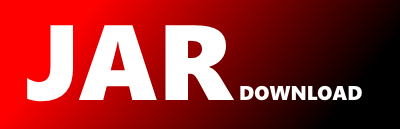
com.antiaction.raptor.sql.mssql.MSSql_I18N Maven / Gradle / Ivy
/*
* Created on 28/02/2010
*
* TODO To change the template for this generated file go to
* Window - Preferences - Java - Code Style - Code Templates
*/
package com.antiaction.raptor.sql.mssql;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.antiaction.raptor.i18n.I18N;
public class MSSql_I18N extends I18N {
private static Logger logger = Logger.getLogger( MSSql_I18N.class.getName() );
protected List pendingTextIdList = new ArrayList();
protected Set pendingTextIdSet = new HashSet();
public static I18N getI18N(Connection conn, int translation_id) {
I18N i18n = new MSSql_I18N();
i18n.translation_id = translation_id;
Map idMap;
String sql;
PreparedStatement stm = null;
ResultSet rs = null;
int language_id;
int text_id;
String text_idstring;
String text_translated;
try {
sql = "SELECT id, text_idstring FROM i18n_text_idstrings ";
sql += "WHERE translation_id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, translation_id );
rs = stm.executeQuery();
while ( rs.next() ) {
text_id = rs.getInt( 1 );
text_idstring = rs.getString( 2 );
i18n.idstrList.add( text_idstring );
i18n.idstrMap.put( text_idstring, text_id );
}
rs.close();
rs = null;
stm.close();
stm = null;
Collections.sort( i18n.idstrList );
sql = "SELECT language_id, text_id, text_translated FROM i18n_text_translated ";
sql += "WHERE translation_id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, translation_id );
rs = stm.executeQuery();
while ( rs.next() ) {
language_id = rs.getInt( 1 );
text_id = rs.getInt( 2 );
text_translated = rs.getString( 3 );
idMap = i18n.langIdMap.get( language_id );
if ( idMap == null ) {
idMap = new HashMap();
i18n.langIdMap.put( language_id, idMap );
}
idMap.put( text_id, text_translated );
}
rs.close();
rs = null;
stm.close();
stm = null;
}
catch (SQLException e) {
i18n = null;
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( rs != null ) {
try {
rs.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
rs = null;
}
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
return i18n;
}
public String getTranslated(int language_id, String text_idstring) {
int text_id = 0;
String translated_text = null;
if ( text_idstring == null || text_idstring.length() == 0 ) {
return null;
}
if ( idstrMap.containsKey( text_idstring ) ) {
text_id = idstrMap.get( text_idstring );
}
else {
synchronized ( pendingTextIdSet ) {
if ( !pendingTextIdSet.contains( text_idstring ) ) {
pendingTextIdList.add( text_idstring );
pendingTextIdSet.add( text_idstring );
}
}
}
if ( langIdMap.containsKey( language_id ) ) {
Map idMap = langIdMap.get( language_id );
if ( idMap != null ) {
if ( idMap.containsKey( text_id ) ) {
translated_text = idMap.get( text_id );
}
}
}
if ( translated_text == null ) {
translated_text = text_idstring;
}
return translated_text;
}
public void flush_changes(Connection conn) {
String text_idstring;
int text_id;
String sql;
PreparedStatement stm = null;
ResultSet rs = null;
synchronized ( pendingTextIdSet ) {
for ( int i=0; i idMap = langIdMap.get( language_id );
if ( idMap != null ) {
if ( idMap.containsKey( text_id ) ) {
old_translated_text = idMap.get( text_id );
}
if ( old_translated_text != null || new_translated_text != null ) {
try {
sql = "DELETE FROM i18n_text_translated ";
sql += "WHERE translation_id = ? AND language_id = ? AND text_id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, translation_id );
stm.setInt( 2, language_id );
stm.setInt( 3, text_id );
stm.executeUpdate();
if ( new_translated_text != null ) {
sql = "INSERT INTO i18n_text_translated(translation_id, language_id, text_id, text_translated) ";
sql += "VALUES(?, ? ,? ,?) ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, translation_id );
stm.setInt( 2, language_id );
stm.setInt( 3, text_id );
stm.setString( 4, new_translated_text );
stm.executeUpdate();
stm.close();
stm = null;
}
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy