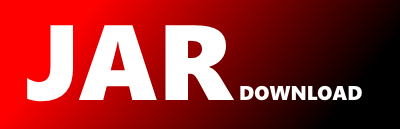
com.amazonaws.services.workspacesweb.model.UserSettingsSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The summary of user settings.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UserSettingsSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*/
private CookieSynchronizationConfiguration cookieSynchronizationConfiguration;
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*/
private String copyAllowed;
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*/
private Integer disconnectTimeoutInMinutes;
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*/
private String downloadAllowed;
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*/
private Integer idleDisconnectTimeoutInMinutes;
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*/
private String pasteAllowed;
/**
*
* Specifies whether the user can print to the local device.
*
*/
private String printAllowed;
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*/
private String uploadAllowed;
/**
*
* The ARN of the user settings.
*
*/
private String userSettingsArn;
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @param cookieSynchronizationConfiguration
* The configuration that specifies which cookies should be synchronized from the end user's local browser to
* the remote browser.
*/
public void setCookieSynchronizationConfiguration(CookieSynchronizationConfiguration cookieSynchronizationConfiguration) {
this.cookieSynchronizationConfiguration = cookieSynchronizationConfiguration;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @return The configuration that specifies which cookies should be synchronized from the end user's local browser
* to the remote browser.
*/
public CookieSynchronizationConfiguration getCookieSynchronizationConfiguration() {
return this.cookieSynchronizationConfiguration;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @param cookieSynchronizationConfiguration
* The configuration that specifies which cookies should be synchronized from the end user's local browser to
* the remote browser.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettingsSummary withCookieSynchronizationConfiguration(CookieSynchronizationConfiguration cookieSynchronizationConfiguration) {
setCookieSynchronizationConfiguration(cookieSynchronizationConfiguration);
return this;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public void setCopyAllowed(String copyAllowed) {
this.copyAllowed = copyAllowed;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @return Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public String getCopyAllowed() {
return this.copyAllowed;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withCopyAllowed(String copyAllowed) {
setCopyAllowed(copyAllowed);
return this;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withCopyAllowed(EnabledType copyAllowed) {
this.copyAllowed = copyAllowed.toString();
return this;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @param disconnectTimeoutInMinutes
* The amount of time that a streaming session remains active after users disconnect.
*/
public void setDisconnectTimeoutInMinutes(Integer disconnectTimeoutInMinutes) {
this.disconnectTimeoutInMinutes = disconnectTimeoutInMinutes;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @return The amount of time that a streaming session remains active after users disconnect.
*/
public Integer getDisconnectTimeoutInMinutes() {
return this.disconnectTimeoutInMinutes;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @param disconnectTimeoutInMinutes
* The amount of time that a streaming session remains active after users disconnect.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettingsSummary withDisconnectTimeoutInMinutes(Integer disconnectTimeoutInMinutes) {
setDisconnectTimeoutInMinutes(disconnectTimeoutInMinutes);
return this;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public void setDownloadAllowed(String downloadAllowed) {
this.downloadAllowed = downloadAllowed;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @return Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public String getDownloadAllowed() {
return this.downloadAllowed;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withDownloadAllowed(String downloadAllowed) {
setDownloadAllowed(downloadAllowed);
return this;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withDownloadAllowed(EnabledType downloadAllowed) {
this.downloadAllowed = downloadAllowed.toString();
return this;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @param idleDisconnectTimeoutInMinutes
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
*/
public void setIdleDisconnectTimeoutInMinutes(Integer idleDisconnectTimeoutInMinutes) {
this.idleDisconnectTimeoutInMinutes = idleDisconnectTimeoutInMinutes;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @return The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
*/
public Integer getIdleDisconnectTimeoutInMinutes() {
return this.idleDisconnectTimeoutInMinutes;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @param idleDisconnectTimeoutInMinutes
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettingsSummary withIdleDisconnectTimeoutInMinutes(Integer idleDisconnectTimeoutInMinutes) {
setIdleDisconnectTimeoutInMinutes(idleDisconnectTimeoutInMinutes);
return this;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public void setPasteAllowed(String pasteAllowed) {
this.pasteAllowed = pasteAllowed;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @return Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public String getPasteAllowed() {
return this.pasteAllowed;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withPasteAllowed(String pasteAllowed) {
setPasteAllowed(pasteAllowed);
return this;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withPasteAllowed(EnabledType pasteAllowed) {
this.pasteAllowed = pasteAllowed.toString();
return this;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @see EnabledType
*/
public void setPrintAllowed(String printAllowed) {
this.printAllowed = printAllowed;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @return Specifies whether the user can print to the local device.
* @see EnabledType
*/
public String getPrintAllowed() {
return this.printAllowed;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withPrintAllowed(String printAllowed) {
setPrintAllowed(printAllowed);
return this;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withPrintAllowed(EnabledType printAllowed) {
this.printAllowed = printAllowed.toString();
return this;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public void setUploadAllowed(String uploadAllowed) {
this.uploadAllowed = uploadAllowed;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @return Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public String getUploadAllowed() {
return this.uploadAllowed;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withUploadAllowed(String uploadAllowed) {
setUploadAllowed(uploadAllowed);
return this;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public UserSettingsSummary withUploadAllowed(EnabledType uploadAllowed) {
this.uploadAllowed = uploadAllowed.toString();
return this;
}
/**
*
* The ARN of the user settings.
*
*
* @param userSettingsArn
* The ARN of the user settings.
*/
public void setUserSettingsArn(String userSettingsArn) {
this.userSettingsArn = userSettingsArn;
}
/**
*
* The ARN of the user settings.
*
*
* @return The ARN of the user settings.
*/
public String getUserSettingsArn() {
return this.userSettingsArn;
}
/**
*
* The ARN of the user settings.
*
*
* @param userSettingsArn
* The ARN of the user settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettingsSummary withUserSettingsArn(String userSettingsArn) {
setUserSettingsArn(userSettingsArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCookieSynchronizationConfiguration() != null)
sb.append("CookieSynchronizationConfiguration: ").append("***Sensitive Data Redacted***").append(",");
if (getCopyAllowed() != null)
sb.append("CopyAllowed: ").append(getCopyAllowed()).append(",");
if (getDisconnectTimeoutInMinutes() != null)
sb.append("DisconnectTimeoutInMinutes: ").append(getDisconnectTimeoutInMinutes()).append(",");
if (getDownloadAllowed() != null)
sb.append("DownloadAllowed: ").append(getDownloadAllowed()).append(",");
if (getIdleDisconnectTimeoutInMinutes() != null)
sb.append("IdleDisconnectTimeoutInMinutes: ").append(getIdleDisconnectTimeoutInMinutes()).append(",");
if (getPasteAllowed() != null)
sb.append("PasteAllowed: ").append(getPasteAllowed()).append(",");
if (getPrintAllowed() != null)
sb.append("PrintAllowed: ").append(getPrintAllowed()).append(",");
if (getUploadAllowed() != null)
sb.append("UploadAllowed: ").append(getUploadAllowed()).append(",");
if (getUserSettingsArn() != null)
sb.append("UserSettingsArn: ").append(getUserSettingsArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UserSettingsSummary == false)
return false;
UserSettingsSummary other = (UserSettingsSummary) obj;
if (other.getCookieSynchronizationConfiguration() == null ^ this.getCookieSynchronizationConfiguration() == null)
return false;
if (other.getCookieSynchronizationConfiguration() != null
&& other.getCookieSynchronizationConfiguration().equals(this.getCookieSynchronizationConfiguration()) == false)
return false;
if (other.getCopyAllowed() == null ^ this.getCopyAllowed() == null)
return false;
if (other.getCopyAllowed() != null && other.getCopyAllowed().equals(this.getCopyAllowed()) == false)
return false;
if (other.getDisconnectTimeoutInMinutes() == null ^ this.getDisconnectTimeoutInMinutes() == null)
return false;
if (other.getDisconnectTimeoutInMinutes() != null && other.getDisconnectTimeoutInMinutes().equals(this.getDisconnectTimeoutInMinutes()) == false)
return false;
if (other.getDownloadAllowed() == null ^ this.getDownloadAllowed() == null)
return false;
if (other.getDownloadAllowed() != null && other.getDownloadAllowed().equals(this.getDownloadAllowed()) == false)
return false;
if (other.getIdleDisconnectTimeoutInMinutes() == null ^ this.getIdleDisconnectTimeoutInMinutes() == null)
return false;
if (other.getIdleDisconnectTimeoutInMinutes() != null
&& other.getIdleDisconnectTimeoutInMinutes().equals(this.getIdleDisconnectTimeoutInMinutes()) == false)
return false;
if (other.getPasteAllowed() == null ^ this.getPasteAllowed() == null)
return false;
if (other.getPasteAllowed() != null && other.getPasteAllowed().equals(this.getPasteAllowed()) == false)
return false;
if (other.getPrintAllowed() == null ^ this.getPrintAllowed() == null)
return false;
if (other.getPrintAllowed() != null && other.getPrintAllowed().equals(this.getPrintAllowed()) == false)
return false;
if (other.getUploadAllowed() == null ^ this.getUploadAllowed() == null)
return false;
if (other.getUploadAllowed() != null && other.getUploadAllowed().equals(this.getUploadAllowed()) == false)
return false;
if (other.getUserSettingsArn() == null ^ this.getUserSettingsArn() == null)
return false;
if (other.getUserSettingsArn() != null && other.getUserSettingsArn().equals(this.getUserSettingsArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCookieSynchronizationConfiguration() == null) ? 0 : getCookieSynchronizationConfiguration().hashCode());
hashCode = prime * hashCode + ((getCopyAllowed() == null) ? 0 : getCopyAllowed().hashCode());
hashCode = prime * hashCode + ((getDisconnectTimeoutInMinutes() == null) ? 0 : getDisconnectTimeoutInMinutes().hashCode());
hashCode = prime * hashCode + ((getDownloadAllowed() == null) ? 0 : getDownloadAllowed().hashCode());
hashCode = prime * hashCode + ((getIdleDisconnectTimeoutInMinutes() == null) ? 0 : getIdleDisconnectTimeoutInMinutes().hashCode());
hashCode = prime * hashCode + ((getPasteAllowed() == null) ? 0 : getPasteAllowed().hashCode());
hashCode = prime * hashCode + ((getPrintAllowed() == null) ? 0 : getPrintAllowed().hashCode());
hashCode = prime * hashCode + ((getUploadAllowed() == null) ? 0 : getUploadAllowed().hashCode());
hashCode = prime * hashCode + ((getUserSettingsArn() == null) ? 0 : getUserSettingsArn().hashCode());
return hashCode;
}
@Override
public UserSettingsSummary clone() {
try {
return (UserSettingsSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.workspacesweb.model.transform.UserSettingsSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}