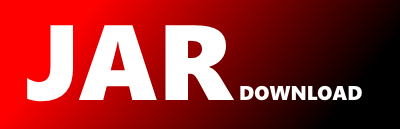
com.annimon.stream.test.hamcrest.OptionalMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-test Show documentation
Show all versions of stream-test Show documentation
Hamcrest and Mockito matchers for Lightweight-Stream-API
package com.annimon.stream.test.hamcrest;
import com.annimon.stream.Optional;
import static org.hamcrest.CoreMatchers.is;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeDiagnosingMatcher;
public class OptionalMatcher {
private OptionalMatcher() { }
public static Matcher> isPresent() {
return new IsPresentMatcher();
}
public static Matcher> isEmpty() {
return new IsEmptyMatcher();
}
public static Matcher> hasValue(T object) {
return hasValueThat(is(object));
}
public static Matcher> hasValueThat(Matcher super T> matcher) {
return new HasValueMatcher(matcher);
}
public static class IsPresentMatcher extends TypeSafeDiagnosingMatcher> {
@Override
protected boolean matchesSafely(Optional> optional, Description mismatchDescription) {
mismatchDescription.appendText("Optional was empty");
return optional.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("Optional value should be present");
}
}
public static class IsEmptyMatcher extends TypeSafeDiagnosingMatcher> {
@Override
protected boolean matchesSafely(Optional> optional, Description mismatchDescription) {
mismatchDescription.appendText("Optional was present");
return !optional.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("Optional value should be empty");
}
}
public static class HasValueMatcher extends TypeSafeDiagnosingMatcher> {
private final Matcher super T> matcher;
public HasValueMatcher(Matcher super T> matcher) {
this.matcher = matcher;
}
@Override
protected boolean matchesSafely(Optional optional, Description mismatchDescription) {
if (!optional.isPresent()) {
mismatchDescription.appendText("Optional was empty");
return false;
}
final T value = optional.get();
mismatchDescription.appendText("Optional value ");
matcher.describeMismatch(value, mismatchDescription);
return matcher.matches(value);
}
@Override
public void describeTo(Description description) {
description.appendText("Optional value ").appendDescriptionOf(matcher);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy