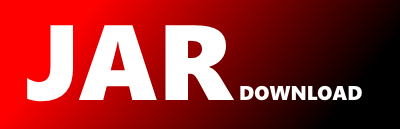
com.anrisoftware.resources.images.mapcachedresolutions.ImagesMapImpl Maven / Gradle / Ivy
/*
* Copyright 2012-2016 Erwin Müller
*
* This file is part of resources-images.
*
* resources-images is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* resources-images is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with resources-images. If not, see .
*/
package com.anrisoftware.resources.images.mapcachedresolutions;
import static java.lang.Math.abs;
import java.awt.Dimension;
import java.util.HashMap;
import java.util.Map;
import javax.inject.Inject;
import com.anrisoftware.resources.api.ResourcesException;
import com.anrisoftware.resources.images.api.ImageResolution;
import com.anrisoftware.resources.images.api.ImageResource;
import com.anrisoftware.resources.images.api.ImagesMap;
/**
* Uses a Java hash map to store the image resources.
*
* @author Erwin Mueller, [email protected]
* @since 1.1
*/
class ImagesMapImpl implements ImagesMap {
private final ImagesMapLogger log;
/**
* Saves the loaded image resources.
*
* The image resources are stored for each name and for each resolution,
* i.e.:
*
*
* [<name:{@link String}> := [<resolution:{@link ImageResolution}> := {@link ImageResource}]]
*
*/
private final Map> images;
/**
* Creates the images map.
*/
@Inject
ImagesMapImpl(ImagesMapLogger logger) {
this.log = logger;
this.images = new HashMap>();
}
@Override
public void putImage(ImageResource image) throws ResourcesException {
String name = image.getName();
ImageResolution resolution = image.getResolution();
Map resolutions = resolutionsMap(name);
if (!resolutions.containsKey(resolution)) {
resolutions.put(resolution, image);
} else {
log.imageAlreadyInMap(this, image);
}
}
private Map resolutionsMap(String name) {
Map resolutions = images.get(name);
if (resolutions == null) {
resolutions = new HashMap();
images.put(name, resolutions);
}
return resolutions;
}
@Override
public ImageResource getImage(String name, Dimension size) {
Map resolutions = resolutionsMap(name);
int diff = Integer.MAX_VALUE;
int newdiff;
int sizeArea = size.width;
int imageArea;
ImageResource foundImage = null;
for (ImageResource image : resolutions.values()) {
imageArea = image.getWidthPx();
newdiff = sizeArea - imageArea;
if (newdiff == 0) {
foundImage = image;
break;
}
if (newdiff < 0 && abs(newdiff) < abs(diff)) {
foundImage = image;
diff = newdiff;
}
if (newdiff > 0 && newdiff < diff) {
foundImage = image;
diff = newdiff;
}
}
return foundImage;
}
@Override
public ImageResource getImage(String name, Dimension size,
ImageResolution resolution) {
Map resolutions;
resolutions = resolutionsMap(name);
ImageResource image = resolutions.get(resolution);
if (image == null) {
log.noImageReturningNearest(this, name);
return getImage(name, size);
}
return image;
}
@Override
public boolean haveImage(String name) {
return images.containsKey(name);
}
@Override
public boolean haveImage(String name, ImageResolution resolution) {
Map resolutions;
resolutions = images.get(name);
return resolutions == null ? false : resolutions
.containsKey(resolution);
}
@Override
public boolean haveImage(String name, ImageResolution resolution,
Dimension size) {
return haveImage(name, resolution);
}
@Override
public String toString() {
return images.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy