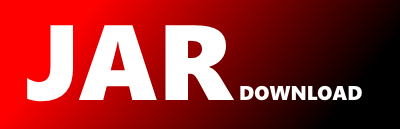
com.amazonaws.services.dynamodbv2.streamsadapter.AdapterRequestCache Maven / Gradle / Ivy
/*
* Copyright 2014-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Amazon Software License (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/asl/
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.streamsadapter;
import java.util.Deque;
import java.util.HashMap;
import java.util.LinkedList;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Cache for mapping Kinesis requests to DynamoDB Streams requests. Evicts oldest cache entry if adding a new entry exceeds the cache capacity.
*/
public class AdapterRequestCache {
/**
* Map for request lookup.
*/
private final HashMap cacheMap = new HashMap();
/**
* Deque for evicting old cache entries.
*/
private final Deque evictQueue = new LinkedList();
/**
* Capacity for the cache.
*/
private final int capacity;
public AdapterRequestCache(int capacity) {
if (capacity <= 0) {
throw new IllegalArgumentException("Capacity must be a positive number");
}
this.capacity = capacity;
}
/**
* Adds an entry to the cache.
*
* @param request
* Kinesis request
* @param requestAdapter
* DynamoDB adapter client wrapper for the Kinesis request
*/
public synchronized void addEntry(AmazonWebServiceRequest request, AmazonWebServiceRequest requestAdapter) {
if (null == request || null == requestAdapter) {
throw new IllegalArgumentException("Request and adapter request must not be null");
}
if (evictQueue.size() == capacity) {
Integer evicted = evictQueue.removeLast();
cacheMap.remove(evicted);
}
evictQueue.addFirst(System.identityHashCode(request));
cacheMap.put(System.identityHashCode(request), requestAdapter);
}
/**
* Gets the actual DynamoDB Streams request made for a Kinesis request.
*
* @param request
* Kinesis request
* @return actual DynamoDB Streams request made for the associated Kinesis request
*/
public synchronized AmazonWebServiceRequest getEntry(AmazonWebServiceRequest request) {
if (null == request) {
throw new IllegalArgumentException("Request must not be null");
}
return cacheMap.get(System.identityHashCode(request));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy