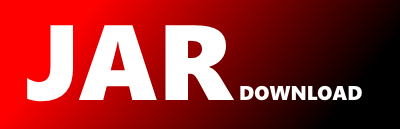
com.amazonaws.services.workspacesweb.model.CreatePortalRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-workspacesweb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.workspacesweb.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreatePortalRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The additional encryption context of the portal.
*
*/
private java.util.Map additionalEncryptionContext;
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*/
private String authenticationType;
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*/
private String clientToken;
/**
*
* The customer managed key of the web portal.
*
*/
private String customerManagedKey;
/**
*
* The name of the web portal. This is not visible to users who log into the web portal.
*
*/
private String displayName;
/**
*
* The type and resources of the underlying instance.
*
*/
private String instanceType;
/**
*
* The maximum number of concurrent sessions for the portal.
*
*/
private Integer maxConcurrentSessions;
/**
*
* The tags to add to the web portal. A tag is a key-value pair.
*
*/
private java.util.List tags;
/**
*
* The additional encryption context of the portal.
*
*
* @return The additional encryption context of the portal.
*/
public java.util.Map getAdditionalEncryptionContext() {
return additionalEncryptionContext;
}
/**
*
* The additional encryption context of the portal.
*
*
* @param additionalEncryptionContext
* The additional encryption context of the portal.
*/
public void setAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
this.additionalEncryptionContext = additionalEncryptionContext;
}
/**
*
* The additional encryption context of the portal.
*
*
* @param additionalEncryptionContext
* The additional encryption context of the portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
setAdditionalEncryptionContext(additionalEncryptionContext);
return this;
}
/**
* Add a single AdditionalEncryptionContext entry
*
* @see CreatePortalRequest#withAdditionalEncryptionContext
* @returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest addAdditionalEncryptionContextEntry(String key, String value) {
if (null == this.additionalEncryptionContext) {
this.additionalEncryptionContext = new java.util.HashMap();
}
if (this.additionalEncryptionContext.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.additionalEncryptionContext.put(key, value);
return this;
}
/**
* Removes all the entries added into AdditionalEncryptionContext.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest clearAdditionalEncryptionContextEntries() {
this.additionalEncryptionContext = null;
return this;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @see AuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @return The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @see AuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreatePortalRequest withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
*
* Standard
web portals are authenticated directly through your identity provider. You need to call
* CreateIdentityProvider
to integrate your identity provider with your web portal. User and group
* access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to Single
* Sign-On). Identity sources (including external identity provider integration), plus user and group access to your
* web portal, can be configured in the IAM Identity Center.
*
*
* @param authenticationType
* The type of authentication integration points used when signing into the web portal. Defaults to
* Standard
.
*
* Standard
web portals are authenticated directly through your identity provider. You need to
* call CreateIdentityProvider
to integrate your identity provider with your web portal. User
* and group access to your web portal is controlled through your identity provider.
*
*
* IAM Identity Center
web portals are authenticated through IAM Identity Center (successor to
* Single Sign-On). Identity sources (including external identity provider integration), plus user and group
* access to your web portal, can be configured in the IAM Identity Center.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreatePortalRequest withAuthenticationType(AuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request
* completes successfully, subsequent retries with the same client token returns the result from the original
* successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* Idempotency ensures that an API request completes only once. With an idempotent request, if the original
* request completes successfully, subsequent retries with the same client token returns the result from the
* original successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request completes
* successfully, subsequent retries with the same client token returns the result from the original successful
* request.
*
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Idempotency
* ensures that an API request completes only once. With an idempotent request, if the original request
* completes successfully, subsequent retries with the same client token returns the result from the original
* successful request.
*
* If you do not specify a client token, one is automatically generated by the Amazon Web Services SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The customer managed key of the web portal.
*
*
* @param customerManagedKey
* The customer managed key of the web portal.
*/
public void setCustomerManagedKey(String customerManagedKey) {
this.customerManagedKey = customerManagedKey;
}
/**
*
* The customer managed key of the web portal.
*
*
* @return The customer managed key of the web portal.
*/
public String getCustomerManagedKey() {
return this.customerManagedKey;
}
/**
*
* The customer managed key of the web portal.
*
*
* @param customerManagedKey
* The customer managed key of the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withCustomerManagedKey(String customerManagedKey) {
setCustomerManagedKey(customerManagedKey);
return this;
}
/**
*
* The name of the web portal. This is not visible to users who log into the web portal.
*
*
* @param displayName
* The name of the web portal. This is not visible to users who log into the web portal.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* The name of the web portal. This is not visible to users who log into the web portal.
*
*
* @return The name of the web portal. This is not visible to users who log into the web portal.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* The name of the web portal. This is not visible to users who log into the web portal.
*
*
* @param displayName
* The name of the web portal. This is not visible to users who log into the web portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @return The type and resources of the underlying instance.
* @see InstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public CreatePortalRequest withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The type and resources of the underlying instance.
*
*
* @param instanceType
* The type and resources of the underlying instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public CreatePortalRequest withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @param maxConcurrentSessions
* The maximum number of concurrent sessions for the portal.
*/
public void setMaxConcurrentSessions(Integer maxConcurrentSessions) {
this.maxConcurrentSessions = maxConcurrentSessions;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @return The maximum number of concurrent sessions for the portal.
*/
public Integer getMaxConcurrentSessions() {
return this.maxConcurrentSessions;
}
/**
*
* The maximum number of concurrent sessions for the portal.
*
*
* @param maxConcurrentSessions
* The maximum number of concurrent sessions for the portal.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withMaxConcurrentSessions(Integer maxConcurrentSessions) {
setMaxConcurrentSessions(maxConcurrentSessions);
return this;
}
/**
*
* The tags to add to the web portal. A tag is a key-value pair.
*
*
* @return The tags to add to the web portal. A tag is a key-value pair.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tags to add to the web portal. A tag is a key-value pair.
*
*
* @param tags
* The tags to add to the web portal. A tag is a key-value pair.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tags to add to the web portal. A tag is a key-value pair.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags to add to the web portal. A tag is a key-value pair.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags to add to the web portal. A tag is a key-value pair.
*
*
* @param tags
* The tags to add to the web portal. A tag is a key-value pair.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePortalRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdditionalEncryptionContext() != null)
sb.append("AdditionalEncryptionContext: ").append(getAdditionalEncryptionContext()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getCustomerManagedKey() != null)
sb.append("CustomerManagedKey: ").append(getCustomerManagedKey()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append("***Sensitive Data Redacted***").append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getMaxConcurrentSessions() != null)
sb.append("MaxConcurrentSessions: ").append(getMaxConcurrentSessions()).append(",");
if (getTags() != null)
sb.append("Tags: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreatePortalRequest == false)
return false;
CreatePortalRequest other = (CreatePortalRequest) obj;
if (other.getAdditionalEncryptionContext() == null ^ this.getAdditionalEncryptionContext() == null)
return false;
if (other.getAdditionalEncryptionContext() != null && other.getAdditionalEncryptionContext().equals(this.getAdditionalEncryptionContext()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getCustomerManagedKey() == null ^ this.getCustomerManagedKey() == null)
return false;
if (other.getCustomerManagedKey() != null && other.getCustomerManagedKey().equals(this.getCustomerManagedKey()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getMaxConcurrentSessions() == null ^ this.getMaxConcurrentSessions() == null)
return false;
if (other.getMaxConcurrentSessions() != null && other.getMaxConcurrentSessions().equals(this.getMaxConcurrentSessions()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdditionalEncryptionContext() == null) ? 0 : getAdditionalEncryptionContext().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getCustomerManagedKey() == null) ? 0 : getCustomerManagedKey().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrentSessions() == null) ? 0 : getMaxConcurrentSessions().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreatePortalRequest clone() {
return (CreatePortalRequest) super.clone();
}
}