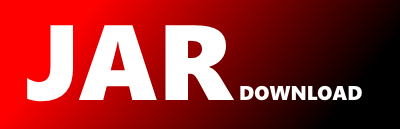
com.android.build.gradle.ndk.internal.ToolchainConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-experimental Show documentation
Show all versions of gradle-experimental Show documentation
Gradle plug-in to build Android applications.
/*
* Copyright (C) 2014 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.build.gradle.ndk.internal;
import com.android.build.gradle.internal.NdkHandler;
import com.android.build.gradle.internal.core.Abi;
import com.android.build.gradle.internal.core.Toolchain;
import com.android.build.gradle.managed.NdkAbiOptions;
import org.gradle.api.Action;
import org.gradle.internal.os.OperatingSystem;
import org.gradle.model.ModelMap;
import org.gradle.nativeplatform.platform.NativePlatform;
import org.gradle.nativeplatform.toolchain.Clang;
import org.gradle.nativeplatform.toolchain.Gcc;
import org.gradle.nativeplatform.toolchain.GccCompatibleToolChain;
import org.gradle.nativeplatform.toolchain.GccPlatformToolChain;
import org.gradle.nativeplatform.toolchain.NativeToolChainRegistry;
import org.gradle.nativeplatform.toolchain.internal.gcc.DefaultGccPlatformToolChain;
import org.gradle.platform.base.PlatformContainer;
import java.util.Collections;
import java.util.List;
/**
* Action to configure toolchain for native binaries.
*/
public class ToolchainConfiguration {
public static void configurePlatforms(PlatformContainer platforms, NdkHandler ndkHandler) {
for (Abi abi : ndkHandler.getSupportedAbis()) {
NativePlatform platform = platforms.maybeCreate(abi.getName(), NativePlatform.class);
// All we care is the name of the platform. It doesn't matter what the
// architecture is, but it must be set to non-x86 so that it does not match
// the default supported platform.
platform.architecture("ppc");
platform.operatingSystem("linux");
}
}
/**
* Configure toolchain for a platform.
*/
public static void configureToolchain(
NativeToolChainRegistry toolchainRegistry,
final String toolchainName,
final ModelMap abiConfigs,
final NdkHandler ndkHandler) {
final Toolchain ndkToolchain = Toolchain.getByName(toolchainName);
toolchainRegistry.create("ndk-" + toolchainName,
(Class extends GccCompatibleToolChain>)
(toolchainName.equals("gcc") ? Gcc.class : Clang.class),
new Action() {
@Override
public void execute(GccCompatibleToolChain toolchain) {
// Configure each platform.
for (final Abi abi : ndkHandler.getSupportedAbis()) {
toolchain.target(abi.getName(), new Action() {
@Override
public void execute(GccPlatformToolChain targetPlatform) {
// Disable usage of response file as clang do not handle file
// with \r\n properly.
if (OperatingSystem.current().isWindows()
&& toolchainName.equals("clang")) {
((DefaultGccPlatformToolChain) targetPlatform)
.setCanUseCommandFile(false);
}
if (Toolchain.GCC.equals(ndkToolchain)) {
String gccPrefix = abi.getGccExecutablePrefix();
targetPlatform.getcCompiler()
.setExecutable(gccPrefix + "-gcc");
targetPlatform.getCppCompiler()
.setExecutable(gccPrefix + "-g++");
targetPlatform.getLinker()
.setExecutable(gccPrefix + "-g++");
targetPlatform.getAssembler()
.setExecutable(gccPrefix + "-as");
targetPlatform.getStaticLibArchiver()
.setExecutable(gccPrefix + "-ar");
}
// By default, gradle will use -Xlinker to pass arguments to the linker.
// Removing it as it prevents -sysroot from being properly set.
targetPlatform.getLinker().withArguments(
new Action>() {
@Override
public void execute(List args) {
args.removeAll(Collections.singleton("-Xlinker"));
}
});
final NdkAbiOptions config = abiConfigs.get(abi.getName());
if (config != null) {
// Specify ABI specific flags.
targetPlatform.getcCompiler().withArguments(
new Action>() {
@Override
public void execute(List args) {
for (String flag : config.getCFlags()) {
args.add(flag);
}
}
});
targetPlatform.getCppCompiler().withArguments(
new Action>() {
@Override
public void execute(List args) {
for (String flag : config.getCppFlags()) {
args.add(flag);
}
}
});
targetPlatform.getLinker().withArguments(
new Action>() {
@Override
public void execute(List args) {
for (String flag : config.getLdFlags()) {
args.add(flag);
}
for (String lib : config.getLdLibs()) {
args.add("-l" + lib);
}
}
});
}
}
});
toolchain.path(ndkHandler.getCCompiler(abi).getParentFile());
}
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy