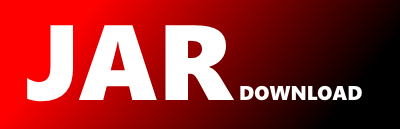
com.amazonaws.services.xray.model.TimeSeriesServiceStatistics Maven / Gradle / Ivy
Show all versions of aws-java-sdk-xray Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.xray.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A list of TimeSeriesStatistic structures.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TimeSeriesServiceStatistics implements Serializable, Cloneable, StructuredPojo {
/**
*
* Timestamp of the window for which statistics are aggregated.
*
*/
private java.util.Date timestamp;
private EdgeStatistics edgeSummaryStatistics;
private ServiceStatistics serviceSummaryStatistics;
/**
*
* The forecasted high and low fault count values.
*
*/
private ForecastStatistics serviceForecastStatistics;
/**
*
* The response time histogram for the selected entities.
*
*/
private java.util.List responseTimeHistogram;
/**
*
* Timestamp of the window for which statistics are aggregated.
*
*
* @param timestamp
* Timestamp of the window for which statistics are aggregated.
*/
public void setTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
}
/**
*
* Timestamp of the window for which statistics are aggregated.
*
*
* @return Timestamp of the window for which statistics are aggregated.
*/
public java.util.Date getTimestamp() {
return this.timestamp;
}
/**
*
* Timestamp of the window for which statistics are aggregated.
*
*
* @param timestamp
* Timestamp of the window for which statistics are aggregated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withTimestamp(java.util.Date timestamp) {
setTimestamp(timestamp);
return this;
}
/**
* @param edgeSummaryStatistics
*/
public void setEdgeSummaryStatistics(EdgeStatistics edgeSummaryStatistics) {
this.edgeSummaryStatistics = edgeSummaryStatistics;
}
/**
* @return
*/
public EdgeStatistics getEdgeSummaryStatistics() {
return this.edgeSummaryStatistics;
}
/**
* @param edgeSummaryStatistics
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withEdgeSummaryStatistics(EdgeStatistics edgeSummaryStatistics) {
setEdgeSummaryStatistics(edgeSummaryStatistics);
return this;
}
/**
* @param serviceSummaryStatistics
*/
public void setServiceSummaryStatistics(ServiceStatistics serviceSummaryStatistics) {
this.serviceSummaryStatistics = serviceSummaryStatistics;
}
/**
* @return
*/
public ServiceStatistics getServiceSummaryStatistics() {
return this.serviceSummaryStatistics;
}
/**
* @param serviceSummaryStatistics
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withServiceSummaryStatistics(ServiceStatistics serviceSummaryStatistics) {
setServiceSummaryStatistics(serviceSummaryStatistics);
return this;
}
/**
*
* The forecasted high and low fault count values.
*
*
* @param serviceForecastStatistics
* The forecasted high and low fault count values.
*/
public void setServiceForecastStatistics(ForecastStatistics serviceForecastStatistics) {
this.serviceForecastStatistics = serviceForecastStatistics;
}
/**
*
* The forecasted high and low fault count values.
*
*
* @return The forecasted high and low fault count values.
*/
public ForecastStatistics getServiceForecastStatistics() {
return this.serviceForecastStatistics;
}
/**
*
* The forecasted high and low fault count values.
*
*
* @param serviceForecastStatistics
* The forecasted high and low fault count values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withServiceForecastStatistics(ForecastStatistics serviceForecastStatistics) {
setServiceForecastStatistics(serviceForecastStatistics);
return this;
}
/**
*
* The response time histogram for the selected entities.
*
*
* @return The response time histogram for the selected entities.
*/
public java.util.List getResponseTimeHistogram() {
return responseTimeHistogram;
}
/**
*
* The response time histogram for the selected entities.
*
*
* @param responseTimeHistogram
* The response time histogram for the selected entities.
*/
public void setResponseTimeHistogram(java.util.Collection responseTimeHistogram) {
if (responseTimeHistogram == null) {
this.responseTimeHistogram = null;
return;
}
this.responseTimeHistogram = new java.util.ArrayList(responseTimeHistogram);
}
/**
*
* The response time histogram for the selected entities.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResponseTimeHistogram(java.util.Collection)} or
* {@link #withResponseTimeHistogram(java.util.Collection)} if you want to override the existing values.
*
*
* @param responseTimeHistogram
* The response time histogram for the selected entities.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withResponseTimeHistogram(HistogramEntry... responseTimeHistogram) {
if (this.responseTimeHistogram == null) {
setResponseTimeHistogram(new java.util.ArrayList(responseTimeHistogram.length));
}
for (HistogramEntry ele : responseTimeHistogram) {
this.responseTimeHistogram.add(ele);
}
return this;
}
/**
*
* The response time histogram for the selected entities.
*
*
* @param responseTimeHistogram
* The response time histogram for the selected entities.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeSeriesServiceStatistics withResponseTimeHistogram(java.util.Collection responseTimeHistogram) {
setResponseTimeHistogram(responseTimeHistogram);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTimestamp() != null)
sb.append("Timestamp: ").append(getTimestamp()).append(",");
if (getEdgeSummaryStatistics() != null)
sb.append("EdgeSummaryStatistics: ").append(getEdgeSummaryStatistics()).append(",");
if (getServiceSummaryStatistics() != null)
sb.append("ServiceSummaryStatistics: ").append(getServiceSummaryStatistics()).append(",");
if (getServiceForecastStatistics() != null)
sb.append("ServiceForecastStatistics: ").append(getServiceForecastStatistics()).append(",");
if (getResponseTimeHistogram() != null)
sb.append("ResponseTimeHistogram: ").append(getResponseTimeHistogram());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TimeSeriesServiceStatistics == false)
return false;
TimeSeriesServiceStatistics other = (TimeSeriesServiceStatistics) obj;
if (other.getTimestamp() == null ^ this.getTimestamp() == null)
return false;
if (other.getTimestamp() != null && other.getTimestamp().equals(this.getTimestamp()) == false)
return false;
if (other.getEdgeSummaryStatistics() == null ^ this.getEdgeSummaryStatistics() == null)
return false;
if (other.getEdgeSummaryStatistics() != null && other.getEdgeSummaryStatistics().equals(this.getEdgeSummaryStatistics()) == false)
return false;
if (other.getServiceSummaryStatistics() == null ^ this.getServiceSummaryStatistics() == null)
return false;
if (other.getServiceSummaryStatistics() != null && other.getServiceSummaryStatistics().equals(this.getServiceSummaryStatistics()) == false)
return false;
if (other.getServiceForecastStatistics() == null ^ this.getServiceForecastStatistics() == null)
return false;
if (other.getServiceForecastStatistics() != null && other.getServiceForecastStatistics().equals(this.getServiceForecastStatistics()) == false)
return false;
if (other.getResponseTimeHistogram() == null ^ this.getResponseTimeHistogram() == null)
return false;
if (other.getResponseTimeHistogram() != null && other.getResponseTimeHistogram().equals(this.getResponseTimeHistogram()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTimestamp() == null) ? 0 : getTimestamp().hashCode());
hashCode = prime * hashCode + ((getEdgeSummaryStatistics() == null) ? 0 : getEdgeSummaryStatistics().hashCode());
hashCode = prime * hashCode + ((getServiceSummaryStatistics() == null) ? 0 : getServiceSummaryStatistics().hashCode());
hashCode = prime * hashCode + ((getServiceForecastStatistics() == null) ? 0 : getServiceForecastStatistics().hashCode());
hashCode = prime * hashCode + ((getResponseTimeHistogram() == null) ? 0 : getResponseTimeHistogram().hashCode());
return hashCode;
}
@Override
public TimeSeriesServiceStatistics clone() {
try {
return (TimeSeriesServiceStatistics) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.xray.model.transform.TimeSeriesServiceStatisticsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}