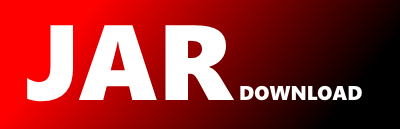
build.lombok.ast_generatedSource.lombok.ast.VariableDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lombok-ast Show documentation
Show all versions of lombok-ast Show documentation
This is a very small fork of lombok.ast as some Android tools needed a few modifications. The normal repository for lombok.ast is here https://github.com/rzwitserloot/lombok.ast and our changes for 0.2.3 are in this pull request: https://github.com/rzwitserloot/lombok.ast/pull/8
The newest version!
//Generated by lombok.ast.template.TemplateProcessor. DO NOT EDIT, DO NOT CHECK IN!
package lombok.ast;
public class VariableDefinition extends lombok.ast.AbstractNode {
private lombok.ast.AbstractNode modifiers = adopt(new lombok.ast.Modifiers());
private lombok.ast.AbstractNode typeReference = null;
private boolean varargs = false;
lombok.ast.ListAccessor variables = ListAccessor.of(this, VariableDefinitionEntry.class, "VariableDefinition.variables");
public Catch upToCatch() {
if (!(this.getParent() instanceof Catch)) return null;
Catch out = (Catch)this.getParent();
if (out.rawExceptionDeclaration() != this) return null;
return out;
}
public ConstructorDeclaration upIfParameterToConstructorDeclaration() {
if (!(this.getParent() instanceof ConstructorDeclaration)) return null;
ConstructorDeclaration out = (ConstructorDeclaration)this.getParent();
if (!out.rawParameters().contains(this)) return null;
return out;
}
public MethodDeclaration upIfParameterToMethodDeclaration() {
if (!(this.getParent() instanceof MethodDeclaration)) return null;
MethodDeclaration out = (MethodDeclaration)this.getParent();
if (!out.rawParameters().contains(this)) return null;
return out;
}
public VariableDeclaration upToVariableDeclaration() {
if (!(this.getParent() instanceof VariableDeclaration)) return null;
VariableDeclaration out = (VariableDeclaration)this.getParent();
if (out.rawDefinition() != this) return null;
return out;
}
public For upToFor() {
if (!(this.getParent() instanceof For)) return null;
For out = (For)this.getParent();
if (out.rawVariableDeclaration() != this) return null;
return out;
}
public ForEach upToForEach() {
if (!(this.getParent() instanceof ForEach)) return null;
ForEach out = (ForEach)this.getParent();
if (out.rawVariable() != this) return null;
return out;
}
public Modifiers astModifiers() {
if (!(this.modifiers instanceof Modifiers)) return null;
return (Modifiers) this.modifiers;
}
public lombok.ast.VariableDefinition astModifiers(Modifiers modifiers) {
return this.rawModifiers(modifiers);
}
private lombok.ast.VariableDefinition rawModifiers(lombok.ast.Node modifiers) {
if (modifiers == this.modifiers) return this;
if (modifiers != null) this.adopt((lombok.ast.AbstractNode)modifiers);
if (this.modifiers != null) this.disown(this.modifiers);
this.modifiers = (lombok.ast.AbstractNode)modifiers;
return this;
}
public TypeReference astTypeReference() {
if (!(this.typeReference instanceof TypeReference)) return null;
return (TypeReference) this.typeReference;
}
public lombok.ast.VariableDefinition astTypeReference(TypeReference typeReference) {
if (typeReference == null) throw new java.lang.NullPointerException("typeReference is mandatory");
return this.rawTypeReference(typeReference);
}
public lombok.ast.Node rawTypeReference() {
return this.typeReference;
}
public lombok.ast.VariableDefinition rawTypeReference(lombok.ast.Node typeReference) {
if (typeReference == this.typeReference) return this;
if (typeReference != null) this.adopt((lombok.ast.AbstractNode)typeReference);
if (this.typeReference != null) this.disown(this.typeReference);
this.typeReference = (lombok.ast.AbstractNode)typeReference;
return this;
}
public boolean astVarargs() {
return this.varargs;
}
public lombok.ast.VariableDefinition astVarargs(boolean varargs) {
this.varargs = varargs;
return this;
}
public lombok.ast.RawListAccessor rawVariables() {
return this.variables.asRaw();
}
public lombok.ast.StrictListAccessor astVariables() {
return this.variables.asStrict();
}
@java.lang.Override public java.util.List getChildren() {
java.util.List result = new java.util.ArrayList();
if (this.modifiers != null) result.add(this.modifiers);
if (this.typeReference != null) result.add(this.typeReference);
result.addAll(this.variables.backingList());
return result;
}
@java.lang.Override public boolean replaceChild(Node original, Node replacement) throws lombok.ast.AstException {
if (this.modifiers == original) {
if (replacement instanceof Modifiers) {
this.astModifiers((Modifiers) replacement);
return true;
} else throw new lombok.ast.AstException(this, String.format(
"Cannot replace node: replacement must be of type %s but is of type %s",
"Modifiers", replacement == null ? "null" : replacement.getClass().getName()));
}
if (this.typeReference == original) {
this.rawTypeReference(replacement);
return true;
}
if (this.rawVariables().replace(original, replacement)) return true;
return false;
}
@java.lang.Override public boolean detach(Node child) {
if (this.modifiers == child) {
this.disown((AbstractNode) child);
this.modifiers = null;
return true;
}
if (this.typeReference == child) {
this.disown((AbstractNode) child);
this.typeReference = null;
return true;
}
if (this.rawVariables().remove(child)) return true;
return false;
}
@java.lang.Override public void accept(lombok.ast.AstVisitor visitor) {
if (visitor.visitVariableDefinition(this)) return;
if (this.modifiers != null) this.modifiers.accept(visitor);
if (this.typeReference != null) this.typeReference.accept(visitor);
for (lombok.ast.Node child : this.variables.asIterable()) {
child.accept(visitor);
}
visitor.afterVisitVariableDefinition(this);
visitor.endVisit(this);
}
@java.lang.Override public VariableDefinition copy() {
VariableDefinition result = new VariableDefinition();
if (this.modifiers != null) result.rawModifiers(this.modifiers.copy());
if (this.typeReference != null) result.rawTypeReference(this.typeReference.copy());
result.varargs = this.varargs;
for (Node n : this.variables.backingList()) {
result.rawVariables().addToEnd(n == null ? null : n.copy());
}
return result;
}
public lombok.ast.TypeDeclaration upUpIfFieldToTypeDeclaration() {
return lombok.ast.VariableDefinitionTemplate.upUpIfFieldToTypeDeclaration(this);
}
public Block upUpIfLocalVariableToBlock() {
return lombok.ast.VariableDefinitionTemplate.upUpIfLocalVariableToBlock(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy