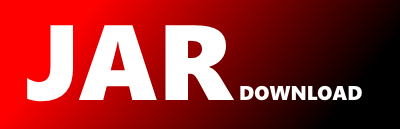
build.lombok.ast_generatedSource.lombok.ast.CompilationUnit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lombok-ast Show documentation
Show all versions of lombok-ast Show documentation
This is a very small fork of lombok.ast as some Android tools needed a few modifications. The normal repository for lombok.ast is here https://github.com/rzwitserloot/lombok.ast and our changes for 0.2.3 are in this pull request: https://github.com/rzwitserloot/lombok.ast/pull/8
The newest version!
//Generated by lombok.ast.template.TemplateProcessor. DO NOT EDIT, DO NOT CHECK IN!
package lombok.ast;
public class CompilationUnit extends lombok.ast.AbstractNode {
private lombok.ast.AbstractNode packageDeclaration = null;
lombok.ast.ListAccessor importDeclarations = ListAccessor.of(this, ImportDeclaration.class, "CompilationUnit.importDeclarations");
lombok.ast.ListAccessor typeDeclarations = ListAccessor.of(this, TypeDeclaration.class, "CompilationUnit.typeDeclarations");
public PackageDeclaration astPackageDeclaration() {
if (!(this.packageDeclaration instanceof PackageDeclaration)) return null;
return (PackageDeclaration) this.packageDeclaration;
}
public lombok.ast.CompilationUnit astPackageDeclaration(PackageDeclaration packageDeclaration) {
return this.rawPackageDeclaration(packageDeclaration);
}
public lombok.ast.Node rawPackageDeclaration() {
return this.packageDeclaration;
}
public lombok.ast.CompilationUnit rawPackageDeclaration(lombok.ast.Node packageDeclaration) {
if (packageDeclaration == this.packageDeclaration) return this;
if (packageDeclaration != null) this.adopt((lombok.ast.AbstractNode)packageDeclaration);
if (this.packageDeclaration != null) this.disown(this.packageDeclaration);
this.packageDeclaration = (lombok.ast.AbstractNode)packageDeclaration;
return this;
}
public lombok.ast.RawListAccessor rawImportDeclarations() {
return this.importDeclarations.asRaw();
}
public lombok.ast.StrictListAccessor astImportDeclarations() {
return this.importDeclarations.asStrict();
}
public lombok.ast.RawListAccessor rawTypeDeclarations() {
return this.typeDeclarations.asRaw();
}
public lombok.ast.StrictListAccessor astTypeDeclarations() {
return this.typeDeclarations.asStrict();
}
@java.lang.Override public java.util.List getChildren() {
java.util.List result = new java.util.ArrayList();
if (this.packageDeclaration != null) result.add(this.packageDeclaration);
result.addAll(this.importDeclarations.backingList());
result.addAll(this.typeDeclarations.backingList());
return result;
}
@java.lang.Override public boolean replaceChild(Node original, Node replacement) throws lombok.ast.AstException {
if (this.packageDeclaration == original) {
this.rawPackageDeclaration(replacement);
return true;
}
if (this.rawImportDeclarations().replace(original, replacement)) return true;
if (this.rawTypeDeclarations().replace(original, replacement)) return true;
return false;
}
@java.lang.Override public boolean detach(Node child) {
if (this.packageDeclaration == child) {
this.disown((AbstractNode) child);
this.packageDeclaration = null;
return true;
}
if (this.rawImportDeclarations().remove(child)) return true;
if (this.rawTypeDeclarations().remove(child)) return true;
return false;
}
@java.lang.Override public void accept(lombok.ast.AstVisitor visitor) {
if (visitor.visitCompilationUnit(this)) return;
if (this.packageDeclaration != null) this.packageDeclaration.accept(visitor);
for (lombok.ast.Node child : this.importDeclarations.asIterable()) {
child.accept(visitor);
}
for (lombok.ast.Node child : this.typeDeclarations.asIterable()) {
child.accept(visitor);
}
visitor.afterVisitCompilationUnit(this);
visitor.endVisit(this);
}
@java.lang.Override public CompilationUnit copy() {
CompilationUnit result = new CompilationUnit();
if (this.packageDeclaration != null) result.rawPackageDeclaration(this.packageDeclaration.copy());
for (Node n : this.importDeclarations.backingList()) {
result.rawImportDeclarations().addToEnd(n == null ? null : n.copy());
}
for (Node n : this.typeDeclarations.backingList()) {
result.rawTypeDeclarations().addToEnd(n == null ? null : n.copy());
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy