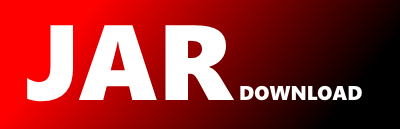
com.anaptecs.jeaf.openapi.WeirdBooking Maven / Gradle / Ivy
/*
* Product Base Definitions
* This component represents the Open API interface of the accounting service.
*
* OpenAPI spec version: 0.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.anaptecs.jeaf.openapi;
import java.util.Objects;
import java.util.Arrays;
import com.anaptecs.jeaf.openapi.Booking;
import com.anaptecs.jeaf.openapi.InventoryType;
import com.anaptecs.jeaf.openapi.WeirdParent;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
/**
* WeirdBooking
*/
public class WeirdBooking extends WeirdParent {
@JsonProperty("booking")
private String booking = null;
@JsonProperty("additionalBookings")
private List additionalBookings = null;
@JsonProperty("versionedObjectSoftLink")
private String versionedObjectSoftLink = null;
@JsonProperty("childProperty")
private Integer childProperty = null;
@JsonProperty("realBooking")
private Booking realBooking = null;
@JsonProperty("inventories")
private List inventories = null;
public WeirdBooking booking(String booking) {
this.booking = booking;
return this;
}
/**
* Internal ID 2nd line 3rd line
* @return booking
**/
@Schema(required = true, description = "Internal ID 2nd line 3rd line ")
public String getBooking() {
return booking;
}
public void setBooking(String booking) {
this.booking = booking;
}
public WeirdBooking additionalBookings(List additionalBookings) {
this.additionalBookings = additionalBookings;
return this;
}
public WeirdBooking addAdditionalBookingsItem(String additionalBookingsItem) {
if (this.additionalBookings == null) {
this.additionalBookings = new ArrayList<>();
}
this.additionalBookings.add(additionalBookingsItem);
return this;
}
/**
* additional bookings
* @return additionalBookings
**/
@Schema(description = "additional bookings ")
public List getAdditionalBookings() {
return additionalBookings;
}
public void setAdditionalBookings(List additionalBookings) {
this.additionalBookings = additionalBookings;
}
public WeirdBooking versionedObjectSoftLink(String versionedObjectSoftLink) {
this.versionedObjectSoftLink = versionedObjectSoftLink;
return this;
}
/**
* soft link 2nd line 3rd line
* @return versionedObjectSoftLink
**/
@Schema(required = true, description = "soft link 2nd line 3rd line ")
public String getVersionedObjectSoftLink() {
return versionedObjectSoftLink;
}
public void setVersionedObjectSoftLink(String versionedObjectSoftLink) {
this.versionedObjectSoftLink = versionedObjectSoftLink;
}
public WeirdBooking childProperty(Integer childProperty) {
this.childProperty = childProperty;
return this;
}
/**
* Get childProperty
* @return childProperty
**/
@Schema(required = true, description = "")
public Integer getChildProperty() {
return childProperty;
}
public void setChildProperty(Integer childProperty) {
this.childProperty = childProperty;
}
public WeirdBooking realBooking(Booking realBooking) {
this.realBooking = realBooking;
return this;
}
/**
* Get realBooking
* @return realBooking
**/
@Schema(description = "")
public Booking getRealBooking() {
return realBooking;
}
public void setRealBooking(Booking realBooking) {
this.realBooking = realBooking;
}
public WeirdBooking inventories(List inventories) {
this.inventories = inventories;
return this;
}
public WeirdBooking addInventoriesItem(InventoryType inventoriesItem) {
if (this.inventories == null) {
this.inventories = new ArrayList<>();
}
this.inventories.add(inventoriesItem);
return this;
}
/**
* <br><br> Breaking Change with 2.1: Association will required at least 1 object.
* @return inventories
**/
@Schema(description = "
Breaking Change with 2.1: Association will required at least 1 object. ")
public List getInventories() {
return inventories;
}
public void setInventories(List inventories) {
this.inventories = inventories;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
WeirdBooking weirdBooking = (WeirdBooking) o;
return Objects.equals(this.booking, weirdBooking.booking) &&
Objects.equals(this.additionalBookings, weirdBooking.additionalBookings) &&
Objects.equals(this.versionedObjectSoftLink, weirdBooking.versionedObjectSoftLink) &&
Objects.equals(this.childProperty, weirdBooking.childProperty) &&
Objects.equals(this.realBooking, weirdBooking.realBooking) &&
Objects.equals(this.inventories, weirdBooking.inventories) &&
super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(booking, additionalBookings, versionedObjectSoftLink, childProperty, realBooking, inventories, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WeirdBooking {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" booking: ").append(toIndentedString(booking)).append("\n");
sb.append(" additionalBookings: ").append(toIndentedString(additionalBookings)).append("\n");
sb.append(" versionedObjectSoftLink: ").append(toIndentedString(versionedObjectSoftLink)).append("\n");
sb.append(" childProperty: ").append(toIndentedString(childProperty)).append("\n");
sb.append(" realBooking: ").append(toIndentedString(realBooking)).append("\n");
sb.append(" inventories: ").append(toIndentedString(inventories)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy