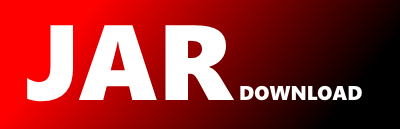
com.android.tools.lint.LintCliXmlParser Maven / Gradle / Ivy
/*
* Copyright (C) 2011 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.tools.lint;
import com.android.annotations.NonNull;
import com.android.annotations.Nullable;
import com.android.tools.lint.client.api.IDomParser;
import com.android.tools.lint.client.api.IssueRegistry;
import com.android.tools.lint.detector.api.Location;
import com.android.tools.lint.detector.api.Location.Handle;
import com.android.tools.lint.detector.api.XmlContext;
import com.android.utils.PositionXmlParser;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.xml.sax.SAXException;
import java.io.File;
import java.io.UnsupportedEncodingException;
/**
* A customization of the {@link PositionXmlParser} which creates position
* objects that directly extend the lint
* {@link com.android.tools.lint.detector.api.Position} class.
*
* It also catches and reports parser errors as lint errors.
*/
public class LintCliXmlParser extends PositionXmlParser implements IDomParser {
@Override
public Document parseXml(@NonNull XmlContext context) {
String xml = null;
try {
// Do we need to provide an input stream for encoding?
xml = context.getContents();
if (xml != null) {
return super.parse(xml);
}
} catch (UnsupportedEncodingException e) {
context.report(
// Must provide an issue since API guarantees that the issue parameter
// is valid
IssueRegistry.PARSER_ERROR, Location.create(context.file),
e.getCause() != null ? e.getCause().getLocalizedMessage() :
e.getLocalizedMessage(),
null);
} catch (SAXException e) {
Location location = Location.create(context.file);
String message = e.getCause() != null ? e.getCause().getLocalizedMessage() :
e.getLocalizedMessage();
if (message.startsWith("The processing instruction target matching "
+ "\"[xX][mM][lL]\" is not allowed.")) {
int prologue = xml.indexOf("