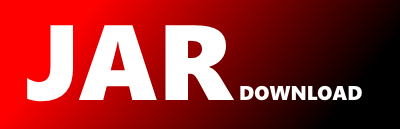
com.google.wireless.android.play.playlog.proto.ClientAnalytics Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: clientanalytics.proto
package com.google.wireless.android.play.playlog.proto;
public final class ClientAnalytics {
private ClientAnalytics() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface LogEventKeyValuesOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string key = 1;
/**
* optional string key = 1;
*/
boolean hasKey();
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
// optional string value = 2;
/**
* optional string value = 2;
*/
boolean hasValue();
/**
* optional string value = 2;
*/
java.lang.String getValue();
/**
* optional string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogEventKeyValues}
*
*
* Semi-arbitrary key-value pairs describing the event. Common entries
* will be extracted into strongly typed fields in the GWS log records.
*
*/
public static final class LogEventKeyValues extends
com.google.protobuf.GeneratedMessage
implements LogEventKeyValuesOrBuilder {
// Use LogEventKeyValues.newBuilder() to construct.
private LogEventKeyValues(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private LogEventKeyValues(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final LogEventKeyValues defaultInstance;
public static LogEventKeyValues getDefaultInstance() {
return defaultInstance;
}
public LogEventKeyValues getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogEventKeyValues(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEventKeyValues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public LogEventKeyValues parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogEventKeyValues(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private java.lang.Object key_;
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
/**
* optional string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* optional string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
key_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogEventKeyValues}
*
*
* Semi-arbitrary key-value pairs describing the event. Common entries
* will be extracted into strongly typed fields in the GWS log records.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEventKeyValues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
if (other.hasValue()) {
bitField0_ |= 0x00000002;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string key = 1;
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
// optional string value = 2;
private java.lang.Object value_ = "";
/**
* optional string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* optional string value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* optional string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.LogEventKeyValues)
}
static {
defaultInstance = new LogEventKeyValues(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.LogEventKeyValues)
}
public interface ActiveExperimentsOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated string client_altering_experiment = 1;
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
java.util.List
getClientAlteringExperimentList();
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
int getClientAlteringExperimentCount();
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
java.lang.String getClientAlteringExperiment(int index);
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
com.google.protobuf.ByteString
getClientAlteringExperimentBytes(int index);
// repeated string other_experiment = 2;
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
java.util.List
getOtherExperimentList();
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
int getOtherExperimentCount();
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
java.lang.String getOtherExperiment(int index);
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
com.google.protobuf.ByteString
getOtherExperimentBytes(int index);
// repeated int32 gws_experiment = 3;
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
java.util.List getGwsExperimentList();
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
int getGwsExperimentCount();
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
int getGwsExperiment(int index);
// repeated int64 play_experiment = 4;
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
java.util.List getPlayExperimentList();
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
int getPlayExperimentCount();
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
long getPlayExperiment(int index);
// repeated int64 unsupported_play_experiment = 5;
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
java.util.List getUnsupportedPlayExperimentList();
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
int getUnsupportedPlayExperimentCount();
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
long getUnsupportedPlayExperiment(int index);
}
/**
* Protobuf type {@code wireless_android_play_playlog.ActiveExperiments}
*
*
* The set of currently active experiments for a given LogEvent.
*
* Next tag: 6
*
*/
public static final class ActiveExperiments extends
com.google.protobuf.GeneratedMessage
implements ActiveExperimentsOrBuilder {
// Use ActiveExperiments.newBuilder() to construct.
private ActiveExperiments(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ActiveExperiments(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ActiveExperiments defaultInstance;
public static ActiveExperiments getDefaultInstance() {
return defaultInstance;
}
public ActiveExperiments getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ActiveExperiments(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
clientAlteringExperiment_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
clientAlteringExperiment_.add(input.readBytes());
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
otherExperiment_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
otherExperiment_.add(input.readBytes());
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
gwsExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
gwsExperiment_.add(input.readInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
gwsExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
gwsExperiment_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
playExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
playExperiment_.add(input.readInt64());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008) && input.getBytesUntilLimit() > 0) {
playExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
playExperiment_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
case 40: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
unsupportedPlayExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
unsupportedPlayExperiment_.add(input.readInt64());
break;
}
case 42: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010) && input.getBytesUntilLimit() > 0) {
unsupportedPlayExperiment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
while (input.getBytesUntilLimit() > 0) {
unsupportedPlayExperiment_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
clientAlteringExperiment_ = new com.google.protobuf.UnmodifiableLazyStringList(clientAlteringExperiment_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
otherExperiment_ = new com.google.protobuf.UnmodifiableLazyStringList(otherExperiment_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
gwsExperiment_ = java.util.Collections.unmodifiableList(gwsExperiment_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
playExperiment_ = java.util.Collections.unmodifiableList(playExperiment_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
unsupportedPlayExperiment_ = java.util.Collections.unmodifiableList(unsupportedPlayExperiment_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ActiveExperiments_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ActiveExperiments parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ActiveExperiments(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated string client_altering_experiment = 1;
public static final int CLIENT_ALTERING_EXPERIMENT_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList clientAlteringExperiment_;
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public java.util.List
getClientAlteringExperimentList() {
return clientAlteringExperiment_;
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public int getClientAlteringExperimentCount() {
return clientAlteringExperiment_.size();
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public java.lang.String getClientAlteringExperiment(int index) {
return clientAlteringExperiment_.get(index);
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public com.google.protobuf.ByteString
getClientAlteringExperimentBytes(int index) {
return clientAlteringExperiment_.getByteString(index);
}
// repeated string other_experiment = 2;
public static final int OTHER_EXPERIMENT_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList otherExperiment_;
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public java.util.List
getOtherExperimentList() {
return otherExperiment_;
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public int getOtherExperimentCount() {
return otherExperiment_.size();
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public java.lang.String getOtherExperiment(int index) {
return otherExperiment_.get(index);
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public com.google.protobuf.ByteString
getOtherExperimentBytes(int index) {
return otherExperiment_.getByteString(index);
}
// repeated int32 gws_experiment = 3;
public static final int GWS_EXPERIMENT_FIELD_NUMBER = 3;
private java.util.List gwsExperiment_;
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public java.util.List
getGwsExperimentList() {
return gwsExperiment_;
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public int getGwsExperimentCount() {
return gwsExperiment_.size();
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public int getGwsExperiment(int index) {
return gwsExperiment_.get(index);
}
// repeated int64 play_experiment = 4;
public static final int PLAY_EXPERIMENT_FIELD_NUMBER = 4;
private java.util.List playExperiment_;
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public java.util.List
getPlayExperimentList() {
return playExperiment_;
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public int getPlayExperimentCount() {
return playExperiment_.size();
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public long getPlayExperiment(int index) {
return playExperiment_.get(index);
}
// repeated int64 unsupported_play_experiment = 5;
public static final int UNSUPPORTED_PLAY_EXPERIMENT_FIELD_NUMBER = 5;
private java.util.List unsupportedPlayExperiment_;
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public java.util.List
getUnsupportedPlayExperimentList() {
return unsupportedPlayExperiment_;
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public int getUnsupportedPlayExperimentCount() {
return unsupportedPlayExperiment_.size();
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public long getUnsupportedPlayExperiment(int index) {
return unsupportedPlayExperiment_.get(index);
}
private void initFields() {
clientAlteringExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
otherExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
gwsExperiment_ = java.util.Collections.emptyList();
playExperiment_ = java.util.Collections.emptyList();
unsupportedPlayExperiment_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < clientAlteringExperiment_.size(); i++) {
output.writeBytes(1, clientAlteringExperiment_.getByteString(i));
}
for (int i = 0; i < otherExperiment_.size(); i++) {
output.writeBytes(2, otherExperiment_.getByteString(i));
}
for (int i = 0; i < gwsExperiment_.size(); i++) {
output.writeInt32(3, gwsExperiment_.get(i));
}
for (int i = 0; i < playExperiment_.size(); i++) {
output.writeInt64(4, playExperiment_.get(i));
}
for (int i = 0; i < unsupportedPlayExperiment_.size(); i++) {
output.writeInt64(5, unsupportedPlayExperiment_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < clientAlteringExperiment_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(clientAlteringExperiment_.getByteString(i));
}
size += dataSize;
size += 1 * getClientAlteringExperimentList().size();
}
{
int dataSize = 0;
for (int i = 0; i < otherExperiment_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(otherExperiment_.getByteString(i));
}
size += dataSize;
size += 1 * getOtherExperimentList().size();
}
{
int dataSize = 0;
for (int i = 0; i < gwsExperiment_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(gwsExperiment_.get(i));
}
size += dataSize;
size += 1 * getGwsExperimentList().size();
}
{
int dataSize = 0;
for (int i = 0; i < playExperiment_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(playExperiment_.get(i));
}
size += dataSize;
size += 1 * getPlayExperimentList().size();
}
{
int dataSize = 0;
for (int i = 0; i < unsupportedPlayExperiment_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(unsupportedPlayExperiment_.get(i));
}
size += dataSize;
size += 1 * getUnsupportedPlayExperimentList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.ActiveExperiments}
*
*
* The set of currently active experiments for a given LogEvent.
*
* Next tag: 6
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ActiveExperiments_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientAlteringExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
otherExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
gwsExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
playExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
unsupportedPlayExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
clientAlteringExperiment_ = new com.google.protobuf.UnmodifiableLazyStringList(
clientAlteringExperiment_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.clientAlteringExperiment_ = clientAlteringExperiment_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
otherExperiment_ = new com.google.protobuf.UnmodifiableLazyStringList(
otherExperiment_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.otherExperiment_ = otherExperiment_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
gwsExperiment_ = java.util.Collections.unmodifiableList(gwsExperiment_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.gwsExperiment_ = gwsExperiment_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
playExperiment_ = java.util.Collections.unmodifiableList(playExperiment_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.playExperiment_ = playExperiment_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
unsupportedPlayExperiment_ = java.util.Collections.unmodifiableList(unsupportedPlayExperiment_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.unsupportedPlayExperiment_ = unsupportedPlayExperiment_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance()) return this;
if (!other.clientAlteringExperiment_.isEmpty()) {
if (clientAlteringExperiment_.isEmpty()) {
clientAlteringExperiment_ = other.clientAlteringExperiment_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureClientAlteringExperimentIsMutable();
clientAlteringExperiment_.addAll(other.clientAlteringExperiment_);
}
onChanged();
}
if (!other.otherExperiment_.isEmpty()) {
if (otherExperiment_.isEmpty()) {
otherExperiment_ = other.otherExperiment_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOtherExperimentIsMutable();
otherExperiment_.addAll(other.otherExperiment_);
}
onChanged();
}
if (!other.gwsExperiment_.isEmpty()) {
if (gwsExperiment_.isEmpty()) {
gwsExperiment_ = other.gwsExperiment_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureGwsExperimentIsMutable();
gwsExperiment_.addAll(other.gwsExperiment_);
}
onChanged();
}
if (!other.playExperiment_.isEmpty()) {
if (playExperiment_.isEmpty()) {
playExperiment_ = other.playExperiment_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensurePlayExperimentIsMutable();
playExperiment_.addAll(other.playExperiment_);
}
onChanged();
}
if (!other.unsupportedPlayExperiment_.isEmpty()) {
if (unsupportedPlayExperiment_.isEmpty()) {
unsupportedPlayExperiment_ = other.unsupportedPlayExperiment_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureUnsupportedPlayExperimentIsMutable();
unsupportedPlayExperiment_.addAll(other.unsupportedPlayExperiment_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated string client_altering_experiment = 1;
private com.google.protobuf.LazyStringList clientAlteringExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureClientAlteringExperimentIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
clientAlteringExperiment_ = new com.google.protobuf.LazyStringArrayList(clientAlteringExperiment_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public java.util.List
getClientAlteringExperimentList() {
return java.util.Collections.unmodifiableList(clientAlteringExperiment_);
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public int getClientAlteringExperimentCount() {
return clientAlteringExperiment_.size();
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public java.lang.String getClientAlteringExperiment(int index) {
return clientAlteringExperiment_.get(index);
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public com.google.protobuf.ByteString
getClientAlteringExperimentBytes(int index) {
return clientAlteringExperiment_.getByteString(index);
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public Builder setClientAlteringExperiment(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureClientAlteringExperimentIsMutable();
clientAlteringExperiment_.set(index, value);
onChanged();
return this;
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public Builder addClientAlteringExperiment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureClientAlteringExperimentIsMutable();
clientAlteringExperiment_.add(value);
onChanged();
return this;
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public Builder addAllClientAlteringExperiment(
java.lang.Iterable values) {
ensureClientAlteringExperimentIsMutable();
super.addAll(values, clientAlteringExperiment_);
onChanged();
return this;
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public Builder clearClientAlteringExperiment() {
clientAlteringExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string client_altering_experiment = 1;
*
*
* The list of IDs of client-behavior-altering experiments that are on.
*
*/
public Builder addClientAlteringExperimentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureClientAlteringExperimentIsMutable();
clientAlteringExperiment_.add(value);
onChanged();
return this;
}
// repeated string other_experiment = 2;
private com.google.protobuf.LazyStringList otherExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureOtherExperimentIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
otherExperiment_ = new com.google.protobuf.LazyStringArrayList(otherExperiment_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public java.util.List
getOtherExperimentList() {
return java.util.Collections.unmodifiableList(otherExperiment_);
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public int getOtherExperimentCount() {
return otherExperiment_.size();
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public java.lang.String getOtherExperiment(int index) {
return otherExperiment_.get(index);
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public com.google.protobuf.ByteString
getOtherExperimentBytes(int index) {
return otherExperiment_.getByteString(index);
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public Builder setOtherExperiment(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOtherExperimentIsMutable();
otherExperiment_.set(index, value);
onChanged();
return this;
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public Builder addOtherExperiment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOtherExperimentIsMutable();
otherExperiment_.add(value);
onChanged();
return this;
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public Builder addAllOtherExperiment(
java.lang.Iterable values) {
ensureOtherExperimentIsMutable();
super.addAll(values, otherExperiment_);
onChanged();
return this;
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public Builder clearOtherExperiment() {
otherExperiment_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string other_experiment = 2;
*
*
* The list of IDs of other experiments that are on. The client must not
* change its behavior in response to these experiments.
*
*/
public Builder addOtherExperimentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureOtherExperimentIsMutable();
otherExperiment_.add(value);
onChanged();
return this;
}
// repeated int32 gws_experiment = 3;
private java.util.List gwsExperiment_ = java.util.Collections.emptyList();
private void ensureGwsExperimentIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
gwsExperiment_ = new java.util.ArrayList(gwsExperiment_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public java.util.List
getGwsExperimentList() {
return java.util.Collections.unmodifiableList(gwsExperiment_);
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public int getGwsExperimentCount() {
return gwsExperiment_.size();
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public int getGwsExperiment(int index) {
return gwsExperiment_.get(index);
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public Builder setGwsExperiment(
int index, int value) {
ensureGwsExperimentIsMutable();
gwsExperiment_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public Builder addGwsExperiment(int value) {
ensureGwsExperimentIsMutable();
gwsExperiment_.add(value);
onChanged();
return this;
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public Builder addAllGwsExperiment(
java.lang.Iterable extends java.lang.Integer> values) {
ensureGwsExperimentIsMutable();
super.addAll(values, gwsExperiment_);
onChanged();
return this;
}
/**
* repeated int32 gws_experiment = 3;
*
*
* The list of GWS experiments that are on. These are not targeted by Playlog.
*
*/
public Builder clearGwsExperiment() {
gwsExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
// repeated int64 play_experiment = 4;
private java.util.List playExperiment_ = java.util.Collections.emptyList();
private void ensurePlayExperimentIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
playExperiment_ = new java.util.ArrayList(playExperiment_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public java.util.List
getPlayExperimentList() {
return java.util.Collections.unmodifiableList(playExperiment_);
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public int getPlayExperimentCount() {
return playExperiment_.size();
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public long getPlayExperiment(int index) {
return playExperiment_.get(index);
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public Builder setPlayExperiment(
int index, long value) {
ensurePlayExperimentIsMutable();
playExperiment_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public Builder addPlayExperiment(long value) {
ensurePlayExperimentIsMutable();
playExperiment_.add(value);
onChanged();
return this;
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public Builder addAllPlayExperiment(
java.lang.Iterable extends java.lang.Long> values) {
ensurePlayExperimentIsMutable();
super.addAll(values, playExperiment_);
onChanged();
return this;
}
/**
* repeated int64 play_experiment = 4;
*
*
* The list of Play experiments that are enabled for this LogEvent.
* Includes both client-side and server-side experiments.
*
*/
public Builder clearPlayExperiment() {
playExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
// repeated int64 unsupported_play_experiment = 5;
private java.util.List unsupportedPlayExperiment_ = java.util.Collections.emptyList();
private void ensureUnsupportedPlayExperimentIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
unsupportedPlayExperiment_ = new java.util.ArrayList(unsupportedPlayExperiment_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public java.util.List
getUnsupportedPlayExperimentList() {
return java.util.Collections.unmodifiableList(unsupportedPlayExperiment_);
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public int getUnsupportedPlayExperimentCount() {
return unsupportedPlayExperiment_.size();
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public long getUnsupportedPlayExperiment(int index) {
return unsupportedPlayExperiment_.get(index);
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public Builder setUnsupportedPlayExperiment(
int index, long value) {
ensureUnsupportedPlayExperimentIsMutable();
unsupportedPlayExperiment_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public Builder addUnsupportedPlayExperiment(long value) {
ensureUnsupportedPlayExperimentIsMutable();
unsupportedPlayExperiment_.add(value);
onChanged();
return this;
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public Builder addAllUnsupportedPlayExperiment(
java.lang.Iterable extends java.lang.Long> values) {
ensureUnsupportedPlayExperimentIsMutable();
super.addAll(values, unsupportedPlayExperiment_);
onChanged();
return this;
}
/**
* repeated int64 unsupported_play_experiment = 5;
*
*
* The list of Play experiments that were sent to the client for this LogEvent
* but is not recognized by the client. May include server-side experiments
* that were active, so correct this list in the logging service.
*
*/
public Builder clearUnsupportedPlayExperiment() {
unsupportedPlayExperiment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.ActiveExperiments)
}
static {
defaultInstance = new ActiveExperiments(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.ActiveExperiments)
}
public interface LogEventOrBuilder extends
com.google.protobuf.GeneratedMessage.
ExtendableMessageOrBuilder {
// optional int64 event_time_ms = 1;
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
boolean hasEventTimeMs();
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
long getEventTimeMs();
// optional int64 event_uptime_ms = 17;
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
boolean hasEventUptimeMs();
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
long getEventUptimeMs();
// optional int64 sequence_position = 21;
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
boolean hasSequencePosition();
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
long getSequencePosition();
// optional string tag = 2;
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
boolean hasTag();
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
java.lang.String getTag();
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
com.google.protobuf.ByteString
getTagBytes();
// optional int32 event_code = 11;
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
boolean hasEventCode();
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
int getEventCode();
// optional int32 event_flow_id = 12;
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
boolean hasEventFlowId();
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
int getEventFlowId();
// optional bool is_user_initiated = 10;
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
boolean hasIsUserInitiated();
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
boolean getIsUserInitiated();
// repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
java.util.List
getValueList();
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues getValue(int index);
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
int getValueCount();
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder>
getValueOrBuilderList();
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder getValueOrBuilder(
int index);
// optional bytes store = 4;
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
boolean hasStore();
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
com.google.protobuf.ByteString getStore();
// optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
boolean hasAppUsage1P();
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent getAppUsage1P();
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder getAppUsage1POrBuilder();
// optional bytes source_extension = 6;
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
boolean hasSourceExtension();
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
com.google.protobuf.ByteString getSourceExtension();
// optional string source_extension_js = 8;
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
boolean hasSourceExtensionJs();
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
java.lang.String getSourceExtensionJs();
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
com.google.protobuf.ByteString
getSourceExtensionJsBytes();
// optional string source_extension_json = 13;
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
boolean hasSourceExtensionJson();
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
java.lang.String getSourceExtensionJson();
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
com.google.protobuf.ByteString
getSourceExtensionJsonBytes();
// optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
boolean hasExp();
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments getExp();
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder getExpOrBuilder();
// optional string test_id = 14;
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
boolean hasTestId();
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
java.lang.String getTestId();
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
com.google.protobuf.ByteString
getTestIdBytes();
// optional sint64 timezone_offset_seconds = 15 [default = 180000];
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
boolean hasTimezoneOffsetSeconds();
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
long getTimezoneOffsetSeconds();
// optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
boolean hasExperimentIds();
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds getExperimentIds();
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder getExperimentIdsOrBuilder();
// optional bytes client_ve = 18;
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
boolean hasClientVe();
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
com.google.protobuf.ByteString getClientVe();
// optional string client_ve_js = 24;
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
boolean hasClientVeJs();
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
java.lang.String getClientVeJs();
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
com.google.protobuf.ByteString
getClientVeJsBytes();
// optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
boolean hasInternalEvent();
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent getInternalEvent();
// repeated int32 test_code = 20;
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
java.util.List getTestCodeList();
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
int getTestCodeCount();
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
int getTestCode(int index);
// optional int64 boot_count = 22;
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
boolean hasBootCount();
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
long getBootCount();
// optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
boolean hasNetworkConnectionInfo();
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo getNetworkConnectionInfo();
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder getNetworkConnectionInfoOrBuilder();
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogEvent}
*
*
* Next tag: 25
*
*/
public static final class LogEvent extends
com.google.protobuf.GeneratedMessage.ExtendableMessage<
LogEvent> implements LogEventOrBuilder {
// Use LogEvent.newBuilder() to construct.
private LogEvent(com.google.protobuf.GeneratedMessage.ExtendableBuilder builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private LogEvent(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final LogEvent defaultInstance;
public static LogEvent getDefaultInstance() {
return defaultInstance;
}
public LogEvent getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
eventTimeMs_ = input.readInt64();
break;
}
case 18: {
bitField0_ |= 0x00000008;
tag_ = input.readBytes();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
value_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
value_.add(input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.PARSER, extensionRegistry));
break;
}
case 34: {
bitField0_ |= 0x00000080;
store_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000200;
sourceExtension_ = input.readBytes();
break;
}
case 58: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder subBuilder = null;
if (((bitField0_ & 0x00001000) == 0x00001000)) {
subBuilder = exp_.toBuilder();
}
exp_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(exp_);
exp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00001000;
break;
}
case 66: {
bitField0_ |= 0x00000400;
sourceExtensionJs_ = input.readBytes();
break;
}
case 74: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = appUsage1P_.toBuilder();
}
appUsage1P_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(appUsage1P_);
appUsage1P_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 80: {
bitField0_ |= 0x00000040;
isUserInitiated_ = input.readBool();
break;
}
case 88: {
bitField0_ |= 0x00000010;
eventCode_ = input.readInt32();
break;
}
case 96: {
bitField0_ |= 0x00000020;
eventFlowId_ = input.readInt32();
break;
}
case 106: {
bitField0_ |= 0x00000800;
sourceExtensionJson_ = input.readBytes();
break;
}
case 114: {
bitField0_ |= 0x00002000;
testId_ = input.readBytes();
break;
}
case 120: {
bitField0_ |= 0x00004000;
timezoneOffsetSeconds_ = input.readSInt64();
break;
}
case 130: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder subBuilder = null;
if (((bitField0_ & 0x00008000) == 0x00008000)) {
subBuilder = experimentIds_.toBuilder();
}
experimentIds_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(experimentIds_);
experimentIds_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00008000;
break;
}
case 136: {
bitField0_ |= 0x00000002;
eventUptimeMs_ = input.readInt64();
break;
}
case 146: {
bitField0_ |= 0x00010000;
clientVe_ = input.readBytes();
break;
}
case 152: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(19, rawValue);
} else {
bitField0_ |= 0x00040000;
internalEvent_ = value;
}
break;
}
case 160: {
if (!((mutable_bitField0_ & 0x00100000) == 0x00100000)) {
testCode_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00100000;
}
testCode_.add(input.readInt32());
break;
}
case 162: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00100000) == 0x00100000) && input.getBytesUntilLimit() > 0) {
testCode_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00100000;
}
while (input.getBytesUntilLimit() > 0) {
testCode_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 168: {
bitField0_ |= 0x00000004;
sequencePosition_ = input.readInt64();
break;
}
case 176: {
bitField0_ |= 0x00080000;
bootCount_ = input.readInt64();
break;
}
case 186: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00100000) == 0x00100000)) {
subBuilder = networkConnectionInfo_.toBuilder();
}
networkConnectionInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(networkConnectionInfo_);
networkConnectionInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00100000;
break;
}
case 194: {
bitField0_ |= 0x00020000;
clientVeJs_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
value_ = java.util.Collections.unmodifiableList(value_);
}
if (((mutable_bitField0_ & 0x00100000) == 0x00100000)) {
testCode_ = java.util.Collections.unmodifiableList(testCode_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEvent_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public LogEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogEvent(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.LogEvent.InternalEvent}
*/
public enum InternalEvent
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NONE = 0;
*/
NONE(0, 0),
/**
* WALL_CLOCK_SET = 1;
*/
WALL_CLOCK_SET(1, 1),
/**
* DEVICE_BOOT = 2;
*/
DEVICE_BOOT(2, 2),
;
/**
* NONE = 0;
*/
public static final int NONE_VALUE = 0;
/**
* WALL_CLOCK_SET = 1;
*/
public static final int WALL_CLOCK_SET_VALUE = 1;
/**
* DEVICE_BOOT = 2;
*/
public static final int DEVICE_BOOT_VALUE = 2;
public final int getNumber() { return value; }
public static InternalEvent valueOf(int value) {
switch (value) {
case 0: return NONE;
case 1: return WALL_CLOCK_SET;
case 2: return DEVICE_BOOT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public InternalEvent findValueByNumber(int number) {
return InternalEvent.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.getDescriptor().getEnumTypes().get(0);
}
private static final InternalEvent[] VALUES = values();
public static InternalEvent valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private InternalEvent(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.LogEvent.InternalEvent)
}
private int bitField0_;
// optional int64 event_time_ms = 1;
public static final int EVENT_TIME_MS_FIELD_NUMBER = 1;
private long eventTimeMs_;
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public boolean hasEventTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public long getEventTimeMs() {
return eventTimeMs_;
}
// optional int64 event_uptime_ms = 17;
public static final int EVENT_UPTIME_MS_FIELD_NUMBER = 17;
private long eventUptimeMs_;
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public boolean hasEventUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public long getEventUptimeMs() {
return eventUptimeMs_;
}
// optional int64 sequence_position = 21;
public static final int SEQUENCE_POSITION_FIELD_NUMBER = 21;
private long sequencePosition_;
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public boolean hasSequencePosition() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public long getSequencePosition() {
return sequencePosition_;
}
// optional string tag = 2;
public static final int TAG_FIELD_NUMBER = 2;
private java.lang.Object tag_;
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public boolean hasTag() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public java.lang.String getTag() {
java.lang.Object ref = tag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tag_ = s;
}
return s;
}
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public com.google.protobuf.ByteString
getTagBytes() {
java.lang.Object ref = tag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int32 event_code = 11;
public static final int EVENT_CODE_FIELD_NUMBER = 11;
private int eventCode_;
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public boolean hasEventCode() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public int getEventCode() {
return eventCode_;
}
// optional int32 event_flow_id = 12;
public static final int EVENT_FLOW_ID_FIELD_NUMBER = 12;
private int eventFlowId_;
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public boolean hasEventFlowId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public int getEventFlowId() {
return eventFlowId_;
}
// optional bool is_user_initiated = 10;
public static final int IS_USER_INITIATED_FIELD_NUMBER = 10;
private boolean isUserInitiated_;
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public boolean hasIsUserInitiated() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public boolean getIsUserInitiated() {
return isUserInitiated_;
}
// repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
public static final int VALUE_FIELD_NUMBER = 3;
private java.util.List value_;
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public java.util.List getValueList() {
return value_;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder>
getValueOrBuilderList() {
return value_;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public int getValueCount() {
return value_.size();
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues getValue(int index) {
return value_.get(index);
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder getValueOrBuilder(
int index) {
return value_.get(index);
}
// optional bytes store = 4;
public static final int STORE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString store_;
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public boolean hasStore() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public com.google.protobuf.ByteString getStore() {
return store_;
}
// optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
public static final int APP_USAGE_1P_FIELD_NUMBER = 9;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent appUsage1P_;
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public boolean hasAppUsage1P() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent getAppUsage1P() {
return appUsage1P_;
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder getAppUsage1POrBuilder() {
return appUsage1P_;
}
// optional bytes source_extension = 6;
public static final int SOURCE_EXTENSION_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString sourceExtension_;
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public boolean hasSourceExtension() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public com.google.protobuf.ByteString getSourceExtension() {
return sourceExtension_;
}
// optional string source_extension_js = 8;
public static final int SOURCE_EXTENSION_JS_FIELD_NUMBER = 8;
private java.lang.Object sourceExtensionJs_;
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public boolean hasSourceExtensionJs() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public java.lang.String getSourceExtensionJs() {
java.lang.Object ref = sourceExtensionJs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sourceExtensionJs_ = s;
}
return s;
}
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public com.google.protobuf.ByteString
getSourceExtensionJsBytes() {
java.lang.Object ref = sourceExtensionJs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceExtensionJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string source_extension_json = 13;
public static final int SOURCE_EXTENSION_JSON_FIELD_NUMBER = 13;
private java.lang.Object sourceExtensionJson_;
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public boolean hasSourceExtensionJson() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public java.lang.String getSourceExtensionJson() {
java.lang.Object ref = sourceExtensionJson_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sourceExtensionJson_ = s;
}
return s;
}
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public com.google.protobuf.ByteString
getSourceExtensionJsonBytes() {
java.lang.Object ref = sourceExtensionJson_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceExtensionJson_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
public static final int EXP_FIELD_NUMBER = 7;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments exp_;
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public boolean hasExp() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments getExp() {
return exp_;
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder getExpOrBuilder() {
return exp_;
}
// optional string test_id = 14;
public static final int TEST_ID_FIELD_NUMBER = 14;
private java.lang.Object testId_;
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public boolean hasTestId() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public java.lang.String getTestId() {
java.lang.Object ref = testId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
testId_ = s;
}
return s;
}
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public com.google.protobuf.ByteString
getTestIdBytes() {
java.lang.Object ref = testId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
testId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional sint64 timezone_offset_seconds = 15 [default = 180000];
public static final int TIMEZONE_OFFSET_SECONDS_FIELD_NUMBER = 15;
private long timezoneOffsetSeconds_;
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public boolean hasTimezoneOffsetSeconds() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public long getTimezoneOffsetSeconds() {
return timezoneOffsetSeconds_;
}
// optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
public static final int EXPERIMENT_IDS_FIELD_NUMBER = 16;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds experimentIds_;
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public boolean hasExperimentIds() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds getExperimentIds() {
return experimentIds_;
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder getExperimentIdsOrBuilder() {
return experimentIds_;
}
// optional bytes client_ve = 18;
public static final int CLIENT_VE_FIELD_NUMBER = 18;
private com.google.protobuf.ByteString clientVe_;
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public boolean hasClientVe() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public com.google.protobuf.ByteString getClientVe() {
return clientVe_;
}
// optional string client_ve_js = 24;
public static final int CLIENT_VE_JS_FIELD_NUMBER = 24;
private java.lang.Object clientVeJs_;
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public boolean hasClientVeJs() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public java.lang.String getClientVeJs() {
java.lang.Object ref = clientVeJs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientVeJs_ = s;
}
return s;
}
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public com.google.protobuf.ByteString
getClientVeJsBytes() {
java.lang.Object ref = clientVeJs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientVeJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
public static final int INTERNAL_EVENT_FIELD_NUMBER = 19;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent internalEvent_;
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public boolean hasInternalEvent() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent getInternalEvent() {
return internalEvent_;
}
// repeated int32 test_code = 20;
public static final int TEST_CODE_FIELD_NUMBER = 20;
private java.util.List testCode_;
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public java.util.List
getTestCodeList() {
return testCode_;
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public int getTestCodeCount() {
return testCode_.size();
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public int getTestCode(int index) {
return testCode_.get(index);
}
// optional int64 boot_count = 22;
public static final int BOOT_COUNT_FIELD_NUMBER = 22;
private long bootCount_;
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public boolean hasBootCount() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public long getBootCount() {
return bootCount_;
}
// optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
public static final int NETWORK_CONNECTION_INFO_FIELD_NUMBER = 23;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo networkConnectionInfo_;
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public boolean hasNetworkConnectionInfo() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo getNetworkConnectionInfo() {
return networkConnectionInfo_;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder getNetworkConnectionInfoOrBuilder() {
return networkConnectionInfo_;
}
private void initFields() {
eventTimeMs_ = 0L;
eventUptimeMs_ = 0L;
sequencePosition_ = 0L;
tag_ = "";
eventCode_ = 0;
eventFlowId_ = 0;
isUserInitiated_ = false;
value_ = java.util.Collections.emptyList();
store_ = com.google.protobuf.ByteString.EMPTY;
appUsage1P_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance();
sourceExtension_ = com.google.protobuf.ByteString.EMPTY;
sourceExtensionJs_ = "";
sourceExtensionJson_ = "";
exp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance();
testId_ = "";
timezoneOffsetSeconds_ = 180000L;
experimentIds_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance();
clientVe_ = com.google.protobuf.ByteString.EMPTY;
clientVeJs_ = "";
internalEvent_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent.NONE;
testCode_ = java.util.Collections.emptyList();
bootCount_ = 0L;
networkConnectionInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!extensionsAreInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
com.google.protobuf.GeneratedMessage
.ExtendableMessage.ExtensionWriter extensionWriter =
newExtensionWriter();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, eventTimeMs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(2, getTagBytes());
}
for (int i = 0; i < value_.size(); i++) {
output.writeMessage(3, value_.get(i));
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(4, store_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(6, sourceExtension_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeMessage(7, exp_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBytes(8, getSourceExtensionJsBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(9, appUsage1P_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(10, isUserInitiated_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(11, eventCode_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt32(12, eventFlowId_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBytes(13, getSourceExtensionJsonBytes());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBytes(14, getTestIdBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeSInt64(15, timezoneOffsetSeconds_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeMessage(16, experimentIds_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(17, eventUptimeMs_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeBytes(18, clientVe_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeEnum(19, internalEvent_.getNumber());
}
for (int i = 0; i < testCode_.size(); i++) {
output.writeInt32(20, testCode_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(21, sequencePosition_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeInt64(22, bootCount_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeMessage(23, networkConnectionInfo_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeBytes(24, getClientVeJsBytes());
}
extensionWriter.writeUntil(536870912, output);
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, eventTimeMs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getTagBytes());
}
for (int i = 0; i < value_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, value_.get(i));
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, store_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, sourceExtension_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, exp_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getSourceExtensionJsBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, appUsage1P_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, isUserInitiated_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, eventCode_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(12, eventFlowId_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, getSourceExtensionJsonBytes());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(14, getTestIdBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(15, timezoneOffsetSeconds_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, experimentIds_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, eventUptimeMs_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(18, clientVe_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(19, internalEvent_.getNumber());
}
{
int dataSize = 0;
for (int i = 0; i < testCode_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(testCode_.get(i));
}
size += dataSize;
size += 2 * getTestCodeList().size();
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, sequencePosition_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, bootCount_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, networkConnectionInfo_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(24, getClientVeJsBytes());
}
size += extensionsSerializedSize();
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogEvent}
*
*
* Next tag: 25
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.ExtendableBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent, Builder> implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEvent_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getValueFieldBuilder();
getAppUsage1PFieldBuilder();
getExpFieldBuilder();
getExperimentIdsFieldBuilder();
getNetworkConnectionInfoFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
eventTimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
eventUptimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
sequencePosition_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
tag_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
eventCode_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
eventFlowId_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
isUserInitiated_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
valueBuilder_.clear();
}
store_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
if (appUsage1PBuilder_ == null) {
appUsage1P_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance();
} else {
appUsage1PBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
sourceExtension_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000400);
sourceExtensionJs_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
sourceExtensionJson_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
if (expBuilder_ == null) {
exp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance();
} else {
expBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
testId_ = "";
bitField0_ = (bitField0_ & ~0x00004000);
timezoneOffsetSeconds_ = 180000L;
bitField0_ = (bitField0_ & ~0x00008000);
if (experimentIdsBuilder_ == null) {
experimentIds_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance();
} else {
experimentIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00010000);
clientVe_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00020000);
clientVeJs_ = "";
bitField0_ = (bitField0_ & ~0x00040000);
internalEvent_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent.NONE;
bitField0_ = (bitField0_ & ~0x00080000);
testCode_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00100000);
bootCount_ = 0L;
bitField0_ = (bitField0_ & ~0x00200000);
if (networkConnectionInfoBuilder_ == null) {
networkConnectionInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance();
} else {
networkConnectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogEvent_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.eventTimeMs_ = eventTimeMs_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.eventUptimeMs_ = eventUptimeMs_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.sequencePosition_ = sequencePosition_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.tag_ = tag_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.eventCode_ = eventCode_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.eventFlowId_ = eventFlowId_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.isUserInitiated_ = isUserInitiated_;
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
value_ = java.util.Collections.unmodifiableList(value_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.store_ = store_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
if (appUsage1PBuilder_ == null) {
result.appUsage1P_ = appUsage1P_;
} else {
result.appUsage1P_ = appUsage1PBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.sourceExtension_ = sourceExtension_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.sourceExtensionJs_ = sourceExtensionJs_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000800;
}
result.sourceExtensionJson_ = sourceExtensionJson_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00001000;
}
if (expBuilder_ == null) {
result.exp_ = exp_;
} else {
result.exp_ = expBuilder_.build();
}
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00002000;
}
result.testId_ = testId_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00004000;
}
result.timezoneOffsetSeconds_ = timezoneOffsetSeconds_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00008000;
}
if (experimentIdsBuilder_ == null) {
result.experimentIds_ = experimentIds_;
} else {
result.experimentIds_ = experimentIdsBuilder_.build();
}
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00010000;
}
result.clientVe_ = clientVe_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00020000;
}
result.clientVeJs_ = clientVeJs_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00040000;
}
result.internalEvent_ = internalEvent_;
if (((bitField0_ & 0x00100000) == 0x00100000)) {
testCode_ = java.util.Collections.unmodifiableList(testCode_);
bitField0_ = (bitField0_ & ~0x00100000);
}
result.testCode_ = testCode_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00080000;
}
result.bootCount_ = bootCount_;
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00100000;
}
if (networkConnectionInfoBuilder_ == null) {
result.networkConnectionInfo_ = networkConnectionInfo_;
} else {
result.networkConnectionInfo_ = networkConnectionInfoBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.getDefaultInstance()) return this;
if (other.hasEventTimeMs()) {
setEventTimeMs(other.getEventTimeMs());
}
if (other.hasEventUptimeMs()) {
setEventUptimeMs(other.getEventUptimeMs());
}
if (other.hasSequencePosition()) {
setSequencePosition(other.getSequencePosition());
}
if (other.hasTag()) {
bitField0_ |= 0x00000008;
tag_ = other.tag_;
onChanged();
}
if (other.hasEventCode()) {
setEventCode(other.getEventCode());
}
if (other.hasEventFlowId()) {
setEventFlowId(other.getEventFlowId());
}
if (other.hasIsUserInitiated()) {
setIsUserInitiated(other.getIsUserInitiated());
}
if (valueBuilder_ == null) {
if (!other.value_.isEmpty()) {
if (value_.isEmpty()) {
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureValueIsMutable();
value_.addAll(other.value_);
}
onChanged();
}
} else {
if (!other.value_.isEmpty()) {
if (valueBuilder_.isEmpty()) {
valueBuilder_.dispose();
valueBuilder_ = null;
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000080);
valueBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getValueFieldBuilder() : null;
} else {
valueBuilder_.addAllMessages(other.value_);
}
}
}
if (other.hasStore()) {
setStore(other.getStore());
}
if (other.hasAppUsage1P()) {
mergeAppUsage1P(other.getAppUsage1P());
}
if (other.hasSourceExtension()) {
setSourceExtension(other.getSourceExtension());
}
if (other.hasSourceExtensionJs()) {
bitField0_ |= 0x00000800;
sourceExtensionJs_ = other.sourceExtensionJs_;
onChanged();
}
if (other.hasSourceExtensionJson()) {
bitField0_ |= 0x00001000;
sourceExtensionJson_ = other.sourceExtensionJson_;
onChanged();
}
if (other.hasExp()) {
mergeExp(other.getExp());
}
if (other.hasTestId()) {
bitField0_ |= 0x00004000;
testId_ = other.testId_;
onChanged();
}
if (other.hasTimezoneOffsetSeconds()) {
setTimezoneOffsetSeconds(other.getTimezoneOffsetSeconds());
}
if (other.hasExperimentIds()) {
mergeExperimentIds(other.getExperimentIds());
}
if (other.hasClientVe()) {
setClientVe(other.getClientVe());
}
if (other.hasClientVeJs()) {
bitField0_ |= 0x00040000;
clientVeJs_ = other.clientVeJs_;
onChanged();
}
if (other.hasInternalEvent()) {
setInternalEvent(other.getInternalEvent());
}
if (!other.testCode_.isEmpty()) {
if (testCode_.isEmpty()) {
testCode_ = other.testCode_;
bitField0_ = (bitField0_ & ~0x00100000);
} else {
ensureTestCodeIsMutable();
testCode_.addAll(other.testCode_);
}
onChanged();
}
if (other.hasBootCount()) {
setBootCount(other.getBootCount());
}
if (other.hasNetworkConnectionInfo()) {
mergeNetworkConnectionInfo(other.getNetworkConnectionInfo());
}
this.mergeExtensionFields(other);
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!extensionsAreInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 event_time_ms = 1;
private long eventTimeMs_ ;
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public boolean hasEventTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public long getEventTimeMs() {
return eventTimeMs_;
}
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public Builder setEventTimeMs(long value) {
bitField0_ |= 0x00000001;
eventTimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 event_time_ms = 1;
*
*
* Epoch time in milliseconds, according to the wall clock.
* See http://go/clearcut-timestamps for details on how this value is used.
* This field is required for Odyssey. Otherwise, events will be silently
* dropped.
*
*/
public Builder clearEventTimeMs() {
bitField0_ = (bitField0_ & ~0x00000001);
eventTimeMs_ = 0L;
onChanged();
return this;
}
// optional int64 event_uptime_ms = 17;
private long eventUptimeMs_ ;
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public boolean hasEventUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public long getEventUptimeMs() {
return eventUptimeMs_;
}
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public Builder setEventUptimeMs(long value) {
bitField0_ |= 0x00000002;
eventUptimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 event_uptime_ms = 17;
*
*
* Time in milliseconds since the device was booted. (On Android this
* is the result of SystemClock.elapsedRealtime(), not
* SystemClock.uptimeMillis() which does not increment when the device is in
* deep sleep.)
*
* See http://go/clearcut-timestamps for details on how this value is used for
* timestamp corrections. It is also copied to
* PlayExtension.client_elapsed_realtime_millis if the routing option
* "include_client_elapsed_realtime_millis" is set to true.
*
*/
public Builder clearEventUptimeMs() {
bitField0_ = (bitField0_ & ~0x00000002);
eventUptimeMs_ = 0L;
onChanged();
return this;
}
// optional int64 sequence_position = 21;
private long sequencePosition_ ;
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public boolean hasSequencePosition() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public long getSequencePosition() {
return sequencePosition_;
}
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public Builder setSequencePosition(long value) {
bitField0_ |= 0x00000004;
sequencePosition_ = value;
onChanged();
return this;
}
/**
* optional int64 sequence_position = 21;
*
*
* Client generated sequence position for this event - defines a total order
* for events from a given device. If event A's sequence position is less than
* event B's sequence position, then event A happened before event B.
*
*/
public Builder clearSequencePosition() {
bitField0_ = (bitField0_ & ~0x00000004);
sequencePosition_ = 0L;
onChanged();
return this;
}
// optional string tag = 2;
private java.lang.Object tag_ = "";
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public boolean hasTag() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public java.lang.String getTag() {
java.lang.Object ref = tag_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
tag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public com.google.protobuf.ByteString
getTagBytes() {
java.lang.Object ref = tag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public Builder setTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
tag_ = value;
onChanged();
return this;
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public Builder clearTag() {
bitField0_ = (bitField0_ & ~0x00000008);
tag_ = getDefaultInstance().getTag();
onChanged();
return this;
}
/**
* optional string tag = 2;
*
*
* Type of event, could be "CLICK" or "PAGE_VIEW" or "DOWNLOAD_STATUS".
* There will be a common repository of cross-vertical events that should be
* used, but any individual app would be free to define their own tags.
*
* STRONGLY DISCOURAGED: use event_code instead.
*
*/
public Builder setTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
tag_ = value;
onChanged();
return this;
}
// optional int32 event_code = 11;
private int eventCode_ ;
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public boolean hasEventCode() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public int getEventCode() {
return eventCode_;
}
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public Builder setEventCode(int value) {
bitField0_ |= 0x00000010;
eventCode_ = value;
onChanged();
return this;
}
/**
* optional int32 event_code = 11;
*
*
* Type of event. Different kinds of events inside a client app should have
* different values of 'event_code'. Different client apps may have
* overlapping sets of 'event_code' values; these values are only meant to
* make sense within the context of a single client app, as identified by
* the 'log_source' field of LogRequest.
*
* A typical way to use 'event_code' is to define the enum of possible values
* inside of the custom logging extension proto.
* TODO(abednego): add an example CL.
*
* This enum type should be annotated with a LogEventRoutingConfig proto.
*
*/
public Builder clearEventCode() {
bitField0_ = (bitField0_ & ~0x00000010);
eventCode_ = 0;
onChanged();
return this;
}
// optional int32 event_flow_id = 12;
private int eventFlowId_ ;
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public boolean hasEventFlowId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public int getEventFlowId() {
return eventFlowId_;
}
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public Builder setEventFlowId(int value) {
bitField0_ |= 0x00000020;
eventFlowId_ = value;
onChanged();
return this;
}
/**
* optional int32 event_flow_id = 12;
*
*
* Identifies a group of events that belong to the same flow or session of
* user interaction, which is a client-specific concept.
*
* These IDs are used in the Clearcut/UMA pipeline for computing latencies
* between pairs of events, where it is necessary to ensure that both events
* belong to the same flow.
*
* For example, the Google Play team may want to give a unique ID to each
* purchase session, including all of the events starting from the initial
* search for an app to the eventual purchase and installation of the app.
* Another example would be the Search app wanting to tie together search
* query events and clicks on search results, especially if several instances
* of the app may be active at the same time.
*
*/
public Builder clearEventFlowId() {
bitField0_ = (bitField0_ & ~0x00000020);
eventFlowId_ = 0;
onChanged();
return this;
}
// optional bool is_user_initiated = 10;
private boolean isUserInitiated_ ;
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public boolean hasIsUserInitiated() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public boolean getIsUserInitiated() {
return isUserInitiated_;
}
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public Builder setIsUserInitiated(boolean value) {
bitField0_ |= 0x00000040;
isUserInitiated_ = value;
onChanged();
return this;
}
/**
* optional bool is_user_initiated = 10;
*
*
* Whether this event was initiated by the user at the time of the event or
* shortly before (vs. a background event like upgrading a database after the
* app was automatically updated).
*
*/
public Builder clearIsUserInitiated() {
bitField0_ = (bitField0_ & ~0x00000040);
isUserInitiated_ = false;
onChanged();
return this;
}
// repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
private java.util.List value_ =
java.util.Collections.emptyList();
private void ensureValueIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
value_ = new java.util.ArrayList(value_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder> valueBuilder_;
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public java.util.List getValueList() {
if (valueBuilder_ == null) {
return java.util.Collections.unmodifiableList(value_);
} else {
return valueBuilder_.getMessageList();
}
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public int getValueCount() {
if (valueBuilder_ == null) {
return value_.size();
} else {
return valueBuilder_.getCount();
}
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues getValue(int index) {
if (valueBuilder_ == null) {
return value_.get(index);
} else {
return valueBuilder_.getMessage(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder setValue(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.set(index, value);
onChanged();
} else {
valueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder setValue(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.set(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder addValue(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(value);
onChanged();
} else {
valueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder addValue(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(index, value);
onChanged();
} else {
valueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder addValue(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder addValue(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder addAllValue(
java.lang.Iterable extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues> values) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
super.addAll(values, value_);
onChanged();
} else {
valueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
valueBuilder_.clear();
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public Builder removeValue(int index) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.remove(index);
onChanged();
} else {
valueBuilder_.remove(index);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder getValueBuilder(
int index) {
return getValueFieldBuilder().getBuilder(index);
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder getValueOrBuilder(
int index) {
if (valueBuilder_ == null) {
return value_.get(index); } else {
return valueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder>
getValueOrBuilderList() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(value_);
}
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder addValueBuilder() {
return getValueFieldBuilder().addBuilder(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder addValueBuilder(
int index) {
return getValueFieldBuilder().addBuilder(
index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogEventKeyValues value = 3;
*
*
* These might contain PII and will not be stored for more than 8 days.
* If possible, please create a named field in the protos below instead.
*
*/
public java.util.List
getValueBuilderList() {
return getValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValues.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventKeyValuesOrBuilder>(
value_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
// optional bytes store = 4;
private com.google.protobuf.ByteString store_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public boolean hasStore() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public com.google.protobuf.ByteString getStore() {
return store_;
}
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public Builder setStore(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
store_ = value;
onChanged();
return this;
}
/**
* optional bytes store = 4;
*
*
* TODO(jsw): Remove. We can't do that as of 2015/08/13 because we still get
* several thousand QPS from ancient Play Store clients who use this field.
*
* Extension for Play Store app logs. Real type is PlayStoreLogEvent. This
* exists to support legacy clients. Use source_extension instead.
*
*/
public Builder clearStore() {
bitField0_ = (bitField0_ & ~0x00000100);
store_ = getDefaultInstance().getStore();
onChanged();
return this;
}
// optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent appUsage1P_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder> appUsage1PBuilder_;
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public boolean hasAppUsage1P() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent getAppUsage1P() {
if (appUsage1PBuilder_ == null) {
return appUsage1P_;
} else {
return appUsage1PBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public Builder setAppUsage1P(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent value) {
if (appUsage1PBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
appUsage1P_ = value;
onChanged();
} else {
appUsage1PBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public Builder setAppUsage1P(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder builderForValue) {
if (appUsage1PBuilder_ == null) {
appUsage1P_ = builderForValue.build();
onChanged();
} else {
appUsage1PBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public Builder mergeAppUsage1P(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent value) {
if (appUsage1PBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
appUsage1P_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance()) {
appUsage1P_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.newBuilder(appUsage1P_).mergeFrom(value).buildPartial();
} else {
appUsage1P_ = value;
}
onChanged();
} else {
appUsage1PBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public Builder clearAppUsage1P() {
if (appUsage1PBuilder_ == null) {
appUsage1P_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance();
onChanged();
} else {
appUsage1PBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder getAppUsage1PBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getAppUsage1PFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder getAppUsage1POrBuilder() {
if (appUsage1PBuilder_ != null) {
return appUsage1PBuilder_.getMessageOrBuilder();
} else {
return appUsage1P_;
}
}
/**
* optional .wireless_android_play_playlog.AppUsage1pLogEvent app_usage_1p = 9;
*
*
* First-party app usage tracking. Used only when log_source = APP_USAGE_1P.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder>
getAppUsage1PFieldBuilder() {
if (appUsage1PBuilder_ == null) {
appUsage1PBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder>(
appUsage1P_,
getParentForChildren(),
isClean());
appUsage1P_ = null;
}
return appUsage1PBuilder_;
}
// optional bytes source_extension = 6;
private com.google.protobuf.ByteString sourceExtension_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public boolean hasSourceExtension() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public com.google.protobuf.ByteString getSourceExtension() {
return sourceExtension_;
}
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public Builder setSourceExtension(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
sourceExtension_ = value;
onChanged();
return this;
}
/**
* optional bytes source_extension = 6;
*
*
* A source specific extension (typically a serialized proto). The type of
* this data is determined by the log_source of the enclosing LogRequest.
* The proto is derived by calling ParseFromString method in
* net/proto2/public/message_lite.h.
*
*/
public Builder clearSourceExtension() {
bitField0_ = (bitField0_ & ~0x00000400);
sourceExtension_ = getDefaultInstance().getSourceExtension();
onChanged();
return this;
}
// optional string source_extension_js = 8;
private java.lang.Object sourceExtensionJs_ = "";
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public boolean hasSourceExtensionJs() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public java.lang.String getSourceExtensionJs() {
java.lang.Object ref = sourceExtensionJs_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
sourceExtensionJs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public com.google.protobuf.ByteString
getSourceExtensionJsBytes() {
java.lang.Object ref = sourceExtensionJs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceExtensionJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder setSourceExtensionJs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
sourceExtensionJs_ = value;
onChanged();
return this;
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder clearSourceExtensionJs() {
bitField0_ = (bitField0_ & ~0x00000800);
sourceExtensionJs_ = getDefaultInstance().getSourceExtensionJs();
onChanged();
return this;
}
/**
* optional string source_extension_js = 8;
*
*
* A jspb version of source_extension, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder setSourceExtensionJsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
sourceExtensionJs_ = value;
onChanged();
return this;
}
// optional string source_extension_json = 13;
private java.lang.Object sourceExtensionJson_ = "";
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public boolean hasSourceExtensionJson() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public java.lang.String getSourceExtensionJson() {
java.lang.Object ref = sourceExtensionJson_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
sourceExtensionJson_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public com.google.protobuf.ByteString
getSourceExtensionJsonBytes() {
java.lang.Object ref = sourceExtensionJson_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceExtensionJson_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public Builder setSourceExtensionJson(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
sourceExtensionJson_ = value;
onChanged();
return this;
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public Builder clearSourceExtensionJson() {
bitField0_ = (bitField0_ & ~0x00001000);
sourceExtensionJson_ = getDefaultInstance().getSourceExtensionJson();
onChanged();
return this;
}
/**
* optional string source_extension_json = 13;
*
*
* A stringified json proto version of source_extension. The json string is
* converted back to proto using the json_format utility at
* net/proto2/util/public/json_format.h, without any flags.
*
*/
public Builder setSourceExtensionJsonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
sourceExtensionJson_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments exp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder> expBuilder_;
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public boolean hasExp() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments getExp() {
if (expBuilder_ == null) {
return exp_;
} else {
return expBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public Builder setExp(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments value) {
if (expBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
exp_ = value;
onChanged();
} else {
expBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public Builder setExp(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder builderForValue) {
if (expBuilder_ == null) {
exp_ = builderForValue.build();
onChanged();
} else {
expBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public Builder mergeExp(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments value) {
if (expBuilder_ == null) {
if (((bitField0_ & 0x00002000) == 0x00002000) &&
exp_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance()) {
exp_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.newBuilder(exp_).mergeFrom(value).buildPartial();
} else {
exp_ = value;
}
onChanged();
} else {
expBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public Builder clearExp() {
if (expBuilder_ == null) {
exp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.getDefaultInstance();
onChanged();
} else {
expBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
return this;
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder getExpBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getExpFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder getExpOrBuilder() {
if (expBuilder_ != null) {
return expBuilder_.getMessageOrBuilder();
} else {
return exp_;
}
}
/**
* optional .wireless_android_play_playlog.ActiveExperiments exp = 7;
*
*
* The set of currently active experiments. If missing, then the server will
* assume that experiment on/off status has not changed since the previous
* LogEvent message in the same LogRequest proto. If missing in the very first
* LogEvent of a LogRequest, then the server will assume that all experiments
* are off.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder>
getExpFieldBuilder() {
if (expBuilder_ == null) {
expBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperiments.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ActiveExperimentsOrBuilder>(
exp_,
getParentForChildren(),
isClean());
exp_ = null;
}
return expBuilder_;
}
// optional string test_id = 14;
private java.lang.Object testId_ = "";
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public boolean hasTestId() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public java.lang.String getTestId() {
java.lang.Object ref = testId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
testId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public com.google.protobuf.ByteString
getTestIdBytes() {
java.lang.Object ref = testId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
testId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public Builder setTestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
testId_ = value;
onChanged();
return this;
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public Builder clearTestId() {
bitField0_ = (bitField0_ & ~0x00004000);
testId_ = getDefaultInstance().getTestId();
onChanged();
return this;
}
/**
* optional string test_id = 14;
*
*
* A string for identifying a test run. You may use this string to group log
* data coming from a test run. Usually logs from test apps or from normal
* apps running on test devices will have this field set.
*
*/
public Builder setTestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
testId_ = value;
onChanged();
return this;
}
// optional sint64 timezone_offset_seconds = 15 [default = 180000];
private long timezoneOffsetSeconds_ = 180000L;
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public boolean hasTimezoneOffsetSeconds() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public long getTimezoneOffsetSeconds() {
return timezoneOffsetSeconds_;
}
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public Builder setTimezoneOffsetSeconds(long value) {
bitField0_ |= 0x00008000;
timezoneOffsetSeconds_ = value;
onChanged();
return this;
}
/**
* optional sint64 timezone_offset_seconds = 15 [default = 180000];
*
*
* User's timezone offset from UTC in seconds. If the user's UTC time
* is X millis then the on-device clock time would be
* X + timezone_offset_seconds * 1000.
* The default value is non-sensical, mainly so that 0 can be distinguished
* from "not specified" using proto nano (and without changing our build
* options to use reference types for primitives).
*
*/
public Builder clearTimezoneOffsetSeconds() {
bitField0_ = (bitField0_ & ~0x00008000);
timezoneOffsetSeconds_ = 180000L;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds experimentIds_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder> experimentIdsBuilder_;
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public boolean hasExperimentIds() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds getExperimentIds() {
if (experimentIdsBuilder_ == null) {
return experimentIds_;
} else {
return experimentIdsBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public Builder setExperimentIds(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds value) {
if (experimentIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
experimentIds_ = value;
onChanged();
} else {
experimentIdsBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public Builder setExperimentIds(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder builderForValue) {
if (experimentIdsBuilder_ == null) {
experimentIds_ = builderForValue.build();
onChanged();
} else {
experimentIdsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public Builder mergeExperimentIds(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds value) {
if (experimentIdsBuilder_ == null) {
if (((bitField0_ & 0x00010000) == 0x00010000) &&
experimentIds_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance()) {
experimentIds_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.newBuilder(experimentIds_).mergeFrom(value).buildPartial();
} else {
experimentIds_ = value;
}
onChanged();
} else {
experimentIdsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public Builder clearExperimentIds() {
if (experimentIdsBuilder_ == null) {
experimentIds_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance();
onChanged();
} else {
experimentIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00010000);
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder getExperimentIdsBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getExperimentIdsFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder getExperimentIdsOrBuilder() {
if (experimentIdsBuilder_ != null) {
return experimentIdsBuilder_.getMessageOrBuilder();
} else {
return experimentIds_;
}
}
/**
* optional .wireless_android_play_playlog.ExperimentIds experiment_ids = 16;
*
*
* serialized DeviceExperimentIds; the experiment ids are propagated
* to GWSLog experiment ids
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder>
getExperimentIdsFieldBuilder() {
if (experimentIdsBuilder_ == null) {
experimentIdsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder>(
experimentIds_,
getParentForChildren(),
isClean());
experimentIds_ = null;
}
return experimentIdsBuilder_;
}
// optional bytes client_ve = 18;
private com.google.protobuf.ByteString clientVe_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public boolean hasClientVe() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public com.google.protobuf.ByteString getClientVe() {
return clientVe_;
}
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public Builder setClientVe(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
clientVe_ = value;
onChanged();
return this;
}
/**
* optional bytes client_ve = 18;
*
*
* Serialized proto message of ClientVisualElementsProto as defined in
* logs/proto/visual_element/client_visual_elements.proto. The client can
* optionally send a log event with this field set. In this case, clearcut
* server needs to deserialize the bytes as a ClientVisualElementsProto and
* sets appropriate fields in the output GWSLogEntryProto.
*
* See http://go/NativeVeLogging for more information.
*
*/
public Builder clearClientVe() {
bitField0_ = (bitField0_ & ~0x00020000);
clientVe_ = getDefaultInstance().getClientVe();
onChanged();
return this;
}
// optional string client_ve_js = 24;
private java.lang.Object clientVeJs_ = "";
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public boolean hasClientVeJs() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public java.lang.String getClientVeJs() {
java.lang.Object ref = clientVeJs_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientVeJs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public com.google.protobuf.ByteString
getClientVeJsBytes() {
java.lang.Object ref = clientVeJs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientVeJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public Builder setClientVeJs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
clientVeJs_ = value;
onChanged();
return this;
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public Builder clearClientVeJs() {
bitField0_ = (bitField0_ & ~0x00040000);
clientVeJs_ = getDefaultInstance().getClientVeJs();
onChanged();
return this;
}
/**
* optional string client_ve_js = 24;
*
*
* Alternative serialized field for the client_ve field defined in 18.
* Serialize using array serialization format. More info at go/jspb.
*
*/
public Builder setClientVeJsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
clientVeJs_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent internalEvent_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent.NONE;
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public boolean hasInternalEvent() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent getInternalEvent() {
return internalEvent_;
}
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public Builder setInternalEvent(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00080000;
internalEvent_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.LogEvent.InternalEvent internal_event = 19;
*/
public Builder clearInternalEvent() {
bitField0_ = (bitField0_ & ~0x00080000);
internalEvent_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.InternalEvent.NONE;
onChanged();
return this;
}
// repeated int32 test_code = 20;
private java.util.List testCode_ = java.util.Collections.emptyList();
private void ensureTestCodeIsMutable() {
if (!((bitField0_ & 0x00100000) == 0x00100000)) {
testCode_ = new java.util.ArrayList(testCode_);
bitField0_ |= 0x00100000;
}
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public java.util.List
getTestCodeList() {
return java.util.Collections.unmodifiableList(testCode_);
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public int getTestCodeCount() {
return testCode_.size();
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public int getTestCode(int index) {
return testCode_.get(index);
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public Builder setTestCode(
int index, int value) {
ensureTestCodeIsMutable();
testCode_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public Builder addTestCode(int value) {
ensureTestCodeIsMutable();
testCode_.add(value);
onChanged();
return this;
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public Builder addAllTestCode(
java.lang.Iterable extends java.lang.Integer> values) {
ensureTestCodeIsMutable();
super.addAll(values, testCode_);
onChanged();
return this;
}
/**
* repeated int32 test_code = 20;
*
*
* Indicates that an interesting thing has occurred. Used to narrow the set
* of samples to consider in analysis. Test codes are copied to
* GwsLogEntryProto.TestCode.
*
* See google3/ads/base/test_codes/test_codes.proto
* for Ads' use of test codes.
*
*/
public Builder clearTestCode() {
testCode_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
// optional int64 boot_count = 22;
private long bootCount_ ;
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public boolean hasBootCount() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public long getBootCount() {
return bootCount_;
}
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public Builder setBootCount(long value) {
bitField0_ |= 0x00200000;
bootCount_ = value;
onChanged();
return this;
}
/**
* optional int64 boot_count = 22;
*
*
* Number of times the device is known to have booted. Taken together,
* {boot-count, uptime} pairs are a monotonically increasing counter which
* can be used to order events accross log sources.
*
*/
public Builder clearBootCount() {
bitField0_ = (bitField0_ & ~0x00200000);
bootCount_ = 0L;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo networkConnectionInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder> networkConnectionInfoBuilder_;
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public boolean hasNetworkConnectionInfo() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo getNetworkConnectionInfo() {
if (networkConnectionInfoBuilder_ == null) {
return networkConnectionInfo_;
} else {
return networkConnectionInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public Builder setNetworkConnectionInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo value) {
if (networkConnectionInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
networkConnectionInfo_ = value;
onChanged();
} else {
networkConnectionInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public Builder setNetworkConnectionInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder builderForValue) {
if (networkConnectionInfoBuilder_ == null) {
networkConnectionInfo_ = builderForValue.build();
onChanged();
} else {
networkConnectionInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public Builder mergeNetworkConnectionInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo value) {
if (networkConnectionInfoBuilder_ == null) {
if (((bitField0_ & 0x00400000) == 0x00400000) &&
networkConnectionInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance()) {
networkConnectionInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.newBuilder(networkConnectionInfo_).mergeFrom(value).buildPartial();
} else {
networkConnectionInfo_ = value;
}
onChanged();
} else {
networkConnectionInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public Builder clearNetworkConnectionInfo() {
if (networkConnectionInfoBuilder_ == null) {
networkConnectionInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance();
onChanged();
} else {
networkConnectionInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
return this;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder getNetworkConnectionInfoBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getNetworkConnectionInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder getNetworkConnectionInfoOrBuilder() {
if (networkConnectionInfoBuilder_ != null) {
return networkConnectionInfoBuilder_.getMessageOrBuilder();
} else {
return networkConnectionInfo_;
}
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo network_connection_info = 23;
*
*
* The current network connectivity info when the event was logged in the
* client
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder>
getNetworkConnectionInfoFieldBuilder() {
if (networkConnectionInfoBuilder_ == null) {
networkConnectionInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder>(
networkConnectionInfo_,
getParentForChildren(),
isClean());
networkConnectionInfo_ = null;
}
return networkConnectionInfoBuilder_;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.LogEvent)
}
static {
defaultInstance = new LogEvent(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.LogEvent)
}
public interface NetworkConnectionInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
boolean hasNetworkType();
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType getNetworkType();
}
/**
* Protobuf type {@code wireless_android_play_playlog.NetworkConnectionInfo}
*
*
* To describe the network connectivity of the client.
* Next tag: 2
*
*/
public static final class NetworkConnectionInfo extends
com.google.protobuf.GeneratedMessage
implements NetworkConnectionInfoOrBuilder {
// Use NetworkConnectionInfo.newBuilder() to construct.
private NetworkConnectionInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NetworkConnectionInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NetworkConnectionInfo defaultInstance;
public static NetworkConnectionInfo getDefaultInstance() {
return defaultInstance;
}
public NetworkConnectionInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NetworkConnectionInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
networkType_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_NetworkConnectionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NetworkConnectionInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NetworkConnectionInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.NetworkConnectionInfo.NetworkType}
*
*
* From android/frameworks/base/core/java/android/net/ConnectivityManager.java
* Note: data will only be recorded for internet connections. This means
* BLUETOOTH corresponds to when bluetooth was used for reverse tethering
* (i.e. using a computer's internet connection on the phone) and NOT for
* general device pairing via bluetooth, such as clockwork.
*
*/
public enum NetworkType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NONE = -1;
*/
NONE(0, -1),
/**
* MOBILE = 0;
*/
MOBILE(1, 0),
/**
* WIFI = 1;
*/
WIFI(2, 1),
/**
* MOBILE_MMS = 2;
*/
MOBILE_MMS(3, 2),
/**
* MOBILE_SUPL = 3;
*/
MOBILE_SUPL(4, 3),
/**
* MOBILE_DUN = 4;
*/
MOBILE_DUN(5, 4),
/**
* MOBILE_HIPRI = 5;
*/
MOBILE_HIPRI(6, 5),
/**
* WIMAX = 6;
*/
WIMAX(7, 6),
/**
* BLUETOOTH = 7;
*/
BLUETOOTH(8, 7),
/**
* DUMMY = 8;
*/
DUMMY(9, 8),
/**
* ETHERNET = 9;
*/
ETHERNET(10, 9),
/**
* MOBILE_FOTA = 10;
*/
MOBILE_FOTA(11, 10),
/**
* MOBILE_IMS = 11;
*/
MOBILE_IMS(12, 11),
/**
* MOBILE_CBS = 12;
*/
MOBILE_CBS(13, 12),
/**
* WIFI_P2P = 13;
*/
WIFI_P2P(14, 13),
/**
* MOBILE_IA = 14;
*/
MOBILE_IA(15, 14),
/**
* MOBILE_EMERGENCY = 15;
*/
MOBILE_EMERGENCY(16, 15),
/**
* PROXY = 16;
*/
PROXY(17, 16),
/**
* VPN = 17;
*/
VPN(18, 17),
;
/**
* NONE = -1;
*/
public static final int NONE_VALUE = -1;
/**
* MOBILE = 0;
*/
public static final int MOBILE_VALUE = 0;
/**
* WIFI = 1;
*/
public static final int WIFI_VALUE = 1;
/**
* MOBILE_MMS = 2;
*/
public static final int MOBILE_MMS_VALUE = 2;
/**
* MOBILE_SUPL = 3;
*/
public static final int MOBILE_SUPL_VALUE = 3;
/**
* MOBILE_DUN = 4;
*/
public static final int MOBILE_DUN_VALUE = 4;
/**
* MOBILE_HIPRI = 5;
*/
public static final int MOBILE_HIPRI_VALUE = 5;
/**
* WIMAX = 6;
*/
public static final int WIMAX_VALUE = 6;
/**
* BLUETOOTH = 7;
*/
public static final int BLUETOOTH_VALUE = 7;
/**
* DUMMY = 8;
*/
public static final int DUMMY_VALUE = 8;
/**
* ETHERNET = 9;
*/
public static final int ETHERNET_VALUE = 9;
/**
* MOBILE_FOTA = 10;
*/
public static final int MOBILE_FOTA_VALUE = 10;
/**
* MOBILE_IMS = 11;
*/
public static final int MOBILE_IMS_VALUE = 11;
/**
* MOBILE_CBS = 12;
*/
public static final int MOBILE_CBS_VALUE = 12;
/**
* WIFI_P2P = 13;
*/
public static final int WIFI_P2P_VALUE = 13;
/**
* MOBILE_IA = 14;
*/
public static final int MOBILE_IA_VALUE = 14;
/**
* MOBILE_EMERGENCY = 15;
*/
public static final int MOBILE_EMERGENCY_VALUE = 15;
/**
* PROXY = 16;
*/
public static final int PROXY_VALUE = 16;
/**
* VPN = 17;
*/
public static final int VPN_VALUE = 17;
public final int getNumber() { return value; }
public static NetworkType valueOf(int value) {
switch (value) {
case -1: return NONE;
case 0: return MOBILE;
case 1: return WIFI;
case 2: return MOBILE_MMS;
case 3: return MOBILE_SUPL;
case 4: return MOBILE_DUN;
case 5: return MOBILE_HIPRI;
case 6: return WIMAX;
case 7: return BLUETOOTH;
case 8: return DUMMY;
case 9: return ETHERNET;
case 10: return MOBILE_FOTA;
case 11: return MOBILE_IMS;
case 12: return MOBILE_CBS;
case 13: return WIFI_P2P;
case 14: return MOBILE_IA;
case 15: return MOBILE_EMERGENCY;
case 16: return PROXY;
case 17: return VPN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public NetworkType findValueByNumber(int number) {
return NetworkType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDescriptor().getEnumTypes().get(0);
}
private static final NetworkType[] VALUES = values();
public static NetworkType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private NetworkType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.NetworkConnectionInfo.NetworkType)
}
private int bitField0_;
// optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
public static final int NETWORK_TYPE_FIELD_NUMBER = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType networkType_;
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public boolean hasNetworkType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType getNetworkType() {
return networkType_;
}
private void initFields() {
networkType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType.NONE;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, networkType_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, networkType_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.NetworkConnectionInfo}
*
*
* To describe the network connectivity of the client.
* Next tag: 2
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_NetworkConnectionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
networkType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType.NONE;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.networkType_ = networkType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.getDefaultInstance()) return this;
if (other.hasNetworkType()) {
setNetworkType(other.getNetworkType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType networkType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType.NONE;
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public boolean hasNetworkType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType getNetworkType() {
return networkType_;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public Builder setNetworkType(com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
networkType_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.NetworkConnectionInfo.NetworkType network_type = 1 [default = NONE];
*
*
* The current network connectivity type when the event was logged in the
* client
*
*/
public Builder clearNetworkType() {
bitField0_ = (bitField0_ & ~0x00000001);
networkType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.NetworkConnectionInfo.NetworkType.NONE;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.NetworkConnectionInfo)
}
static {
defaultInstance = new NetworkConnectionInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.NetworkConnectionInfo)
}
public interface ExperimentIdsOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional bytes clear_blob = 1;
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
boolean hasClearBlob();
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
com.google.protobuf.ByteString getClearBlob();
// optional string clear_blob_js = 4;
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
boolean hasClearBlobJs();
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
java.lang.String getClearBlobJs();
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
com.google.protobuf.ByteString
getClearBlobJsBytes();
// repeated bytes encrypted_blob = 2;
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
java.util.List getEncryptedBlobList();
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
int getEncryptedBlobCount();
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
com.google.protobuf.ByteString getEncryptedBlob(int index);
// optional bool users_match = 3;
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
boolean hasUsersMatch();
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
boolean getUsersMatch();
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExperimentIds}
*
*
* The experiment ids, both in the clear and encrypted.
*
*/
public static final class ExperimentIds extends
com.google.protobuf.GeneratedMessage
implements ExperimentIdsOrBuilder {
// Use ExperimentIds.newBuilder() to construct.
private ExperimentIds(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ExperimentIds(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ExperimentIds defaultInstance;
public static ExperimentIds getDefaultInstance() {
return defaultInstance;
}
public ExperimentIds getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExperimentIds(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
clearBlob_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
encryptedBlob_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
encryptedBlob_.add(input.readBytes());
break;
}
case 24: {
bitField0_ |= 0x00000004;
usersMatch_ = input.readBool();
break;
}
case 34: {
bitField0_ |= 0x00000002;
clearBlobJs_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
encryptedBlob_ = java.util.Collections.unmodifiableList(encryptedBlob_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIds_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIds_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ExperimentIds parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExperimentIds(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional bytes clear_blob = 1;
public static final int CLEAR_BLOB_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString clearBlob_;
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public boolean hasClearBlob() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public com.google.protobuf.ByteString getClearBlob() {
return clearBlob_;
}
// optional string clear_blob_js = 4;
public static final int CLEAR_BLOB_JS_FIELD_NUMBER = 4;
private java.lang.Object clearBlobJs_;
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public boolean hasClearBlobJs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public java.lang.String getClearBlobJs() {
java.lang.Object ref = clearBlobJs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clearBlobJs_ = s;
}
return s;
}
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public com.google.protobuf.ByteString
getClearBlobJsBytes() {
java.lang.Object ref = clearBlobJs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clearBlobJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated bytes encrypted_blob = 2;
public static final int ENCRYPTED_BLOB_FIELD_NUMBER = 2;
private java.util.List encryptedBlob_;
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public java.util.List
getEncryptedBlobList() {
return encryptedBlob_;
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public int getEncryptedBlobCount() {
return encryptedBlob_.size();
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public com.google.protobuf.ByteString getEncryptedBlob(int index) {
return encryptedBlob_.get(index);
}
// optional bool users_match = 3;
public static final int USERS_MATCH_FIELD_NUMBER = 3;
private boolean usersMatch_;
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public boolean hasUsersMatch() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public boolean getUsersMatch() {
return usersMatch_;
}
private void initFields() {
clearBlob_ = com.google.protobuf.ByteString.EMPTY;
clearBlobJs_ = "";
encryptedBlob_ = java.util.Collections.emptyList();
usersMatch_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, clearBlob_);
}
for (int i = 0; i < encryptedBlob_.size(); i++) {
output.writeBytes(2, encryptedBlob_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, usersMatch_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(4, getClearBlobJsBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, clearBlob_);
}
{
int dataSize = 0;
for (int i = 0; i < encryptedBlob_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(encryptedBlob_.get(i));
}
size += dataSize;
size += 1 * getEncryptedBlobList().size();
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, usersMatch_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getClearBlobJsBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExperimentIds}
*
*
* The experiment ids, both in the clear and encrypted.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIds_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIds_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clearBlob_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
clearBlobJs_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
encryptedBlob_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
usersMatch_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIds_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clearBlob_ = clearBlob_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.clearBlobJs_ = clearBlobJs_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
encryptedBlob_ = java.util.Collections.unmodifiableList(encryptedBlob_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.encryptedBlob_ = encryptedBlob_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.usersMatch_ = usersMatch_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds.getDefaultInstance()) return this;
if (other.hasClearBlob()) {
setClearBlob(other.getClearBlob());
}
if (other.hasClearBlobJs()) {
bitField0_ |= 0x00000002;
clearBlobJs_ = other.clearBlobJs_;
onChanged();
}
if (!other.encryptedBlob_.isEmpty()) {
if (encryptedBlob_.isEmpty()) {
encryptedBlob_ = other.encryptedBlob_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureEncryptedBlobIsMutable();
encryptedBlob_.addAll(other.encryptedBlob_);
}
onChanged();
}
if (other.hasUsersMatch()) {
setUsersMatch(other.getUsersMatch());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIds) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional bytes clear_blob = 1;
private com.google.protobuf.ByteString clearBlob_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public boolean hasClearBlob() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public com.google.protobuf.ByteString getClearBlob() {
return clearBlob_;
}
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder setClearBlob(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clearBlob_ = value;
onChanged();
return this;
}
/**
* optional bytes clear_blob = 1;
*
*
* The client selected experiment ids in the clear (e.g. from Chrome).
* serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder clearClearBlob() {
bitField0_ = (bitField0_ & ~0x00000001);
clearBlob_ = getDefaultInstance().getClearBlob();
onChanged();
return this;
}
// optional string clear_blob_js = 4;
private java.lang.Object clearBlobJs_ = "";
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public boolean hasClearBlobJs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public java.lang.String getClearBlobJs() {
java.lang.Object ref = clearBlobJs_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clearBlobJs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public com.google.protobuf.ByteString
getClearBlobJsBytes() {
java.lang.Object ref = clearBlobJs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clearBlobJs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder setClearBlobJs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
clearBlobJs_ = value;
onChanged();
return this;
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder clearClearBlobJs() {
bitField0_ = (bitField0_ & ~0x00000002);
clearBlobJs_ = getDefaultInstance().getClearBlobJs();
onChanged();
return this;
}
/**
* optional string clear_blob_js = 4;
*
*
* A jspb version of clear_blob, using array serialization format.
* More info on JSPB at go/jspb.
*
*/
public Builder setClearBlobJsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
clearBlobJs_ = value;
onChanged();
return this;
}
// repeated bytes encrypted_blob = 2;
private java.util.List encryptedBlob_ = java.util.Collections.emptyList();
private void ensureEncryptedBlobIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
encryptedBlob_ = new java.util.ArrayList(encryptedBlob_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public java.util.List
getEncryptedBlobList() {
return java.util.Collections.unmodifiableList(encryptedBlob_);
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public int getEncryptedBlobCount() {
return encryptedBlob_.size();
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public com.google.protobuf.ByteString getEncryptedBlob(int index) {
return encryptedBlob_.get(index);
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder setEncryptedBlob(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureEncryptedBlobIsMutable();
encryptedBlob_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder addEncryptedBlob(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureEncryptedBlobIsMutable();
encryptedBlob_.add(value);
onChanged();
return this;
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder addAllEncryptedBlob(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureEncryptedBlobIsMutable();
super.addAll(values, encryptedBlob_);
onChanged();
return this;
}
/**
* repeated bytes encrypted_blob = 2;
*
*
* encrypted serialized experiments.heterodyne.DeviceExperimentIds
*
*/
public Builder clearEncryptedBlob() {
encryptedBlob_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
// optional bool users_match = 3;
private boolean usersMatch_ ;
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public boolean hasUsersMatch() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public boolean getUsersMatch() {
return usersMatch_;
}
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public Builder setUsersMatch(boolean value) {
bitField0_ |= 0x00000008;
usersMatch_ = value;
onChanged();
return this;
}
/**
* optional bool users_match = 3;
*
*
* indicates whether the Phenotype committed user matches the upload account
*
*/
public Builder clearUsersMatch() {
bitField0_ = (bitField0_ & ~0x00000008);
usersMatch_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.ExperimentIds)
}
static {
defaultInstance = new ExperimentIds(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.ExperimentIds)
}
public interface AndroidClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int64 android_id = 1;
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
boolean hasAndroidId();
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
long getAndroidId();
// optional string logging_id = 2;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
boolean hasLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
java.lang.String getLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
com.google.protobuf.ByteString
getLoggingIdBytes();
// optional int64 device_id = 18;
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
boolean hasDeviceId();
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
long getDeviceId();
// optional int32 sdk_version = 3;
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
boolean hasSdkVersion();
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
int getSdkVersion();
// optional string model = 4;
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
boolean hasModel();
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
java.lang.String getModel();
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
com.google.protobuf.ByteString
getModelBytes();
// optional string product = 5;
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
boolean hasProduct();
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
java.lang.String getProduct();
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
com.google.protobuf.ByteString
getProductBytes();
// optional string hardware = 8;
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
boolean hasHardware();
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
java.lang.String getHardware();
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
com.google.protobuf.ByteString
getHardwareBytes();
// optional string device = 9;
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
boolean hasDevice();
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
java.lang.String getDevice();
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
com.google.protobuf.ByteString
getDeviceBytes();
// optional string os_build = 6;
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
boolean hasOsBuild();
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
java.lang.String getOsBuild();
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
com.google.protobuf.ByteString
getOsBuildBytes();
// optional string application_build = 7;
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
boolean hasApplicationBuild();
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
java.lang.String getApplicationBuild();
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
com.google.protobuf.ByteString
getApplicationBuildBytes();
// optional string mcc_mnc = 10;
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
boolean hasMccMnc();
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
java.lang.String getMccMnc();
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
com.google.protobuf.ByteString
getMccMncBytes();
// optional string locale = 11;
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
boolean hasLocale();
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
java.lang.String getLocale();
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
com.google.protobuf.ByteString
getLocaleBytes();
// optional string country = 12;
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
boolean hasCountry();
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
java.lang.String getCountry();
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
com.google.protobuf.ByteString
getCountryBytes();
// optional string manufacturer = 13;
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
boolean hasManufacturer();
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
java.lang.String getManufacturer();
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
com.google.protobuf.ByteString
getManufacturerBytes();
// optional string brand = 14;
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
boolean hasBrand();
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
java.lang.String getBrand();
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
com.google.protobuf.ByteString
getBrandBytes();
// optional string board = 15;
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
boolean hasBoard();
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
java.lang.String getBoard();
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
com.google.protobuf.ByteString
getBoardBytes();
// optional string radio_version = 16;
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
boolean hasRadioVersion();
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
java.lang.String getRadioVersion();
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
com.google.protobuf.ByteString
getRadioVersionBytes();
// optional string fingerprint = 17;
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
boolean hasFingerprint();
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
java.lang.String getFingerprint();
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
com.google.protobuf.ByteString
getFingerprintBytes();
// optional int32 gms_core_version_code = 19;
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
boolean hasGmsCoreVersionCode();
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
int getGmsCoreVersionCode();
// optional bool is_sidewinder_device = 20;
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
boolean hasIsSidewinderDevice();
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
boolean getIsSidewinderDevice();
// optional bytes chimera_config_info = 21;
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
boolean hasChimeraConfigInfo();
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
com.google.protobuf.ByteString getChimeraConfigInfo();
// optional bool using_log_source_int = 22;
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
boolean hasUsingLogSourceInt();
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
boolean getUsingLogSourceInt();
}
/**
* Protobuf type {@code wireless_android_play_playlog.AndroidClientInfo}
*
*
* Attributes specific to Android clients.
*
* Next tag: 23
*
*/
public static final class AndroidClientInfo extends
com.google.protobuf.GeneratedMessage
implements AndroidClientInfoOrBuilder {
// Use AndroidClientInfo.newBuilder() to construct.
private AndroidClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private AndroidClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final AndroidClientInfo defaultInstance;
public static AndroidClientInfo getDefaultInstance() {
return defaultInstance;
}
public AndroidClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AndroidClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
androidId_ = input.readInt64();
break;
}
case 18: {
bitField0_ |= 0x00000002;
loggingId_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000008;
sdkVersion_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000010;
model_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000020;
product_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000100;
osBuild_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000200;
applicationBuild_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000040;
hardware_ = input.readBytes();
break;
}
case 74: {
bitField0_ |= 0x00000080;
device_ = input.readBytes();
break;
}
case 82: {
bitField0_ |= 0x00000400;
mccMnc_ = input.readBytes();
break;
}
case 90: {
bitField0_ |= 0x00000800;
locale_ = input.readBytes();
break;
}
case 98: {
bitField0_ |= 0x00001000;
country_ = input.readBytes();
break;
}
case 106: {
bitField0_ |= 0x00002000;
manufacturer_ = input.readBytes();
break;
}
case 114: {
bitField0_ |= 0x00004000;
brand_ = input.readBytes();
break;
}
case 122: {
bitField0_ |= 0x00008000;
board_ = input.readBytes();
break;
}
case 130: {
bitField0_ |= 0x00010000;
radioVersion_ = input.readBytes();
break;
}
case 138: {
bitField0_ |= 0x00020000;
fingerprint_ = input.readBytes();
break;
}
case 144: {
bitField0_ |= 0x00000004;
deviceId_ = input.readInt64();
break;
}
case 152: {
bitField0_ |= 0x00040000;
gmsCoreVersionCode_ = input.readInt32();
break;
}
case 160: {
bitField0_ |= 0x00080000;
isSidewinderDevice_ = input.readBool();
break;
}
case 170: {
bitField0_ |= 0x00100000;
chimeraConfigInfo_ = input.readBytes();
break;
}
case 176: {
bitField0_ |= 0x00200000;
usingLogSourceInt_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AndroidClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public AndroidClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AndroidClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int64 android_id = 1;
public static final int ANDROID_ID_FIELD_NUMBER = 1;
private long androidId_;
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public boolean hasAndroidId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public long getAndroidId() {
return androidId_;
}
// optional string logging_id = 2;
public static final int LOGGING_ID_FIELD_NUMBER = 2;
private java.lang.Object loggingId_;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loggingId_ = s;
}
return s;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int64 device_id = 18;
public static final int DEVICE_ID_FIELD_NUMBER = 18;
private long deviceId_;
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public long getDeviceId() {
return deviceId_;
}
// optional int32 sdk_version = 3;
public static final int SDK_VERSION_FIELD_NUMBER = 3;
private int sdkVersion_;
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public boolean hasSdkVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public int getSdkVersion() {
return sdkVersion_;
}
// optional string model = 4;
public static final int MODEL_FIELD_NUMBER = 4;
private java.lang.Object model_;
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
model_ = s;
}
return s;
}
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string product = 5;
public static final int PRODUCT_FIELD_NUMBER = 5;
private java.lang.Object product_;
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public boolean hasProduct() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public java.lang.String getProduct() {
java.lang.Object ref = product_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
product_ = s;
}
return s;
}
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public com.google.protobuf.ByteString
getProductBytes() {
java.lang.Object ref = product_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
product_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string hardware = 8;
public static final int HARDWARE_FIELD_NUMBER = 8;
private java.lang.Object hardware_;
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public boolean hasHardware() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public java.lang.String getHardware() {
java.lang.Object ref = hardware_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hardware_ = s;
}
return s;
}
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public com.google.protobuf.ByteString
getHardwareBytes() {
java.lang.Object ref = hardware_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hardware_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string device = 9;
public static final int DEVICE_FIELD_NUMBER = 9;
private java.lang.Object device_;
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public boolean hasDevice() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
device_ = s;
}
return s;
}
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_build = 6;
public static final int OS_BUILD_FIELD_NUMBER = 6;
private java.lang.Object osBuild_;
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public boolean hasOsBuild() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public java.lang.String getOsBuild() {
java.lang.Object ref = osBuild_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osBuild_ = s;
}
return s;
}
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public com.google.protobuf.ByteString
getOsBuildBytes() {
java.lang.Object ref = osBuild_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string application_build = 7;
public static final int APPLICATION_BUILD_FIELD_NUMBER = 7;
private java.lang.Object applicationBuild_;
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
applicationBuild_ = s;
}
return s;
}
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string mcc_mnc = 10;
public static final int MCC_MNC_FIELD_NUMBER = 10;
private java.lang.Object mccMnc_;
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public boolean hasMccMnc() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public java.lang.String getMccMnc() {
java.lang.Object ref = mccMnc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mccMnc_ = s;
}
return s;
}
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public com.google.protobuf.ByteString
getMccMncBytes() {
java.lang.Object ref = mccMnc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mccMnc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string locale = 11;
public static final int LOCALE_FIELD_NUMBER = 11;
private java.lang.Object locale_;
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
locale_ = s;
}
return s;
}
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string country = 12;
public static final int COUNTRY_FIELD_NUMBER = 12;
private java.lang.Object country_;
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string manufacturer = 13;
public static final int MANUFACTURER_FIELD_NUMBER = 13;
private java.lang.Object manufacturer_;
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public boolean hasManufacturer() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
manufacturer_ = s;
}
return s;
}
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string brand = 14;
public static final int BRAND_FIELD_NUMBER = 14;
private java.lang.Object brand_;
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public boolean hasBrand() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public java.lang.String getBrand() {
java.lang.Object ref = brand_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
brand_ = s;
}
return s;
}
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public com.google.protobuf.ByteString
getBrandBytes() {
java.lang.Object ref = brand_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
brand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string board = 15;
public static final int BOARD_FIELD_NUMBER = 15;
private java.lang.Object board_;
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public boolean hasBoard() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public java.lang.String getBoard() {
java.lang.Object ref = board_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
board_ = s;
}
return s;
}
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public com.google.protobuf.ByteString
getBoardBytes() {
java.lang.Object ref = board_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
board_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string radio_version = 16;
public static final int RADIO_VERSION_FIELD_NUMBER = 16;
private java.lang.Object radioVersion_;
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public boolean hasRadioVersion() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public java.lang.String getRadioVersion() {
java.lang.Object ref = radioVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
radioVersion_ = s;
}
return s;
}
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public com.google.protobuf.ByteString
getRadioVersionBytes() {
java.lang.Object ref = radioVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
radioVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string fingerprint = 17;
public static final int FINGERPRINT_FIELD_NUMBER = 17;
private java.lang.Object fingerprint_;
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fingerprint_ = s;
}
return s;
}
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional int32 gms_core_version_code = 19;
public static final int GMS_CORE_VERSION_CODE_FIELD_NUMBER = 19;
private int gmsCoreVersionCode_;
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public boolean hasGmsCoreVersionCode() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public int getGmsCoreVersionCode() {
return gmsCoreVersionCode_;
}
// optional bool is_sidewinder_device = 20;
public static final int IS_SIDEWINDER_DEVICE_FIELD_NUMBER = 20;
private boolean isSidewinderDevice_;
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public boolean hasIsSidewinderDevice() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public boolean getIsSidewinderDevice() {
return isSidewinderDevice_;
}
// optional bytes chimera_config_info = 21;
public static final int CHIMERA_CONFIG_INFO_FIELD_NUMBER = 21;
private com.google.protobuf.ByteString chimeraConfigInfo_;
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public boolean hasChimeraConfigInfo() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public com.google.protobuf.ByteString getChimeraConfigInfo() {
return chimeraConfigInfo_;
}
// optional bool using_log_source_int = 22;
public static final int USING_LOG_SOURCE_INT_FIELD_NUMBER = 22;
private boolean usingLogSourceInt_;
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public boolean hasUsingLogSourceInt() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public boolean getUsingLogSourceInt() {
return usingLogSourceInt_;
}
private void initFields() {
androidId_ = 0L;
loggingId_ = "";
deviceId_ = 0L;
sdkVersion_ = 0;
model_ = "";
product_ = "";
hardware_ = "";
device_ = "";
osBuild_ = "";
applicationBuild_ = "";
mccMnc_ = "";
locale_ = "";
country_ = "";
manufacturer_ = "";
brand_ = "";
board_ = "";
radioVersion_ = "";
fingerprint_ = "";
gmsCoreVersionCode_ = 0;
isSidewinderDevice_ = false;
chimeraConfigInfo_ = com.google.protobuf.ByteString.EMPTY;
usingLogSourceInt_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, androidId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(3, sdkVersion_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(4, getModelBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(5, getProductBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBytes(6, getOsBuildBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(7, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(8, getHardwareBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(9, getDeviceBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBytes(10, getMccMncBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBytes(11, getLocaleBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBytes(12, getCountryBytes());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBytes(13, getManufacturerBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBytes(14, getBrandBytes());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBytes(15, getBoardBytes());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeBytes(16, getRadioVersionBytes());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeBytes(17, getFingerprintBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(18, deviceId_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeInt32(19, gmsCoreVersionCode_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeBool(20, isSidewinderDevice_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeBytes(21, chimeraConfigInfo_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeBool(22, usingLogSourceInt_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, androidId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, sdkVersion_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getModelBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getProductBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getOsBuildBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getHardwareBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, getDeviceBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, getMccMncBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, getLocaleBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, getCountryBytes());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, getManufacturerBytes());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(14, getBrandBytes());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, getBoardBytes());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(16, getRadioVersionBytes());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(17, getFingerprintBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, deviceId_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, gmsCoreVersionCode_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(20, isSidewinderDevice_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(21, chimeraConfigInfo_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(22, usingLogSourceInt_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.AndroidClientInfo}
*
*
* Attributes specific to Android clients.
*
* Next tag: 23
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AndroidClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
androidId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
loggingId_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
deviceId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
sdkVersion_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
model_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
product_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
hardware_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
device_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
osBuild_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
applicationBuild_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
mccMnc_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
locale_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
country_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
manufacturer_ = "";
bitField0_ = (bitField0_ & ~0x00002000);
brand_ = "";
bitField0_ = (bitField0_ & ~0x00004000);
board_ = "";
bitField0_ = (bitField0_ & ~0x00008000);
radioVersion_ = "";
bitField0_ = (bitField0_ & ~0x00010000);
fingerprint_ = "";
bitField0_ = (bitField0_ & ~0x00020000);
gmsCoreVersionCode_ = 0;
bitField0_ = (bitField0_ & ~0x00040000);
isSidewinderDevice_ = false;
bitField0_ = (bitField0_ & ~0x00080000);
chimeraConfigInfo_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00100000);
usingLogSourceInt_ = false;
bitField0_ = (bitField0_ & ~0x00200000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.androidId_ = androidId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.loggingId_ = loggingId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.deviceId_ = deviceId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.sdkVersion_ = sdkVersion_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.model_ = model_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.product_ = product_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.hardware_ = hardware_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.device_ = device_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.osBuild_ = osBuild_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.applicationBuild_ = applicationBuild_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.mccMnc_ = mccMnc_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.locale_ = locale_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.country_ = country_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00002000;
}
result.manufacturer_ = manufacturer_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00004000;
}
result.brand_ = brand_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00008000;
}
result.board_ = board_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00010000;
}
result.radioVersion_ = radioVersion_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00020000;
}
result.fingerprint_ = fingerprint_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00040000;
}
result.gmsCoreVersionCode_ = gmsCoreVersionCode_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00080000;
}
result.isSidewinderDevice_ = isSidewinderDevice_;
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00100000;
}
result.chimeraConfigInfo_ = chimeraConfigInfo_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00200000;
}
result.usingLogSourceInt_ = usingLogSourceInt_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance()) return this;
if (other.hasAndroidId()) {
setAndroidId(other.getAndroidId());
}
if (other.hasLoggingId()) {
bitField0_ |= 0x00000002;
loggingId_ = other.loggingId_;
onChanged();
}
if (other.hasDeviceId()) {
setDeviceId(other.getDeviceId());
}
if (other.hasSdkVersion()) {
setSdkVersion(other.getSdkVersion());
}
if (other.hasModel()) {
bitField0_ |= 0x00000010;
model_ = other.model_;
onChanged();
}
if (other.hasProduct()) {
bitField0_ |= 0x00000020;
product_ = other.product_;
onChanged();
}
if (other.hasHardware()) {
bitField0_ |= 0x00000040;
hardware_ = other.hardware_;
onChanged();
}
if (other.hasDevice()) {
bitField0_ |= 0x00000080;
device_ = other.device_;
onChanged();
}
if (other.hasOsBuild()) {
bitField0_ |= 0x00000100;
osBuild_ = other.osBuild_;
onChanged();
}
if (other.hasApplicationBuild()) {
bitField0_ |= 0x00000200;
applicationBuild_ = other.applicationBuild_;
onChanged();
}
if (other.hasMccMnc()) {
bitField0_ |= 0x00000400;
mccMnc_ = other.mccMnc_;
onChanged();
}
if (other.hasLocale()) {
bitField0_ |= 0x00000800;
locale_ = other.locale_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00001000;
country_ = other.country_;
onChanged();
}
if (other.hasManufacturer()) {
bitField0_ |= 0x00002000;
manufacturer_ = other.manufacturer_;
onChanged();
}
if (other.hasBrand()) {
bitField0_ |= 0x00004000;
brand_ = other.brand_;
onChanged();
}
if (other.hasBoard()) {
bitField0_ |= 0x00008000;
board_ = other.board_;
onChanged();
}
if (other.hasRadioVersion()) {
bitField0_ |= 0x00010000;
radioVersion_ = other.radioVersion_;
onChanged();
}
if (other.hasFingerprint()) {
bitField0_ |= 0x00020000;
fingerprint_ = other.fingerprint_;
onChanged();
}
if (other.hasGmsCoreVersionCode()) {
setGmsCoreVersionCode(other.getGmsCoreVersionCode());
}
if (other.hasIsSidewinderDevice()) {
setIsSidewinderDevice(other.getIsSidewinderDevice());
}
if (other.hasChimeraConfigInfo()) {
setChimeraConfigInfo(other.getChimeraConfigInfo());
}
if (other.hasUsingLogSourceInt()) {
setUsingLogSourceInt(other.getUsingLogSourceInt());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 android_id = 1;
private long androidId_ ;
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public boolean hasAndroidId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public long getAndroidId() {
return androidId_;
}
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public Builder setAndroidId(long value) {
bitField0_ |= 0x00000001;
androidId_ = value;
onChanged();
return this;
}
/**
* optional int64 android_id = 1;
*
*
* "Gservices" android ID. Considered PII. Never logged in archival logs.
*
*/
public Builder clearAndroidId() {
bitField0_ = (bitField0_ & ~0x00000001);
androidId_ = 0L;
onChanged();
return this;
}
// optional string logging_id = 2;
private java.lang.Object loggingId_ = "";
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
loggingId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder clearLoggingId() {
bitField0_ = (bitField0_ & ~0x00000002);
loggingId_ = getDefaultInstance().getLoggingId();
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
// optional int64 device_id = 18;
private long deviceId_ ;
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public long getDeviceId() {
return deviceId_;
}
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public Builder setDeviceId(long value) {
bitField0_ |= 0x00000004;
deviceId_ = value;
onChanged();
return this;
}
/**
* optional int64 device_id = 18;
*
*
* Device ID referenced by android.provider.Settings.Secure.ANDROID_ID.
* Considered PII. Never logged in archival logs.
*
*/
public Builder clearDeviceId() {
bitField0_ = (bitField0_ & ~0x00000004);
deviceId_ = 0L;
onChanged();
return this;
}
// optional int32 sdk_version = 3;
private int sdkVersion_ ;
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public boolean hasSdkVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public int getSdkVersion() {
return sdkVersion_;
}
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public Builder setSdkVersion(int value) {
bitField0_ |= 0x00000008;
sdkVersion_ = value;
onChanged();
return this;
}
/**
* optional int32 sdk_version = 3;
*
*
* This comes from android.os.Build.VERSION.SDK_INT.
*
*/
public Builder clearSdkVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
sdkVersion_ = 0;
onChanged();
return this;
}
// optional string model = 4;
private java.lang.Object model_ = "";
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
model_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public Builder setModel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
model_ = value;
onChanged();
return this;
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public Builder clearModel() {
bitField0_ = (bitField0_ & ~0x00000010);
model_ = getDefaultInstance().getModel();
onChanged();
return this;
}
/**
* optional string model = 4;
*
*
* Textual description of the client platform. e.g., "Nexus 4".
* This comes from android.os.Build.MODEL.
*
*/
public Builder setModelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
model_ = value;
onChanged();
return this;
}
// optional string product = 5;
private java.lang.Object product_ = "";
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public boolean hasProduct() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public java.lang.String getProduct() {
java.lang.Object ref = product_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
product_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public com.google.protobuf.ByteString
getProductBytes() {
java.lang.Object ref = product_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
product_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public Builder setProduct(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
product_ = value;
onChanged();
return this;
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public Builder clearProduct() {
bitField0_ = (bitField0_ & ~0x00000020);
product_ = getDefaultInstance().getProduct();
onChanged();
return this;
}
/**
* optional string product = 5;
*
*
* The name of the overall product. e.g., "occam".
* This comes from android.os.Build.Product.
*
*/
public Builder setProductBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
product_ = value;
onChanged();
return this;
}
// optional string hardware = 8;
private java.lang.Object hardware_ = "";
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public boolean hasHardware() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public java.lang.String getHardware() {
java.lang.Object ref = hardware_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
hardware_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public com.google.protobuf.ByteString
getHardwareBytes() {
java.lang.Object ref = hardware_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hardware_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public Builder setHardware(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
hardware_ = value;
onChanged();
return this;
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public Builder clearHardware() {
bitField0_ = (bitField0_ & ~0x00000040);
hardware_ = getDefaultInstance().getHardware();
onChanged();
return this;
}
/**
* optional string hardware = 8;
*
*
* The name of the hardware (from the kernel command line or /proc).
* This comes from android.os.Build.Hardware. e.g., "mako".
*
*/
public Builder setHardwareBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
hardware_ = value;
onChanged();
return this;
}
// optional string device = 9;
private java.lang.Object device_ = "";
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public boolean hasDevice() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
device_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public Builder setDevice(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
device_ = value;
onChanged();
return this;
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public Builder clearDevice() {
bitField0_ = (bitField0_ & ~0x00000080);
device_ = getDefaultInstance().getDevice();
onChanged();
return this;
}
/**
* optional string device = 9;
*
*
* The name of the industrial design. e.g., "mako".
* This comes from android.os.Build.Device.
*
*/
public Builder setDeviceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
device_ = value;
onChanged();
return this;
}
// optional string os_build = 6;
private java.lang.Object osBuild_ = "";
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public boolean hasOsBuild() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public java.lang.String getOsBuild() {
java.lang.Object ref = osBuild_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osBuild_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public com.google.protobuf.ByteString
getOsBuildBytes() {
java.lang.Object ref = osBuild_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public Builder setOsBuild(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
osBuild_ = value;
onChanged();
return this;
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public Builder clearOsBuild() {
bitField0_ = (bitField0_ & ~0x00000100);
osBuild_ = getDefaultInstance().getOsBuild();
onChanged();
return this;
}
/**
* optional string os_build = 6;
*
*
* This comes from android.os.Build.ID. e.g., something like "JRN54F".
*
*/
public Builder setOsBuildBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
osBuild_ = value;
onChanged();
return this;
}
// optional string application_build = 7;
private java.lang.Object applicationBuild_ = "";
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
applicationBuild_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public Builder setApplicationBuild(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
applicationBuild_ = value;
onChanged();
return this;
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public Builder clearApplicationBuild() {
bitField0_ = (bitField0_ & ~0x00000200);
applicationBuild_ = getDefaultInstance().getApplicationBuild();
onChanged();
return this;
}
/**
* optional string application_build = 7;
*
*
* The client application version. The java int version in the android package
* converted to string.
*
*/
public Builder setApplicationBuildBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
applicationBuild_ = value;
onChanged();
return this;
}
// optional string mcc_mnc = 10;
private java.lang.Object mccMnc_ = "";
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public boolean hasMccMnc() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public java.lang.String getMccMnc() {
java.lang.Object ref = mccMnc_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
mccMnc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public com.google.protobuf.ByteString
getMccMncBytes() {
java.lang.Object ref = mccMnc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mccMnc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public Builder setMccMnc(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
mccMnc_ = value;
onChanged();
return this;
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public Builder clearMccMnc() {
bitField0_ = (bitField0_ & ~0x00000400);
mccMnc_ = getDefaultInstance().getMccMnc();
onChanged();
return this;
}
/**
* optional string mcc_mnc = 10;
*
*
* The mobile country code / mobile network code (MCC/MNC).
* e.g., 310004 for Verizon USA.
*
*/
public Builder setMccMncBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
mccMnc_ = value;
onChanged();
return this;
}
// optional string locale = 11;
private java.lang.Object locale_ = "";
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
locale_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public Builder setLocale(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
locale_ = value;
onChanged();
return this;
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public Builder clearLocale() {
bitField0_ = (bitField0_ & ~0x00000800);
locale_ = getDefaultInstance().getLocale();
onChanged();
return this;
}
/**
* optional string locale = 11;
*
*
* The chosen locale from the client. e.g., "en_US", "ko_KR", "en_GB".
*
*/
public Builder setLocaleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
locale_ = value;
onChanged();
return this;
}
// optional string country = 12;
private java.lang.Object country_ = "";
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00001000);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 12;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
country_ = value;
onChanged();
return this;
}
// optional string manufacturer = 13;
private java.lang.Object manufacturer_ = "";
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public boolean hasManufacturer() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
manufacturer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public Builder setManufacturer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
manufacturer_ = value;
onChanged();
return this;
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public Builder clearManufacturer() {
bitField0_ = (bitField0_ & ~0x00002000);
manufacturer_ = getDefaultInstance().getManufacturer();
onChanged();
return this;
}
/**
* optional string manufacturer = 13;
*
*
* The manufacturer of the hardware.
* This comes from android.os.Build.MANUFACTURER
*
*/
public Builder setManufacturerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
manufacturer_ = value;
onChanged();
return this;
}
// optional string brand = 14;
private java.lang.Object brand_ = "";
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public boolean hasBrand() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public java.lang.String getBrand() {
java.lang.Object ref = brand_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
brand_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public com.google.protobuf.ByteString
getBrandBytes() {
java.lang.Object ref = brand_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
brand_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public Builder setBrand(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
brand_ = value;
onChanged();
return this;
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public Builder clearBrand() {
bitField0_ = (bitField0_ & ~0x00004000);
brand_ = getDefaultInstance().getBrand();
onChanged();
return this;
}
/**
* optional string brand = 14;
*
*
* The brand the software is customized for, if any. Often a carrier name.
* e.g. "google"
* from http://developer.android.com/reference/android/os/Build.html#BRAND
*
*/
public Builder setBrandBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
brand_ = value;
onChanged();
return this;
}
// optional string board = 15;
private java.lang.Object board_ = "";
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public boolean hasBoard() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public java.lang.String getBoard() {
java.lang.Object ref = board_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
board_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public com.google.protobuf.ByteString
getBoardBytes() {
java.lang.Object ref = board_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
board_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public Builder setBoard(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
board_ = value;
onChanged();
return this;
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public Builder clearBoard() {
bitField0_ = (bitField0_ & ~0x00008000);
board_ = getDefaultInstance().getBoard();
onChanged();
return this;
}
/**
* optional string board = 15;
*
*
* The name of the underlying board
* e.g. "tuna"
* from http://developer.android.com/reference/android/os/Build.html#BOARD
*
*/
public Builder setBoardBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
board_ = value;
onChanged();
return this;
}
// optional string radio_version = 16;
private java.lang.Object radioVersion_ = "";
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public boolean hasRadioVersion() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public java.lang.String getRadioVersion() {
java.lang.Object ref = radioVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
radioVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public com.google.protobuf.ByteString
getRadioVersionBytes() {
java.lang.Object ref = radioVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
radioVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public Builder setRadioVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
radioVersion_ = value;
onChanged();
return this;
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public Builder clearRadioVersion() {
bitField0_ = (bitField0_ & ~0x00010000);
radioVersion_ = getDefaultInstance().getRadioVersion();
onChanged();
return this;
}
/**
* optional string radio_version = 16;
*
*
* Radio version as reported by device, if available at reporting time
* e.g. "I9250XXLJ1"
* from http://developer.android.com/reference/android/os/Build.html#getRadioVersion()
*
*/
public Builder setRadioVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
radioVersion_ = value;
onChanged();
return this;
}
// optional string fingerprint = 17;
private java.lang.Object fingerprint_ = "";
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public Builder setFingerprint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
fingerprint_ = value;
onChanged();
return this;
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public Builder clearFingerprint() {
bitField0_ = (bitField0_ & ~0x00020000);
fingerprint_ = getDefaultInstance().getFingerprint();
onChanged();
return this;
}
/**
* optional string fingerprint = 17;
*
*
* Device model/build fingerprint.
* This comes from android.os.Build.FINGERPRINT.
*
*/
public Builder setFingerprintBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
fingerprint_ = value;
onChanged();
return this;
}
// optional int32 gms_core_version_code = 19;
private int gmsCoreVersionCode_ ;
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public boolean hasGmsCoreVersionCode() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public int getGmsCoreVersionCode() {
return gmsCoreVersionCode_;
}
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public Builder setGmsCoreVersionCode(int value) {
bitField0_ |= 0x00040000;
gmsCoreVersionCode_ = value;
onChanged();
return this;
}
/**
* optional int32 gms_core_version_code = 19;
*
*
* android.content.pm.PackageInfo.versionCode of com.google.android.gms.
* e.g., 6031030
*
*/
public Builder clearGmsCoreVersionCode() {
bitField0_ = (bitField0_ & ~0x00040000);
gmsCoreVersionCode_ = 0;
onChanged();
return this;
}
// optional bool is_sidewinder_device = 20;
private boolean isSidewinderDevice_ ;
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public boolean hasIsSidewinderDevice() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public boolean getIsSidewinderDevice() {
return isSidewinderDevice_;
}
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public Builder setIsSidewinderDevice(boolean value) {
bitField0_ |= 0x00080000;
isSidewinderDevice_ = value;
onChanged();
return this;
}
/**
* optional bool is_sidewinder_device = 20;
*
*
* Whether the device is a Sidewinder device. See go/sidewinder-summary.
*
*/
public Builder clearIsSidewinderDevice() {
bitField0_ = (bitField0_ & ~0x00080000);
isSidewinderDevice_ = false;
onChanged();
return this;
}
// optional bytes chimera_config_info = 21;
private com.google.protobuf.ByteString chimeraConfigInfo_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public boolean hasChimeraConfigInfo() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public com.google.protobuf.ByteString getChimeraConfigInfo() {
return chimeraConfigInfo_;
}
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public Builder setChimeraConfigInfo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00100000;
chimeraConfigInfo_ = value;
onChanged();
return this;
}
/**
* optional bytes chimera_config_info = 21;
*
*
* The Chimera module config info describing the module sets on the device
* when the event is logged in the client.
* This is a proto of ChimeraConfigInfo residing in
* google3/logs/proto/wireless/android/play/playlog/chimera_modules.proto.
*
*/
public Builder clearChimeraConfigInfo() {
bitField0_ = (bitField0_ & ~0x00100000);
chimeraConfigInfo_ = getDefaultInstance().getChimeraConfigInfo();
onChanged();
return this;
}
// optional bool using_log_source_int = 22;
private boolean usingLogSourceInt_ ;
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public boolean hasUsingLogSourceInt() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public boolean getUsingLogSourceInt() {
return usingLogSourceInt_;
}
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public Builder setUsingLogSourceInt(boolean value) {
bitField0_ |= 0x00200000;
usingLogSourceInt_ = value;
onChanged();
return this;
}
/**
* optional bool using_log_source_int = 22;
*
*
* Indicates if the device is passing in the LogRequest.LogSource integer
* value instead of passing in a string for log_source_name.
* This field is not set by the client, but by the server after parsing the
* request.
*
*/
public Builder clearUsingLogSourceInt() {
bitField0_ = (bitField0_ & ~0x00200000);
usingLogSourceInt_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.AndroidClientInfo)
}
static {
defaultInstance = new AndroidClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.AndroidClientInfo)
}
public interface BrowserInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string locale = 1;
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
boolean hasLocale();
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
java.lang.String getLocale();
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
com.google.protobuf.ByteString
getLocaleBytes();
// optional string browser = 2;
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
boolean hasBrowser();
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
java.lang.String getBrowser();
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
com.google.protobuf.ByteString
getBrowserBytes();
// optional string browser_version = 3;
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
boolean hasBrowserVersion();
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
java.lang.String getBrowserVersion();
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
com.google.protobuf.ByteString
getBrowserVersionBytes();
// optional string flash_version = 4;
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
boolean hasFlashVersion();
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
java.lang.String getFlashVersion();
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
com.google.protobuf.ByteString
getFlashVersionBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.BrowserInfo}
*
*
* Attributes specific to web/mobile browsers.
*
* Next tag: 5
*
*/
public static final class BrowserInfo extends
com.google.protobuf.GeneratedMessage
implements BrowserInfoOrBuilder {
// Use BrowserInfo.newBuilder() to construct.
private BrowserInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private BrowserInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final BrowserInfo defaultInstance;
public static BrowserInfo getDefaultInstance() {
return defaultInstance;
}
public BrowserInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BrowserInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
locale_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
browser_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
browserVersion_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
flashVersion_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BrowserInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BrowserInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public BrowserInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BrowserInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string locale = 1;
public static final int LOCALE_FIELD_NUMBER = 1;
private java.lang.Object locale_;
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
locale_ = s;
}
return s;
}
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string browser = 2;
public static final int BROWSER_FIELD_NUMBER = 2;
private java.lang.Object browser_;
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public boolean hasBrowser() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public java.lang.String getBrowser() {
java.lang.Object ref = browser_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
browser_ = s;
}
return s;
}
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public com.google.protobuf.ByteString
getBrowserBytes() {
java.lang.Object ref = browser_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
browser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string browser_version = 3;
public static final int BROWSER_VERSION_FIELD_NUMBER = 3;
private java.lang.Object browserVersion_;
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public boolean hasBrowserVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public java.lang.String getBrowserVersion() {
java.lang.Object ref = browserVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
browserVersion_ = s;
}
return s;
}
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public com.google.protobuf.ByteString
getBrowserVersionBytes() {
java.lang.Object ref = browserVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
browserVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string flash_version = 4;
public static final int FLASH_VERSION_FIELD_NUMBER = 4;
private java.lang.Object flashVersion_;
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public boolean hasFlashVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public java.lang.String getFlashVersion() {
java.lang.Object ref = flashVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
flashVersion_ = s;
}
return s;
}
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public com.google.protobuf.ByteString
getFlashVersionBytes() {
java.lang.Object ref = flashVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
flashVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
locale_ = "";
browser_ = "";
browserVersion_ = "";
flashVersion_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getLocaleBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getBrowserBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getBrowserVersionBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getFlashVersionBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getLocaleBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getBrowserBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getBrowserVersionBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getFlashVersionBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.BrowserInfo}
*
*
* Attributes specific to web/mobile browsers.
*
* Next tag: 5
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BrowserInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BrowserInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
locale_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
browser_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
browserVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
flashVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BrowserInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.locale_ = locale_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.browser_ = browser_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.browserVersion_ = browserVersion_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.flashVersion_ = flashVersion_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance()) return this;
if (other.hasLocale()) {
bitField0_ |= 0x00000001;
locale_ = other.locale_;
onChanged();
}
if (other.hasBrowser()) {
bitField0_ |= 0x00000002;
browser_ = other.browser_;
onChanged();
}
if (other.hasBrowserVersion()) {
bitField0_ |= 0x00000004;
browserVersion_ = other.browserVersion_;
onChanged();
}
if (other.hasFlashVersion()) {
bitField0_ |= 0x00000008;
flashVersion_ = other.flashVersion_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string locale = 1;
private java.lang.Object locale_ = "";
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
locale_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public Builder setLocale(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
locale_ = value;
onChanged();
return this;
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public Builder clearLocale() {
bitField0_ = (bitField0_ & ~0x00000001);
locale_ = getDefaultInstance().getLocale();
onChanged();
return this;
}
/**
* optional string locale = 1;
*
*
* Locale of the browser. Usually the same as the Accept-Language header but
* can be overridden by the hl query-string parameter.
*
*/
public Builder setLocaleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
locale_ = value;
onChanged();
return this;
}
// optional string browser = 2;
private java.lang.Object browser_ = "";
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public boolean hasBrowser() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public java.lang.String getBrowser() {
java.lang.Object ref = browser_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
browser_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public com.google.protobuf.ByteString
getBrowserBytes() {
java.lang.Object ref = browser_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
browser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public Builder setBrowser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
browser_ = value;
onChanged();
return this;
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public Builder clearBrowser() {
bitField0_ = (bitField0_ & ~0x00000002);
browser_ = getDefaultInstance().getBrowser();
onChanged();
return this;
}
/**
* optional string browser = 2;
*
*
* Name of the browser. This comes from analytics.browserInfo.NAME.
*
*/
public Builder setBrowserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
browser_ = value;
onChanged();
return this;
}
// optional string browser_version = 3;
private java.lang.Object browserVersion_ = "";
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public boolean hasBrowserVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public java.lang.String getBrowserVersion() {
java.lang.Object ref = browserVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
browserVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public com.google.protobuf.ByteString
getBrowserVersionBytes() {
java.lang.Object ref = browserVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
browserVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public Builder setBrowserVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
browserVersion_ = value;
onChanged();
return this;
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public Builder clearBrowserVersion() {
bitField0_ = (bitField0_ & ~0x00000004);
browserVersion_ = getDefaultInstance().getBrowserVersion();
onChanged();
return this;
}
/**
* optional string browser_version = 3;
*
*
* Version of the browser. This comes from goog.userAgent.product.VERSION.
*
*/
public Builder setBrowserVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
browserVersion_ = value;
onChanged();
return this;
}
// optional string flash_version = 4;
private java.lang.Object flashVersion_ = "";
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public boolean hasFlashVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public java.lang.String getFlashVersion() {
java.lang.Object ref = flashVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
flashVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public com.google.protobuf.ByteString
getFlashVersionBytes() {
java.lang.Object ref = flashVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
flashVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public Builder setFlashVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
flashVersion_ = value;
onChanged();
return this;
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public Builder clearFlashVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
flashVersion_ = getDefaultInstance().getFlashVersion();
onChanged();
return this;
}
/**
* optional string flash_version = 4;
*
*
* Version of flash the browser is using.
* This comes from goog.userAgent.flash.VERSION. Should not be included if
* goog.userAgent.flash.HAS_FLASH is false.
*
*/
public Builder setFlashVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
flashVersion_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.BrowserInfo)
}
static {
defaultInstance = new BrowserInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.BrowserInfo)
}
public interface DesktopClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string client_id = 1;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
boolean hasClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
java.lang.String getClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
com.google.protobuf.ByteString
getClientIdBytes();
// optional string logging_id = 2;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
boolean hasLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
java.lang.String getLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
com.google.protobuf.ByteString
getLoggingIdBytes();
// optional string os = 3;
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
boolean hasOs();
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
java.lang.String getOs();
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
com.google.protobuf.ByteString
getOsBytes();
// optional string os_major_version = 4;
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
boolean hasOsMajorVersion();
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
java.lang.String getOsMajorVersion();
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
com.google.protobuf.ByteString
getOsMajorVersionBytes();
// optional string os_full_version = 5;
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
boolean hasOsFullVersion();
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
java.lang.String getOsFullVersion();
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
com.google.protobuf.ByteString
getOsFullVersionBytes();
// optional string application_build = 6;
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
boolean hasApplicationBuild();
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
java.lang.String getApplicationBuild();
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
com.google.protobuf.ByteString
getApplicationBuildBytes();
// optional string country = 7;
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
boolean hasCountry();
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
java.lang.String getCountry();
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
com.google.protobuf.ByteString
getCountryBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.DesktopClientInfo}
*
*
* Attributes specific to desktop clients.
*
* Next tag: 8
*
*/
public static final class DesktopClientInfo extends
com.google.protobuf.GeneratedMessage
implements DesktopClientInfoOrBuilder {
// Use DesktopClientInfo.newBuilder() to construct.
private DesktopClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DesktopClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DesktopClientInfo defaultInstance;
public static DesktopClientInfo getDefaultInstance() {
return defaultInstance;
}
public DesktopClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DesktopClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
clientId_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
loggingId_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
os_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
osMajorVersion_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
osFullVersion_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
applicationBuild_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
country_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DesktopClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DesktopClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DesktopClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string client_id = 1;
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private java.lang.Object clientId_;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientId_ = s;
}
return s;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string logging_id = 2;
public static final int LOGGING_ID_FIELD_NUMBER = 2;
private java.lang.Object loggingId_;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loggingId_ = s;
}
return s;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os = 3;
public static final int OS_FIELD_NUMBER = 3;
private java.lang.Object os_;
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
os_ = s;
}
return s;
}
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_major_version = 4;
public static final int OS_MAJOR_VERSION_FIELD_NUMBER = 4;
private java.lang.Object osMajorVersion_;
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public boolean hasOsMajorVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public java.lang.String getOsMajorVersion() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osMajorVersion_ = s;
}
return s;
}
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public com.google.protobuf.ByteString
getOsMajorVersionBytes() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osMajorVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_full_version = 5;
public static final int OS_FULL_VERSION_FIELD_NUMBER = 5;
private java.lang.Object osFullVersion_;
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public boolean hasOsFullVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public java.lang.String getOsFullVersion() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osFullVersion_ = s;
}
return s;
}
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public com.google.protobuf.ByteString
getOsFullVersionBytes() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osFullVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string application_build = 6;
public static final int APPLICATION_BUILD_FIELD_NUMBER = 6;
private java.lang.Object applicationBuild_;
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
applicationBuild_ = s;
}
return s;
}
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string country = 7;
public static final int COUNTRY_FIELD_NUMBER = 7;
private java.lang.Object country_;
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
clientId_ = "";
loggingId_ = "";
os_ = "";
osMajorVersion_ = "";
osFullVersion_ = "";
applicationBuild_ = "";
country_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getOsBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getOsMajorVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getOsFullVersionBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, getCountryBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getOsBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getOsMajorVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getOsFullVersionBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getCountryBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.DesktopClientInfo}
*
*
* Attributes specific to desktop clients.
*
* Next tag: 8
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DesktopClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
loggingId_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
os_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
osMajorVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
osFullVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
applicationBuild_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
country_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clientId_ = clientId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.loggingId_ = loggingId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.os_ = os_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.osMajorVersion_ = osMajorVersion_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.osFullVersion_ = osFullVersion_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.applicationBuild_ = applicationBuild_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.country_ = country_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance()) return this;
if (other.hasClientId()) {
bitField0_ |= 0x00000001;
clientId_ = other.clientId_;
onChanged();
}
if (other.hasLoggingId()) {
bitField0_ |= 0x00000002;
loggingId_ = other.loggingId_;
onChanged();
}
if (other.hasOs()) {
bitField0_ |= 0x00000004;
os_ = other.os_;
onChanged();
}
if (other.hasOsMajorVersion()) {
bitField0_ |= 0x00000008;
osMajorVersion_ = other.osMajorVersion_;
onChanged();
}
if (other.hasOsFullVersion()) {
bitField0_ |= 0x00000010;
osFullVersion_ = other.osFullVersion_;
onChanged();
}
if (other.hasApplicationBuild()) {
bitField0_ |= 0x00000020;
applicationBuild_ = other.applicationBuild_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00000040;
country_ = other.country_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string client_id = 1;
private java.lang.Object clientId_ = "";
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder clearClientId() {
bitField0_ = (bitField0_ & ~0x00000001);
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
// optional string logging_id = 2;
private java.lang.Object loggingId_ = "";
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
loggingId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder clearLoggingId() {
bitField0_ = (bitField0_ & ~0x00000002);
loggingId_ = getDefaultInstance().getLoggingId();
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
// optional string os = 3;
private java.lang.Object os_ = "";
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
os_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder setOs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
os_ = value;
onChanged();
return this;
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder clearOs() {
bitField0_ = (bitField0_ & ~0x00000004);
os_ = getDefaultInstance().getOs();
onChanged();
return this;
}
/**
* optional string os = 3;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder setOsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
os_ = value;
onChanged();
return this;
}
// optional string os_major_version = 4;
private java.lang.Object osMajorVersion_ = "";
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public boolean hasOsMajorVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public java.lang.String getOsMajorVersion() {
java.lang.Object ref = osMajorVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osMajorVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public com.google.protobuf.ByteString
getOsMajorVersionBytes() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osMajorVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public Builder setOsMajorVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
osMajorVersion_ = value;
onChanged();
return this;
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public Builder clearOsMajorVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
osMajorVersion_ = getDefaultInstance().getOsMajorVersion();
onChanged();
return this;
}
/**
* optional string os_major_version = 4;
*
*
* e.g., "10.7", "Vista", "Win7"
*
*/
public Builder setOsMajorVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
osMajorVersion_ = value;
onChanged();
return this;
}
// optional string os_full_version = 5;
private java.lang.Object osFullVersion_ = "";
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public boolean hasOsFullVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public java.lang.String getOsFullVersion() {
java.lang.Object ref = osFullVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osFullVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public com.google.protobuf.ByteString
getOsFullVersionBytes() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osFullVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public Builder setOsFullVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
osFullVersion_ = value;
onChanged();
return this;
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public Builder clearOsFullVersion() {
bitField0_ = (bitField0_ & ~0x00000010);
osFullVersion_ = getDefaultInstance().getOsFullVersion();
onChanged();
return this;
}
/**
* optional string os_full_version = 5;
*
*
* e.g., "10.7.3", "Win7 SP1"
*
*/
public Builder setOsFullVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
osFullVersion_ = value;
onChanged();
return this;
}
// optional string application_build = 6;
private java.lang.Object applicationBuild_ = "";
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
applicationBuild_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public Builder setApplicationBuild(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
applicationBuild_ = value;
onChanged();
return this;
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public Builder clearApplicationBuild() {
bitField0_ = (bitField0_ & ~0x00000020);
applicationBuild_ = getDefaultInstance().getApplicationBuild();
onChanged();
return this;
}
/**
* optional string application_build = 6;
*
*
* The client application version.
*
*/
public Builder setApplicationBuildBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
applicationBuild_ = value;
onChanged();
return this;
}
// optional string country = 7;
private java.lang.Object country_ = "";
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00000040);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 7;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
country_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.DesktopClientInfo)
}
static {
defaultInstance = new DesktopClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.DesktopClientInfo)
}
public interface IosClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string client_id = 1;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
boolean hasClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
java.lang.String getClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
com.google.protobuf.ByteString
getClientIdBytes();
// optional string logging_id = 2;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
boolean hasLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
java.lang.String getLoggingId();
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
com.google.protobuf.ByteString
getLoggingIdBytes();
// optional string os_major_version = 3;
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
boolean hasOsMajorVersion();
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
java.lang.String getOsMajorVersion();
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
com.google.protobuf.ByteString
getOsMajorVersionBytes();
// optional string os_full_version = 4;
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
boolean hasOsFullVersion();
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
java.lang.String getOsFullVersion();
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
com.google.protobuf.ByteString
getOsFullVersionBytes();
// optional string application_build = 5;
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
boolean hasApplicationBuild();
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
java.lang.String getApplicationBuild();
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
com.google.protobuf.ByteString
getApplicationBuildBytes();
// optional string country = 6;
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
boolean hasCountry();
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
java.lang.String getCountry();
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
com.google.protobuf.ByteString
getCountryBytes();
// optional string model = 7;
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
boolean hasModel();
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
java.lang.String getModel();
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
com.google.protobuf.ByteString
getModelBytes();
// optional string language_code = 8;
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
boolean hasLanguageCode();
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
java.lang.String getLanguageCode();
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
com.google.protobuf.ByteString
getLanguageCodeBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.IosClientInfo}
*
*
* Attributes specific to iOS clients.
*
* NOTE(vjalan): Fields of this proto are made available as conditions for iOS
* client-side experimentation in Heterodyne. Fields which contain PII should be
* annotated with experiments.heterodyne.ignore_for_application_properties to
* ensure that they cannot be used as conditions.
*
* Next tag: 9
*
*/
public static final class IosClientInfo extends
com.google.protobuf.GeneratedMessage
implements IosClientInfoOrBuilder {
// Use IosClientInfo.newBuilder() to construct.
private IosClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private IosClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final IosClientInfo defaultInstance;
public static IosClientInfo getDefaultInstance() {
return defaultInstance;
}
public IosClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IosClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
clientId_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
loggingId_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
osMajorVersion_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
osFullVersion_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
applicationBuild_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
country_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
model_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000080;
languageCode_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_IosClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_IosClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public IosClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IosClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string client_id = 1;
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private java.lang.Object clientId_;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientId_ = s;
}
return s;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string logging_id = 2;
public static final int LOGGING_ID_FIELD_NUMBER = 2;
private java.lang.Object loggingId_;
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loggingId_ = s;
}
return s;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_major_version = 3;
public static final int OS_MAJOR_VERSION_FIELD_NUMBER = 3;
private java.lang.Object osMajorVersion_;
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public boolean hasOsMajorVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public java.lang.String getOsMajorVersion() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osMajorVersion_ = s;
}
return s;
}
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public com.google.protobuf.ByteString
getOsMajorVersionBytes() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osMajorVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_full_version = 4;
public static final int OS_FULL_VERSION_FIELD_NUMBER = 4;
private java.lang.Object osFullVersion_;
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public boolean hasOsFullVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public java.lang.String getOsFullVersion() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osFullVersion_ = s;
}
return s;
}
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public com.google.protobuf.ByteString
getOsFullVersionBytes() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osFullVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string application_build = 5;
public static final int APPLICATION_BUILD_FIELD_NUMBER = 5;
private java.lang.Object applicationBuild_;
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
applicationBuild_ = s;
}
return s;
}
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string country = 6;
public static final int COUNTRY_FIELD_NUMBER = 6;
private java.lang.Object country_;
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string model = 7;
public static final int MODEL_FIELD_NUMBER = 7;
private java.lang.Object model_;
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
model_ = s;
}
return s;
}
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string language_code = 8;
public static final int LANGUAGE_CODE_FIELD_NUMBER = 8;
private java.lang.Object languageCode_;
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public boolean hasLanguageCode() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public java.lang.String getLanguageCode() {
java.lang.Object ref = languageCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
languageCode_ = s;
}
return s;
}
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public com.google.protobuf.ByteString
getLanguageCodeBytes() {
java.lang.Object ref = languageCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
clientId_ = "";
loggingId_ = "";
osMajorVersion_ = "";
osFullVersion_ = "";
applicationBuild_ = "";
country_ = "";
model_ = "";
languageCode_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getOsMajorVersionBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getOsFullVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getCountryBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, getModelBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, getLanguageCodeBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getOsMajorVersionBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getOsFullVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getCountryBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getModelBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getLanguageCodeBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.IosClientInfo}
*
*
* Attributes specific to iOS clients.
*
* NOTE(vjalan): Fields of this proto are made available as conditions for iOS
* client-side experimentation in Heterodyne. Fields which contain PII should be
* annotated with experiments.heterodyne.ignore_for_application_properties to
* ensure that they cannot be used as conditions.
*
* Next tag: 9
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_IosClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_IosClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
loggingId_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
osMajorVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
osFullVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
applicationBuild_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
country_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
model_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
languageCode_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_IosClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clientId_ = clientId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.loggingId_ = loggingId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.osMajorVersion_ = osMajorVersion_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.osFullVersion_ = osFullVersion_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.applicationBuild_ = applicationBuild_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.country_ = country_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.model_ = model_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.languageCode_ = languageCode_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance()) return this;
if (other.hasClientId()) {
bitField0_ |= 0x00000001;
clientId_ = other.clientId_;
onChanged();
}
if (other.hasLoggingId()) {
bitField0_ |= 0x00000002;
loggingId_ = other.loggingId_;
onChanged();
}
if (other.hasOsMajorVersion()) {
bitField0_ |= 0x00000004;
osMajorVersion_ = other.osMajorVersion_;
onChanged();
}
if (other.hasOsFullVersion()) {
bitField0_ |= 0x00000008;
osFullVersion_ = other.osFullVersion_;
onChanged();
}
if (other.hasApplicationBuild()) {
bitField0_ |= 0x00000010;
applicationBuild_ = other.applicationBuild_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00000020;
country_ = other.country_;
onChanged();
}
if (other.hasModel()) {
bitField0_ |= 0x00000040;
model_ = other.model_;
onChanged();
}
if (other.hasLanguageCode()) {
bitField0_ |= 0x00000080;
languageCode_ = other.languageCode_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string client_id = 1;
private java.lang.Object clientId_ = "";
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder clearClientId() {
bitField0_ = (bitField0_ & ~0x00000001);
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII.
*
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
// optional string logging_id = 2;
private java.lang.Object loggingId_ = "";
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
loggingId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder clearLoggingId() {
bitField0_ = (bitField0_ & ~0x00000002);
loggingId_ = getDefaultInstance().getLoggingId();
onChanged();
return this;
}
/**
* optional string logging_id = 2;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
// optional string os_major_version = 3;
private java.lang.Object osMajorVersion_ = "";
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public boolean hasOsMajorVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public java.lang.String getOsMajorVersion() {
java.lang.Object ref = osMajorVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osMajorVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public com.google.protobuf.ByteString
getOsMajorVersionBytes() {
java.lang.Object ref = osMajorVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osMajorVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public Builder setOsMajorVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
osMajorVersion_ = value;
onChanged();
return this;
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public Builder clearOsMajorVersion() {
bitField0_ = (bitField0_ & ~0x00000004);
osMajorVersion_ = getDefaultInstance().getOsMajorVersion();
onChanged();
return this;
}
/**
* optional string os_major_version = 3;
*
*
* The major OS version of an iOS client.
*
*/
public Builder setOsMajorVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
osMajorVersion_ = value;
onChanged();
return this;
}
// optional string os_full_version = 4;
private java.lang.Object osFullVersion_ = "";
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public boolean hasOsFullVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public java.lang.String getOsFullVersion() {
java.lang.Object ref = osFullVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osFullVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public com.google.protobuf.ByteString
getOsFullVersionBytes() {
java.lang.Object ref = osFullVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osFullVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public Builder setOsFullVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
osFullVersion_ = value;
onChanged();
return this;
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public Builder clearOsFullVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
osFullVersion_ = getDefaultInstance().getOsFullVersion();
onChanged();
return this;
}
/**
* optional string os_full_version = 4;
*
*
* The minor OS version of an iOS client.
*
*/
public Builder setOsFullVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
osFullVersion_ = value;
onChanged();
return this;
}
// optional string application_build = 5;
private java.lang.Object applicationBuild_ = "";
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
applicationBuild_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public Builder setApplicationBuild(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
applicationBuild_ = value;
onChanged();
return this;
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public Builder clearApplicationBuild() {
bitField0_ = (bitField0_ & ~0x00000010);
applicationBuild_ = getDefaultInstance().getApplicationBuild();
onChanged();
return this;
}
/**
* optional string application_build = 5;
*
*
* The client application version.
*
*/
public Builder setApplicationBuildBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
applicationBuild_ = value;
onChanged();
return this;
}
// optional string country = 6;
private java.lang.Object country_ = "";
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00000020);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 6;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* This is typically populated based on the value of:
* [[NSLocale currentLocale] objectForKey:NSLocaleCountryCode]
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
country_ = value;
onChanged();
return this;
}
// optional string model = 7;
private java.lang.Object model_ = "";
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
model_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public Builder setModel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
model_ = value;
onChanged();
return this;
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public Builder clearModel() {
bitField0_ = (bitField0_ & ~0x00000040);
model_ = getDefaultInstance().getModel();
onChanged();
return this;
}
/**
* optional string model = 7;
*
*
* The unix name (uname) for the model from an iOS client. e.g., "iPhone6,1"
* for iPhone 5s.
*
*/
public Builder setModelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
model_ = value;
onChanged();
return this;
}
// optional string language_code = 8;
private java.lang.Object languageCode_ = "";
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public boolean hasLanguageCode() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public java.lang.String getLanguageCode() {
java.lang.Object ref = languageCode_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
languageCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public com.google.protobuf.ByteString
getLanguageCodeBytes() {
java.lang.Object ref = languageCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public Builder setLanguageCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
languageCode_ = value;
onChanged();
return this;
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public Builder clearLanguageCode() {
bitField0_ = (bitField0_ & ~0x00000080);
languageCode_ = getDefaultInstance().getLanguageCode();
onChanged();
return this;
}
/**
* optional string language_code = 8;
*
*
* The current language displayed to user. e.g., "en", "fr", "en-AU", or "de"
* This comes from [[NSBundle mainBundle] preferredLocalizations]; and is:
* "[language designator]" or "[language designator]-[region designator]"
* format based off of what localization is available and displayed to user.
*
*/
public Builder setLanguageCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
languageCode_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.IosClientInfo)
}
static {
defaultInstance = new IosClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.IosClientInfo)
}
public interface PancettaClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string device_id = 1;
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
boolean hasDeviceId();
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
java.lang.String getDeviceId();
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
com.google.protobuf.ByteString
getDeviceIdBytes();
// optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
boolean hasOs();
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType getOs();
// optional string app_id = 3;
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
boolean hasAppId();
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
java.lang.String getAppId();
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
com.google.protobuf.ByteString
getAppIdBytes();
// optional string app_version = 4;
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
boolean hasAppVersion();
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
java.lang.String getAppVersion();
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
com.google.protobuf.ByteString
getAppVersionBytes();
// optional string mcc_mnc = 5;
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
boolean hasMccMnc();
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
java.lang.String getMccMnc();
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
com.google.protobuf.ByteString
getMccMncBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.PancettaClientInfo}
*
*
* Attributes specific to Pancetta Chrome app clients. Pancetta clients will be
* Chrome apps running on desktops or a headless network access point. The apps
* are used to upload data from wearables to Pancetta servers.
*
* Next tag: 6
*
*/
public static final class PancettaClientInfo extends
com.google.protobuf.GeneratedMessage
implements PancettaClientInfoOrBuilder {
// Use PancettaClientInfo.newBuilder() to construct.
private PancettaClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PancettaClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PancettaClientInfo defaultInstance;
public static PancettaClientInfo getDefaultInstance() {
return defaultInstance;
}
public PancettaClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PancettaClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
deviceId_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
os_ = value;
}
break;
}
case 26: {
bitField0_ |= 0x00000004;
appId_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
appVersion_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
mccMnc_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PancettaClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PancettaClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PancettaClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.PancettaClientInfo.OsType}
*
*
* Operating system.
* Next tag: 7
*
*/
public enum OsType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OS_TYPE_UNKNOWN = 0;
*/
OS_TYPE_UNKNOWN(0, 0),
/**
* OS_TYPE_MAC = 1;
*/
OS_TYPE_MAC(1, 1),
/**
* OS_TYPE_WINDOWS = 2;
*/
OS_TYPE_WINDOWS(2, 2),
/**
* OS_TYPE_ANDROID = 3;
*/
OS_TYPE_ANDROID(3, 3),
/**
* OS_TYPE_CROS = 4;
*/
OS_TYPE_CROS(4, 4),
/**
* OS_TYPE_LINUX = 5;
*/
OS_TYPE_LINUX(5, 5),
/**
* OS_TYPE_OPENBSD = 6;
*/
OS_TYPE_OPENBSD(6, 6),
;
/**
* OS_TYPE_UNKNOWN = 0;
*/
public static final int OS_TYPE_UNKNOWN_VALUE = 0;
/**
* OS_TYPE_MAC = 1;
*/
public static final int OS_TYPE_MAC_VALUE = 1;
/**
* OS_TYPE_WINDOWS = 2;
*/
public static final int OS_TYPE_WINDOWS_VALUE = 2;
/**
* OS_TYPE_ANDROID = 3;
*/
public static final int OS_TYPE_ANDROID_VALUE = 3;
/**
* OS_TYPE_CROS = 4;
*/
public static final int OS_TYPE_CROS_VALUE = 4;
/**
* OS_TYPE_LINUX = 5;
*/
public static final int OS_TYPE_LINUX_VALUE = 5;
/**
* OS_TYPE_OPENBSD = 6;
*/
public static final int OS_TYPE_OPENBSD_VALUE = 6;
public final int getNumber() { return value; }
public static OsType valueOf(int value) {
switch (value) {
case 0: return OS_TYPE_UNKNOWN;
case 1: return OS_TYPE_MAC;
case 2: return OS_TYPE_WINDOWS;
case 3: return OS_TYPE_ANDROID;
case 4: return OS_TYPE_CROS;
case 5: return OS_TYPE_LINUX;
case 6: return OS_TYPE_OPENBSD;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OsType findValueByNumber(int number) {
return OsType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDescriptor().getEnumTypes().get(0);
}
private static final OsType[] VALUES = values();
public static OsType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private OsType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.PancettaClientInfo.OsType)
}
private int bitField0_;
// optional string device_id = 1;
public static final int DEVICE_ID_FIELD_NUMBER = 1;
private java.lang.Object deviceId_;
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public java.lang.String getDeviceId() {
java.lang.Object ref = deviceId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceId_ = s;
}
return s;
}
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public com.google.protobuf.ByteString
getDeviceIdBytes() {
java.lang.Object ref = deviceId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
public static final int OS_FIELD_NUMBER = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType os_;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType getOs() {
return os_;
}
// optional string app_id = 3;
public static final int APP_ID_FIELD_NUMBER = 3;
private java.lang.Object appId_;
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
}
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string app_version = 4;
public static final int APP_VERSION_FIELD_NUMBER = 4;
private java.lang.Object appVersion_;
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public boolean hasAppVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public java.lang.String getAppVersion() {
java.lang.Object ref = appVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appVersion_ = s;
}
return s;
}
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public com.google.protobuf.ByteString
getAppVersionBytes() {
java.lang.Object ref = appVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string mcc_mnc = 5;
public static final int MCC_MNC_FIELD_NUMBER = 5;
private java.lang.Object mccMnc_;
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public boolean hasMccMnc() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public java.lang.String getMccMnc() {
java.lang.Object ref = mccMnc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mccMnc_ = s;
}
return s;
}
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public com.google.protobuf.ByteString
getMccMncBytes() {
java.lang.Object ref = mccMnc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mccMnc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
deviceId_ = "";
os_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType.OS_TYPE_UNKNOWN;
appId_ = "";
appVersion_ = "";
mccMnc_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getDeviceIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, os_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getAppIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getAppVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getMccMncBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getDeviceIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, os_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getAppIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getAppVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getMccMncBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.PancettaClientInfo}
*
*
* Attributes specific to Pancetta Chrome app clients. Pancetta clients will be
* Chrome apps running on desktops or a headless network access point. The apps
* are used to upload data from wearables to Pancetta servers.
*
* Next tag: 6
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PancettaClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
deviceId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
os_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType.OS_TYPE_UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000002);
appId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
appVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
mccMnc_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.deviceId_ = deviceId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.os_ = os_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.appId_ = appId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.appVersion_ = appVersion_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.mccMnc_ = mccMnc_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance()) return this;
if (other.hasDeviceId()) {
bitField0_ |= 0x00000001;
deviceId_ = other.deviceId_;
onChanged();
}
if (other.hasOs()) {
setOs(other.getOs());
}
if (other.hasAppId()) {
bitField0_ |= 0x00000004;
appId_ = other.appId_;
onChanged();
}
if (other.hasAppVersion()) {
bitField0_ |= 0x00000008;
appVersion_ = other.appVersion_;
onChanged();
}
if (other.hasMccMnc()) {
bitField0_ |= 0x00000010;
mccMnc_ = other.mccMnc_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string device_id = 1;
private java.lang.Object deviceId_ = "";
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public java.lang.String getDeviceId() {
java.lang.Object ref = deviceId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
deviceId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public com.google.protobuf.ByteString
getDeviceIdBytes() {
java.lang.Object ref = deviceId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public Builder setDeviceId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
deviceId_ = value;
onChanged();
return this;
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public Builder clearDeviceId() {
bitField0_ = (bitField0_ & ~0x00000001);
deviceId_ = getDefaultInstance().getDeviceId();
onChanged();
return this;
}
/**
* optional string device_id = 1;
*
*
* Device ID that should be considered PII. This should only be filled by
* Pancetta's headless network access point which is manufactured and
* distributed by Google.
*
*/
public Builder setDeviceIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
deviceId_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType os_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType.OS_TYPE_UNKNOWN;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType getOs() {
return os_;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public Builder setOs(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
os_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo.OsType os = 2;
*
*
* The OS that Chrome is running on.
*
*/
public Builder clearOs() {
bitField0_ = (bitField0_ & ~0x00000002);
os_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.OsType.OS_TYPE_UNKNOWN;
onChanged();
return this;
}
// optional string app_id = 3;
private java.lang.Object appId_ = "";
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
appId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public Builder setAppId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
appId_ = value;
onChanged();
return this;
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public Builder clearAppId() {
bitField0_ = (bitField0_ & ~0x00000004);
appId_ = getDefaultInstance().getAppId();
onChanged();
return this;
}
/**
* optional string app_id = 3;
*
*
* The Chrome app id.
*
*/
public Builder setAppIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
appId_ = value;
onChanged();
return this;
}
// optional string app_version = 4;
private java.lang.Object appVersion_ = "";
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public boolean hasAppVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public java.lang.String getAppVersion() {
java.lang.Object ref = appVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
appVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public com.google.protobuf.ByteString
getAppVersionBytes() {
java.lang.Object ref = appVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public Builder setAppVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
appVersion_ = value;
onChanged();
return this;
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public Builder clearAppVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
appVersion_ = getDefaultInstance().getAppVersion();
onChanged();
return this;
}
/**
* optional string app_version = 4;
*
*
* The Chrome app version.
*
*/
public Builder setAppVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
appVersion_ = value;
onChanged();
return this;
}
// optional string mcc_mnc = 5;
private java.lang.Object mccMnc_ = "";
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public boolean hasMccMnc() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public java.lang.String getMccMnc() {
java.lang.Object ref = mccMnc_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
mccMnc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public com.google.protobuf.ByteString
getMccMncBytes() {
java.lang.Object ref = mccMnc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mccMnc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public Builder setMccMnc(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
mccMnc_ = value;
onChanged();
return this;
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public Builder clearMccMnc() {
bitField0_ = (bitField0_ & ~0x00000010);
mccMnc_ = getDefaultInstance().getMccMnc();
onChanged();
return this;
}
/**
* optional string mcc_mnc = 5;
*
*
* Optional. Mobile Country Code and Mobile Network Code if possible.
* This is for the headless network access point.
*
*/
public Builder setMccMncBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
mccMnc_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.PancettaClientInfo)
}
static {
defaultInstance = new PancettaClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.PancettaClientInfo)
}
public interface PlayCeClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string client_id = 1;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
boolean hasClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
java.lang.String getClientId();
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
com.google.protobuf.ByteString
getClientIdBytes();
// optional string logging_id = 7;
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
boolean hasLoggingId();
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
java.lang.String getLoggingId();
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
com.google.protobuf.ByteString
getLoggingIdBytes();
// optional string make = 3;
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
boolean hasMake();
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
java.lang.String getMake();
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
com.google.protobuf.ByteString
getMakeBytes();
// optional string model = 4;
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
boolean hasModel();
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
java.lang.String getModel();
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
com.google.protobuf.ByteString
getModelBytes();
// optional string application_build = 5;
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
boolean hasApplicationBuild();
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
java.lang.String getApplicationBuild();
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
com.google.protobuf.ByteString
getApplicationBuildBytes();
// optional string platform_version = 6;
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
boolean hasPlatformVersion();
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
java.lang.String getPlatformVersion();
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
com.google.protobuf.ByteString
getPlatformVersionBytes();
// optional string country = 8;
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
boolean hasCountry();
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
java.lang.String getCountry();
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
com.google.protobuf.ByteString
getCountryBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.PlayCeClientInfo}
*
*
* Attributes specific to third-party CE devices.
* CE devices scope
* - Roku
* - Smart TV (Non Android-TV)
* - PlayStation 3/4
* - XBox 360/One
*
* We do not specify types of devices since it is defined by the pair of
* device_make and device_model (e.g.: make=roku + model=2500X = streaming box,
* make=lge + model=42LN5700 = Smart TV, make=tivo + model=roamio = Set Top Box)
* Next tag: 9
*
*/
public static final class PlayCeClientInfo extends
com.google.protobuf.GeneratedMessage
implements PlayCeClientInfoOrBuilder {
// Use PlayCeClientInfo.newBuilder() to construct.
private PlayCeClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PlayCeClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PlayCeClientInfo defaultInstance;
public static PlayCeClientInfo getDefaultInstance() {
return defaultInstance;
}
public PlayCeClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PlayCeClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
clientId_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
make_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
model_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
applicationBuild_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
platformVersion_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000002;
loggingId_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000040;
country_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PlayCeClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PlayCeClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PlayCeClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string client_id = 1;
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private java.lang.Object clientId_;
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientId_ = s;
}
return s;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string logging_id = 7;
public static final int LOGGING_ID_FIELD_NUMBER = 7;
private java.lang.Object loggingId_;
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loggingId_ = s;
}
return s;
}
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string make = 3;
public static final int MAKE_FIELD_NUMBER = 3;
private java.lang.Object make_;
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public boolean hasMake() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public java.lang.String getMake() {
java.lang.Object ref = make_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
make_ = s;
}
return s;
}
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public com.google.protobuf.ByteString
getMakeBytes() {
java.lang.Object ref = make_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
make_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string model = 4;
public static final int MODEL_FIELD_NUMBER = 4;
private java.lang.Object model_;
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
model_ = s;
}
return s;
}
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string application_build = 5;
public static final int APPLICATION_BUILD_FIELD_NUMBER = 5;
private java.lang.Object applicationBuild_;
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
applicationBuild_ = s;
}
return s;
}
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string platform_version = 6;
public static final int PLATFORM_VERSION_FIELD_NUMBER = 6;
private java.lang.Object platformVersion_;
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public boolean hasPlatformVersion() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public java.lang.String getPlatformVersion() {
java.lang.Object ref = platformVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
platformVersion_ = s;
}
return s;
}
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public com.google.protobuf.ByteString
getPlatformVersionBytes() {
java.lang.Object ref = platformVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platformVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string country = 8;
public static final int COUNTRY_FIELD_NUMBER = 8;
private java.lang.Object country_;
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
clientId_ = "";
loggingId_ = "";
make_ = "";
model_ = "";
applicationBuild_ = "";
platformVersion_ = "";
country_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMakeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getModelBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getPlatformVersionBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(7, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(8, getCountryBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getClientIdBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMakeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getModelBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getApplicationBuildBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getPlatformVersionBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getLoggingIdBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getCountryBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.PlayCeClientInfo}
*
*
* Attributes specific to third-party CE devices.
* CE devices scope
* - Roku
* - Smart TV (Non Android-TV)
* - PlayStation 3/4
* - XBox 360/One
*
* We do not specify types of devices since it is defined by the pair of
* device_make and device_model (e.g.: make=roku + model=2500X = streaming box,
* make=lge + model=42LN5700 = Smart TV, make=tivo + model=roamio = Set Top Box)
* Next tag: 9
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PlayCeClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
loggingId_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
make_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
model_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
applicationBuild_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
platformVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
country_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clientId_ = clientId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.loggingId_ = loggingId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.make_ = make_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.model_ = model_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.applicationBuild_ = applicationBuild_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.platformVersion_ = platformVersion_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.country_ = country_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance()) return this;
if (other.hasClientId()) {
bitField0_ |= 0x00000001;
clientId_ = other.clientId_;
onChanged();
}
if (other.hasLoggingId()) {
bitField0_ |= 0x00000002;
loggingId_ = other.loggingId_;
onChanged();
}
if (other.hasMake()) {
bitField0_ |= 0x00000004;
make_ = other.make_;
onChanged();
}
if (other.hasModel()) {
bitField0_ |= 0x00000008;
model_ = other.model_;
onChanged();
}
if (other.hasApplicationBuild()) {
bitField0_ |= 0x00000010;
applicationBuild_ = other.applicationBuild_;
onChanged();
}
if (other.hasPlatformVersion()) {
bitField0_ |= 0x00000020;
platformVersion_ = other.platformVersion_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00000040;
country_ = other.country_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string client_id = 1;
private java.lang.Object clientId_ = "";
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public Builder clearClientId() {
bitField0_ = (bitField0_ & ~0x00000001);
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
* optional string client_id = 1;
*
*
* Sticky ID that should be considered PII. This is the device serial number
* or another device identifier that, together with make and model, uniquely
* identifies the device.
*
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientId_ = value;
onChanged();
return this;
}
// optional string logging_id = 7;
private java.lang.Object loggingId_ = "";
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public boolean hasLoggingId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public java.lang.String getLoggingId() {
java.lang.Object ref = loggingId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
loggingId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public com.google.protobuf.ByteString
getLoggingIdBytes() {
java.lang.Object ref = loggingId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loggingId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder clearLoggingId() {
bitField0_ = (bitField0_ & ~0x00000002);
loggingId_ = getDefaultInstance().getLoggingId();
onChanged();
return this;
}
/**
* optional string logging_id = 7;
*
*
* Pseudonymous, random id (sticky) per device. May only be logged in temp
* logs if GWSLogEntryProto.UserInfo.UserId is not set.
*
*/
public Builder setLoggingIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loggingId_ = value;
onChanged();
return this;
}
// optional string make = 3;
private java.lang.Object make_ = "";
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public boolean hasMake() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public java.lang.String getMake() {
java.lang.Object ref = make_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
make_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public com.google.protobuf.ByteString
getMakeBytes() {
java.lang.Object ref = make_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
make_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public Builder setMake(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
make_ = value;
onChanged();
return this;
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public Builder clearMake() {
bitField0_ = (bitField0_ & ~0x00000004);
make_ = getDefaultInstance().getMake();
onChanged();
return this;
}
/**
* optional string make = 3;
*
*
* Device make. E.g.: samsung, lge, google, sony, microsoft.
* Case insensitive. RoKu==roku.
*
*/
public Builder setMakeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
make_ = value;
onChanged();
return this;
}
// optional string model = 4;
private java.lang.Object model_ = "";
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
model_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public Builder setModel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
model_ = value;
onChanged();
return this;
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public Builder clearModel() {
bitField0_ = (bitField0_ & ~0x00000008);
model_ = getDefaultInstance().getModel();
onChanged();
return this;
}
/**
* optional string model = 4;
*
*
* Device model. E.g.: 2500X. Case insensitive. 2500X==2500x.
*
*/
public Builder setModelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
model_ = value;
onChanged();
return this;
}
// optional string application_build = 5;
private java.lang.Object applicationBuild_ = "";
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public boolean hasApplicationBuild() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public java.lang.String getApplicationBuild() {
java.lang.Object ref = applicationBuild_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
applicationBuild_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public com.google.protobuf.ByteString
getApplicationBuildBytes() {
java.lang.Object ref = applicationBuild_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationBuild_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public Builder setApplicationBuild(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
applicationBuild_ = value;
onChanged();
return this;
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public Builder clearApplicationBuild() {
bitField0_ = (bitField0_ & ~0x00000010);
applicationBuild_ = getDefaultInstance().getApplicationBuild();
onChanged();
return this;
}
/**
* optional string application_build = 5;
*
*
* App version/build. Each application defines it for itself.
* E.g.: GPM20140708-171921 on make=roku and model=2500X corresponds to a
* combination of BrightScript and C++ code compiled for mips
* from the git snapshot of the GPM app at the given time (20140708-171921).
*
*/
public Builder setApplicationBuildBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
applicationBuild_ = value;
onChanged();
return this;
}
// optional string platform_version = 6;
private java.lang.Object platformVersion_ = "";
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public boolean hasPlatformVersion() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public java.lang.String getPlatformVersion() {
java.lang.Object ref = platformVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
platformVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public com.google.protobuf.ByteString
getPlatformVersionBytes() {
java.lang.Object ref = platformVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platformVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public Builder setPlatformVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
platformVersion_ = value;
onChanged();
return this;
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public Builder clearPlatformVersion() {
bitField0_ = (bitField0_ & ~0x00000020);
platformVersion_ = getDefaultInstance().getPlatformVersion();
onChanged();
return this;
}
/**
* optional string platform_version = 6;
*
*
* Platform version. E.g.: 5.5. (Roku FW version for Play Movies launch). Can
* include letters (e.g.: 5.5.3254b for a beta build #3254 of the FW release
* 5.5).
*
*/
public Builder setPlatformVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
platformVersion_ = value;
onChanged();
return this;
}
// optional string country = 8;
private java.lang.Object country_ = "";
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00000040);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
* Where this comes from is currently app-specific
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
country_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.PlayCeClientInfo)
}
static {
defaultInstance = new PlayCeClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.PlayCeClientInfo)
}
public interface VrClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
boolean hasVrClientType();
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType getVrClientType();
// optional string sdk_version = 2;
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
boolean hasSdkVersion();
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
java.lang.String getSdkVersion();
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
com.google.protobuf.ByteString
getSdkVersionBytes();
// optional string fingerprint = 3;
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
boolean hasFingerprint();
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
java.lang.String getFingerprint();
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
com.google.protobuf.ByteString
getFingerprintBytes();
// optional string gvr_version = 4;
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
boolean hasGvrVersion();
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
java.lang.String getGvrVersion();
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
com.google.protobuf.ByteString
getGvrVersionBytes();
// optional string manufacturer = 5;
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
boolean hasManufacturer();
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
java.lang.String getManufacturer();
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
com.google.protobuf.ByteString
getManufacturerBytes();
// optional string model = 6;
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
boolean hasModel();
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
java.lang.String getModel();
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
com.google.protobuf.ByteString
getModelBytes();
// optional string language = 7;
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
boolean hasLanguage();
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
java.lang.String getLanguage();
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
com.google.protobuf.ByteString
getLanguageBytes();
// optional string country = 8;
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
boolean hasCountry();
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
java.lang.String getCountry();
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
com.google.protobuf.ByteString
getCountryBytes();
// optional string unity_version = 9;
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
boolean hasUnityVersion();
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
java.lang.String getUnityVersion();
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
com.google.protobuf.ByteString
getUnityVersionBytes();
// optional string unity_sdk_version = 10;
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
boolean hasUnitySdkVersion();
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
java.lang.String getUnitySdkVersion();
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
com.google.protobuf.ByteString
getUnitySdkVersionBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.VrClientInfo}
*
*
* Attributes specific to VR clients. These are applications built on top of
* the cross-platform Google Virtual Reality Toolkit (aka GVR). They run on a
* variety of platforms including Android Cardboard, IOS Cardboard, Oculus, etc
*
* This may be an Android phone for Cardboard, or may be a full VR headset.
*
* Next tag: 11
*
*/
public static final class VrClientInfo extends
com.google.protobuf.GeneratedMessage
implements VrClientInfoOrBuilder {
// Use VrClientInfo.newBuilder() to construct.
private VrClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private VrClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final VrClientInfo defaultInstance;
public static VrClientInfo getDefaultInstance() {
return defaultInstance;
}
public VrClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VrClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
vrClientType_ = value;
}
break;
}
case 18: {
bitField0_ |= 0x00000002;
sdkVersion_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
fingerprint_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
gvrVersion_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
manufacturer_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
model_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
language_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000080;
country_ = input.readBytes();
break;
}
case 74: {
bitField0_ |= 0x00000100;
unityVersion_ = input.readBytes();
break;
}
case 82: {
bitField0_ |= 0x00000200;
unitySdkVersion_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_VrClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_VrClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public VrClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VrClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.VrClientInfo.VrClientType}
*
*
* The type of VR client that made this request.
* Next enum tag: 6
*
*/
public enum VrClientType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*
*
* VR client type is unknown.
*
*/
UNKNOWN(0, 0),
/**
* ANDROID_CARDBOARD_SDK = 1;
*
*
* Client type is an Android device using the Cardboard SDK.
*
*/
ANDROID_CARDBOARD_SDK(1, 1),
/**
* IOS_CARDBOARD_SDK = 2;
*
*
* Client type is an iOS device using the Cardboard SDK.
*
*/
IOS_CARDBOARD_SDK(2, 2),
/**
* ANDROID_UNITY_SDK = 3;
*
*
* Client type is an Android device using Unity SDK.
*
*/
ANDROID_UNITY_SDK(3, 3),
/**
* IOS_UNITY_SDK = 4;
*
*
* Client type is an iOS device using Unity SDK.
*
*/
IOS_UNITY_SDK(4, 4),
/**
* WINDOWS = 5;
*
*
* Client type is a Windows machine (e.g. VrApp running Oculus or Valve).
*
*/
WINDOWS(5, 5),
;
/**
* UNKNOWN = 0;
*
*
* VR client type is unknown.
*
*/
public static final int UNKNOWN_VALUE = 0;
/**
* ANDROID_CARDBOARD_SDK = 1;
*
*
* Client type is an Android device using the Cardboard SDK.
*
*/
public static final int ANDROID_CARDBOARD_SDK_VALUE = 1;
/**
* IOS_CARDBOARD_SDK = 2;
*
*
* Client type is an iOS device using the Cardboard SDK.
*
*/
public static final int IOS_CARDBOARD_SDK_VALUE = 2;
/**
* ANDROID_UNITY_SDK = 3;
*
*
* Client type is an Android device using Unity SDK.
*
*/
public static final int ANDROID_UNITY_SDK_VALUE = 3;
/**
* IOS_UNITY_SDK = 4;
*
*
* Client type is an iOS device using Unity SDK.
*
*/
public static final int IOS_UNITY_SDK_VALUE = 4;
/**
* WINDOWS = 5;
*
*
* Client type is a Windows machine (e.g. VrApp running Oculus or Valve).
*
*/
public static final int WINDOWS_VALUE = 5;
public final int getNumber() { return value; }
public static VrClientType valueOf(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return ANDROID_CARDBOARD_SDK;
case 2: return IOS_CARDBOARD_SDK;
case 3: return ANDROID_UNITY_SDK;
case 4: return IOS_UNITY_SDK;
case 5: return WINDOWS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public VrClientType findValueByNumber(int number) {
return VrClientType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDescriptor().getEnumTypes().get(0);
}
private static final VrClientType[] VALUES = values();
public static VrClientType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private VrClientType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.VrClientInfo.VrClientType)
}
private int bitField0_;
// optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
public static final int VR_CLIENT_TYPE_FIELD_NUMBER = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType vrClientType_;
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public boolean hasVrClientType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType getVrClientType() {
return vrClientType_;
}
// optional string sdk_version = 2;
public static final int SDK_VERSION_FIELD_NUMBER = 2;
private java.lang.Object sdkVersion_;
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public boolean hasSdkVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public java.lang.String getSdkVersion() {
java.lang.Object ref = sdkVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sdkVersion_ = s;
}
return s;
}
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public com.google.protobuf.ByteString
getSdkVersionBytes() {
java.lang.Object ref = sdkVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string fingerprint = 3;
public static final int FINGERPRINT_FIELD_NUMBER = 3;
private java.lang.Object fingerprint_;
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fingerprint_ = s;
}
return s;
}
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string gvr_version = 4;
public static final int GVR_VERSION_FIELD_NUMBER = 4;
private java.lang.Object gvrVersion_;
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public boolean hasGvrVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public java.lang.String getGvrVersion() {
java.lang.Object ref = gvrVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
gvrVersion_ = s;
}
return s;
}
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public com.google.protobuf.ByteString
getGvrVersionBytes() {
java.lang.Object ref = gvrVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gvrVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string manufacturer = 5;
public static final int MANUFACTURER_FIELD_NUMBER = 5;
private java.lang.Object manufacturer_;
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public boolean hasManufacturer() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
manufacturer_ = s;
}
return s;
}
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string model = 6;
public static final int MODEL_FIELD_NUMBER = 6;
private java.lang.Object model_;
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
model_ = s;
}
return s;
}
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string language = 7;
public static final int LANGUAGE_FIELD_NUMBER = 7;
private java.lang.Object language_;
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string country = 8;
public static final int COUNTRY_FIELD_NUMBER = 8;
private java.lang.Object country_;
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string unity_version = 9;
public static final int UNITY_VERSION_FIELD_NUMBER = 9;
private java.lang.Object unityVersion_;
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public boolean hasUnityVersion() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public java.lang.String getUnityVersion() {
java.lang.Object ref = unityVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unityVersion_ = s;
}
return s;
}
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public com.google.protobuf.ByteString
getUnityVersionBytes() {
java.lang.Object ref = unityVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unityVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string unity_sdk_version = 10;
public static final int UNITY_SDK_VERSION_FIELD_NUMBER = 10;
private java.lang.Object unitySdkVersion_;
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public boolean hasUnitySdkVersion() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public java.lang.String getUnitySdkVersion() {
java.lang.Object ref = unitySdkVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
unitySdkVersion_ = s;
}
return s;
}
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public com.google.protobuf.ByteString
getUnitySdkVersionBytes() {
java.lang.Object ref = unitySdkVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unitySdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
vrClientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType.UNKNOWN;
sdkVersion_ = "";
fingerprint_ = "";
gvrVersion_ = "";
manufacturer_ = "";
model_ = "";
language_ = "";
country_ = "";
unityVersion_ = "";
unitySdkVersion_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, vrClientType_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getSdkVersionBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getFingerprintBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getGvrVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getManufacturerBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getModelBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, getLanguageBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, getCountryBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBytes(9, getUnityVersionBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(10, getUnitySdkVersionBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, vrClientType_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getSdkVersionBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getFingerprintBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getGvrVersionBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getManufacturerBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getModelBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getLanguageBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getCountryBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, getUnityVersionBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, getUnitySdkVersionBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.VrClientInfo}
*
*
* Attributes specific to VR clients. These are applications built on top of
* the cross-platform Google Virtual Reality Toolkit (aka GVR). They run on a
* variety of platforms including Android Cardboard, IOS Cardboard, Oculus, etc
*
* This may be an Android phone for Cardboard, or may be a full VR headset.
*
* Next tag: 11
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_VrClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_VrClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
vrClientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType.UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000001);
sdkVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
fingerprint_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
gvrVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
manufacturer_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
model_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
language_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
country_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
unityVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
unitySdkVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_VrClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.vrClientType_ = vrClientType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.sdkVersion_ = sdkVersion_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.fingerprint_ = fingerprint_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.gvrVersion_ = gvrVersion_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.manufacturer_ = manufacturer_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.model_ = model_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.language_ = language_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.country_ = country_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.unityVersion_ = unityVersion_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.unitySdkVersion_ = unitySdkVersion_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance()) return this;
if (other.hasVrClientType()) {
setVrClientType(other.getVrClientType());
}
if (other.hasSdkVersion()) {
bitField0_ |= 0x00000002;
sdkVersion_ = other.sdkVersion_;
onChanged();
}
if (other.hasFingerprint()) {
bitField0_ |= 0x00000004;
fingerprint_ = other.fingerprint_;
onChanged();
}
if (other.hasGvrVersion()) {
bitField0_ |= 0x00000008;
gvrVersion_ = other.gvrVersion_;
onChanged();
}
if (other.hasManufacturer()) {
bitField0_ |= 0x00000010;
manufacturer_ = other.manufacturer_;
onChanged();
}
if (other.hasModel()) {
bitField0_ |= 0x00000020;
model_ = other.model_;
onChanged();
}
if (other.hasLanguage()) {
bitField0_ |= 0x00000040;
language_ = other.language_;
onChanged();
}
if (other.hasCountry()) {
bitField0_ |= 0x00000080;
country_ = other.country_;
onChanged();
}
if (other.hasUnityVersion()) {
bitField0_ |= 0x00000100;
unityVersion_ = other.unityVersion_;
onChanged();
}
if (other.hasUnitySdkVersion()) {
bitField0_ |= 0x00000200;
unitySdkVersion_ = other.unitySdkVersion_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType vrClientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType.UNKNOWN;
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public boolean hasVrClientType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType getVrClientType() {
return vrClientType_;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public Builder setVrClientType(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
vrClientType_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo.VrClientType vr_client_type = 1;
*
*
* The VR client type for this client. One of the enum values defined above.
*
*/
public Builder clearVrClientType() {
bitField0_ = (bitField0_ & ~0x00000001);
vrClientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.VrClientType.UNKNOWN;
onChanged();
return this;
}
// optional string sdk_version = 2;
private java.lang.Object sdkVersion_ = "";
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public boolean hasSdkVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public java.lang.String getSdkVersion() {
java.lang.Object ref = sdkVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
sdkVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public com.google.protobuf.ByteString
getSdkVersionBytes() {
java.lang.Object ref = sdkVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public Builder setSdkVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
sdkVersion_ = value;
onChanged();
return this;
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public Builder clearSdkVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
sdkVersion_ = getDefaultInstance().getSdkVersion();
onChanged();
return this;
}
/**
* optional string sdk_version = 2;
*
*
* The VR client OS SDK version, in a format appropriate for the platform.
*
*/
public Builder setSdkVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
sdkVersion_ = value;
onChanged();
return this;
}
// optional string fingerprint = 3;
private java.lang.Object fingerprint_ = "";
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public Builder setFingerprint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fingerprint_ = value;
onChanged();
return this;
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public Builder clearFingerprint() {
bitField0_ = (bitField0_ & ~0x00000004);
fingerprint_ = getDefaultInstance().getFingerprint();
onChanged();
return this;
}
/**
* optional string fingerprint = 3;
*
*
* The VR device build fingerprint, in a format appropriate for the platform.
*
*/
public Builder setFingerprintBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fingerprint_ = value;
onChanged();
return this;
}
// optional string gvr_version = 4;
private java.lang.Object gvrVersion_ = "";
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public boolean hasGvrVersion() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public java.lang.String getGvrVersion() {
java.lang.Object ref = gvrVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
gvrVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public com.google.protobuf.ByteString
getGvrVersionBytes() {
java.lang.Object ref = gvrVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gvrVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public Builder setGvrVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
gvrVersion_ = value;
onChanged();
return this;
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public Builder clearGvrVersion() {
bitField0_ = (bitField0_ & ~0x00000008);
gvrVersion_ = getDefaultInstance().getGvrVersion();
onChanged();
return this;
}
/**
* optional string gvr_version = 4;
*
*
* Version of the Google VR Toolkit (aka GVR).
*
*/
public Builder setGvrVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
gvrVersion_ = value;
onChanged();
return this;
}
// optional string manufacturer = 5;
private java.lang.Object manufacturer_ = "";
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public boolean hasManufacturer() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public java.lang.String getManufacturer() {
java.lang.Object ref = manufacturer_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
manufacturer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public com.google.protobuf.ByteString
getManufacturerBytes() {
java.lang.Object ref = manufacturer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
manufacturer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public Builder setManufacturer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
manufacturer_ = value;
onChanged();
return this;
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public Builder clearManufacturer() {
bitField0_ = (bitField0_ & ~0x00000010);
manufacturer_ = getDefaultInstance().getManufacturer();
onChanged();
return this;
}
/**
* optional string manufacturer = 5;
*
*
* The manufacturer of the client, for example Samsung.
*
*/
public Builder setManufacturerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
manufacturer_ = value;
onChanged();
return this;
}
// optional string model = 6;
private java.lang.Object model_ = "";
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public boolean hasModel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
model_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public Builder setModel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
model_ = value;
onChanged();
return this;
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public Builder clearModel() {
bitField0_ = (bitField0_ & ~0x00000020);
model_ = getDefaultInstance().getModel();
onChanged();
return this;
}
/**
* optional string model = 6;
*
*
* The model of the client, for example Nexus 6.
*
*/
public Builder setModelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
model_ = value;
onChanged();
return this;
}
// optional string language = 7;
private java.lang.Object language_ = "";
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
language_ = value;
onChanged();
return this;
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public Builder clearLanguage() {
bitField0_ = (bitField0_ & ~0x00000040);
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* optional string language = 7;
*
*
* The chosen language from the client. e.g., "en", "ko". We originally
* logged locale, but after a privacy review switched to only log language.
*
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
language_ = value;
onChanged();
return this;
}
// optional string country = 8;
private java.lang.Object country_ = "";
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00000080);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 8;
*
*
* The chosen country from the client. e.g., "US", "KR", "JP".
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
country_ = value;
onChanged();
return this;
}
// optional string unity_version = 9;
private java.lang.Object unityVersion_ = "";
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public boolean hasUnityVersion() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public java.lang.String getUnityVersion() {
java.lang.Object ref = unityVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
unityVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public com.google.protobuf.ByteString
getUnityVersionBytes() {
java.lang.Object ref = unityVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unityVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public Builder setUnityVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
unityVersion_ = value;
onChanged();
return this;
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public Builder clearUnityVersion() {
bitField0_ = (bitField0_ & ~0x00000100);
unityVersion_ = getDefaultInstance().getUnityVersion();
onChanged();
return this;
}
/**
* optional string unity_version = 9;
*
*
* The version of the Unity runtime.
*
*/
public Builder setUnityVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
unityVersion_ = value;
onChanged();
return this;
}
// optional string unity_sdk_version = 10;
private java.lang.Object unitySdkVersion_ = "";
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public boolean hasUnitySdkVersion() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public java.lang.String getUnitySdkVersion() {
java.lang.Object ref = unitySdkVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
unitySdkVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public com.google.protobuf.ByteString
getUnitySdkVersionBytes() {
java.lang.Object ref = unitySdkVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
unitySdkVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public Builder setUnitySdkVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
unitySdkVersion_ = value;
onChanged();
return this;
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public Builder clearUnitySdkVersion() {
bitField0_ = (bitField0_ & ~0x00000200);
unitySdkVersion_ = getDefaultInstance().getUnitySdkVersion();
onChanged();
return this;
}
/**
* optional string unity_sdk_version = 10;
*
*
* The version of Google's VR Unity SDK.
*
*/
public Builder setUnitySdkVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
unitySdkVersion_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.VrClientInfo)
}
static {
defaultInstance = new VrClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.VrClientInfo)
}
public interface JsClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string os = 1;
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
boolean hasOs();
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
java.lang.String getOs();
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
com.google.protobuf.ByteString
getOsBytes();
// optional string os_version = 2;
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
boolean hasOsVersion();
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
java.lang.String getOsVersion();
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
com.google.protobuf.ByteString
getOsVersionBytes();
// optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
boolean hasDeviceType();
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType getDeviceType();
// optional string country = 4;
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
boolean hasCountry();
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
java.lang.String getCountry();
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
com.google.protobuf.ByteString
getCountryBytes();
// optional string locale = 5;
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
boolean hasLocale();
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
java.lang.String getLocale();
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
com.google.protobuf.ByteString
getLocaleBytes();
// optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
boolean hasOsType();
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType getOsType();
}
/**
* Protobuf type {@code wireless_android_play_playlog.JsClientInfo}
*
*
* Attributes specific to JavaScript clients.
*
* Next tag: 7
*
*/
public static final class JsClientInfo extends
com.google.protobuf.GeneratedMessage
implements JsClientInfoOrBuilder {
// Use JsClientInfo.newBuilder() to construct.
private JsClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private JsClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final JsClientInfo defaultInstance;
public static JsClientInfo getDefaultInstance() {
return defaultInstance;
}
public JsClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JsClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
os_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
osVersion_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
deviceType_ = value;
}
break;
}
case 34: {
bitField0_ |= 0x00000008;
country_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
locale_ = input.readBytes();
break;
}
case 48: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(6, rawValue);
} else {
bitField0_ |= 0x00000020;
osType_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_JsClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_JsClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public JsClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JsClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.JsClientInfo.OsType}
*/
public enum OsType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OS_UNKNOWN = 0;
*/
OS_UNKNOWN(0, 0),
/**
* MAC = 1;
*/
MAC(1, 1),
/**
* WINDOWS = 2;
*/
WINDOWS(2, 2),
/**
* ANDROID = 3;
*/
ANDROID(3, 3),
/**
* LINUX = 4;
*/
LINUX(4, 4),
/**
* CHROME_OS = 5;
*/
CHROME_OS(5, 5),
/**
* IPAD = 6;
*/
IPAD(6, 6),
/**
* IPHONE = 7;
*/
IPHONE(7, 7),
/**
* IPOD = 8;
*/
IPOD(8, 8),
;
/**
* OS_UNKNOWN = 0;
*/
public static final int OS_UNKNOWN_VALUE = 0;
/**
* MAC = 1;
*/
public static final int MAC_VALUE = 1;
/**
* WINDOWS = 2;
*/
public static final int WINDOWS_VALUE = 2;
/**
* ANDROID = 3;
*/
public static final int ANDROID_VALUE = 3;
/**
* LINUX = 4;
*/
public static final int LINUX_VALUE = 4;
/**
* CHROME_OS = 5;
*/
public static final int CHROME_OS_VALUE = 5;
/**
* IPAD = 6;
*/
public static final int IPAD_VALUE = 6;
/**
* IPHONE = 7;
*/
public static final int IPHONE_VALUE = 7;
/**
* IPOD = 8;
*/
public static final int IPOD_VALUE = 8;
public final int getNumber() { return value; }
public static OsType valueOf(int value) {
switch (value) {
case 0: return OS_UNKNOWN;
case 1: return MAC;
case 2: return WINDOWS;
case 3: return ANDROID;
case 4: return LINUX;
case 5: return CHROME_OS;
case 6: return IPAD;
case 7: return IPHONE;
case 8: return IPOD;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OsType findValueByNumber(int number) {
return OsType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDescriptor().getEnumTypes().get(0);
}
private static final OsType[] VALUES = values();
public static OsType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private OsType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.JsClientInfo.OsType)
}
/**
* Protobuf enum {@code wireless_android_play_playlog.JsClientInfo.DeviceType}
*/
public enum DeviceType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0, 0),
/**
* MOBILE = 1;
*/
MOBILE(1, 1),
/**
* TABLET = 2;
*/
TABLET(2, 2),
/**
* DESKTOP = 3;
*/
DESKTOP(3, 3),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* MOBILE = 1;
*/
public static final int MOBILE_VALUE = 1;
/**
* TABLET = 2;
*/
public static final int TABLET_VALUE = 2;
/**
* DESKTOP = 3;
*/
public static final int DESKTOP_VALUE = 3;
public final int getNumber() { return value; }
public static DeviceType valueOf(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return MOBILE;
case 2: return TABLET;
case 3: return DESKTOP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DeviceType findValueByNumber(int number) {
return DeviceType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDescriptor().getEnumTypes().get(1);
}
private static final DeviceType[] VALUES = values();
public static DeviceType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private DeviceType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.JsClientInfo.DeviceType)
}
private int bitField0_;
// optional string os = 1;
public static final int OS_FIELD_NUMBER = 1;
private java.lang.Object os_;
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
os_ = s;
}
return s;
}
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os_version = 2;
public static final int OS_VERSION_FIELD_NUMBER = 2;
private java.lang.Object osVersion_;
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public boolean hasOsVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osVersion_ = s;
}
return s;
}
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
public static final int DEVICE_TYPE_FIELD_NUMBER = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType deviceType_;
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public boolean hasDeviceType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType getDeviceType() {
return deviceType_;
}
// optional string country = 4;
public static final int COUNTRY_FIELD_NUMBER = 4;
private java.lang.Object country_;
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
country_ = s;
}
return s;
}
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string locale = 5;
public static final int LOCALE_FIELD_NUMBER = 5;
private java.lang.Object locale_;
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
locale_ = s;
}
return s;
}
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
public static final int OS_TYPE_FIELD_NUMBER = 6;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType osType_;
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public boolean hasOsType() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType getOsType() {
return osType_;
}
private void initFields() {
os_ = "";
osVersion_ = "";
deviceType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType.UNKNOWN;
country_ = "";
locale_ = "";
osType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType.OS_UNKNOWN;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getOsBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getOsVersionBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, deviceType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getCountryBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getLocaleBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeEnum(6, osType_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getOsBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getOsVersionBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, deviceType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getCountryBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getLocaleBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, osType_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.JsClientInfo}
*
*
* Attributes specific to JavaScript clients.
*
* Next tag: 7
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_JsClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_JsClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
os_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
osVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
deviceType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType.UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000004);
country_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
locale_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
osType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType.OS_UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_JsClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.os_ = os_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.osVersion_ = osVersion_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.deviceType_ = deviceType_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.country_ = country_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.locale_ = locale_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.osType_ = osType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance()) return this;
if (other.hasOs()) {
bitField0_ |= 0x00000001;
os_ = other.os_;
onChanged();
}
if (other.hasOsVersion()) {
bitField0_ |= 0x00000002;
osVersion_ = other.osVersion_;
onChanged();
}
if (other.hasDeviceType()) {
setDeviceType(other.getDeviceType());
}
if (other.hasCountry()) {
bitField0_ |= 0x00000008;
country_ = other.country_;
onChanged();
}
if (other.hasLocale()) {
bitField0_ |= 0x00000010;
locale_ = other.locale_;
onChanged();
}
if (other.hasOsType()) {
setOsType(other.getOsType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string os = 1;
private java.lang.Object os_ = "";
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
os_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder setOs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
os_ = value;
onChanged();
return this;
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder clearOs() {
bitField0_ = (bitField0_ & ~0x00000001);
os_ = getDefaultInstance().getOs();
onChanged();
return this;
}
/**
* optional string os = 1;
*
*
* e.g., "mac", "windows", "linux"
*
*/
public Builder setOsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
os_ = value;
onChanged();
return this;
}
// optional string os_version = 2;
private java.lang.Object osVersion_ = "";
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public boolean hasOsVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public Builder setOsVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
osVersion_ = value;
onChanged();
return this;
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public Builder clearOsVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
osVersion_ = getDefaultInstance().getOsVersion();
onChanged();
return this;
}
/**
* optional string os_version = 2;
*
*
* The OS version (not available for everything).
*
*/
public Builder setOsVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
osVersion_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType deviceType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType.UNKNOWN;
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public boolean hasDeviceType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType getDeviceType() {
return deviceType_;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public Builder setDeviceType(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
deviceType_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.DeviceType device_type = 3;
*/
public Builder clearDeviceType() {
bitField0_ = (bitField0_ & ~0x00000004);
deviceType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.DeviceType.UNKNOWN;
onChanged();
return this;
}
// optional string country = 4;
private java.lang.Object country_ = "";
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public boolean hasCountry() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public java.lang.String getCountry() {
java.lang.Object ref = country_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
country_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public com.google.protobuf.ByteString
getCountryBytes() {
java.lang.Object ref = country_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
country_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public Builder setCountry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
country_ = value;
onChanged();
return this;
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public Builder clearCountry() {
bitField0_ = (bitField0_ & ~0x00000008);
country_ = getDefaultInstance().getCountry();
onChanged();
return this;
}
/**
* optional string country = 4;
*
*
* The country from the client. e.g., "US", "KR", "JP".
* Only populated if the client explicitly sets it.
*
*/
public Builder setCountryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
country_ = value;
onChanged();
return this;
}
// optional string locale = 5;
private java.lang.Object locale_ = "";
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public boolean hasLocale() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public java.lang.String getLocale() {
java.lang.Object ref = locale_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
locale_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public com.google.protobuf.ByteString
getLocaleBytes() {
java.lang.Object ref = locale_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
locale_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public Builder setLocale(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
locale_ = value;
onChanged();
return this;
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public Builder clearLocale() {
bitField0_ = (bitField0_ & ~0x00000010);
locale_ = getDefaultInstance().getLocale();
onChanged();
return this;
}
/**
* optional string locale = 5;
*
*
* The locale used to display the page, e.g., "de_DE", "en", "en_US"; this may
* differ from the browser's locale if the user overrode the language
* settings.
* Only populated if the client explicitly sets it.
*
*/
public Builder setLocaleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
locale_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType osType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType.OS_UNKNOWN;
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public boolean hasOsType() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType getOsType() {
return osType_;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public Builder setOsType(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
osType_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo.OsType os_type = 6;
*
*
* e.g., MAC, WINDOWS, ANDROID etc.
*
*/
public Builder clearOsType() {
bitField0_ = (bitField0_ & ~0x00000020);
osType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.OsType.OS_UNKNOWN;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.JsClientInfo)
}
static {
defaultInstance = new JsClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.JsClientInfo)
}
public interface ClientInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
boolean hasClientType();
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType getClientType();
// optional string remote_host = 6;
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
boolean hasRemoteHost();
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
java.lang.String getRemoteHost();
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
com.google.protobuf.ByteString
getRemoteHostBytes();
// optional string remote_host6 = 7;
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
boolean hasRemoteHost6();
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
java.lang.String getRemoteHost6();
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
com.google.protobuf.ByteString
getRemoteHost6Bytes();
// optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
boolean hasAndroidClientInfo();
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo getAndroidClientInfo();
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder getAndroidClientInfoOrBuilder();
// optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
boolean hasDesktopClientInfo();
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo getDesktopClientInfo();
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder getDesktopClientInfoOrBuilder();
// optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
boolean hasIosClientInfo();
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo getIosClientInfo();
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder getIosClientInfoOrBuilder();
// optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
boolean hasPlayCeClientInfo();
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo getPlayCeClientInfo();
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder getPlayCeClientInfoOrBuilder();
// optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
boolean hasVrClientInfo();
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo getVrClientInfo();
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder getVrClientInfoOrBuilder();
// optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
boolean hasPancettaClientInfo();
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo getPancettaClientInfo();
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder getPancettaClientInfoOrBuilder();
// optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
boolean hasBrowserInfo();
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo getBrowserInfo();
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder getBrowserInfoOrBuilder();
// optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
boolean hasJsClientInfo();
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo getJsClientInfo();
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder getJsClientInfoOrBuilder();
}
/**
* Protobuf type {@code wireless_android_play_playlog.ClientInfo}
*
*
* Next tag: 12
*
*/
public static final class ClientInfo extends
com.google.protobuf.GeneratedMessage
implements ClientInfoOrBuilder {
// Use ClientInfo.newBuilder() to construct.
private ClientInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ClientInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ClientInfo defaultInstance;
public static ClientInfo getDefaultInstance() {
return defaultInstance;
}
public ClientInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ClientInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
clientType_ = value;
}
break;
}
case 18: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = androidClientInfo_.toBuilder();
}
androidClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(androidClientInfo_);
androidClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 26: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = desktopClientInfo_.toBuilder();
}
desktopClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(desktopClientInfo_);
desktopClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 34: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = iosClientInfo_.toBuilder();
}
iosClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(iosClientInfo_);
iosClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 42: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = playCeClientInfo_.toBuilder();
}
playCeClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(playCeClientInfo_);
playCeClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 50: {
bitField0_ |= 0x00000002;
remoteHost_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000004;
remoteHost6_ = input.readBytes();
break;
}
case 66: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = vrClientInfo_.toBuilder();
}
vrClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(vrClientInfo_);
vrClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 74: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = browserInfo_.toBuilder();
}
browserInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(browserInfo_);
browserInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 82: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = pancettaClientInfo_.toBuilder();
}
pancettaClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pancettaClientInfo_);
pancettaClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 90: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
subBuilder = jsClientInfo_.toBuilder();
}
jsClientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jsClientInfo_);
jsClientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ClientInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ClientInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.ClientInfo.ClientType}
*
*
* The type of client that made this request.
* Next enum tag: 10
*
*/
public enum ClientType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*
*
* Client type is unknown.
*
*/
UNKNOWN(0, 0),
/**
* JS = 1;
*
*
* Client type is Javascript.
*
*/
JS(1, 1),
/**
* DESKTOP = 2;
*
*
* Desktop client type.
*
*/
DESKTOP(2, 2),
/**
* IOS = 3;
*
*
* iOS client type.
*
*/
IOS(3, 3),
/**
* ANDROID = 4;
*
*
* Android client type.
*
*/
ANDROID(4, 4),
/**
* PLAY_CE = 5;
*
*
* This is to be used by third party consumer electronics devices
* with GooglePlay apps, e.g.: Roku, Smart TVs etc.
*
*/
PLAY_CE(5, 5),
/**
* PYTHON = 6;
*
*
* Python client type.
*
*/
PYTHON(6, 6),
/**
* VR = 7;
*
*
* VR client type.
*
*/
VR(7, 7),
/**
* PANCETTA = 8;
*
*
* Pancetta client type. go/pancetta
*
*/
PANCETTA(8, 8),
/**
* DRIVE_FS = 9;
*
*
* DriveFS client type. go/drive-fs
*
*/
DRIVE_FS(9, 9),
;
/**
* UNKNOWN = 0;
*
*
* Client type is unknown.
*
*/
public static final int UNKNOWN_VALUE = 0;
/**
* JS = 1;
*
*
* Client type is Javascript.
*
*/
public static final int JS_VALUE = 1;
/**
* DESKTOP = 2;
*
*
* Desktop client type.
*
*/
public static final int DESKTOP_VALUE = 2;
/**
* IOS = 3;
*
*
* iOS client type.
*
*/
public static final int IOS_VALUE = 3;
/**
* ANDROID = 4;
*
*
* Android client type.
*
*/
public static final int ANDROID_VALUE = 4;
/**
* PLAY_CE = 5;
*
*
* This is to be used by third party consumer electronics devices
* with GooglePlay apps, e.g.: Roku, Smart TVs etc.
*
*/
public static final int PLAY_CE_VALUE = 5;
/**
* PYTHON = 6;
*
*
* Python client type.
*
*/
public static final int PYTHON_VALUE = 6;
/**
* VR = 7;
*
*
* VR client type.
*
*/
public static final int VR_VALUE = 7;
/**
* PANCETTA = 8;
*
*
* Pancetta client type. go/pancetta
*
*/
public static final int PANCETTA_VALUE = 8;
/**
* DRIVE_FS = 9;
*
*
* DriveFS client type. go/drive-fs
*
*/
public static final int DRIVE_FS_VALUE = 9;
public final int getNumber() { return value; }
public static ClientType valueOf(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return JS;
case 2: return DESKTOP;
case 3: return IOS;
case 4: return ANDROID;
case 5: return PLAY_CE;
case 6: return PYTHON;
case 7: return VR;
case 8: return PANCETTA;
case 9: return DRIVE_FS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ClientType findValueByNumber(int number) {
return ClientType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDescriptor().getEnumTypes().get(0);
}
private static final ClientType[] VALUES = values();
public static ClientType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ClientType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.ClientInfo.ClientType)
}
private int bitField0_;
// optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
public static final int CLIENT_TYPE_FIELD_NUMBER = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType clientType_;
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public boolean hasClientType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType getClientType() {
return clientType_;
}
// optional string remote_host = 6;
public static final int REMOTE_HOST_FIELD_NUMBER = 6;
private java.lang.Object remoteHost_;
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public boolean hasRemoteHost() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public java.lang.String getRemoteHost() {
java.lang.Object ref = remoteHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
remoteHost_ = s;
}
return s;
}
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public com.google.protobuf.ByteString
getRemoteHostBytes() {
java.lang.Object ref = remoteHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string remote_host6 = 7;
public static final int REMOTE_HOST6_FIELD_NUMBER = 7;
private java.lang.Object remoteHost6_;
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public boolean hasRemoteHost6() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public java.lang.String getRemoteHost6() {
java.lang.Object ref = remoteHost6_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
remoteHost6_ = s;
}
return s;
}
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public com.google.protobuf.ByteString
getRemoteHost6Bytes() {
java.lang.Object ref = remoteHost6_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteHost6_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
public static final int ANDROID_CLIENT_INFO_FIELD_NUMBER = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo androidClientInfo_;
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public boolean hasAndroidClientInfo() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo getAndroidClientInfo() {
return androidClientInfo_;
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder getAndroidClientInfoOrBuilder() {
return androidClientInfo_;
}
// optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
public static final int DESKTOP_CLIENT_INFO_FIELD_NUMBER = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo desktopClientInfo_;
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public boolean hasDesktopClientInfo() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo getDesktopClientInfo() {
return desktopClientInfo_;
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder getDesktopClientInfoOrBuilder() {
return desktopClientInfo_;
}
// optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
public static final int IOS_CLIENT_INFO_FIELD_NUMBER = 4;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo iosClientInfo_;
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public boolean hasIosClientInfo() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo getIosClientInfo() {
return iosClientInfo_;
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder getIosClientInfoOrBuilder() {
return iosClientInfo_;
}
// optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
public static final int PLAY_CE_CLIENT_INFO_FIELD_NUMBER = 5;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo playCeClientInfo_;
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public boolean hasPlayCeClientInfo() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo getPlayCeClientInfo() {
return playCeClientInfo_;
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder getPlayCeClientInfoOrBuilder() {
return playCeClientInfo_;
}
// optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
public static final int VR_CLIENT_INFO_FIELD_NUMBER = 8;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo vrClientInfo_;
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public boolean hasVrClientInfo() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo getVrClientInfo() {
return vrClientInfo_;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder getVrClientInfoOrBuilder() {
return vrClientInfo_;
}
// optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
public static final int PANCETTA_CLIENT_INFO_FIELD_NUMBER = 10;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo pancettaClientInfo_;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public boolean hasPancettaClientInfo() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo getPancettaClientInfo() {
return pancettaClientInfo_;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder getPancettaClientInfoOrBuilder() {
return pancettaClientInfo_;
}
// optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
public static final int BROWSER_INFO_FIELD_NUMBER = 9;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo browserInfo_;
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public boolean hasBrowserInfo() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo getBrowserInfo() {
return browserInfo_;
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder getBrowserInfoOrBuilder() {
return browserInfo_;
}
// optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
public static final int JS_CLIENT_INFO_FIELD_NUMBER = 11;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo jsClientInfo_;
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public boolean hasJsClientInfo() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo getJsClientInfo() {
return jsClientInfo_;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder getJsClientInfoOrBuilder() {
return jsClientInfo_;
}
private void initFields() {
clientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType.UNKNOWN;
remoteHost_ = "";
remoteHost6_ = "";
androidClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance();
desktopClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance();
iosClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance();
playCeClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance();
vrClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance();
pancettaClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance();
browserInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance();
jsClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, clientType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(2, androidClientInfo_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(3, desktopClientInfo_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(4, iosClientInfo_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(5, playCeClientInfo_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(6, getRemoteHostBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(7, getRemoteHost6Bytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(8, vrClientInfo_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(9, browserInfo_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(10, pancettaClientInfo_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeMessage(11, jsClientInfo_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, clientType_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, androidClientInfo_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, desktopClientInfo_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, iosClientInfo_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, playCeClientInfo_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getRemoteHostBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getRemoteHost6Bytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, vrClientInfo_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, browserInfo_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, pancettaClientInfo_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, jsClientInfo_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.ClientInfo}
*
*
* Next tag: 12
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ClientInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ClientInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getAndroidClientInfoFieldBuilder();
getDesktopClientInfoFieldBuilder();
getIosClientInfoFieldBuilder();
getPlayCeClientInfoFieldBuilder();
getVrClientInfoFieldBuilder();
getPancettaClientInfoFieldBuilder();
getBrowserInfoFieldBuilder();
getJsClientInfoFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType.UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000001);
remoteHost_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
remoteHost6_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
if (androidClientInfoBuilder_ == null) {
androidClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance();
} else {
androidClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (desktopClientInfoBuilder_ == null) {
desktopClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance();
} else {
desktopClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (iosClientInfoBuilder_ == null) {
iosClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance();
} else {
iosClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (playCeClientInfoBuilder_ == null) {
playCeClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance();
} else {
playCeClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (vrClientInfoBuilder_ == null) {
vrClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance();
} else {
vrClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (pancettaClientInfoBuilder_ == null) {
pancettaClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance();
} else {
pancettaClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (browserInfoBuilder_ == null) {
browserInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance();
} else {
browserInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (jsClientInfoBuilder_ == null) {
jsClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance();
} else {
jsClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ClientInfo_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clientType_ = clientType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.remoteHost_ = remoteHost_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.remoteHost6_ = remoteHost6_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (androidClientInfoBuilder_ == null) {
result.androidClientInfo_ = androidClientInfo_;
} else {
result.androidClientInfo_ = androidClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (desktopClientInfoBuilder_ == null) {
result.desktopClientInfo_ = desktopClientInfo_;
} else {
result.desktopClientInfo_ = desktopClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (iosClientInfoBuilder_ == null) {
result.iosClientInfo_ = iosClientInfo_;
} else {
result.iosClientInfo_ = iosClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (playCeClientInfoBuilder_ == null) {
result.playCeClientInfo_ = playCeClientInfo_;
} else {
result.playCeClientInfo_ = playCeClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
if (vrClientInfoBuilder_ == null) {
result.vrClientInfo_ = vrClientInfo_;
} else {
result.vrClientInfo_ = vrClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (pancettaClientInfoBuilder_ == null) {
result.pancettaClientInfo_ = pancettaClientInfo_;
} else {
result.pancettaClientInfo_ = pancettaClientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
if (browserInfoBuilder_ == null) {
result.browserInfo_ = browserInfo_;
} else {
result.browserInfo_ = browserInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
if (jsClientInfoBuilder_ == null) {
result.jsClientInfo_ = jsClientInfo_;
} else {
result.jsClientInfo_ = jsClientInfoBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance()) return this;
if (other.hasClientType()) {
setClientType(other.getClientType());
}
if (other.hasRemoteHost()) {
bitField0_ |= 0x00000002;
remoteHost_ = other.remoteHost_;
onChanged();
}
if (other.hasRemoteHost6()) {
bitField0_ |= 0x00000004;
remoteHost6_ = other.remoteHost6_;
onChanged();
}
if (other.hasAndroidClientInfo()) {
mergeAndroidClientInfo(other.getAndroidClientInfo());
}
if (other.hasDesktopClientInfo()) {
mergeDesktopClientInfo(other.getDesktopClientInfo());
}
if (other.hasIosClientInfo()) {
mergeIosClientInfo(other.getIosClientInfo());
}
if (other.hasPlayCeClientInfo()) {
mergePlayCeClientInfo(other.getPlayCeClientInfo());
}
if (other.hasVrClientInfo()) {
mergeVrClientInfo(other.getVrClientInfo());
}
if (other.hasPancettaClientInfo()) {
mergePancettaClientInfo(other.getPancettaClientInfo());
}
if (other.hasBrowserInfo()) {
mergeBrowserInfo(other.getBrowserInfo());
}
if (other.hasJsClientInfo()) {
mergeJsClientInfo(other.getJsClientInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType clientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType.UNKNOWN;
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public boolean hasClientType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType getClientType() {
return clientType_;
}
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public Builder setClientType(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
clientType_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.ClientInfo.ClientType client_type = 1;
*
*
* The client type for this client. One of the enum values defined above.
*
*/
public Builder clearClientType() {
bitField0_ = (bitField0_ & ~0x00000001);
clientType_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.ClientType.UNKNOWN;
onChanged();
return this;
}
// optional string remote_host = 6;
private java.lang.Object remoteHost_ = "";
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public boolean hasRemoteHost() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public java.lang.String getRemoteHost() {
java.lang.Object ref = remoteHost_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
remoteHost_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public com.google.protobuf.ByteString
getRemoteHostBytes() {
java.lang.Object ref = remoteHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder setRemoteHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
remoteHost_ = value;
onChanged();
return this;
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder clearRemoteHost() {
bitField0_ = (bitField0_ & ~0x00000002);
remoteHost_ = getDefaultInstance().getRemoteHost();
onChanged();
return this;
}
/**
* optional string remote_host = 6;
*
*
* Identical to GWSLogEntryProto.RemoteHost.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder setRemoteHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
remoteHost_ = value;
onChanged();
return this;
}
// optional string remote_host6 = 7;
private java.lang.Object remoteHost6_ = "";
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public boolean hasRemoteHost6() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public java.lang.String getRemoteHost6() {
java.lang.Object ref = remoteHost6_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
remoteHost6_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public com.google.protobuf.ByteString
getRemoteHost6Bytes() {
java.lang.Object ref = remoteHost6_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteHost6_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder setRemoteHost6(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
remoteHost6_ = value;
onChanged();
return this;
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder clearRemoteHost6() {
bitField0_ = (bitField0_ & ~0x00000004);
remoteHost6_ = getDefaultInstance().getRemoteHost6();
onChanged();
return this;
}
/**
* optional string remote_host6 = 7;
*
*
* Identical to GWSLogEntryProto.RemoteHost6.
* Set by the Clearcut server when ClientInfo is written to Footprints.
* Do not set this value in client code; the server will ignore it.
*
*/
public Builder setRemoteHost6Bytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
remoteHost6_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo androidClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder> androidClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public boolean hasAndroidClientInfo() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo getAndroidClientInfo() {
if (androidClientInfoBuilder_ == null) {
return androidClientInfo_;
} else {
return androidClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public Builder setAndroidClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo value) {
if (androidClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
androidClientInfo_ = value;
onChanged();
} else {
androidClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public Builder setAndroidClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder builderForValue) {
if (androidClientInfoBuilder_ == null) {
androidClientInfo_ = builderForValue.build();
onChanged();
} else {
androidClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public Builder mergeAndroidClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo value) {
if (androidClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
androidClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance()) {
androidClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.newBuilder(androidClientInfo_).mergeFrom(value).buildPartial();
} else {
androidClientInfo_ = value;
}
onChanged();
} else {
androidClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public Builder clearAndroidClientInfo() {
if (androidClientInfoBuilder_ == null) {
androidClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.getDefaultInstance();
onChanged();
} else {
androidClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder getAndroidClientInfoBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getAndroidClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder getAndroidClientInfoOrBuilder() {
if (androidClientInfoBuilder_ != null) {
return androidClientInfoBuilder_.getMessageOrBuilder();
} else {
return androidClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.AndroidClientInfo android_client_info = 2;
*
*
* Only one of the following *_client_info fields will exist in any request.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder>
getAndroidClientInfoFieldBuilder() {
if (androidClientInfoBuilder_ == null) {
androidClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AndroidClientInfoOrBuilder>(
androidClientInfo_,
getParentForChildren(),
isClean());
androidClientInfo_ = null;
}
return androidClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo desktopClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder> desktopClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public boolean hasDesktopClientInfo() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo getDesktopClientInfo() {
if (desktopClientInfoBuilder_ == null) {
return desktopClientInfo_;
} else {
return desktopClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public Builder setDesktopClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo value) {
if (desktopClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
desktopClientInfo_ = value;
onChanged();
} else {
desktopClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public Builder setDesktopClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder builderForValue) {
if (desktopClientInfoBuilder_ == null) {
desktopClientInfo_ = builderForValue.build();
onChanged();
} else {
desktopClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public Builder mergeDesktopClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo value) {
if (desktopClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
desktopClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance()) {
desktopClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.newBuilder(desktopClientInfo_).mergeFrom(value).buildPartial();
} else {
desktopClientInfo_ = value;
}
onChanged();
} else {
desktopClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public Builder clearDesktopClientInfo() {
if (desktopClientInfoBuilder_ == null) {
desktopClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.getDefaultInstance();
onChanged();
} else {
desktopClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder getDesktopClientInfoBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getDesktopClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder getDesktopClientInfoOrBuilder() {
if (desktopClientInfoBuilder_ != null) {
return desktopClientInfoBuilder_.getMessageOrBuilder();
} else {
return desktopClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.DesktopClientInfo desktop_client_info = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder>
getDesktopClientInfoFieldBuilder() {
if (desktopClientInfoBuilder_ == null) {
desktopClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DesktopClientInfoOrBuilder>(
desktopClientInfo_,
getParentForChildren(),
isClean());
desktopClientInfo_ = null;
}
return desktopClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo iosClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder> iosClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public boolean hasIosClientInfo() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo getIosClientInfo() {
if (iosClientInfoBuilder_ == null) {
return iosClientInfo_;
} else {
return iosClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public Builder setIosClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo value) {
if (iosClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
iosClientInfo_ = value;
onChanged();
} else {
iosClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public Builder setIosClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder builderForValue) {
if (iosClientInfoBuilder_ == null) {
iosClientInfo_ = builderForValue.build();
onChanged();
} else {
iosClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public Builder mergeIosClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo value) {
if (iosClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
iosClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance()) {
iosClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.newBuilder(iosClientInfo_).mergeFrom(value).buildPartial();
} else {
iosClientInfo_ = value;
}
onChanged();
} else {
iosClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public Builder clearIosClientInfo() {
if (iosClientInfoBuilder_ == null) {
iosClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.getDefaultInstance();
onChanged();
} else {
iosClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder getIosClientInfoBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getIosClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder getIosClientInfoOrBuilder() {
if (iosClientInfoBuilder_ != null) {
return iosClientInfoBuilder_.getMessageOrBuilder();
} else {
return iosClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.IosClientInfo ios_client_info = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder>
getIosClientInfoFieldBuilder() {
if (iosClientInfoBuilder_ == null) {
iosClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.IosClientInfoOrBuilder>(
iosClientInfo_,
getParentForChildren(),
isClean());
iosClientInfo_ = null;
}
return iosClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo playCeClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder> playCeClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public boolean hasPlayCeClientInfo() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo getPlayCeClientInfo() {
if (playCeClientInfoBuilder_ == null) {
return playCeClientInfo_;
} else {
return playCeClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public Builder setPlayCeClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo value) {
if (playCeClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
playCeClientInfo_ = value;
onChanged();
} else {
playCeClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public Builder setPlayCeClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder builderForValue) {
if (playCeClientInfoBuilder_ == null) {
playCeClientInfo_ = builderForValue.build();
onChanged();
} else {
playCeClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public Builder mergePlayCeClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo value) {
if (playCeClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
playCeClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance()) {
playCeClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.newBuilder(playCeClientInfo_).mergeFrom(value).buildPartial();
} else {
playCeClientInfo_ = value;
}
onChanged();
} else {
playCeClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public Builder clearPlayCeClientInfo() {
if (playCeClientInfoBuilder_ == null) {
playCeClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.getDefaultInstance();
onChanged();
} else {
playCeClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder getPlayCeClientInfoBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getPlayCeClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder getPlayCeClientInfoOrBuilder() {
if (playCeClientInfoBuilder_ != null) {
return playCeClientInfoBuilder_.getMessageOrBuilder();
} else {
return playCeClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.PlayCeClientInfo play_ce_client_info = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder>
getPlayCeClientInfoFieldBuilder() {
if (playCeClientInfoBuilder_ == null) {
playCeClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PlayCeClientInfoOrBuilder>(
playCeClientInfo_,
getParentForChildren(),
isClean());
playCeClientInfo_ = null;
}
return playCeClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo vrClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder> vrClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public boolean hasVrClientInfo() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo getVrClientInfo() {
if (vrClientInfoBuilder_ == null) {
return vrClientInfo_;
} else {
return vrClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public Builder setVrClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo value) {
if (vrClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vrClientInfo_ = value;
onChanged();
} else {
vrClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public Builder setVrClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder builderForValue) {
if (vrClientInfoBuilder_ == null) {
vrClientInfo_ = builderForValue.build();
onChanged();
} else {
vrClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public Builder mergeVrClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo value) {
if (vrClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
vrClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance()) {
vrClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.newBuilder(vrClientInfo_).mergeFrom(value).buildPartial();
} else {
vrClientInfo_ = value;
}
onChanged();
} else {
vrClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public Builder clearVrClientInfo() {
if (vrClientInfoBuilder_ == null) {
vrClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.getDefaultInstance();
onChanged();
} else {
vrClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder getVrClientInfoBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getVrClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder getVrClientInfoOrBuilder() {
if (vrClientInfoBuilder_ != null) {
return vrClientInfoBuilder_.getMessageOrBuilder();
} else {
return vrClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.VrClientInfo vr_client_info = 8;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder>
getVrClientInfoFieldBuilder() {
if (vrClientInfoBuilder_ == null) {
vrClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.VrClientInfoOrBuilder>(
vrClientInfo_,
getParentForChildren(),
isClean());
vrClientInfo_ = null;
}
return vrClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo pancettaClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder> pancettaClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public boolean hasPancettaClientInfo() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo getPancettaClientInfo() {
if (pancettaClientInfoBuilder_ == null) {
return pancettaClientInfo_;
} else {
return pancettaClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public Builder setPancettaClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo value) {
if (pancettaClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pancettaClientInfo_ = value;
onChanged();
} else {
pancettaClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public Builder setPancettaClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder builderForValue) {
if (pancettaClientInfoBuilder_ == null) {
pancettaClientInfo_ = builderForValue.build();
onChanged();
} else {
pancettaClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public Builder mergePancettaClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo value) {
if (pancettaClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
pancettaClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance()) {
pancettaClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.newBuilder(pancettaClientInfo_).mergeFrom(value).buildPartial();
} else {
pancettaClientInfo_ = value;
}
onChanged();
} else {
pancettaClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public Builder clearPancettaClientInfo() {
if (pancettaClientInfoBuilder_ == null) {
pancettaClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.getDefaultInstance();
onChanged();
} else {
pancettaClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder getPancettaClientInfoBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getPancettaClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder getPancettaClientInfoOrBuilder() {
if (pancettaClientInfoBuilder_ != null) {
return pancettaClientInfoBuilder_.getMessageOrBuilder();
} else {
return pancettaClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.PancettaClientInfo pancetta_client_info = 10;
*
*
* Pancetta contact: pancetta-cloud-eng@.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder>
getPancettaClientInfoFieldBuilder() {
if (pancettaClientInfoBuilder_ == null) {
pancettaClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.PancettaClientInfoOrBuilder>(
pancettaClientInfo_,
getParentForChildren(),
isClean());
pancettaClientInfo_ = null;
}
return pancettaClientInfoBuilder_;
}
// optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo browserInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder> browserInfoBuilder_;
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public boolean hasBrowserInfo() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo getBrowserInfo() {
if (browserInfoBuilder_ == null) {
return browserInfo_;
} else {
return browserInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public Builder setBrowserInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo value) {
if (browserInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
browserInfo_ = value;
onChanged();
} else {
browserInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public Builder setBrowserInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder builderForValue) {
if (browserInfoBuilder_ == null) {
browserInfo_ = builderForValue.build();
onChanged();
} else {
browserInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public Builder mergeBrowserInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo value) {
if (browserInfoBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
browserInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance()) {
browserInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.newBuilder(browserInfo_).mergeFrom(value).buildPartial();
} else {
browserInfo_ = value;
}
onChanged();
} else {
browserInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public Builder clearBrowserInfo() {
if (browserInfoBuilder_ == null) {
browserInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.getDefaultInstance();
onChanged();
} else {
browserInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder getBrowserInfoBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getBrowserInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder getBrowserInfoOrBuilder() {
if (browserInfoBuilder_ != null) {
return browserInfoBuilder_.getMessageOrBuilder();
} else {
return browserInfo_;
}
}
/**
* optional .wireless_android_play_playlog.BrowserInfo browser_info = 9;
*
*
* The browser used to make the request, if accessing through any web client.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder>
getBrowserInfoFieldBuilder() {
if (browserInfoBuilder_ == null) {
browserInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BrowserInfoOrBuilder>(
browserInfo_,
getParentForChildren(),
isClean());
browserInfo_ = null;
}
return browserInfoBuilder_;
}
// optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo jsClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder> jsClientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public boolean hasJsClientInfo() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo getJsClientInfo() {
if (jsClientInfoBuilder_ == null) {
return jsClientInfo_;
} else {
return jsClientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public Builder setJsClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo value) {
if (jsClientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jsClientInfo_ = value;
onChanged();
} else {
jsClientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public Builder setJsClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder builderForValue) {
if (jsClientInfoBuilder_ == null) {
jsClientInfo_ = builderForValue.build();
onChanged();
} else {
jsClientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public Builder mergeJsClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo value) {
if (jsClientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
jsClientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance()) {
jsClientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.newBuilder(jsClientInfo_).mergeFrom(value).buildPartial();
} else {
jsClientInfo_ = value;
}
onChanged();
} else {
jsClientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public Builder clearJsClientInfo() {
if (jsClientInfoBuilder_ == null) {
jsClientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.getDefaultInstance();
onChanged();
} else {
jsClientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder getJsClientInfoBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getJsClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder getJsClientInfoOrBuilder() {
if (jsClientInfoBuilder_ != null) {
return jsClientInfoBuilder_.getMessageOrBuilder();
} else {
return jsClientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.JsClientInfo js_client_info = 11;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder>
getJsClientInfoFieldBuilder() {
if (jsClientInfoBuilder_ == null) {
jsClientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.JsClientInfoOrBuilder>(
jsClientInfo_,
getParentForChildren(),
isClean());
jsClientInfo_ = null;
}
return jsClientInfoBuilder_;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.ClientInfo)
}
static {
defaultInstance = new ClientInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.ClientInfo)
}
public interface ExperimentIdListOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated string id = 1;
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
java.util.List
getIdList();
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
int getIdCount();
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
java.lang.String getId(int index);
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
com.google.protobuf.ByteString
getIdBytes(int index);
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExperimentIdList}
*
*
* The list of IDs of experiments that are currently on for this client. All
* other experiments are off.
*
* Next tag: 2
*
*/
public static final class ExperimentIdList extends
com.google.protobuf.GeneratedMessage
implements ExperimentIdListOrBuilder {
// Use ExperimentIdList.newBuilder() to construct.
private ExperimentIdList(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ExperimentIdList(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ExperimentIdList defaultInstance;
public static ExperimentIdList getDefaultInstance() {
return defaultInstance;
}
public ExperimentIdList getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExperimentIdList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
id_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
id_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
id_ = new com.google.protobuf.UnmodifiableLazyStringList(id_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIdList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ExperimentIdList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExperimentIdList(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated string id = 1;
public static final int ID_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList id_;
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public java.util.List
getIdList() {
return id_;
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public int getIdCount() {
return id_.size();
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public java.lang.String getId(int index) {
return id_.get(index);
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public com.google.protobuf.ByteString
getIdBytes(int index) {
return id_.getByteString(index);
}
private void initFields() {
id_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < id_.size(); i++) {
output.writeBytes(1, id_.getByteString(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < id_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(id_.getByteString(i));
}
size += dataSize;
size += 1 * getIdList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExperimentIdList}
*
*
* The list of IDs of experiments that are currently on for this client. All
* other experiments are off.
*
* Next tag: 2
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIdList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
id_ = new com.google.protobuf.UnmodifiableLazyStringList(
id_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.id_ = id_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance()) return this;
if (!other.id_.isEmpty()) {
if (id_.isEmpty()) {
id_ = other.id_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdIsMutable();
id_.addAll(other.id_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated string id = 1;
private com.google.protobuf.LazyStringList id_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
id_ = new com.google.protobuf.LazyStringArrayList(id_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public java.util.List
getIdList() {
return java.util.Collections.unmodifiableList(id_);
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public int getIdCount() {
return id_.size();
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public java.lang.String getId(int index) {
return id_.get(index);
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public com.google.protobuf.ByteString
getIdBytes(int index) {
return id_.getByteString(index);
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public Builder setId(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdIsMutable();
id_.set(index, value);
onChanged();
return this;
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public Builder addId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdIsMutable();
id_.add(value);
onChanged();
return this;
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public Builder addAllId(
java.lang.Iterable values) {
ensureIdIsMutable();
super.addAll(values, id_);
onChanged();
return this;
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public Builder clearId() {
id_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string id = 1;
*
*
* IDs are sorted and do not contain duplicates.
*
*/
public Builder addIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdIsMutable();
id_.add(value);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.ExperimentIdList)
}
static {
defaultInstance = new ExperimentIdList(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.ExperimentIdList)
}
public interface AppUsage1pLogEventOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int32 app_type = 1;
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
boolean hasAppType();
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
int getAppType();
// optional string android_package_name = 2;
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
boolean hasAndroidPackageName();
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
java.lang.String getAndroidPackageName();
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
com.google.protobuf.ByteString
getAndroidPackageNameBytes();
// optional string version = 3;
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
boolean hasVersion();
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
java.lang.String getVersion();
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
com.google.protobuf.ByteString
getVersionBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.AppUsage1pLogEvent}
*
*
* First party app usage log event extension.
* Used only when log_source = APP_USAGE_1P.
*
* TODO(jsw): Investigate whether anyone is actually using this, and if not,
* remove it.
*
* Next tag: 4
*
*/
public static final class AppUsage1pLogEvent extends
com.google.protobuf.GeneratedMessage
implements AppUsage1pLogEventOrBuilder {
// Use AppUsage1pLogEvent.newBuilder() to construct.
private AppUsage1pLogEvent(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private AppUsage1pLogEvent(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final AppUsage1pLogEvent defaultInstance;
public static AppUsage1pLogEvent getDefaultInstance() {
return defaultInstance;
}
public AppUsage1pLogEvent getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AppUsage1pLogEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
appType_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
androidPackageName_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
version_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public AppUsage1pLogEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AppUsage1pLogEvent(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int32 app_type = 1;
public static final int APP_TYPE_FIELD_NUMBER = 1;
private int appType_;
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public boolean hasAppType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public int getAppType() {
return appType_;
}
// optional string android_package_name = 2;
public static final int ANDROID_PACKAGE_NAME_FIELD_NUMBER = 2;
private java.lang.Object androidPackageName_;
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public boolean hasAndroidPackageName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public java.lang.String getAndroidPackageName() {
java.lang.Object ref = androidPackageName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
androidPackageName_ = s;
}
return s;
}
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public com.google.protobuf.ByteString
getAndroidPackageNameBytes() {
java.lang.Object ref = androidPackageName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
androidPackageName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string version = 3;
public static final int VERSION_FIELD_NUMBER = 3;
private java.lang.Object version_;
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
version_ = s;
}
return s;
}
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
appType_ = 0;
androidPackageName_ = "";
version_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, appType_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getAndroidPackageNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getVersionBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, appType_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getAndroidPackageNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getVersionBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.AppUsage1pLogEvent}
*
*
* First party app usage log event extension.
* Used only when log_source = APP_USAGE_1P.
*
* TODO(jsw): Investigate whether anyone is actually using this, and if not,
* remove it.
*
* Next tag: 4
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
appType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
androidPackageName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
version_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.appType_ = appType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.androidPackageName_ = androidPackageName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.version_ = version_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent.getDefaultInstance()) return this;
if (other.hasAppType()) {
setAppType(other.getAppType());
}
if (other.hasAndroidPackageName()) {
bitField0_ |= 0x00000002;
androidPackageName_ = other.androidPackageName_;
onChanged();
}
if (other.hasVersion()) {
bitField0_ |= 0x00000004;
version_ = other.version_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.AppUsage1pLogEvent) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int32 app_type = 1;
private int appType_ ;
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public boolean hasAppType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public int getAppType() {
return appType_;
}
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public Builder setAppType(int value) {
bitField0_ |= 0x00000001;
appType_ = value;
onChanged();
return this;
}
/**
* optional int32 app_type = 1;
*
*
* A wireless.android.stats.playlog.activeusers.AppType.Id value. See
* google3/wireless/android/stats/playlog/activeusers/apptype.proto.
*
*/
public Builder clearAppType() {
bitField0_ = (bitField0_ & ~0x00000001);
appType_ = 0;
onChanged();
return this;
}
// optional string android_package_name = 2;
private java.lang.Object androidPackageName_ = "";
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public boolean hasAndroidPackageName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public java.lang.String getAndroidPackageName() {
java.lang.Object ref = androidPackageName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
androidPackageName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public com.google.protobuf.ByteString
getAndroidPackageNameBytes() {
java.lang.Object ref = androidPackageName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
androidPackageName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public Builder setAndroidPackageName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
androidPackageName_ = value;
onChanged();
return this;
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public Builder clearAndroidPackageName() {
bitField0_ = (bitField0_ & ~0x00000002);
androidPackageName_ = getDefaultInstance().getAndroidPackageName();
onChanged();
return this;
}
/**
* optional string android_package_name = 2;
*
*
* Package name of the app (on Android).
*
*/
public Builder setAndroidPackageNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
androidPackageName_ = value;
onChanged();
return this;
}
// optional string version = 3;
private java.lang.Object version_ = "";
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
version_ = value;
onChanged();
return this;
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000004);
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
* optional string version = 3;
*
*
* Version of the app.
*
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
version_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.AppUsage1pLogEvent)
}
static {
defaultInstance = new AppUsage1pLogEvent(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.AppUsage1pLogEvent)
}
public interface BatchedLogRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .wireless_android_play_playlog.LogRequest log_request = 1;
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
java.util.List
getLogRequestList();
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest getLogRequest(int index);
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
int getLogRequestCount();
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder>
getLogRequestOrBuilderList();
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder getLogRequestOrBuilder(
int index);
}
/**
* Protobuf type {@code wireless_android_play_playlog.BatchedLogRequest}
*
*
* A BatchedLogRequest represents a batched collection of log requests.
*
* Next tag: 2
*
*/
public static final class BatchedLogRequest extends
com.google.protobuf.GeneratedMessage
implements BatchedLogRequestOrBuilder {
// Use BatchedLogRequest.newBuilder() to construct.
private BatchedLogRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private BatchedLogRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final BatchedLogRequest defaultInstance;
public static BatchedLogRequest getDefaultInstance() {
return defaultInstance;
}
public BatchedLogRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BatchedLogRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
logRequest_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logRequest_.add(input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
logRequest_ = java.util.Collections.unmodifiableList(logRequest_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BatchedLogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public BatchedLogRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BatchedLogRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .wireless_android_play_playlog.LogRequest log_request = 1;
public static final int LOG_REQUEST_FIELD_NUMBER = 1;
private java.util.List logRequest_;
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public java.util.List getLogRequestList() {
return logRequest_;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder>
getLogRequestOrBuilderList() {
return logRequest_;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public int getLogRequestCount() {
return logRequest_.size();
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest getLogRequest(int index) {
return logRequest_.get(index);
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder getLogRequestOrBuilder(
int index) {
return logRequest_.get(index);
}
private void initFields() {
logRequest_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getLogRequestCount(); i++) {
if (!getLogRequest(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < logRequest_.size(); i++) {
output.writeMessage(1, logRequest_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < logRequest_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, logRequest_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.BatchedLogRequest}
*
*
* A BatchedLogRequest represents a batched collection of log requests.
*
* Next tag: 2
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BatchedLogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getLogRequestFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (logRequestBuilder_ == null) {
logRequest_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logRequestBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest(this);
int from_bitField0_ = bitField0_;
if (logRequestBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
logRequest_ = java.util.Collections.unmodifiableList(logRequest_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logRequest_ = logRequest_;
} else {
result.logRequest_ = logRequestBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest.getDefaultInstance()) return this;
if (logRequestBuilder_ == null) {
if (!other.logRequest_.isEmpty()) {
if (logRequest_.isEmpty()) {
logRequest_ = other.logRequest_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogRequestIsMutable();
logRequest_.addAll(other.logRequest_);
}
onChanged();
}
} else {
if (!other.logRequest_.isEmpty()) {
if (logRequestBuilder_.isEmpty()) {
logRequestBuilder_.dispose();
logRequestBuilder_ = null;
logRequest_ = other.logRequest_;
bitField0_ = (bitField0_ & ~0x00000001);
logRequestBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getLogRequestFieldBuilder() : null;
} else {
logRequestBuilder_.addAllMessages(other.logRequest_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getLogRequestCount(); i++) {
if (!getLogRequest(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.BatchedLogRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .wireless_android_play_playlog.LogRequest log_request = 1;
private java.util.List logRequest_ =
java.util.Collections.emptyList();
private void ensureLogRequestIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
logRequest_ = new java.util.ArrayList(logRequest_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder> logRequestBuilder_;
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public java.util.List getLogRequestList() {
if (logRequestBuilder_ == null) {
return java.util.Collections.unmodifiableList(logRequest_);
} else {
return logRequestBuilder_.getMessageList();
}
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public int getLogRequestCount() {
if (logRequestBuilder_ == null) {
return logRequest_.size();
} else {
return logRequestBuilder_.getCount();
}
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest getLogRequest(int index) {
if (logRequestBuilder_ == null) {
return logRequest_.get(index);
} else {
return logRequestBuilder_.getMessage(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder setLogRequest(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest value) {
if (logRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogRequestIsMutable();
logRequest_.set(index, value);
onChanged();
} else {
logRequestBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder setLogRequest(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder builderForValue) {
if (logRequestBuilder_ == null) {
ensureLogRequestIsMutable();
logRequest_.set(index, builderForValue.build());
onChanged();
} else {
logRequestBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder addLogRequest(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest value) {
if (logRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogRequestIsMutable();
logRequest_.add(value);
onChanged();
} else {
logRequestBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder addLogRequest(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest value) {
if (logRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogRequestIsMutable();
logRequest_.add(index, value);
onChanged();
} else {
logRequestBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder addLogRequest(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder builderForValue) {
if (logRequestBuilder_ == null) {
ensureLogRequestIsMutable();
logRequest_.add(builderForValue.build());
onChanged();
} else {
logRequestBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder addLogRequest(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder builderForValue) {
if (logRequestBuilder_ == null) {
ensureLogRequestIsMutable();
logRequest_.add(index, builderForValue.build());
onChanged();
} else {
logRequestBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder addAllLogRequest(
java.lang.Iterable extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest> values) {
if (logRequestBuilder_ == null) {
ensureLogRequestIsMutable();
super.addAll(values, logRequest_);
onChanged();
} else {
logRequestBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder clearLogRequest() {
if (logRequestBuilder_ == null) {
logRequest_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logRequestBuilder_.clear();
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public Builder removeLogRequest(int index) {
if (logRequestBuilder_ == null) {
ensureLogRequestIsMutable();
logRequest_.remove(index);
onChanged();
} else {
logRequestBuilder_.remove(index);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder getLogRequestBuilder(
int index) {
return getLogRequestFieldBuilder().getBuilder(index);
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder getLogRequestOrBuilder(
int index) {
if (logRequestBuilder_ == null) {
return logRequest_.get(index); } else {
return logRequestBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder>
getLogRequestOrBuilderList() {
if (logRequestBuilder_ != null) {
return logRequestBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logRequest_);
}
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder addLogRequestBuilder() {
return getLogRequestFieldBuilder().addBuilder(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder addLogRequestBuilder(
int index) {
return getLogRequestFieldBuilder().addBuilder(
index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogRequest log_request = 1;
*/
public java.util.List
getLogRequestBuilderList() {
return getLogRequestFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder>
getLogRequestFieldBuilder() {
if (logRequestBuilder_ == null) {
logRequestBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder>(
logRequest_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
logRequest_ = null;
}
return logRequestBuilder_;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.BatchedLogRequest)
}
static {
defaultInstance = new BatchedLogRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.BatchedLogRequest)
}
public interface LogRequestOrBuilder extends
com.google.protobuf.GeneratedMessage.
ExtendableMessageOrBuilder {
// optional int64 request_time_ms = 4;
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
boolean hasRequestTimeMs();
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
long getRequestTimeMs();
// optional int64 request_uptime_ms = 8;
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
boolean hasRequestUptimeMs();
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
long getRequestUptimeMs();
// optional .wireless_android_play_playlog.ClientInfo client_info = 1;
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
boolean hasClientInfo();
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo getClientInfo();
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder getClientInfoOrBuilder();
// optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
boolean hasLogSource();
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource getLogSource();
// optional string log_source_name = 6;
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
boolean hasLogSourceName();
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
java.lang.String getLogSourceName();
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
com.google.protobuf.ByteString
getLogSourceNameBytes();
// optional string zwieback_cookie = 7;
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
boolean hasZwiebackCookie();
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
java.lang.String getZwiebackCookie();
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
com.google.protobuf.ByteString
getZwiebackCookieBytes();
// repeated .wireless_android_play_playlog.LogEvent log_event = 3;
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
java.util.List
getLogEventList();
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent getLogEvent(int index);
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
int getLogEventCount();
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder>
getLogEventOrBuilderList();
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder getLogEventOrBuilder(
int index);
// repeated bytes serialized_log_events = 5;
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
java.util.List getSerializedLogEventsList();
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
int getSerializedLogEventsCount();
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
com.google.protobuf.ByteString getSerializedLogEvents(int index);
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
boolean hasQosTier();
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier();
// optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
boolean hasScheduler();
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType getScheduler();
// optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
boolean hasDeviceStatus();
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus getDeviceStatus();
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder getDeviceStatusOrBuilder();
// optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
boolean hasExternalTimestamp();
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp getExternalTimestamp();
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder getExternalTimestampOrBuilder();
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogRequest}
*
*
* A LogRequest represents a batched collection of loggable events, each event
* to be processed and sent to zero or more destinations (e.g. Sawmill log types
* or Footprints) by the Clearcut server.
*
* Next tag: 13
*
*/
public static final class LogRequest extends
com.google.protobuf.GeneratedMessage.ExtendableMessage<
LogRequest> implements LogRequestOrBuilder {
// Use LogRequest.newBuilder() to construct.
private LogRequest(com.google.protobuf.GeneratedMessage.ExtendableBuilder builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private LogRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final LogRequest defaultInstance;
public static LogRequest getDefaultInstance() {
return defaultInstance;
}
public LogRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = clientInfo_.toBuilder();
}
clientInfo_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientInfo_);
clientInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 16: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000008;
logSource_ = value;
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
logEvent_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
logEvent_.add(input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.PARSER, extensionRegistry));
break;
}
case 32: {
bitField0_ |= 0x00000001;
requestTimeMs_ = input.readInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
serializedLogEvents_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
serializedLogEvents_.add(input.readBytes());
break;
}
case 50: {
bitField0_ |= 0x00000010;
logSourceName_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000020;
zwiebackCookie_ = input.readBytes();
break;
}
case 64: {
bitField0_ |= 0x00000002;
requestUptimeMs_ = input.readInt64();
break;
}
case 72: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(9, rawValue);
} else {
bitField0_ |= 0x00000040;
qosTier_ = value;
}
break;
}
case 80: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(10, rawValue);
} else {
bitField0_ |= 0x00000080;
scheduler_ = value;
}
break;
}
case 90: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = deviceStatus_.toBuilder();
}
deviceStatus_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(deviceStatus_);
deviceStatus_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 98: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = externalTimestamp_.toBuilder();
}
externalTimestamp_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(externalTimestamp_);
externalTimestamp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
logEvent_ = java.util.Collections.unmodifiableList(logEvent_);
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
serializedLogEvents_ = java.util.Collections.unmodifiableList(serializedLogEvents_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public LogRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.LogRequest.LogSource}
*/
public enum LogSource
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = -1;
*/
UNKNOWN(0, -1),
/**
* STORE = 0;
*/
STORE(1, 0),
/**
* WEB_STORE = 65;
*
*
* Google Play Store Android App
*
*/
WEB_STORE(2, 65),
/**
* WORK_STORE = 132;
*
*
* Google Play Web Store (https://play.google.com/store)
*
*/
WORK_STORE(3, 132),
/**
* WORK_STORE_APP = 261;
*
*
* Play for Work on the web client (Worksky)
*
*/
WORK_STORE_APP(4, 261),
/**
* EDU_STORE = 15;
*
*
* Play for Work on the Google Play Store Android App
*
*/
EDU_STORE(5, 15),
/**
* MUSIC = 1;
*
*
* for collecting stats on Play for Education
*
*/
MUSIC(6, 1),
/**
* BOOKS = 2;
*/
BOOKS(7, 2),
/**
* VIDEO = 3;
*/
VIDEO(8, 3),
/**
* MOVIES = 74;
*
*
* Android Video (go/avi, android-video-oncall@)
*
*/
MOVIES(9, 74),
/**
* MAGAZINES = 4;
*/
MAGAZINES(10, 4),
/**
* GAMES = 5;
*/
GAMES(11, 5),
/**
* LB_A = 6;
*/
LB_A(12, 6),
/**
* ANDROID_IDE = 7;
*
*
* for collecting app usage stats (Lockbox project)
*
*/
ANDROID_IDE(13, 7),
/**
* LB_P = 8;
*
*
* Android IDE (deprecated, see ANDROID_STUDIO below)
*
*/
LB_P(14, 8),
/**
* LB_S = 9;
*
*
* for collecting phone call events and stats (Lockbox project)
*
*/
LB_S(15, 9),
/**
* GMS_CORE = 10;
*
*
* for collecting SMS events and stats (Lockbox project)
*
*/
GMS_CORE(16, 10),
/**
* APP_USAGE_1P = 11;
*
*
* Usage of GMS Core itself and its internal stats
*
*/
APP_USAGE_1P(17, 11),
/**
* ICING = 12;
*
*
* First-party app usage tracking.
*
*/
ICING(18, 12),
/**
* HERREVAD = 13;
*
*
* Icing stats (AppDataSearch go/icing)
*
*/
HERREVAD(19, 13),
/**
* GOOGLE_TV = 14;
*
*
* for collecting Nova Network quality data
*
*/
GOOGLE_TV(20, 14),
/**
* GMS_CORE_PEOPLE = 16;
*
*
* Google TV, not Eureka.
*
*/
GMS_CORE_PEOPLE(21, 16),
/**
* LE = 17;
*
*
* GMS core people (aka menagerie, zhengfu@, android-contacts@)
*
*/
LE(22, 17),
/**
* GOOGLE_ANALYTICS = 18;
*
*
* Location Engine statistics
*
*/
GOOGLE_ANALYTICS(23, 18),
/**
* LB_D = 19;
*
*
* Google Analytics SDK health monitoring
*
*/
LB_D(24, 19),
/**
* ANDROID_GSA = 20;
*
*
* for collecting device state changes (Lockbox project)
*
*/
ANDROID_GSA(25, 20),
/**
* LB_T = 21;
*
*
* Android GSA (Google Search App) stats
*
*/
LB_T(26, 21),
/**
* PERSONAL_LOGGER = 22;
*
*
* for collecting Android RunningTaskInfo (Lockbox project)
*
*/
PERSONAL_LOGGER(27, 22),
/**
* PERSONAL_BROWSER_LOGGER = 37;
*
*
* PixelPerfect activity logger
*
*/
PERSONAL_BROWSER_LOGGER(28, 37),
/**
* GMS_CORE_WALLET_MERCHANT_ERROR = 23;
*
*
* PixelPerfect Chrome extension logger
*
*/
GMS_CORE_WALLET_MERCHANT_ERROR(29, 23),
/**
* LB_C = 24;
*
*
* GMS core wallet merchant errors
*
*/
LB_C(30, 24),
/**
* LB_IA = 52;
*
*
* Android contacts going from Now to HappyHour.log_event_extension_test
*
*/
LB_IA(31, 52),
/**
* LB_CB = 237;
*
*
* Android installed apps going from Now to HappyHour.
*
*/
LB_CB(32, 237),
/**
* LB_DM = 268;
*
*
* Android contacts for backup/restore purpose.
*
*/
LB_DM(33, 268),
/**
* ANDROID_AUTH = 25;
*
*
* Android device metadata used for contacts backup/restore
* purpose.
*
*/
ANDROID_AUTH(34, 25),
/**
* ANDROID_CAMERA = 26;
*
*
* for collecting GMS core android auth data
*
*/
ANDROID_CAMERA(35, 26),
/**
* CW = 27;
*
*
* Android camera usage statistics
*
*/
CW(36, 27),
/**
* CW_COUNTERS = 243;
*
*
* Clockwork interaction logs
*
*/
CW_COUNTERS(37, 243),
/**
* GEL = 28;
*
*
* Clockwork counters
*
*/
GEL(38, 28),
/**
* DNA_PROBER = 29;
*
*
* Google experience launcher logs (http://go/signac).
*
*/
DNA_PROBER(39, 29),
/**
* UDR = 30;
*
*
* DNA logger (for internal research), go/dna2014.
*
*/
UDR(40, 30),
/**
* GMS_CORE_WALLET = 31;
*
*
* For collecting Android app usage data.
*
*/
GMS_CORE_WALLET(41, 31),
/**
* SOCIAL = 32;
*
*
* GMS core wallet analytics
*
*/
SOCIAL(42, 32),
/**
* INSTORE_WALLET = 33;
*
*
* Traffic from Social clients: gplus-log-quality@
*
*/
INSTORE_WALLET(43, 33),
/**
* NOVA = 34;
*
*
* Instore play payments
*
*/
NOVA(44, 34),
/**
* LB_CA = 35;
*
*
* Nova client logs.
*
*/
LB_CA(45, 35),
/**
* LATIN_IME = 36;
*
*
* DEPRECATED: Android contact account data going from Now to Footprints.
*
*/
LATIN_IME(46, 36),
/**
* NEWS_WEATHER = 38;
*
*
* Google Keyboard (http://go/google-keyboard)
*
*/
NEWS_WEATHER(47, 38),
/**
* HANGOUT = 39;
*
*
* News & Weather Android/iOS apps
*
*/
HANGOUT(48, 39),
/**
* HANGOUT_LOG_REQUEST = 50;
*
*
* Deprecated source for HangoutLogEntry (rtc-analytics-eng@)
*
*/
HANGOUT_LOG_REQUEST(49, 50),
/**
* COPRESENCE = 40;
*
*
* Hangouts data using HangoutLogRequest (rtc-analytics-eng@)
*
*/
COPRESENCE(50, 40),
/**
* SOCIAL_AFFINITY = 41;
*
*
* Copresence (http://go/whisper)
*
*/
SOCIAL_AFFINITY(51, 41),
/**
* PHOTOS = 42;
*
*
* Social Affinity logs (go/disco-logging-v2).
*
*/
PHOTOS(52, 42),
/**
* GCM = 43;
*
*
* Google Photos (go/photos).
*
*/
GCM(53, 43),
/**
* GOKART = 44;
*
*
* Google Cloud Messaging logs (go/gcm).
*
*/
GOKART(54, 44),
/**
* FINDR = 45;
*
*
* GoKart (go/gokart, Drive Android API)
*
*/
FINDR(55, 45),
/**
* ANDROID_MESSAGING = 46;
*
*
* Findr app (go/findr).
*
*/
ANDROID_MESSAGING(56, 46),
/**
* SOCIAL_WEB = 47;
*
*
* Android messenger(Bugle).
*
*/
SOCIAL_WEB(57, 47),
/**
* BACKDROP = 48;
*
*
* Traffic from Social clients: gplus-log-quality@.
*
*/
BACKDROP(58, 48),
/**
* TELEMATICS = 49;
*
*
* Backdrop device logs (go/tv-imax).
*
*/
TELEMATICS(59, 49),
/**
* GVC_HARVESTER = 51;
*
*
* Telematics app (go/telematics).
*
*/
GVC_HARVESTER(60, 51),
/**
* CAR = 53;
*
*
* GVC Harvester (go/harvesterdesigndoc)
*
*/
CAR(61, 53),
/**
* PIXEL_PERFECT = 54;
*
*
* Android Automotive (go/gearhead).
*
*/
PIXEL_PERFECT(62, 54),
/**
* DRIVE = 55;
*
*
* PixelPerfect app client log (go/pixelperfect-analytics)
*
*/
DRIVE(63, 55),
/**
* DOCS = 56;
*
*
* Google Drive (go/cakemix, nickread@, cakemix-drive@)
*
*/
DOCS(64, 56),
/**
* SHEETS = 57;
*
*
* Google Docs (go/cakemix, haluk@, bulach@, tstrauss@)
*
*/
SHEETS(65, 57),
/**
* SLIDES = 58;
*
*
* Google Sheets (go/cakemix, tgleason@, bulach@, tstrauss@)
*
*/
SLIDES(66, 58),
/**
* IME = 59;
*
*
* Google Slides (go/cakemix, yugacohler@, bulach@, tstrauss@)
*
*/
IME(67, 59),
/**
* WARP = 60;
*
*
* Google Android IME logs (go/goopy).
*
*/
WARP(68, 60),
/**
* NFC_PROGRAMMER = 61;
*
*
* Warp (go/warp-project)
*
*/
NFC_PROGRAMMER(69, 61),
/**
* NETSTATS = 62;
*
*
* NfcProgrammer (go/aedu-platform-overview)
*
*/
NETSTATS(70, 62),
/**
* NEWSSTAND = 63;
*
*
* Collection of Network Statistics (go/n-s).
*
*/
NEWSSTAND(71, 63),
/**
* KIDS_COMMUNICATOR = 64;
*
*
* Google Play Newsstand.
*
*/
KIDS_COMMUNICATOR(72, 64),
/**
* WIFI_ASSISTANT = 66;
*
*
* Kids Communicator (http://goto/kidscommunicator_dd)
*
*/
WIFI_ASSISTANT(73, 66),
/**
* CAST_SENDER_SDK = 67;
*
*
* WiFi Assistant client logs.
*
*/
CAST_SENDER_SDK(74, 67),
/**
* CRONET_SOCIAL = 68;
*
*
* Cast Sender SDK (go/dongle)
*
*/
CRONET_SOCIAL(75, 68),
/**
* PHENOTYPE = 69;
*
*
* Social cronet metrics.
*
*/
PHENOTYPE(76, 69),
/**
* PHENOTYPE_COUNTERS = 70;
*
*
* The client side of experiment framework (go/ph, peiling@, phenotype-eng@).
*
*/
PHENOTYPE_COUNTERS(77, 70),
/**
* CHROME_INFRA = 71;
*
*
* The counters for Phenotype (go/ph, peiling@, phenotype-eng@).
*
*/
CHROME_INFRA(78, 71),
/**
* JUSTSPEAK = 72;
*
*
* Chrome Infrastructure events
*
*/
JUSTSPEAK(79, 72),
/**
* PERF_PROFILE = 73;
*
*
* JustSpeak (go/justspeak)
*
*/
PERF_PROFILE(80, 73),
/**
* KATNISS = 75;
*
*
* Android Wide Profiling (go/android-wide-profiling)
*
*/
KATNISS(81, 75),
/**
* SOCIAL_APPINVITE = 76;
*
*
* Android TV search (go/atv-search)
*
*/
SOCIAL_APPINVITE(82, 76),
/**
* GMM_COUNTERS = 77;
*
*
* Google Plus Platform App Invite (go/appinvite)
*
*/
GMM_COUNTERS(83, 77),
/**
* BOND_ONEGOOGLE = 78;
*
*
* Google Maps Mobile (go/gmm).
*
*/
BOND_ONEGOOGLE(84, 78),
/**
* MAPS_API = 79;
*
*
* Bond OneGoogleBar integration (go/bond-ogb)
*
*/
MAPS_API(85, 79),
/**
* CRONET_ANDROID_YT = 196;
*
*
* Android and iOS Maps API (go/mapview & go/meridian)
*
*/
CRONET_ANDROID_YT(86, 196),
/**
* CRONET_ANDROID_GSA = 80;
*
*
* Cronet UMA logs from Android YT (go/cronet-uma)
*
*/
CRONET_ANDROID_GSA(87, 80),
/**
* GOOGLE_FIT_WEARABLE = 81;
*
*
* Cronet UMA logs from Android GSA (go/cronet-uma)
*
*/
GOOGLE_FIT_WEARABLE(88, 81),
/**
* FITNESS_ANDROID = 169;
*
*
* Google Fit Wearable App (go/heart)
*
*/
FITNESS_ANDROID(89, 169),
/**
* FITNESS_GMS_CORE = 170;
*
*
* Google Fit Android App (go/heart)
*
*/
FITNESS_GMS_CORE(90, 170),
/**
* GOOGLE_EXPRESS = 82;
*
*
* Google Fit GMSCore (go/heart)
*
*/
GOOGLE_EXPRESS(91, 82),
/**
* GOOGLE_EXPRESS_DEV = 215;
*
*
* Google Express (go/gsx)
*
*/
GOOGLE_EXPRESS_DEV(92, 215),
/**
* GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES = 228;
*
*
* Google Express (go/gsx) For DEV mall server
*
*/
GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES(93, 228),
/**
* GOOGLE_EXPRESS_ANDROID_PRIMES = 229;
*
*
* Google Express (go/gsx) Courier App metric logging (go/primes)
*
*/
GOOGLE_EXPRESS_ANDROID_PRIMES(94, 229),
/**
* GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES = 240;
*
*
* Google Express (go/gsx) App metric logging (go/primes)
*
*/
GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES(95, 240),
/**
* SENSE = 83;
*
*
* Google Express (go/gsx) Store OMS App metric logging (go/primes)
*
*/
SENSE(96, 83),
/**
* ANDROID_BACKUP = 84;
*
*
* Sense (go/sense)
*
*/
ANDROID_BACKUP(97, 84),
/**
* VR = 85;
*
*
* Android Backup Service.
*
*/
VR(98, 85),
/**
* IME_COUNTERS = 86;
*
*
* Virtual Reality and Google Cardboard (go/vr-team)
*
*/
IME_COUNTERS(99, 86),
/**
* SETUP_WIZARD = 87;
*
*
* The counters for Google Android IME (go/goopy).
*
*/
SETUP_WIZARD(100, 87),
/**
* EMERGENCY_ASSIST = 88;
*
*
* Setup Wizard (go/suw)
*
*/
EMERGENCY_ASSIST(101, 88),
/**
* TRON = 89;
*
*
* Barton (go/barton-deprecation)
*
*/
TRON(102, 89),
/**
* TRON_COUNTERS = 90;
*
*
* The event logs for Android Framework (go/tron, cwren@, khigaki@, tron-eng@).
*
*/
TRON_COUNTERS(103, 90),
/**
* BATTERY_STATS = 91;
*
*
* The counters for Android Framework (go/tron, cwren@, khigaki@, tron-eng@).
*
*/
BATTERY_STATS(104, 91),
/**
* DISK_STATS = 92;
*
*
* For collecting battery performance data from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
DISK_STATS(105, 92),
/**
* GRAPHICS_STATS = 107;
*
*
* For collecting disk utilization stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
GRAPHICS_STATS(106, 107),
/**
* PROC_STATS = 93;
*
*
* For collecting rendering performance stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
PROC_STATS(107, 93),
/**
* DROP_BOX = 131;
*
*
* For collecting memory utilization stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
DROP_BOX(108, 131),
/**
* FINGERPRINT_STATS = 181;
*
*
* For collecting DropBox logs from Android clients.
*
*/
FINGERPRINT_STATS(109, 181),
/**
* NOTIFICATION_STATS = 182;
*
*
* For collecting fingerprint stats from Android clients. (go/android-fingerprintstats)
*
*/
NOTIFICATION_STATS(110, 182),
/**
* TAP_AND_PAY_GCORE = 94;
*
*
* For collecting notification stats from Android clients. (go/android-notificationstats)
*
*/
TAP_AND_PAY_GCORE(111, 94),
/**
* A11YLOGGER = 95;
*
*
* Tap and Pay client service layer (go/tp2-eng)
*
*/
A11YLOGGER(112, 95),
/**
* GCM_COUNTERS = 96;
*
*
* Accessibility Settings Logger (go/a11ylogger)
*
*/
GCM_COUNTERS(113, 96),
/**
* PLACES_NO_GLS_CONSENT = 97;
*
*
* Google Cloud Messaging counters (go/gcm).
*
*/
PLACES_NO_GLS_CONSENT(114, 97),
/**
* TACHYON_LOG_REQUEST = 98;
*
*
* Mobile Places API logs without GLS consent (go/ios-places-api).
*
*/
TACHYON_LOG_REQUEST(115, 98),
/**
* TACHYON_COUNTERS = 99;
*
*
* WebRTC Tachyon (go/tachyon)
*
*/
TACHYON_COUNTERS(116, 99),
/**
* VISION = 100;
*
*
* WebRTC Tachyon (go/tachyon)
*
*/
VISION(117, 100),
/**
* SOCIAL_USER_LOCATION = 101;
*
*
* Vision (go/mobile-vision)
*
*/
SOCIAL_USER_LOCATION(118, 101),
/**
* LAUNCHPAD_TOYS = 102;
*
*
* Location Sharing (go/location-sharing)
*
*/
LAUNCHPAD_TOYS(119, 102),
/**
* METALOG_COUNTERS = 103;
*
*
* Launchpad Toys (go/launchpadtoys)
*
*/
METALOG_COUNTERS(120, 103),
/**
* MOBILESDK_CLIENT = 104;
*
*
* Counters for metalog (go/clearcut-metalog, iamawong@, clearcut-eng@)
*
*/
MOBILESDK_CLIENT(121, 104),
/**
* ANDROID_VERIFY_APPS = 105;
*
*
* go/greenhouse
*
*/
ANDROID_VERIFY_APPS(122, 105),
/**
* ADSHIELD = 106;
*
*
* go/safety-net
*
*/
ADSHIELD(123, 106),
/**
* SHERLOG = 108;
*
*
* go/adshield
*
*/
SHERLOG(124, 108),
/**
* LE_ULR_COUNTERS = 109;
*
*
* Sherlog data (go/sherlog)
*
*/
LE_ULR_COUNTERS(125, 109),
/**
* GMM_UE3 = 110;
*
*
* Counters for ULR (go/gcore-ulr). Location custom events use LE.
*
*/
GMM_UE3(126, 110),
/**
* CALENDAR = 111;
*
*
* Logs for GMM analytics and sessions (go/gca-analytics)
*
*/
CALENDAR(127, 111),
/**
* ENDER = 112;
*
*
* Google Android Calendar
*
*/
ENDER(128, 112),
/**
* FAMILY_COMPASS = 113;
*
*
* Logs for Ender (go/ender)
*
*/
FAMILY_COMPASS(129, 113),
/**
* TRANSOM = 114;
*
*
* go/familycompass
*
*/
TRANSOM(130, 114),
/**
* TRANSOM_COUNTERS = 115;
*
*
* Transom client-side metrics (go/transom)
*
*/
TRANSOM_COUNTERS(131, 115),
/**
* LB_AS = 116;
*
*
* Counters for transom metrics
*
*/
LB_AS(132, 116),
/**
* LB_CFG = 117;
*
*
* App usage collected by the new UsageStats API (Lockbox project).
*
*/
LB_CFG(133, 117),
/**
* IOS_GSA = 118;
*
*
* Device config collected by the new UsageStats API (Lockbox project).
*
*/
IOS_GSA(134, 118),
/**
* TAP_AND_PAY_APP = 119;
*
*
* iOS GSA (Google Search App) stats
*
*/
TAP_AND_PAY_APP(135, 119),
/**
* TAP_AND_PAY_APP_COUNTERS = 265;
*
*
* Tap and Pay android app, aka Android pay app (go/tp2)
*
*/
TAP_AND_PAY_APP_COUNTERS(136, 265),
/**
* FLYDROID = 120;
*
*
* Tap and Pay android app counters
*
*/
FLYDROID(137, 120),
/**
* CPANEL_APP = 121;
*
*
* Logs for Flydroid (go/flydroid).
*
*/
CPANEL_APP(138, 121),
/**
* ANDROID_SNET_GCORE = 122;
*
*
* CPanel latency on mobile.
*
*/
ANDROID_SNET_GCORE(139, 122),
/**
* ANDROID_SNET_IDLE = 123;
*
*
* go/safety-net
*
*/
ANDROID_SNET_IDLE(140, 123),
/**
* ANDROID_SNET_JAR = 124;
*
*
* go/safety-net
*
*/
ANDROID_SNET_JAR(141, 124),
/**
* CONTEXT_MANAGER = 125;
*
*
* go/safety-net
*
*/
CONTEXT_MANAGER(142, 125),
/**
* CLASSROOM = 126;
*
*
* Logs for Context Manager (go/context-manager)
*
*/
CLASSROOM(143, 126),
/**
* TAILORMADE = 127;
*
*
* App usage of the go/classroom app.
*
*/
TAILORMADE(144, 127),
/**
* KEEP = 128;
*
*
* Logs for Tailormade app (go/a1-tm)
*
*/
KEEP(145, 128),
/**
* GMM_BRIIM_COUNTERS = 129;
*
*
* Logs for Keep (go/keep).
*
*/
GMM_BRIIM_COUNTERS(146, 129),
/**
* CHROMECAST_APP_LOG = 130;
*
*
* GMM BRIIM counters (go/gmm-briim)
*
*/
CHROMECAST_APP_LOG(147, 130),
/**
* ADWORDS_MOBILE = 133;
*
*
* Logs for chromecast app (go/chromecastapplogs)
*
*/
ADWORDS_MOBILE(148, 133),
/**
* LEANBACK_EVENT = 134;
*
*
* Logs for AdWords mobile app (go/awm)
*
*/
LEANBACK_EVENT(149, 134),
/**
* ANDROID_GMAIL = 135;
*
*
* Logs for Android TV Leanback Launcher (go/android-tv)
*
*/
ANDROID_GMAIL(150, 135),
/**
* SAMPLE_SHM = 136;
*
*
* Logs for Gmail (go/android-gmail-clearcut, shahrk@, android-mail-team@)
*
*/
SAMPLE_SHM(151, 136),
/**
* GPLUS_ANDROID_PRIMES = 140;
*
*
* Logs for system health metric and memory metric sample app (go/primes)
*
*/
GPLUS_ANDROID_PRIMES(152, 140),
/**
* GMAIL_ANDROID_PRIMES = 150;
*/
GMAIL_ANDROID_PRIMES(154, 150),
/**
* CALENDAR_ANDROID_PRIMES = 151;
*/
CALENDAR_ANDROID_PRIMES(156, 151),
/**
* DOCS_ANDROID_PRIMES = 152;
*/
DOCS_ANDROID_PRIMES(158, 152),
/**
* YT_MAIN_APP_ANDROID_PRIMES = 154;
*/
YT_MAIN_APP_ANDROID_PRIMES(160, 154),
/**
* YT_KIDS_ANDROID_PRIMES = 155;
*
*
* Logs for system health metric for the YouTube main app (go/primes)
*
*/
YT_KIDS_ANDROID_PRIMES(161, 155),
/**
* YT_GAMING_ANDROID_PRIMES = 156;
*
*
* Logs for system health metric for the YouTube kids app (go/primes)
*
*/
YT_GAMING_ANDROID_PRIMES(162, 156),
/**
* YT_MUSIC_ANDROID_PRIMES = 157;
*
*
* Logs for system health metric for the YouTube gaming app (go/primes)
*
*/
YT_MUSIC_ANDROID_PRIMES(163, 157),
/**
* YT_LITE_ANDROID_PRIMES = 158;
*
*
* Logs for system health metric for the YouTube music app (go/primes)
*
*/
YT_LITE_ANDROID_PRIMES(164, 158),
/**
* YT_CREATOR_ANDROID_PRIMES = 171;
*
*
* Logs for system health metric for the YouTube lite app (go/primes)
*
*/
YT_CREATOR_ANDROID_PRIMES(165, 171),
/**
* JAM_ANDROID_PRIMES = 159;
*
*
* Logs for system health metric for the YouTube Creator Studio app (go/primes)
*
*/
JAM_ANDROID_PRIMES(166, 159),
/**
* JAM_KIOSK_ANDROID_PRIMES = 160;
*
*
* Logs for system health metric for Jam (go/jam, go/primes)
*
*/
JAM_KIOSK_ANDROID_PRIMES(167, 160),
/**
* PHOTOS_ANDROID_PRIMES = 165;
*
*
* Logs for system health metric for Jam Kiosk (go/jam, go/primes)
*
*/
PHOTOS_ANDROID_PRIMES(168, 165),
/**
* DRIVE_ANDROID_PRIMES = 166;
*
*
* Logs for system health metric for Photos (go/primes)
*
*/
DRIVE_ANDROID_PRIMES(169, 166),
/**
* SHEETS_ANDROID_PRIMES = 167;
*
*
* Logs for system health metric for Drive (go/primes)
*
*/
SHEETS_ANDROID_PRIMES(170, 167),
/**
* SLIDES_ANDROID_PRIMES = 168;
*
*
* Logs for system health metric for Sheets (go/primes)
*
*/
SLIDES_ANDROID_PRIMES(171, 168),
/**
* SNAPSEED_ANDROID_PRIMES = 178;
*
*
* Logs for system health metric for Slides (go/primes)
*
*/
SNAPSEED_ANDROID_PRIMES(172, 178),
/**
* HANGOUTS_ANDROID_PRIMES = 179;
*
*
* Logs for system health metric for Snapseed (go/primes, go/snapseed).
*
*/
HANGOUTS_ANDROID_PRIMES(173, 179),
/**
* INBOX_ANDROID_PRIMES = 180;
*
*
* Logs for system health metric for Shem (go/primes, go/rtc-shem).
*
*/
INBOX_ANDROID_PRIMES(174, 180),
/**
* INBOX_IOS_PRIMES = 262;
*
*
* Logs for system health metric for Inbox (go/primes, go/bt-speed)
*
*/
INBOX_IOS_PRIMES(175, 262),
/**
* SDP_IOS_PRIMES = 287;
*
*
* Logs for ios system health metric for Inbox (go/primes-ios, go/bt-speed)
*
*/
SDP_IOS_PRIMES(176, 287),
/**
* GMSCORE_ANDROID_PRIMES = 193;
*
*
* Logs for ios system health metric for sdp (go/primes-ios, go/bt-speed)
*
*/
GMSCORE_ANDROID_PRIMES(177, 193),
/**
* PLAY_MUSIC_ANDROID_WEAR_PRIMES = 200;
*
*
* Logs for system health metric for GmsCore (go/primes)
*
*/
PLAY_MUSIC_ANDROID_WEAR_PRIMES(178, 200),
/**
* GEARHEAD_ANDROID_PRIMES = 201;
*
*
* Logs for system health metric for Play Music for Android Wear (music-android-wear@, go/wear-play-music, go/primes)
*
*/
GEARHEAD_ANDROID_PRIMES(179, 201),
/**
* INSTORE_CONSUMER_PRIMES = 207;
*
*
* Logs for system health metric for the Android Auto (GearHead) app (go/primes, go/gearhead)
*
*/
INSTORE_CONSUMER_PRIMES(180, 207),
/**
* SAMPLE_IOS_PRIMES = 202;
*
*
* Logs for system health metric for Hands Free (go/primes, go/instore-wallet)
*
*/
SAMPLE_IOS_PRIMES(181, 202),
/**
* CPANEL_ANDROID_PRIMES = 213;
*
*
* Logs for ios system health metric and memory metric sample app (go/primes-ios)
*
*/
CPANEL_ANDROID_PRIMES(182, 213),
/**
* HUDDLE_ANDROID_PRIMES = 214;
*
*
* Logs for android system health metric (go/cpanel-mobile)
*
*/
HUDDLE_ANDROID_PRIMES(183, 214),
/**
* AWX_ANDROID_PRIMES = 222;
*
*
* Primes for Huddle (go/huddle)
*
*/
AWX_ANDROID_PRIMES(184, 222),
/**
* GHS_ANDROID_PRIMES = 223;
*
*
* Primes for Google AdWords Express android app (go/primes, go/awx-android)
*
*/
GHS_ANDROID_PRIMES(185, 223),
/**
* ADWORDS_MOBILE_ANDROID_PRIMES = 224;
*
*
* Primes for Google Home Services android app (go/primes, go/sab-awx-android)
*
*/
ADWORDS_MOBILE_ANDROID_PRIMES(186, 224),
/**
* TAP_AND_PAY_ANDROID_PRIMES = 227;
*
*
* AdWords system health (go/awm, go/primes)
*
*/
TAP_AND_PAY_ANDROID_PRIMES(187, 227),
/**
* WALLET_APP_ANDROID_PRIMES = 232;
*
*
* Primes for Tap and Pay android app, aka Android pay app (go/tp2)
*
*/
WALLET_APP_ANDROID_PRIMES(188, 232),
/**
* WALLET_APP_IOS_PRIMES = 233;
*
*
* Primes for Wallet android app (go/gmoney)
*
*/
WALLET_APP_IOS_PRIMES(189, 233),
/**
* ANALYTICS_ANDROID_PRIMES = 235;
*
*
* Primes for Wallet iOS app (go/gmoney)
*
*/
ANALYTICS_ANDROID_PRIMES(190, 235),
/**
* SPACES_ANDROID_PRIMES = 236;
*
*
* Logs for system health metric for Analytics Android app (go/primes)
*
*/
SPACES_ANDROID_PRIMES(191, 236),
/**
* SPACES_IOS_PRIMES = 276;
*
*
* Logs for the Spaces Android App (go/spaces)
*
*/
SPACES_IOS_PRIMES(192, 276),
/**
* SOCIETY_ANDROID_PRIMES = 238;
*
*
* Logs for the Spaces iOS App (go/spaces)
*
*/
SOCIETY_ANDROID_PRIMES(193, 238),
/**
* GMM_BRIIM_PRIMES = 239;
*
*
* Logs for system health metric for Society (go/primes, go/society)
*
*/
GMM_BRIIM_PRIMES(194, 239),
/**
* CW_PRIMES = 242;
*
*
* Logs for system health metric for GMM BRIIM (go/gmm-briim)
*
*/
CW_PRIMES(195, 242),
/**
* FAMILYLINK_ANDROID_PRIMES = 244;
*
*
* Clockwork go/primes logs
*
*/
FAMILYLINK_ANDROID_PRIMES(196, 244),
/**
* FAMILYLINK_IOS_PRIMES = 291;
*
*
* Logs for system health metric for FamilyLink (go/primes)
*
*/
FAMILYLINK_IOS_PRIMES(197, 291),
/**
* WH_PRIMES = 248;
*
*
* Logs for ios system health metric for FamilyLink (go/primes-ios, go/familylink-ios-app)
*
*/
WH_PRIMES(198, 248),
/**
* NOVA_ANDROID_PRIMES = 249;
*
*
* Primes for Westinghouse (go/westinghouse, go/primes)
*
*/
NOVA_ANDROID_PRIMES(199, 249),
/**
* PHOTOS_DRAPER_ANDROID_PRIMES = 253;
*
*
* Nova client app system health metrics (go/primes)
*
*/
PHOTOS_DRAPER_ANDROID_PRIMES(200, 253),
/**
* GMM_PRIMES = 254;
*
*
* Logs for system health metric for Photos Draper (go/primes, go/photos-draper)
*
*/
GMM_PRIMES(201, 254),
/**
* TRANSLATE_ANDROID_PRIMES = 255;
*
*
* Logs for system health metric for aGMM (go/gmm)
*
*/
TRANSLATE_ANDROID_PRIMES(202, 255),
/**
* TRANSLATE_IOS_PRIMES = 256;
*
*
* Logs for system health metric for Translate on Android (go/primes)
*
*/
TRANSLATE_IOS_PRIMES(203, 256),
/**
* FLYDROID_ANDROID_PRIMES = 259;
*
*
* Logs for system health metric for Translate on iOS (go/primes)
*
*/
FLYDROID_ANDROID_PRIMES(204, 259),
/**
* CONSUMERIQ_PRIMES = 260;
*
*
* Log for system health metric for Freighter (go/freighter)
*
*/
CONSUMERIQ_PRIMES(205, 260),
/**
* GMB_ANDROID_PRIMES = 263;
*
*
* Log for system health metric for Consumer IQ (go/primes)
*
*/
GMB_ANDROID_PRIMES(206, 263),
/**
* CLOUDDPC_PRIMES = 304;
*
*
* Primes for Google My Business android app (go/gmb-android, go/primes).
*
*/
CLOUDDPC_PRIMES(207, 304),
/**
* CLOUDDPC_ARC_PRIMES = 305;
*
*
* Primes for CloudDpc (go/clouddpc)
*
*/
CLOUDDPC_ARC_PRIMES(208, 305),
/**
* ICORE = 137;
*
*
* Primes for CloudDpc for ARC (go/clouddpc)
*
*/
ICORE(209, 137),
/**
* PANCETTA_MOBILE_HOST = 138;
*
*
* iOS logging (go/ios-logging)
*
*/
PANCETTA_MOBILE_HOST(210, 138),
/**
* PANCETTA_MOBILE_HOST_COUNTERS = 139;
*
*
* Logs for Pancetta mobile hosts (go/pancetta)
*
*/
PANCETTA_MOBILE_HOST_COUNTERS(211, 139),
/**
* CROSSDEVICENOTIFICATION = 141;
*
*
* Counters for Pancetta mobile hosts (go/pancetta)
*
*/
CROSSDEVICENOTIFICATION(212, 141),
/**
* CROSSDEVICENOTIFICATION_DEV = 142;
*
*
* Logs for cross device notification service (go/hotpocket)
*
*/
CROSSDEVICENOTIFICATION_DEV(213, 142),
/**
* MAPS_API_COUNTERS = 143;
*
*
* Dev logs for cross device notification service (go/hotpocket)
*
*/
MAPS_API_COUNTERS(214, 143),
/**
* GPU = 144;
*
*
* Android and iOS Maps API (go/mapview & go/meridian)
*
*/
GPU(215, 144),
/**
* ON_THE_GO = 145;
*
*
* Logs for Geo Photo Uploader (go/gpu-site)
*
*/
ON_THE_GO(216, 145),
/**
* ON_THE_GO_COUNTERS = 146;
*
*
* Logs for OnTheGo (go/onthego)
*
*/
ON_THE_GO_COUNTERS(217, 146),
/**
* GMS_CORE_PEOPLE_AUTOCOMPLETE = 147;
*
*
* Counters for OnTheGo (go/onthego)
*
*/
GMS_CORE_PEOPLE_AUTOCOMPLETE(218, 147),
/**
* FLYDROID_COUNTERS = 148;
*
*
* Logs for Gcore People Autocomplete (go/yenta)
*
*/
FLYDROID_COUNTERS(219, 148),
/**
* FIREBALL = 149;
*
*
* Logs for Flydroid (go/flydroid).
*
*/
FIREBALL(220, 149),
/**
* FIREBALL_COUNTERS = 257;
*
*
* Logs for Fireball (go/fireballdev)
*
*/
FIREBALL_COUNTERS(221, 257),
/**
* CRONET_FIREBALL = 303;
*
*
* Fireball counters (go/fireballdev)
*
*/
CRONET_FIREBALL(222, 303),
/**
* FIREBALL_PRIMES = 266;
*
*
* Fireball cronet (go/fireballdev)
*
*/
FIREBALL_PRIMES(223, 266),
/**
* FIREBALL_IOS_PRIMES = 313;
*
*
* Logs for system health for Fireball (go/fireball-systemhealth-plan)
*
*/
FIREBALL_IOS_PRIMES(224, 313),
/**
* GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES = 314;
*
*
* Logs for system health for Fireball iOS (go/fireball-systemhealth-plan)
*
*/
GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES(225, 314),
/**
* PYROCLASM = 153;
*
*
* Primes for Google Handwriting Input (go/hwrime)
*
*/
PYROCLASM(226, 153),
/**
* ANDROID_GSA_COUNTERS = 161;
*
*
* Logs for Pyroclasm (go/pyroclasm)
*
*/
ANDROID_GSA_COUNTERS(227, 161),
/**
* JAM_IMPRESSIONS = 162;
*
*
* Logs for AGSA counters.
*
*/
JAM_IMPRESSIONS(228, 162),
/**
* JAM_KIOSK_IMPRESSIONS = 163;
*
*
* Impressions logs for Jam (go/jam).
*
*/
JAM_KIOSK_IMPRESSIONS(229, 163),
/**
* PAYMENTS_OCR = 164;
*
*
* Impressions logs for Jam Kiosk (go/jam).
*
*/
PAYMENTS_OCR(230, 164),
/**
* UNICORN_FAMILY_MANAGEMENT = 172;
*
*
* Logs for payments ocr (go/cc-ocr-metrics).
*
*/
UNICORN_FAMILY_MANAGEMENT(231, 172),
/**
* AUDITOR = 173;
*
*
* Logs for Family management (and onboarding) go/familymanagement
*
*/
AUDITOR(232, 173),
/**
* NQLOOKUP = 174;
*
*
* Logs for Accesssibility Auditor (go/a11y-checker)
*
*/
NQLOOKUP(233, 174),
/**
* ANDROID_GSA_HIGH_PRIORITY_EVENTS = 175;
*
*
* Logs for NQLookup (go/nqlookup, contact [email protected])
*
*/
ANDROID_GSA_HIGH_PRIORITY_EVENTS(234, 175),
/**
* ANDROID_DIALER = 176;
*
*
* Logs for high priority events in AGSA (go/click-tracking-agsa).
*
*/
ANDROID_DIALER(235, 176),
/**
* CLEARCUT_DEMO = 177;
*
*
* Logs for Android Dialer (go/android-dialer)
*
*/
CLEARCUT_DEMO(236, 177),
/**
* APPMANAGER = 183;
*
*
* Logs for Clearcut's demo application
*
*/
APPMANAGER(237, 183),
/**
* SMARTLOCK_COUNTERS = 184;
*
*
* Logs for Firebase App Manager (go/appmanager)
*
*/
SMARTLOCK_COUNTERS(238, 184),
/**
* EXPEDITIONS_GUIDE = 185;
*
*
* Counters for Smart Lock (go/smartlock)
*
*/
EXPEDITIONS_GUIDE(239, 185),
/**
* FUSE = 186;
*
*
* Guide-aggregated logs for go/expeditions .
*
*/
FUSE(240, 186),
/**
* PIXEL_PERFECT_CLIENT_STATE_LOGGER = 187;
*
*
* Logs for Fuse (go/fuse).
*
*/
PIXEL_PERFECT_CLIENT_STATE_LOGGER(241, 187),
/**
* PLATFORM_STATS_COUNTERS = 188;
*
*
* Logs for PixelPerfect client app (go/pxp-client)
*
*/
PLATFORM_STATS_COUNTERS(242, 188),
/**
* DRIVE_VIEWER = 189;
*
*
* Counters for platform stats.(go/androidplatformstats, clearcut-eng@)
*
*/
DRIVE_VIEWER(243, 189),
/**
* PDF_VIEWER = 190;
*
*
* Google Drive Viewer Android and iOS apps (go/picoprojector)
*
*/
PDF_VIEWER(244, 190),
/**
* BIGTOP = 191;
*
*
* Google PDF Viewer Android app (go/picoprojector)
*
*/
BIGTOP(245, 191),
/**
* VOICE = 192;
*
*
* Logs for Bigtop (externally known as Inbox, go/bigtop).
*
*/
VOICE(246, 192),
/**
* MYFIBER = 194;
*
*
* Logs for Google Voice (go/googlevoice).
*
*/
MYFIBER(247, 194),
/**
* RECORDED_PAGES = 195;
*
*
* Logs for My Fiber mobile applications (go/myfibernative)
*
*/
RECORDED_PAGES(248, 195),
/**
* MOB_DOG = 197;
*
*
* Logs for RecordedPages (go/recorded-pages)
*
*/
MOB_DOG(249, 197),
/**
* WALLET_APP = 198;
*
*
* Logs for MobDog (go/mobdog)
*
*/
WALLET_APP(250, 198),
/**
* GBOARD = 199;
*
*
* Logs for the Wallet app (go/gmoney)
*
*/
GBOARD(251, 199),
/**
* CRONET_GMM = 203;
*
*
* Logs for GBoard (go/gboard)
*
*/
CRONET_GMM(252, 203),
/**
* TRUSTED_FACE = 204;
*
*
* Cronet UMA logs from GMM (go/cronet-uma)
*
*/
TRUSTED_FACE(253, 204),
/**
* MATCHSTICK = 205;
*
*
* Logs for Trusted Face (go/smartlock)
*
*/
MATCHSTICK(254, 205),
/**
* APP_CATALOG = 206;
*
*
* Logs for Matchstick library (go/matchstick)
*
*/
APP_CATALOG(255, 206),
/**
* BLUETOOTH = 208;
*
*
* Logs for AppCatalog aka "Get My Google Apps" (go/growth)
*
*/
BLUETOOTH(256, 208),
/**
* WIFI = 209;
*
*
* Logs for Bluetooth (go/fluoride)
*
*/
WIFI(257, 209),
/**
* TELECOM = 210;
*
*
* Logs for WiFi (go/connectivity-site)
*
*/
TELECOM(258, 210),
/**
* TELEPHONY = 211;
*
*
* Logs for Telcom (go/connectivity-site)
*
*/
TELEPHONY(259, 211),
/**
* IDENTITY_FRONTEND = 212;
*
*
* Logs for Telephony (go/connectivity-site)
*
*/
IDENTITY_FRONTEND(260, 212),
/**
* SESAME = 216;
*
*
* Logs for the Identity frontend (go/ifus-readme)
*
*/
SESAME(261, 216),
/**
* GOOGLE_KEYBOARD_CONTENT = 217;
*
*
* Logs for Sesame (go/sesame-logging)
*
*/
GOOGLE_KEYBOARD_CONTENT(262, 217),
/**
* MADDEN = 218;
*
*
* Google Keyboard's Latin IME content logs (go/google-keyboard)
*
*/
MADDEN(263, 218),
/**
* INK = 219;
*
*
* Logs for Madden (go/madden)
*
*/
INK(264, 219),
/**
* ANDROID_CONTACTS = 220;
*
*
* Logs for Ink (go/ink)
*
*/
ANDROID_CONTACTS(265, 220),
/**
* GOOGLE_KEYBOARD_COUNTERS = 221;
*
*
* Logs for Android Contacts (go/android-contacts)
*
*/
GOOGLE_KEYBOARD_COUNTERS(266, 221),
/**
* CLEARCUT_PROBER = 225;
*
*
* Google Keyboard histogram counters (go/google-keyboard)
*
*/
CLEARCUT_PROBER(267, 225),
/**
* PLAY_CONSOLE_APP = 226;
*
*
* Clearcut prober (go/clearcut-prober)
*
*/
PLAY_CONSOLE_APP(268, 226),
/**
* PLAY_CONSOLE_APP_PRIMES = 264;
*
*
* Logs for the Google Play Developer Console app
*
*/
PLAY_CONSOLE_APP_PRIMES(269, 264),
/**
* SPECTRUM = 230;
*
*
* Log for system health metrics for the Google Play Developer Console app
*
*/
SPECTRUM(270, 230),
/**
* SPECTRUM_COUNTERS = 231;
*
*
* Spectrum (go/spectrum-site)
*
*/
SPECTRUM_COUNTERS(271, 231),
/**
* SPECTRUM_ANDROID_PRIMES = 267;
*
*
* Spectrum (go/spectrum-site)
*
*/
SPECTRUM_ANDROID_PRIMES(272, 267),
/**
* IOS_SPOTLIGHT_SEARCH_LIBRARY = 234;
*
*
* Spectrum (go/spectrum-site)
*
*/
IOS_SPOTLIGHT_SEARCH_LIBRARY(273, 234),
/**
* BOQ_WEB = 241;
*
*
* Logs for the iOS Spotlight Search Library (go/tempeh-dd)
*
*/
BOQ_WEB(274, 241),
/**
* ORCHESTRATION_CLIENT = 245;
*
*
* Logs for client-side analytics of Boq Web (go/boq-web)
*
*/
ORCHESTRATION_CLIENT(275, 245),
/**
* ORCHESTRATION_CLIENT_DEV = 246;
*
*
* Orchestration client logs for prod environment (go/megalogs-design)
*
*/
ORCHESTRATION_CLIENT_DEV(276, 246),
/**
* GOOGLE_NOW_LAUNCHER = 247;
*
*
* Orchestration client logs for dev environment (go/megalogs-design)
*
*/
GOOGLE_NOW_LAUNCHER(277, 247),
/**
* SCOOBY_SPAM_REPORT_LOG = 250;
*
*
* Logs for Google Now Launcher (go/gnl-logging)
*
*/
SCOOBY_SPAM_REPORT_LOG(278, 250),
/**
* IOS_GROWTH = 251;
*
*
* Logs of spam reports by scooby clients. (go/scooby)
*
*/
IOS_GROWTH(279, 251),
/**
* APPS_NOTIFY = 252;
*
*
* go/growthkit-metrics
*
*/
APPS_NOTIFY(280, 252),
/**
* SMARTKEY_APP = 269;
*
*
* Drive web push notifications (go/apps-notify)
*
*/
SMARTKEY_APP(281, 269),
/**
* CLINICAL_STUDIES = 270;
*
*
* Smart Key App for project Bolt (go/smartlock)
*
*/
CLINICAL_STUDIES(282, 270),
/**
* FITNESS_ANDROID_PRIMES = 271;
*
*
* Lifescience Clinical Studies logs (go/apollox)
*
*/
FITNESS_ANDROID_PRIMES(283, 271),
/**
* IMPROV_APPS = 272;
*
*
* System health metric for Google Fit Android App (go/heart, go/primes)
*
*/
IMPROV_APPS(284, 272),
/**
* FAMILYLINK = 273;
*
*
* Logs for Improv apps (go/improvlogging)
*
*/
FAMILYLINK(285, 273),
/**
* FAMILYLINK_COUNTERS = 274;
*
*
* Family Link (go/familylink-dd)
*
*/
FAMILYLINK_COUNTERS(286, 274),
/**
* SOCIETY = 275;
*
*
* Family Link counters (go/familylink-dd)
*
*/
SOCIETY(287, 275),
/**
* DIALER_ANDROID_PRIMES = 277;
*
*
* NBU Society app (go/society)
*
*/
DIALER_ANDROID_PRIMES(288, 277),
/**
* YOUTUBE_DIRECTOR_APP = 278;
*
*
* Android Dialer Primes (go/dialer)
*
*/
YOUTUBE_DIRECTOR_APP(289, 278),
/**
* TACHYON_ANDROID_PRIMES = 279;
*
*
* YouTube Director App (go/youtube-director)
*
*/
TACHYON_ANDROID_PRIMES(290, 279),
/**
* DRIVE_FS = 280;
*
*
* Android Tachyon Client
*
*/
DRIVE_FS(291, 280),
/**
* YT_MAIN = 281;
*
*
* DriveFS (go/drive-fs)
*
*/
YT_MAIN(292, 281),
/**
* WING_MARKETPLACE_ANDROID_PRIMES = 282;
*
*
* Logs for YT's main application
*
*/
WING_MARKETPLACE_ANDROID_PRIMES(293, 282),
/**
* DYNAMITE = 283;
*
*
* Primes for Wing Marketplace Android app (go/wing-marketplace-android, go/primes)
*
*/
DYNAMITE(294, 283),
/**
* CORP_ANDROID_FOOD = 284;
*
*
* Logs for Dynamite (go/dynamite)
*
*/
CORP_ANDROID_FOOD(295, 284),
/**
* ANDROID_MESSAGING_PRIMES = 285;
*
*
* Logs for the internal Android Food app (g3doc/java/com/google/corp/bizapps/rews/food/android)
*
*/
ANDROID_MESSAGING_PRIMES(296, 285),
/**
* GPLUS_IOS_PRIMES = 286;
*
*
* Primes for Android Messenger (go/bugledev, go/primes)
*
*/
GPLUS_IOS_PRIMES(297, 286),
/**
* CHROMECAST_ANDROID_PRIMES = 288;
*
*
* Primes for Google+ iOS app (go/gplus-ios, go/primes)
*
*/
CHROMECAST_ANDROID_PRIMES(298, 288),
/**
* APPSTREAMING = 289;
*
*
* Primes for Chromecast Android app (go/cast-apps)
*
*/
APPSTREAMING(299, 289),
/**
* GMB_ANDROID = 290;
*
*
* Logs for AppStreaming Client Framework Side (go/piccard)
*
*/
GMB_ANDROID(300, 290),
/**
* VOICE_IOS_PRIMES = 292;
*
*
* Logs for GMB Android app (go/gmb-android).
*
*/
VOICE_IOS_PRIMES(301, 292),
/**
* VOICE_ANDROID_PRIMES = 293;
*
*
* Primes for iOS Google Voice (go/googlevoice).
*
*/
VOICE_ANDROID_PRIMES(302, 293),
/**
* PAISA = 294;
*
*
* Primes for Android Google Voice (go/googlevoice).
*
*/
PAISA(303, 294),
/**
* GMB_IOS = 295;
*
*
* NBU Paisa app (go/paisa)
*
*/
GMB_IOS(304, 295),
/**
* SCOOBY_EVENTS = 296;
*
*
* Logs for GMB iOS App (go/gmb-ios).
*
*/
SCOOBY_EVENTS(305, 296),
/**
* SNAPSEED_IOS_PRIMES = 297;
*
*
* Call logs events by scooby clients. (go/scooby)
*
*/
SNAPSEED_IOS_PRIMES(306, 297),
/**
* YOUTUBE_DIRECTOR_IOS_PRIMES = 298;
*
*
* Primes for Snapseed IOS (go/Snapseed)
*
*/
YOUTUBE_DIRECTOR_IOS_PRIMES(307, 298),
/**
* WALLPAPER_PICKER = 299;
*
*
* YouTube Director App (go/youtube-director)
*
*/
WALLPAPER_PICKER(308, 299),
/**
* CHIME = 300;
*
*
* Android wallpaper picker (go/magic-wallpapers)
*
*/
CHIME(309, 300),
/**
* BEACON_GCORE = 301;
*
*
* Chime (go/chime)
*
*/
BEACON_GCORE(310, 301),
/**
* ANDROID_STUDIO = 302;
*
*
* Logs for debugging beacon module (go/beacons)
*
*/
ANDROID_STUDIO(311, 302),
/**
* DOCS_OFFLINE = 306;
*
*
* Android Studio (go/android-studio)
*
*/
DOCS_OFFLINE(312, 306),
/**
* FREIGHTER = 307;
*
*
* Logs for Docs Offline on Desktop (go/jetpax)
*
*/
FREIGHTER(313, 307),
/**
* DOCS_IOS_PRIMES = 308;
*
*
* Logs for Freighter (http://go/freighter)
*
*/
DOCS_IOS_PRIMES(314, 308),
/**
* SLIDES_IOS_PRIMES = 309;
*
*
* Logs for the Google Docs iOS application
*
*/
SLIDES_IOS_PRIMES(315, 309),
/**
* SHEETS_IOS_PRIMES = 310;
*
*
* Logs for the Google Docs iOS application
*
*/
SHEETS_IOS_PRIMES(316, 310),
/**
* IPCONNECTIVITY = 311;
*
*
* Logs for the Google Docs iOS application
*
*/
IPCONNECTIVITY(317, 311),
/**
* CURATOR = 312;
*
*
* Logs for Networking (go/connectivity-site)
*
*/
CURATOR(318, 312),
;
/**
* GPLUS_ANDROID_SYSTEM_HEALTH = 140;
*
*
* Logs for system health metric for Gplus (go/primes)
*
*/
public static final LogSource GPLUS_ANDROID_SYSTEM_HEALTH = GPLUS_ANDROID_PRIMES;
/**
* GMAIL_ANDROID_SYSTEM_HEALTH = 150;
*
*
* Logs for system health metric for GMail (go/primes)
*
*/
public static final LogSource GMAIL_ANDROID_SYSTEM_HEALTH = GMAIL_ANDROID_PRIMES;
/**
* CALENDAR_ANDROID_SYSTEM_HEALTH = 151;
*
*
* Logs for system health metric for Calendar (go/primes)
*
*/
public static final LogSource CALENDAR_ANDROID_SYSTEM_HEALTH = CALENDAR_ANDROID_PRIMES;
/**
* DOCS_ANDROID_SYSTEM_HEALTH = 152;
*
*
* Logs for system health metric for Docs (go/primes)
*
*/
public static final LogSource DOCS_ANDROID_SYSTEM_HEALTH = DOCS_ANDROID_PRIMES;
/**
* UNKNOWN = -1;
*/
public static final int UNKNOWN_VALUE = -1;
/**
* STORE = 0;
*/
public static final int STORE_VALUE = 0;
/**
* WEB_STORE = 65;
*
*
* Google Play Store Android App
*
*/
public static final int WEB_STORE_VALUE = 65;
/**
* WORK_STORE = 132;
*
*
* Google Play Web Store (https://play.google.com/store)
*
*/
public static final int WORK_STORE_VALUE = 132;
/**
* WORK_STORE_APP = 261;
*
*
* Play for Work on the web client (Worksky)
*
*/
public static final int WORK_STORE_APP_VALUE = 261;
/**
* EDU_STORE = 15;
*
*
* Play for Work on the Google Play Store Android App
*
*/
public static final int EDU_STORE_VALUE = 15;
/**
* MUSIC = 1;
*
*
* for collecting stats on Play for Education
*
*/
public static final int MUSIC_VALUE = 1;
/**
* BOOKS = 2;
*/
public static final int BOOKS_VALUE = 2;
/**
* VIDEO = 3;
*/
public static final int VIDEO_VALUE = 3;
/**
* MOVIES = 74;
*
*
* Android Video (go/avi, android-video-oncall@)
*
*/
public static final int MOVIES_VALUE = 74;
/**
* MAGAZINES = 4;
*/
public static final int MAGAZINES_VALUE = 4;
/**
* GAMES = 5;
*/
public static final int GAMES_VALUE = 5;
/**
* LB_A = 6;
*/
public static final int LB_A_VALUE = 6;
/**
* ANDROID_IDE = 7;
*
*
* for collecting app usage stats (Lockbox project)
*
*/
public static final int ANDROID_IDE_VALUE = 7;
/**
* LB_P = 8;
*
*
* Android IDE (deprecated, see ANDROID_STUDIO below)
*
*/
public static final int LB_P_VALUE = 8;
/**
* LB_S = 9;
*
*
* for collecting phone call events and stats (Lockbox project)
*
*/
public static final int LB_S_VALUE = 9;
/**
* GMS_CORE = 10;
*
*
* for collecting SMS events and stats (Lockbox project)
*
*/
public static final int GMS_CORE_VALUE = 10;
/**
* APP_USAGE_1P = 11;
*
*
* Usage of GMS Core itself and its internal stats
*
*/
public static final int APP_USAGE_1P_VALUE = 11;
/**
* ICING = 12;
*
*
* First-party app usage tracking.
*
*/
public static final int ICING_VALUE = 12;
/**
* HERREVAD = 13;
*
*
* Icing stats (AppDataSearch go/icing)
*
*/
public static final int HERREVAD_VALUE = 13;
/**
* GOOGLE_TV = 14;
*
*
* for collecting Nova Network quality data
*
*/
public static final int GOOGLE_TV_VALUE = 14;
/**
* GMS_CORE_PEOPLE = 16;
*
*
* Google TV, not Eureka.
*
*/
public static final int GMS_CORE_PEOPLE_VALUE = 16;
/**
* LE = 17;
*
*
* GMS core people (aka menagerie, zhengfu@, android-contacts@)
*
*/
public static final int LE_VALUE = 17;
/**
* GOOGLE_ANALYTICS = 18;
*
*
* Location Engine statistics
*
*/
public static final int GOOGLE_ANALYTICS_VALUE = 18;
/**
* LB_D = 19;
*
*
* Google Analytics SDK health monitoring
*
*/
public static final int LB_D_VALUE = 19;
/**
* ANDROID_GSA = 20;
*
*
* for collecting device state changes (Lockbox project)
*
*/
public static final int ANDROID_GSA_VALUE = 20;
/**
* LB_T = 21;
*
*
* Android GSA (Google Search App) stats
*
*/
public static final int LB_T_VALUE = 21;
/**
* PERSONAL_LOGGER = 22;
*
*
* for collecting Android RunningTaskInfo (Lockbox project)
*
*/
public static final int PERSONAL_LOGGER_VALUE = 22;
/**
* PERSONAL_BROWSER_LOGGER = 37;
*
*
* PixelPerfect activity logger
*
*/
public static final int PERSONAL_BROWSER_LOGGER_VALUE = 37;
/**
* GMS_CORE_WALLET_MERCHANT_ERROR = 23;
*
*
* PixelPerfect Chrome extension logger
*
*/
public static final int GMS_CORE_WALLET_MERCHANT_ERROR_VALUE = 23;
/**
* LB_C = 24;
*
*
* GMS core wallet merchant errors
*
*/
public static final int LB_C_VALUE = 24;
/**
* LB_IA = 52;
*
*
* Android contacts going from Now to HappyHour.log_event_extension_test
*
*/
public static final int LB_IA_VALUE = 52;
/**
* LB_CB = 237;
*
*
* Android installed apps going from Now to HappyHour.
*
*/
public static final int LB_CB_VALUE = 237;
/**
* LB_DM = 268;
*
*
* Android contacts for backup/restore purpose.
*
*/
public static final int LB_DM_VALUE = 268;
/**
* ANDROID_AUTH = 25;
*
*
* Android device metadata used for contacts backup/restore
* purpose.
*
*/
public static final int ANDROID_AUTH_VALUE = 25;
/**
* ANDROID_CAMERA = 26;
*
*
* for collecting GMS core android auth data
*
*/
public static final int ANDROID_CAMERA_VALUE = 26;
/**
* CW = 27;
*
*
* Android camera usage statistics
*
*/
public static final int CW_VALUE = 27;
/**
* CW_COUNTERS = 243;
*
*
* Clockwork interaction logs
*
*/
public static final int CW_COUNTERS_VALUE = 243;
/**
* GEL = 28;
*
*
* Clockwork counters
*
*/
public static final int GEL_VALUE = 28;
/**
* DNA_PROBER = 29;
*
*
* Google experience launcher logs (http://go/signac).
*
*/
public static final int DNA_PROBER_VALUE = 29;
/**
* UDR = 30;
*
*
* DNA logger (for internal research), go/dna2014.
*
*/
public static final int UDR_VALUE = 30;
/**
* GMS_CORE_WALLET = 31;
*
*
* For collecting Android app usage data.
*
*/
public static final int GMS_CORE_WALLET_VALUE = 31;
/**
* SOCIAL = 32;
*
*
* GMS core wallet analytics
*
*/
public static final int SOCIAL_VALUE = 32;
/**
* INSTORE_WALLET = 33;
*
*
* Traffic from Social clients: gplus-log-quality@
*
*/
public static final int INSTORE_WALLET_VALUE = 33;
/**
* NOVA = 34;
*
*
* Instore play payments
*
*/
public static final int NOVA_VALUE = 34;
/**
* LB_CA = 35;
*
*
* Nova client logs.
*
*/
public static final int LB_CA_VALUE = 35;
/**
* LATIN_IME = 36;
*
*
* DEPRECATED: Android contact account data going from Now to Footprints.
*
*/
public static final int LATIN_IME_VALUE = 36;
/**
* NEWS_WEATHER = 38;
*
*
* Google Keyboard (http://go/google-keyboard)
*
*/
public static final int NEWS_WEATHER_VALUE = 38;
/**
* HANGOUT = 39;
*
*
* News & Weather Android/iOS apps
*
*/
public static final int HANGOUT_VALUE = 39;
/**
* HANGOUT_LOG_REQUEST = 50;
*
*
* Deprecated source for HangoutLogEntry (rtc-analytics-eng@)
*
*/
public static final int HANGOUT_LOG_REQUEST_VALUE = 50;
/**
* COPRESENCE = 40;
*
*
* Hangouts data using HangoutLogRequest (rtc-analytics-eng@)
*
*/
public static final int COPRESENCE_VALUE = 40;
/**
* SOCIAL_AFFINITY = 41;
*
*
* Copresence (http://go/whisper)
*
*/
public static final int SOCIAL_AFFINITY_VALUE = 41;
/**
* PHOTOS = 42;
*
*
* Social Affinity logs (go/disco-logging-v2).
*
*/
public static final int PHOTOS_VALUE = 42;
/**
* GCM = 43;
*
*
* Google Photos (go/photos).
*
*/
public static final int GCM_VALUE = 43;
/**
* GOKART = 44;
*
*
* Google Cloud Messaging logs (go/gcm).
*
*/
public static final int GOKART_VALUE = 44;
/**
* FINDR = 45;
*
*
* GoKart (go/gokart, Drive Android API)
*
*/
public static final int FINDR_VALUE = 45;
/**
* ANDROID_MESSAGING = 46;
*
*
* Findr app (go/findr).
*
*/
public static final int ANDROID_MESSAGING_VALUE = 46;
/**
* SOCIAL_WEB = 47;
*
*
* Android messenger(Bugle).
*
*/
public static final int SOCIAL_WEB_VALUE = 47;
/**
* BACKDROP = 48;
*
*
* Traffic from Social clients: gplus-log-quality@.
*
*/
public static final int BACKDROP_VALUE = 48;
/**
* TELEMATICS = 49;
*
*
* Backdrop device logs (go/tv-imax).
*
*/
public static final int TELEMATICS_VALUE = 49;
/**
* GVC_HARVESTER = 51;
*
*
* Telematics app (go/telematics).
*
*/
public static final int GVC_HARVESTER_VALUE = 51;
/**
* CAR = 53;
*
*
* GVC Harvester (go/harvesterdesigndoc)
*
*/
public static final int CAR_VALUE = 53;
/**
* PIXEL_PERFECT = 54;
*
*
* Android Automotive (go/gearhead).
*
*/
public static final int PIXEL_PERFECT_VALUE = 54;
/**
* DRIVE = 55;
*
*
* PixelPerfect app client log (go/pixelperfect-analytics)
*
*/
public static final int DRIVE_VALUE = 55;
/**
* DOCS = 56;
*
*
* Google Drive (go/cakemix, nickread@, cakemix-drive@)
*
*/
public static final int DOCS_VALUE = 56;
/**
* SHEETS = 57;
*
*
* Google Docs (go/cakemix, haluk@, bulach@, tstrauss@)
*
*/
public static final int SHEETS_VALUE = 57;
/**
* SLIDES = 58;
*
*
* Google Sheets (go/cakemix, tgleason@, bulach@, tstrauss@)
*
*/
public static final int SLIDES_VALUE = 58;
/**
* IME = 59;
*
*
* Google Slides (go/cakemix, yugacohler@, bulach@, tstrauss@)
*
*/
public static final int IME_VALUE = 59;
/**
* WARP = 60;
*
*
* Google Android IME logs (go/goopy).
*
*/
public static final int WARP_VALUE = 60;
/**
* NFC_PROGRAMMER = 61;
*
*
* Warp (go/warp-project)
*
*/
public static final int NFC_PROGRAMMER_VALUE = 61;
/**
* NETSTATS = 62;
*
*
* NfcProgrammer (go/aedu-platform-overview)
*
*/
public static final int NETSTATS_VALUE = 62;
/**
* NEWSSTAND = 63;
*
*
* Collection of Network Statistics (go/n-s).
*
*/
public static final int NEWSSTAND_VALUE = 63;
/**
* KIDS_COMMUNICATOR = 64;
*
*
* Google Play Newsstand.
*
*/
public static final int KIDS_COMMUNICATOR_VALUE = 64;
/**
* WIFI_ASSISTANT = 66;
*
*
* Kids Communicator (http://goto/kidscommunicator_dd)
*
*/
public static final int WIFI_ASSISTANT_VALUE = 66;
/**
* CAST_SENDER_SDK = 67;
*
*
* WiFi Assistant client logs.
*
*/
public static final int CAST_SENDER_SDK_VALUE = 67;
/**
* CRONET_SOCIAL = 68;
*
*
* Cast Sender SDK (go/dongle)
*
*/
public static final int CRONET_SOCIAL_VALUE = 68;
/**
* PHENOTYPE = 69;
*
*
* Social cronet metrics.
*
*/
public static final int PHENOTYPE_VALUE = 69;
/**
* PHENOTYPE_COUNTERS = 70;
*
*
* The client side of experiment framework (go/ph, peiling@, phenotype-eng@).
*
*/
public static final int PHENOTYPE_COUNTERS_VALUE = 70;
/**
* CHROME_INFRA = 71;
*
*
* The counters for Phenotype (go/ph, peiling@, phenotype-eng@).
*
*/
public static final int CHROME_INFRA_VALUE = 71;
/**
* JUSTSPEAK = 72;
*
*
* Chrome Infrastructure events
*
*/
public static final int JUSTSPEAK_VALUE = 72;
/**
* PERF_PROFILE = 73;
*
*
* JustSpeak (go/justspeak)
*
*/
public static final int PERF_PROFILE_VALUE = 73;
/**
* KATNISS = 75;
*
*
* Android Wide Profiling (go/android-wide-profiling)
*
*/
public static final int KATNISS_VALUE = 75;
/**
* SOCIAL_APPINVITE = 76;
*
*
* Android TV search (go/atv-search)
*
*/
public static final int SOCIAL_APPINVITE_VALUE = 76;
/**
* GMM_COUNTERS = 77;
*
*
* Google Plus Platform App Invite (go/appinvite)
*
*/
public static final int GMM_COUNTERS_VALUE = 77;
/**
* BOND_ONEGOOGLE = 78;
*
*
* Google Maps Mobile (go/gmm).
*
*/
public static final int BOND_ONEGOOGLE_VALUE = 78;
/**
* MAPS_API = 79;
*
*
* Bond OneGoogleBar integration (go/bond-ogb)
*
*/
public static final int MAPS_API_VALUE = 79;
/**
* CRONET_ANDROID_YT = 196;
*
*
* Android and iOS Maps API (go/mapview & go/meridian)
*
*/
public static final int CRONET_ANDROID_YT_VALUE = 196;
/**
* CRONET_ANDROID_GSA = 80;
*
*
* Cronet UMA logs from Android YT (go/cronet-uma)
*
*/
public static final int CRONET_ANDROID_GSA_VALUE = 80;
/**
* GOOGLE_FIT_WEARABLE = 81;
*
*
* Cronet UMA logs from Android GSA (go/cronet-uma)
*
*/
public static final int GOOGLE_FIT_WEARABLE_VALUE = 81;
/**
* FITNESS_ANDROID = 169;
*
*
* Google Fit Wearable App (go/heart)
*
*/
public static final int FITNESS_ANDROID_VALUE = 169;
/**
* FITNESS_GMS_CORE = 170;
*
*
* Google Fit Android App (go/heart)
*
*/
public static final int FITNESS_GMS_CORE_VALUE = 170;
/**
* GOOGLE_EXPRESS = 82;
*
*
* Google Fit GMSCore (go/heart)
*
*/
public static final int GOOGLE_EXPRESS_VALUE = 82;
/**
* GOOGLE_EXPRESS_DEV = 215;
*
*
* Google Express (go/gsx)
*
*/
public static final int GOOGLE_EXPRESS_DEV_VALUE = 215;
/**
* GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES = 228;
*
*
* Google Express (go/gsx) For DEV mall server
*
*/
public static final int GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES_VALUE = 228;
/**
* GOOGLE_EXPRESS_ANDROID_PRIMES = 229;
*
*
* Google Express (go/gsx) Courier App metric logging (go/primes)
*
*/
public static final int GOOGLE_EXPRESS_ANDROID_PRIMES_VALUE = 229;
/**
* GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES = 240;
*
*
* Google Express (go/gsx) App metric logging (go/primes)
*
*/
public static final int GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES_VALUE = 240;
/**
* SENSE = 83;
*
*
* Google Express (go/gsx) Store OMS App metric logging (go/primes)
*
*/
public static final int SENSE_VALUE = 83;
/**
* ANDROID_BACKUP = 84;
*
*
* Sense (go/sense)
*
*/
public static final int ANDROID_BACKUP_VALUE = 84;
/**
* VR = 85;
*
*
* Android Backup Service.
*
*/
public static final int VR_VALUE = 85;
/**
* IME_COUNTERS = 86;
*
*
* Virtual Reality and Google Cardboard (go/vr-team)
*
*/
public static final int IME_COUNTERS_VALUE = 86;
/**
* SETUP_WIZARD = 87;
*
*
* The counters for Google Android IME (go/goopy).
*
*/
public static final int SETUP_WIZARD_VALUE = 87;
/**
* EMERGENCY_ASSIST = 88;
*
*
* Setup Wizard (go/suw)
*
*/
public static final int EMERGENCY_ASSIST_VALUE = 88;
/**
* TRON = 89;
*
*
* Barton (go/barton-deprecation)
*
*/
public static final int TRON_VALUE = 89;
/**
* TRON_COUNTERS = 90;
*
*
* The event logs for Android Framework (go/tron, cwren@, khigaki@, tron-eng@).
*
*/
public static final int TRON_COUNTERS_VALUE = 90;
/**
* BATTERY_STATS = 91;
*
*
* The counters for Android Framework (go/tron, cwren@, khigaki@, tron-eng@).
*
*/
public static final int BATTERY_STATS_VALUE = 91;
/**
* DISK_STATS = 92;
*
*
* For collecting battery performance data from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
public static final int DISK_STATS_VALUE = 92;
/**
* GRAPHICS_STATS = 107;
*
*
* For collecting disk utilization stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
public static final int GRAPHICS_STATS_VALUE = 107;
/**
* PROC_STATS = 93;
*
*
* For collecting rendering performance stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
public static final int PROC_STATS_VALUE = 93;
/**
* DROP_BOX = 131;
*
*
* For collecting memory utilization stats from Android clients. (go/androidplatformstats, belland@, clearcut-eng@)
*
*/
public static final int DROP_BOX_VALUE = 131;
/**
* FINGERPRINT_STATS = 181;
*
*
* For collecting DropBox logs from Android clients.
*
*/
public static final int FINGERPRINT_STATS_VALUE = 181;
/**
* NOTIFICATION_STATS = 182;
*
*
* For collecting fingerprint stats from Android clients. (go/android-fingerprintstats)
*
*/
public static final int NOTIFICATION_STATS_VALUE = 182;
/**
* TAP_AND_PAY_GCORE = 94;
*
*
* For collecting notification stats from Android clients. (go/android-notificationstats)
*
*/
public static final int TAP_AND_PAY_GCORE_VALUE = 94;
/**
* A11YLOGGER = 95;
*
*
* Tap and Pay client service layer (go/tp2-eng)
*
*/
public static final int A11YLOGGER_VALUE = 95;
/**
* GCM_COUNTERS = 96;
*
*
* Accessibility Settings Logger (go/a11ylogger)
*
*/
public static final int GCM_COUNTERS_VALUE = 96;
/**
* PLACES_NO_GLS_CONSENT = 97;
*
*
* Google Cloud Messaging counters (go/gcm).
*
*/
public static final int PLACES_NO_GLS_CONSENT_VALUE = 97;
/**
* TACHYON_LOG_REQUEST = 98;
*
*
* Mobile Places API logs without GLS consent (go/ios-places-api).
*
*/
public static final int TACHYON_LOG_REQUEST_VALUE = 98;
/**
* TACHYON_COUNTERS = 99;
*
*
* WebRTC Tachyon (go/tachyon)
*
*/
public static final int TACHYON_COUNTERS_VALUE = 99;
/**
* VISION = 100;
*
*
* WebRTC Tachyon (go/tachyon)
*
*/
public static final int VISION_VALUE = 100;
/**
* SOCIAL_USER_LOCATION = 101;
*
*
* Vision (go/mobile-vision)
*
*/
public static final int SOCIAL_USER_LOCATION_VALUE = 101;
/**
* LAUNCHPAD_TOYS = 102;
*
*
* Location Sharing (go/location-sharing)
*
*/
public static final int LAUNCHPAD_TOYS_VALUE = 102;
/**
* METALOG_COUNTERS = 103;
*
*
* Launchpad Toys (go/launchpadtoys)
*
*/
public static final int METALOG_COUNTERS_VALUE = 103;
/**
* MOBILESDK_CLIENT = 104;
*
*
* Counters for metalog (go/clearcut-metalog, iamawong@, clearcut-eng@)
*
*/
public static final int MOBILESDK_CLIENT_VALUE = 104;
/**
* ANDROID_VERIFY_APPS = 105;
*
*
* go/greenhouse
*
*/
public static final int ANDROID_VERIFY_APPS_VALUE = 105;
/**
* ADSHIELD = 106;
*
*
* go/safety-net
*
*/
public static final int ADSHIELD_VALUE = 106;
/**
* SHERLOG = 108;
*
*
* go/adshield
*
*/
public static final int SHERLOG_VALUE = 108;
/**
* LE_ULR_COUNTERS = 109;
*
*
* Sherlog data (go/sherlog)
*
*/
public static final int LE_ULR_COUNTERS_VALUE = 109;
/**
* GMM_UE3 = 110;
*
*
* Counters for ULR (go/gcore-ulr). Location custom events use LE.
*
*/
public static final int GMM_UE3_VALUE = 110;
/**
* CALENDAR = 111;
*
*
* Logs for GMM analytics and sessions (go/gca-analytics)
*
*/
public static final int CALENDAR_VALUE = 111;
/**
* ENDER = 112;
*
*
* Google Android Calendar
*
*/
public static final int ENDER_VALUE = 112;
/**
* FAMILY_COMPASS = 113;
*
*
* Logs for Ender (go/ender)
*
*/
public static final int FAMILY_COMPASS_VALUE = 113;
/**
* TRANSOM = 114;
*
*
* go/familycompass
*
*/
public static final int TRANSOM_VALUE = 114;
/**
* TRANSOM_COUNTERS = 115;
*
*
* Transom client-side metrics (go/transom)
*
*/
public static final int TRANSOM_COUNTERS_VALUE = 115;
/**
* LB_AS = 116;
*
*
* Counters for transom metrics
*
*/
public static final int LB_AS_VALUE = 116;
/**
* LB_CFG = 117;
*
*
* App usage collected by the new UsageStats API (Lockbox project).
*
*/
public static final int LB_CFG_VALUE = 117;
/**
* IOS_GSA = 118;
*
*
* Device config collected by the new UsageStats API (Lockbox project).
*
*/
public static final int IOS_GSA_VALUE = 118;
/**
* TAP_AND_PAY_APP = 119;
*
*
* iOS GSA (Google Search App) stats
*
*/
public static final int TAP_AND_PAY_APP_VALUE = 119;
/**
* TAP_AND_PAY_APP_COUNTERS = 265;
*
*
* Tap and Pay android app, aka Android pay app (go/tp2)
*
*/
public static final int TAP_AND_PAY_APP_COUNTERS_VALUE = 265;
/**
* FLYDROID = 120;
*
*
* Tap and Pay android app counters
*
*/
public static final int FLYDROID_VALUE = 120;
/**
* CPANEL_APP = 121;
*
*
* Logs for Flydroid (go/flydroid).
*
*/
public static final int CPANEL_APP_VALUE = 121;
/**
* ANDROID_SNET_GCORE = 122;
*
*
* CPanel latency on mobile.
*
*/
public static final int ANDROID_SNET_GCORE_VALUE = 122;
/**
* ANDROID_SNET_IDLE = 123;
*
*
* go/safety-net
*
*/
public static final int ANDROID_SNET_IDLE_VALUE = 123;
/**
* ANDROID_SNET_JAR = 124;
*
*
* go/safety-net
*
*/
public static final int ANDROID_SNET_JAR_VALUE = 124;
/**
* CONTEXT_MANAGER = 125;
*
*
* go/safety-net
*
*/
public static final int CONTEXT_MANAGER_VALUE = 125;
/**
* CLASSROOM = 126;
*
*
* Logs for Context Manager (go/context-manager)
*
*/
public static final int CLASSROOM_VALUE = 126;
/**
* TAILORMADE = 127;
*
*
* App usage of the go/classroom app.
*
*/
public static final int TAILORMADE_VALUE = 127;
/**
* KEEP = 128;
*
*
* Logs for Tailormade app (go/a1-tm)
*
*/
public static final int KEEP_VALUE = 128;
/**
* GMM_BRIIM_COUNTERS = 129;
*
*
* Logs for Keep (go/keep).
*
*/
public static final int GMM_BRIIM_COUNTERS_VALUE = 129;
/**
* CHROMECAST_APP_LOG = 130;
*
*
* GMM BRIIM counters (go/gmm-briim)
*
*/
public static final int CHROMECAST_APP_LOG_VALUE = 130;
/**
* ADWORDS_MOBILE = 133;
*
*
* Logs for chromecast app (go/chromecastapplogs)
*
*/
public static final int ADWORDS_MOBILE_VALUE = 133;
/**
* LEANBACK_EVENT = 134;
*
*
* Logs for AdWords mobile app (go/awm)
*
*/
public static final int LEANBACK_EVENT_VALUE = 134;
/**
* ANDROID_GMAIL = 135;
*
*
* Logs for Android TV Leanback Launcher (go/android-tv)
*
*/
public static final int ANDROID_GMAIL_VALUE = 135;
/**
* SAMPLE_SHM = 136;
*
*
* Logs for Gmail (go/android-gmail-clearcut, shahrk@, android-mail-team@)
*
*/
public static final int SAMPLE_SHM_VALUE = 136;
/**
* GPLUS_ANDROID_PRIMES = 140;
*
*
* Logs for system health metric and memory metric sample app (go/primes)
*
*/
public static final int GPLUS_ANDROID_PRIMES_VALUE = 140;
/**
* GPLUS_ANDROID_SYSTEM_HEALTH = 140;
*
*
* Logs for system health metric for Gplus (go/primes)
*
*/
public static final int GPLUS_ANDROID_SYSTEM_HEALTH_VALUE = 140;
/**
* GMAIL_ANDROID_PRIMES = 150;
*/
public static final int GMAIL_ANDROID_PRIMES_VALUE = 150;
/**
* GMAIL_ANDROID_SYSTEM_HEALTH = 150;
*
*
* Logs for system health metric for GMail (go/primes)
*
*/
public static final int GMAIL_ANDROID_SYSTEM_HEALTH_VALUE = 150;
/**
* CALENDAR_ANDROID_PRIMES = 151;
*/
public static final int CALENDAR_ANDROID_PRIMES_VALUE = 151;
/**
* CALENDAR_ANDROID_SYSTEM_HEALTH = 151;
*
*
* Logs for system health metric for Calendar (go/primes)
*
*/
public static final int CALENDAR_ANDROID_SYSTEM_HEALTH_VALUE = 151;
/**
* DOCS_ANDROID_PRIMES = 152;
*/
public static final int DOCS_ANDROID_PRIMES_VALUE = 152;
/**
* DOCS_ANDROID_SYSTEM_HEALTH = 152;
*
*
* Logs for system health metric for Docs (go/primes)
*
*/
public static final int DOCS_ANDROID_SYSTEM_HEALTH_VALUE = 152;
/**
* YT_MAIN_APP_ANDROID_PRIMES = 154;
*/
public static final int YT_MAIN_APP_ANDROID_PRIMES_VALUE = 154;
/**
* YT_KIDS_ANDROID_PRIMES = 155;
*
*
* Logs for system health metric for the YouTube main app (go/primes)
*
*/
public static final int YT_KIDS_ANDROID_PRIMES_VALUE = 155;
/**
* YT_GAMING_ANDROID_PRIMES = 156;
*
*
* Logs for system health metric for the YouTube kids app (go/primes)
*
*/
public static final int YT_GAMING_ANDROID_PRIMES_VALUE = 156;
/**
* YT_MUSIC_ANDROID_PRIMES = 157;
*
*
* Logs for system health metric for the YouTube gaming app (go/primes)
*
*/
public static final int YT_MUSIC_ANDROID_PRIMES_VALUE = 157;
/**
* YT_LITE_ANDROID_PRIMES = 158;
*
*
* Logs for system health metric for the YouTube music app (go/primes)
*
*/
public static final int YT_LITE_ANDROID_PRIMES_VALUE = 158;
/**
* YT_CREATOR_ANDROID_PRIMES = 171;
*
*
* Logs for system health metric for the YouTube lite app (go/primes)
*
*/
public static final int YT_CREATOR_ANDROID_PRIMES_VALUE = 171;
/**
* JAM_ANDROID_PRIMES = 159;
*
*
* Logs for system health metric for the YouTube Creator Studio app (go/primes)
*
*/
public static final int JAM_ANDROID_PRIMES_VALUE = 159;
/**
* JAM_KIOSK_ANDROID_PRIMES = 160;
*
*
* Logs for system health metric for Jam (go/jam, go/primes)
*
*/
public static final int JAM_KIOSK_ANDROID_PRIMES_VALUE = 160;
/**
* PHOTOS_ANDROID_PRIMES = 165;
*
*
* Logs for system health metric for Jam Kiosk (go/jam, go/primes)
*
*/
public static final int PHOTOS_ANDROID_PRIMES_VALUE = 165;
/**
* DRIVE_ANDROID_PRIMES = 166;
*
*
* Logs for system health metric for Photos (go/primes)
*
*/
public static final int DRIVE_ANDROID_PRIMES_VALUE = 166;
/**
* SHEETS_ANDROID_PRIMES = 167;
*
*
* Logs for system health metric for Drive (go/primes)
*
*/
public static final int SHEETS_ANDROID_PRIMES_VALUE = 167;
/**
* SLIDES_ANDROID_PRIMES = 168;
*
*
* Logs for system health metric for Sheets (go/primes)
*
*/
public static final int SLIDES_ANDROID_PRIMES_VALUE = 168;
/**
* SNAPSEED_ANDROID_PRIMES = 178;
*
*
* Logs for system health metric for Slides (go/primes)
*
*/
public static final int SNAPSEED_ANDROID_PRIMES_VALUE = 178;
/**
* HANGOUTS_ANDROID_PRIMES = 179;
*
*
* Logs for system health metric for Snapseed (go/primes, go/snapseed).
*
*/
public static final int HANGOUTS_ANDROID_PRIMES_VALUE = 179;
/**
* INBOX_ANDROID_PRIMES = 180;
*
*
* Logs for system health metric for Shem (go/primes, go/rtc-shem).
*
*/
public static final int INBOX_ANDROID_PRIMES_VALUE = 180;
/**
* INBOX_IOS_PRIMES = 262;
*
*
* Logs for system health metric for Inbox (go/primes, go/bt-speed)
*
*/
public static final int INBOX_IOS_PRIMES_VALUE = 262;
/**
* SDP_IOS_PRIMES = 287;
*
*
* Logs for ios system health metric for Inbox (go/primes-ios, go/bt-speed)
*
*/
public static final int SDP_IOS_PRIMES_VALUE = 287;
/**
* GMSCORE_ANDROID_PRIMES = 193;
*
*
* Logs for ios system health metric for sdp (go/primes-ios, go/bt-speed)
*
*/
public static final int GMSCORE_ANDROID_PRIMES_VALUE = 193;
/**
* PLAY_MUSIC_ANDROID_WEAR_PRIMES = 200;
*
*
* Logs for system health metric for GmsCore (go/primes)
*
*/
public static final int PLAY_MUSIC_ANDROID_WEAR_PRIMES_VALUE = 200;
/**
* GEARHEAD_ANDROID_PRIMES = 201;
*
*
* Logs for system health metric for Play Music for Android Wear (music-android-wear@, go/wear-play-music, go/primes)
*
*/
public static final int GEARHEAD_ANDROID_PRIMES_VALUE = 201;
/**
* INSTORE_CONSUMER_PRIMES = 207;
*
*
* Logs for system health metric for the Android Auto (GearHead) app (go/primes, go/gearhead)
*
*/
public static final int INSTORE_CONSUMER_PRIMES_VALUE = 207;
/**
* SAMPLE_IOS_PRIMES = 202;
*
*
* Logs for system health metric for Hands Free (go/primes, go/instore-wallet)
*
*/
public static final int SAMPLE_IOS_PRIMES_VALUE = 202;
/**
* CPANEL_ANDROID_PRIMES = 213;
*
*
* Logs for ios system health metric and memory metric sample app (go/primes-ios)
*
*/
public static final int CPANEL_ANDROID_PRIMES_VALUE = 213;
/**
* HUDDLE_ANDROID_PRIMES = 214;
*
*
* Logs for android system health metric (go/cpanel-mobile)
*
*/
public static final int HUDDLE_ANDROID_PRIMES_VALUE = 214;
/**
* AWX_ANDROID_PRIMES = 222;
*
*
* Primes for Huddle (go/huddle)
*
*/
public static final int AWX_ANDROID_PRIMES_VALUE = 222;
/**
* GHS_ANDROID_PRIMES = 223;
*
*
* Primes for Google AdWords Express android app (go/primes, go/awx-android)
*
*/
public static final int GHS_ANDROID_PRIMES_VALUE = 223;
/**
* ADWORDS_MOBILE_ANDROID_PRIMES = 224;
*
*
* Primes for Google Home Services android app (go/primes, go/sab-awx-android)
*
*/
public static final int ADWORDS_MOBILE_ANDROID_PRIMES_VALUE = 224;
/**
* TAP_AND_PAY_ANDROID_PRIMES = 227;
*
*
* AdWords system health (go/awm, go/primes)
*
*/
public static final int TAP_AND_PAY_ANDROID_PRIMES_VALUE = 227;
/**
* WALLET_APP_ANDROID_PRIMES = 232;
*
*
* Primes for Tap and Pay android app, aka Android pay app (go/tp2)
*
*/
public static final int WALLET_APP_ANDROID_PRIMES_VALUE = 232;
/**
* WALLET_APP_IOS_PRIMES = 233;
*
*
* Primes for Wallet android app (go/gmoney)
*
*/
public static final int WALLET_APP_IOS_PRIMES_VALUE = 233;
/**
* ANALYTICS_ANDROID_PRIMES = 235;
*
*
* Primes for Wallet iOS app (go/gmoney)
*
*/
public static final int ANALYTICS_ANDROID_PRIMES_VALUE = 235;
/**
* SPACES_ANDROID_PRIMES = 236;
*
*
* Logs for system health metric for Analytics Android app (go/primes)
*
*/
public static final int SPACES_ANDROID_PRIMES_VALUE = 236;
/**
* SPACES_IOS_PRIMES = 276;
*
*
* Logs for the Spaces Android App (go/spaces)
*
*/
public static final int SPACES_IOS_PRIMES_VALUE = 276;
/**
* SOCIETY_ANDROID_PRIMES = 238;
*
*
* Logs for the Spaces iOS App (go/spaces)
*
*/
public static final int SOCIETY_ANDROID_PRIMES_VALUE = 238;
/**
* GMM_BRIIM_PRIMES = 239;
*
*
* Logs for system health metric for Society (go/primes, go/society)
*
*/
public static final int GMM_BRIIM_PRIMES_VALUE = 239;
/**
* CW_PRIMES = 242;
*
*
* Logs for system health metric for GMM BRIIM (go/gmm-briim)
*
*/
public static final int CW_PRIMES_VALUE = 242;
/**
* FAMILYLINK_ANDROID_PRIMES = 244;
*
*
* Clockwork go/primes logs
*
*/
public static final int FAMILYLINK_ANDROID_PRIMES_VALUE = 244;
/**
* FAMILYLINK_IOS_PRIMES = 291;
*
*
* Logs for system health metric for FamilyLink (go/primes)
*
*/
public static final int FAMILYLINK_IOS_PRIMES_VALUE = 291;
/**
* WH_PRIMES = 248;
*
*
* Logs for ios system health metric for FamilyLink (go/primes-ios, go/familylink-ios-app)
*
*/
public static final int WH_PRIMES_VALUE = 248;
/**
* NOVA_ANDROID_PRIMES = 249;
*
*
* Primes for Westinghouse (go/westinghouse, go/primes)
*
*/
public static final int NOVA_ANDROID_PRIMES_VALUE = 249;
/**
* PHOTOS_DRAPER_ANDROID_PRIMES = 253;
*
*
* Nova client app system health metrics (go/primes)
*
*/
public static final int PHOTOS_DRAPER_ANDROID_PRIMES_VALUE = 253;
/**
* GMM_PRIMES = 254;
*
*
* Logs for system health metric for Photos Draper (go/primes, go/photos-draper)
*
*/
public static final int GMM_PRIMES_VALUE = 254;
/**
* TRANSLATE_ANDROID_PRIMES = 255;
*
*
* Logs for system health metric for aGMM (go/gmm)
*
*/
public static final int TRANSLATE_ANDROID_PRIMES_VALUE = 255;
/**
* TRANSLATE_IOS_PRIMES = 256;
*
*
* Logs for system health metric for Translate on Android (go/primes)
*
*/
public static final int TRANSLATE_IOS_PRIMES_VALUE = 256;
/**
* FLYDROID_ANDROID_PRIMES = 259;
*
*
* Logs for system health metric for Translate on iOS (go/primes)
*
*/
public static final int FLYDROID_ANDROID_PRIMES_VALUE = 259;
/**
* CONSUMERIQ_PRIMES = 260;
*
*
* Log for system health metric for Freighter (go/freighter)
*
*/
public static final int CONSUMERIQ_PRIMES_VALUE = 260;
/**
* GMB_ANDROID_PRIMES = 263;
*
*
* Log for system health metric for Consumer IQ (go/primes)
*
*/
public static final int GMB_ANDROID_PRIMES_VALUE = 263;
/**
* CLOUDDPC_PRIMES = 304;
*
*
* Primes for Google My Business android app (go/gmb-android, go/primes).
*
*/
public static final int CLOUDDPC_PRIMES_VALUE = 304;
/**
* CLOUDDPC_ARC_PRIMES = 305;
*
*
* Primes for CloudDpc (go/clouddpc)
*
*/
public static final int CLOUDDPC_ARC_PRIMES_VALUE = 305;
/**
* ICORE = 137;
*
*
* Primes for CloudDpc for ARC (go/clouddpc)
*
*/
public static final int ICORE_VALUE = 137;
/**
* PANCETTA_MOBILE_HOST = 138;
*
*
* iOS logging (go/ios-logging)
*
*/
public static final int PANCETTA_MOBILE_HOST_VALUE = 138;
/**
* PANCETTA_MOBILE_HOST_COUNTERS = 139;
*
*
* Logs for Pancetta mobile hosts (go/pancetta)
*
*/
public static final int PANCETTA_MOBILE_HOST_COUNTERS_VALUE = 139;
/**
* CROSSDEVICENOTIFICATION = 141;
*
*
* Counters for Pancetta mobile hosts (go/pancetta)
*
*/
public static final int CROSSDEVICENOTIFICATION_VALUE = 141;
/**
* CROSSDEVICENOTIFICATION_DEV = 142;
*
*
* Logs for cross device notification service (go/hotpocket)
*
*/
public static final int CROSSDEVICENOTIFICATION_DEV_VALUE = 142;
/**
* MAPS_API_COUNTERS = 143;
*
*
* Dev logs for cross device notification service (go/hotpocket)
*
*/
public static final int MAPS_API_COUNTERS_VALUE = 143;
/**
* GPU = 144;
*
*
* Android and iOS Maps API (go/mapview & go/meridian)
*
*/
public static final int GPU_VALUE = 144;
/**
* ON_THE_GO = 145;
*
*
* Logs for Geo Photo Uploader (go/gpu-site)
*
*/
public static final int ON_THE_GO_VALUE = 145;
/**
* ON_THE_GO_COUNTERS = 146;
*
*
* Logs for OnTheGo (go/onthego)
*
*/
public static final int ON_THE_GO_COUNTERS_VALUE = 146;
/**
* GMS_CORE_PEOPLE_AUTOCOMPLETE = 147;
*
*
* Counters for OnTheGo (go/onthego)
*
*/
public static final int GMS_CORE_PEOPLE_AUTOCOMPLETE_VALUE = 147;
/**
* FLYDROID_COUNTERS = 148;
*
*
* Logs for Gcore People Autocomplete (go/yenta)
*
*/
public static final int FLYDROID_COUNTERS_VALUE = 148;
/**
* FIREBALL = 149;
*
*
* Logs for Flydroid (go/flydroid).
*
*/
public static final int FIREBALL_VALUE = 149;
/**
* FIREBALL_COUNTERS = 257;
*
*
* Logs for Fireball (go/fireballdev)
*
*/
public static final int FIREBALL_COUNTERS_VALUE = 257;
/**
* CRONET_FIREBALL = 303;
*
*
* Fireball counters (go/fireballdev)
*
*/
public static final int CRONET_FIREBALL_VALUE = 303;
/**
* FIREBALL_PRIMES = 266;
*
*
* Fireball cronet (go/fireballdev)
*
*/
public static final int FIREBALL_PRIMES_VALUE = 266;
/**
* FIREBALL_IOS_PRIMES = 313;
*
*
* Logs for system health for Fireball (go/fireball-systemhealth-plan)
*
*/
public static final int FIREBALL_IOS_PRIMES_VALUE = 313;
/**
* GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES = 314;
*
*
* Logs for system health for Fireball iOS (go/fireball-systemhealth-plan)
*
*/
public static final int GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES_VALUE = 314;
/**
* PYROCLASM = 153;
*
*
* Primes for Google Handwriting Input (go/hwrime)
*
*/
public static final int PYROCLASM_VALUE = 153;
/**
* ANDROID_GSA_COUNTERS = 161;
*
*
* Logs for Pyroclasm (go/pyroclasm)
*
*/
public static final int ANDROID_GSA_COUNTERS_VALUE = 161;
/**
* JAM_IMPRESSIONS = 162;
*
*
* Logs for AGSA counters.
*
*/
public static final int JAM_IMPRESSIONS_VALUE = 162;
/**
* JAM_KIOSK_IMPRESSIONS = 163;
*
*
* Impressions logs for Jam (go/jam).
*
*/
public static final int JAM_KIOSK_IMPRESSIONS_VALUE = 163;
/**
* PAYMENTS_OCR = 164;
*
*
* Impressions logs for Jam Kiosk (go/jam).
*
*/
public static final int PAYMENTS_OCR_VALUE = 164;
/**
* UNICORN_FAMILY_MANAGEMENT = 172;
*
*
* Logs for payments ocr (go/cc-ocr-metrics).
*
*/
public static final int UNICORN_FAMILY_MANAGEMENT_VALUE = 172;
/**
* AUDITOR = 173;
*
*
* Logs for Family management (and onboarding) go/familymanagement
*
*/
public static final int AUDITOR_VALUE = 173;
/**
* NQLOOKUP = 174;
*
*
* Logs for Accesssibility Auditor (go/a11y-checker)
*
*/
public static final int NQLOOKUP_VALUE = 174;
/**
* ANDROID_GSA_HIGH_PRIORITY_EVENTS = 175;
*
*
* Logs for NQLookup (go/nqlookup, contact [email protected])
*
*/
public static final int ANDROID_GSA_HIGH_PRIORITY_EVENTS_VALUE = 175;
/**
* ANDROID_DIALER = 176;
*
*
* Logs for high priority events in AGSA (go/click-tracking-agsa).
*
*/
public static final int ANDROID_DIALER_VALUE = 176;
/**
* CLEARCUT_DEMO = 177;
*
*
* Logs for Android Dialer (go/android-dialer)
*
*/
public static final int CLEARCUT_DEMO_VALUE = 177;
/**
* APPMANAGER = 183;
*
*
* Logs for Clearcut's demo application
*
*/
public static final int APPMANAGER_VALUE = 183;
/**
* SMARTLOCK_COUNTERS = 184;
*
*
* Logs for Firebase App Manager (go/appmanager)
*
*/
public static final int SMARTLOCK_COUNTERS_VALUE = 184;
/**
* EXPEDITIONS_GUIDE = 185;
*
*
* Counters for Smart Lock (go/smartlock)
*
*/
public static final int EXPEDITIONS_GUIDE_VALUE = 185;
/**
* FUSE = 186;
*
*
* Guide-aggregated logs for go/expeditions .
*
*/
public static final int FUSE_VALUE = 186;
/**
* PIXEL_PERFECT_CLIENT_STATE_LOGGER = 187;
*
*
* Logs for Fuse (go/fuse).
*
*/
public static final int PIXEL_PERFECT_CLIENT_STATE_LOGGER_VALUE = 187;
/**
* PLATFORM_STATS_COUNTERS = 188;
*
*
* Logs for PixelPerfect client app (go/pxp-client)
*
*/
public static final int PLATFORM_STATS_COUNTERS_VALUE = 188;
/**
* DRIVE_VIEWER = 189;
*
*
* Counters for platform stats.(go/androidplatformstats, clearcut-eng@)
*
*/
public static final int DRIVE_VIEWER_VALUE = 189;
/**
* PDF_VIEWER = 190;
*
*
* Google Drive Viewer Android and iOS apps (go/picoprojector)
*
*/
public static final int PDF_VIEWER_VALUE = 190;
/**
* BIGTOP = 191;
*
*
* Google PDF Viewer Android app (go/picoprojector)
*
*/
public static final int BIGTOP_VALUE = 191;
/**
* VOICE = 192;
*
*
* Logs for Bigtop (externally known as Inbox, go/bigtop).
*
*/
public static final int VOICE_VALUE = 192;
/**
* MYFIBER = 194;
*
*
* Logs for Google Voice (go/googlevoice).
*
*/
public static final int MYFIBER_VALUE = 194;
/**
* RECORDED_PAGES = 195;
*
*
* Logs for My Fiber mobile applications (go/myfibernative)
*
*/
public static final int RECORDED_PAGES_VALUE = 195;
/**
* MOB_DOG = 197;
*
*
* Logs for RecordedPages (go/recorded-pages)
*
*/
public static final int MOB_DOG_VALUE = 197;
/**
* WALLET_APP = 198;
*
*
* Logs for MobDog (go/mobdog)
*
*/
public static final int WALLET_APP_VALUE = 198;
/**
* GBOARD = 199;
*
*
* Logs for the Wallet app (go/gmoney)
*
*/
public static final int GBOARD_VALUE = 199;
/**
* CRONET_GMM = 203;
*
*
* Logs for GBoard (go/gboard)
*
*/
public static final int CRONET_GMM_VALUE = 203;
/**
* TRUSTED_FACE = 204;
*
*
* Cronet UMA logs from GMM (go/cronet-uma)
*
*/
public static final int TRUSTED_FACE_VALUE = 204;
/**
* MATCHSTICK = 205;
*
*
* Logs for Trusted Face (go/smartlock)
*
*/
public static final int MATCHSTICK_VALUE = 205;
/**
* APP_CATALOG = 206;
*
*
* Logs for Matchstick library (go/matchstick)
*
*/
public static final int APP_CATALOG_VALUE = 206;
/**
* BLUETOOTH = 208;
*
*
* Logs for AppCatalog aka "Get My Google Apps" (go/growth)
*
*/
public static final int BLUETOOTH_VALUE = 208;
/**
* WIFI = 209;
*
*
* Logs for Bluetooth (go/fluoride)
*
*/
public static final int WIFI_VALUE = 209;
/**
* TELECOM = 210;
*
*
* Logs for WiFi (go/connectivity-site)
*
*/
public static final int TELECOM_VALUE = 210;
/**
* TELEPHONY = 211;
*
*
* Logs for Telcom (go/connectivity-site)
*
*/
public static final int TELEPHONY_VALUE = 211;
/**
* IDENTITY_FRONTEND = 212;
*
*
* Logs for Telephony (go/connectivity-site)
*
*/
public static final int IDENTITY_FRONTEND_VALUE = 212;
/**
* SESAME = 216;
*
*
* Logs for the Identity frontend (go/ifus-readme)
*
*/
public static final int SESAME_VALUE = 216;
/**
* GOOGLE_KEYBOARD_CONTENT = 217;
*
*
* Logs for Sesame (go/sesame-logging)
*
*/
public static final int GOOGLE_KEYBOARD_CONTENT_VALUE = 217;
/**
* MADDEN = 218;
*
*
* Google Keyboard's Latin IME content logs (go/google-keyboard)
*
*/
public static final int MADDEN_VALUE = 218;
/**
* INK = 219;
*
*
* Logs for Madden (go/madden)
*
*/
public static final int INK_VALUE = 219;
/**
* ANDROID_CONTACTS = 220;
*
*
* Logs for Ink (go/ink)
*
*/
public static final int ANDROID_CONTACTS_VALUE = 220;
/**
* GOOGLE_KEYBOARD_COUNTERS = 221;
*
*
* Logs for Android Contacts (go/android-contacts)
*
*/
public static final int GOOGLE_KEYBOARD_COUNTERS_VALUE = 221;
/**
* CLEARCUT_PROBER = 225;
*
*
* Google Keyboard histogram counters (go/google-keyboard)
*
*/
public static final int CLEARCUT_PROBER_VALUE = 225;
/**
* PLAY_CONSOLE_APP = 226;
*
*
* Clearcut prober (go/clearcut-prober)
*
*/
public static final int PLAY_CONSOLE_APP_VALUE = 226;
/**
* PLAY_CONSOLE_APP_PRIMES = 264;
*
*
* Logs for the Google Play Developer Console app
*
*/
public static final int PLAY_CONSOLE_APP_PRIMES_VALUE = 264;
/**
* SPECTRUM = 230;
*
*
* Log for system health metrics for the Google Play Developer Console app
*
*/
public static final int SPECTRUM_VALUE = 230;
/**
* SPECTRUM_COUNTERS = 231;
*
*
* Spectrum (go/spectrum-site)
*
*/
public static final int SPECTRUM_COUNTERS_VALUE = 231;
/**
* SPECTRUM_ANDROID_PRIMES = 267;
*
*
* Spectrum (go/spectrum-site)
*
*/
public static final int SPECTRUM_ANDROID_PRIMES_VALUE = 267;
/**
* IOS_SPOTLIGHT_SEARCH_LIBRARY = 234;
*
*
* Spectrum (go/spectrum-site)
*
*/
public static final int IOS_SPOTLIGHT_SEARCH_LIBRARY_VALUE = 234;
/**
* BOQ_WEB = 241;
*
*
* Logs for the iOS Spotlight Search Library (go/tempeh-dd)
*
*/
public static final int BOQ_WEB_VALUE = 241;
/**
* ORCHESTRATION_CLIENT = 245;
*
*
* Logs for client-side analytics of Boq Web (go/boq-web)
*
*/
public static final int ORCHESTRATION_CLIENT_VALUE = 245;
/**
* ORCHESTRATION_CLIENT_DEV = 246;
*
*
* Orchestration client logs for prod environment (go/megalogs-design)
*
*/
public static final int ORCHESTRATION_CLIENT_DEV_VALUE = 246;
/**
* GOOGLE_NOW_LAUNCHER = 247;
*
*
* Orchestration client logs for dev environment (go/megalogs-design)
*
*/
public static final int GOOGLE_NOW_LAUNCHER_VALUE = 247;
/**
* SCOOBY_SPAM_REPORT_LOG = 250;
*
*
* Logs for Google Now Launcher (go/gnl-logging)
*
*/
public static final int SCOOBY_SPAM_REPORT_LOG_VALUE = 250;
/**
* IOS_GROWTH = 251;
*
*
* Logs of spam reports by scooby clients. (go/scooby)
*
*/
public static final int IOS_GROWTH_VALUE = 251;
/**
* APPS_NOTIFY = 252;
*
*
* go/growthkit-metrics
*
*/
public static final int APPS_NOTIFY_VALUE = 252;
/**
* SMARTKEY_APP = 269;
*
*
* Drive web push notifications (go/apps-notify)
*
*/
public static final int SMARTKEY_APP_VALUE = 269;
/**
* CLINICAL_STUDIES = 270;
*
*
* Smart Key App for project Bolt (go/smartlock)
*
*/
public static final int CLINICAL_STUDIES_VALUE = 270;
/**
* FITNESS_ANDROID_PRIMES = 271;
*
*
* Lifescience Clinical Studies logs (go/apollox)
*
*/
public static final int FITNESS_ANDROID_PRIMES_VALUE = 271;
/**
* IMPROV_APPS = 272;
*
*
* System health metric for Google Fit Android App (go/heart, go/primes)
*
*/
public static final int IMPROV_APPS_VALUE = 272;
/**
* FAMILYLINK = 273;
*
*
* Logs for Improv apps (go/improvlogging)
*
*/
public static final int FAMILYLINK_VALUE = 273;
/**
* FAMILYLINK_COUNTERS = 274;
*
*
* Family Link (go/familylink-dd)
*
*/
public static final int FAMILYLINK_COUNTERS_VALUE = 274;
/**
* SOCIETY = 275;
*
*
* Family Link counters (go/familylink-dd)
*
*/
public static final int SOCIETY_VALUE = 275;
/**
* DIALER_ANDROID_PRIMES = 277;
*
*
* NBU Society app (go/society)
*
*/
public static final int DIALER_ANDROID_PRIMES_VALUE = 277;
/**
* YOUTUBE_DIRECTOR_APP = 278;
*
*
* Android Dialer Primes (go/dialer)
*
*/
public static final int YOUTUBE_DIRECTOR_APP_VALUE = 278;
/**
* TACHYON_ANDROID_PRIMES = 279;
*
*
* YouTube Director App (go/youtube-director)
*
*/
public static final int TACHYON_ANDROID_PRIMES_VALUE = 279;
/**
* DRIVE_FS = 280;
*
*
* Android Tachyon Client
*
*/
public static final int DRIVE_FS_VALUE = 280;
/**
* YT_MAIN = 281;
*
*
* DriveFS (go/drive-fs)
*
*/
public static final int YT_MAIN_VALUE = 281;
/**
* WING_MARKETPLACE_ANDROID_PRIMES = 282;
*
*
* Logs for YT's main application
*
*/
public static final int WING_MARKETPLACE_ANDROID_PRIMES_VALUE = 282;
/**
* DYNAMITE = 283;
*
*
* Primes for Wing Marketplace Android app (go/wing-marketplace-android, go/primes)
*
*/
public static final int DYNAMITE_VALUE = 283;
/**
* CORP_ANDROID_FOOD = 284;
*
*
* Logs for Dynamite (go/dynamite)
*
*/
public static final int CORP_ANDROID_FOOD_VALUE = 284;
/**
* ANDROID_MESSAGING_PRIMES = 285;
*
*
* Logs for the internal Android Food app (g3doc/java/com/google/corp/bizapps/rews/food/android)
*
*/
public static final int ANDROID_MESSAGING_PRIMES_VALUE = 285;
/**
* GPLUS_IOS_PRIMES = 286;
*
*
* Primes for Android Messenger (go/bugledev, go/primes)
*
*/
public static final int GPLUS_IOS_PRIMES_VALUE = 286;
/**
* CHROMECAST_ANDROID_PRIMES = 288;
*
*
* Primes for Google+ iOS app (go/gplus-ios, go/primes)
*
*/
public static final int CHROMECAST_ANDROID_PRIMES_VALUE = 288;
/**
* APPSTREAMING = 289;
*
*
* Primes for Chromecast Android app (go/cast-apps)
*
*/
public static final int APPSTREAMING_VALUE = 289;
/**
* GMB_ANDROID = 290;
*
*
* Logs for AppStreaming Client Framework Side (go/piccard)
*
*/
public static final int GMB_ANDROID_VALUE = 290;
/**
* VOICE_IOS_PRIMES = 292;
*
*
* Logs for GMB Android app (go/gmb-android).
*
*/
public static final int VOICE_IOS_PRIMES_VALUE = 292;
/**
* VOICE_ANDROID_PRIMES = 293;
*
*
* Primes for iOS Google Voice (go/googlevoice).
*
*/
public static final int VOICE_ANDROID_PRIMES_VALUE = 293;
/**
* PAISA = 294;
*
*
* Primes for Android Google Voice (go/googlevoice).
*
*/
public static final int PAISA_VALUE = 294;
/**
* GMB_IOS = 295;
*
*
* NBU Paisa app (go/paisa)
*
*/
public static final int GMB_IOS_VALUE = 295;
/**
* SCOOBY_EVENTS = 296;
*
*
* Logs for GMB iOS App (go/gmb-ios).
*
*/
public static final int SCOOBY_EVENTS_VALUE = 296;
/**
* SNAPSEED_IOS_PRIMES = 297;
*
*
* Call logs events by scooby clients. (go/scooby)
*
*/
public static final int SNAPSEED_IOS_PRIMES_VALUE = 297;
/**
* YOUTUBE_DIRECTOR_IOS_PRIMES = 298;
*
*
* Primes for Snapseed IOS (go/Snapseed)
*
*/
public static final int YOUTUBE_DIRECTOR_IOS_PRIMES_VALUE = 298;
/**
* WALLPAPER_PICKER = 299;
*
*
* YouTube Director App (go/youtube-director)
*
*/
public static final int WALLPAPER_PICKER_VALUE = 299;
/**
* CHIME = 300;
*
*
* Android wallpaper picker (go/magic-wallpapers)
*
*/
public static final int CHIME_VALUE = 300;
/**
* BEACON_GCORE = 301;
*
*
* Chime (go/chime)
*
*/
public static final int BEACON_GCORE_VALUE = 301;
/**
* ANDROID_STUDIO = 302;
*
*
* Logs for debugging beacon module (go/beacons)
*
*/
public static final int ANDROID_STUDIO_VALUE = 302;
/**
* DOCS_OFFLINE = 306;
*
*
* Android Studio (go/android-studio)
*
*/
public static final int DOCS_OFFLINE_VALUE = 306;
/**
* FREIGHTER = 307;
*
*
* Logs for Docs Offline on Desktop (go/jetpax)
*
*/
public static final int FREIGHTER_VALUE = 307;
/**
* DOCS_IOS_PRIMES = 308;
*
*
* Logs for Freighter (http://go/freighter)
*
*/
public static final int DOCS_IOS_PRIMES_VALUE = 308;
/**
* SLIDES_IOS_PRIMES = 309;
*
*
* Logs for the Google Docs iOS application
*
*/
public static final int SLIDES_IOS_PRIMES_VALUE = 309;
/**
* SHEETS_IOS_PRIMES = 310;
*
*
* Logs for the Google Docs iOS application
*
*/
public static final int SHEETS_IOS_PRIMES_VALUE = 310;
/**
* IPCONNECTIVITY = 311;
*
*
* Logs for the Google Docs iOS application
*
*/
public static final int IPCONNECTIVITY_VALUE = 311;
/**
* CURATOR = 312;
*
*
* Logs for Networking (go/connectivity-site)
*
*/
public static final int CURATOR_VALUE = 312;
public final int getNumber() { return value; }
public static LogSource valueOf(int value) {
switch (value) {
case -1: return UNKNOWN;
case 0: return STORE;
case 65: return WEB_STORE;
case 132: return WORK_STORE;
case 261: return WORK_STORE_APP;
case 15: return EDU_STORE;
case 1: return MUSIC;
case 2: return BOOKS;
case 3: return VIDEO;
case 74: return MOVIES;
case 4: return MAGAZINES;
case 5: return GAMES;
case 6: return LB_A;
case 7: return ANDROID_IDE;
case 8: return LB_P;
case 9: return LB_S;
case 10: return GMS_CORE;
case 11: return APP_USAGE_1P;
case 12: return ICING;
case 13: return HERREVAD;
case 14: return GOOGLE_TV;
case 16: return GMS_CORE_PEOPLE;
case 17: return LE;
case 18: return GOOGLE_ANALYTICS;
case 19: return LB_D;
case 20: return ANDROID_GSA;
case 21: return LB_T;
case 22: return PERSONAL_LOGGER;
case 37: return PERSONAL_BROWSER_LOGGER;
case 23: return GMS_CORE_WALLET_MERCHANT_ERROR;
case 24: return LB_C;
case 52: return LB_IA;
case 237: return LB_CB;
case 268: return LB_DM;
case 25: return ANDROID_AUTH;
case 26: return ANDROID_CAMERA;
case 27: return CW;
case 243: return CW_COUNTERS;
case 28: return GEL;
case 29: return DNA_PROBER;
case 30: return UDR;
case 31: return GMS_CORE_WALLET;
case 32: return SOCIAL;
case 33: return INSTORE_WALLET;
case 34: return NOVA;
case 35: return LB_CA;
case 36: return LATIN_IME;
case 38: return NEWS_WEATHER;
case 39: return HANGOUT;
case 50: return HANGOUT_LOG_REQUEST;
case 40: return COPRESENCE;
case 41: return SOCIAL_AFFINITY;
case 42: return PHOTOS;
case 43: return GCM;
case 44: return GOKART;
case 45: return FINDR;
case 46: return ANDROID_MESSAGING;
case 47: return SOCIAL_WEB;
case 48: return BACKDROP;
case 49: return TELEMATICS;
case 51: return GVC_HARVESTER;
case 53: return CAR;
case 54: return PIXEL_PERFECT;
case 55: return DRIVE;
case 56: return DOCS;
case 57: return SHEETS;
case 58: return SLIDES;
case 59: return IME;
case 60: return WARP;
case 61: return NFC_PROGRAMMER;
case 62: return NETSTATS;
case 63: return NEWSSTAND;
case 64: return KIDS_COMMUNICATOR;
case 66: return WIFI_ASSISTANT;
case 67: return CAST_SENDER_SDK;
case 68: return CRONET_SOCIAL;
case 69: return PHENOTYPE;
case 70: return PHENOTYPE_COUNTERS;
case 71: return CHROME_INFRA;
case 72: return JUSTSPEAK;
case 73: return PERF_PROFILE;
case 75: return KATNISS;
case 76: return SOCIAL_APPINVITE;
case 77: return GMM_COUNTERS;
case 78: return BOND_ONEGOOGLE;
case 79: return MAPS_API;
case 196: return CRONET_ANDROID_YT;
case 80: return CRONET_ANDROID_GSA;
case 81: return GOOGLE_FIT_WEARABLE;
case 169: return FITNESS_ANDROID;
case 170: return FITNESS_GMS_CORE;
case 82: return GOOGLE_EXPRESS;
case 215: return GOOGLE_EXPRESS_DEV;
case 228: return GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES;
case 229: return GOOGLE_EXPRESS_ANDROID_PRIMES;
case 240: return GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES;
case 83: return SENSE;
case 84: return ANDROID_BACKUP;
case 85: return VR;
case 86: return IME_COUNTERS;
case 87: return SETUP_WIZARD;
case 88: return EMERGENCY_ASSIST;
case 89: return TRON;
case 90: return TRON_COUNTERS;
case 91: return BATTERY_STATS;
case 92: return DISK_STATS;
case 107: return GRAPHICS_STATS;
case 93: return PROC_STATS;
case 131: return DROP_BOX;
case 181: return FINGERPRINT_STATS;
case 182: return NOTIFICATION_STATS;
case 94: return TAP_AND_PAY_GCORE;
case 95: return A11YLOGGER;
case 96: return GCM_COUNTERS;
case 97: return PLACES_NO_GLS_CONSENT;
case 98: return TACHYON_LOG_REQUEST;
case 99: return TACHYON_COUNTERS;
case 100: return VISION;
case 101: return SOCIAL_USER_LOCATION;
case 102: return LAUNCHPAD_TOYS;
case 103: return METALOG_COUNTERS;
case 104: return MOBILESDK_CLIENT;
case 105: return ANDROID_VERIFY_APPS;
case 106: return ADSHIELD;
case 108: return SHERLOG;
case 109: return LE_ULR_COUNTERS;
case 110: return GMM_UE3;
case 111: return CALENDAR;
case 112: return ENDER;
case 113: return FAMILY_COMPASS;
case 114: return TRANSOM;
case 115: return TRANSOM_COUNTERS;
case 116: return LB_AS;
case 117: return LB_CFG;
case 118: return IOS_GSA;
case 119: return TAP_AND_PAY_APP;
case 265: return TAP_AND_PAY_APP_COUNTERS;
case 120: return FLYDROID;
case 121: return CPANEL_APP;
case 122: return ANDROID_SNET_GCORE;
case 123: return ANDROID_SNET_IDLE;
case 124: return ANDROID_SNET_JAR;
case 125: return CONTEXT_MANAGER;
case 126: return CLASSROOM;
case 127: return TAILORMADE;
case 128: return KEEP;
case 129: return GMM_BRIIM_COUNTERS;
case 130: return CHROMECAST_APP_LOG;
case 133: return ADWORDS_MOBILE;
case 134: return LEANBACK_EVENT;
case 135: return ANDROID_GMAIL;
case 136: return SAMPLE_SHM;
case 140: return GPLUS_ANDROID_PRIMES;
case 150: return GMAIL_ANDROID_PRIMES;
case 151: return CALENDAR_ANDROID_PRIMES;
case 152: return DOCS_ANDROID_PRIMES;
case 154: return YT_MAIN_APP_ANDROID_PRIMES;
case 155: return YT_KIDS_ANDROID_PRIMES;
case 156: return YT_GAMING_ANDROID_PRIMES;
case 157: return YT_MUSIC_ANDROID_PRIMES;
case 158: return YT_LITE_ANDROID_PRIMES;
case 171: return YT_CREATOR_ANDROID_PRIMES;
case 159: return JAM_ANDROID_PRIMES;
case 160: return JAM_KIOSK_ANDROID_PRIMES;
case 165: return PHOTOS_ANDROID_PRIMES;
case 166: return DRIVE_ANDROID_PRIMES;
case 167: return SHEETS_ANDROID_PRIMES;
case 168: return SLIDES_ANDROID_PRIMES;
case 178: return SNAPSEED_ANDROID_PRIMES;
case 179: return HANGOUTS_ANDROID_PRIMES;
case 180: return INBOX_ANDROID_PRIMES;
case 262: return INBOX_IOS_PRIMES;
case 287: return SDP_IOS_PRIMES;
case 193: return GMSCORE_ANDROID_PRIMES;
case 200: return PLAY_MUSIC_ANDROID_WEAR_PRIMES;
case 201: return GEARHEAD_ANDROID_PRIMES;
case 207: return INSTORE_CONSUMER_PRIMES;
case 202: return SAMPLE_IOS_PRIMES;
case 213: return CPANEL_ANDROID_PRIMES;
case 214: return HUDDLE_ANDROID_PRIMES;
case 222: return AWX_ANDROID_PRIMES;
case 223: return GHS_ANDROID_PRIMES;
case 224: return ADWORDS_MOBILE_ANDROID_PRIMES;
case 227: return TAP_AND_PAY_ANDROID_PRIMES;
case 232: return WALLET_APP_ANDROID_PRIMES;
case 233: return WALLET_APP_IOS_PRIMES;
case 235: return ANALYTICS_ANDROID_PRIMES;
case 236: return SPACES_ANDROID_PRIMES;
case 276: return SPACES_IOS_PRIMES;
case 238: return SOCIETY_ANDROID_PRIMES;
case 239: return GMM_BRIIM_PRIMES;
case 242: return CW_PRIMES;
case 244: return FAMILYLINK_ANDROID_PRIMES;
case 291: return FAMILYLINK_IOS_PRIMES;
case 248: return WH_PRIMES;
case 249: return NOVA_ANDROID_PRIMES;
case 253: return PHOTOS_DRAPER_ANDROID_PRIMES;
case 254: return GMM_PRIMES;
case 255: return TRANSLATE_ANDROID_PRIMES;
case 256: return TRANSLATE_IOS_PRIMES;
case 259: return FLYDROID_ANDROID_PRIMES;
case 260: return CONSUMERIQ_PRIMES;
case 263: return GMB_ANDROID_PRIMES;
case 304: return CLOUDDPC_PRIMES;
case 305: return CLOUDDPC_ARC_PRIMES;
case 137: return ICORE;
case 138: return PANCETTA_MOBILE_HOST;
case 139: return PANCETTA_MOBILE_HOST_COUNTERS;
case 141: return CROSSDEVICENOTIFICATION;
case 142: return CROSSDEVICENOTIFICATION_DEV;
case 143: return MAPS_API_COUNTERS;
case 144: return GPU;
case 145: return ON_THE_GO;
case 146: return ON_THE_GO_COUNTERS;
case 147: return GMS_CORE_PEOPLE_AUTOCOMPLETE;
case 148: return FLYDROID_COUNTERS;
case 149: return FIREBALL;
case 257: return FIREBALL_COUNTERS;
case 303: return CRONET_FIREBALL;
case 266: return FIREBALL_PRIMES;
case 313: return FIREBALL_IOS_PRIMES;
case 314: return GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES;
case 153: return PYROCLASM;
case 161: return ANDROID_GSA_COUNTERS;
case 162: return JAM_IMPRESSIONS;
case 163: return JAM_KIOSK_IMPRESSIONS;
case 164: return PAYMENTS_OCR;
case 172: return UNICORN_FAMILY_MANAGEMENT;
case 173: return AUDITOR;
case 174: return NQLOOKUP;
case 175: return ANDROID_GSA_HIGH_PRIORITY_EVENTS;
case 176: return ANDROID_DIALER;
case 177: return CLEARCUT_DEMO;
case 183: return APPMANAGER;
case 184: return SMARTLOCK_COUNTERS;
case 185: return EXPEDITIONS_GUIDE;
case 186: return FUSE;
case 187: return PIXEL_PERFECT_CLIENT_STATE_LOGGER;
case 188: return PLATFORM_STATS_COUNTERS;
case 189: return DRIVE_VIEWER;
case 190: return PDF_VIEWER;
case 191: return BIGTOP;
case 192: return VOICE;
case 194: return MYFIBER;
case 195: return RECORDED_PAGES;
case 197: return MOB_DOG;
case 198: return WALLET_APP;
case 199: return GBOARD;
case 203: return CRONET_GMM;
case 204: return TRUSTED_FACE;
case 205: return MATCHSTICK;
case 206: return APP_CATALOG;
case 208: return BLUETOOTH;
case 209: return WIFI;
case 210: return TELECOM;
case 211: return TELEPHONY;
case 212: return IDENTITY_FRONTEND;
case 216: return SESAME;
case 217: return GOOGLE_KEYBOARD_CONTENT;
case 218: return MADDEN;
case 219: return INK;
case 220: return ANDROID_CONTACTS;
case 221: return GOOGLE_KEYBOARD_COUNTERS;
case 225: return CLEARCUT_PROBER;
case 226: return PLAY_CONSOLE_APP;
case 264: return PLAY_CONSOLE_APP_PRIMES;
case 230: return SPECTRUM;
case 231: return SPECTRUM_COUNTERS;
case 267: return SPECTRUM_ANDROID_PRIMES;
case 234: return IOS_SPOTLIGHT_SEARCH_LIBRARY;
case 241: return BOQ_WEB;
case 245: return ORCHESTRATION_CLIENT;
case 246: return ORCHESTRATION_CLIENT_DEV;
case 247: return GOOGLE_NOW_LAUNCHER;
case 250: return SCOOBY_SPAM_REPORT_LOG;
case 251: return IOS_GROWTH;
case 252: return APPS_NOTIFY;
case 269: return SMARTKEY_APP;
case 270: return CLINICAL_STUDIES;
case 271: return FITNESS_ANDROID_PRIMES;
case 272: return IMPROV_APPS;
case 273: return FAMILYLINK;
case 274: return FAMILYLINK_COUNTERS;
case 275: return SOCIETY;
case 277: return DIALER_ANDROID_PRIMES;
case 278: return YOUTUBE_DIRECTOR_APP;
case 279: return TACHYON_ANDROID_PRIMES;
case 280: return DRIVE_FS;
case 281: return YT_MAIN;
case 282: return WING_MARKETPLACE_ANDROID_PRIMES;
case 283: return DYNAMITE;
case 284: return CORP_ANDROID_FOOD;
case 285: return ANDROID_MESSAGING_PRIMES;
case 286: return GPLUS_IOS_PRIMES;
case 288: return CHROMECAST_ANDROID_PRIMES;
case 289: return APPSTREAMING;
case 290: return GMB_ANDROID;
case 292: return VOICE_IOS_PRIMES;
case 293: return VOICE_ANDROID_PRIMES;
case 294: return PAISA;
case 295: return GMB_IOS;
case 296: return SCOOBY_EVENTS;
case 297: return SNAPSEED_IOS_PRIMES;
case 298: return YOUTUBE_DIRECTOR_IOS_PRIMES;
case 299: return WALLPAPER_PICKER;
case 300: return CHIME;
case 301: return BEACON_GCORE;
case 302: return ANDROID_STUDIO;
case 306: return DOCS_OFFLINE;
case 307: return FREIGHTER;
case 308: return DOCS_IOS_PRIMES;
case 309: return SLIDES_IOS_PRIMES;
case 310: return SHEETS_IOS_PRIMES;
case 311: return IPCONNECTIVITY;
case 312: return CURATOR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LogSource findValueByNumber(int number) {
return LogSource.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDescriptor().getEnumTypes().get(0);
}
private static final LogSource[] VALUES = {
UNKNOWN, STORE, WEB_STORE, WORK_STORE, WORK_STORE_APP, EDU_STORE, MUSIC, BOOKS, VIDEO, MOVIES, MAGAZINES, GAMES, LB_A, ANDROID_IDE, LB_P, LB_S, GMS_CORE, APP_USAGE_1P, ICING, HERREVAD, GOOGLE_TV, GMS_CORE_PEOPLE, LE, GOOGLE_ANALYTICS, LB_D, ANDROID_GSA, LB_T, PERSONAL_LOGGER, PERSONAL_BROWSER_LOGGER, GMS_CORE_WALLET_MERCHANT_ERROR, LB_C, LB_IA, LB_CB, LB_DM, ANDROID_AUTH, ANDROID_CAMERA, CW, CW_COUNTERS, GEL, DNA_PROBER, UDR, GMS_CORE_WALLET, SOCIAL, INSTORE_WALLET, NOVA, LB_CA, LATIN_IME, NEWS_WEATHER, HANGOUT, HANGOUT_LOG_REQUEST, COPRESENCE, SOCIAL_AFFINITY, PHOTOS, GCM, GOKART, FINDR, ANDROID_MESSAGING, SOCIAL_WEB, BACKDROP, TELEMATICS, GVC_HARVESTER, CAR, PIXEL_PERFECT, DRIVE, DOCS, SHEETS, SLIDES, IME, WARP, NFC_PROGRAMMER, NETSTATS, NEWSSTAND, KIDS_COMMUNICATOR, WIFI_ASSISTANT, CAST_SENDER_SDK, CRONET_SOCIAL, PHENOTYPE, PHENOTYPE_COUNTERS, CHROME_INFRA, JUSTSPEAK, PERF_PROFILE, KATNISS, SOCIAL_APPINVITE, GMM_COUNTERS, BOND_ONEGOOGLE, MAPS_API, CRONET_ANDROID_YT, CRONET_ANDROID_GSA, GOOGLE_FIT_WEARABLE, FITNESS_ANDROID, FITNESS_GMS_CORE, GOOGLE_EXPRESS, GOOGLE_EXPRESS_DEV, GOOGLE_EXPRESS_COURIER_ANDROID_PRIMES, GOOGLE_EXPRESS_ANDROID_PRIMES, GOOGLE_EXPRESS_STOREOMS_ANDROID_PRIMES, SENSE, ANDROID_BACKUP, VR, IME_COUNTERS, SETUP_WIZARD, EMERGENCY_ASSIST, TRON, TRON_COUNTERS, BATTERY_STATS, DISK_STATS, GRAPHICS_STATS, PROC_STATS, DROP_BOX, FINGERPRINT_STATS, NOTIFICATION_STATS, TAP_AND_PAY_GCORE, A11YLOGGER, GCM_COUNTERS, PLACES_NO_GLS_CONSENT, TACHYON_LOG_REQUEST, TACHYON_COUNTERS, VISION, SOCIAL_USER_LOCATION, LAUNCHPAD_TOYS, METALOG_COUNTERS, MOBILESDK_CLIENT, ANDROID_VERIFY_APPS, ADSHIELD, SHERLOG, LE_ULR_COUNTERS, GMM_UE3, CALENDAR, ENDER, FAMILY_COMPASS, TRANSOM, TRANSOM_COUNTERS, LB_AS, LB_CFG, IOS_GSA, TAP_AND_PAY_APP, TAP_AND_PAY_APP_COUNTERS, FLYDROID, CPANEL_APP, ANDROID_SNET_GCORE, ANDROID_SNET_IDLE, ANDROID_SNET_JAR, CONTEXT_MANAGER, CLASSROOM, TAILORMADE, KEEP, GMM_BRIIM_COUNTERS, CHROMECAST_APP_LOG, ADWORDS_MOBILE, LEANBACK_EVENT, ANDROID_GMAIL, SAMPLE_SHM, GPLUS_ANDROID_PRIMES, GPLUS_ANDROID_SYSTEM_HEALTH, GMAIL_ANDROID_PRIMES, GMAIL_ANDROID_SYSTEM_HEALTH, CALENDAR_ANDROID_PRIMES, CALENDAR_ANDROID_SYSTEM_HEALTH, DOCS_ANDROID_PRIMES, DOCS_ANDROID_SYSTEM_HEALTH, YT_MAIN_APP_ANDROID_PRIMES, YT_KIDS_ANDROID_PRIMES, YT_GAMING_ANDROID_PRIMES, YT_MUSIC_ANDROID_PRIMES, YT_LITE_ANDROID_PRIMES, YT_CREATOR_ANDROID_PRIMES, JAM_ANDROID_PRIMES, JAM_KIOSK_ANDROID_PRIMES, PHOTOS_ANDROID_PRIMES, DRIVE_ANDROID_PRIMES, SHEETS_ANDROID_PRIMES, SLIDES_ANDROID_PRIMES, SNAPSEED_ANDROID_PRIMES, HANGOUTS_ANDROID_PRIMES, INBOX_ANDROID_PRIMES, INBOX_IOS_PRIMES, SDP_IOS_PRIMES, GMSCORE_ANDROID_PRIMES, PLAY_MUSIC_ANDROID_WEAR_PRIMES, GEARHEAD_ANDROID_PRIMES, INSTORE_CONSUMER_PRIMES, SAMPLE_IOS_PRIMES, CPANEL_ANDROID_PRIMES, HUDDLE_ANDROID_PRIMES, AWX_ANDROID_PRIMES, GHS_ANDROID_PRIMES, ADWORDS_MOBILE_ANDROID_PRIMES, TAP_AND_PAY_ANDROID_PRIMES, WALLET_APP_ANDROID_PRIMES, WALLET_APP_IOS_PRIMES, ANALYTICS_ANDROID_PRIMES, SPACES_ANDROID_PRIMES, SPACES_IOS_PRIMES, SOCIETY_ANDROID_PRIMES, GMM_BRIIM_PRIMES, CW_PRIMES, FAMILYLINK_ANDROID_PRIMES, FAMILYLINK_IOS_PRIMES, WH_PRIMES, NOVA_ANDROID_PRIMES, PHOTOS_DRAPER_ANDROID_PRIMES, GMM_PRIMES, TRANSLATE_ANDROID_PRIMES, TRANSLATE_IOS_PRIMES, FLYDROID_ANDROID_PRIMES, CONSUMERIQ_PRIMES, GMB_ANDROID_PRIMES, CLOUDDPC_PRIMES, CLOUDDPC_ARC_PRIMES, ICORE, PANCETTA_MOBILE_HOST, PANCETTA_MOBILE_HOST_COUNTERS, CROSSDEVICENOTIFICATION, CROSSDEVICENOTIFICATION_DEV, MAPS_API_COUNTERS, GPU, ON_THE_GO, ON_THE_GO_COUNTERS, GMS_CORE_PEOPLE_AUTOCOMPLETE, FLYDROID_COUNTERS, FIREBALL, FIREBALL_COUNTERS, CRONET_FIREBALL, FIREBALL_PRIMES, FIREBALL_IOS_PRIMES, GOOGLE_HANDWRITING_INPUT_ANDROID_PRIMES, PYROCLASM, ANDROID_GSA_COUNTERS, JAM_IMPRESSIONS, JAM_KIOSK_IMPRESSIONS, PAYMENTS_OCR, UNICORN_FAMILY_MANAGEMENT, AUDITOR, NQLOOKUP, ANDROID_GSA_HIGH_PRIORITY_EVENTS, ANDROID_DIALER, CLEARCUT_DEMO, APPMANAGER, SMARTLOCK_COUNTERS, EXPEDITIONS_GUIDE, FUSE, PIXEL_PERFECT_CLIENT_STATE_LOGGER, PLATFORM_STATS_COUNTERS, DRIVE_VIEWER, PDF_VIEWER, BIGTOP, VOICE, MYFIBER, RECORDED_PAGES, MOB_DOG, WALLET_APP, GBOARD, CRONET_GMM, TRUSTED_FACE, MATCHSTICK, APP_CATALOG, BLUETOOTH, WIFI, TELECOM, TELEPHONY, IDENTITY_FRONTEND, SESAME, GOOGLE_KEYBOARD_CONTENT, MADDEN, INK, ANDROID_CONTACTS, GOOGLE_KEYBOARD_COUNTERS, CLEARCUT_PROBER, PLAY_CONSOLE_APP, PLAY_CONSOLE_APP_PRIMES, SPECTRUM, SPECTRUM_COUNTERS, SPECTRUM_ANDROID_PRIMES, IOS_SPOTLIGHT_SEARCH_LIBRARY, BOQ_WEB, ORCHESTRATION_CLIENT, ORCHESTRATION_CLIENT_DEV, GOOGLE_NOW_LAUNCHER, SCOOBY_SPAM_REPORT_LOG, IOS_GROWTH, APPS_NOTIFY, SMARTKEY_APP, CLINICAL_STUDIES, FITNESS_ANDROID_PRIMES, IMPROV_APPS, FAMILYLINK, FAMILYLINK_COUNTERS, SOCIETY, DIALER_ANDROID_PRIMES, YOUTUBE_DIRECTOR_APP, TACHYON_ANDROID_PRIMES, DRIVE_FS, YT_MAIN, WING_MARKETPLACE_ANDROID_PRIMES, DYNAMITE, CORP_ANDROID_FOOD, ANDROID_MESSAGING_PRIMES, GPLUS_IOS_PRIMES, CHROMECAST_ANDROID_PRIMES, APPSTREAMING, GMB_ANDROID, VOICE_IOS_PRIMES, VOICE_ANDROID_PRIMES, PAISA, GMB_IOS, SCOOBY_EVENTS, SNAPSEED_IOS_PRIMES, YOUTUBE_DIRECTOR_IOS_PRIMES, WALLPAPER_PICKER, CHIME, BEACON_GCORE, ANDROID_STUDIO, DOCS_OFFLINE, FREIGHTER, DOCS_IOS_PRIMES, SLIDES_IOS_PRIMES, SHEETS_IOS_PRIMES, IPCONNECTIVITY, CURATOR,
};
public static LogSource valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private LogSource(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.LogRequest.LogSource)
}
/**
* Protobuf enum {@code wireless_android_play_playlog.LogRequest.SchedulerType}
*
*
* Different types schedulers for log upload.
*
*/
public enum SchedulerType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_SCHEDULER = 0;
*/
UNKNOWN_SCHEDULER(0, 0),
/**
* ASAP = 1;
*/
ASAP(1, 1),
/**
* DEFAULT_PERIODIC = 2;
*
*
* As soon as possible used when log store is full.
*
*/
DEFAULT_PERIODIC(2, 2),
/**
* QOS_FAST_ONEOFF = 3;
*/
QOS_FAST_ONEOFF(3, 3),
/**
* QOS_DEFAULT_PERIODIC = 4;
*/
QOS_DEFAULT_PERIODIC(4, 4),
/**
* QOS_UNMETERED_PERIODIC = 5;
*/
QOS_UNMETERED_PERIODIC(5, 5),
;
/**
* UNKNOWN_SCHEDULER = 0;
*/
public static final int UNKNOWN_SCHEDULER_VALUE = 0;
/**
* ASAP = 1;
*/
public static final int ASAP_VALUE = 1;
/**
* DEFAULT_PERIODIC = 2;
*
*
* As soon as possible used when log store is full.
*
*/
public static final int DEFAULT_PERIODIC_VALUE = 2;
/**
* QOS_FAST_ONEOFF = 3;
*/
public static final int QOS_FAST_ONEOFF_VALUE = 3;
/**
* QOS_DEFAULT_PERIODIC = 4;
*/
public static final int QOS_DEFAULT_PERIODIC_VALUE = 4;
/**
* QOS_UNMETERED_PERIODIC = 5;
*/
public static final int QOS_UNMETERED_PERIODIC_VALUE = 5;
public final int getNumber() { return value; }
public static SchedulerType valueOf(int value) {
switch (value) {
case 0: return UNKNOWN_SCHEDULER;
case 1: return ASAP;
case 2: return DEFAULT_PERIODIC;
case 3: return QOS_FAST_ONEOFF;
case 4: return QOS_DEFAULT_PERIODIC;
case 5: return QOS_UNMETERED_PERIODIC;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SchedulerType findValueByNumber(int number) {
return SchedulerType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDescriptor().getEnumTypes().get(1);
}
private static final SchedulerType[] VALUES = values();
public static SchedulerType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private SchedulerType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.LogRequest.SchedulerType)
}
private int bitField0_;
// optional int64 request_time_ms = 4;
public static final int REQUEST_TIME_MS_FIELD_NUMBER = 4;
private long requestTimeMs_;
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public boolean hasRequestTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public long getRequestTimeMs() {
return requestTimeMs_;
}
// optional int64 request_uptime_ms = 8;
public static final int REQUEST_UPTIME_MS_FIELD_NUMBER = 8;
private long requestUptimeMs_;
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public boolean hasRequestUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public long getRequestUptimeMs() {
return requestUptimeMs_;
}
// optional .wireless_android_play_playlog.ClientInfo client_info = 1;
public static final int CLIENT_INFO_FIELD_NUMBER = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo clientInfo_;
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public boolean hasClientInfo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo getClientInfo() {
return clientInfo_;
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder getClientInfoOrBuilder() {
return clientInfo_;
}
// optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
public static final int LOG_SOURCE_FIELD_NUMBER = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource logSource_;
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public boolean hasLogSource() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource getLogSource() {
return logSource_;
}
// optional string log_source_name = 6;
public static final int LOG_SOURCE_NAME_FIELD_NUMBER = 6;
private java.lang.Object logSourceName_;
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public boolean hasLogSourceName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public java.lang.String getLogSourceName() {
java.lang.Object ref = logSourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
logSourceName_ = s;
}
return s;
}
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes() {
java.lang.Object ref = logSourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logSourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string zwieback_cookie = 7;
public static final int ZWIEBACK_COOKIE_FIELD_NUMBER = 7;
private java.lang.Object zwiebackCookie_;
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public boolean hasZwiebackCookie() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public java.lang.String getZwiebackCookie() {
java.lang.Object ref = zwiebackCookie_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
zwiebackCookie_ = s;
}
return s;
}
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public com.google.protobuf.ByteString
getZwiebackCookieBytes() {
java.lang.Object ref = zwiebackCookie_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
zwiebackCookie_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .wireless_android_play_playlog.LogEvent log_event = 3;
public static final int LOG_EVENT_FIELD_NUMBER = 3;
private java.util.List logEvent_;
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public java.util.List getLogEventList() {
return logEvent_;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder>
getLogEventOrBuilderList() {
return logEvent_;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public int getLogEventCount() {
return logEvent_.size();
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent getLogEvent(int index) {
return logEvent_.get(index);
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder getLogEventOrBuilder(
int index) {
return logEvent_.get(index);
}
// repeated bytes serialized_log_events = 5;
public static final int SERIALIZED_LOG_EVENTS_FIELD_NUMBER = 5;
private java.util.List serializedLogEvents_;
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public java.util.List
getSerializedLogEventsList() {
return serializedLogEvents_;
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public int getSerializedLogEventsCount() {
return serializedLogEvents_.size();
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public com.google.protobuf.ByteString getSerializedLogEvents(int index) {
return serializedLogEvents_.get(index);
}
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
public static final int QOS_TIER_FIELD_NUMBER = 9;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier qosTier_;
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier() {
return qosTier_;
}
// optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
public static final int SCHEDULER_FIELD_NUMBER = 10;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType scheduler_;
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public boolean hasScheduler() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType getScheduler() {
return scheduler_;
}
// optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
public static final int DEVICE_STATUS_FIELD_NUMBER = 11;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus deviceStatus_;
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public boolean hasDeviceStatus() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus getDeviceStatus() {
return deviceStatus_;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder getDeviceStatusOrBuilder() {
return deviceStatus_;
}
// optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
public static final int EXTERNAL_TIMESTAMP_FIELD_NUMBER = 12;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp externalTimestamp_;
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public boolean hasExternalTimestamp() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp getExternalTimestamp() {
return externalTimestamp_;
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder getExternalTimestampOrBuilder() {
return externalTimestamp_;
}
private void initFields() {
requestTimeMs_ = 0L;
requestUptimeMs_ = 0L;
clientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance();
logSource_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource.UNKNOWN;
logSourceName_ = "";
zwiebackCookie_ = "";
logEvent_ = java.util.Collections.emptyList();
serializedLogEvents_ = java.util.Collections.emptyList();
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
scheduler_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType.UNKNOWN_SCHEDULER;
deviceStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance();
externalTimestamp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getLogEventCount(); i++) {
if (!getLogEvent(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (!extensionsAreInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
com.google.protobuf.GeneratedMessage
.ExtendableMessage.ExtensionWriter extensionWriter =
newExtensionWriter();
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(1, clientInfo_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(2, logSource_.getNumber());
}
for (int i = 0; i < logEvent_.size(); i++) {
output.writeMessage(3, logEvent_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(4, requestTimeMs_);
}
for (int i = 0; i < serializedLogEvents_.size(); i++) {
output.writeBytes(5, serializedLogEvents_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(6, getLogSourceNameBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(7, getZwiebackCookieBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(8, requestUptimeMs_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeEnum(9, qosTier_.getNumber());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeEnum(10, scheduler_.getNumber());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(11, deviceStatus_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(12, externalTimestamp_);
}
extensionWriter.writeUntil(536870912, output);
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, clientInfo_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, logSource_.getNumber());
}
for (int i = 0; i < logEvent_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, logEvent_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, requestTimeMs_);
}
{
int dataSize = 0;
for (int i = 0; i < serializedLogEvents_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(serializedLogEvents_.get(i));
}
size += dataSize;
size += 1 * getSerializedLogEventsList().size();
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getLogSourceNameBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getZwiebackCookieBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, requestUptimeMs_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, qosTier_.getNumber());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, scheduler_.getNumber());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, deviceStatus_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, externalTimestamp_);
}
size += extensionsSerializedSize();
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogRequest}
*
*
* A LogRequest represents a batched collection of loggable events, each event
* to be processed and sent to zero or more destinations (e.g. Sawmill log types
* or Footprints) by the Clearcut server.
*
* Next tag: 13
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.ExtendableBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest, Builder> implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getClientInfoFieldBuilder();
getLogEventFieldBuilder();
getDeviceStatusFieldBuilder();
getExternalTimestampFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
requestTimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
requestUptimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
if (clientInfoBuilder_ == null) {
clientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance();
} else {
clientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
logSource_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource.UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000008);
logSourceName_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
zwiebackCookie_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
if (logEventBuilder_ == null) {
logEvent_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
logEventBuilder_.clear();
}
serializedLogEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
bitField0_ = (bitField0_ & ~0x00000100);
scheduler_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType.UNKNOWN_SCHEDULER;
bitField0_ = (bitField0_ & ~0x00000200);
if (deviceStatusBuilder_ == null) {
deviceStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance();
} else {
deviceStatusBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
if (externalTimestampBuilder_ == null) {
externalTimestamp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance();
} else {
externalTimestampBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogRequest_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.requestTimeMs_ = requestTimeMs_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.requestUptimeMs_ = requestUptimeMs_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (clientInfoBuilder_ == null) {
result.clientInfo_ = clientInfo_;
} else {
result.clientInfo_ = clientInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.logSource_ = logSource_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.logSourceName_ = logSourceName_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.zwiebackCookie_ = zwiebackCookie_;
if (logEventBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
logEvent_ = java.util.Collections.unmodifiableList(logEvent_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.logEvent_ = logEvent_;
} else {
result.logEvent_ = logEventBuilder_.build();
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
serializedLogEvents_ = java.util.Collections.unmodifiableList(serializedLogEvents_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.serializedLogEvents_ = serializedLogEvents_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
result.qosTier_ = qosTier_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
result.scheduler_ = scheduler_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
if (deviceStatusBuilder_ == null) {
result.deviceStatus_ = deviceStatus_;
} else {
result.deviceStatus_ = deviceStatusBuilder_.build();
}
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
if (externalTimestampBuilder_ == null) {
result.externalTimestamp_ = externalTimestamp_;
} else {
result.externalTimestamp_ = externalTimestampBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.getDefaultInstance()) return this;
if (other.hasRequestTimeMs()) {
setRequestTimeMs(other.getRequestTimeMs());
}
if (other.hasRequestUptimeMs()) {
setRequestUptimeMs(other.getRequestUptimeMs());
}
if (other.hasClientInfo()) {
mergeClientInfo(other.getClientInfo());
}
if (other.hasLogSource()) {
setLogSource(other.getLogSource());
}
if (other.hasLogSourceName()) {
bitField0_ |= 0x00000010;
logSourceName_ = other.logSourceName_;
onChanged();
}
if (other.hasZwiebackCookie()) {
bitField0_ |= 0x00000020;
zwiebackCookie_ = other.zwiebackCookie_;
onChanged();
}
if (logEventBuilder_ == null) {
if (!other.logEvent_.isEmpty()) {
if (logEvent_.isEmpty()) {
logEvent_ = other.logEvent_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureLogEventIsMutable();
logEvent_.addAll(other.logEvent_);
}
onChanged();
}
} else {
if (!other.logEvent_.isEmpty()) {
if (logEventBuilder_.isEmpty()) {
logEventBuilder_.dispose();
logEventBuilder_ = null;
logEvent_ = other.logEvent_;
bitField0_ = (bitField0_ & ~0x00000040);
logEventBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getLogEventFieldBuilder() : null;
} else {
logEventBuilder_.addAllMessages(other.logEvent_);
}
}
}
if (!other.serializedLogEvents_.isEmpty()) {
if (serializedLogEvents_.isEmpty()) {
serializedLogEvents_ = other.serializedLogEvents_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureSerializedLogEventsIsMutable();
serializedLogEvents_.addAll(other.serializedLogEvents_);
}
onChanged();
}
if (other.hasQosTier()) {
setQosTier(other.getQosTier());
}
if (other.hasScheduler()) {
setScheduler(other.getScheduler());
}
if (other.hasDeviceStatus()) {
mergeDeviceStatus(other.getDeviceStatus());
}
if (other.hasExternalTimestamp()) {
mergeExternalTimestamp(other.getExternalTimestamp());
}
this.mergeExtensionFields(other);
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getLogEventCount(); i++) {
if (!getLogEvent(i).isInitialized()) {
return false;
}
}
if (!extensionsAreInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 request_time_ms = 4;
private long requestTimeMs_ ;
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public boolean hasRequestTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public long getRequestTimeMs() {
return requestTimeMs_;
}
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public Builder setRequestTimeMs(long value) {
bitField0_ |= 0x00000001;
requestTimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 request_time_ms = 4;
*
*
* "Now", in milliseconds, according to the same clock as the one used to set
* the 'event_time_ms' values in the LogEvent protos above.
*
*/
public Builder clearRequestTimeMs() {
bitField0_ = (bitField0_ & ~0x00000001);
requestTimeMs_ = 0L;
onChanged();
return this;
}
// optional int64 request_uptime_ms = 8;
private long requestUptimeMs_ ;
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public boolean hasRequestUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public long getRequestUptimeMs() {
return requestUptimeMs_;
}
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public Builder setRequestUptimeMs(long value) {
bitField0_ |= 0x00000002;
requestUptimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 request_uptime_ms = 8;
*
*
* Current time since boot in milliseconds, including time spent in sleep,
* according to the same clock as the one used to set
* the 'event_uptime_ms' values in the LogEvent protos above.
*
*/
public Builder clearRequestUptimeMs() {
bitField0_ = (bitField0_ & ~0x00000002);
requestUptimeMs_ = 0L;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.ClientInfo client_info = 1;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo clientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder> clientInfoBuilder_;
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public boolean hasClientInfo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo getClientInfo() {
if (clientInfoBuilder_ == null) {
return clientInfo_;
} else {
return clientInfoBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public Builder setClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientInfo_ = value;
onChanged();
} else {
clientInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public Builder setClientInfo(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder builderForValue) {
if (clientInfoBuilder_ == null) {
clientInfo_ = builderForValue.build();
onChanged();
} else {
clientInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public Builder mergeClientInfo(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo value) {
if (clientInfoBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
clientInfo_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance()) {
clientInfo_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.newBuilder(clientInfo_).mergeFrom(value).buildPartial();
} else {
clientInfo_ = value;
}
onChanged();
} else {
clientInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public Builder clearClientInfo() {
if (clientInfoBuilder_ == null) {
clientInfo_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.getDefaultInstance();
onChanged();
} else {
clientInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder getClientInfoBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getClientInfoFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder getClientInfoOrBuilder() {
if (clientInfoBuilder_ != null) {
return clientInfoBuilder_.getMessageOrBuilder();
} else {
return clientInfo_;
}
}
/**
* optional .wireless_android_play_playlog.ClientInfo client_info = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder>
getClientInfoFieldBuilder() {
if (clientInfoBuilder_ == null) {
clientInfoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfo.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ClientInfoOrBuilder>(
clientInfo_,
getParentForChildren(),
isClean());
clientInfo_ = null;
}
return clientInfoBuilder_;
}
// optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource logSource_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource.UNKNOWN;
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public boolean hasLogSource() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource getLogSource() {
return logSource_;
}
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public Builder setLogSource(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
logSource_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.LogRequest.LogSource log_source = 2 [default = UNKNOWN];
*/
public Builder clearLogSource() {
bitField0_ = (bitField0_ & ~0x00000008);
logSource_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.LogSource.UNKNOWN;
onChanged();
return this;
}
// optional string log_source_name = 6;
private java.lang.Object logSourceName_ = "";
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public boolean hasLogSourceName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public java.lang.String getLogSourceName() {
java.lang.Object ref = logSourceName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
logSourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes() {
java.lang.Object ref = logSourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logSourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public Builder setLogSourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
logSourceName_ = value;
onChanged();
return this;
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public Builder clearLogSourceName() {
bitField0_ = (bitField0_ & ~0x00000010);
logSourceName_ = getDefaultInstance().getLogSourceName();
onChanged();
return this;
}
/**
* optional string log_source_name = 6;
*
*
* If log_source is not present and log_source_name is present, the value of
* log_source_name is parsed as a LogSource value and set to log_source.
*
*/
public Builder setLogSourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
logSourceName_ = value;
onChanged();
return this;
}
// optional string zwieback_cookie = 7;
private java.lang.Object zwiebackCookie_ = "";
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public boolean hasZwiebackCookie() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public java.lang.String getZwiebackCookie() {
java.lang.Object ref = zwiebackCookie_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
zwiebackCookie_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public com.google.protobuf.ByteString
getZwiebackCookieBytes() {
java.lang.Object ref = zwiebackCookie_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
zwiebackCookie_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public Builder setZwiebackCookie(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
zwiebackCookie_ = value;
onChanged();
return this;
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public Builder clearZwiebackCookie() {
bitField0_ = (bitField0_ & ~0x00000020);
zwiebackCookie_ = getDefaultInstance().getZwiebackCookie();
onChanged();
return this;
}
/**
* optional string zwieback_cookie = 7;
*
*
* cookie from pseudonymous id service at the time the event was posted
*
*/
public Builder setZwiebackCookieBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
zwiebackCookie_ = value;
onChanged();
return this;
}
// repeated .wireless_android_play_playlog.LogEvent log_event = 3;
private java.util.List logEvent_ =
java.util.Collections.emptyList();
private void ensureLogEventIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
logEvent_ = new java.util.ArrayList(logEvent_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder> logEventBuilder_;
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public java.util.List getLogEventList() {
if (logEventBuilder_ == null) {
return java.util.Collections.unmodifiableList(logEvent_);
} else {
return logEventBuilder_.getMessageList();
}
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public int getLogEventCount() {
if (logEventBuilder_ == null) {
return logEvent_.size();
} else {
return logEventBuilder_.getCount();
}
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent getLogEvent(int index) {
if (logEventBuilder_ == null) {
return logEvent_.get(index);
} else {
return logEventBuilder_.getMessage(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder setLogEvent(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent value) {
if (logEventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEventIsMutable();
logEvent_.set(index, value);
onChanged();
} else {
logEventBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder setLogEvent(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder builderForValue) {
if (logEventBuilder_ == null) {
ensureLogEventIsMutable();
logEvent_.set(index, builderForValue.build());
onChanged();
} else {
logEventBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder addLogEvent(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent value) {
if (logEventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEventIsMutable();
logEvent_.add(value);
onChanged();
} else {
logEventBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder addLogEvent(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent value) {
if (logEventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEventIsMutable();
logEvent_.add(index, value);
onChanged();
} else {
logEventBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder addLogEvent(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder builderForValue) {
if (logEventBuilder_ == null) {
ensureLogEventIsMutable();
logEvent_.add(builderForValue.build());
onChanged();
} else {
logEventBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder addLogEvent(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder builderForValue) {
if (logEventBuilder_ == null) {
ensureLogEventIsMutable();
logEvent_.add(index, builderForValue.build());
onChanged();
} else {
logEventBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder addAllLogEvent(
java.lang.Iterable extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent> values) {
if (logEventBuilder_ == null) {
ensureLogEventIsMutable();
super.addAll(values, logEvent_);
onChanged();
} else {
logEventBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder clearLogEvent() {
if (logEventBuilder_ == null) {
logEvent_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
logEventBuilder_.clear();
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public Builder removeLogEvent(int index) {
if (logEventBuilder_ == null) {
ensureLogEventIsMutable();
logEvent_.remove(index);
onChanged();
} else {
logEventBuilder_.remove(index);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder getLogEventBuilder(
int index) {
return getLogEventFieldBuilder().getBuilder(index);
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder getLogEventOrBuilder(
int index) {
if (logEventBuilder_ == null) {
return logEvent_.get(index); } else {
return logEventBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder>
getLogEventOrBuilderList() {
if (logEventBuilder_ != null) {
return logEventBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logEvent_);
}
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder addLogEventBuilder() {
return getLogEventFieldBuilder().addBuilder(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder addLogEventBuilder(
int index) {
return getLogEventFieldBuilder().addBuilder(
index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.LogEvent log_event = 3;
*/
public java.util.List
getLogEventBuilderList() {
return getLogEventFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder>
getLogEventFieldBuilder() {
if (logEventBuilder_ == null) {
logEventBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEvent.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogEventOrBuilder>(
logEvent_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
logEvent_ = null;
}
return logEventBuilder_;
}
// repeated bytes serialized_log_events = 5;
private java.util.List serializedLogEvents_ = java.util.Collections.emptyList();
private void ensureSerializedLogEventsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
serializedLogEvents_ = new java.util.ArrayList(serializedLogEvents_);
bitField0_ |= 0x00000080;
}
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public java.util.List
getSerializedLogEventsList() {
return java.util.Collections.unmodifiableList(serializedLogEvents_);
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public int getSerializedLogEventsCount() {
return serializedLogEvents_.size();
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public com.google.protobuf.ByteString getSerializedLogEvents(int index) {
return serializedLogEvents_.get(index);
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public Builder setSerializedLogEvents(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSerializedLogEventsIsMutable();
serializedLogEvents_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public Builder addSerializedLogEvents(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSerializedLogEventsIsMutable();
serializedLogEvents_.add(value);
onChanged();
return this;
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public Builder addAllSerializedLogEvents(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSerializedLogEventsIsMutable();
super.addAll(values, serializedLogEvents_);
onChanged();
return this;
}
/**
* repeated bytes serialized_log_events = 5;
*
*
* Can be used instead of 'log_event' in order to save battery in cases where
* LogEvent protos are already serialized, and deserializing is undesirable.
* If used, this is a collection of byte streams each produced using a
* CodedOutputStream.
*
*/
public Builder clearSerializedLogEvents() {
serializedLogEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier() {
return qosTier_;
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public Builder setQosTier(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
qosTier_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 9 [default = DEFAULT];
*
*
* Quality of Service tier for log upload.
*
*/
public Builder clearQosTier() {
bitField0_ = (bitField0_ & ~0x00000100);
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType scheduler_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType.UNKNOWN_SCHEDULER;
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public boolean hasScheduler() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType getScheduler() {
return scheduler_;
}
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public Builder setScheduler(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
scheduler_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.LogRequest.SchedulerType scheduler = 10;
*
*
* The type of log upload scheduler.
*
*/
public Builder clearScheduler() {
bitField0_ = (bitField0_ & ~0x00000200);
scheduler_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogRequest.SchedulerType.UNKNOWN_SCHEDULER;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus deviceStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder> deviceStatusBuilder_;
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public boolean hasDeviceStatus() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus getDeviceStatus() {
if (deviceStatusBuilder_ == null) {
return deviceStatus_;
} else {
return deviceStatusBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public Builder setDeviceStatus(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus value) {
if (deviceStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deviceStatus_ = value;
onChanged();
} else {
deviceStatusBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public Builder setDeviceStatus(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder builderForValue) {
if (deviceStatusBuilder_ == null) {
deviceStatus_ = builderForValue.build();
onChanged();
} else {
deviceStatusBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public Builder mergeDeviceStatus(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus value) {
if (deviceStatusBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
deviceStatus_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance()) {
deviceStatus_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.newBuilder(deviceStatus_).mergeFrom(value).buildPartial();
} else {
deviceStatus_ = value;
}
onChanged();
} else {
deviceStatusBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public Builder clearDeviceStatus() {
if (deviceStatusBuilder_ == null) {
deviceStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance();
onChanged();
} else {
deviceStatusBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder getDeviceStatusBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getDeviceStatusFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder getDeviceStatusOrBuilder() {
if (deviceStatusBuilder_ != null) {
return deviceStatusBuilder_.getMessageOrBuilder();
} else {
return deviceStatus_;
}
}
/**
* optional .wireless_android_play_playlog.DeviceStatus device_status = 11;
*
*
* Device status.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder>
getDeviceStatusFieldBuilder() {
if (deviceStatusBuilder_ == null) {
deviceStatusBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder>(
deviceStatus_,
getParentForChildren(),
isClean());
deviceStatus_ = null;
}
return deviceStatusBuilder_;
}
// optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp externalTimestamp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder> externalTimestampBuilder_;
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public boolean hasExternalTimestamp() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp getExternalTimestamp() {
if (externalTimestampBuilder_ == null) {
return externalTimestamp_;
} else {
return externalTimestampBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public Builder setExternalTimestamp(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp value) {
if (externalTimestampBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
externalTimestamp_ = value;
onChanged();
} else {
externalTimestampBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public Builder setExternalTimestamp(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder builderForValue) {
if (externalTimestampBuilder_ == null) {
externalTimestamp_ = builderForValue.build();
onChanged();
} else {
externalTimestampBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public Builder mergeExternalTimestamp(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp value) {
if (externalTimestampBuilder_ == null) {
if (((bitField0_ & 0x00000800) == 0x00000800) &&
externalTimestamp_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance()) {
externalTimestamp_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.newBuilder(externalTimestamp_).mergeFrom(value).buildPartial();
} else {
externalTimestamp_ = value;
}
onChanged();
} else {
externalTimestampBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public Builder clearExternalTimestamp() {
if (externalTimestampBuilder_ == null) {
externalTimestamp_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance();
onChanged();
} else {
externalTimestampBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder getExternalTimestampBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getExternalTimestampFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder getExternalTimestampOrBuilder() {
if (externalTimestampBuilder_ != null) {
return externalTimestampBuilder_.getMessageOrBuilder();
} else {
return externalTimestamp_;
}
}
/**
* optional .wireless_android_play_playlog.ExternalTimestamp external_timestamp = 12;
*
*
* A timestamp taken from a source external to the device. For example, a GPS
* timestamp. This can be more accurate than the device wall clock, but is not
* guaranteed to be. That will depend on the source, and on the device
* hardware and software that obtains the timestamp.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder>
getExternalTimestampFieldBuilder() {
if (externalTimestampBuilder_ == null) {
externalTimestampBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder>(
externalTimestamp_,
getParentForChildren(),
isClean());
externalTimestamp_ = null;
}
return externalTimestampBuilder_;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.LogRequest)
}
static {
defaultInstance = new LogRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.LogRequest)
}
public interface DeviceStatusOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional bool is_unmetered = 1;
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
boolean hasIsUnmetered();
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
boolean getIsUnmetered();
// optional bool is_charging = 2;
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
boolean hasIsCharging();
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
boolean getIsCharging();
// optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
boolean hasAutoTimeStatus();
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime getAutoTimeStatus();
// optional bool is_googler_device = 4;
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
boolean hasIsGooglerDevice();
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
boolean getIsGooglerDevice();
}
/**
* Protobuf type {@code wireless_android_play_playlog.DeviceStatus}
*
*
* DeviceStatus captures device status including network connection status,
* battery status, and etc.
* Next tag: 5
*
*/
public static final class DeviceStatus extends
com.google.protobuf.GeneratedMessage
implements DeviceStatusOrBuilder {
// Use DeviceStatus.newBuilder() to construct.
private DeviceStatus(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DeviceStatus(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DeviceStatus defaultInstance;
public static DeviceStatus getDefaultInstance() {
return defaultInstance;
}
public DeviceStatus getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeviceStatus(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
isUnmetered_ = input.readBool();
break;
}
case 16: {
bitField0_ |= 0x00000002;
isCharging_ = input.readBool();
break;
}
case 24: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
autoTimeStatus_ = value;
}
break;
}
case 32: {
bitField0_ |= 0x00000008;
isGooglerDevice_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DeviceStatus_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DeviceStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DeviceStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeviceStatus(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.DeviceStatus.AutomaticTime}
*
*
* Status of automatic date and time setting.
*
*/
public enum AutomaticTime
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*
*
* Device is using an older version of GmsCore (before U), or there was a
* problem getting the setting.
*
*/
UNKNOWN(0, 0),
/**
* AUTO_TIME_OFF = 1;
*
*
* Automatic date and time was not enabled.
*
*/
AUTO_TIME_OFF(1, 1),
/**
* AUTO_TIME_ON = 2;
*
*
* Automatic date and time was enabled.
*
*/
AUTO_TIME_ON(2, 2),
;
/**
* UNKNOWN = 0;
*
*
* Device is using an older version of GmsCore (before U), or there was a
* problem getting the setting.
*
*/
public static final int UNKNOWN_VALUE = 0;
/**
* AUTO_TIME_OFF = 1;
*
*
* Automatic date and time was not enabled.
*
*/
public static final int AUTO_TIME_OFF_VALUE = 1;
/**
* AUTO_TIME_ON = 2;
*
*
* Automatic date and time was enabled.
*
*/
public static final int AUTO_TIME_ON_VALUE = 2;
public final int getNumber() { return value; }
public static AutomaticTime valueOf(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return AUTO_TIME_OFF;
case 2: return AUTO_TIME_ON;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AutomaticTime findValueByNumber(int number) {
return AutomaticTime.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDescriptor().getEnumTypes().get(0);
}
private static final AutomaticTime[] VALUES = values();
public static AutomaticTime valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private AutomaticTime(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.DeviceStatus.AutomaticTime)
}
private int bitField0_;
// optional bool is_unmetered = 1;
public static final int IS_UNMETERED_FIELD_NUMBER = 1;
private boolean isUnmetered_;
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public boolean hasIsUnmetered() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public boolean getIsUnmetered() {
return isUnmetered_;
}
// optional bool is_charging = 2;
public static final int IS_CHARGING_FIELD_NUMBER = 2;
private boolean isCharging_;
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public boolean hasIsCharging() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public boolean getIsCharging() {
return isCharging_;
}
// optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
public static final int AUTO_TIME_STATUS_FIELD_NUMBER = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime autoTimeStatus_;
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public boolean hasAutoTimeStatus() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime getAutoTimeStatus() {
return autoTimeStatus_;
}
// optional bool is_googler_device = 4;
public static final int IS_GOOGLER_DEVICE_FIELD_NUMBER = 4;
private boolean isGooglerDevice_;
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public boolean hasIsGooglerDevice() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public boolean getIsGooglerDevice() {
return isGooglerDevice_;
}
private void initFields() {
isUnmetered_ = false;
isCharging_ = false;
autoTimeStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime.UNKNOWN;
isGooglerDevice_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(1, isUnmetered_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, isCharging_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, autoTimeStatus_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, isGooglerDevice_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, isUnmetered_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, isCharging_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, autoTimeStatus_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, isGooglerDevice_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.DeviceStatus}
*
*
* DeviceStatus captures device status including network connection status,
* battery status, and etc.
* Next tag: 5
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DeviceStatus_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DeviceStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
isUnmetered_ = false;
bitField0_ = (bitField0_ & ~0x00000001);
isCharging_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
autoTimeStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime.UNKNOWN;
bitField0_ = (bitField0_ & ~0x00000004);
isGooglerDevice_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_DeviceStatus_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.isUnmetered_ = isUnmetered_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.isCharging_ = isCharging_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.autoTimeStatus_ = autoTimeStatus_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.isGooglerDevice_ = isGooglerDevice_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.getDefaultInstance()) return this;
if (other.hasIsUnmetered()) {
setIsUnmetered(other.getIsUnmetered());
}
if (other.hasIsCharging()) {
setIsCharging(other.getIsCharging());
}
if (other.hasAutoTimeStatus()) {
setAutoTimeStatus(other.getAutoTimeStatus());
}
if (other.hasIsGooglerDevice()) {
setIsGooglerDevice(other.getIsGooglerDevice());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional bool is_unmetered = 1;
private boolean isUnmetered_ ;
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public boolean hasIsUnmetered() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public boolean getIsUnmetered() {
return isUnmetered_;
}
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public Builder setIsUnmetered(boolean value) {
bitField0_ |= 0x00000001;
isUnmetered_ = value;
onChanged();
return this;
}
/**
* optional bool is_unmetered = 1;
*
*
* Whether device network connection is unmetered.
*
*/
public Builder clearIsUnmetered() {
bitField0_ = (bitField0_ & ~0x00000001);
isUnmetered_ = false;
onChanged();
return this;
}
// optional bool is_charging = 2;
private boolean isCharging_ ;
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public boolean hasIsCharging() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public boolean getIsCharging() {
return isCharging_;
}
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public Builder setIsCharging(boolean value) {
bitField0_ |= 0x00000002;
isCharging_ = value;
onChanged();
return this;
}
/**
* optional bool is_charging = 2;
*
*
* Whether device is under charge. See link
* http://developer.android.com/training/monitoring-device-state/battery-monitoring.html
* This boolean returns true when status equals to
* BatteryManager.BATTERY_STATUS_CHARGING || BatteryManager.BATTERY_STATUS_FULL
*
*/
public Builder clearIsCharging() {
bitField0_ = (bitField0_ & ~0x00000002);
isCharging_ = false;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime autoTimeStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime.UNKNOWN;
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public boolean hasAutoTimeStatus() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime getAutoTimeStatus() {
return autoTimeStatus_;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public Builder setAutoTimeStatus(com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
autoTimeStatus_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.DeviceStatus.AutomaticTime auto_time_status = 3;
*
*
* Whether automatic date and time is enabled on the device. See link
* http://developer.android.com/reference/android/provider/Settings.Global.html#AUTO_TIME
* Note, this does not guarantee correct device clock if NTP clock sync has
* not occurred.
*
*/
public Builder clearAutoTimeStatus() {
bitField0_ = (bitField0_ & ~0x00000004);
autoTimeStatus_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.DeviceStatus.AutomaticTime.UNKNOWN;
onChanged();
return this;
}
// optional bool is_googler_device = 4;
private boolean isGooglerDevice_ ;
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public boolean hasIsGooglerDevice() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public boolean getIsGooglerDevice() {
return isGooglerDevice_;
}
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public Builder setIsGooglerDevice(boolean value) {
bitField0_ |= 0x00000008;
isGooglerDevice_ = value;
onChanged();
return this;
}
/**
* optional bool is_googler_device = 4;
*
*
* Is true if the device contains an account that has the @google.com domain.
*
*/
public Builder clearIsGooglerDevice() {
bitField0_ = (bitField0_ & ~0x00000008);
isGooglerDevice_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.DeviceStatus)
}
static {
defaultInstance = new DeviceStatus(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.DeviceStatus)
}
public interface ExternalTimestampOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int64 time_ms = 1;
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
boolean hasTimeMs();
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
long getTimeMs();
// optional int64 uptime_ms = 2;
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
boolean hasUptimeMs();
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
long getUptimeMs();
// optional string source = 3;
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
boolean hasSource();
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
java.lang.String getSource();
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
com.google.protobuf.ByteString
getSourceBytes();
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExternalTimestamp}
*
*
* ExternalTimestamp captures a timestamp obtained by an external source. This
* can be GPS or clock sync over the network. The timestamp is typically old, so
* the device uptime when the timestamp was obtained is also included. The
* source of this timestamp must guarantee that the timestamp is not older than
* the most recent device boot.
* Next tag: 4
*
*/
public static final class ExternalTimestamp extends
com.google.protobuf.GeneratedMessage
implements ExternalTimestampOrBuilder {
// Use ExternalTimestamp.newBuilder() to construct.
private ExternalTimestamp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ExternalTimestamp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ExternalTimestamp defaultInstance;
public static ExternalTimestamp getDefaultInstance() {
return defaultInstance;
}
public ExternalTimestamp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExternalTimestamp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
timeMs_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
uptimeMs_ = input.readInt64();
break;
}
case 26: {
bitField0_ |= 0x00000004;
source_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExternalTimestamp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ExternalTimestamp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExternalTimestamp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int64 time_ms = 1;
public static final int TIME_MS_FIELD_NUMBER = 1;
private long timeMs_;
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public boolean hasTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public long getTimeMs() {
return timeMs_;
}
// optional int64 uptime_ms = 2;
public static final int UPTIME_MS_FIELD_NUMBER = 2;
private long uptimeMs_;
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public boolean hasUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public long getUptimeMs() {
return uptimeMs_;
}
// optional string source = 3;
public static final int SOURCE_FIELD_NUMBER = 3;
private java.lang.Object source_;
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public boolean hasSource() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
source_ = s;
}
return s;
}
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
timeMs_ = 0L;
uptimeMs_ = 0L;
source_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, timeMs_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, uptimeMs_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getSourceBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, timeMs_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, uptimeMs_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getSourceBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.ExternalTimestamp}
*
*
* ExternalTimestamp captures a timestamp obtained by an external source. This
* can be GPS or clock sync over the network. The timestamp is typically old, so
* the device uptime when the timestamp was obtained is also included. The
* source of this timestamp must guarantee that the timestamp is not older than
* the most recent device boot.
* Next tag: 4
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestampOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExternalTimestamp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
timeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
uptimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
source_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.timeMs_ = timeMs_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.uptimeMs_ = uptimeMs_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.source_ = source_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp.getDefaultInstance()) return this;
if (other.hasTimeMs()) {
setTimeMs(other.getTimeMs());
}
if (other.hasUptimeMs()) {
setUptimeMs(other.getUptimeMs());
}
if (other.hasSource()) {
bitField0_ |= 0x00000004;
source_ = other.source_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExternalTimestamp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 time_ms = 1;
private long timeMs_ ;
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public boolean hasTimeMs() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public long getTimeMs() {
return timeMs_;
}
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public Builder setTimeMs(long value) {
bitField0_ |= 0x00000001;
timeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 time_ms = 1;
*
*
* A timestamp given by an external source.
*
*/
public Builder clearTimeMs() {
bitField0_ = (bitField0_ & ~0x00000001);
timeMs_ = 0L;
onChanged();
return this;
}
// optional int64 uptime_ms = 2;
private long uptimeMs_ ;
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public boolean hasUptimeMs() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public long getUptimeMs() {
return uptimeMs_;
}
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public Builder setUptimeMs(long value) {
bitField0_ |= 0x00000002;
uptimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 uptime_ms = 2;
*
*
* The device uptime when the timestamp was recorded.
*
*/
public Builder clearUptimeMs() {
bitField0_ = (bitField0_ & ~0x00000002);
uptimeMs_ = 0L;
onChanged();
return this;
}
// optional string source = 3;
private java.lang.Object source_ = "";
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public boolean hasSource() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
source_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public Builder setSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
source_ = value;
onChanged();
return this;
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public Builder clearSource() {
bitField0_ = (bitField0_ & ~0x00000004);
source_ = getDefaultInstance().getSource();
onChanged();
return this;
}
/**
* optional string source = 3;
*
*
* The name of the source that provided the timestamp.
*
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
source_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.ExternalTimestamp)
}
static {
defaultInstance = new ExternalTimestamp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.ExternalTimestamp)
}
public interface QosTierConfigurationOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string log_source_name = 1;
/**
* optional string log_source_name = 1;
*/
boolean hasLogSourceName();
/**
* optional string log_source_name = 1;
*/
java.lang.String getLogSourceName();
/**
* optional string log_source_name = 1;
*/
com.google.protobuf.ByteString
getLogSourceNameBytes();
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
boolean hasQosTier();
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier();
}
/**
* Protobuf type {@code wireless_android_play_playlog.QosTierConfiguration}
*
*
* QosTierConfiguration configures what Quality of Service tier should be
* applied to a given log source.
*
* Next tag: 3
*
*/
public static final class QosTierConfiguration extends
com.google.protobuf.GeneratedMessage
implements QosTierConfigurationOrBuilder {
// Use QosTierConfiguration.newBuilder() to construct.
private QosTierConfiguration(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private QosTierConfiguration(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final QosTierConfiguration defaultInstance;
public static QosTierConfiguration getDefaultInstance() {
return defaultInstance;
}
public QosTierConfiguration getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QosTierConfiguration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
logSourceName_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier value = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
qosTier_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTierConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public QosTierConfiguration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QosTierConfiguration(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code wireless_android_play_playlog.QosTierConfiguration.QosTier}
*
*
* Quality of Service tiers for Clearcut log upload.
*
*/
public enum QosTier
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DEFAULT = 0;
*
*
* Use GcmNetworkManager to schedule logs upload every hour.
*
*/
DEFAULT(0, 0),
/**
* UNMETERED_ONLY = 1;
*
*
* Log will be uploaded when the device is on an unmetered network.
* Note that devices that rarely or never connect to unmetered networks
* will end up losing many or all of these messages.
*
*/
UNMETERED_ONLY(1, 1),
/**
* UNMETERED_OR_DAILY = 2;
*
*
* Similar to the previous one, but if an upload opportunity does not arise
* near to the end of the day, Clearcut will upload the data as soon as
* the device is online (even if on a metered network).
*
*/
UNMETERED_OR_DAILY(2, 2),
/**
* FAST_IF_RADIO_AWAKE = 3;
*
*
* Log will be uploaded as soon as the device is using the radio.
* If not, log will be uploaded at the next DEFAULT-tier upload time.
*
*/
FAST_IF_RADIO_AWAKE(3, 3),
/**
* NEVER = 4;
*
*
* This is for server to override client QosTier setting.
* If a certain log source's QosTier is set to NEVER, then Clearcut will
* drop log events of that log source immediately and won't save them in
* log store.
*
*/
NEVER(4, 4),
;
/**
* DEFAULT = 0;
*
*
* Use GcmNetworkManager to schedule logs upload every hour.
*
*/
public static final int DEFAULT_VALUE = 0;
/**
* UNMETERED_ONLY = 1;
*
*
* Log will be uploaded when the device is on an unmetered network.
* Note that devices that rarely or never connect to unmetered networks
* will end up losing many or all of these messages.
*
*/
public static final int UNMETERED_ONLY_VALUE = 1;
/**
* UNMETERED_OR_DAILY = 2;
*
*
* Similar to the previous one, but if an upload opportunity does not arise
* near to the end of the day, Clearcut will upload the data as soon as
* the device is online (even if on a metered network).
*
*/
public static final int UNMETERED_OR_DAILY_VALUE = 2;
/**
* FAST_IF_RADIO_AWAKE = 3;
*
*
* Log will be uploaded as soon as the device is using the radio.
* If not, log will be uploaded at the next DEFAULT-tier upload time.
*
*/
public static final int FAST_IF_RADIO_AWAKE_VALUE = 3;
/**
* NEVER = 4;
*
*
* This is for server to override client QosTier setting.
* If a certain log source's QosTier is set to NEVER, then Clearcut will
* drop log events of that log source immediately and won't save them in
* log store.
*
*/
public static final int NEVER_VALUE = 4;
public final int getNumber() { return value; }
public static QosTier valueOf(int value) {
switch (value) {
case 0: return DEFAULT;
case 1: return UNMETERED_ONLY;
case 2: return UNMETERED_OR_DAILY;
case 3: return FAST_IF_RADIO_AWAKE;
case 4: return NEVER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public QosTier findValueByNumber(int number) {
return QosTier.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.getDescriptor().getEnumTypes().get(0);
}
private static final QosTier[] VALUES = values();
public static QosTier valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private QosTier(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wireless_android_play_playlog.QosTierConfiguration.QosTier)
}
private int bitField0_;
// optional string log_source_name = 1;
public static final int LOG_SOURCE_NAME_FIELD_NUMBER = 1;
private java.lang.Object logSourceName_;
/**
* optional string log_source_name = 1;
*/
public boolean hasLogSourceName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string log_source_name = 1;
*/
public java.lang.String getLogSourceName() {
java.lang.Object ref = logSourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
logSourceName_ = s;
}
return s;
}
}
/**
* optional string log_source_name = 1;
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes() {
java.lang.Object ref = logSourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logSourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
public static final int QOS_TIER_FIELD_NUMBER = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier qosTier_;
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier() {
return qosTier_;
}
private void initFields() {
logSourceName_ = "";
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getLogSourceNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, qosTier_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getLogSourceNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, qosTier_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.QosTierConfiguration}
*
*
* QosTierConfiguration configures what Quality of Service tier should be
* applied to a given log source.
*
* Next tag: 3
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTierConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
logSourceName_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.logSourceName_ = logSourceName_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.qosTier_ = qosTier_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.getDefaultInstance()) return this;
if (other.hasLogSourceName()) {
bitField0_ |= 0x00000001;
logSourceName_ = other.logSourceName_;
onChanged();
}
if (other.hasQosTier()) {
setQosTier(other.getQosTier());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string log_source_name = 1;
private java.lang.Object logSourceName_ = "";
/**
* optional string log_source_name = 1;
*/
public boolean hasLogSourceName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string log_source_name = 1;
*/
public java.lang.String getLogSourceName() {
java.lang.Object ref = logSourceName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
logSourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string log_source_name = 1;
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes() {
java.lang.Object ref = logSourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logSourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string log_source_name = 1;
*/
public Builder setLogSourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
logSourceName_ = value;
onChanged();
return this;
}
/**
* optional string log_source_name = 1;
*/
public Builder clearLogSourceName() {
bitField0_ = (bitField0_ & ~0x00000001);
logSourceName_ = getDefaultInstance().getLogSourceName();
onChanged();
return this;
}
/**
* optional string log_source_name = 1;
*/
public Builder setLogSourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
logSourceName_ = value;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier getQosTier() {
return qosTier_;
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public Builder setQosTier(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
qosTier_ = value;
onChanged();
return this;
}
/**
* optional .wireless_android_play_playlog.QosTierConfiguration.QosTier qos_tier = 2;
*/
public Builder clearQosTier() {
bitField0_ = (bitField0_ & ~0x00000002);
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.QosTier.DEFAULT;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.QosTierConfiguration)
}
static {
defaultInstance = new QosTierConfiguration(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.QosTierConfiguration)
}
public interface QosTiersOverrideOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
java.util.List
getQosTierConfigurationList();
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration getQosTierConfiguration(int index);
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
int getQosTierConfigurationCount();
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder>
getQosTierConfigurationOrBuilderList();
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder getQosTierConfigurationOrBuilder(
int index);
// optional int64 qos_tier_fingerprint = 2;
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
boolean hasQosTierFingerprint();
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
long getQosTierFingerprint();
}
/**
* Protobuf type {@code wireless_android_play_playlog.QosTiersOverride}
*
*
* Next tag: 3
*
*/
public static final class QosTiersOverride extends
com.google.protobuf.GeneratedMessage
implements QosTiersOverrideOrBuilder {
// Use QosTiersOverride.newBuilder() to construct.
private QosTiersOverride(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private QosTiersOverride(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final QosTiersOverride defaultInstance;
public static QosTiersOverride getDefaultInstance() {
return defaultInstance;
}
public QosTiersOverride getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QosTiersOverride(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
qosTierConfiguration_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
qosTierConfiguration_.add(input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
qosTierFingerprint_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
qosTierConfiguration_ = java.util.Collections.unmodifiableList(qosTierConfiguration_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTiersOverride_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public QosTiersOverride parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QosTiersOverride(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
public static final int QOS_TIER_CONFIGURATION_FIELD_NUMBER = 1;
private java.util.List qosTierConfiguration_;
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public java.util.List getQosTierConfigurationList() {
return qosTierConfiguration_;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder>
getQosTierConfigurationOrBuilderList() {
return qosTierConfiguration_;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public int getQosTierConfigurationCount() {
return qosTierConfiguration_.size();
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration getQosTierConfiguration(int index) {
return qosTierConfiguration_.get(index);
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder getQosTierConfigurationOrBuilder(
int index) {
return qosTierConfiguration_.get(index);
}
// optional int64 qos_tier_fingerprint = 2;
public static final int QOS_TIER_FINGERPRINT_FIELD_NUMBER = 2;
private long qosTierFingerprint_;
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public boolean hasQosTierFingerprint() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public long getQosTierFingerprint() {
return qosTierFingerprint_;
}
private void initFields() {
qosTierConfiguration_ = java.util.Collections.emptyList();
qosTierFingerprint_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < qosTierConfiguration_.size(); i++) {
output.writeMessage(1, qosTierConfiguration_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(2, qosTierFingerprint_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < qosTierConfiguration_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, qosTierConfiguration_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, qosTierFingerprint_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.QosTiersOverride}
*
*
* Next tag: 3
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTiersOverride_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getQosTierConfigurationFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (qosTierConfigurationBuilder_ == null) {
qosTierConfiguration_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
qosTierConfigurationBuilder_.clear();
}
qosTierFingerprint_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (qosTierConfigurationBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
qosTierConfiguration_ = java.util.Collections.unmodifiableList(qosTierConfiguration_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.qosTierConfiguration_ = qosTierConfiguration_;
} else {
result.qosTierConfiguration_ = qosTierConfigurationBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.qosTierFingerprint_ = qosTierFingerprint_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance()) return this;
if (qosTierConfigurationBuilder_ == null) {
if (!other.qosTierConfiguration_.isEmpty()) {
if (qosTierConfiguration_.isEmpty()) {
qosTierConfiguration_ = other.qosTierConfiguration_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.addAll(other.qosTierConfiguration_);
}
onChanged();
}
} else {
if (!other.qosTierConfiguration_.isEmpty()) {
if (qosTierConfigurationBuilder_.isEmpty()) {
qosTierConfigurationBuilder_.dispose();
qosTierConfigurationBuilder_ = null;
qosTierConfiguration_ = other.qosTierConfiguration_;
bitField0_ = (bitField0_ & ~0x00000001);
qosTierConfigurationBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getQosTierConfigurationFieldBuilder() : null;
} else {
qosTierConfigurationBuilder_.addAllMessages(other.qosTierConfiguration_);
}
}
}
if (other.hasQosTierFingerprint()) {
setQosTierFingerprint(other.getQosTierFingerprint());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
private java.util.List qosTierConfiguration_ =
java.util.Collections.emptyList();
private void ensureQosTierConfigurationIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
qosTierConfiguration_ = new java.util.ArrayList(qosTierConfiguration_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder> qosTierConfigurationBuilder_;
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public java.util.List getQosTierConfigurationList() {
if (qosTierConfigurationBuilder_ == null) {
return java.util.Collections.unmodifiableList(qosTierConfiguration_);
} else {
return qosTierConfigurationBuilder_.getMessageList();
}
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public int getQosTierConfigurationCount() {
if (qosTierConfigurationBuilder_ == null) {
return qosTierConfiguration_.size();
} else {
return qosTierConfigurationBuilder_.getCount();
}
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration getQosTierConfiguration(int index) {
if (qosTierConfigurationBuilder_ == null) {
return qosTierConfiguration_.get(index);
} else {
return qosTierConfigurationBuilder_.getMessage(index);
}
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder setQosTierConfiguration(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration value) {
if (qosTierConfigurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.set(index, value);
onChanged();
} else {
qosTierConfigurationBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder setQosTierConfiguration(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder builderForValue) {
if (qosTierConfigurationBuilder_ == null) {
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.set(index, builderForValue.build());
onChanged();
} else {
qosTierConfigurationBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder addQosTierConfiguration(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration value) {
if (qosTierConfigurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.add(value);
onChanged();
} else {
qosTierConfigurationBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder addQosTierConfiguration(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration value) {
if (qosTierConfigurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.add(index, value);
onChanged();
} else {
qosTierConfigurationBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder addQosTierConfiguration(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder builderForValue) {
if (qosTierConfigurationBuilder_ == null) {
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.add(builderForValue.build());
onChanged();
} else {
qosTierConfigurationBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder addQosTierConfiguration(
int index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder builderForValue) {
if (qosTierConfigurationBuilder_ == null) {
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.add(index, builderForValue.build());
onChanged();
} else {
qosTierConfigurationBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder addAllQosTierConfiguration(
java.lang.Iterable extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration> values) {
if (qosTierConfigurationBuilder_ == null) {
ensureQosTierConfigurationIsMutable();
super.addAll(values, qosTierConfiguration_);
onChanged();
} else {
qosTierConfigurationBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder clearQosTierConfiguration() {
if (qosTierConfigurationBuilder_ == null) {
qosTierConfiguration_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
qosTierConfigurationBuilder_.clear();
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public Builder removeQosTierConfiguration(int index) {
if (qosTierConfigurationBuilder_ == null) {
ensureQosTierConfigurationIsMutable();
qosTierConfiguration_.remove(index);
onChanged();
} else {
qosTierConfigurationBuilder_.remove(index);
}
return this;
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder getQosTierConfigurationBuilder(
int index) {
return getQosTierConfigurationFieldBuilder().getBuilder(index);
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder getQosTierConfigurationOrBuilder(
int index) {
if (qosTierConfigurationBuilder_ == null) {
return qosTierConfiguration_.get(index); } else {
return qosTierConfigurationBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public java.util.List extends com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder>
getQosTierConfigurationOrBuilderList() {
if (qosTierConfigurationBuilder_ != null) {
return qosTierConfigurationBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(qosTierConfiguration_);
}
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder addQosTierConfigurationBuilder() {
return getQosTierConfigurationFieldBuilder().addBuilder(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder addQosTierConfigurationBuilder(
int index) {
return getQosTierConfigurationFieldBuilder().addBuilder(
index, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.getDefaultInstance());
}
/**
* repeated .wireless_android_play_playlog.QosTierConfiguration qos_tier_configuration = 1;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
* This usually happens when server is burdened with fast qos tiers.
*
*/
public java.util.List
getQosTierConfigurationBuilderList() {
return getQosTierConfigurationFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder>
getQosTierConfigurationFieldBuilder() {
if (qosTierConfigurationBuilder_ == null) {
qosTierConfigurationBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfiguration.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTierConfigurationOrBuilder>(
qosTierConfiguration_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
qosTierConfiguration_ = null;
}
return qosTierConfigurationBuilder_;
}
// optional int64 qos_tier_fingerprint = 2;
private long qosTierFingerprint_ ;
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public boolean hasQosTierFingerprint() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public long getQosTierFingerprint() {
return qosTierFingerprint_;
}
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public Builder setQosTierFingerprint(long value) {
bitField0_ |= 0x00000002;
qosTierFingerprint_ = value;
onChanged();
return this;
}
/**
* optional int64 qos_tier_fingerprint = 2;
*
*
* The fingerprint of the qos_tier_configuration field.
*
*/
public Builder clearQosTierFingerprint() {
bitField0_ = (bitField0_ & ~0x00000002);
qosTierFingerprint_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.QosTiersOverride)
}
static {
defaultInstance = new QosTiersOverride(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.QosTiersOverride)
}
public interface LogSourceBatchingBlacklistOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated string log_source_name = 1;
/**
* repeated string log_source_name = 1;
*/
java.util.List
getLogSourceNameList();
/**
* repeated string log_source_name = 1;
*/
int getLogSourceNameCount();
/**
* repeated string log_source_name = 1;
*/
java.lang.String getLogSourceName(int index);
/**
* repeated string log_source_name = 1;
*/
com.google.protobuf.ByteString
getLogSourceNameBytes(int index);
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogSourceBatchingBlacklist}
*
*
* Next tag: 2
*
*/
public static final class LogSourceBatchingBlacklist extends
com.google.protobuf.GeneratedMessage
implements LogSourceBatchingBlacklistOrBuilder {
// Use LogSourceBatchingBlacklist.newBuilder() to construct.
private LogSourceBatchingBlacklist(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private LogSourceBatchingBlacklist(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final LogSourceBatchingBlacklist defaultInstance;
public static LogSourceBatchingBlacklist getDefaultInstance() {
return defaultInstance;
}
public LogSourceBatchingBlacklist getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogSourceBatchingBlacklist(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
logSourceName_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
logSourceName_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
logSourceName_ = new com.google.protobuf.UnmodifiableLazyStringList(logSourceName_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public LogSourceBatchingBlacklist parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogSourceBatchingBlacklist(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated string log_source_name = 1;
public static final int LOG_SOURCE_NAME_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList logSourceName_;
/**
* repeated string log_source_name = 1;
*/
public java.util.List
getLogSourceNameList() {
return logSourceName_;
}
/**
* repeated string log_source_name = 1;
*/
public int getLogSourceNameCount() {
return logSourceName_.size();
}
/**
* repeated string log_source_name = 1;
*/
public java.lang.String getLogSourceName(int index) {
return logSourceName_.get(index);
}
/**
* repeated string log_source_name = 1;
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes(int index) {
return logSourceName_.getByteString(index);
}
private void initFields() {
logSourceName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < logSourceName_.size(); i++) {
output.writeBytes(1, logSourceName_.getByteString(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < logSourceName_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(logSourceName_.getByteString(i));
}
size += dataSize;
size += 1 * getLogSourceNameList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogSourceBatchingBlacklist}
*
*
* Next tag: 2
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
logSourceName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
logSourceName_ = new com.google.protobuf.UnmodifiableLazyStringList(
logSourceName_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logSourceName_ = logSourceName_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance()) return this;
if (!other.logSourceName_.isEmpty()) {
if (logSourceName_.isEmpty()) {
logSourceName_ = other.logSourceName_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogSourceNameIsMutable();
logSourceName_.addAll(other.logSourceName_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated string log_source_name = 1;
private com.google.protobuf.LazyStringList logSourceName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureLogSourceNameIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
logSourceName_ = new com.google.protobuf.LazyStringArrayList(logSourceName_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string log_source_name = 1;
*/
public java.util.List
getLogSourceNameList() {
return java.util.Collections.unmodifiableList(logSourceName_);
}
/**
* repeated string log_source_name = 1;
*/
public int getLogSourceNameCount() {
return logSourceName_.size();
}
/**
* repeated string log_source_name = 1;
*/
public java.lang.String getLogSourceName(int index) {
return logSourceName_.get(index);
}
/**
* repeated string log_source_name = 1;
*/
public com.google.protobuf.ByteString
getLogSourceNameBytes(int index) {
return logSourceName_.getByteString(index);
}
/**
* repeated string log_source_name = 1;
*/
public Builder setLogSourceName(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLogSourceNameIsMutable();
logSourceName_.set(index, value);
onChanged();
return this;
}
/**
* repeated string log_source_name = 1;
*/
public Builder addLogSourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLogSourceNameIsMutable();
logSourceName_.add(value);
onChanged();
return this;
}
/**
* repeated string log_source_name = 1;
*/
public Builder addAllLogSourceName(
java.lang.Iterable values) {
ensureLogSourceNameIsMutable();
super.addAll(values, logSourceName_);
onChanged();
return this;
}
/**
* repeated string log_source_name = 1;
*/
public Builder clearLogSourceName() {
logSourceName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string log_source_name = 1;
*/
public Builder addLogSourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureLogSourceNameIsMutable();
logSourceName_.add(value);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.LogSourceBatchingBlacklist)
}
static {
defaultInstance = new LogSourceBatchingBlacklist(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.LogSourceBatchingBlacklist)
}
public interface LogResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional int64 next_request_wait_millis = 1 [default = -1];
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
boolean hasNextRequestWaitMillis();
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
long getNextRequestWaitMillis();
// optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
boolean hasExperiments();
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList getExperiments();
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder getExperimentsOrBuilder();
// optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
boolean hasQosTier();
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride getQosTier();
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder getQosTierOrBuilder();
// optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
boolean hasLogSourceBatchingBlacklist();
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist getLogSourceBatchingBlacklist();
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder getLogSourceBatchingBlacklistOrBuilder();
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogResponse}
*
*
* Next tag: 5
*
*/
public static final class LogResponse extends
com.google.protobuf.GeneratedMessage
implements LogResponseOrBuilder {
// Use LogResponse.newBuilder() to construct.
private LogResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private LogResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final LogResponse defaultInstance;
public static LogResponse getDefaultInstance() {
return defaultInstance;
}
public LogResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
nextRequestWaitMillis_ = input.readInt64();
break;
}
case 18: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = experiments_.toBuilder();
}
experiments_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(experiments_);
experiments_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = qosTier_.toBuilder();
}
qosTier_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(qosTier_);
qosTier_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = logSourceBatchingBlacklist_.toBuilder();
}
logSourceBatchingBlacklist_ = input.readMessage(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(logSourceBatchingBlacklist_);
logSourceBatchingBlacklist_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public LogResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int64 next_request_wait_millis = 1 [default = -1];
public static final int NEXT_REQUEST_WAIT_MILLIS_FIELD_NUMBER = 1;
private long nextRequestWaitMillis_;
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public boolean hasNextRequestWaitMillis() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public long getNextRequestWaitMillis() {
return nextRequestWaitMillis_;
}
// optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
public static final int EXPERIMENTS_FIELD_NUMBER = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList experiments_;
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public boolean hasExperiments() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList getExperiments() {
return experiments_;
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder getExperimentsOrBuilder() {
return experiments_;
}
// optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
public static final int QOS_TIER_FIELD_NUMBER = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride qosTier_;
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride getQosTier() {
return qosTier_;
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder getQosTierOrBuilder() {
return qosTier_;
}
// optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
public static final int LOG_SOURCE_BATCHING_BLACKLIST_FIELD_NUMBER = 4;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist logSourceBatchingBlacklist_;
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public boolean hasLogSourceBatchingBlacklist() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist getLogSourceBatchingBlacklist() {
return logSourceBatchingBlacklist_;
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder getLogSourceBatchingBlacklistOrBuilder() {
return logSourceBatchingBlacklist_;
}
private void initFields() {
nextRequestWaitMillis_ = -1L;
experiments_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance();
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance();
logSourceBatchingBlacklist_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, nextRequestWaitMillis_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, experiments_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, qosTier_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, logSourceBatchingBlacklist_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, nextRequestWaitMillis_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, experiments_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, qosTier_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, logSourceBatchingBlacklist_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wireless_android_play_playlog.LogResponse}
*
*
* Next tag: 5
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.class, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.Builder.class);
}
// Construct using com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getExperimentsFieldBuilder();
getQosTierFieldBuilder();
getLogSourceBatchingBlacklistFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
nextRequestWaitMillis_ = -1L;
bitField0_ = (bitField0_ & ~0x00000001);
if (experimentsBuilder_ == null) {
experiments_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance();
} else {
experimentsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (qosTierBuilder_ == null) {
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance();
} else {
qosTierBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (logSourceBatchingBlacklistBuilder_ == null) {
logSourceBatchingBlacklist_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance();
} else {
logSourceBatchingBlacklistBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.internal_static_wireless_android_play_playlog_LogResponse_descriptor;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse getDefaultInstanceForType() {
return com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.getDefaultInstance();
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse build() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse buildPartial() {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse result = new com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.nextRequestWaitMillis_ = nextRequestWaitMillis_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (experimentsBuilder_ == null) {
result.experiments_ = experiments_;
} else {
result.experiments_ = experimentsBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (qosTierBuilder_ == null) {
result.qosTier_ = qosTier_;
} else {
result.qosTier_ = qosTierBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (logSourceBatchingBlacklistBuilder_ == null) {
result.logSourceBatchingBlacklist_ = logSourceBatchingBlacklist_;
} else {
result.logSourceBatchingBlacklist_ = logSourceBatchingBlacklistBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse) {
return mergeFrom((com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse other) {
if (other == com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse.getDefaultInstance()) return this;
if (other.hasNextRequestWaitMillis()) {
setNextRequestWaitMillis(other.getNextRequestWaitMillis());
}
if (other.hasExperiments()) {
mergeExperiments(other.getExperiments());
}
if (other.hasQosTier()) {
mergeQosTier(other.getQosTier());
}
if (other.hasLogSourceBatchingBlacklist()) {
mergeLogSourceBatchingBlacklist(other.getLogSourceBatchingBlacklist());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int64 next_request_wait_millis = 1 [default = -1];
private long nextRequestWaitMillis_ = -1L;
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public boolean hasNextRequestWaitMillis() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public long getNextRequestWaitMillis() {
return nextRequestWaitMillis_;
}
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public Builder setNextRequestWaitMillis(long value) {
bitField0_ |= 0x00000001;
nextRequestWaitMillis_ = value;
onChanged();
return this;
}
/**
* optional int64 next_request_wait_millis = 1 [default = -1];
*
*
* Client should wait for next_request_wait_millis before sending the next
* log request.
*
*/
public Builder clearNextRequestWaitMillis() {
bitField0_ = (bitField0_ & ~0x00000001);
nextRequestWaitMillis_ = -1L;
onChanged();
return this;
}
// optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList experiments_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder> experimentsBuilder_;
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public boolean hasExperiments() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList getExperiments() {
if (experimentsBuilder_ == null) {
return experiments_;
} else {
return experimentsBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public Builder setExperiments(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList value) {
if (experimentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
experiments_ = value;
onChanged();
} else {
experimentsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public Builder setExperiments(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder builderForValue) {
if (experimentsBuilder_ == null) {
experiments_ = builderForValue.build();
onChanged();
} else {
experimentsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public Builder mergeExperiments(com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList value) {
if (experimentsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
experiments_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance()) {
experiments_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.newBuilder(experiments_).mergeFrom(value).buildPartial();
} else {
experiments_ = value;
}
onChanged();
} else {
experimentsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public Builder clearExperiments() {
if (experimentsBuilder_ == null) {
experiments_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.getDefaultInstance();
onChanged();
} else {
experimentsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder getExperimentsBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getExperimentsFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder getExperimentsOrBuilder() {
if (experimentsBuilder_ != null) {
return experimentsBuilder_.getMessageOrBuilder();
} else {
return experiments_;
}
}
/**
* optional .wireless_android_play_playlog.ExperimentIdList experiments = 2;
*
*
* If present, this is the new set of enabled experiments for this client.
* Otherwise, the client should assume that there has been no change in the
* set of enabled experiments.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder>
getExperimentsFieldBuilder() {
if (experimentsBuilder_ == null) {
experimentsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdList.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.ExperimentIdListOrBuilder>(
experiments_,
getParentForChildren(),
isClean());
experiments_ = null;
}
return experimentsBuilder_;
}
// optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder> qosTierBuilder_;
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public boolean hasQosTier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride getQosTier() {
if (qosTierBuilder_ == null) {
return qosTier_;
} else {
return qosTierBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public Builder setQosTier(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride value) {
if (qosTierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
qosTier_ = value;
onChanged();
} else {
qosTierBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public Builder setQosTier(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder builderForValue) {
if (qosTierBuilder_ == null) {
qosTier_ = builderForValue.build();
onChanged();
} else {
qosTierBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public Builder mergeQosTier(com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride value) {
if (qosTierBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
qosTier_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance()) {
qosTier_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.newBuilder(qosTier_).mergeFrom(value).buildPartial();
} else {
qosTier_ = value;
}
onChanged();
} else {
qosTierBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public Builder clearQosTier() {
if (qosTierBuilder_ == null) {
qosTier_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.getDefaultInstance();
onChanged();
} else {
qosTierBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder getQosTierBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getQosTierFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder getQosTierOrBuilder() {
if (qosTierBuilder_ != null) {
return qosTierBuilder_.getMessageOrBuilder();
} else {
return qosTier_;
}
}
/**
* optional .wireless_android_play_playlog.QosTiersOverride qos_tier = 3;
*
*
* Quality of Service tiers enforced by server for overriding client
* qos_tier setting in event logging.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder>
getQosTierFieldBuilder() {
if (qosTierBuilder_ == null) {
qosTierBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverride.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.QosTiersOverrideOrBuilder>(
qosTier_,
getParentForChildren(),
isClean());
qosTier_ = null;
}
return qosTierBuilder_;
}
// optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
private com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist logSourceBatchingBlacklist_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder> logSourceBatchingBlacklistBuilder_;
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public boolean hasLogSourceBatchingBlacklist() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist getLogSourceBatchingBlacklist() {
if (logSourceBatchingBlacklistBuilder_ == null) {
return logSourceBatchingBlacklist_;
} else {
return logSourceBatchingBlacklistBuilder_.getMessage();
}
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public Builder setLogSourceBatchingBlacklist(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist value) {
if (logSourceBatchingBlacklistBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
logSourceBatchingBlacklist_ = value;
onChanged();
} else {
logSourceBatchingBlacklistBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public Builder setLogSourceBatchingBlacklist(
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder builderForValue) {
if (logSourceBatchingBlacklistBuilder_ == null) {
logSourceBatchingBlacklist_ = builderForValue.build();
onChanged();
} else {
logSourceBatchingBlacklistBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public Builder mergeLogSourceBatchingBlacklist(com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist value) {
if (logSourceBatchingBlacklistBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
logSourceBatchingBlacklist_ != com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance()) {
logSourceBatchingBlacklist_ =
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.newBuilder(logSourceBatchingBlacklist_).mergeFrom(value).buildPartial();
} else {
logSourceBatchingBlacklist_ = value;
}
onChanged();
} else {
logSourceBatchingBlacklistBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public Builder clearLogSourceBatchingBlacklist() {
if (logSourceBatchingBlacklistBuilder_ == null) {
logSourceBatchingBlacklist_ = com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.getDefaultInstance();
onChanged();
} else {
logSourceBatchingBlacklistBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder getLogSourceBatchingBlacklistBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getLogSourceBatchingBlacklistFieldBuilder().getBuilder();
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
public com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder getLogSourceBatchingBlacklistOrBuilder() {
if (logSourceBatchingBlacklistBuilder_ != null) {
return logSourceBatchingBlacklistBuilder_.getMessageOrBuilder();
} else {
return logSourceBatchingBlacklist_;
}
}
/**
* optional .wireless_android_play_playlog.LogSourceBatchingBlacklist log_source_batching_blacklist = 4;
*
*
* Enforced by server for telling Clearcut uploader not to group log events
* from certain log sources. If it is not set, client will try to group log
* events together with the same upload context without considering log
* sources restrictions. Upload context includes following info.
* 1. PlayLoggerContext.isAnonymous
* 2. PlayLoggerContext.uploadAccountName
* 3. PlayLoggerContext.zwiebackCookie
* 4. PlayLoggerContext.qosTier
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder>
getLogSourceBatchingBlacklistFieldBuilder() {
if (logSourceBatchingBlacklistBuilder_ == null) {
logSourceBatchingBlacklistBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklist.Builder, com.google.wireless.android.play.playlog.proto.ClientAnalytics.LogSourceBatchingBlacklistOrBuilder>(
logSourceBatchingBlacklist_,
getParentForChildren(),
isClean());
logSourceBatchingBlacklist_ = null;
}
return logSourceBatchingBlacklistBuilder_;
}
// @@protoc_insertion_point(builder_scope:wireless_android_play_playlog.LogResponse)
}
static {
defaultInstance = new LogResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:wireless_android_play_playlog.LogResponse)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_LogEventKeyValues_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_ActiveExperiments_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_LogEvent_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_LogEvent_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_NetworkConnectionInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_ExperimentIds_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_ExperimentIds_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_AndroidClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_BrowserInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_BrowserInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_DesktopClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_IosClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_IosClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_PancettaClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_PlayCeClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_VrClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_VrClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_JsClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_JsClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_ClientInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_ClientInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_ExperimentIdList_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_BatchedLogRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_LogRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_LogRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_DeviceStatus_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_DeviceStatus_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_ExternalTimestamp_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_QosTierConfiguration_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_QosTiersOverride_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_wireless_android_play_playlog_LogResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_wireless_android_play_playlog_LogResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\025clientanalytics.proto\022\035wireless_androi" +
"d_play_playlog\"/\n\021LogEventKeyValues\022\013\n\003k" +
"ey\030\001 \001(\t\022\r\n\005value\030\002 \001(\t\"\247\001\n\021ActiveExperi" +
"ments\022\"\n\032client_altering_experiment\030\001 \003(" +
"\t\022\030\n\020other_experiment\030\002 \003(\t\022\026\n\016gws_exper" +
"iment\030\003 \003(\005\022\027\n\017play_experiment\030\004 \003(\003\022#\n\033" +
"unsupported_play_experiment\030\005 \003(\003\"\227\007\n\010Lo" +
"gEvent\022\025\n\revent_time_ms\030\001 \001(\003\022\027\n\017event_u" +
"ptime_ms\030\021 \001(\003\022\031\n\021sequence_position\030\025 \001(" +
"\003\022\013\n\003tag\030\002 \001(\t\022\022\n\nevent_code\030\013 \001(\005\022\025\n\rev",
"ent_flow_id\030\014 \001(\005\022\031\n\021is_user_initiated\030\n" +
" \001(\010\022?\n\005value\030\003 \003(\01320.wireless_android_p" +
"lay_playlog.LogEventKeyValues\022\r\n\005store\030\004" +
" \001(\014\022G\n\014app_usage_1p\030\t \001(\01321.wireless_an" +
"droid_play_playlog.AppUsage1pLogEvent\022\030\n" +
"\020source_extension\030\006 \001(\014\022\033\n\023source_extens" +
"ion_js\030\010 \001(\t\022\035\n\025source_extension_json\030\r " +
"\001(\t\022=\n\003exp\030\007 \001(\01320.wireless_android_play" +
"_playlog.ActiveExperiments\022\017\n\007test_id\030\016 " +
"\001(\t\022\'\n\027timezone_offset_seconds\030\017 \001(\022:\00618",
"0000\022D\n\016experiment_ids\030\020 \001(\0132,.wireless_" +
"android_play_playlog.ExperimentIds\022\021\n\tcl" +
"ient_ve\030\022 \001(\014\022\024\n\014client_ve_js\030\030 \001(\t\022M\n\016i" +
"nternal_event\030\023 \001(\01625.wireless_android_p" +
"lay_playlog.LogEvent.InternalEvent\022\021\n\tte" +
"st_code\030\024 \003(\005\022\022\n\nboot_count\030\026 \001(\003\022U\n\027net" +
"work_connection_info\030\027 \001(\01324.wireless_an" +
"droid_play_playlog.NetworkConnectionInfo" +
"\">\n\rInternalEvent\022\010\n\004NONE\020\000\022\022\n\016WALL_CLOC" +
"K_SET\020\001\022\017\n\013DEVICE_BOOT\020\002*\t\010\350\007\020\200\200\200\200\002\"\234\003\n\025",
"NetworkConnectionInfo\022\\\n\014network_type\030\001 " +
"\001(\[email protected]_android_play_playlog.Netw" +
"orkConnectionInfo.NetworkType:\004NONE\"\244\002\n\013" +
"NetworkType\022\021\n\004NONE\020\377\377\377\377\377\377\377\377\377\001\022\n\n\006MOBILE" +
"\020\000\022\010\n\004WIFI\020\001\022\016\n\nMOBILE_MMS\020\002\022\017\n\013MOBILE_S" +
"UPL\020\003\022\016\n\nMOBILE_DUN\020\004\022\020\n\014MOBILE_HIPRI\020\005\022" +
"\t\n\005WIMAX\020\006\022\r\n\tBLUETOOTH\020\007\022\t\n\005DUMMY\020\010\022\014\n\010" +
"ETHERNET\020\t\022\017\n\013MOBILE_FOTA\020\n\022\016\n\nMOBILE_IM" +
"S\020\013\022\016\n\nMOBILE_CBS\020\014\022\014\n\010WIFI_P2P\020\r\022\r\n\tMOB" +
"ILE_IA\020\016\022\024\n\020MOBILE_EMERGENCY\020\017\022\t\n\005PROXY\020",
"\020\022\007\n\003VPN\020\021\"g\n\rExperimentIds\022\022\n\nclear_blo" +
"b\030\001 \001(\014\022\025\n\rclear_blob_js\030\004 \001(\t\022\026\n\016encryp" +
"ted_blob\030\002 \003(\014\022\023\n\013users_match\030\003 \001(\010\"\334\003\n\021" +
"AndroidClientInfo\022\022\n\nandroid_id\030\001 \001(\003\022\022\n" +
"\nlogging_id\030\002 \001(\t\022\021\n\tdevice_id\030\022 \001(\003\022\023\n\013" +
"sdk_version\030\003 \001(\005\022\r\n\005model\030\004 \001(\t\022\017\n\007prod" +
"uct\030\005 \001(\t\022\020\n\010hardware\030\010 \001(\t\022\016\n\006device\030\t " +
"\001(\t\022\020\n\010os_build\030\006 \001(\t\022\031\n\021application_bui" +
"ld\030\007 \001(\t\022\017\n\007mcc_mnc\030\n \001(\t\022\016\n\006locale\030\013 \001(" +
"\t\022\017\n\007country\030\014 \001(\t\022\024\n\014manufacturer\030\r \001(\t",
"\022\r\n\005brand\030\016 \001(\t\022\r\n\005board\030\017 \001(\t\022\025\n\rradio_" +
"version\030\020 \001(\t\022\023\n\013fingerprint\030\021 \001(\t\022\035\n\025gm" +
"s_core_version_code\030\023 \001(\005\022\034\n\024is_sidewind" +
"er_device\030\024 \001(\010\022\033\n\023chimera_config_info\030\025" +
" \001(\014\022\034\n\024using_log_source_int\030\026 \001(\010\"^\n\013Br" +
"owserInfo\022\016\n\006locale\030\001 \001(\t\022\017\n\007browser\030\002 \001" +
"(\t\022\027\n\017browser_version\030\003 \001(\t\022\025\n\rflash_ver" +
"sion\030\004 \001(\t\"\245\001\n\021DesktopClientInfo\022\021\n\tclie" +
"nt_id\030\001 \001(\t\022\022\n\nlogging_id\030\002 \001(\t\022\n\n\002os\030\003 " +
"\001(\t\022\030\n\020os_major_version\030\004 \001(\t\022\027\n\017os_full",
"_version\030\005 \001(\t\022\031\n\021application_build\030\006 \001(" +
"\t\022\017\n\007country\030\007 \001(\t\"\273\001\n\rIosClientInfo\022\021\n\t" +
"client_id\030\001 \001(\t\022\022\n\nlogging_id\030\002 \001(\t\022\030\n\020o" +
"s_major_version\030\003 \001(\t\022\027\n\017os_full_version" +
"\030\004 \001(\t\022\031\n\021application_build\030\005 \001(\t\022\017\n\007cou" +
"ntry\030\006 \001(\t\022\r\n\005model\030\007 \001(\t\022\025\n\rlanguage_co" +
"de\030\010 \001(\t\"\270\002\n\022PancettaClientInfo\022\021\n\tdevic" +
"e_id\030\001 \001(\t\022D\n\002os\030\002 \001(\01628.wireless_androi" +
"d_play_playlog.PancettaClientInfo.OsType" +
"\022\016\n\006app_id\030\003 \001(\t\022\023\n\013app_version\030\004 \001(\t\022\017\n",
"\007mcc_mnc\030\005 \001(\t\"\222\001\n\006OsType\022\023\n\017OS_TYPE_UNK" +
"NOWN\020\000\022\017\n\013OS_TYPE_MAC\020\001\022\023\n\017OS_TYPE_WINDO" +
"WS\020\002\022\023\n\017OS_TYPE_ANDROID\020\003\022\020\n\014OS_TYPE_CRO" +
"S\020\004\022\021\n\rOS_TYPE_LINUX\020\005\022\023\n\017OS_TYPE_OPENBS" +
"D\020\006\"\234\001\n\020PlayCeClientInfo\022\021\n\tclient_id\030\001 " +
"\001(\t\022\022\n\nlogging_id\030\007 \001(\t\022\014\n\004make\030\003 \001(\t\022\r\n" +
"\005model\030\004 \001(\t\022\031\n\021application_build\030\005 \001(\t\022" +
"\030\n\020platform_version\030\006 \001(\t\022\017\n\007country\030\010 \001" +
"(\t\"\240\003\n\014VrClientInfo\022P\n\016vr_client_type\030\001 " +
"\001(\01628.wireless_android_play_playlog.VrCl",
"ientInfo.VrClientType\022\023\n\013sdk_version\030\002 \001" +
"(\t\022\023\n\013fingerprint\030\003 \001(\t\022\023\n\013gvr_version\030\004" +
" \001(\t\022\024\n\014manufacturer\030\005 \001(\t\022\r\n\005model\030\006 \001(" +
"\t\022\020\n\010language\030\007 \001(\t\022\017\n\007country\030\010 \001(\t\022\025\n\r" +
"unity_version\030\t \001(\t\022\031\n\021unity_sdk_version" +
"\030\n \001(\t\"\204\001\n\014VrClientType\022\013\n\007UNKNOWN\020\000\022\031\n\025" +
"ANDROID_CARDBOARD_SDK\020\001\022\025\n\021IOS_CARDBOARD" +
"_SDK\020\002\022\025\n\021ANDROID_UNITY_SDK\020\003\022\021\n\rIOS_UNI" +
"TY_SDK\020\004\022\013\n\007WINDOWS\020\005\"\230\003\n\014JsClientInfo\022\n" +
"\n\002os\030\001 \001(\t\022\022\n\nos_version\030\002 \001(\t\022K\n\013device",
"_type\030\003 \001(\01626.wireless_android_play_play" +
"log.JsClientInfo.DeviceType\022\017\n\007country\030\004" +
" \001(\t\022\016\n\006locale\030\005 \001(\t\022C\n\007os_type\030\006 \001(\01622." +
"wireless_android_play_playlog.JsClientIn" +
"fo.OsType\"u\n\006OsType\022\016\n\nOS_UNKNOWN\020\000\022\007\n\003M" +
"AC\020\001\022\013\n\007WINDOWS\020\002\022\013\n\007ANDROID\020\003\022\t\n\005LINUX\020" +
"\004\022\r\n\tCHROME_OS\020\005\022\010\n\004IPAD\020\006\022\n\n\006IPHONE\020\007\022\010" +
"\n\004IPOD\020\010\">\n\nDeviceType\022\013\n\007UNKNOWN\020\000\022\n\n\006M" +
"OBILE\020\001\022\n\n\006TABLET\020\002\022\013\n\007DESKTOP\020\003\"\326\006\n\nCli" +
"entInfo\022I\n\013client_type\030\001 \001(\01624.wireless_",
"android_play_playlog.ClientInfo.ClientTy" +
"pe\022\023\n\013remote_host\030\006 \001(\t\022\024\n\014remote_host6\030" +
"\007 \001(\t\022M\n\023android_client_info\030\002 \001(\01320.wir" +
"eless_android_play_playlog.AndroidClient" +
"Info\022M\n\023desktop_client_info\030\003 \001(\01320.wire" +
"less_android_play_playlog.DesktopClientI" +
"nfo\022E\n\017ios_client_info\030\004 \001(\0132,.wireless_" +
"android_play_playlog.IosClientInfo\022L\n\023pl" +
"ay_ce_client_info\030\005 \001(\0132/.wireless_andro" +
"id_play_playlog.PlayCeClientInfo\022C\n\016vr_c",
"lient_info\030\010 \001(\0132+.wireless_android_play" +
"_playlog.VrClientInfo\022O\n\024pancetta_client" +
"_info\030\n \001(\01321.wireless_android_play_play" +
"log.PancettaClientInfo\022@\n\014browser_info\030\t" +
" \001(\0132*.wireless_android_play_playlog.Bro" +
"wserInfo\022C\n\016js_client_info\030\013 \001(\0132+.wirel" +
"ess_android_play_playlog.JsClientInfo\"\201\001" +
"\n\nClientType\022\013\n\007UNKNOWN\020\000\022\006\n\002JS\020\001\022\013\n\007DES" +
"KTOP\020\002\022\007\n\003IOS\020\003\022\013\n\007ANDROID\020\004\022\013\n\007PLAY_CE\020" +
"\005\022\n\n\006PYTHON\020\006\022\006\n\002VR\020\007\022\014\n\010PANCETTA\020\010\022\014\n\010D",
"RIVE_FS\020\t\"\036\n\020ExperimentIdList\022\n\n\002id\030\001 \003(" +
"\t\"U\n\022AppUsage1pLogEvent\022\020\n\010app_type\030\001 \001(" +
"\005\022\034\n\024android_package_name\030\002 \001(\t\022\017\n\007versi" +
"on\030\003 \001(\t\"S\n\021BatchedLogRequest\022>\n\013log_req" +
"uest\030\001 \003(\0132).wireless_android_play_playl" +
"og.LogRequest\"\320:\n\nLogRequest\022\027\n\017request_" +
"time_ms\030\004 \001(\003\022\031\n\021request_uptime_ms\030\010 \001(\003" +
"\022>\n\013client_info\030\001 \001(\0132).wireless_android" +
"_play_playlog.ClientInfo\022P\n\nlog_source\030\002" +
" \001(\01623.wireless_android_play_playlog.Log",
"Request.LogSource:\007UNKNOWN\022\027\n\017log_source" +
"_name\030\006 \001(\t\022\027\n\017zwieback_cookie\030\007 \001(\t\022:\n\t" +
"log_event\030\003 \003(\0132\'.wireless_android_play_" +
"playlog.LogEvent\022\035\n\025serialized_log_event" +
"s\030\005 \003(\014\022V\n\010qos_tier\030\t \001(\0162;.wireless_and" +
"roid_play_playlog.QosTierConfiguration.Q" +
"osTier:\007DEFAULT\022J\n\tscheduler\030\n \001(\01627.wir" +
"eless_android_play_playlog.LogRequest.Sc" +
"hedulerType\022B\n\rdevice_status\030\013 \001(\0132+.wir" +
"eless_android_play_playlog.DeviceStatus\022",
"L\n\022external_timestamp\030\014 \001(\01320.wireless_a" +
"ndroid_play_playlog.ExternalTimestamp\"\2314" +
"\n\tLogSource\022\024\n\007UNKNOWN\020\377\377\377\377\377\377\377\377\377\001\022\t\n\005STO" +
"RE\020\000\022\r\n\tWEB_STORE\020A\022\017\n\nWORK_STORE\020\204\001\022\023\n\016" +
"WORK_STORE_APP\020\205\002\022\r\n\tEDU_STORE\020\017\022\t\n\005MUSI" +
"C\020\001\022\t\n\005BOOKS\020\002\022\t\n\005VIDEO\020\003\022\n\n\006MOVIES\020J\022\r\n" +
"\tMAGAZINES\020\004\022\t\n\005GAMES\020\005\022\010\n\004LB_A\020\006\022\017\n\013AND" +
"ROID_IDE\020\007\022\010\n\004LB_P\020\010\022\010\n\004LB_S\020\t\022\014\n\010GMS_CO" +
"RE\020\n\022\020\n\014APP_USAGE_1P\020\013\022\t\n\005ICING\020\014\022\014\n\010HER" +
"REVAD\020\r\022\r\n\tGOOGLE_TV\020\016\022\023\n\017GMS_CORE_PEOPL",
"E\020\020\022\006\n\002LE\020\021\022\024\n\020GOOGLE_ANALYTICS\020\022\022\010\n\004LB_" +
"D\020\023\022\017\n\013ANDROID_GSA\020\024\022\010\n\004LB_T\020\025\022\023\n\017PERSON" +
"AL_LOGGER\020\026\022\033\n\027PERSONAL_BROWSER_LOGGER\020%" +
"\022\"\n\036GMS_CORE_WALLET_MERCHANT_ERROR\020\027\022\010\n\004" +
"LB_C\020\030\022\t\n\005LB_IA\0204\022\n\n\005LB_CB\020\355\001\022\n\n\005LB_DM\020\214" +
"\002\022\020\n\014ANDROID_AUTH\020\031\022\022\n\016ANDROID_CAMERA\020\032\022" +
"\006\n\002CW\020\033\022\020\n\013CW_COUNTERS\020\363\001\022\007\n\003GEL\020\034\022\016\n\nDN" +
"A_PROBER\020\035\022\007\n\003UDR\020\036\022\023\n\017GMS_CORE_WALLET\020\037" +
"\022\n\n\006SOCIAL\020 \022\022\n\016INSTORE_WALLET\020!\022\010\n\004NOVA" +
"\020\"\022\t\n\005LB_CA\020#\022\r\n\tLATIN_IME\020$\022\020\n\014NEWS_WEA",
"THER\020&\022\013\n\007HANGOUT\020\'\022\027\n\023HANGOUT_LOG_REQUE" +
"ST\0202\022\016\n\nCOPRESENCE\020(\022\023\n\017SOCIAL_AFFINITY\020" +
")\022\n\n\006PHOTOS\020*\022\007\n\003GCM\020+\022\n\n\006GOKART\020,\022\t\n\005FI" +
"NDR\020-\022\025\n\021ANDROID_MESSAGING\020.\022\016\n\nSOCIAL_W" +
"EB\020/\022\014\n\010BACKDROP\0200\022\016\n\nTELEMATICS\0201\022\021\n\rGV" +
"C_HARVESTER\0203\022\007\n\003CAR\0205\022\021\n\rPIXEL_PERFECT\020" +
"6\022\t\n\005DRIVE\0207\022\010\n\004DOCS\0208\022\n\n\006SHEETS\0209\022\n\n\006SL" +
"IDES\020:\022\007\n\003IME\020;\022\010\n\004WARP\020<\022\022\n\016NFC_PROGRAM" +
"MER\020=\022\014\n\010NETSTATS\020>\022\r\n\tNEWSSTAND\020?\022\025\n\021KI" +
"DS_COMMUNICATOR\020@\022\022\n\016WIFI_ASSISTANT\020B\022\023\n",
"\017CAST_SENDER_SDK\020C\022\021\n\rCRONET_SOCIAL\020D\022\r\n" +
"\tPHENOTYPE\020E\022\026\n\022PHENOTYPE_COUNTERS\020F\022\020\n\014" +
"CHROME_INFRA\020G\022\r\n\tJUSTSPEAK\020H\022\020\n\014PERF_PR" +
"OFILE\020I\022\013\n\007KATNISS\020K\022\024\n\020SOCIAL_APPINVITE" +
"\020L\022\020\n\014GMM_COUNTERS\020M\022\022\n\016BOND_ONEGOOGLE\020N" +
"\022\014\n\010MAPS_API\020O\022\026\n\021CRONET_ANDROID_YT\020\304\001\022\026" +
"\n\022CRONET_ANDROID_GSA\020P\022\027\n\023GOOGLE_FIT_WEA" +
"RABLE\020Q\022\024\n\017FITNESS_ANDROID\020\251\001\022\025\n\020FITNESS" +
"_GMS_CORE\020\252\001\022\022\n\016GOOGLE_EXPRESS\020R\022\027\n\022GOOG" +
"LE_EXPRESS_DEV\020\327\001\022*\n%GOOGLE_EXPRESS_COUR",
"IER_ANDROID_PRIMES\020\344\001\022\"\n\035GOOGLE_EXPRESS_" +
"ANDROID_PRIMES\020\345\001\022+\n&GOOGLE_EXPRESS_STOR" +
"EOMS_ANDROID_PRIMES\020\360\001\022\t\n\005SENSE\020S\022\022\n\016AND" +
"ROID_BACKUP\020T\022\006\n\002VR\020U\022\020\n\014IME_COUNTERS\020V\022" +
"\020\n\014SETUP_WIZARD\020W\022\024\n\020EMERGENCY_ASSIST\020X\022" +
"\010\n\004TRON\020Y\022\021\n\rTRON_COUNTERS\020Z\022\021\n\rBATTERY_" +
"STATS\020[\022\016\n\nDISK_STATS\020\\\022\022\n\016GRAPHICS_STAT" +
"S\020k\022\016\n\nPROC_STATS\020]\022\r\n\010DROP_BOX\020\203\001\022\026\n\021FI" +
"NGERPRINT_STATS\020\265\001\022\027\n\022NOTIFICATION_STATS" +
"\020\266\001\022\025\n\021TAP_AND_PAY_GCORE\020^\022\016\n\nA11YLOGGER",
"\020_\022\020\n\014GCM_COUNTERS\020`\022\031\n\025PLACES_NO_GLS_CO" +
"NSENT\020a\022\027\n\023TACHYON_LOG_REQUEST\020b\022\024\n\020TACH" +
"YON_COUNTERS\020c\022\n\n\006VISION\020d\022\030\n\024SOCIAL_USE" +
"R_LOCATION\020e\022\022\n\016LAUNCHPAD_TOYS\020f\022\024\n\020META" +
"LOG_COUNTERS\020g\022\024\n\020MOBILESDK_CLIENT\020h\022\027\n\023" +
"ANDROID_VERIFY_APPS\020i\022\014\n\010ADSHIELD\020j\022\013\n\007S" +
"HERLOG\020l\022\023\n\017LE_ULR_COUNTERS\020m\022\013\n\007GMM_UE3" +
"\020n\022\014\n\010CALENDAR\020o\022\t\n\005ENDER\020p\022\022\n\016FAMILY_CO" +
"MPASS\020q\022\013\n\007TRANSOM\020r\022\024\n\020TRANSOM_COUNTERS" +
"\020s\022\t\n\005LB_AS\020t\022\n\n\006LB_CFG\020u\022\013\n\007IOS_GSA\020v\022\023",
"\n\017TAP_AND_PAY_APP\020w\022\035\n\030TAP_AND_PAY_APP_C" +
"OUNTERS\020\211\002\022\014\n\010FLYDROID\020x\022\016\n\nCPANEL_APP\020y" +
"\022\026\n\022ANDROID_SNET_GCORE\020z\022\025\n\021ANDROID_SNET" +
"_IDLE\020{\022\024\n\020ANDROID_SNET_JAR\020|\022\023\n\017CONTEXT" +
"_MANAGER\020}\022\r\n\tCLASSROOM\020~\022\016\n\nTAILORMADE\020" +
"\177\022\t\n\004KEEP\020\200\001\022\027\n\022GMM_BRIIM_COUNTERS\020\201\001\022\027\n" +
"\022CHROMECAST_APP_LOG\020\202\001\022\023\n\016ADWORDS_MOBILE" +
"\020\205\001\022\023\n\016LEANBACK_EVENT\020\206\001\022\022\n\rANDROID_GMAI" +
"L\020\207\001\022\017\n\nSAMPLE_SHM\020\210\001\022\031\n\024GPLUS_ANDROID_P" +
"RIMES\020\214\001\022 \n\033GPLUS_ANDROID_SYSTEM_HEALTH\020",
"\214\001\022\031\n\024GMAIL_ANDROID_PRIMES\020\226\001\022 \n\033GMAIL_A" +
"NDROID_SYSTEM_HEALTH\020\226\001\022\034\n\027CALENDAR_ANDR" +
"OID_PRIMES\020\227\001\022#\n\036CALENDAR_ANDROID_SYSTEM" +
"_HEALTH\020\227\001\022\030\n\023DOCS_ANDROID_PRIMES\020\230\001\022\037\n\032" +
"DOCS_ANDROID_SYSTEM_HEALTH\020\230\001\022\037\n\032YT_MAIN" +
"_APP_ANDROID_PRIMES\020\232\001\022\033\n\026YT_KIDS_ANDROI" +
"D_PRIMES\020\233\001\022\035\n\030YT_GAMING_ANDROID_PRIMES\020" +
"\234\001\022\034\n\027YT_MUSIC_ANDROID_PRIMES\020\235\001\022\033\n\026YT_L" +
"ITE_ANDROID_PRIMES\020\236\001\022\036\n\031YT_CREATOR_ANDR" +
"OID_PRIMES\020\253\001\022\027\n\022JAM_ANDROID_PRIMES\020\237\001\022\035",
"\n\030JAM_KIOSK_ANDROID_PRIMES\020\240\001\022\032\n\025PHOTOS_" +
"ANDROID_PRIMES\020\245\001\022\031\n\024DRIVE_ANDROID_PRIME" +
"S\020\246\001\022\032\n\025SHEETS_ANDROID_PRIMES\020\247\001\022\032\n\025SLID" +
"ES_ANDROID_PRIMES\020\250\001\022\034\n\027SNAPSEED_ANDROID" +
"_PRIMES\020\262\001\022\034\n\027HANGOUTS_ANDROID_PRIMES\020\263\001" +
"\022\031\n\024INBOX_ANDROID_PRIMES\020\264\001\022\025\n\020INBOX_IOS" +
"_PRIMES\020\206\002\022\023\n\016SDP_IOS_PRIMES\020\237\002\022\033\n\026GMSCO" +
"RE_ANDROID_PRIMES\020\301\001\022#\n\036PLAY_MUSIC_ANDRO" +
"ID_WEAR_PRIMES\020\310\001\022\034\n\027GEARHEAD_ANDROID_PR" +
"IMES\020\311\001\022\034\n\027INSTORE_CONSUMER_PRIMES\020\317\001\022\026\n",
"\021SAMPLE_IOS_PRIMES\020\312\001\022\032\n\025CPANEL_ANDROID_" +
"PRIMES\020\325\001\022\032\n\025HUDDLE_ANDROID_PRIMES\020\326\001\022\027\n" +
"\022AWX_ANDROID_PRIMES\020\336\001\022\027\n\022GHS_ANDROID_PR" +
"IMES\020\337\001\022\"\n\035ADWORDS_MOBILE_ANDROID_PRIMES" +
"\020\340\001\022\037\n\032TAP_AND_PAY_ANDROID_PRIMES\020\343\001\022\036\n\031" +
"WALLET_APP_ANDROID_PRIMES\020\350\001\022\032\n\025WALLET_A" +
"PP_IOS_PRIMES\020\351\001\022\035\n\030ANALYTICS_ANDROID_PR" +
"IMES\020\353\001\022\032\n\025SPACES_ANDROID_PRIMES\020\354\001\022\026\n\021S" +
"PACES_IOS_PRIMES\020\224\002\022\033\n\026SOCIETY_ANDROID_P" +
"RIMES\020\356\001\022\025\n\020GMM_BRIIM_PRIMES\020\357\001\022\016\n\tCW_PR",
"IMES\020\362\001\022\036\n\031FAMILYLINK_ANDROID_PRIMES\020\364\001\022" +
"\032\n\025FAMILYLINK_IOS_PRIMES\020\243\002\022\016\n\tWH_PRIMES" +
"\020\370\001\022\030\n\023NOVA_ANDROID_PRIMES\020\371\001\022!\n\034PHOTOS_" +
"DRAPER_ANDROID_PRIMES\020\375\001\022\017\n\nGMM_PRIMES\020\376" +
"\001\022\035\n\030TRANSLATE_ANDROID_PRIMES\020\377\001\022\031\n\024TRAN" +
"SLATE_IOS_PRIMES\020\200\002\022\034\n\027FLYDROID_ANDROID_" +
"PRIMES\020\203\002\022\026\n\021CONSUMERIQ_PRIMES\020\204\002\022\027\n\022GMB" +
"_ANDROID_PRIMES\020\207\002\022\024\n\017CLOUDDPC_PRIMES\020\260\002" +
"\022\030\n\023CLOUDDPC_ARC_PRIMES\020\261\002\022\n\n\005ICORE\020\211\001\022\031" +
"\n\024PANCETTA_MOBILE_HOST\020\212\001\022\"\n\035PANCETTA_MO",
"BILE_HOST_COUNTERS\020\213\001\022\034\n\027CROSSDEVICENOTI" +
"FICATION\020\215\001\022 \n\033CROSSDEVICENOTIFICATION_D" +
"EV\020\216\001\022\026\n\021MAPS_API_COUNTERS\020\217\001\022\010\n\003GPU\020\220\001\022" +
"\016\n\tON_THE_GO\020\221\001\022\027\n\022ON_THE_GO_COUNTERS\020\222\001" +
"\022!\n\034GMS_CORE_PEOPLE_AUTOCOMPLETE\020\223\001\022\026\n\021F" +
"LYDROID_COUNTERS\020\224\001\022\r\n\010FIREBALL\020\225\001\022\026\n\021FI" +
"REBALL_COUNTERS\020\201\002\022\024\n\017CRONET_FIREBALL\020\257\002" +
"\022\024\n\017FIREBALL_PRIMES\020\212\002\022\030\n\023FIREBALL_IOS_P" +
"RIMES\020\271\002\022,\n\'GOOGLE_HANDWRITING_INPUT_AND" +
"ROID_PRIMES\020\272\002\022\016\n\tPYROCLASM\020\231\001\022\031\n\024ANDROI",
"D_GSA_COUNTERS\020\241\001\022\024\n\017JAM_IMPRESSIONS\020\242\001\022" +
"\032\n\025JAM_KIOSK_IMPRESSIONS\020\243\001\022\021\n\014PAYMENTS_" +
"OCR\020\244\001\022\036\n\031UNICORN_FAMILY_MANAGEMENT\020\254\001\022\014" +
"\n\007AUDITOR\020\255\001\022\r\n\010NQLOOKUP\020\256\001\022%\n ANDROID_G" +
"SA_HIGH_PRIORITY_EVENTS\020\257\001\022\023\n\016ANDROID_DI" +
"ALER\020\260\001\022\022\n\rCLEARCUT_DEMO\020\261\001\022\017\n\nAPPMANAGE" +
"R\020\267\001\022\027\n\022SMARTLOCK_COUNTERS\020\270\001\022\026\n\021EXPEDIT" +
"IONS_GUIDE\020\271\001\022\t\n\004FUSE\020\272\001\022&\n!PIXEL_PERFEC" +
"T_CLIENT_STATE_LOGGER\020\273\001\022\034\n\027PLATFORM_STA" +
"TS_COUNTERS\020\274\001\022\021\n\014DRIVE_VIEWER\020\275\001\022\017\n\nPDF",
"_VIEWER\020\276\001\022\013\n\006BIGTOP\020\277\001\022\n\n\005VOICE\020\300\001\022\014\n\007M" +
"YFIBER\020\302\001\022\023\n\016RECORDED_PAGES\020\303\001\022\014\n\007MOB_DO" +
"G\020\305\001\022\017\n\nWALLET_APP\020\306\001\022\013\n\006GBOARD\020\307\001\022\017\n\nCR" +
"ONET_GMM\020\313\001\022\021\n\014TRUSTED_FACE\020\314\001\022\017\n\nMATCHS" +
"TICK\020\315\001\022\020\n\013APP_CATALOG\020\316\001\022\016\n\tBLUETOOTH\020\320" +
"\001\022\t\n\004WIFI\020\321\001\022\014\n\007TELECOM\020\322\001\022\016\n\tTELEPHONY\020" +
"\323\001\022\026\n\021IDENTITY_FRONTEND\020\324\001\022\013\n\006SESAME\020\330\001\022" +
"\034\n\027GOOGLE_KEYBOARD_CONTENT\020\331\001\022\013\n\006MADDEN\020" +
"\332\001\022\010\n\003INK\020\333\001\022\025\n\020ANDROID_CONTACTS\020\334\001\022\035\n\030G" +
"OOGLE_KEYBOARD_COUNTERS\020\335\001\022\024\n\017CLEARCUT_P",
"ROBER\020\341\001\022\025\n\020PLAY_CONSOLE_APP\020\342\001\022\034\n\027PLAY_" +
"CONSOLE_APP_PRIMES\020\210\002\022\r\n\010SPECTRUM\020\346\001\022\026\n\021" +
"SPECTRUM_COUNTERS\020\347\001\022\034\n\027SPECTRUM_ANDROID" +
"_PRIMES\020\213\002\022!\n\034IOS_SPOTLIGHT_SEARCH_LIBRA" +
"RY\020\352\001\022\014\n\007BOQ_WEB\020\361\001\022\031\n\024ORCHESTRATION_CLI" +
"ENT\020\365\001\022\035\n\030ORCHESTRATION_CLIENT_DEV\020\366\001\022\030\n" +
"\023GOOGLE_NOW_LAUNCHER\020\367\001\022\033\n\026SCOOBY_SPAM_R" +
"EPORT_LOG\020\372\001\022\017\n\nIOS_GROWTH\020\373\001\022\020\n\013APPS_NO" +
"TIFY\020\374\001\022\021\n\014SMARTKEY_APP\020\215\002\022\025\n\020CLINICAL_S" +
"TUDIES\020\216\002\022\033\n\026FITNESS_ANDROID_PRIMES\020\217\002\022\020",
"\n\013IMPROV_APPS\020\220\002\022\017\n\nFAMILYLINK\020\221\002\022\030\n\023FAM" +
"ILYLINK_COUNTERS\020\222\002\022\014\n\007SOCIETY\020\223\002\022\032\n\025DIA" +
"LER_ANDROID_PRIMES\020\225\002\022\031\n\024YOUTUBE_DIRECTO" +
"R_APP\020\226\002\022\033\n\026TACHYON_ANDROID_PRIMES\020\227\002\022\r\n" +
"\010DRIVE_FS\020\230\002\022\014\n\007YT_MAIN\020\231\002\022$\n\037WING_MARKE" +
"TPLACE_ANDROID_PRIMES\020\232\002\022\r\n\010DYNAMITE\020\233\002\022" +
"\026\n\021CORP_ANDROID_FOOD\020\234\002\022\035\n\030ANDROID_MESSA" +
"GING_PRIMES\020\235\002\022\025\n\020GPLUS_IOS_PRIMES\020\236\002\022\036\n" +
"\031CHROMECAST_ANDROID_PRIMES\020\240\002\022\021\n\014APPSTRE" +
"AMING\020\241\002\022\020\n\013GMB_ANDROID\020\242\002\022\025\n\020VOICE_IOS_",
"PRIMES\020\244\002\022\031\n\024VOICE_ANDROID_PRIMES\020\245\002\022\n\n\005" +
"PAISA\020\246\002\022\014\n\007GMB_IOS\020\247\002\022\022\n\rSCOOBY_EVENTS\020" +
"\250\002\022\030\n\023SNAPSEED_IOS_PRIMES\020\251\002\022 \n\033YOUTUBE_" +
"DIRECTOR_IOS_PRIMES\020\252\002\022\025\n\020WALLPAPER_PICK" +
"ER\020\253\002\022\n\n\005CHIME\020\254\002\022\021\n\014BEACON_GCORE\020\255\002\022\023\n\016" +
"ANDROID_STUDIO\020\256\002\022\021\n\014DOCS_OFFLINE\020\262\002\022\016\n\t" +
"FREIGHTER\020\263\002\022\024\n\017DOCS_IOS_PRIMES\020\264\002\022\026\n\021SL" +
"IDES_IOS_PRIMES\020\265\002\022\026\n\021SHEETS_IOS_PRIMES\020" +
"\266\002\022\023\n\016IPCONNECTIVITY\020\267\002\022\014\n\007CURATOR\020\270\002\032\002\020" +
"\001\"\221\001\n\rSchedulerType\022\025\n\021UNKNOWN_SCHEDULER",
"\020\000\022\010\n\004ASAP\020\001\022\024\n\020DEFAULT_PERIODIC\020\002\022\023\n\017QO" +
"S_FAST_ONEOFF\020\003\022\030\n\024QOS_DEFAULT_PERIODIC\020" +
"\004\022\032\n\026QOS_UNMETERED_PERIODIC\020\005*\t\010\350\007\020\200\200\200\200\002" +
"\"\354\001\n\014DeviceStatus\022\024\n\014is_unmetered\030\001 \001(\010\022" +
"\023\n\013is_charging\030\002 \001(\010\022S\n\020auto_time_status" +
"\030\003 \001(\01629.wireless_android_play_playlog.D" +
"eviceStatus.AutomaticTime\022\031\n\021is_googler_" +
"device\030\004 \001(\010\"A\n\rAutomaticTime\022\013\n\007UNKNOWN" +
"\020\000\022\021\n\rAUTO_TIME_OFF\020\001\022\020\n\014AUTO_TIME_ON\020\002\"" +
"G\n\021ExternalTimestamp\022\017\n\007time_ms\030\001 \001(\003\022\021\n",
"\tuptime_ms\030\002 \001(\003\022\016\n\006source\030\003 \001(\t\"\346\001\n\024Qos" +
"TierConfiguration\022\027\n\017log_source_name\030\001 \001" +
"(\t\022M\n\010qos_tier\030\002 \001(\0162;.wireless_android_" +
"play_playlog.QosTierConfiguration.QosTie" +
"r\"f\n\007QosTier\022\013\n\007DEFAULT\020\000\022\022\n\016UNMETERED_O" +
"NLY\020\001\022\026\n\022UNMETERED_OR_DAILY\020\002\022\027\n\023FAST_IF" +
"_RADIO_AWAKE\020\003\022\t\n\005NEVER\020\004\"\205\001\n\020QosTiersOv" +
"erride\022S\n\026qos_tier_configuration\030\001 \003(\01323" +
".wireless_android_play_playlog.QosTierCo" +
"nfiguration\022\034\n\024qos_tier_fingerprint\030\002 \001(",
"\003\"5\n\032LogSourceBatchingBlacklist\022\027\n\017log_s" +
"ource_name\030\001 \003(\t\"\236\002\n\013LogResponse\022$\n\030next" +
"_request_wait_millis\030\001 \001(\003:\002-1\022D\n\013experi" +
"ments\030\002 \001(\0132/.wireless_android_play_play" +
"log.ExperimentIdList\022A\n\010qos_tier\030\003 \001(\0132/" +
".wireless_android_play_playlog.QosTiersO" +
"verride\022`\n\035log_source_batching_blacklist" +
"\030\004 \001(\01329.wireless_android_play_playlog.L" +
"ogSourceBatchingBlacklistBA\n.com.google." +
"wireless.android.play.playlog.protoB\017Cli",
"entAnalytics"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_wireless_android_play_playlog_LogEventKeyValues_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_LogEventKeyValues_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_wireless_android_play_playlog_ActiveExperiments_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_ActiveExperiments_descriptor,
new java.lang.String[] { "ClientAlteringExperiment", "OtherExperiment", "GwsExperiment", "PlayExperiment", "UnsupportedPlayExperiment", });
internal_static_wireless_android_play_playlog_LogEvent_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_wireless_android_play_playlog_LogEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_LogEvent_descriptor,
new java.lang.String[] { "EventTimeMs", "EventUptimeMs", "SequencePosition", "Tag", "EventCode", "EventFlowId", "IsUserInitiated", "Value", "Store", "AppUsage1P", "SourceExtension", "SourceExtensionJs", "SourceExtensionJson", "Exp", "TestId", "TimezoneOffsetSeconds", "ExperimentIds", "ClientVe", "ClientVeJs", "InternalEvent", "TestCode", "BootCount", "NetworkConnectionInfo", });
internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_wireless_android_play_playlog_NetworkConnectionInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_NetworkConnectionInfo_descriptor,
new java.lang.String[] { "NetworkType", });
internal_static_wireless_android_play_playlog_ExperimentIds_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_wireless_android_play_playlog_ExperimentIds_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_ExperimentIds_descriptor,
new java.lang.String[] { "ClearBlob", "ClearBlobJs", "EncryptedBlob", "UsersMatch", });
internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_wireless_android_play_playlog_AndroidClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_AndroidClientInfo_descriptor,
new java.lang.String[] { "AndroidId", "LoggingId", "DeviceId", "SdkVersion", "Model", "Product", "Hardware", "Device", "OsBuild", "ApplicationBuild", "MccMnc", "Locale", "Country", "Manufacturer", "Brand", "Board", "RadioVersion", "Fingerprint", "GmsCoreVersionCode", "IsSidewinderDevice", "ChimeraConfigInfo", "UsingLogSourceInt", });
internal_static_wireless_android_play_playlog_BrowserInfo_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_wireless_android_play_playlog_BrowserInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_BrowserInfo_descriptor,
new java.lang.String[] { "Locale", "Browser", "BrowserVersion", "FlashVersion", });
internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_wireless_android_play_playlog_DesktopClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_DesktopClientInfo_descriptor,
new java.lang.String[] { "ClientId", "LoggingId", "Os", "OsMajorVersion", "OsFullVersion", "ApplicationBuild", "Country", });
internal_static_wireless_android_play_playlog_IosClientInfo_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_wireless_android_play_playlog_IosClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_IosClientInfo_descriptor,
new java.lang.String[] { "ClientId", "LoggingId", "OsMajorVersion", "OsFullVersion", "ApplicationBuild", "Country", "Model", "LanguageCode", });
internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_wireless_android_play_playlog_PancettaClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_PancettaClientInfo_descriptor,
new java.lang.String[] { "DeviceId", "Os", "AppId", "AppVersion", "MccMnc", });
internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_wireless_android_play_playlog_PlayCeClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_PlayCeClientInfo_descriptor,
new java.lang.String[] { "ClientId", "LoggingId", "Make", "Model", "ApplicationBuild", "PlatformVersion", "Country", });
internal_static_wireless_android_play_playlog_VrClientInfo_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_wireless_android_play_playlog_VrClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_VrClientInfo_descriptor,
new java.lang.String[] { "VrClientType", "SdkVersion", "Fingerprint", "GvrVersion", "Manufacturer", "Model", "Language", "Country", "UnityVersion", "UnitySdkVersion", });
internal_static_wireless_android_play_playlog_JsClientInfo_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_wireless_android_play_playlog_JsClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_JsClientInfo_descriptor,
new java.lang.String[] { "Os", "OsVersion", "DeviceType", "Country", "Locale", "OsType", });
internal_static_wireless_android_play_playlog_ClientInfo_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_wireless_android_play_playlog_ClientInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_ClientInfo_descriptor,
new java.lang.String[] { "ClientType", "RemoteHost", "RemoteHost6", "AndroidClientInfo", "DesktopClientInfo", "IosClientInfo", "PlayCeClientInfo", "VrClientInfo", "PancettaClientInfo", "BrowserInfo", "JsClientInfo", });
internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_wireless_android_play_playlog_ExperimentIdList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_ExperimentIdList_descriptor,
new java.lang.String[] { "Id", });
internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_AppUsage1pLogEvent_descriptor,
new java.lang.String[] { "AppType", "AndroidPackageName", "Version", });
internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_wireless_android_play_playlog_BatchedLogRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_BatchedLogRequest_descriptor,
new java.lang.String[] { "LogRequest", });
internal_static_wireless_android_play_playlog_LogRequest_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_wireless_android_play_playlog_LogRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_LogRequest_descriptor,
new java.lang.String[] { "RequestTimeMs", "RequestUptimeMs", "ClientInfo", "LogSource", "LogSourceName", "ZwiebackCookie", "LogEvent", "SerializedLogEvents", "QosTier", "Scheduler", "DeviceStatus", "ExternalTimestamp", });
internal_static_wireless_android_play_playlog_DeviceStatus_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_wireless_android_play_playlog_DeviceStatus_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_DeviceStatus_descriptor,
new java.lang.String[] { "IsUnmetered", "IsCharging", "AutoTimeStatus", "IsGooglerDevice", });
internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_wireless_android_play_playlog_ExternalTimestamp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_ExternalTimestamp_descriptor,
new java.lang.String[] { "TimeMs", "UptimeMs", "Source", });
internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_wireless_android_play_playlog_QosTierConfiguration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_QosTierConfiguration_descriptor,
new java.lang.String[] { "LogSourceName", "QosTier", });
internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_wireless_android_play_playlog_QosTiersOverride_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_QosTiersOverride_descriptor,
new java.lang.String[] { "QosTierConfiguration", "QosTierFingerprint", });
internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor =
getDescriptor().getMessageTypes().get(22);
internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_LogSourceBatchingBlacklist_descriptor,
new java.lang.String[] { "LogSourceName", });
internal_static_wireless_android_play_playlog_LogResponse_descriptor =
getDescriptor().getMessageTypes().get(23);
internal_static_wireless_android_play_playlog_LogResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_wireless_android_play_playlog_LogResponse_descriptor,
new java.lang.String[] { "NextRequestWaitMillis", "Experiments", "QosTier", "LogSourceBatchingBlacklist", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy