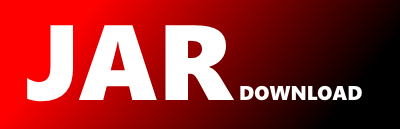
com.amazonaws.services.xray.AWSXRayAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-xray Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.xray;
import javax.annotation.Generated;
import com.amazonaws.services.xray.model.*;
/**
* Interface for accessing AWS X-Ray asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.xray.AbstractAWSXRayAsync} instead.
*
*
*
* Amazon Web Services X-Ray provides APIs for managing debug traces and retrieving service maps and other data created
* by processing those traces.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSXRayAsync extends AWSXRay {
/**
*
* Retrieves a list of traces specified by ID. Each trace is a collection of segment documents that originates from
* a single request. Use GetTraceSummaries
to get a list of trace IDs.
*
*
* @param batchGetTracesRequest
* @return A Java Future containing the result of the BatchGetTraces operation returned by the service.
* @sample AWSXRayAsync.BatchGetTraces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetTracesAsync(BatchGetTracesRequest batchGetTracesRequest);
/**
*
* Retrieves a list of traces specified by ID. Each trace is a collection of segment documents that originates from
* a single request. Use GetTraceSummaries
to get a list of trace IDs.
*
*
* @param batchGetTracesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetTraces operation returned by the service.
* @sample AWSXRayAsyncHandler.BatchGetTraces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetTracesAsync(BatchGetTracesRequest batchGetTracesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a group resource with a name and a filter expression.
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AWSXRayAsync.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest);
/**
*
* Creates a group resource with a name and a filter expression.
*
*
* @param createGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AWSXRayAsyncHandler.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a rule to control sampling behavior for instrumented applications. Services retrieve rules with GetSamplingRules, and evaluate
* each rule in ascending order of priority for each request. If a rule matches, the service records a trace,
* borrowing it from the reservoir size. After 10 seconds, the service reports back to X-Ray with GetSamplingTargets to get
* updated versions of each in-use rule. The updated rule contains a trace quota that the service can use instead of
* borrowing from the reservoir.
*
*
* @param createSamplingRuleRequest
* @return A Java Future containing the result of the CreateSamplingRule operation returned by the service.
* @sample AWSXRayAsync.CreateSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSamplingRuleAsync(CreateSamplingRuleRequest createSamplingRuleRequest);
/**
*
* Creates a rule to control sampling behavior for instrumented applications. Services retrieve rules with GetSamplingRules, and evaluate
* each rule in ascending order of priority for each request. If a rule matches, the service records a trace,
* borrowing it from the reservoir size. After 10 seconds, the service reports back to X-Ray with GetSamplingTargets to get
* updated versions of each in-use rule. The updated rule contains a trace quota that the service can use instead of
* borrowing from the reservoir.
*
*
* @param createSamplingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSamplingRule operation returned by the service.
* @sample AWSXRayAsyncHandler.CreateSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSamplingRuleAsync(CreateSamplingRuleRequest createSamplingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a group resource.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AWSXRayAsync.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest);
/**
*
* Deletes a group resource.
*
*
* @param deleteGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AWSXRayAsyncHandler.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a sampling rule.
*
*
* @param deleteSamplingRuleRequest
* @return A Java Future containing the result of the DeleteSamplingRule operation returned by the service.
* @sample AWSXRayAsync.DeleteSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSamplingRuleAsync(DeleteSamplingRuleRequest deleteSamplingRuleRequest);
/**
*
* Deletes a sampling rule.
*
*
* @param deleteSamplingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSamplingRule operation returned by the service.
* @sample AWSXRayAsyncHandler.DeleteSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSamplingRuleAsync(DeleteSamplingRuleRequest deleteSamplingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current encryption configuration for X-Ray data.
*
*
* @param getEncryptionConfigRequest
* @return A Java Future containing the result of the GetEncryptionConfig operation returned by the service.
* @sample AWSXRayAsync.GetEncryptionConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEncryptionConfigAsync(GetEncryptionConfigRequest getEncryptionConfigRequest);
/**
*
* Retrieves the current encryption configuration for X-Ray data.
*
*
* @param getEncryptionConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEncryptionConfig operation returned by the service.
* @sample AWSXRayAsyncHandler.GetEncryptionConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEncryptionConfigAsync(GetEncryptionConfigRequest getEncryptionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves group resource details.
*
*
* @param getGroupRequest
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* @sample AWSXRayAsync.GetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupAsync(GetGroupRequest getGroupRequest);
/**
*
* Retrieves group resource details.
*
*
* @param getGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* @sample AWSXRayAsyncHandler.GetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupAsync(GetGroupRequest getGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all active group details.
*
*
* @param getGroupsRequest
* @return A Java Future containing the result of the GetGroups operation returned by the service.
* @sample AWSXRayAsync.GetGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupsAsync(GetGroupsRequest getGroupsRequest);
/**
*
* Retrieves all active group details.
*
*
* @param getGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroups operation returned by the service.
* @sample AWSXRayAsyncHandler.GetGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupsAsync(GetGroupsRequest getGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the summary information of an insight. This includes impact to clients and root cause services, the top
* anomalous services, the category, the state of the insight, and the start and end time of the insight.
*
*
* @param getInsightRequest
* @return A Java Future containing the result of the GetInsight operation returned by the service.
* @sample AWSXRayAsync.GetInsight
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightAsync(GetInsightRequest getInsightRequest);
/**
*
* Retrieves the summary information of an insight. This includes impact to clients and root cause services, the top
* anomalous services, the category, the state of the insight, and the start and end time of the insight.
*
*
* @param getInsightRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInsight operation returned by the service.
* @sample AWSXRayAsyncHandler.GetInsight
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightAsync(GetInsightRequest getInsightRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* X-Ray reevaluates insights periodically until they're resolved, and records each intermediate state as an event.
* You can review an insight's events in the Impact Timeline on the Inspect page in the X-Ray console.
*
*
* @param getInsightEventsRequest
* @return A Java Future containing the result of the GetInsightEvents operation returned by the service.
* @sample AWSXRayAsync.GetInsightEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightEventsAsync(GetInsightEventsRequest getInsightEventsRequest);
/**
*
* X-Ray reevaluates insights periodically until they're resolved, and records each intermediate state as an event.
* You can review an insight's events in the Impact Timeline on the Inspect page in the X-Ray console.
*
*
* @param getInsightEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInsightEvents operation returned by the service.
* @sample AWSXRayAsyncHandler.GetInsightEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightEventsAsync(GetInsightEventsRequest getInsightEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a service graph structure filtered by the specified insight. The service graph is limited to only
* structural information. For a complete service graph, use this API with the GetServiceGraph API.
*
*
* @param getInsightImpactGraphRequest
* @return A Java Future containing the result of the GetInsightImpactGraph operation returned by the service.
* @sample AWSXRayAsync.GetInsightImpactGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightImpactGraphAsync(GetInsightImpactGraphRequest getInsightImpactGraphRequest);
/**
*
* Retrieves a service graph structure filtered by the specified insight. The service graph is limited to only
* structural information. For a complete service graph, use this API with the GetServiceGraph API.
*
*
* @param getInsightImpactGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInsightImpactGraph operation returned by the service.
* @sample AWSXRayAsyncHandler.GetInsightImpactGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightImpactGraphAsync(GetInsightImpactGraphRequest getInsightImpactGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the summaries of all insights in the specified group matching the provided filter values.
*
*
* @param getInsightSummariesRequest
* @return A Java Future containing the result of the GetInsightSummaries operation returned by the service.
* @sample AWSXRayAsync.GetInsightSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightSummariesAsync(GetInsightSummariesRequest getInsightSummariesRequest);
/**
*
* Retrieves the summaries of all insights in the specified group matching the provided filter values.
*
*
* @param getInsightSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInsightSummaries operation returned by the service.
* @sample AWSXRayAsyncHandler.GetInsightSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInsightSummariesAsync(GetInsightSummariesRequest getInsightSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves all sampling rules.
*
*
* @param getSamplingRulesRequest
* @return A Java Future containing the result of the GetSamplingRules operation returned by the service.
* @sample AWSXRayAsync.GetSamplingRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSamplingRulesAsync(GetSamplingRulesRequest getSamplingRulesRequest);
/**
*
* Retrieves all sampling rules.
*
*
* @param getSamplingRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSamplingRules operation returned by the service.
* @sample AWSXRayAsyncHandler.GetSamplingRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSamplingRulesAsync(GetSamplingRulesRequest getSamplingRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about recent sampling results for all sampling rules.
*
*
* @param getSamplingStatisticSummariesRequest
* @return A Java Future containing the result of the GetSamplingStatisticSummaries operation returned by the
* service.
* @sample AWSXRayAsync.GetSamplingStatisticSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future getSamplingStatisticSummariesAsync(
GetSamplingStatisticSummariesRequest getSamplingStatisticSummariesRequest);
/**
*
* Retrieves information about recent sampling results for all sampling rules.
*
*
* @param getSamplingStatisticSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSamplingStatisticSummaries operation returned by the
* service.
* @sample AWSXRayAsyncHandler.GetSamplingStatisticSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future getSamplingStatisticSummariesAsync(
GetSamplingStatisticSummariesRequest getSamplingStatisticSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Requests a sampling quota for rules that the service is using to sample requests.
*
*
* @param getSamplingTargetsRequest
* @return A Java Future containing the result of the GetSamplingTargets operation returned by the service.
* @sample AWSXRayAsync.GetSamplingTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSamplingTargetsAsync(GetSamplingTargetsRequest getSamplingTargetsRequest);
/**
*
* Requests a sampling quota for rules that the service is using to sample requests.
*
*
* @param getSamplingTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSamplingTargets operation returned by the service.
* @sample AWSXRayAsyncHandler.GetSamplingTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSamplingTargetsAsync(GetSamplingTargetsRequest getSamplingTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a document that describes services that process incoming requests, and downstream services that they
* call as a result. Root services process incoming requests and make calls to downstream services. Root services
* are applications that use the Amazon Web Services X-Ray
* SDK. Downstream services can be other applications, Amazon Web Services resources, HTTP web APIs, or SQL
* databases.
*
*
* @param getServiceGraphRequest
* @return A Java Future containing the result of the GetServiceGraph operation returned by the service.
* @sample AWSXRayAsync.GetServiceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getServiceGraphAsync(GetServiceGraphRequest getServiceGraphRequest);
/**
*
* Retrieves a document that describes services that process incoming requests, and downstream services that they
* call as a result. Root services process incoming requests and make calls to downstream services. Root services
* are applications that use the Amazon Web Services X-Ray
* SDK. Downstream services can be other applications, Amazon Web Services resources, HTTP web APIs, or SQL
* databases.
*
*
* @param getServiceGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServiceGraph operation returned by the service.
* @sample AWSXRayAsyncHandler.GetServiceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getServiceGraphAsync(GetServiceGraphRequest getServiceGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get an aggregation of service statistics defined by a specific time range.
*
*
* @param getTimeSeriesServiceStatisticsRequest
* @return A Java Future containing the result of the GetTimeSeriesServiceStatistics operation returned by the
* service.
* @sample AWSXRayAsync.GetTimeSeriesServiceStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future getTimeSeriesServiceStatisticsAsync(
GetTimeSeriesServiceStatisticsRequest getTimeSeriesServiceStatisticsRequest);
/**
*
* Get an aggregation of service statistics defined by a specific time range.
*
*
* @param getTimeSeriesServiceStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTimeSeriesServiceStatistics operation returned by the
* service.
* @sample AWSXRayAsyncHandler.GetTimeSeriesServiceStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future getTimeSeriesServiceStatisticsAsync(
GetTimeSeriesServiceStatisticsRequest getTimeSeriesServiceStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a service graph for one or more specific trace IDs.
*
*
* @param getTraceGraphRequest
* @return A Java Future containing the result of the GetTraceGraph operation returned by the service.
* @sample AWSXRayAsync.GetTraceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceGraphAsync(GetTraceGraphRequest getTraceGraphRequest);
/**
*
* Retrieves a service graph for one or more specific trace IDs.
*
*
* @param getTraceGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTraceGraph operation returned by the service.
* @sample AWSXRayAsyncHandler.GetTraceGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceGraphAsync(GetTraceGraphRequest getTraceGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves IDs and annotations for traces available for a specified time frame using an optional filter. To get
* the full traces, pass the trace IDs to BatchGetTraces
.
*
*
* A filter expression can target traced requests that hit specific service nodes or edges, have errors, or come
* from a known user. For example, the following filter expression targets traces that pass through
* api.example.com
:
*
*
* service("api.example.com")
*
*
* This filter expression finds traces that have an annotation named account
with the value
* 12345
:
*
*
* annotation.account = "12345"
*
*
* For a full list of indexed fields and keywords that you can use in filter expressions, see Using Filter Expressions in
* the Amazon Web Services X-Ray Developer Guide.
*
*
* @param getTraceSummariesRequest
* @return A Java Future containing the result of the GetTraceSummaries operation returned by the service.
* @sample AWSXRayAsync.GetTraceSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceSummariesAsync(GetTraceSummariesRequest getTraceSummariesRequest);
/**
*
* Retrieves IDs and annotations for traces available for a specified time frame using an optional filter. To get
* the full traces, pass the trace IDs to BatchGetTraces
.
*
*
* A filter expression can target traced requests that hit specific service nodes or edges, have errors, or come
* from a known user. For example, the following filter expression targets traces that pass through
* api.example.com
:
*
*
* service("api.example.com")
*
*
* This filter expression finds traces that have an annotation named account
with the value
* 12345
:
*
*
* annotation.account = "12345"
*
*
* For a full list of indexed fields and keywords that you can use in filter expressions, see Using Filter Expressions in
* the Amazon Web Services X-Ray Developer Guide.
*
*
* @param getTraceSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTraceSummaries operation returned by the service.
* @sample AWSXRayAsyncHandler.GetTraceSummaries
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTraceSummariesAsync(GetTraceSummariesRequest getTraceSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags that are applied to the specified Amazon Web Services X-Ray group or sampling rule.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSXRayAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of tags that are applied to the specified Amazon Web Services X-Ray group or sampling rule.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSXRayAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the encryption configuration for X-Ray data.
*
*
* @param putEncryptionConfigRequest
* @return A Java Future containing the result of the PutEncryptionConfig operation returned by the service.
* @sample AWSXRayAsync.PutEncryptionConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEncryptionConfigAsync(PutEncryptionConfigRequest putEncryptionConfigRequest);
/**
*
* Updates the encryption configuration for X-Ray data.
*
*
* @param putEncryptionConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEncryptionConfig operation returned by the service.
* @sample AWSXRayAsyncHandler.PutEncryptionConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEncryptionConfigAsync(PutEncryptionConfigRequest putEncryptionConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used by the Amazon Web Services X-Ray daemon to upload telemetry.
*
*
* @param putTelemetryRecordsRequest
* @return A Java Future containing the result of the PutTelemetryRecords operation returned by the service.
* @sample AWSXRayAsync.PutTelemetryRecords
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTelemetryRecordsAsync(PutTelemetryRecordsRequest putTelemetryRecordsRequest);
/**
*
* Used by the Amazon Web Services X-Ray daemon to upload telemetry.
*
*
* @param putTelemetryRecordsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTelemetryRecords operation returned by the service.
* @sample AWSXRayAsyncHandler.PutTelemetryRecords
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTelemetryRecordsAsync(PutTelemetryRecordsRequest putTelemetryRecordsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads segment documents to Amazon Web Services X-Ray. The X-Ray SDK generates segment documents and sends them to
* the X-Ray daemon, which uploads them in batches. A segment document can be a completed segment, an in-progress
* segment, or an array of subsegments.
*
*
* Segments must include the following fields. For the full segment document schema, see Amazon Web Services X-Ray
* Segment Documents in the Amazon Web Services X-Ray Developer Guide.
*
*
* Required segment document fields
*
*
* -
*
* name
- The name of the service that handled the request.
*
*
* -
*
* id
- A 64-bit identifier for the segment, unique among segments in the same trace, in 16 hexadecimal
* digits.
*
*
* -
*
* trace_id
- A unique identifier that connects all segments and subsegments originating from a single
* client request.
*
*
* -
*
* start_time
- Time the segment or subsegment was created, in floating point seconds in epoch time,
* accurate to milliseconds. For example, 1480615200.010
or 1.480615200010E9
.
*
*
* -
*
* end_time
- Time the segment or subsegment was closed. For example, 1480615200.090
or
* 1.480615200090E9
. Specify either an end_time
or in_progress
.
*
*
* -
*
* in_progress
- Set to true
instead of specifying an end_time
to record that
* a segment has been started, but is not complete. Send an in-progress segment when your application receives a
* request that will take a long time to serve, to trace that the request was received. When the response is sent,
* send the complete segment to overwrite the in-progress segment.
*
*
*
*
* A trace_id
consists of three numbers separated by hyphens. For example,
* 1-58406520-a006649127e371903a2de979. This includes:
*
*
* Trace ID Format
*
*
* -
*
* The version number, for instance, 1
.
*
*
* -
*
* The time of the original request, in Unix epoch time, in 8 hexadecimal digits. For example, 10:00AM December 2nd,
* 2016 PST in epoch time is 1480615200
seconds, or 58406520
in hexadecimal.
*
*
* -
*
* A 96-bit identifier for the trace, globally unique, in 24 hexadecimal digits.
*
*
*
*
* @param putTraceSegmentsRequest
* @return A Java Future containing the result of the PutTraceSegments operation returned by the service.
* @sample AWSXRayAsync.PutTraceSegments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTraceSegmentsAsync(PutTraceSegmentsRequest putTraceSegmentsRequest);
/**
*
* Uploads segment documents to Amazon Web Services X-Ray. The X-Ray SDK generates segment documents and sends them to
* the X-Ray daemon, which uploads them in batches. A segment document can be a completed segment, an in-progress
* segment, or an array of subsegments.
*
*
* Segments must include the following fields. For the full segment document schema, see Amazon Web Services X-Ray
* Segment Documents in the Amazon Web Services X-Ray Developer Guide.
*
*
* Required segment document fields
*
*
* -
*
* name
- The name of the service that handled the request.
*
*
* -
*
* id
- A 64-bit identifier for the segment, unique among segments in the same trace, in 16 hexadecimal
* digits.
*
*
* -
*
* trace_id
- A unique identifier that connects all segments and subsegments originating from a single
* client request.
*
*
* -
*
* start_time
- Time the segment or subsegment was created, in floating point seconds in epoch time,
* accurate to milliseconds. For example, 1480615200.010
or 1.480615200010E9
.
*
*
* -
*
* end_time
- Time the segment or subsegment was closed. For example, 1480615200.090
or
* 1.480615200090E9
. Specify either an end_time
or in_progress
.
*
*
* -
*
* in_progress
- Set to true
instead of specifying an end_time
to record that
* a segment has been started, but is not complete. Send an in-progress segment when your application receives a
* request that will take a long time to serve, to trace that the request was received. When the response is sent,
* send the complete segment to overwrite the in-progress segment.
*
*
*
*
* A trace_id
consists of three numbers separated by hyphens. For example,
* 1-58406520-a006649127e371903a2de979. This includes:
*
*
* Trace ID Format
*
*
* -
*
* The version number, for instance, 1
.
*
*
* -
*
* The time of the original request, in Unix epoch time, in 8 hexadecimal digits. For example, 10:00AM December 2nd,
* 2016 PST in epoch time is 1480615200
seconds, or 58406520
in hexadecimal.
*
*
* -
*
* A 96-bit identifier for the trace, globally unique, in 24 hexadecimal digits.
*
*
*
*
* @param putTraceSegmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTraceSegments operation returned by the service.
* @sample AWSXRayAsyncHandler.PutTraceSegments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTraceSegmentsAsync(PutTraceSegmentsRequest putTraceSegmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies tags to an existing Amazon Web Services X-Ray group or sampling rule.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSXRayAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Applies tags to an existing Amazon Web Services X-Ray group or sampling rule.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSXRayAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from an Amazon Web Services X-Ray group or sampling rule. You cannot edit or delete system tags
* (those with an aws:
prefix).
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSXRayAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from an Amazon Web Services X-Ray group or sampling rule. You cannot edit or delete system tags
* (those with an aws:
prefix).
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSXRayAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a group resource.
*
*
* @param updateGroupRequest
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AWSXRayAsync.UpdateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest);
/**
*
* Updates a group resource.
*
*
* @param updateGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AWSXRayAsyncHandler.UpdateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies a sampling rule's configuration.
*
*
* @param updateSamplingRuleRequest
* @return A Java Future containing the result of the UpdateSamplingRule operation returned by the service.
* @sample AWSXRayAsync.UpdateSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSamplingRuleAsync(UpdateSamplingRuleRequest updateSamplingRuleRequest);
/**
*
* Modifies a sampling rule's configuration.
*
*
* @param updateSamplingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSamplingRule operation returned by the service.
* @sample AWSXRayAsyncHandler.UpdateSamplingRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSamplingRuleAsync(UpdateSamplingRuleRequest updateSamplingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}