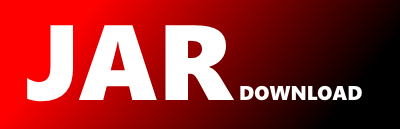
com.amazonaws.services.workspacesweb.AmazonWorkSpacesWeb Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.workspacesweb.model.*;
/**
* Interface for accessing Amazon WorkSpaces Web.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.workspacesweb.AbstractAmazonWorkSpacesWeb} instead.
*
*
*
* Amazon WorkSpaces Secure Browser is a low cost, fully managed WorkSpace built specifically to facilitate secure,
* web-based workloads. WorkSpaces Secure Browser makes it easy for customers to safely provide their employees with
* access to internal websites and SaaS web applications without the administrative burden of appliances or specialized
* client software. WorkSpaces Secure Browser provides simple policy tools tailored for user interactions, while
* offloading common tasks like capacity management, scaling, and maintaining browser images.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonWorkSpacesWeb {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "workspaces-web";
/**
*
* Associates a browser settings resource with a web portal.
*
*
* @param associateBrowserSettingsRequest
* @return Result of the AssociateBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateBrowserSettings
* @see AWS API Documentation
*/
AssociateBrowserSettingsResult associateBrowserSettings(AssociateBrowserSettingsRequest associateBrowserSettingsRequest);
/**
*
* Associates an IP access settings resource with a web portal.
*
*
* @param associateIpAccessSettingsRequest
* @return Result of the AssociateIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateIpAccessSettings
* @see AWS API Documentation
*/
AssociateIpAccessSettingsResult associateIpAccessSettings(AssociateIpAccessSettingsRequest associateIpAccessSettingsRequest);
/**
*
* Associates a network settings resource with a web portal.
*
*
* @param associateNetworkSettingsRequest
* @return Result of the AssociateNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateNetworkSettings
* @see AWS API Documentation
*/
AssociateNetworkSettingsResult associateNetworkSettings(AssociateNetworkSettingsRequest associateNetworkSettingsRequest);
/**
*
* Associates a trust store with a web portal.
*
*
* @param associateTrustStoreRequest
* @return Result of the AssociateTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateTrustStore
* @see AWS API Documentation
*/
AssociateTrustStoreResult associateTrustStore(AssociateTrustStoreRequest associateTrustStoreRequest);
/**
*
* Associates a user access logging settings resource with a web portal.
*
*
* @param associateUserAccessLoggingSettingsRequest
* @return Result of the AssociateUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
AssociateUserAccessLoggingSettingsResult associateUserAccessLoggingSettings(
AssociateUserAccessLoggingSettingsRequest associateUserAccessLoggingSettingsRequest);
/**
*
* Associates a user settings resource with a web portal.
*
*
* @param associateUserSettingsRequest
* @return Result of the AssociateUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.AssociateUserSettings
* @see AWS API Documentation
*/
AssociateUserSettingsResult associateUserSettings(AssociateUserSettingsRequest associateUserSettingsRequest);
/**
*
* Creates a browser settings resource that can be associated with a web portal. Once associated with a web portal,
* browser settings control how the browser will behave once a user starts a streaming session for the web portal.
*
*
* @param createBrowserSettingsRequest
* @return Result of the CreateBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateBrowserSettings
* @see AWS API Documentation
*/
CreateBrowserSettingsResult createBrowserSettings(CreateBrowserSettingsRequest createBrowserSettingsRequest);
/**
*
* Creates an identity provider resource that is then associated with a web portal.
*
*
* @param createIdentityProviderRequest
* @return Result of the CreateIdentityProvider operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateIdentityProvider
* @see AWS API Documentation
*/
CreateIdentityProviderResult createIdentityProvider(CreateIdentityProviderRequest createIdentityProviderRequest);
/**
*
* Creates an IP access settings resource that can be associated with a web portal.
*
*
* @param createIpAccessSettingsRequest
* @return Result of the CreateIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateIpAccessSettings
* @see AWS API Documentation
*/
CreateIpAccessSettingsResult createIpAccessSettings(CreateIpAccessSettingsRequest createIpAccessSettingsRequest);
/**
*
* Creates a network settings resource that can be associated with a web portal. Once associated with a web portal,
* network settings define how streaming instances will connect with your specified VPC.
*
*
* @param createNetworkSettingsRequest
* @return Result of the CreateNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateNetworkSettings
* @see AWS API Documentation
*/
CreateNetworkSettingsResult createNetworkSettings(CreateNetworkSettingsRequest createNetworkSettingsRequest);
/**
*
* Creates a web portal.
*
*
* @param createPortalRequest
* @return Result of the CreatePortal operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreatePortal
* @see AWS
* API Documentation
*/
CreatePortalResult createPortal(CreatePortalRequest createPortalRequest);
/**
*
* Creates a trust store that can be associated with a web portal. A trust store contains certificate authority (CA)
* certificates. Once associated with a web portal, the browser in a streaming session will recognize certificates
* that have been issued using any of the CAs in the trust store. If your organization has internal websites that
* use certificates issued by private CAs, you should add the private CA certificate to the trust store.
*
*
* @param createTrustStoreRequest
* @return Result of the CreateTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateTrustStore
* @see AWS API Documentation
*/
CreateTrustStoreResult createTrustStore(CreateTrustStoreRequest createTrustStoreRequest);
/**
*
* Creates a user access logging settings resource that can be associated with a web portal.
*
*
* @param createUserAccessLoggingSettingsRequest
* @return Result of the CreateUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateUserAccessLoggingSettings
* @see AWS API Documentation
*/
CreateUserAccessLoggingSettingsResult createUserAccessLoggingSettings(CreateUserAccessLoggingSettingsRequest createUserAccessLoggingSettingsRequest);
/**
*
* Creates a user settings resource that can be associated with a web portal. Once associated with a web portal,
* user settings control how users can transfer data between a streaming session and the their local devices.
*
*
* @param createUserSettingsRequest
* @return Result of the CreateUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.CreateUserSettings
* @see AWS API Documentation
*/
CreateUserSettingsResult createUserSettings(CreateUserSettingsRequest createUserSettingsRequest);
/**
*
* Deletes browser settings.
*
*
* @param deleteBrowserSettingsRequest
* @return Result of the DeleteBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteBrowserSettings
* @see AWS API Documentation
*/
DeleteBrowserSettingsResult deleteBrowserSettings(DeleteBrowserSettingsRequest deleteBrowserSettingsRequest);
/**
*
* Deletes the identity provider.
*
*
* @param deleteIdentityProviderRequest
* @return Result of the DeleteIdentityProvider operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteIdentityProvider
* @see AWS API Documentation
*/
DeleteIdentityProviderResult deleteIdentityProvider(DeleteIdentityProviderRequest deleteIdentityProviderRequest);
/**
*
* Deletes IP access settings.
*
*
* @param deleteIpAccessSettingsRequest
* @return Result of the DeleteIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteIpAccessSettings
* @see AWS API Documentation
*/
DeleteIpAccessSettingsResult deleteIpAccessSettings(DeleteIpAccessSettingsRequest deleteIpAccessSettingsRequest);
/**
*
* Deletes network settings.
*
*
* @param deleteNetworkSettingsRequest
* @return Result of the DeleteNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteNetworkSettings
* @see AWS API Documentation
*/
DeleteNetworkSettingsResult deleteNetworkSettings(DeleteNetworkSettingsRequest deleteNetworkSettingsRequest);
/**
*
* Deletes a web portal.
*
*
* @param deletePortalRequest
* @return Result of the DeletePortal operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeletePortal
* @see AWS
* API Documentation
*/
DeletePortalResult deletePortal(DeletePortalRequest deletePortalRequest);
/**
*
* Deletes the trust store.
*
*
* @param deleteTrustStoreRequest
* @return Result of the DeleteTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteTrustStore
* @see AWS API Documentation
*/
DeleteTrustStoreResult deleteTrustStore(DeleteTrustStoreRequest deleteTrustStoreRequest);
/**
*
* Deletes user access logging settings.
*
*
* @param deleteUserAccessLoggingSettingsRequest
* @return Result of the DeleteUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteUserAccessLoggingSettings
* @see AWS API Documentation
*/
DeleteUserAccessLoggingSettingsResult deleteUserAccessLoggingSettings(DeleteUserAccessLoggingSettingsRequest deleteUserAccessLoggingSettingsRequest);
/**
*
* Deletes user settings.
*
*
* @param deleteUserSettingsRequest
* @return Result of the DeleteUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DeleteUserSettings
* @see AWS API Documentation
*/
DeleteUserSettingsResult deleteUserSettings(DeleteUserSettingsRequest deleteUserSettingsRequest);
/**
*
* Disassociates browser settings from a web portal.
*
*
* @param disassociateBrowserSettingsRequest
* @return Result of the DisassociateBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateBrowserSettings
* @see AWS API Documentation
*/
DisassociateBrowserSettingsResult disassociateBrowserSettings(DisassociateBrowserSettingsRequest disassociateBrowserSettingsRequest);
/**
*
* Disassociates IP access settings from a web portal.
*
*
* @param disassociateIpAccessSettingsRequest
* @return Result of the DisassociateIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateIpAccessSettings
* @see AWS API Documentation
*/
DisassociateIpAccessSettingsResult disassociateIpAccessSettings(DisassociateIpAccessSettingsRequest disassociateIpAccessSettingsRequest);
/**
*
* Disassociates network settings from a web portal.
*
*
* @param disassociateNetworkSettingsRequest
* @return Result of the DisassociateNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateNetworkSettings
* @see AWS API Documentation
*/
DisassociateNetworkSettingsResult disassociateNetworkSettings(DisassociateNetworkSettingsRequest disassociateNetworkSettingsRequest);
/**
*
* Disassociates a trust store from a web portal.
*
*
* @param disassociateTrustStoreRequest
* @return Result of the DisassociateTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateTrustStore
* @see AWS API Documentation
*/
DisassociateTrustStoreResult disassociateTrustStore(DisassociateTrustStoreRequest disassociateTrustStoreRequest);
/**
*
* Disassociates user access logging settings from a web portal.
*
*
* @param disassociateUserAccessLoggingSettingsRequest
* @return Result of the DisassociateUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
DisassociateUserAccessLoggingSettingsResult disassociateUserAccessLoggingSettings(
DisassociateUserAccessLoggingSettingsRequest disassociateUserAccessLoggingSettingsRequest);
/**
*
* Disassociates user settings from a web portal.
*
*
* @param disassociateUserSettingsRequest
* @return Result of the DisassociateUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.DisassociateUserSettings
* @see AWS API Documentation
*/
DisassociateUserSettingsResult disassociateUserSettings(DisassociateUserSettingsRequest disassociateUserSettingsRequest);
/**
*
* Gets browser settings.
*
*
* @param getBrowserSettingsRequest
* @return Result of the GetBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetBrowserSettings
* @see AWS API Documentation
*/
GetBrowserSettingsResult getBrowserSettings(GetBrowserSettingsRequest getBrowserSettingsRequest);
/**
*
* Gets the identity provider.
*
*
* @param getIdentityProviderRequest
* @return Result of the GetIdentityProvider operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetIdentityProvider
* @see AWS API Documentation
*/
GetIdentityProviderResult getIdentityProvider(GetIdentityProviderRequest getIdentityProviderRequest);
/**
*
* Gets the IP access settings.
*
*
* @param getIpAccessSettingsRequest
* @return Result of the GetIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetIpAccessSettings
* @see AWS API Documentation
*/
GetIpAccessSettingsResult getIpAccessSettings(GetIpAccessSettingsRequest getIpAccessSettingsRequest);
/**
*
* Gets the network settings.
*
*
* @param getNetworkSettingsRequest
* @return Result of the GetNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetNetworkSettings
* @see AWS API Documentation
*/
GetNetworkSettingsResult getNetworkSettings(GetNetworkSettingsRequest getNetworkSettingsRequest);
/**
*
* Gets the web portal.
*
*
* @param getPortalRequest
* @return Result of the GetPortal operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetPortal
* @see AWS API
* Documentation
*/
GetPortalResult getPortal(GetPortalRequest getPortalRequest);
/**
*
* Gets the service provider metadata.
*
*
* @param getPortalServiceProviderMetadataRequest
* @return Result of the GetPortalServiceProviderMetadata operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetPortalServiceProviderMetadata
* @see AWS API Documentation
*/
GetPortalServiceProviderMetadataResult getPortalServiceProviderMetadata(GetPortalServiceProviderMetadataRequest getPortalServiceProviderMetadataRequest);
/**
*
* Gets the trust store.
*
*
* @param getTrustStoreRequest
* @return Result of the GetTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetTrustStore
* @see AWS
* API Documentation
*/
GetTrustStoreResult getTrustStore(GetTrustStoreRequest getTrustStoreRequest);
/**
*
* Gets the trust store certificate.
*
*
* @param getTrustStoreCertificateRequest
* @return Result of the GetTrustStoreCertificate operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetTrustStoreCertificate
* @see AWS API Documentation
*/
GetTrustStoreCertificateResult getTrustStoreCertificate(GetTrustStoreCertificateRequest getTrustStoreCertificateRequest);
/**
*
* Gets user access logging settings.
*
*
* @param getUserAccessLoggingSettingsRequest
* @return Result of the GetUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetUserAccessLoggingSettings
* @see AWS API Documentation
*/
GetUserAccessLoggingSettingsResult getUserAccessLoggingSettings(GetUserAccessLoggingSettingsRequest getUserAccessLoggingSettingsRequest);
/**
*
* Gets user settings.
*
*
* @param getUserSettingsRequest
* @return Result of the GetUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.GetUserSettings
* @see AWS
* API Documentation
*/
GetUserSettingsResult getUserSettings(GetUserSettingsRequest getUserSettingsRequest);
/**
*
* Retrieves a list of browser settings.
*
*
* @param listBrowserSettingsRequest
* @return Result of the ListBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListBrowserSettings
* @see AWS API Documentation
*/
ListBrowserSettingsResult listBrowserSettings(ListBrowserSettingsRequest listBrowserSettingsRequest);
/**
*
* Retrieves a list of identity providers for a specific web portal.
*
*
* @param listIdentityProvidersRequest
* @return Result of the ListIdentityProviders operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListIdentityProviders
* @see AWS API Documentation
*/
ListIdentityProvidersResult listIdentityProviders(ListIdentityProvidersRequest listIdentityProvidersRequest);
/**
*
* Retrieves a list of IP access settings.
*
*
* @param listIpAccessSettingsRequest
* @return Result of the ListIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListIpAccessSettings
* @see AWS API Documentation
*/
ListIpAccessSettingsResult listIpAccessSettings(ListIpAccessSettingsRequest listIpAccessSettingsRequest);
/**
*
* Retrieves a list of network settings.
*
*
* @param listNetworkSettingsRequest
* @return Result of the ListNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListNetworkSettings
* @see AWS API Documentation
*/
ListNetworkSettingsResult listNetworkSettings(ListNetworkSettingsRequest listNetworkSettingsRequest);
/**
*
* Retrieves a list or web portals.
*
*
* @param listPortalsRequest
* @return Result of the ListPortals operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListPortals
* @see AWS API
* Documentation
*/
ListPortalsResult listPortals(ListPortalsRequest listPortalsRequest);
/**
*
* Retrieves a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves a list of trust store certificates.
*
*
* @param listTrustStoreCertificatesRequest
* @return Result of the ListTrustStoreCertificates operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListTrustStoreCertificates
* @see AWS API Documentation
*/
ListTrustStoreCertificatesResult listTrustStoreCertificates(ListTrustStoreCertificatesRequest listTrustStoreCertificatesRequest);
/**
*
* Retrieves a list of trust stores.
*
*
* @param listTrustStoresRequest
* @return Result of the ListTrustStores operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListTrustStores
* @see AWS
* API Documentation
*/
ListTrustStoresResult listTrustStores(ListTrustStoresRequest listTrustStoresRequest);
/**
*
* Retrieves a list of user access logging settings.
*
*
* @param listUserAccessLoggingSettingsRequest
* @return Result of the ListUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
ListUserAccessLoggingSettingsResult listUserAccessLoggingSettings(ListUserAccessLoggingSettingsRequest listUserAccessLoggingSettingsRequest);
/**
*
* Retrieves a list of user settings.
*
*
* @param listUserSettingsRequest
* @return Result of the ListUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.ListUserSettings
* @see AWS API Documentation
*/
ListUserSettingsResult listUserSettings(ListUserSettingsRequest listUserSettingsRequest);
/**
*
* Adds or overwrites one or more tags for the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @throws TooManyTagsException
* There are too many tags.
* @sample AmazonWorkSpacesWeb.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates browser settings.
*
*
* @param updateBrowserSettingsRequest
* @return Result of the UpdateBrowserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateBrowserSettings
* @see AWS API Documentation
*/
UpdateBrowserSettingsResult updateBrowserSettings(UpdateBrowserSettingsRequest updateBrowserSettingsRequest);
/**
*
* Updates the identity provider.
*
*
* @param updateIdentityProviderRequest
* @return Result of the UpdateIdentityProvider operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateIdentityProvider
* @see AWS API Documentation
*/
UpdateIdentityProviderResult updateIdentityProvider(UpdateIdentityProviderRequest updateIdentityProviderRequest);
/**
*
* Updates IP access settings.
*
*
* @param updateIpAccessSettingsRequest
* @return Result of the UpdateIpAccessSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateIpAccessSettings
* @see AWS API Documentation
*/
UpdateIpAccessSettingsResult updateIpAccessSettings(UpdateIpAccessSettingsRequest updateIpAccessSettingsRequest);
/**
*
* Updates network settings.
*
*
* @param updateNetworkSettingsRequest
* @return Result of the UpdateNetworkSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateNetworkSettings
* @see AWS API Documentation
*/
UpdateNetworkSettingsResult updateNetworkSettings(UpdateNetworkSettingsRequest updateNetworkSettingsRequest);
/**
*
* Updates a web portal.
*
*
* @param updatePortalRequest
* @return Result of the UpdatePortal operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @throws ConflictException
* There is a conflict.
* @sample AmazonWorkSpacesWeb.UpdatePortal
* @see AWS
* API Documentation
*/
UpdatePortalResult updatePortal(UpdatePortalRequest updatePortalRequest);
/**
*
* Updates the trust store.
*
*
* @param updateTrustStoreRequest
* @return Result of the UpdateTrustStore operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateTrustStore
* @see AWS API Documentation
*/
UpdateTrustStoreResult updateTrustStore(UpdateTrustStoreRequest updateTrustStoreRequest);
/**
*
* Updates the user access logging settings.
*
*
* @param updateUserAccessLoggingSettingsRequest
* @return Result of the UpdateUserAccessLoggingSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateUserAccessLoggingSettings
* @see AWS API Documentation
*/
UpdateUserAccessLoggingSettingsResult updateUserAccessLoggingSettings(UpdateUserAccessLoggingSettingsRequest updateUserAccessLoggingSettingsRequest);
/**
*
* Updates the user settings.
*
*
* @param updateUserSettingsRequest
* @return Result of the UpdateUserSettings operation returned by the service.
* @throws InternalServerException
* There is an internal server error.
* @throws ResourceNotFoundException
* The resource cannot be found.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* There is a throttling error.
* @throws ValidationException
* There is a validation error.
* @sample AmazonWorkSpacesWeb.UpdateUserSettings
* @see AWS API Documentation
*/
UpdateUserSettingsResult updateUserSettings(UpdateUserSettingsRequest updateUserSettingsRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}