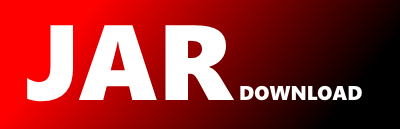
build.lombok.ast_generatedSource.lombok.ast.Modifiers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lombok-ast Show documentation
Show all versions of lombok-ast Show documentation
This is a very small fork of lombok.ast as some Android tools needed a few modifications. The normal repository for lombok.ast is here https://github.com/rzwitserloot/lombok.ast and our changes for 0.2.3 are in this pull request: https://github.com/rzwitserloot/lombok.ast/pull/8
The newest version!
//Generated by lombok.ast.template.TemplateProcessor. DO NOT EDIT, DO NOT CHECK IN!
package lombok.ast;
public class Modifiers extends lombok.ast.AbstractNode {
lombok.ast.ListAccessor annotations = ListAccessor.of(this, Annotation.class, "Modifiers.annotations");
lombok.ast.ListAccessor keywords = ListAccessor.of(this, KeywordModifier.class, "Modifiers.keywords");
public InterfaceDeclaration upToInterfaceDeclaration() {
if (!(this.getParent() instanceof InterfaceDeclaration)) return null;
InterfaceDeclaration out = (InterfaceDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public TypeDeclaration upToTypeDeclaration() {
if (!(this.getParent() instanceof TypeDeclaration)) return null;
TypeDeclaration out = (TypeDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public AnnotationDeclaration upToAnnotationDeclaration() {
if (!(this.getParent() instanceof AnnotationDeclaration)) return null;
AnnotationDeclaration out = (AnnotationDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public ClassDeclaration upToClassDeclaration() {
if (!(this.getParent() instanceof ClassDeclaration)) return null;
ClassDeclaration out = (ClassDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public MethodDeclaration upToMethodDeclaration() {
if (!(this.getParent() instanceof MethodDeclaration)) return null;
MethodDeclaration out = (MethodDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public VariableDefinition upToVariableDefinition() {
if (!(this.getParent() instanceof VariableDefinition)) return null;
VariableDefinition out = (VariableDefinition)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public EnumDeclaration upToEnumDeclaration() {
if (!(this.getParent() instanceof EnumDeclaration)) return null;
EnumDeclaration out = (EnumDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public ConstructorDeclaration upToConstructorDeclaration() {
if (!(this.getParent() instanceof ConstructorDeclaration)) return null;
ConstructorDeclaration out = (ConstructorDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public AnnotationMethodDeclaration upToAnnotationMethodDeclaration() {
if (!(this.getParent() instanceof AnnotationMethodDeclaration)) return null;
AnnotationMethodDeclaration out = (AnnotationMethodDeclaration)this.getParent();
if (out.astModifiers() != this) return null;
return out;
}
public lombok.ast.RawListAccessor rawAnnotations() {
return this.annotations.asRaw();
}
public lombok.ast.StrictListAccessor astAnnotations() {
return this.annotations.asStrict();
}
public lombok.ast.RawListAccessor rawKeywords() {
return this.keywords.asRaw();
}
public lombok.ast.StrictListAccessor astKeywords() {
return this.keywords.asStrict();
}
@java.lang.Override public java.util.List getChildren() {
java.util.List result = new java.util.ArrayList();
result.addAll(this.annotations.backingList());
result.addAll(this.keywords.backingList());
return result;
}
@java.lang.Override public boolean replaceChild(Node original, Node replacement) throws lombok.ast.AstException {
if (this.rawAnnotations().replace(original, replacement)) return true;
if (this.rawKeywords().replace(original, replacement)) return true;
return false;
}
@java.lang.Override public boolean detach(Node child) {
if (this.rawAnnotations().remove(child)) return true;
if (this.rawKeywords().remove(child)) return true;
return false;
}
@java.lang.Override public void accept(lombok.ast.AstVisitor visitor) {
if (visitor.visitModifiers(this)) return;
for (lombok.ast.Node child : this.annotations.asIterable()) {
child.accept(visitor);
}
for (lombok.ast.Node child : this.keywords.asIterable()) {
child.accept(visitor);
}
visitor.afterVisitModifiers(this);
visitor.endVisit(this);
}
@java.lang.Override public Modifiers copy() {
Modifiers result = new Modifiers();
for (Node n : this.annotations.backingList()) {
result.rawAnnotations().addToEnd(n == null ? null : n.copy());
}
for (Node n : this.keywords.backingList()) {
result.rawKeywords().addToEnd(n == null ? null : n.copy());
}
return result;
}
/**
* Returns the keyword-based modifiers the way {@link java.lang.reflect.Modifiers} works.
* Only those keywords that are explicitly in the AST are reported; to also include implicit flags, such
* as for example the idea that methods in interfaces are always public and abstract even if not marked as such,
* use {@link #getEffectiveModifierFlags()}.
*/
public int getExplicitModifierFlags() {
return lombok.ast.ModifiersTemplate.getExplicitModifierFlags(this);
}
/**
* Returns the keyword-based modifiers the way {@link java.lang.reflect.Modifiers} works. Also sets flags that are implicitly true due to the nature
* of the node that the modifiers are attached to (for example, inner interfaces are implicitly static and thus if the Modifiers object is a child of
* such a declaration, its static bit will be set. Similarly, method declarations in interfaces are abstract and public whether or not those keywords
* have been applied to the node).
*/
public int getEffectiveModifierFlags() {
return lombok.ast.ModifiersTemplate.getEffectiveModifierFlags(this);
}
public boolean isPublic() {
return lombok.ast.ModifiersTemplate.isPublic(this);
}
public boolean isProtected() {
return lombok.ast.ModifiersTemplate.isProtected(this);
}
public boolean isPrivate() {
return lombok.ast.ModifiersTemplate.isPrivate(this);
}
public boolean isPackagePrivate() {
return lombok.ast.ModifiersTemplate.isPackagePrivate(this);
}
public boolean isStatic() {
return lombok.ast.ModifiersTemplate.isStatic(this);
}
public boolean isFinal() {
return lombok.ast.ModifiersTemplate.isFinal(this);
}
public boolean isAbstract() {
return lombok.ast.ModifiersTemplate.isAbstract(this);
}
public boolean isTransient() {
return lombok.ast.ModifiersTemplate.isTransient(this);
}
public boolean isEmpty() {
return lombok.ast.ModifiersTemplate.isEmpty(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy