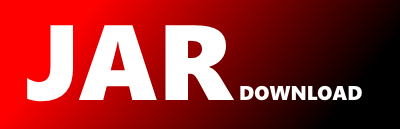
com.amazonaws.services.workspacesweb.model.CreateUserSettingsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateUserSettingsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The additional encryption context of the user settings.
*
*/
private java.util.Map additionalEncryptionContext;
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*/
private String clientToken;
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*/
private CookieSynchronizationConfiguration cookieSynchronizationConfiguration;
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*/
private String copyAllowed;
/**
*
* The customer managed key used to encrypt sensitive information in the user settings.
*
*/
private String customerManagedKey;
/**
*
* Specifies whether the user can use deep links that open automatically when connecting to a session.
*
*/
private String deepLinkAllowed;
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*/
private Integer disconnectTimeoutInMinutes;
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*/
private String downloadAllowed;
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*/
private Integer idleDisconnectTimeoutInMinutes;
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*/
private String pasteAllowed;
/**
*
* Specifies whether the user can print to the local device.
*
*/
private String printAllowed;
/**
*
* The tags to add to the user settings resource. A tag is a key-value pair.
*
*/
private java.util.List tags;
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*/
private String uploadAllowed;
/**
*
* The additional encryption context of the user settings.
*
*
* @return The additional encryption context of the user settings.
*/
public java.util.Map getAdditionalEncryptionContext() {
return additionalEncryptionContext;
}
/**
*
* The additional encryption context of the user settings.
*
*
* @param additionalEncryptionContext
* The additional encryption context of the user settings.
*/
public void setAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
this.additionalEncryptionContext = additionalEncryptionContext;
}
/**
*
* The additional encryption context of the user settings.
*
*
* @param additionalEncryptionContext
* The additional encryption context of the user settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
setAdditionalEncryptionContext(additionalEncryptionContext);
return this;
}
/**
* Add a single AdditionalEncryptionContext entry
*
* @see CreateUserSettingsRequest#withAdditionalEncryptionContext
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest addAdditionalEncryptionContextEntry(String key, String value) {
if (null == this.additionalEncryptionContext) {
this.additionalEncryptionContext = new java.util.HashMap();
}
if (this.additionalEncryptionContext.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.additionalEncryptionContext.put(key, value);
return this;
}
/**
* Removes all the entries added into AdditionalEncryptionContext.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest clearAdditionalEncryptionContextEntries() {
this.additionalEncryptionContext = null;
return this;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request
* completes successfully, subsequent retries with the same client token returns the result from the original
* successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* Idempotency ensures that an API request completes only once. With an idempotent request, if the original
* request completes successfully, subsequent retries with the same client token returns the result from the
* original successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request
* completes successfully, subsequent retries with the same client token returns the result from the original
* successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @param cookieSynchronizationConfiguration
* The configuration that specifies which cookies should be synchronized from the end user's local browser to
* the remote browser.
*/
public void setCookieSynchronizationConfiguration(CookieSynchronizationConfiguration cookieSynchronizationConfiguration) {
this.cookieSynchronizationConfiguration = cookieSynchronizationConfiguration;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @return The configuration that specifies which cookies should be synchronized from the end user's local browser
* to the remote browser.
*/
public CookieSynchronizationConfiguration getCookieSynchronizationConfiguration() {
return this.cookieSynchronizationConfiguration;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @param cookieSynchronizationConfiguration
* The configuration that specifies which cookies should be synchronized from the end user's local browser to
* the remote browser.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withCookieSynchronizationConfiguration(CookieSynchronizationConfiguration cookieSynchronizationConfiguration) {
setCookieSynchronizationConfiguration(cookieSynchronizationConfiguration);
return this;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public void setCopyAllowed(String copyAllowed) {
this.copyAllowed = copyAllowed;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @return Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public String getCopyAllowed() {
return this.copyAllowed;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withCopyAllowed(String copyAllowed) {
setCopyAllowed(copyAllowed);
return this;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* @param copyAllowed
* Specifies whether the user can copy text from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withCopyAllowed(EnabledType copyAllowed) {
this.copyAllowed = copyAllowed.toString();
return this;
}
/**
*
* The customer managed key used to encrypt sensitive information in the user settings.
*
*
* @param customerManagedKey
* The customer managed key used to encrypt sensitive information in the user settings.
*/
public void setCustomerManagedKey(String customerManagedKey) {
this.customerManagedKey = customerManagedKey;
}
/**
*
* The customer managed key used to encrypt sensitive information in the user settings.
*
*
* @return The customer managed key used to encrypt sensitive information in the user settings.
*/
public String getCustomerManagedKey() {
return this.customerManagedKey;
}
/**
*
* The customer managed key used to encrypt sensitive information in the user settings.
*
*
* @param customerManagedKey
* The customer managed key used to encrypt sensitive information in the user settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withCustomerManagedKey(String customerManagedKey) {
setCustomerManagedKey(customerManagedKey);
return this;
}
/**
*
* Specifies whether the user can use deep links that open automatically when connecting to a session.
*
*
* @param deepLinkAllowed
* Specifies whether the user can use deep links that open automatically when connecting to a session.
* @see EnabledType
*/
public void setDeepLinkAllowed(String deepLinkAllowed) {
this.deepLinkAllowed = deepLinkAllowed;
}
/**
*
* Specifies whether the user can use deep links that open automatically when connecting to a session.
*
*
* @return Specifies whether the user can use deep links that open automatically when connecting to a session.
* @see EnabledType
*/
public String getDeepLinkAllowed() {
return this.deepLinkAllowed;
}
/**
*
* Specifies whether the user can use deep links that open automatically when connecting to a session.
*
*
* @param deepLinkAllowed
* Specifies whether the user can use deep links that open automatically when connecting to a session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withDeepLinkAllowed(String deepLinkAllowed) {
setDeepLinkAllowed(deepLinkAllowed);
return this;
}
/**
*
* Specifies whether the user can use deep links that open automatically when connecting to a session.
*
*
* @param deepLinkAllowed
* Specifies whether the user can use deep links that open automatically when connecting to a session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withDeepLinkAllowed(EnabledType deepLinkAllowed) {
this.deepLinkAllowed = deepLinkAllowed.toString();
return this;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @param disconnectTimeoutInMinutes
* The amount of time that a streaming session remains active after users disconnect.
*/
public void setDisconnectTimeoutInMinutes(Integer disconnectTimeoutInMinutes) {
this.disconnectTimeoutInMinutes = disconnectTimeoutInMinutes;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @return The amount of time that a streaming session remains active after users disconnect.
*/
public Integer getDisconnectTimeoutInMinutes() {
return this.disconnectTimeoutInMinutes;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @param disconnectTimeoutInMinutes
* The amount of time that a streaming session remains active after users disconnect.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withDisconnectTimeoutInMinutes(Integer disconnectTimeoutInMinutes) {
setDisconnectTimeoutInMinutes(disconnectTimeoutInMinutes);
return this;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public void setDownloadAllowed(String downloadAllowed) {
this.downloadAllowed = downloadAllowed;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @return Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public String getDownloadAllowed() {
return this.downloadAllowed;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withDownloadAllowed(String downloadAllowed) {
setDownloadAllowed(downloadAllowed);
return this;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* @param downloadAllowed
* Specifies whether the user can download files from the streaming session to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withDownloadAllowed(EnabledType downloadAllowed) {
this.downloadAllowed = downloadAllowed.toString();
return this;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @param idleDisconnectTimeoutInMinutes
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
*/
public void setIdleDisconnectTimeoutInMinutes(Integer idleDisconnectTimeoutInMinutes) {
this.idleDisconnectTimeoutInMinutes = idleDisconnectTimeoutInMinutes;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @return The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
*/
public Integer getIdleDisconnectTimeoutInMinutes() {
return this.idleDisconnectTimeoutInMinutes;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @param idleDisconnectTimeoutInMinutes
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withIdleDisconnectTimeoutInMinutes(Integer idleDisconnectTimeoutInMinutes) {
setIdleDisconnectTimeoutInMinutes(idleDisconnectTimeoutInMinutes);
return this;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public void setPasteAllowed(String pasteAllowed) {
this.pasteAllowed = pasteAllowed;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @return Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public String getPasteAllowed() {
return this.pasteAllowed;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withPasteAllowed(String pasteAllowed) {
setPasteAllowed(pasteAllowed);
return this;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* @param pasteAllowed
* Specifies whether the user can paste text from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withPasteAllowed(EnabledType pasteAllowed) {
this.pasteAllowed = pasteAllowed.toString();
return this;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @see EnabledType
*/
public void setPrintAllowed(String printAllowed) {
this.printAllowed = printAllowed;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @return Specifies whether the user can print to the local device.
* @see EnabledType
*/
public String getPrintAllowed() {
return this.printAllowed;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withPrintAllowed(String printAllowed) {
setPrintAllowed(printAllowed);
return this;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* @param printAllowed
* Specifies whether the user can print to the local device.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withPrintAllowed(EnabledType printAllowed) {
this.printAllowed = printAllowed.toString();
return this;
}
/**
*
* The tags to add to the user settings resource. A tag is a key-value pair.
*
*
* @return The tags to add to the user settings resource. A tag is a key-value pair.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tags to add to the user settings resource. A tag is a key-value pair.
*
*
* @param tags
* The tags to add to the user settings resource. A tag is a key-value pair.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tags to add to the user settings resource. A tag is a key-value pair.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags to add to the user settings resource. A tag is a key-value pair.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags to add to the user settings resource. A tag is a key-value pair.
*
*
* @param tags
* The tags to add to the user settings resource. A tag is a key-value pair.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserSettingsRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public void setUploadAllowed(String uploadAllowed) {
this.uploadAllowed = uploadAllowed;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @return Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public String getUploadAllowed() {
return this.uploadAllowed;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withUploadAllowed(String uploadAllowed) {
setUploadAllowed(uploadAllowed);
return this;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* @param uploadAllowed
* Specifies whether the user can upload files from the local device to the streaming session.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnabledType
*/
public CreateUserSettingsRequest withUploadAllowed(EnabledType uploadAllowed) {
this.uploadAllowed = uploadAllowed.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdditionalEncryptionContext() != null)
sb.append("AdditionalEncryptionContext: ").append(getAdditionalEncryptionContext()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getCookieSynchronizationConfiguration() != null)
sb.append("CookieSynchronizationConfiguration: ").append("***Sensitive Data Redacted***").append(",");
if (getCopyAllowed() != null)
sb.append("CopyAllowed: ").append(getCopyAllowed()).append(",");
if (getCustomerManagedKey() != null)
sb.append("CustomerManagedKey: ").append(getCustomerManagedKey()).append(",");
if (getDeepLinkAllowed() != null)
sb.append("DeepLinkAllowed: ").append(getDeepLinkAllowed()).append(",");
if (getDisconnectTimeoutInMinutes() != null)
sb.append("DisconnectTimeoutInMinutes: ").append(getDisconnectTimeoutInMinutes()).append(",");
if (getDownloadAllowed() != null)
sb.append("DownloadAllowed: ").append(getDownloadAllowed()).append(",");
if (getIdleDisconnectTimeoutInMinutes() != null)
sb.append("IdleDisconnectTimeoutInMinutes: ").append(getIdleDisconnectTimeoutInMinutes()).append(",");
if (getPasteAllowed() != null)
sb.append("PasteAllowed: ").append(getPasteAllowed()).append(",");
if (getPrintAllowed() != null)
sb.append("PrintAllowed: ").append(getPrintAllowed()).append(",");
if (getTags() != null)
sb.append("Tags: ").append("***Sensitive Data Redacted***").append(",");
if (getUploadAllowed() != null)
sb.append("UploadAllowed: ").append(getUploadAllowed());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateUserSettingsRequest == false)
return false;
CreateUserSettingsRequest other = (CreateUserSettingsRequest) obj;
if (other.getAdditionalEncryptionContext() == null ^ this.getAdditionalEncryptionContext() == null)
return false;
if (other.getAdditionalEncryptionContext() != null && other.getAdditionalEncryptionContext().equals(this.getAdditionalEncryptionContext()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getCookieSynchronizationConfiguration() == null ^ this.getCookieSynchronizationConfiguration() == null)
return false;
if (other.getCookieSynchronizationConfiguration() != null
&& other.getCookieSynchronizationConfiguration().equals(this.getCookieSynchronizationConfiguration()) == false)
return false;
if (other.getCopyAllowed() == null ^ this.getCopyAllowed() == null)
return false;
if (other.getCopyAllowed() != null && other.getCopyAllowed().equals(this.getCopyAllowed()) == false)
return false;
if (other.getCustomerManagedKey() == null ^ this.getCustomerManagedKey() == null)
return false;
if (other.getCustomerManagedKey() != null && other.getCustomerManagedKey().equals(this.getCustomerManagedKey()) == false)
return false;
if (other.getDeepLinkAllowed() == null ^ this.getDeepLinkAllowed() == null)
return false;
if (other.getDeepLinkAllowed() != null && other.getDeepLinkAllowed().equals(this.getDeepLinkAllowed()) == false)
return false;
if (other.getDisconnectTimeoutInMinutes() == null ^ this.getDisconnectTimeoutInMinutes() == null)
return false;
if (other.getDisconnectTimeoutInMinutes() != null && other.getDisconnectTimeoutInMinutes().equals(this.getDisconnectTimeoutInMinutes()) == false)
return false;
if (other.getDownloadAllowed() == null ^ this.getDownloadAllowed() == null)
return false;
if (other.getDownloadAllowed() != null && other.getDownloadAllowed().equals(this.getDownloadAllowed()) == false)
return false;
if (other.getIdleDisconnectTimeoutInMinutes() == null ^ this.getIdleDisconnectTimeoutInMinutes() == null)
return false;
if (other.getIdleDisconnectTimeoutInMinutes() != null
&& other.getIdleDisconnectTimeoutInMinutes().equals(this.getIdleDisconnectTimeoutInMinutes()) == false)
return false;
if (other.getPasteAllowed() == null ^ this.getPasteAllowed() == null)
return false;
if (other.getPasteAllowed() != null && other.getPasteAllowed().equals(this.getPasteAllowed()) == false)
return false;
if (other.getPrintAllowed() == null ^ this.getPrintAllowed() == null)
return false;
if (other.getPrintAllowed() != null && other.getPrintAllowed().equals(this.getPrintAllowed()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getUploadAllowed() == null ^ this.getUploadAllowed() == null)
return false;
if (other.getUploadAllowed() != null && other.getUploadAllowed().equals(this.getUploadAllowed()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdditionalEncryptionContext() == null) ? 0 : getAdditionalEncryptionContext().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getCookieSynchronizationConfiguration() == null) ? 0 : getCookieSynchronizationConfiguration().hashCode());
hashCode = prime * hashCode + ((getCopyAllowed() == null) ? 0 : getCopyAllowed().hashCode());
hashCode = prime * hashCode + ((getCustomerManagedKey() == null) ? 0 : getCustomerManagedKey().hashCode());
hashCode = prime * hashCode + ((getDeepLinkAllowed() == null) ? 0 : getDeepLinkAllowed().hashCode());
hashCode = prime * hashCode + ((getDisconnectTimeoutInMinutes() == null) ? 0 : getDisconnectTimeoutInMinutes().hashCode());
hashCode = prime * hashCode + ((getDownloadAllowed() == null) ? 0 : getDownloadAllowed().hashCode());
hashCode = prime * hashCode + ((getIdleDisconnectTimeoutInMinutes() == null) ? 0 : getIdleDisconnectTimeoutInMinutes().hashCode());
hashCode = prime * hashCode + ((getPasteAllowed() == null) ? 0 : getPasteAllowed().hashCode());
hashCode = prime * hashCode + ((getPrintAllowed() == null) ? 0 : getPrintAllowed().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getUploadAllowed() == null) ? 0 : getUploadAllowed().hashCode());
return hashCode;
}
@Override
public CreateUserSettingsRequest clone() {
return (CreateUserSettingsRequest) super.clone();
}
}