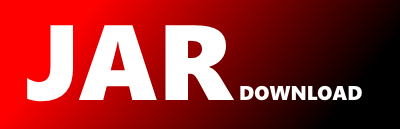
com.amazonaws.services.xray.model.TraceSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-xray Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.xray.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Metadata generated from the segment documents in a trace.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TraceSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the request that generated the trace's segments and subsegments.
*
*/
private String id;
/**
*
* The length of time in seconds between the start time of the root segment and the end time of the last segment
* that completed.
*
*/
private Double duration;
/**
*
* The length of time in seconds between the start and end times of the root segment. If the service performs work
* asynchronously, the response time measures the time before the response is sent to the user, while the duration
* measures the amount of time before the last traced activity completes.
*
*/
private Double responseTime;
/**
*
* One or more of the segment documents has a 500 series error.
*
*/
private Boolean hasFault;
/**
*
* One or more of the segment documents has a 400 series error.
*
*/
private Boolean hasError;
/**
*
* One or more of the segment documents has a 429 throttling error.
*
*/
private Boolean hasThrottle;
/**
*
* One or more of the segment documents is in progress.
*
*/
private Boolean isPartial;
/**
*
* Information about the HTTP request served by the trace.
*
*/
private Http http;
/**
*
* Annotations from the trace's segment documents.
*
*/
private java.util.Map> annotations;
/**
*
* Users from the trace's segment documents.
*
*/
private java.util.List users;
/**
*
* Service IDs from the trace's segment documents.
*
*/
private java.util.List serviceIds;
/**
*
* The unique identifier for the request that generated the trace's segments and subsegments.
*
*
* @param id
* The unique identifier for the request that generated the trace's segments and subsegments.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier for the request that generated the trace's segments and subsegments.
*
*
* @return The unique identifier for the request that generated the trace's segments and subsegments.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier for the request that generated the trace's segments and subsegments.
*
*
* @param id
* The unique identifier for the request that generated the trace's segments and subsegments.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withId(String id) {
setId(id);
return this;
}
/**
*
* The length of time in seconds between the start time of the root segment and the end time of the last segment
* that completed.
*
*
* @param duration
* The length of time in seconds between the start time of the root segment and the end time of the last
* segment that completed.
*/
public void setDuration(Double duration) {
this.duration = duration;
}
/**
*
* The length of time in seconds between the start time of the root segment and the end time of the last segment
* that completed.
*
*
* @return The length of time in seconds between the start time of the root segment and the end time of the last
* segment that completed.
*/
public Double getDuration() {
return this.duration;
}
/**
*
* The length of time in seconds between the start time of the root segment and the end time of the last segment
* that completed.
*
*
* @param duration
* The length of time in seconds between the start time of the root segment and the end time of the last
* segment that completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withDuration(Double duration) {
setDuration(duration);
return this;
}
/**
*
* The length of time in seconds between the start and end times of the root segment. If the service performs work
* asynchronously, the response time measures the time before the response is sent to the user, while the duration
* measures the amount of time before the last traced activity completes.
*
*
* @param responseTime
* The length of time in seconds between the start and end times of the root segment. If the service performs
* work asynchronously, the response time measures the time before the response is sent to the user, while
* the duration measures the amount of time before the last traced activity completes.
*/
public void setResponseTime(Double responseTime) {
this.responseTime = responseTime;
}
/**
*
* The length of time in seconds between the start and end times of the root segment. If the service performs work
* asynchronously, the response time measures the time before the response is sent to the user, while the duration
* measures the amount of time before the last traced activity completes.
*
*
* @return The length of time in seconds between the start and end times of the root segment. If the service
* performs work asynchronously, the response time measures the time before the response is sent to the
* user, while the duration measures the amount of time before the last traced activity completes.
*/
public Double getResponseTime() {
return this.responseTime;
}
/**
*
* The length of time in seconds between the start and end times of the root segment. If the service performs work
* asynchronously, the response time measures the time before the response is sent to the user, while the duration
* measures the amount of time before the last traced activity completes.
*
*
* @param responseTime
* The length of time in seconds between the start and end times of the root segment. If the service performs
* work asynchronously, the response time measures the time before the response is sent to the user, while
* the duration measures the amount of time before the last traced activity completes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withResponseTime(Double responseTime) {
setResponseTime(responseTime);
return this;
}
/**
*
* One or more of the segment documents has a 500 series error.
*
*
* @param hasFault
* One or more of the segment documents has a 500 series error.
*/
public void setHasFault(Boolean hasFault) {
this.hasFault = hasFault;
}
/**
*
* One or more of the segment documents has a 500 series error.
*
*
* @return One or more of the segment documents has a 500 series error.
*/
public Boolean getHasFault() {
return this.hasFault;
}
/**
*
* One or more of the segment documents has a 500 series error.
*
*
* @param hasFault
* One or more of the segment documents has a 500 series error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withHasFault(Boolean hasFault) {
setHasFault(hasFault);
return this;
}
/**
*
* One or more of the segment documents has a 500 series error.
*
*
* @return One or more of the segment documents has a 500 series error.
*/
public Boolean isHasFault() {
return this.hasFault;
}
/**
*
* One or more of the segment documents has a 400 series error.
*
*
* @param hasError
* One or more of the segment documents has a 400 series error.
*/
public void setHasError(Boolean hasError) {
this.hasError = hasError;
}
/**
*
* One or more of the segment documents has a 400 series error.
*
*
* @return One or more of the segment documents has a 400 series error.
*/
public Boolean getHasError() {
return this.hasError;
}
/**
*
* One or more of the segment documents has a 400 series error.
*
*
* @param hasError
* One or more of the segment documents has a 400 series error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withHasError(Boolean hasError) {
setHasError(hasError);
return this;
}
/**
*
* One or more of the segment documents has a 400 series error.
*
*
* @return One or more of the segment documents has a 400 series error.
*/
public Boolean isHasError() {
return this.hasError;
}
/**
*
* One or more of the segment documents has a 429 throttling error.
*
*
* @param hasThrottle
* One or more of the segment documents has a 429 throttling error.
*/
public void setHasThrottle(Boolean hasThrottle) {
this.hasThrottle = hasThrottle;
}
/**
*
* One or more of the segment documents has a 429 throttling error.
*
*
* @return One or more of the segment documents has a 429 throttling error.
*/
public Boolean getHasThrottle() {
return this.hasThrottle;
}
/**
*
* One or more of the segment documents has a 429 throttling error.
*
*
* @param hasThrottle
* One or more of the segment documents has a 429 throttling error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withHasThrottle(Boolean hasThrottle) {
setHasThrottle(hasThrottle);
return this;
}
/**
*
* One or more of the segment documents has a 429 throttling error.
*
*
* @return One or more of the segment documents has a 429 throttling error.
*/
public Boolean isHasThrottle() {
return this.hasThrottle;
}
/**
*
* One or more of the segment documents is in progress.
*
*
* @param isPartial
* One or more of the segment documents is in progress.
*/
public void setIsPartial(Boolean isPartial) {
this.isPartial = isPartial;
}
/**
*
* One or more of the segment documents is in progress.
*
*
* @return One or more of the segment documents is in progress.
*/
public Boolean getIsPartial() {
return this.isPartial;
}
/**
*
* One or more of the segment documents is in progress.
*
*
* @param isPartial
* One or more of the segment documents is in progress.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withIsPartial(Boolean isPartial) {
setIsPartial(isPartial);
return this;
}
/**
*
* One or more of the segment documents is in progress.
*
*
* @return One or more of the segment documents is in progress.
*/
public Boolean isPartial() {
return this.isPartial;
}
/**
*
* Information about the HTTP request served by the trace.
*
*
* @param http
* Information about the HTTP request served by the trace.
*/
public void setHttp(Http http) {
this.http = http;
}
/**
*
* Information about the HTTP request served by the trace.
*
*
* @return Information about the HTTP request served by the trace.
*/
public Http getHttp() {
return this.http;
}
/**
*
* Information about the HTTP request served by the trace.
*
*
* @param http
* Information about the HTTP request served by the trace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withHttp(Http http) {
setHttp(http);
return this;
}
/**
*
* Annotations from the trace's segment documents.
*
*
* @return Annotations from the trace's segment documents.
*/
public java.util.Map> getAnnotations() {
return annotations;
}
/**
*
* Annotations from the trace's segment documents.
*
*
* @param annotations
* Annotations from the trace's segment documents.
*/
public void setAnnotations(java.util.Map> annotations) {
this.annotations = annotations;
}
/**
*
* Annotations from the trace's segment documents.
*
*
* @param annotations
* Annotations from the trace's segment documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withAnnotations(java.util.Map> annotations) {
setAnnotations(annotations);
return this;
}
public TraceSummary addAnnotationsEntry(String key, java.util.List value) {
if (null == this.annotations) {
this.annotations = new java.util.HashMap>();
}
if (this.annotations.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.annotations.put(key, value);
return this;
}
/**
* Removes all the entries added into Annotations.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary clearAnnotationsEntries() {
this.annotations = null;
return this;
}
/**
*
* Users from the trace's segment documents.
*
*
* @return Users from the trace's segment documents.
*/
public java.util.List getUsers() {
return users;
}
/**
*
* Users from the trace's segment documents.
*
*
* @param users
* Users from the trace's segment documents.
*/
public void setUsers(java.util.Collection users) {
if (users == null) {
this.users = null;
return;
}
this.users = new java.util.ArrayList(users);
}
/**
*
* Users from the trace's segment documents.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUsers(java.util.Collection)} or {@link #withUsers(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param users
* Users from the trace's segment documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withUsers(TraceUser... users) {
if (this.users == null) {
setUsers(new java.util.ArrayList(users.length));
}
for (TraceUser ele : users) {
this.users.add(ele);
}
return this;
}
/**
*
* Users from the trace's segment documents.
*
*
* @param users
* Users from the trace's segment documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withUsers(java.util.Collection users) {
setUsers(users);
return this;
}
/**
*
* Service IDs from the trace's segment documents.
*
*
* @return Service IDs from the trace's segment documents.
*/
public java.util.List getServiceIds() {
return serviceIds;
}
/**
*
* Service IDs from the trace's segment documents.
*
*
* @param serviceIds
* Service IDs from the trace's segment documents.
*/
public void setServiceIds(java.util.Collection serviceIds) {
if (serviceIds == null) {
this.serviceIds = null;
return;
}
this.serviceIds = new java.util.ArrayList(serviceIds);
}
/**
*
* Service IDs from the trace's segment documents.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceIds(java.util.Collection)} or {@link #withServiceIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param serviceIds
* Service IDs from the trace's segment documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withServiceIds(ServiceId... serviceIds) {
if (this.serviceIds == null) {
setServiceIds(new java.util.ArrayList(serviceIds.length));
}
for (ServiceId ele : serviceIds) {
this.serviceIds.add(ele);
}
return this;
}
/**
*
* Service IDs from the trace's segment documents.
*
*
* @param serviceIds
* Service IDs from the trace's segment documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TraceSummary withServiceIds(java.util.Collection serviceIds) {
setServiceIds(serviceIds);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getDuration() != null)
sb.append("Duration: ").append(getDuration()).append(",");
if (getResponseTime() != null)
sb.append("ResponseTime: ").append(getResponseTime()).append(",");
if (getHasFault() != null)
sb.append("HasFault: ").append(getHasFault()).append(",");
if (getHasError() != null)
sb.append("HasError: ").append(getHasError()).append(",");
if (getHasThrottle() != null)
sb.append("HasThrottle: ").append(getHasThrottle()).append(",");
if (getIsPartial() != null)
sb.append("IsPartial: ").append(getIsPartial()).append(",");
if (getHttp() != null)
sb.append("Http: ").append(getHttp()).append(",");
if (getAnnotations() != null)
sb.append("Annotations: ").append(getAnnotations()).append(",");
if (getUsers() != null)
sb.append("Users: ").append(getUsers()).append(",");
if (getServiceIds() != null)
sb.append("ServiceIds: ").append(getServiceIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TraceSummary == false)
return false;
TraceSummary other = (TraceSummary) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getDuration() == null ^ this.getDuration() == null)
return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false)
return false;
if (other.getResponseTime() == null ^ this.getResponseTime() == null)
return false;
if (other.getResponseTime() != null && other.getResponseTime().equals(this.getResponseTime()) == false)
return false;
if (other.getHasFault() == null ^ this.getHasFault() == null)
return false;
if (other.getHasFault() != null && other.getHasFault().equals(this.getHasFault()) == false)
return false;
if (other.getHasError() == null ^ this.getHasError() == null)
return false;
if (other.getHasError() != null && other.getHasError().equals(this.getHasError()) == false)
return false;
if (other.getHasThrottle() == null ^ this.getHasThrottle() == null)
return false;
if (other.getHasThrottle() != null && other.getHasThrottle().equals(this.getHasThrottle()) == false)
return false;
if (other.getIsPartial() == null ^ this.getIsPartial() == null)
return false;
if (other.getIsPartial() != null && other.getIsPartial().equals(this.getIsPartial()) == false)
return false;
if (other.getHttp() == null ^ this.getHttp() == null)
return false;
if (other.getHttp() != null && other.getHttp().equals(this.getHttp()) == false)
return false;
if (other.getAnnotations() == null ^ this.getAnnotations() == null)
return false;
if (other.getAnnotations() != null && other.getAnnotations().equals(this.getAnnotations()) == false)
return false;
if (other.getUsers() == null ^ this.getUsers() == null)
return false;
if (other.getUsers() != null && other.getUsers().equals(this.getUsers()) == false)
return false;
if (other.getServiceIds() == null ^ this.getServiceIds() == null)
return false;
if (other.getServiceIds() != null && other.getServiceIds().equals(this.getServiceIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getResponseTime() == null) ? 0 : getResponseTime().hashCode());
hashCode = prime * hashCode + ((getHasFault() == null) ? 0 : getHasFault().hashCode());
hashCode = prime * hashCode + ((getHasError() == null) ? 0 : getHasError().hashCode());
hashCode = prime * hashCode + ((getHasThrottle() == null) ? 0 : getHasThrottle().hashCode());
hashCode = prime * hashCode + ((getIsPartial() == null) ? 0 : getIsPartial().hashCode());
hashCode = prime * hashCode + ((getHttp() == null) ? 0 : getHttp().hashCode());
hashCode = prime * hashCode + ((getAnnotations() == null) ? 0 : getAnnotations().hashCode());
hashCode = prime * hashCode + ((getUsers() == null) ? 0 : getUsers().hashCode());
hashCode = prime * hashCode + ((getServiceIds() == null) ? 0 : getServiceIds().hashCode());
return hashCode;
}
@Override
public TraceSummary clone() {
try {
return (TraceSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.xray.model.transform.TraceSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}