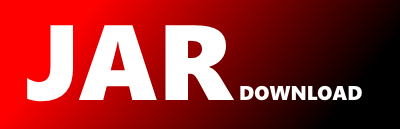
com.ancientlightstudios.example.features.server.FeaturesExtensionServer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-kotlin-openapi-test Show documentation
Show all versions of quarkus-kotlin-openapi-test Show documentation
An example application using the Kotlin OpenAPI generator.
// THIS IS A GENERATED FILE. DO NOT EDIT!
package com.ancientlightstudios.example.features.server
import com.ancientlightstudios.example.custom.*
import com.ancientlightstudios.quarkus.kotlin.openapi.*
import com.ancientlightstudios.quarkus.kotlin.openapi.extension.*
import com.fasterxml.jackson.databind.ObjectMapper
import jakarta.ws.rs.Consumes
import jakarta.ws.rs.POST
import jakarta.ws.rs.Path
import jakarta.ws.rs.QueryParam
import jakarta.ws.rs.core.Context
import jakarta.ws.rs.core.HttpHeaders
import kotlinx.coroutines.slf4j.MDCContext
import kotlinx.coroutines.withContext
import org.jboss.resteasy.reactive.RestResponse
import org.slf4j.Logger
import org.slf4j.LoggerFactory
import org.slf4j.MDC
@Path(value = "")
class FeaturesExtensionServer(
private val delegate: FeaturesExtensionServerDelegate,
private val objectMapper: ObjectMapper
) {
private val log: Logger = LoggerFactory.getLogger(FeaturesExtensionServer::class.java)
@POST
@Path(value = "/features/extension/instant")
@Consumes(value = ["application/json"])
suspend fun instantExtension(
@QueryParam("headerValue") headerValue: String?,
body: String?,
@Context headers: HttpHeaders
): RestResponse<*> {
MDC.put("request-method", "post")
MDC.put("request-path", "/features/extension/instant")
val headerValueMaybe = Maybe.Success("request.query.headerValue", headerValue)
.asInstant()
.required()
val bodyMaybe = Maybe.Success("request.body", body)
.asJson(objectMapper)
.asInstant()
.required()
return withContext(MDCContext()) {
log.info("[post] /features/extension/instant - processing request.")
val request = maybeAllOf("request", headerValueMaybe, bodyMaybe) {
InstantExtensionRequest((headerValueMaybe as Maybe.Success).value, (bodyMaybe as Maybe.Success).value)
}
val context = InstantExtensionContext(request, objectMapper, headers)
try {
delegate.run {
context.instantExtension()
}
} catch (requestHandledSignal: RequestHandledSignal) {
log.info("[post] /features/extension/instant - responding with status code ${requestHandledSignal.response.status}.")
requestHandledSignal.response
} catch (e: Exception) {
log.error("[post] /features/extension/instant - unexpected exception occurred. Please check the delegate implementation to make sure it never throws an exception. Response is now undefined.")
throw e
}
}
}
@POST
@Path(value = "/features/extension/uuid")
@Consumes(value = ["application/json"])
suspend fun uuidExtension(
@QueryParam("headerValue") headerValue: String?,
body: String?,
@Context headers: HttpHeaders
): RestResponse<*> {
MDC.put("request-method", "post")
MDC.put("request-path", "/features/extension/uuid")
val headerValueMaybe = Maybe.Success("request.query.headerValue", headerValue)
.asUUID()
.required()
val bodyMaybe = Maybe.Success("request.body", body)
.asJson(objectMapper)
.asUUID()
.required()
return withContext(MDCContext()) {
log.info("[post] /features/extension/uuid - processing request.")
val request = maybeAllOf("request", headerValueMaybe, bodyMaybe) {
UuidExtensionRequest((headerValueMaybe as Maybe.Success).value, (bodyMaybe as Maybe.Success).value)
}
val context = UuidExtensionContext(request, objectMapper, headers)
try {
delegate.run {
context.uuidExtension()
}
} catch (requestHandledSignal: RequestHandledSignal) {
log.info("[post] /features/extension/uuid - responding with status code ${requestHandledSignal.response.status}.")
requestHandledSignal.response
} catch (e: Exception) {
log.error("[post] /features/extension/uuid - unexpected exception occurred. Please check the delegate implementation to make sure it never throws an exception. Response is now undefined.")
throw e
}
}
}
@POST
@Path(value = "/features/extension/localDate")
@Consumes(value = ["application/json"])
suspend fun localDateExtension(
@QueryParam("headerValue") headerValue: String?,
body: String?,
@Context headers: HttpHeaders
): RestResponse<*> {
MDC.put("request-method", "post")
MDC.put("request-path", "/features/extension/localDate")
val headerValueMaybe = Maybe.Success("request.query.headerValue", headerValue)
.asLocalDate()
.required()
val bodyMaybe = Maybe.Success("request.body", body)
.asJson(objectMapper)
.asLocalDate()
.required()
return withContext(MDCContext()) {
log.info("[post] /features/extension/localDate - processing request.")
val request = maybeAllOf("request", headerValueMaybe, bodyMaybe) {
LocalDateExtensionRequest((headerValueMaybe as Maybe.Success).value, (bodyMaybe as Maybe.Success).value)
}
val context = LocalDateExtensionContext(request, objectMapper, headers)
try {
delegate.run {
context.localDateExtension()
}
} catch (requestHandledSignal: RequestHandledSignal) {
log.info("[post] /features/extension/localDate - responding with status code ${requestHandledSignal.response.status}.")
requestHandledSignal.response
} catch (e: Exception) {
log.error("[post] /features/extension/localDate - unexpected exception occurred. Please check the delegate implementation to make sure it never throws an exception. Response is now undefined.")
throw e
}
}
}
}