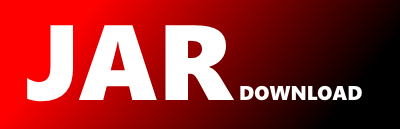
com.amazonaws.services.xray.AWSXRayAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-xray Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.xray;
import javax.annotation.Generated;
import com.amazonaws.services.xray.model.*;
/**
* Interface for accessing AWS X-Ray asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.xray.AbstractAWSXRayAsync} instead.
*
*
*
* AWS X-Ray provides APIs for managing debug traces and retrieving service maps and other data created by processing
* those traces.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSXRayAsync extends AWSXRay {
/**
*
* Retrieves a list of traces specified by ID. Each trace is a collection of segment documents that originates from
* a single request. Use GetTraceSummaries
to get a list of trace IDs.
*
*
* @param batchGetTracesRequest
* @return A Java Future containing the result of the BatchGetTraces operation returned by the service.
* @sample AWSXRayAsync.BatchGetTraces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetTracesAsync(BatchGetTracesRequest batchGetTracesRequest);
/**
*
* Retrieves a list of traces specified by ID. Each trace is a collection of segment documents that originates from
* a single request. Use GetTraceSummaries
to get a list of trace IDs.
*
*
* @param batchGetTracesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetTraces operation returned by the service.
* @sample AWSXRayAsyncHandler.BatchGetTraces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetTracesAsync(BatchGetTracesRequest batchGetTracesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a document that describes services that process incoming requests, and downstream services that they
* call as a result. Root services process incoming requests and make calls to downstream services. Root services
* are applications that use the AWS X-Ray SDK. Downstream services can be other applications, AWS resources, HTTP
* web APIs, or SQL databases.
*
*
* @param getServiceGraphRequest
* @return A Java Future containing the result of the GetServiceGraph operation returned by the service.
* @sample AWSXRayAsync.GetServiceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getServiceGraphAsync(GetServiceGraphRequest getServiceGraphRequest);
/**
*
* Retrieves a document that describes services that process incoming requests, and downstream services that they
* call as a result. Root services process incoming requests and make calls to downstream services. Root services
* are applications that use the AWS X-Ray SDK. Downstream services can be other applications, AWS resources, HTTP
* web APIs, or SQL databases.
*
*
* @param getServiceGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServiceGraph operation returned by the service.
* @sample AWSXRayAsyncHandler.GetServiceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getServiceGraphAsync(GetServiceGraphRequest getServiceGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a service graph for one or more specific trace IDs.
*
*
* @param getTraceGraphRequest
* @return A Java Future containing the result of the GetTraceGraph operation returned by the service.
* @sample AWSXRayAsync.GetTraceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceGraphAsync(GetTraceGraphRequest getTraceGraphRequest);
/**
*
* Retrieves a service graph for one or more specific trace IDs.
*
*
* @param getTraceGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTraceGraph operation returned by the service.
* @sample AWSXRayAsyncHandler.GetTraceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceGraphAsync(GetTraceGraphRequest getTraceGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves IDs and metadata for traces available for a specified time frame using an optional filter. To get the
* full traces, pass the trace IDs to BatchGetTraces
.
*
*
* @param getTraceSummariesRequest
* @return A Java Future containing the result of the GetTraceSummaries operation returned by the service.
* @sample AWSXRayAsync.GetTraceSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceSummariesAsync(GetTraceSummariesRequest getTraceSummariesRequest);
/**
*
* Retrieves IDs and metadata for traces available for a specified time frame using an optional filter. To get the
* full traces, pass the trace IDs to BatchGetTraces
.
*
*
* @param getTraceSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTraceSummaries operation returned by the service.
* @sample AWSXRayAsyncHandler.GetTraceSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceSummariesAsync(GetTraceSummariesRequest getTraceSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used by the AWS X-Ray daemon to upload telemetry.
*
*
* @param putTelemetryRecordsRequest
* @return A Java Future containing the result of the PutTelemetryRecords operation returned by the service.
* @sample AWSXRayAsync.PutTelemetryRecords
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTelemetryRecordsAsync(PutTelemetryRecordsRequest putTelemetryRecordsRequest);
/**
*
* Used by the AWS X-Ray daemon to upload telemetry.
*
*
* @param putTelemetryRecordsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTelemetryRecords operation returned by the service.
* @sample AWSXRayAsyncHandler.PutTelemetryRecords
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTelemetryRecordsAsync(PutTelemetryRecordsRequest putTelemetryRecordsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads segment documents to AWS X-Ray. The X-Ray SDK generates segment documents and sends them to the X-Ray
* daemon, which uploads them in batches. A segment document can be a completed segment, an in-progress segment, or
* an array of subsegments.
*
*
* @param putTraceSegmentsRequest
* @return A Java Future containing the result of the PutTraceSegments operation returned by the service.
* @sample AWSXRayAsync.PutTraceSegments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTraceSegmentsAsync(PutTraceSegmentsRequest putTraceSegmentsRequest);
/**
*
* Uploads segment documents to AWS X-Ray. The X-Ray SDK generates segment documents and sends them to the X-Ray
* daemon, which uploads them in batches. A segment document can be a completed segment, an in-progress segment, or
* an array of subsegments.
*
*
* @param putTraceSegmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTraceSegments operation returned by the service.
* @sample AWSXRayAsyncHandler.PutTraceSegments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTraceSegmentsAsync(PutTraceSegmentsRequest putTraceSegmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}