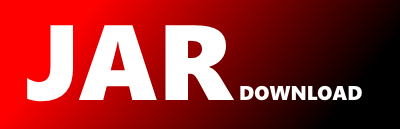
build.lombok.ast_generatedSource.lombok.ast.InterfaceDeclaration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lombok-ast Show documentation
Show all versions of lombok-ast Show documentation
This is a very small fork of lombok.ast as some Android tools needed a few modifications. The normal repository for lombok.ast is here https://github.com/rzwitserloot/lombok.ast and our changes for 0.2.3 are in this pull request: https://github.com/rzwitserloot/lombok.ast/pull/8
The newest version!
//Generated by lombok.ast.template.TemplateProcessor. DO NOT EDIT, DO NOT CHECK IN!
package lombok.ast;
public class InterfaceDeclaration extends lombok.ast.AbstractNode implements lombok.ast.TypeMember, lombok.ast.Statement, lombok.ast.TypeDeclaration, lombok.ast.JavadocContainer {
private lombok.ast.AbstractNode javadoc = null;
private lombok.ast.AbstractNode modifiers = adopt(new lombok.ast.Modifiers());
private lombok.ast.AbstractNode name = adopt(new lombok.ast.Identifier());
lombok.ast.ListAccessor typeVariables = ListAccessor.of(this, TypeVariable.class, "InterfaceDeclaration.typeVariables");
lombok.ast.ListAccessor extending = ListAccessor.of(this, TypeReference.class, "InterfaceDeclaration.extending");
private lombok.ast.AbstractNode body = null;
public TypeBody upToTypeBody() {
if (!(this.getParent() instanceof TypeBody)) return null;
TypeBody out = (TypeBody)this.getParent();
if (!out.rawMembers().contains(this)) return null;
return out;
}
public Block upToBlock() {
if (!(this.getParent() instanceof Block)) return null;
Block out = (Block)this.getParent();
if (!out.rawContents().contains(this)) return null;
return out;
}
public CompilationUnit upIfTopLevelToCompilationUnit() {
if (!(this.getParent() instanceof CompilationUnit)) return null;
CompilationUnit out = (CompilationUnit)this.getParent();
if (!out.rawTypeDeclarations().contains(this)) return null;
return out;
}
public Comment astJavadoc() {
if (!(this.javadoc instanceof Comment)) return null;
return (Comment) this.javadoc;
}
public lombok.ast.InterfaceDeclaration astJavadoc(Comment javadoc) {
return this.rawJavadoc(javadoc);
}
public lombok.ast.Node rawJavadoc() {
return this.javadoc;
}
public lombok.ast.InterfaceDeclaration rawJavadoc(lombok.ast.Node javadoc) {
if (javadoc == this.javadoc) return this;
if (javadoc != null) this.adopt((lombok.ast.AbstractNode)javadoc);
if (this.javadoc != null) this.disown(this.javadoc);
this.javadoc = (lombok.ast.AbstractNode)javadoc;
return this;
}
public Modifiers astModifiers() {
if (!(this.modifiers instanceof Modifiers)) return null;
return (Modifiers) this.modifiers;
}
public lombok.ast.InterfaceDeclaration astModifiers(Modifiers modifiers) {
return this.rawModifiers(modifiers);
}
private lombok.ast.InterfaceDeclaration rawModifiers(lombok.ast.Node modifiers) {
if (modifiers == this.modifiers) return this;
if (modifiers != null) this.adopt((lombok.ast.AbstractNode)modifiers);
if (this.modifiers != null) this.disown(this.modifiers);
this.modifiers = (lombok.ast.AbstractNode)modifiers;
return this;
}
public Identifier astName() {
if (!(this.name instanceof Identifier)) return null;
return (Identifier) this.name;
}
public lombok.ast.InterfaceDeclaration astName(Identifier name) {
return this.rawName(name);
}
private lombok.ast.InterfaceDeclaration rawName(lombok.ast.Node name) {
if (name == this.name) return this;
if (name != null) this.adopt((lombok.ast.AbstractNode)name);
if (this.name != null) this.disown(this.name);
this.name = (lombok.ast.AbstractNode)name;
return this;
}
public lombok.ast.RawListAccessor rawTypeVariables() {
return this.typeVariables.asRaw();
}
public lombok.ast.StrictListAccessor astTypeVariables() {
return this.typeVariables.asStrict();
}
public lombok.ast.RawListAccessor rawExtending() {
return this.extending.asRaw();
}
public lombok.ast.StrictListAccessor astExtending() {
return this.extending.asStrict();
}
public NormalTypeBody astBody() {
if (!(this.body instanceof NormalTypeBody)) return null;
return (NormalTypeBody) this.body;
}
public lombok.ast.InterfaceDeclaration astBody(NormalTypeBody body) {
if (body == null) throw new java.lang.NullPointerException("body is mandatory");
return this.rawBody(body);
}
public lombok.ast.Node rawBody() {
return this.body;
}
public lombok.ast.InterfaceDeclaration rawBody(lombok.ast.Node body) {
if (body == this.body) return this;
if (body != null) this.adopt((lombok.ast.AbstractNode)body);
if (this.body != null) this.disown(this.body);
this.body = (lombok.ast.AbstractNode)body;
return this;
}
@java.lang.Override public java.util.List getChildren() {
java.util.List result = new java.util.ArrayList();
if (this.javadoc != null) result.add(this.javadoc);
if (this.modifiers != null) result.add(this.modifiers);
if (this.name != null) result.add(this.name);
result.addAll(this.typeVariables.backingList());
result.addAll(this.extending.backingList());
if (this.body != null) result.add(this.body);
return result;
}
@java.lang.Override public boolean replaceChild(Node original, Node replacement) throws lombok.ast.AstException {
if (this.javadoc == original) {
this.rawJavadoc(replacement);
return true;
}
if (this.modifiers == original) {
if (replacement instanceof Modifiers) {
this.astModifiers((Modifiers) replacement);
return true;
} else throw new lombok.ast.AstException(this, String.format(
"Cannot replace node: replacement must be of type %s but is of type %s",
"Modifiers", replacement == null ? "null" : replacement.getClass().getName()));
}
if (this.name == original) {
if (replacement instanceof Identifier) {
this.astName((Identifier) replacement);
return true;
} else throw new lombok.ast.AstException(this, String.format(
"Cannot replace node: replacement must be of type %s but is of type %s",
"Identifier", replacement == null ? "null" : replacement.getClass().getName()));
}
if (this.rawTypeVariables().replace(original, replacement)) return true;
if (this.rawExtending().replace(original, replacement)) return true;
if (this.body == original) {
this.rawBody(replacement);
return true;
}
return false;
}
@java.lang.Override public boolean detach(Node child) {
if (this.javadoc == child) {
this.disown((AbstractNode) child);
this.javadoc = null;
return true;
}
if (this.modifiers == child) {
this.disown((AbstractNode) child);
this.modifiers = null;
return true;
}
if (this.name == child) {
this.disown((AbstractNode) child);
this.name = null;
return true;
}
if (this.rawTypeVariables().remove(child)) return true;
if (this.rawExtending().remove(child)) return true;
if (this.body == child) {
this.disown((AbstractNode) child);
this.body = null;
return true;
}
return false;
}
@java.lang.Override public void accept(lombok.ast.AstVisitor visitor) {
if (visitor.visitInterfaceDeclaration(this)) return;
if (this.javadoc != null) this.javadoc.accept(visitor);
if (this.modifiers != null) this.modifiers.accept(visitor);
if (this.name != null) this.name.accept(visitor);
for (lombok.ast.Node child : this.typeVariables.asIterable()) {
child.accept(visitor);
}
for (lombok.ast.Node child : this.extending.asIterable()) {
child.accept(visitor);
}
if (this.body != null) this.body.accept(visitor);
visitor.afterVisitInterfaceDeclaration(this);
visitor.endVisit(this);
}
@java.lang.Override public InterfaceDeclaration copy() {
InterfaceDeclaration result = new InterfaceDeclaration();
if (this.javadoc != null) result.rawJavadoc(this.javadoc.copy());
if (this.modifiers != null) result.rawModifiers(this.modifiers.copy());
if (this.name != null) result.rawName(this.name.copy());
for (Node n : this.typeVariables.backingList()) {
result.rawTypeVariables().addToEnd(n == null ? null : n.copy());
}
for (Node n : this.extending.backingList()) {
result.rawExtending().addToEnd(n == null ? null : n.copy());
}
if (this.body != null) result.rawBody(this.body.copy());
return result;
}
public java.lang.String getDescription() {
return lombok.ast.InterfaceDeclarationTemplate.getDescription(this);
}
public boolean isInterface() {
return lombok.ast.InterfaceDeclarationTemplate.isInterface(this);
}
public lombok.ast.TypeDeclaration upUpToTypeDeclaration() {
return lombok.ast.TypeMemberMixin.upUpToTypeDeclaration(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy