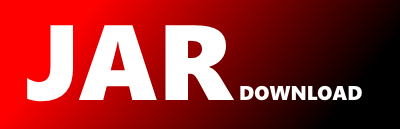
com.antiaction.raptor.dao.EntityBase Maven / Gradle / Ivy
/*
* Created on 16/08/2008
*
* TODO To change the template for this generated file go to
* Window - Preferences - Java - Code Style - Code Templates
*/
package com.antiaction.raptor.dao;
import java.sql.Connection;
import java.sql.ResultSet;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import com.antiaction.raptor.sql.DBWrapper;
public abstract class EntityBase {
/** Spawned from this Entity type. */
public EntityTypeBase entityType = null;
/*
* Initialized directly from storage layer.
*/
/** Associated tree id. */
public int tree_id = 0;
/** Entity id. */
public int id = 0;
/** Entity type id. */
public int type_id = 0;
/** Entity name. */
public String name = null;
/** Children. */
public int children = 0;
/*
* Attributes.
*/
public List attributeList;
public Map attributeMap;
public Map attributeOrdinalMap;
public List getAttributeList() {
return Collections.unmodifiableList( attributeList );
}
public AttributeBase getAttributeByQName(String name) {
int type_id = entityType.getAttributeTypeOrdinalQName( name );
return getAttributeById( type_id );
}
public AttributeBase getAttributeByName(String name) {
int type_id = entityType.getAttributeTypeOrdinalName( name );
return getAttributeById( type_id );
}
public abstract void loadAttributes(DBWrapper db, Connection conn, ResultSet rs);
public abstract AttributeBase getAttributeById(int id);
public abstract void store(SecurityEntityBase user);
public abstract void storeAction(SecurityEntityBase user, String action, StringBuilder script);
public EntityBase copyTo(SecurityEntityBase current_user, int dest_id, boolean recursive) {
Connection conn = null;
EntityBase copy_top_content = null;
ViewBase view = entityType.contentProvider.getView();
/*
try {
// FIXME
//conn = view.env.db.getConnection();
//copy_top_content = view.contentProvider.cloneContent( current_user, conn, this, dest_id );
}
catch (SQLException e) {
e.printStackTrace();
}
if ( conn != null ) {
try {
conn.close();
}
catch (SQLException e) {
// debug
e.printStackTrace();
}
conn = null;
}
*/
return copy_top_content;
}
public int moveTo(SecurityEntityBase current_user, int dest_id) {
Connection conn = null;
ViewBase view = entityType.contentProvider.getView();
/*
try {
// FIXME
//conn = view.env.db.getConnection();
//view.env.db.tree_move( conn, tree_id, id, dest_id );
}
catch (SQLException e) {
e.printStackTrace();
}
if ( conn != null ) {
try {
conn.close();
}
catch (SQLException e) {
// debug
e.printStackTrace();
}
conn = null;
}
*/
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy