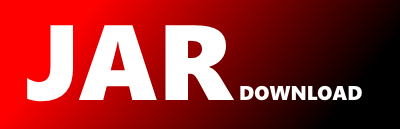
com.anrisoftware.prefdialog.annotations.ComboBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prefdialog-api Show documentation
Show all versions of prefdialog-api Show documentation
Preferences dialog public application interface.
The newest version!
/*
* Copyright 2013-2016 Erwin Müller
*
* This file is part of prefdialog-api.
*
* prefdialog-api is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* prefdialog-api is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with prefdialog-api. If not, see .
*/
package com.anrisoftware.prefdialog.annotations;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Documented;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import javax.swing.ComboBoxEditor;
import javax.swing.ComboBoxModel;
import javax.swing.ListCellRenderer;
/**
* Field to let the user choose from multiple values in a combo-box.
*
* Either a list of values can be set from a different field or a custom
* {@link ComboBoxModel} can be used. It is also possible to set a custom
* {@link ListCellRenderer}. The initial value can be set and is used as the
* initial value of the field.
*
* Examples: a) sets the field with a list of the values
*
*
* @FieldComponent
* @ComboBox(elements = "someFieldElements")
* public String someField;
* // as array
* public String[] someFieldElements = { ... };
* // or as list
* public List<String> someFieldElements = { ... };
*
*
* b) sets the field with an instance of the custom model. If no instance is
* set, the model must have a public standard constructor available for
* instantiation. The new instance is set in the parent object.
*
*
* @FieldComponent
* @ComboBox(model = "customModel")
* public String someField;
*
* public ComboBoxModel customModel;
*
*
* c) sets the custom model class. The custom model must have a public standard
* constructor available for instantiation.
*
*
* @FieldComponent
* @ComboBox(modelClass = CustomComboBoxModel.class)
* public String someField;
*
*
* d) sets the field with an instance of the custom renderer. If no instance is
* set, the renderer must have a public standard constructor available for
* instantiation. The new instance is set in the parent object.
*
*
* @FieldComponent
* @ComboBox(renderer = "customRenderer")
* public String someField;
*
* public ListCellRenderer customRenderer;
*
*
* e) sets the custom renderer class. The custom renderer must have a public
* standard constructor available for instantiation.
*
*
* @FieldComponent
* @ComboBox(rendererClass = CustomListCellRenderer.class)
* public String someField;
*
*
* f) make the combo box editable.
*
*
* @FieldComponent
* @ComboBox(model = "customModel", editable = true)
* public String someField;
*
* public ComboBoxModel customModel = new CustomComboBoxModel();
*
*
* @author Erwin Mueller, [email protected]
* @since 1.0
*/
@Target({ FIELD, METHOD })
@Retention(RUNTIME)
@FieldAnnotation
@Documented
public @interface ComboBox {
/**
* The name of the field name to use for the elements of the combo box. The
* can be an array or an {@link Iterable}. Not needed if the model or model
* class is set with {@link #model()} or {@link #modelClass()}. Defaults to
* an empty name which means no field is set.
*/
String elements() default "";
/**
* If the field should be editable. Set to {@code true} if the combo box
* should be editable or {@code false} if not. Defaults to {@code false}.
*/
boolean editable() default false;
/**
* The name of the field name to use for the custom {@link ComboBoxModel}.
* Defaults to an empty name which means no field is set.
*/
String model() default "";
/**
* The custom {@link ComboBoxModel} to use with this combo box. The model
* must have the default constructor available for instantiation.
*/
@SuppressWarnings("rawtypes")
Class extends ComboBoxModel>[] modelClass() default {};
/**
* The name of the field name to use for the custom {@link ListCellRenderer}
* . Defaults to an empty name which means no field is set.
*/
String renderer() default "";
/**
* The custom {@link ListCellRenderer} to use with this combo box. The
* renderer must have the default constructor available for instantiation.
*/
Class extends ListCellRenderer>>[] rendererClass() default {};
/**
* The name of the field name to use for the custom {@link ComboBoxEditor}.
* Defaults to an empty name which means no field is set.
*
* @since 3.0
*/
String editor() default "";
/**
* The custom {@link ComboBoxEditor} to use with this combo box. The editor
* must have the default constructor available for instantiation.
*
* @since 3.0
*/
Class extends ComboBoxEditor>[] editorClass() default {};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy