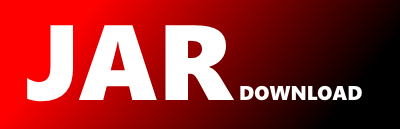
com.anji.plus.gaea.push.utils.TemplateAnalysisUtil Maven / Gradle / Ivy
package com.anji.plus.gaea.push.utils;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.anji.plus.gaea.push.domain.common.SmsTemplateAccount;
import com.anji.plus.gaea.push.domain.po.TemplatePO;
import com.anji.plus.gaea.push.domain.vo.TemplateVO;
import com.anji.plus.gaea.push.domain.common.TParam;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
*
* 工具类
*
*
* @author anji gaea teams
* @since 2020-10-16
*/
@Slf4j
public class TemplateAnalysisUtil {
private static String htmlHead = "Document ";
private static String htmlEnd = "";
private static String[] keyWord = new String[]{"javascript", "vbscript", "expression", "applet", "meta", "xml", "blink", "link", "script", "embed", "object", "iframe", "frame", "frameset", "ilayer", "layer", "bgsound", "title", "base", "onabort", "onactivate", "onafterprint", "onafterupdate", "onbeforeactivate", "onbeforecopy", "onbeforecut", "onbeforedeactivate", "onbeforeeditfocus", "onbeforepaste", "onbeforeprint", "onbeforeunload", "onbeforeupdate", "onblur", "onbounce", "oncellchange", "onchange", "onclick", "oncontextmenu", "oncontrolselect", "oncopy", "oncut", "ondataavailable", "ondatasetchanged", "ondatasetcomplete", "ondblclick", "ondeactivate", "ondrag", "ondragend", "ondragenter", "ondragleave", "ondragover", "ondragstart", "ondrop", "onerror", "onerrorupdate", "onfilterchange", "onfinish", "onfocus", "onfocusin", "onfocusout", "onhelp", "onkeydown", "onkeypress", "onkeyup", "onlayoutcomplete", "onload", "onlosecapture", "onmousedown", "onmouseenter", "onmouseleave", "onmousemove", "onmouseout", "onmouseover", "onmouseup", "onmousewheel", "onmove", "onmoveend", "onmovestart", "onpaste", "onpropertychange", "onreadystatechange", "onreset", "onresize", "onresizeend", "onresizestart", "onrowenter", "onrowexit", "onrowsdelete", "onrowsinserted", "onscroll", "onselect", "onselectionchange", "onselectstart", "onstart", "onstop", "onsubmit", "onunload"};
static Pattern param = Pattern.compile("\\{\\s*[a-zA-Z0-9]+\\s*((\\.|\\:)\\s*[a-zA-Z0-9]+)?\\s*\\}");
static Pattern list = Pattern.compile("\\{\\s*((listbegin\\s*\\:\\s*[a-zA-Z0-9]+\\s*\\:\\s*[a-zA-Z0-9]+)|(listend))+\\s*\\}");
private final static String cid = "cid:";
private final static String text_type = "text";
private final static String pic_type = "pic";
private final static String paramSplit = "#%#";
/**
* 分析模板
* // .$|()[{^?*+\\ 特殊字符作为分隔符时需要使用\\进行转义
* 表示空格 " \\s", "[ ]", "[\\s]"
* 1、 表示空格 " \\s", "[ ]", "[\\s]"
*
* 表示多个空格 "\\s+", "[ ]+", "[\\s]+"
*
* 2、 表示数字 "\\d", "[\\d]", "[0-9]"
*
* 表示多个数字,同理,在后面加上"+"
*
* @param userTemplate
* @param isPreView
* @return
*/
public static TemplatePO analysisTemplate(String userTemplate, boolean isPreView, boolean isMail) {
TemplatePO templatePO = new TemplatePO();
templatePO.setTemplateShow(userTemplate);
String templete = userTemplate;
templete = templete.toLowerCase();
if (isMail) {
// templete = templete.replaceAll("\\s+", "");
templete = templete.replaceAll("\n+", "
");
templete = templete.replaceAll("<\\s*((a))", "");
templete = templete.replaceAll("(javascript)|(vbscript)|(expression)|(applet)|(meta)|(xml)|(blink)|(link)|(script)|(embed)|(object)|(iframe)|(frame)|(frameset)|(ilayer)|(layer)|(bgsound)|(title)|(base)|(onabort)|(onactivate)|(onafterprint)|(onafterupdate)|(onbeforeactivate)|(onbeforecopy)|(onbeforecut)|(onbeforedeactivate)|(onbeforeeditfocus)|(onbeforepaste)|(onbeforeprint)|(onbeforeunload)|(onbeforeupdate)|(onblur)|(onbounce)|(oncellchange)|(onchange)|(onclick)|(oncontextmenu)|(oncontrolselect)|(oncopy)|(oncut)|(ondataavailable)|(ondatasetchanged)|(ondatasetcomplete)|(ondblclick)|(ondeactivate)|(ondrag)|(ondragend)|(ondragenter)|(ondragleave)|(ondragover)|(ondragstart)|(ondrop)|(onerror)|(onerrorupdate)|(onfilterchange)|(onfinish)|(onfocus)|(onfocusin)|(onfocusout)|(onhelp)|(onkeydown)|(onkeypress)|(onkeyup)|(onlayoutcomplete)|(onload)|(onlosecapture)|(onmousedown)|(onmouseenter)|(onmouseleave)|(onmousemove)|(onmouseout)|(onmouseover)|(onmouseup)|(onmousewheel)|(onmove)|(onmoveend)|(onmovestart)|(onpaste)|(onpropertychange)|(onreadystatechange)|(onreset)|(onresize)|(onresizeend)|(onresizestart)|(onrowenter)|(onrowexit)|(onrowsdelete)|(onrowsinserted)|(onscroll)|(onselect)|(onselectionchange)|(onselectstart)|(onstart)|(onstop)|(onsubmit)|(onunload)", "");
templete = templete.replaceAll("<\\s*((a|A)|([S|s][C|c][R|r][I|i][P|p][T|t]))", "");
}
templete = templete.replaceAll("", "");
templete = templete.replaceAll("", "");
templete = templete.replaceAll("\\\\\\s*\\{", "##{##");
templete = templete.replaceAll("\\\\\\s*\\}", "##}##");
templatePO.setTemplate(templete);
List listParamsList = new ArrayList();
Map paramMap = new HashMap();
templete = analysisList(templete, listParamsList, paramMap);
List paramList = new ArrayList();
templete = analysisParam(templete, paramList);
for (String param : paramList) {
if (param.indexOf(cid) != -1) {
param = param.replace(cid, "");
paramMap.put(param, pic_type);
} else
paramMap.put(param, text_type);
}
templete = templete.replaceAll("##\\{##", "{");
templete = templete.replaceAll("##\\}##", "}");
System.out.println(templete);
templatePO.setParamMap(paramMap);
templatePO.setTemplate(templete);
return templatePO;
}
/**
* 转换 paramMap
*
* @param map
* @return
*/
public static Map conversionParaMap(Map map) {
Map paramMap = new HashMap();
if (Objects.nonNull(map)) {
for (Object key : map.keySet()) {
//value 是 TParam类 代表是list
if (map.get(key) instanceof TParam) {
//初始化子map
TParam subMap = (TParam) map.get(key);
//itemVar 的value 为 map的key
String listKey = subMap.getListParam();
//list 不为空 且 listKey不为空
if (!subMap.getItemParamList().isEmpty() && StringUtils.isNoneBlank(listKey)) {
Map listMap = new HashMap();
for (Object itemKey : subMap.getItemParamList()) {
listMap.put(itemKey, text_type);
}
//遍历结束 赋值
List listMapList = new ArrayList();
listMapList.add(listMap);
//将遍历的listMapList放入内部
paramMap.put(listKey, listMapList);
}
} else {
paramMap.put(key, map.get(key));
}
}
} else {
return null;
}
return paramMap;
}
/**
* 分析数组
*
* @param template
* @param listParamList
* @param paramMap
* @return
*/
private static String analysisList(String template, List listParamList, Map paramMap) {
//正则匹配
Matcher listMatch = list.matcher(template);
String temp;
String matcherStr;
int index;
String[] paramArr;
String listTemplate;
while (listMatch.find()) {
TParam listParam = new TParam();
matcherStr = temp = listMatch.group();
if ((index = temp.indexOf("{")) != 0)
temp = temp.substring(index);
temp = temp.replaceAll("\\s+", "");
temp = temp.substring(1, temp.length() - 1);
System.out.println(temp);
if ("listend".equals(temp)) { //是否结束
TParam listParamf = listParamList.get(listParamList.size() - 1);
int listEndIndex = template.indexOf(matcherStr) + matcherStr.length();
int contentListEnd = template.indexOf(matcherStr);
listTemplate = template.substring(listParamf.getBegin(), listEndIndex);
List paramList = new ArrayList();
String listTemplateBuild = analysisParam(template.substring(listParamf.getContentBegin(), contentListEnd), paramList);
String listItemVar = listParamf.getItemVar() + ".";
for (String param : paramList) {
if (param.indexOf(listItemVar) != -1) {
param = param.replace(listItemVar, "");
listParamf.getItemParamList().add(param);
} else if (param.indexOf(cid) != -1) {
param = param.replace(cid, "");
listParamf.getParamMap().put(param, pic_type);
} else {
listParamf.getParamMap().put(param, text_type);
}
}
paramMap.put(listParamf.getListParam(), listParamf);
listParamf.setListTemplate(listTemplateBuild);
template = template.replace(listTemplate, paramSplit + listParamf.getListParam() + paramSplit);
// System.out.println("template:"+template);
// System.out.println("templateList:"+listTemplate);
// System.out.println("templateListbuild:"+listTemplateBuild);
} else {
paramArr = temp.split(":");
int listBeginIndex = template.indexOf(matcherStr);
listParam.setBegin(listBeginIndex);
listParam.setContentBegin(listBeginIndex + matcherStr.length());
listParam.setListParam(paramArr[1]);
listParam.setItemVar(paramArr[2]);
listParam.setItemParamList(new ArrayList());
listParam.setParamMap(new HashMap());
listParamList.add(listParam);
}
System.out.println("list: " + temp);
}
//{listbegin:listName:itemName}
//{listend}
return template;
}
/**
* 分析list遍历
*
* @param template
* @param paramList
* @return
*/
private static String analysisParam(String template, List paramList) {
String temp;
String matcherStr;
int index;
Matcher matcher = param.matcher(template);
while (matcher.find()) {
matcherStr = temp = matcher.group();
if ((index = temp.indexOf("{")) != 0)
temp = temp.substring(index);
matcherStr = temp;
temp = temp.replaceAll("\\s+", "");
temp = temp.substring(1, temp.length() - 1);
if ("listend".equals(temp))
continue;
paramList.add(temp);
// paramMap.put(temp,temp);
template = template.replace(matcherStr, paramSplit + temp + paramSplit);
}
return template;
}
/**
* 短信模板信息Param
*
* @param template
* @return
*/
public static String getTemplateParam(String template) {
Map map = new HashMap();
String[] split = template.split("\\$\\{");
Arrays.stream(split).forEach(item -> {
if (item.contains("}")) {
String substring = item.substring(0, item.indexOf("}"));
map.put(substring, "text");
}
});
return JSONObject.toJSONString(map);
}
private static String getJSONObject() {
JSONObject message = new JSONObject();
message.put("msgType", "text");
message.put("title", "alert告警信息");
//消息点击链接地址,当发送消息为小程序时支持小程序跳转链接
message.put("messageUrl", "http://www.messageUrl.com");
message.put("picUrl", "http://www.picUrl.png");
message.put("singleUrl", "http://www.singleUrl.com");
message.put("singleTitle", "alert singleTitle 告警信息");
StringBuffer dingTalkMEssage = new StringBuffer();
dingTalkMEssage.append(" \n### 告警时间:");
dingTalkMEssage.append("2020-10-19 14:24:18");
dingTalkMEssage.append(" \n#### 告警名称:");
dingTalkMEssage.append("睛灵调外接口Stable失败");
dingTalkMEssage.append(" \n#### 告警等级:");
dingTalkMEssage.append("ERROR告警");
dingTalkMEssage.append(" \n#### 告警项目:");
dingTalkMEssage.append("睛灵监控");
dingTalkMEssage.append(" \n#### 告警信息");
dingTalkMEssage.append("\n#### ");
dingTalkMEssage.append("时间");
dingTalkMEssage.append("\n#### ");
dingTalkMEssage.append(" \n#### 维度:");
dingTalkMEssage.append("serviceName=api-service funcCode=dingTalk bizld=error status=0 desc=java.net.ConnectException: Connection refused (Connection refused) addition=null");
dingTalkMEssage.append(" \n#### 维度:");
dingTalkMEssage.append("status=0");
message.put("text", dingTalkMEssage);
message.put("content", dingTalkMEssage);
System.out.println(message);
return message.toJSONString();
}
/**
* 将paramMap转换成map
*
* @param paraMap
* @return
*/
public static Map getPreParam(Map paraMap) {
Map param = new HashMap();
for (Map.Entry entry : paraMap.entrySet()) {
if (entry.getValue() instanceof TParam) {
TParam tparam = (TParam) entry.getValue();
List