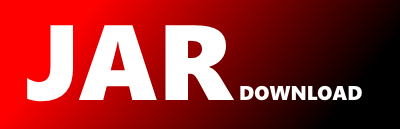
net.spy.memcached.protocol.binary.BinaryOperationFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticache-java-cluster-client Show documentation
Show all versions of elasticache-java-cluster-client Show documentation
Amazon ElastiCache Cluster Client is an enhanced Java library to connect to ElastiCache clusters. This client library has been built upon Spymemcached and is released under the Amazon Software License.
/**
* Copyright (C) 2006-2009 Dustin Sallings
* Copyright (C) 2009-2011 Couchbase, Inc.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALING
* IN THE SOFTWARE.
*
*
* Portions Copyright (C) 2012-2012 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Amazon Software License (the "License"). You may not use this
* file except in compliance with the License. A copy of the License is located at
* http://aws.amazon.com/asl/
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, express or
* implied. See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.spy.memcached.protocol.binary;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Map;
import javax.security.auth.callback.CallbackHandler;
import net.spy.memcached.ops.BaseOperationFactory;
import net.spy.memcached.ops.CASOperation;
import net.spy.memcached.ops.ConcatenationOperation;
import net.spy.memcached.ops.ConcatenationType;
import net.spy.memcached.ops.ConfigurationType;
import net.spy.memcached.ops.DeleteOperation;
import net.spy.memcached.ops.FlushOperation;
import net.spy.memcached.ops.GetAndTouchOperation;
import net.spy.memcached.ops.GetConfigOperation;
import net.spy.memcached.ops.GetOperation;
import net.spy.memcached.ops.GetOperation.Callback;
import net.spy.memcached.ops.DeleteConfigOperation;
import net.spy.memcached.ops.GetlOperation;
import net.spy.memcached.ops.GetsOperation;
import net.spy.memcached.ops.KeyedOperation;
import net.spy.memcached.ops.MultiGetOperationCallback;
import net.spy.memcached.ops.MultiGetsOperationCallback;
import net.spy.memcached.ops.Mutator;
import net.spy.memcached.ops.MutatorOperation;
import net.spy.memcached.ops.NoopOperation;
import net.spy.memcached.ops.Operation;
import net.spy.memcached.ops.OperationCallback;
import net.spy.memcached.ops.SASLAuthOperation;
import net.spy.memcached.ops.SASLMechsOperation;
import net.spy.memcached.ops.SASLStepOperation;
import net.spy.memcached.ops.SetConfigOperation;
import net.spy.memcached.ops.StatsOperation;
import net.spy.memcached.ops.StoreOperation;
import net.spy.memcached.ops.StoreType;
import net.spy.memcached.ops.TapOperation;
import net.spy.memcached.ops.UnlockOperation;
import net.spy.memcached.ops.VersionOperation;
import net.spy.memcached.tapmessage.RequestMessage;
import net.spy.memcached.tapmessage.TapOpcode;
/**
* Factory for binary operations.
*/
public class BinaryOperationFactory extends BaseOperationFactory {
public DeleteOperation
delete(String key, OperationCallback operationCallback) {
return new DeleteOperationImpl(key, operationCallback);
}
public UnlockOperation unlock(String key, long casId,
OperationCallback cb) {
return new UnlockOperationImpl(key, casId, cb);
}
public FlushOperation flush(int delay, OperationCallback cb) {
return new FlushOperationImpl(cb);
}
public GetAndTouchOperation getAndTouch(String key, int expiration,
GetAndTouchOperation.Callback cb) {
return new GetAndTouchOperationImpl(key, expiration, cb);
}
public GetOperation get(String key, Callback callback) {
return new GetOperationImpl(key, callback);
}
public GetOperation get(Collection value, Callback cb) {
return new MultiGetOperationImpl(value, cb);
}
public GetlOperation getl(String key, int exp, GetlOperation.Callback cb) {
return new GetlOperationImpl(key, exp, cb);
}
public GetsOperation gets(String key, GetsOperation.Callback cb) {
return new GetsOperationImpl(key, cb);
}
public GetConfigOperation getConfig(ConfigurationType type, GetConfigOperation.Callback callback) {
return new GetConfigOperationImpl(type, callback);
}
public SetConfigOperation setConfig(ConfigurationType type, int flags, byte[] data, OperationCallback cb){
return new SetConfigOperationImpl(type, flags, data, cb);
}
public DeleteConfigOperation deleteConfig(ConfigurationType type, OperationCallback cb) {
return new DeleteConfigOperationImpl(type, cb);
}
public MutatorOperation mutate(Mutator m, String key, long by, long def,
int exp, OperationCallback cb) {
return new MutatorOperationImpl(m, key, by, def, exp, cb);
}
public StatsOperation stats(String arg,
net.spy.memcached.ops.StatsOperation.Callback cb) {
return new StatsOperationImpl(arg, cb);
}
public StoreOperation store(StoreType storeType, String key, int flags,
int exp, byte[] data, OperationCallback cb) {
return new StoreOperationImpl(storeType, key, flags, exp, data, 0, cb);
}
public KeyedOperation touch(String key, int expiration,
OperationCallback cb) {
return new TouchOperationImpl(key, expiration, cb);
}
public VersionOperation version(OperationCallback cb) {
return new VersionOperationImpl(cb);
}
public NoopOperation noop(OperationCallback cb) {
return new NoopOperationImpl(cb);
}
public CASOperation cas(StoreType type, String key, long casId, int flags,
int exp, byte[] data, OperationCallback cb) {
return new StoreOperationImpl(type, key, flags, exp, data, casId, cb);
}
public ConcatenationOperation cat(ConcatenationType catType, long casId,
String key, byte[] data, OperationCallback cb) {
return new ConcatenationOperationImpl(catType, key, data, casId, cb);
}
@Override
protected Collection extends Operation> cloneGet(KeyedOperation op) {
Collection rv = new ArrayList();
GetOperation.Callback getCb = null;
GetsOperation.Callback getsCb = null;
if (op.getCallback() instanceof GetOperation.Callback) {
getCb =
new MultiGetOperationCallback(op.getCallback(), op.getKeys().size());
} else {
getsCb =
new MultiGetsOperationCallback(op.getCallback(), op.getKeys().size());
}
for (String k : op.getKeys()) {
rv.add(getCb == null ? gets(k, getsCb) : get(k, getCb));
}
return rv;
}
public SASLAuthOperation saslAuth(String[] mech, String serverName,
Map props, CallbackHandler cbh, OperationCallback cb) {
return new SASLAuthOperationImpl(mech, serverName, props, cbh, cb);
}
public SASLMechsOperation saslMechs(OperationCallback cb) {
return new SASLMechsOperationImpl(cb);
}
public SASLStepOperation saslStep(String[] mech, byte[] challenge,
String serverName, Map props, CallbackHandler cbh,
OperationCallback cb) {
return new SASLStepOperationImpl(mech, challenge, serverName, props, cbh,
cb);
}
public TapOperation tapBackfill(String id, long date, OperationCallback cb) {
return new TapBackfillOperationImpl(id, date, cb);
}
public TapOperation tapCustom(String id, RequestMessage message,
OperationCallback cb) {
return new TapCustomOperationImpl(id, message, cb);
}
public TapOperation
tapAck(TapOpcode opcode, int opaque, OperationCallback cb) {
return new TapAckOperationImpl(opcode, opaque, cb);
}
public TapOperation tapDump(String id, OperationCallback cb) {
return new TapDumpOperationImpl(id, cb);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy