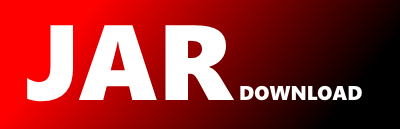
com.anaptecs.jeaf.openapi.PrimitiveArraysObjectWithRestrictions Maven / Gradle / Ivy
/*
* Product Base Definitions
* This component represents the Open API interface of the accounting service.
*
* OpenAPI spec version: 0.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.anaptecs.jeaf.openapi;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
/**
* PrimitiveArraysObjectWithRestrictions
*/
public class PrimitiveArraysObjectWithRestrictions {
@JsonProperty("aBooleanArray")
private List aBooleanArray = null;
@JsonProperty("bBooleanArray")
private List bBooleanArray = null;
@JsonProperty("cBooleanArray")
private List cBooleanArray = null;
@JsonProperty("aByteArray")
private byte[] aByteArray = null;
@JsonProperty("bByteArray")
private byte[] bByteArray = null;
@JsonProperty("aShortArray")
private List aShortArray = null;
@JsonProperty("bShortArray")
private List bShortArray = null;
@JsonProperty("aIntegerArray")
private List aIntegerArray = null;
@JsonProperty("bIntegerArray")
private List bIntegerArray = null;
@JsonProperty("cIntegerArray")
private List cIntegerArray = null;
@JsonProperty("aLongArray")
private List aLongArray = new ArrayList<>();
@JsonProperty("bLongArray")
private List bLongArray = null;
@JsonProperty("aBigIntegerArray")
private List aBigIntegerArray = null;
@JsonProperty("aCharacterArray")
private List aCharacterArray = null;
@JsonProperty("bCharacterArray")
private List bCharacterArray = null;
@JsonProperty("aFloatArray")
private List aFloatArray = null;
@JsonProperty("bFloatArray")
private List bFloatArray = null;
@JsonProperty("aDoubleArray")
private List aDoubleArray = null;
@JsonProperty("bDoubleArray")
private List bDoubleArray = null;
@JsonProperty("aBigDecimalArray")
private List aBigDecimalArray = null;
@JsonProperty("aStringArray")
private List aStringArray = null;
@JsonProperty("bStringArray")
private List bStringArray = null;
public PrimitiveArraysObjectWithRestrictions aBooleanArray(List aBooleanArray) {
this.aBooleanArray = aBooleanArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addABooleanArrayItem(Boolean aBooleanArrayItem) {
if (this.aBooleanArray == null) {
this.aBooleanArray = new ArrayList<>();
}
this.aBooleanArray.add(aBooleanArrayItem);
return this;
}
/**
* Get aBooleanArray
* @return aBooleanArray
**/
@Schema(description = "")
public List getABooleanArray() {
return aBooleanArray;
}
public void setABooleanArray(List aBooleanArray) {
this.aBooleanArray = aBooleanArray;
}
public PrimitiveArraysObjectWithRestrictions bBooleanArray(List bBooleanArray) {
this.bBooleanArray = bBooleanArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBBooleanArrayItem(Boolean bBooleanArrayItem) {
if (this.bBooleanArray == null) {
this.bBooleanArray = new ArrayList<>();
}
this.bBooleanArray.add(bBooleanArrayItem);
return this;
}
/**
* Get bBooleanArray
* @return bBooleanArray
**/
@Schema(description = "")
public List getBBooleanArray() {
return bBooleanArray;
}
public void setBBooleanArray(List bBooleanArray) {
this.bBooleanArray = bBooleanArray;
}
public PrimitiveArraysObjectWithRestrictions cBooleanArray(List cBooleanArray) {
this.cBooleanArray = cBooleanArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addCBooleanArrayItem(Boolean cBooleanArrayItem) {
if (this.cBooleanArray == null) {
this.cBooleanArray = new ArrayList<>();
}
this.cBooleanArray.add(cBooleanArrayItem);
return this;
}
/**
* Get cBooleanArray
* @return cBooleanArray
**/
@Schema(description = "")
public List getCBooleanArray() {
return cBooleanArray;
}
public void setCBooleanArray(List cBooleanArray) {
this.cBooleanArray = cBooleanArray;
}
public PrimitiveArraysObjectWithRestrictions aByteArray(byte[] aByteArray) {
this.aByteArray = aByteArray;
return this;
}
/**
* Get aByteArray
* @return aByteArray
**/
@Schema(description = "")
public byte[] getAByteArray() {
return aByteArray;
}
public void setAByteArray(byte[] aByteArray) {
this.aByteArray = aByteArray;
}
public PrimitiveArraysObjectWithRestrictions bByteArray(byte[] bByteArray) {
this.bByteArray = bByteArray;
return this;
}
/**
* Get bByteArray
* @return bByteArray
**/
@Schema(description = "")
public byte[] getBByteArray() {
return bByteArray;
}
public void setBByteArray(byte[] bByteArray) {
this.bByteArray = bByteArray;
}
public PrimitiveArraysObjectWithRestrictions aShortArray(List aShortArray) {
this.aShortArray = aShortArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addAShortArrayItem(Integer aShortArrayItem) {
if (this.aShortArray == null) {
this.aShortArray = new ArrayList<>();
}
this.aShortArray.add(aShortArrayItem);
return this;
}
/**
* Get aShortArray
* @return aShortArray
**/
@Schema(description = "")
public List getAShortArray() {
return aShortArray;
}
public void setAShortArray(List aShortArray) {
this.aShortArray = aShortArray;
}
public PrimitiveArraysObjectWithRestrictions bShortArray(List bShortArray) {
this.bShortArray = bShortArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBShortArrayItem(Integer bShortArrayItem) {
if (this.bShortArray == null) {
this.bShortArray = new ArrayList<>();
}
this.bShortArray.add(bShortArrayItem);
return this;
}
/**
* Get bShortArray
* @return bShortArray
**/
@Schema(description = "")
public List getBShortArray() {
return bShortArray;
}
public void setBShortArray(List bShortArray) {
this.bShortArray = bShortArray;
}
public PrimitiveArraysObjectWithRestrictions aIntegerArray(List aIntegerArray) {
this.aIntegerArray = aIntegerArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addAIntegerArrayItem(Integer aIntegerArrayItem) {
if (this.aIntegerArray == null) {
this.aIntegerArray = new ArrayList<>();
}
this.aIntegerArray.add(aIntegerArrayItem);
return this;
}
/**
* Get aIntegerArray
* @return aIntegerArray
**/
@Schema(description = "")
public List getAIntegerArray() {
return aIntegerArray;
}
public void setAIntegerArray(List aIntegerArray) {
this.aIntegerArray = aIntegerArray;
}
public PrimitiveArraysObjectWithRestrictions bIntegerArray(List bIntegerArray) {
this.bIntegerArray = bIntegerArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBIntegerArrayItem(Integer bIntegerArrayItem) {
if (this.bIntegerArray == null) {
this.bIntegerArray = new ArrayList<>();
}
this.bIntegerArray.add(bIntegerArrayItem);
return this;
}
/**
* Get bIntegerArray
* @return bIntegerArray
**/
@Schema(description = "")
public List getBIntegerArray() {
return bIntegerArray;
}
public void setBIntegerArray(List bIntegerArray) {
this.bIntegerArray = bIntegerArray;
}
public PrimitiveArraysObjectWithRestrictions cIntegerArray(List cIntegerArray) {
this.cIntegerArray = cIntegerArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addCIntegerArrayItem(Integer cIntegerArrayItem) {
if (this.cIntegerArray == null) {
this.cIntegerArray = new ArrayList<>();
}
this.cIntegerArray.add(cIntegerArrayItem);
return this;
}
/**
* Get cIntegerArray
* @return cIntegerArray
**/
@Schema(description = "")
public List getCIntegerArray() {
return cIntegerArray;
}
public void setCIntegerArray(List cIntegerArray) {
this.cIntegerArray = cIntegerArray;
}
public PrimitiveArraysObjectWithRestrictions aLongArray(List aLongArray) {
this.aLongArray = aLongArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addALongArrayItem(Long aLongArrayItem) {
this.aLongArray.add(aLongArrayItem);
return this;
}
/**
* Get aLongArray
* @return aLongArray
**/
@Schema(required = true, description = "")
public List getALongArray() {
return aLongArray;
}
public void setALongArray(List aLongArray) {
this.aLongArray = aLongArray;
}
public PrimitiveArraysObjectWithRestrictions bLongArray(List bLongArray) {
this.bLongArray = bLongArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBLongArrayItem(Long bLongArrayItem) {
if (this.bLongArray == null) {
this.bLongArray = new ArrayList<>();
}
this.bLongArray.add(bLongArrayItem);
return this;
}
/**
* Get bLongArray
* @return bLongArray
**/
@Schema(description = "")
public List getBLongArray() {
return bLongArray;
}
public void setBLongArray(List bLongArray) {
this.bLongArray = bLongArray;
}
public PrimitiveArraysObjectWithRestrictions aBigIntegerArray(List aBigIntegerArray) {
this.aBigIntegerArray = aBigIntegerArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addABigIntegerArrayItem(Long aBigIntegerArrayItem) {
if (this.aBigIntegerArray == null) {
this.aBigIntegerArray = new ArrayList<>();
}
this.aBigIntegerArray.add(aBigIntegerArrayItem);
return this;
}
/**
* Get aBigIntegerArray
* @return aBigIntegerArray
**/
@Schema(description = "")
public List getABigIntegerArray() {
return aBigIntegerArray;
}
public void setABigIntegerArray(List aBigIntegerArray) {
this.aBigIntegerArray = aBigIntegerArray;
}
public PrimitiveArraysObjectWithRestrictions aCharacterArray(List aCharacterArray) {
this.aCharacterArray = aCharacterArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addACharacterArrayItem(String aCharacterArrayItem) {
if (this.aCharacterArray == null) {
this.aCharacterArray = new ArrayList<>();
}
this.aCharacterArray.add(aCharacterArrayItem);
return this;
}
/**
* Get aCharacterArray
* @return aCharacterArray
**/
@Schema(description = "")
public List getACharacterArray() {
return aCharacterArray;
}
public void setACharacterArray(List aCharacterArray) {
this.aCharacterArray = aCharacterArray;
}
public PrimitiveArraysObjectWithRestrictions bCharacterArray(List bCharacterArray) {
this.bCharacterArray = bCharacterArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBCharacterArrayItem(String bCharacterArrayItem) {
if (this.bCharacterArray == null) {
this.bCharacterArray = new ArrayList<>();
}
this.bCharacterArray.add(bCharacterArrayItem);
return this;
}
/**
* Get bCharacterArray
* @return bCharacterArray
**/
@Schema(description = "")
public List getBCharacterArray() {
return bCharacterArray;
}
public void setBCharacterArray(List bCharacterArray) {
this.bCharacterArray = bCharacterArray;
}
public PrimitiveArraysObjectWithRestrictions aFloatArray(List aFloatArray) {
this.aFloatArray = aFloatArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addAFloatArrayItem(Float aFloatArrayItem) {
if (this.aFloatArray == null) {
this.aFloatArray = new ArrayList<>();
}
this.aFloatArray.add(aFloatArrayItem);
return this;
}
/**
* Get aFloatArray
* @return aFloatArray
**/
@Schema(description = "")
public List getAFloatArray() {
return aFloatArray;
}
public void setAFloatArray(List aFloatArray) {
this.aFloatArray = aFloatArray;
}
public PrimitiveArraysObjectWithRestrictions bFloatArray(List bFloatArray) {
this.bFloatArray = bFloatArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBFloatArrayItem(Float bFloatArrayItem) {
if (this.bFloatArray == null) {
this.bFloatArray = new ArrayList<>();
}
this.bFloatArray.add(bFloatArrayItem);
return this;
}
/**
* Get bFloatArray
* @return bFloatArray
**/
@Schema(description = "")
public List getBFloatArray() {
return bFloatArray;
}
public void setBFloatArray(List bFloatArray) {
this.bFloatArray = bFloatArray;
}
public PrimitiveArraysObjectWithRestrictions aDoubleArray(List aDoubleArray) {
this.aDoubleArray = aDoubleArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addADoubleArrayItem(Double aDoubleArrayItem) {
if (this.aDoubleArray == null) {
this.aDoubleArray = new ArrayList<>();
}
this.aDoubleArray.add(aDoubleArrayItem);
return this;
}
/**
* Get aDoubleArray
* @return aDoubleArray
**/
@Schema(description = "")
public List getADoubleArray() {
return aDoubleArray;
}
public void setADoubleArray(List aDoubleArray) {
this.aDoubleArray = aDoubleArray;
}
public PrimitiveArraysObjectWithRestrictions bDoubleArray(List bDoubleArray) {
this.bDoubleArray = bDoubleArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBDoubleArrayItem(Double bDoubleArrayItem) {
if (this.bDoubleArray == null) {
this.bDoubleArray = new ArrayList<>();
}
this.bDoubleArray.add(bDoubleArrayItem);
return this;
}
/**
* Get bDoubleArray
* @return bDoubleArray
**/
@Schema(description = "")
public List getBDoubleArray() {
return bDoubleArray;
}
public void setBDoubleArray(List bDoubleArray) {
this.bDoubleArray = bDoubleArray;
}
public PrimitiveArraysObjectWithRestrictions aBigDecimalArray(List aBigDecimalArray) {
this.aBigDecimalArray = aBigDecimalArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addABigDecimalArrayItem(Double aBigDecimalArrayItem) {
if (this.aBigDecimalArray == null) {
this.aBigDecimalArray = new ArrayList<>();
}
this.aBigDecimalArray.add(aBigDecimalArrayItem);
return this;
}
/**
* Get aBigDecimalArray
* @return aBigDecimalArray
**/
@Schema(description = "")
public List getABigDecimalArray() {
return aBigDecimalArray;
}
public void setABigDecimalArray(List aBigDecimalArray) {
this.aBigDecimalArray = aBigDecimalArray;
}
public PrimitiveArraysObjectWithRestrictions aStringArray(List aStringArray) {
this.aStringArray = aStringArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addAStringArrayItem(String aStringArrayItem) {
if (this.aStringArray == null) {
this.aStringArray = new ArrayList<>();
}
this.aStringArray.add(aStringArrayItem);
return this;
}
/**
* Get aStringArray
* @return aStringArray
**/
@Schema(description = "")
public List getAStringArray() {
return aStringArray;
}
public void setAStringArray(List aStringArray) {
this.aStringArray = aStringArray;
}
public PrimitiveArraysObjectWithRestrictions bStringArray(List bStringArray) {
this.bStringArray = bStringArray;
return this;
}
public PrimitiveArraysObjectWithRestrictions addBStringArrayItem(String bStringArrayItem) {
if (this.bStringArray == null) {
this.bStringArray = new ArrayList<>();
}
this.bStringArray.add(bStringArrayItem);
return this;
}
/**
* Get bStringArray
* @return bStringArray
**/
@Schema(description = "")
public List getBStringArray() {
return bStringArray;
}
public void setBStringArray(List bStringArray) {
this.bStringArray = bStringArray;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PrimitiveArraysObjectWithRestrictions primitiveArraysObjectWithRestrictions = (PrimitiveArraysObjectWithRestrictions) o;
return Objects.equals(this.aBooleanArray, primitiveArraysObjectWithRestrictions.aBooleanArray) &&
Objects.equals(this.bBooleanArray, primitiveArraysObjectWithRestrictions.bBooleanArray) &&
Objects.equals(this.cBooleanArray, primitiveArraysObjectWithRestrictions.cBooleanArray) &&
Arrays.equals(this.aByteArray, primitiveArraysObjectWithRestrictions.aByteArray) &&
Arrays.equals(this.bByteArray, primitiveArraysObjectWithRestrictions.bByteArray) &&
Objects.equals(this.aShortArray, primitiveArraysObjectWithRestrictions.aShortArray) &&
Objects.equals(this.bShortArray, primitiveArraysObjectWithRestrictions.bShortArray) &&
Objects.equals(this.aIntegerArray, primitiveArraysObjectWithRestrictions.aIntegerArray) &&
Objects.equals(this.bIntegerArray, primitiveArraysObjectWithRestrictions.bIntegerArray) &&
Objects.equals(this.cIntegerArray, primitiveArraysObjectWithRestrictions.cIntegerArray) &&
Objects.equals(this.aLongArray, primitiveArraysObjectWithRestrictions.aLongArray) &&
Objects.equals(this.bLongArray, primitiveArraysObjectWithRestrictions.bLongArray) &&
Objects.equals(this.aBigIntegerArray, primitiveArraysObjectWithRestrictions.aBigIntegerArray) &&
Objects.equals(this.aCharacterArray, primitiveArraysObjectWithRestrictions.aCharacterArray) &&
Objects.equals(this.bCharacterArray, primitiveArraysObjectWithRestrictions.bCharacterArray) &&
Objects.equals(this.aFloatArray, primitiveArraysObjectWithRestrictions.aFloatArray) &&
Objects.equals(this.bFloatArray, primitiveArraysObjectWithRestrictions.bFloatArray) &&
Objects.equals(this.aDoubleArray, primitiveArraysObjectWithRestrictions.aDoubleArray) &&
Objects.equals(this.bDoubleArray, primitiveArraysObjectWithRestrictions.bDoubleArray) &&
Objects.equals(this.aBigDecimalArray, primitiveArraysObjectWithRestrictions.aBigDecimalArray) &&
Objects.equals(this.aStringArray, primitiveArraysObjectWithRestrictions.aStringArray) &&
Objects.equals(this.bStringArray, primitiveArraysObjectWithRestrictions.bStringArray);
}
@Override
public int hashCode() {
return Objects.hash(aBooleanArray, bBooleanArray, cBooleanArray, Arrays.hashCode(aByteArray), Arrays.hashCode(bByteArray), aShortArray, bShortArray, aIntegerArray, bIntegerArray, cIntegerArray, aLongArray, bLongArray, aBigIntegerArray, aCharacterArray, bCharacterArray, aFloatArray, bFloatArray, aDoubleArray, bDoubleArray, aBigDecimalArray, aStringArray, bStringArray);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PrimitiveArraysObjectWithRestrictions {\n");
sb.append(" aBooleanArray: ").append(toIndentedString(aBooleanArray)).append("\n");
sb.append(" bBooleanArray: ").append(toIndentedString(bBooleanArray)).append("\n");
sb.append(" cBooleanArray: ").append(toIndentedString(cBooleanArray)).append("\n");
sb.append(" aByteArray: ").append(toIndentedString(aByteArray)).append("\n");
sb.append(" bByteArray: ").append(toIndentedString(bByteArray)).append("\n");
sb.append(" aShortArray: ").append(toIndentedString(aShortArray)).append("\n");
sb.append(" bShortArray: ").append(toIndentedString(bShortArray)).append("\n");
sb.append(" aIntegerArray: ").append(toIndentedString(aIntegerArray)).append("\n");
sb.append(" bIntegerArray: ").append(toIndentedString(bIntegerArray)).append("\n");
sb.append(" cIntegerArray: ").append(toIndentedString(cIntegerArray)).append("\n");
sb.append(" aLongArray: ").append(toIndentedString(aLongArray)).append("\n");
sb.append(" bLongArray: ").append(toIndentedString(bLongArray)).append("\n");
sb.append(" aBigIntegerArray: ").append(toIndentedString(aBigIntegerArray)).append("\n");
sb.append(" aCharacterArray: ").append(toIndentedString(aCharacterArray)).append("\n");
sb.append(" bCharacterArray: ").append(toIndentedString(bCharacterArray)).append("\n");
sb.append(" aFloatArray: ").append(toIndentedString(aFloatArray)).append("\n");
sb.append(" bFloatArray: ").append(toIndentedString(bFloatArray)).append("\n");
sb.append(" aDoubleArray: ").append(toIndentedString(aDoubleArray)).append("\n");
sb.append(" bDoubleArray: ").append(toIndentedString(bDoubleArray)).append("\n");
sb.append(" aBigDecimalArray: ").append(toIndentedString(aBigDecimalArray)).append("\n");
sb.append(" aStringArray: ").append(toIndentedString(aStringArray)).append("\n");
sb.append(" bStringArray: ").append(toIndentedString(bStringArray)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy