containing the result of the DisassociateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.DisassociateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateUserSettingsAsync(DisassociateUserSettingsRequest disassociateUserSettingsRequest);
/**
*
* Disassociates user settings from a web portal.
*
*
* @param disassociateUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.DisassociateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateUserSettingsAsync(DisassociateUserSettingsRequest disassociateUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets browser settings.
*
*
* @param getBrowserSettingsRequest
* @return A Java Future containing the result of the GetBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getBrowserSettingsAsync(GetBrowserSettingsRequest getBrowserSettingsRequest);
/**
*
* Gets browser settings.
*
*
* @param getBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getBrowserSettingsAsync(GetBrowserSettingsRequest getBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the identity provider.
*
*
* @param getIdentityProviderRequest
* @return A Java Future containing the result of the GetIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdentityProviderAsync(GetIdentityProviderRequest getIdentityProviderRequest);
/**
*
* Gets the identity provider.
*
*
* @param getIdentityProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdentityProviderAsync(GetIdentityProviderRequest getIdentityProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the IP access settings.
*
*
* @param getIpAccessSettingsRequest
* @return A Java Future containing the result of the GetIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getIpAccessSettingsAsync(GetIpAccessSettingsRequest getIpAccessSettingsRequest);
/**
*
* Gets the IP access settings.
*
*
* @param getIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getIpAccessSettingsAsync(GetIpAccessSettingsRequest getIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the network settings.
*
*
* @param getNetworkSettingsRequest
* @return A Java Future containing the result of the GetNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getNetworkSettingsAsync(GetNetworkSettingsRequest getNetworkSettingsRequest);
/**
*
* Gets the network settings.
*
*
* @param getNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getNetworkSettingsAsync(GetNetworkSettingsRequest getNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the web portal.
*
*
* @param getPortalRequest
* @return A Java Future containing the result of the GetPortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetPortal
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPortalAsync(GetPortalRequest getPortalRequest);
/**
*
* Gets the web portal.
*
*
* @param getPortalRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetPortal
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPortalAsync(GetPortalRequest getPortalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the service provider metadata.
*
*
* @param getPortalServiceProviderMetadataRequest
* @return A Java Future containing the result of the GetPortalServiceProviderMetadata operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.GetPortalServiceProviderMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getPortalServiceProviderMetadataAsync(
GetPortalServiceProviderMetadataRequest getPortalServiceProviderMetadataRequest);
/**
*
* Gets the service provider metadata.
*
*
* @param getPortalServiceProviderMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPortalServiceProviderMetadata operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetPortalServiceProviderMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getPortalServiceProviderMetadataAsync(
GetPortalServiceProviderMetadataRequest getPortalServiceProviderMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the trust store.
*
*
* @param getTrustStoreRequest
* @return A Java Future containing the result of the GetTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetTrustStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTrustStoreAsync(GetTrustStoreRequest getTrustStoreRequest);
/**
*
* Gets the trust store.
*
*
* @param getTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetTrustStore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTrustStoreAsync(GetTrustStoreRequest getTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the trust store certificate.
*
*
* @param getTrustStoreCertificateRequest
* @return A Java Future containing the result of the GetTrustStoreCertificate operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetTrustStoreCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future getTrustStoreCertificateAsync(GetTrustStoreCertificateRequest getTrustStoreCertificateRequest);
/**
*
* Gets the trust store certificate.
*
*
* @param getTrustStoreCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTrustStoreCertificate operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetTrustStoreCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future getTrustStoreCertificateAsync(GetTrustStoreCertificateRequest getTrustStoreCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets user access logging settings.
*
*
* @param getUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the GetUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.GetUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getUserAccessLoggingSettingsAsync(
GetUserAccessLoggingSettingsRequest getUserAccessLoggingSettingsRequest);
/**
*
* Gets user access logging settings.
*
*
* @param getUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getUserAccessLoggingSettingsAsync(
GetUserAccessLoggingSettingsRequest getUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets user settings.
*
*
* @param getUserSettingsRequest
* @return A Java Future containing the result of the GetUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.GetUserSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUserSettingsAsync(GetUserSettingsRequest getUserSettingsRequest);
/**
*
* Gets user settings.
*
*
* @param getUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.GetUserSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUserSettingsAsync(GetUserSettingsRequest getUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of browser settings.
*
*
* @param listBrowserSettingsRequest
* @return A Java Future containing the result of the ListBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listBrowserSettingsAsync(ListBrowserSettingsRequest listBrowserSettingsRequest);
/**
*
* Retrieves a list of browser settings.
*
*
* @param listBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listBrowserSettingsAsync(ListBrowserSettingsRequest listBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of identity providers for a specific web portal.
*
*
* @param listIdentityProvidersRequest
* @return A Java Future containing the result of the ListIdentityProviders operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListIdentityProviders
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdentityProvidersAsync(ListIdentityProvidersRequest listIdentityProvidersRequest);
/**
*
* Retrieves a list of identity providers for a specific web portal.
*
*
* @param listIdentityProvidersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdentityProviders operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListIdentityProviders
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdentityProvidersAsync(ListIdentityProvidersRequest listIdentityProvidersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of IP access settings.
*
*
* @param listIpAccessSettingsRequest
* @return A Java Future containing the result of the ListIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listIpAccessSettingsAsync(ListIpAccessSettingsRequest listIpAccessSettingsRequest);
/**
*
* Retrieves a list of IP access settings.
*
*
* @param listIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listIpAccessSettingsAsync(ListIpAccessSettingsRequest listIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of network settings.
*
*
* @param listNetworkSettingsRequest
* @return A Java Future containing the result of the ListNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listNetworkSettingsAsync(ListNetworkSettingsRequest listNetworkSettingsRequest);
/**
*
* Retrieves a list of network settings.
*
*
* @param listNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listNetworkSettingsAsync(ListNetworkSettingsRequest listNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list or web portals.
*
*
* @param listPortalsRequest
* @return A Java Future containing the result of the ListPortals operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListPortals
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPortalsAsync(ListPortalsRequest listPortalsRequest);
/**
*
* Retrieves a list or web portals.
*
*
* @param listPortalsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPortals operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListPortals
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPortalsAsync(ListPortalsRequest listPortalsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of trust store certificates.
*
*
* @param listTrustStoreCertificatesRequest
* @return A Java Future containing the result of the ListTrustStoreCertificates operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListTrustStoreCertificates
* @see AWS API Documentation
*/
java.util.concurrent.Future listTrustStoreCertificatesAsync(
ListTrustStoreCertificatesRequest listTrustStoreCertificatesRequest);
/**
*
* Retrieves a list of trust store certificates.
*
*
* @param listTrustStoreCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrustStoreCertificates operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListTrustStoreCertificates
* @see AWS API Documentation
*/
java.util.concurrent.Future listTrustStoreCertificatesAsync(
ListTrustStoreCertificatesRequest listTrustStoreCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of trust stores.
*
*
* @param listTrustStoresRequest
* @return A Java Future containing the result of the ListTrustStores operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListTrustStores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTrustStoresAsync(ListTrustStoresRequest listTrustStoresRequest);
/**
*
* Retrieves a list of trust stores.
*
*
* @param listTrustStoresRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrustStores operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListTrustStores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTrustStoresAsync(ListTrustStoresRequest listTrustStoresRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of user access logging settings.
*
*
* @param listUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the ListUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listUserAccessLoggingSettingsAsync(
ListUserAccessLoggingSettingsRequest listUserAccessLoggingSettingsRequest);
/**
*
* Retrieves a list of user access logging settings.
*
*
* @param listUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listUserAccessLoggingSettingsAsync(
ListUserAccessLoggingSettingsRequest listUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of user settings.
*
*
* @param listUserSettingsRequest
* @return A Java Future containing the result of the ListUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.ListUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listUserSettingsAsync(ListUserSettingsRequest listUserSettingsRequest);
/**
*
* Retrieves a list of user settings.
*
*
* @param listUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.ListUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future listUserSettingsAsync(ListUserSettingsRequest listUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites one or more tags for the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or overwrites one or more tags for the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates browser settings.
*
*
* @param updateBrowserSettingsRequest
* @return A Java Future containing the result of the UpdateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBrowserSettingsAsync(UpdateBrowserSettingsRequest updateBrowserSettingsRequest);
/**
*
* Updates browser settings.
*
*
* @param updateBrowserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBrowserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateBrowserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBrowserSettingsAsync(UpdateBrowserSettingsRequest updateBrowserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the identity provider.
*
*
* @param updateIdentityProviderRequest
* @return A Java Future containing the result of the UpdateIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdentityProviderAsync(UpdateIdentityProviderRequest updateIdentityProviderRequest);
/**
*
* Updates the identity provider.
*
*
* @param updateIdentityProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIdentityProvider operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateIdentityProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdentityProviderAsync(UpdateIdentityProviderRequest updateIdentityProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates IP access settings.
*
*
* @param updateIpAccessSettingsRequest
* @return A Java Future containing the result of the UpdateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIpAccessSettingsAsync(UpdateIpAccessSettingsRequest updateIpAccessSettingsRequest);
/**
*
* Updates IP access settings.
*
*
* @param updateIpAccessSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIpAccessSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateIpAccessSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIpAccessSettingsAsync(UpdateIpAccessSettingsRequest updateIpAccessSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates network settings.
*
*
* @param updateNetworkSettingsRequest
* @return A Java Future containing the result of the UpdateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNetworkSettingsAsync(UpdateNetworkSettingsRequest updateNetworkSettingsRequest);
/**
*
* Updates network settings.
*
*
* @param updateNetworkSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateNetworkSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateNetworkSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNetworkSettingsAsync(UpdateNetworkSettingsRequest updateNetworkSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a web portal.
*
*
* @param updatePortalRequest
* @return A Java Future containing the result of the UpdatePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdatePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePortalAsync(UpdatePortalRequest updatePortalRequest);
/**
*
* Updates a web portal.
*
*
* @param updatePortalRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePortal operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdatePortal
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePortalAsync(UpdatePortalRequest updatePortalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the trust store.
*
*
* @param updateTrustStoreRequest
* @return A Java Future containing the result of the UpdateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTrustStoreAsync(UpdateTrustStoreRequest updateTrustStoreRequest);
/**
*
* Updates the trust store.
*
*
* @param updateTrustStoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTrustStore operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateTrustStore
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTrustStoreAsync(UpdateTrustStoreRequest updateTrustStoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the user access logging settings.
*
*
* @param updateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the UpdateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsync.UpdateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateUserAccessLoggingSettingsAsync(
UpdateUserAccessLoggingSettingsRequest updateUserAccessLoggingSettingsRequest);
/**
*
* Updates the user access logging settings.
*
*
* @param updateUserAccessLoggingSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUserAccessLoggingSettings operation returned by the
* service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateUserAccessLoggingSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateUserAccessLoggingSettingsAsync(
UpdateUserAccessLoggingSettingsRequest updateUserAccessLoggingSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the user settings.
*
*
* @param updateUserSettingsRequest
* @return A Java Future containing the result of the UpdateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsync.UpdateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateUserSettingsAsync(UpdateUserSettingsRequest updateUserSettingsRequest);
/**
*
* Updates the user settings.
*
*
* @param updateUserSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUserSettings operation returned by the service.
* @sample AmazonWorkSpacesWebAsyncHandler.UpdateUserSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateUserSettingsAsync(UpdateUserSettingsRequest updateUserSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}