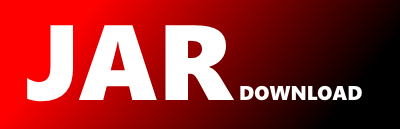
com.antiaction.raptor.dao.AttributeBase Maven / Gradle / Ivy
/*
* Created on 16/08/2008
*
* TODO To change the template for this generated file go to
* Window - Preferences - Java - Code Style - Code Templates
*/
package com.antiaction.raptor.dao;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.List;
import com.antiaction.raptor.sql.DBWrapper;
public abstract class AttributeBase {
/** Spawned from this Attribute type. */
public AttributeTypeBase attributeType = null;
/*
* Initialized directly from storage layer.
*/
/** Associated tree id. */
public int tree_id = 0;
/** Attribute id. */
public int id = 0;
/** Entity id. */
public int entity_id = 0;
/** Attribute type id. */
public int type_id = 0;
protected Integer val_int = null;
protected Timestamp val_datetime = null;
protected String val_varchar = null;
protected String val_text = null;
protected List options = null;
protected List excluded = null;
protected List included = null;
/*
* Methods.
*/
public Integer getInteger() {
throw new UnsupportedOperationException();
}
public void setInteger(Integer integer) {
throw new UnsupportedOperationException();
}
public Timestamp getTimestamp() {
throw new UnsupportedOperationException();
}
public void setTimestamp(Timestamp timestamp) {
throw new UnsupportedOperationException();
}
public String getVarchar() {
throw new UnsupportedOperationException();
}
public void setVarchar(String varchar) {
throw new UnsupportedOperationException();
}
public String getText() {
throw new UnsupportedOperationException();
}
public void setText(String text) {
throw new UnsupportedOperationException();
}
public List getOptions() {
throw new UnsupportedOperationException();
}
public List getExcluded() {
throw new UnsupportedOperationException();
}
public List getIncluded() {
throw new UnsupportedOperationException();
}
public void loadState(DBWrapper db, Connection conn, ResultSet rs) throws SQLException {
tree_id = rs.getInt( "tree_id" );
id = rs.getInt( "id" );
entity_id = rs.getInt( "entity_id" );
type_id = rs.getInt( "type_id" );
val_int = rs.getInt( "val_int" );
if ( rs.wasNull() ) {
val_int = null;
}
val_datetime = rs.getTimestamp( "val_datetime" );
if ( rs.wasNull() ) {
val_datetime = null;
}
val_varchar = rs.getString( "val_varchar" );
if ( rs.wasNull() ) {
val_varchar = null;
}
val_text = rs.getString( "val_text" );
if ( rs.wasNull() ) {
val_text = null;
}
}
public void saveState(DBWrapper db, Connection conn) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy