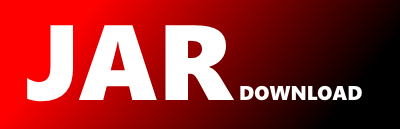
com.amazonaws.services.workspacesweb.model.PortalSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The summary of the portal.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PortalSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*/
private String authenticationType;
/**
*
* The ARN of the browser settings that is associated with the web portal.
*
*/
private String browserSettingsArn;
/**
*
* The browser type of the web portal.
*
*/
private String browserType;
/**
*
* The creation date of the web portal.
*
*/
private java.util.Date creationDate;
/**
*
* The name of the web portal.
*
*/
private String displayName;
/**
*
* The type and resources of the underlying instance.
*
*/
private String instanceType;
/**
*
* The ARN of the IP access settings.
*
*/
private String ipAccessSettingsArn;
/**
*
* The maximum number of concurrent sessions for the portal.
*
*/
private Integer maxConcurrentSessions;
/**
*
* The ARN of the network settings that is associated with the web portal.
*
*/
private String networkSettingsArn;
/**
*
* The ARN of the web portal.
*
*/
private String portalArn;
/**
*
* The endpoint URL of the web portal that users access in order to start streaming sessions.
*
*/
private String portalEndpoint;
/**
*
* The status of the web portal.
*
*/
private String portalStatus;
/**
*
* The renderer that is used in streaming sessions.
*
*/
private String rendererType;
/**
*
* The ARN of the trust that is associated with this web portal.
*
*/
private String trustStoreArn;
/**
*
* The ARN of the user access logging settings that is associated with the web portal.
*
*/
private String userAccessLoggingSettingsArn;
/**
*
* The ARN of the user settings that is associated with the web portal.
*
*/
private String userSettingsArn;
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @see AuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @return The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @see AuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public PortalSummary withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public PortalSummary withAuthenticationType(AuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
*
* The ARN of the browser settings that is associated with the web portal.
*
*
* @param browserSettingsArn
* The ARN of the browser settings that is associated with the web portal.
*/
public void setBrowserSettingsArn(String browserSettingsArn) {
this.browserSettingsArn = browserSettingsArn;
}
/**
*
* The ARN of the browser settings that is associated with the web portal.
*
*
* @return The ARN of the browser settings that is associated with the web portal.
*/
public String getBrowserSettingsArn() {
return this.browserSettingsArn;
}
/**
*
* The ARN of the browser settings that is associated with the web portal.
*
*
* @param browserSettingsArn
* The ARN of the browser settings that is associated with the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withBrowserSettingsArn(String browserSettingsArn) {
setBrowserSettingsArn(browserSettingsArn);
return this;
}
/**
*
* The browser type of the web portal.
*
*
* @param browserType
* The browser type of the web portal.
* @see BrowserType
*/
public void setBrowserType(String browserType) {
this.browserType = browserType;
}
/**
*
* The browser type of the web portal.
*
*
* @return The browser type of the web portal.
* @see BrowserType
*/
public String getBrowserType() {
return this.browserType;
}
/**
*
* The browser type of the web portal.
*
*
* @param browserType
* The browser type of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BrowserType
*/
public PortalSummary withBrowserType(String browserType) {
setBrowserType(browserType);
return this;
}
/**
*
* The browser type of the web portal.
*
*
* @param browserType
* The browser type of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BrowserType
*/
public PortalSummary withBrowserType(BrowserType browserType) {
this.browserType = browserType.toString();
return this;
}
/**
*
* The creation date of the web portal.
*
*
* @param creationDate
* The creation date of the web portal.
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The creation date of the web portal.
*
*
* @return The creation date of the web portal.
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The creation date of the web portal.
*
*
* @param creationDate
* The creation date of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
*
* The name of the web portal.
*
*
* @param displayName
* The name of the web portal.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* The name of the web portal.
*
*
* @return The name of the web portal.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* The name of the web portal.
*
*
* @param displayName
* The name of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @return The type and resources of the underlying instance.
* @see InstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public PortalSummary withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public PortalSummary withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The ARN of the IP access settings.
*
*
* @param ipAccessSettingsArn
* The ARN of the IP access settings.
*/
public void setIpAccessSettingsArn(String ipAccessSettingsArn) {
this.ipAccessSettingsArn = ipAccessSettingsArn;
}
/**
*
* The ARN of the IP access settings.
*
*
* @return The ARN of the IP access settings.
*/
public String getIpAccessSettingsArn() {
return this.ipAccessSettingsArn;
}
/**
*
* The ARN of the IP access settings.
*
*
* @param ipAccessSettingsArn
* The ARN of the IP access settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withIpAccessSettingsArn(String ipAccessSettingsArn) {
setIpAccessSettingsArn(ipAccessSettingsArn);
return this;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @param maxConcurrentSessions
* The maximum number of concurrent sessions for the portal.
*/
public void setMaxConcurrentSessions(Integer maxConcurrentSessions) {
this.maxConcurrentSessions = maxConcurrentSessions;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @return The maximum number of concurrent sessions for the portal.
*/
public Integer getMaxConcurrentSessions() {
return this.maxConcurrentSessions;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @param maxConcurrentSessions
* The maximum number of concurrent sessions for the portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withMaxConcurrentSessions(Integer maxConcurrentSessions) {
setMaxConcurrentSessions(maxConcurrentSessions);
return this;
}
/**
*
* The ARN of the network settings that is associated with the web portal.
*
*
* @param networkSettingsArn
* The ARN of the network settings that is associated with the web portal.
*/
public void setNetworkSettingsArn(String networkSettingsArn) {
this.networkSettingsArn = networkSettingsArn;
}
/**
*
* The ARN of the network settings that is associated with the web portal.
*
*
* @return The ARN of the network settings that is associated with the web portal.
*/
public String getNetworkSettingsArn() {
return this.networkSettingsArn;
}
/**
*
* The ARN of the network settings that is associated with the web portal.
*
*
* @param networkSettingsArn
* The ARN of the network settings that is associated with the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withNetworkSettingsArn(String networkSettingsArn) {
setNetworkSettingsArn(networkSettingsArn);
return this;
}
/**
*
* The ARN of the web portal.
*
*
* @param portalArn
* The ARN of the web portal.
*/
public void setPortalArn(String portalArn) {
this.portalArn = portalArn;
}
/**
*
* The ARN of the web portal.
*
*
* @return The ARN of the web portal.
*/
public String getPortalArn() {
return this.portalArn;
}
/**
*
* The ARN of the web portal.
*
*
* @param portalArn
* The ARN of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withPortalArn(String portalArn) {
setPortalArn(portalArn);
return this;
}
/**
*
* The endpoint URL of the web portal that users access in order to start streaming sessions.
*
*
* @param portalEndpoint
* The endpoint URL of the web portal that users access in order to start streaming sessions.
*/
public void setPortalEndpoint(String portalEndpoint) {
this.portalEndpoint = portalEndpoint;
}
/**
*
* The endpoint URL of the web portal that users access in order to start streaming sessions.
*
*
* @return The endpoint URL of the web portal that users access in order to start streaming sessions.
*/
public String getPortalEndpoint() {
return this.portalEndpoint;
}
/**
*
* The endpoint URL of the web portal that users access in order to start streaming sessions.
*
*
* @param portalEndpoint
* The endpoint URL of the web portal that users access in order to start streaming sessions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withPortalEndpoint(String portalEndpoint) {
setPortalEndpoint(portalEndpoint);
return this;
}
/**
*
* The status of the web portal.
*
*
* @param portalStatus
* The status of the web portal.
* @see PortalStatus
*/
public void setPortalStatus(String portalStatus) {
this.portalStatus = portalStatus;
}
/**
*
* The status of the web portal.
*
*
* @return The status of the web portal.
* @see PortalStatus
*/
public String getPortalStatus() {
return this.portalStatus;
}
/**
*
* The status of the web portal.
*
*
* @param portalStatus
* The status of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PortalStatus
*/
public PortalSummary withPortalStatus(String portalStatus) {
setPortalStatus(portalStatus);
return this;
}
/**
*
* The status of the web portal.
*
*
* @param portalStatus
* The status of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PortalStatus
*/
public PortalSummary withPortalStatus(PortalStatus portalStatus) {
this.portalStatus = portalStatus.toString();
return this;
}
/**
*
* The renderer that is used in streaming sessions.
*
*
* @param rendererType
* The renderer that is used in streaming sessions.
* @see RendererType
*/
public void setRendererType(String rendererType) {
this.rendererType = rendererType;
}
/**
*
* The renderer that is used in streaming sessions.
*
*
* @return The renderer that is used in streaming sessions.
* @see RendererType
*/
public String getRendererType() {
return this.rendererType;
}
/**
*
* The renderer that is used in streaming sessions.
*
*
* @param rendererType
* The renderer that is used in streaming sessions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RendererType
*/
public PortalSummary withRendererType(String rendererType) {
setRendererType(rendererType);
return this;
}
/**
*
* The renderer that is used in streaming sessions.
*
*
* @param rendererType
* The renderer that is used in streaming sessions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RendererType
*/
public PortalSummary withRendererType(RendererType rendererType) {
this.rendererType = rendererType.toString();
return this;
}
/**
*
* The ARN of the trust that is associated with this web portal.
*
*
* @param trustStoreArn
* The ARN of the trust that is associated with this web portal.
*/
public void setTrustStoreArn(String trustStoreArn) {
this.trustStoreArn = trustStoreArn;
}
/**
*
* The ARN of the trust that is associated with this web portal.
*
*
* @return The ARN of the trust that is associated with this web portal.
*/
public String getTrustStoreArn() {
return this.trustStoreArn;
}
/**
*
* The ARN of the trust that is associated with this web portal.
*
*
* @param trustStoreArn
* The ARN of the trust that is associated with this web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withTrustStoreArn(String trustStoreArn) {
setTrustStoreArn(trustStoreArn);
return this;
}
/**
*
* The ARN of the user access logging settings that is associated with the web portal.
*
*
* @param userAccessLoggingSettingsArn
* The ARN of the user access logging settings that is associated with the web portal.
*/
public void setUserAccessLoggingSettingsArn(String userAccessLoggingSettingsArn) {
this.userAccessLoggingSettingsArn = userAccessLoggingSettingsArn;
}
/**
*
* The ARN of the user access logging settings that is associated with the web portal.
*
*
* @return The ARN of the user access logging settings that is associated with the web portal.
*/
public String getUserAccessLoggingSettingsArn() {
return this.userAccessLoggingSettingsArn;
}
/**
*
* The ARN of the user access logging settings that is associated with the web portal.
*
*
* @param userAccessLoggingSettingsArn
* The ARN of the user access logging settings that is associated with the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withUserAccessLoggingSettingsArn(String userAccessLoggingSettingsArn) {
setUserAccessLoggingSettingsArn(userAccessLoggingSettingsArn);
return this;
}
/**
*
* The ARN of the user settings that is associated with the web portal.
*
*
* @param userSettingsArn
* The ARN of the user settings that is associated with the web portal.
*/
public void setUserSettingsArn(String userSettingsArn) {
this.userSettingsArn = userSettingsArn;
}
/**
*
* The ARN of the user settings that is associated with the web portal.
*
*
* @return The ARN of the user settings that is associated with the web portal.
*/
public String getUserSettingsArn() {
return this.userSettingsArn;
}
/**
*
* The ARN of the user settings that is associated with the web portal.
*
*
* @param userSettingsArn
* The ARN of the user settings that is associated with the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortalSummary withUserSettingsArn(String userSettingsArn) {
setUserSettingsArn(userSettingsArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType()).append(",");
if (getBrowserSettingsArn() != null)
sb.append("BrowserSettingsArn: ").append(getBrowserSettingsArn()).append(",");
if (getBrowserType() != null)
sb.append("BrowserType: ").append(getBrowserType()).append(",");
if (getCreationDate() != null)
sb.append("CreationDate: ").append(getCreationDate()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append("***Sensitive Data Redacted***").append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getIpAccessSettingsArn() != null)
sb.append("IpAccessSettingsArn: ").append(getIpAccessSettingsArn()).append(",");
if (getMaxConcurrentSessions() != null)
sb.append("MaxConcurrentSessions: ").append(getMaxConcurrentSessions()).append(",");
if (getNetworkSettingsArn() != null)
sb.append("NetworkSettingsArn: ").append(getNetworkSettingsArn()).append(",");
if (getPortalArn() != null)
sb.append("PortalArn: ").append(getPortalArn()).append(",");
if (getPortalEndpoint() != null)
sb.append("PortalEndpoint: ").append(getPortalEndpoint()).append(",");
if (getPortalStatus() != null)
sb.append("PortalStatus: ").append(getPortalStatus()).append(",");
if (getRendererType() != null)
sb.append("RendererType: ").append(getRendererType()).append(",");
if (getTrustStoreArn() != null)
sb.append("TrustStoreArn: ").append(getTrustStoreArn()).append(",");
if (getUserAccessLoggingSettingsArn() != null)
sb.append("UserAccessLoggingSettingsArn: ").append(getUserAccessLoggingSettingsArn()).append(",");
if (getUserSettingsArn() != null)
sb.append("UserSettingsArn: ").append(getUserSettingsArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PortalSummary == false)
return false;
PortalSummary other = (PortalSummary) obj;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
if (other.getBrowserSettingsArn() == null ^ this.getBrowserSettingsArn() == null)
return false;
if (other.getBrowserSettingsArn() != null && other.getBrowserSettingsArn().equals(this.getBrowserSettingsArn()) == false)
return false;
if (other.getBrowserType() == null ^ this.getBrowserType() == null)
return false;
if (other.getBrowserType() != null && other.getBrowserType().equals(this.getBrowserType()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getIpAccessSettingsArn() == null ^ this.getIpAccessSettingsArn() == null)
return false;
if (other.getIpAccessSettingsArn() != null && other.getIpAccessSettingsArn().equals(this.getIpAccessSettingsArn()) == false)
return false;
if (other.getMaxConcurrentSessions() == null ^ this.getMaxConcurrentSessions() == null)
return false;
if (other.getMaxConcurrentSessions() != null && other.getMaxConcurrentSessions().equals(this.getMaxConcurrentSessions()) == false)
return false;
if (other.getNetworkSettingsArn() == null ^ this.getNetworkSettingsArn() == null)
return false;
if (other.getNetworkSettingsArn() != null && other.getNetworkSettingsArn().equals(this.getNetworkSettingsArn()) == false)
return false;
if (other.getPortalArn() == null ^ this.getPortalArn() == null)
return false;
if (other.getPortalArn() != null && other.getPortalArn().equals(this.getPortalArn()) == false)
return false;
if (other.getPortalEndpoint() == null ^ this.getPortalEndpoint() == null)
return false;
if (other.getPortalEndpoint() != null && other.getPortalEndpoint().equals(this.getPortalEndpoint()) == false)
return false;
if (other.getPortalStatus() == null ^ this.getPortalStatus() == null)
return false;
if (other.getPortalStatus() != null && other.getPortalStatus().equals(this.getPortalStatus()) == false)
return false;
if (other.getRendererType() == null ^ this.getRendererType() == null)
return false;
if (other.getRendererType() != null && other.getRendererType().equals(this.getRendererType()) == false)
return false;
if (other.getTrustStoreArn() == null ^ this.getTrustStoreArn() == null)
return false;
if (other.getTrustStoreArn() != null && other.getTrustStoreArn().equals(this.getTrustStoreArn()) == false)
return false;
if (other.getUserAccessLoggingSettingsArn() == null ^ this.getUserAccessLoggingSettingsArn() == null)
return false;
if (other.getUserAccessLoggingSettingsArn() != null && other.getUserAccessLoggingSettingsArn().equals(this.getUserAccessLoggingSettingsArn()) == false)
return false;
if (other.getUserSettingsArn() == null ^ this.getUserSettingsArn() == null)
return false;
if (other.getUserSettingsArn() != null && other.getUserSettingsArn().equals(this.getUserSettingsArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
hashCode = prime * hashCode + ((getBrowserSettingsArn() == null) ? 0 : getBrowserSettingsArn().hashCode());
hashCode = prime * hashCode + ((getBrowserType() == null) ? 0 : getBrowserType().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getIpAccessSettingsArn() == null) ? 0 : getIpAccessSettingsArn().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrentSessions() == null) ? 0 : getMaxConcurrentSessions().hashCode());
hashCode = prime * hashCode + ((getNetworkSettingsArn() == null) ? 0 : getNetworkSettingsArn().hashCode());
hashCode = prime * hashCode + ((getPortalArn() == null) ? 0 : getPortalArn().hashCode());
hashCode = prime * hashCode + ((getPortalEndpoint() == null) ? 0 : getPortalEndpoint().hashCode());
hashCode = prime * hashCode + ((getPortalStatus() == null) ? 0 : getPortalStatus().hashCode());
hashCode = prime * hashCode + ((getRendererType() == null) ? 0 : getRendererType().hashCode());
hashCode = prime * hashCode + ((getTrustStoreArn() == null) ? 0 : getTrustStoreArn().hashCode());
hashCode = prime * hashCode + ((getUserAccessLoggingSettingsArn() == null) ? 0 : getUserAccessLoggingSettingsArn().hashCode());
hashCode = prime * hashCode + ((getUserSettingsArn() == null) ? 0 : getUserSettingsArn().hashCode());
return hashCode;
}
@Override
public PortalSummary clone() {
try {
return (PortalSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.workspacesweb.model.transform.PortalSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}