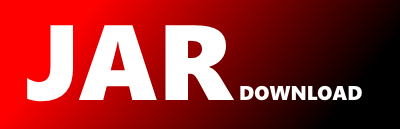
com.anji.plus.gaea.oss.ossbuilder.builders.NFSClient Maven / Gradle / Ivy
package com.anji.plus.gaea.oss.ossbuilder.builders;
import com.anji.plus.gaea.oss.config.OSSNFSProperties;
import com.anji.plus.gaea.oss.config.OSSProperties;
import com.anji.plus.gaea.oss.exceptions.GaeaOSSException;
import com.anji.plus.gaea.oss.exceptions.GaeaOSSExceptionBuilder;
import com.anji.plus.gaea.oss.ossbuilder.GaeaOSSTemplate;
import org.apache.commons.io.FileUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import org.springframework.web.multipart.MultipartFile;
import java.util.List;
/**
* 文件存储 使用服务器本地文件夹
* @Author: lide
* @since 2022/3/22 14:16
*/
public class NFSClient implements GaeaOSSTemplate {
private static Logger logger = LoggerFactory.getLogger(NFSClient.class);
private String nfsLocalStore = "/app/disk/upload";
// 允许的文件后缀 白名单
private String fileTypeWhileList;
@Override
public String getFileTypeWhileList() {
return fileTypeWhileList;
}
public NFSClient(OSSProperties ossProperties){
this.fileTypeWhileList = ossProperties.getFileTypeWhileList();
if (ossProperties.getNfs() != null && !StringUtils.isEmpty(ossProperties.getNfs().getPath())) {
this.nfsLocalStore = ossProperties.getNfs().getPath();
}
if (!StringUtils.endsWithIgnoreCase(this.nfsLocalStore, java.io.File.separator)) {
this.nfsLocalStore = this.nfsLocalStore + java.io.File.separator;
}
java.io.File localDir = new java.io.File(this.nfsLocalStore);
if (!localDir.exists()) {
localDir.mkdirs();
}
logger.info("初始化文件存储,激活服务器本地文件存储,路径{}", this.nfsLocalStore);
}
@Override
public String uploadFileByInputStream(MultipartFile file, String fileObjectName) throws GaeaOSSException {
//判断文件后缀名是否在白名单中,如果不在报异常,中止文件保存
checkFileSuffixName(file);
java.io.File objectFile = null;
try {
// 本地文件保存路径
String filePath = nfsLocalStore + fileObjectName;
objectFile = new java.io.File(filePath);
file.transferTo(objectFile);
} catch (Exception e) {
logger.error("save file to local store error:", e);
throw GaeaOSSExceptionBuilder.build("save file to local store error", e);
} finally {
objectFile = null;
}
return fileObjectName;
}
@Override
public byte[] downloadFile(String fileObjectName) throws GaeaOSSException {
byte[] fileBytes = null;
java.io.File objectFile = null;
try {
// 本地文件保存路径
String filePath = nfsLocalStore + fileObjectName;
objectFile = new java.io.File(filePath);
fileBytes = FileUtils.readFileToByteArray(objectFile);
} catch (Exception e) {
logger.error("read file from local store error:", e);
throw GaeaOSSExceptionBuilder.build("read file from local store error, objectName="+ fileObjectName);
} finally {
objectFile = null;
}
return fileBytes;
}
@Override
public void deleteFile(String fileObjectName) {
try{
// 本地文件保存路径
String filePath = nfsLocalStore + fileObjectName;
java.io.File file = new java.io.File(filePath);
if (file.exists()) {
file.delete();
}
}catch (Exception e){
e.printStackTrace();
}
}
@Override
public void deleteFiles(List fileObjectNames) {
if(CollectionUtils.isEmpty(fileObjectNames)){
return;
}
fileObjectNames.stream().forEach(fileObjectName -> {
this.deleteFile(fileObjectName);
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy