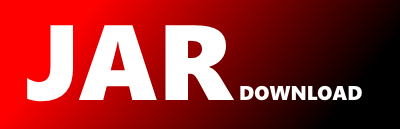
com.amazonaws.services.lambda.runtime.events.APIGatewayProxyResponseEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-lambda-java-events Show documentation
Show all versions of aws-lambda-java-events Show documentation
Event interface definitions AWS services supported by AWS Lambda.
package com.amazonaws.services.lambda.runtime.events;
import java.io.Serializable;
import java.util.Map;
/**
* Class that represents an APIGatewayProxyResponseEvent object
*/
public class APIGatewayProxyResponseEvent implements Serializable, Cloneable {
private static final long serialVersionUID = 2263167344670024172L;
private Integer statusCode;
private Map headers;
private String body;
/**
* default constructor
*/
public APIGatewayProxyResponseEvent() {}
/**
* @return The HTTP status code for the request
*/
public Integer getStatusCode() {
return statusCode;
}
/**
* @param statusCode The HTTP status code for the request
*/
public void setStatusCode(Integer statusCode) {
this.statusCode = statusCode;
}
/**
* @param statusCode The HTTP status code for the request
* @return APIGatewayProxyResponseEvent object
*/
public APIGatewayProxyResponseEvent withStatusCode(Integer statusCode) {
this.setStatusCode(statusCode);
return this;
}
/**
* @return The Http headers return in the response
*/
public Map getHeaders() {
return headers;
}
/**
* @param headers The Http headers return in the response
*/
public void setHeaders(Map headers) {
this.headers = headers;
}
/**
* @param headers The Http headers return in the response
* @return APIGatewayProxyResponseEvent
*/
public APIGatewayProxyResponseEvent withHeaders(Map headers) {
this.setHeaders(headers);
return this;
}
/**
* @return The response body
*/
public String getBody() {
return body;
}
/**
* @param body The response body
*/
public void setBody(String body) {
this.body = body;
}
/**
* @param body The response body
* @return APIGatewayProxyResponseEvent object
*/
public APIGatewayProxyResponseEvent withBody(String body) {
this.setBody(body);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStatusCode() != null)
sb.append("statusCode: ").append(getStatusCode()).append(",");
if (getHeaders() != null)
sb.append("headers: ").append(getHeaders().toString()).append(",");
if (getBody() != null)
sb.append("body: ").append(getBody());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof APIGatewayProxyResponseEvent == false)
return false;
APIGatewayProxyResponseEvent other = (APIGatewayProxyResponseEvent) obj;
if (other.getStatusCode() == null ^ this.getStatusCode() == null)
return false;
if (other.getStatusCode() != null && other.getStatusCode().equals(this.getStatusCode()) == false)
return false;
if (other.getHeaders() == null ^ this.getHeaders() == null)
return false;
if (other.getHeaders() != null && other.getHeaders().equals(this.getHeaders()) == false)
return false;
if (other.getBody() == null ^ this.getBody() == null)
return false;
if (other.getBody() != null && other.getBody().equals(this.getBody()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStatusCode() == null) ? 0 : getStatusCode().hashCode());
hashCode = prime * hashCode + ((getHeaders() == null) ? 0 : getHeaders().hashCode());
hashCode = prime * hashCode + ((getBody() == null) ? 0 : getBody().hashCode());
return hashCode;
}
@Override
public APIGatewayProxyResponseEvent clone() {
try {
return (APIGatewayProxyResponseEvent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone()", e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy