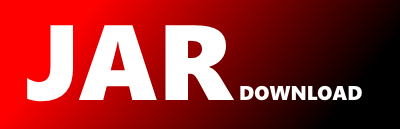
com.antiaction.raptor.sql.mssql.MSSql_SecurityTreePermission Maven / Gradle / Ivy
/*
* Created on 08/04/2010
*
* TODO To change the template for this generated file go to
* Window - Preferences - Java - Code Style - Code Templates
*/
package com.antiaction.raptor.sql.mssql;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.antiaction.raptor.dao.SecurityPermission;
public class MSSql_SecurityTreePermission {
private static Logger logger = Logger.getLogger( MSSql_SecurityTreePermission.class.getName() );
public static int insert(Connection conn, SecurityPermission permission) {
String sql;
PreparedStatement stm = null;
ResultSet rs = null;
try {
sql = "INSERT INTO security_tree_permission(user_tree_id, user_id, on_tree_id, on_id) ";
sql += "VALUES(?, ?, ?, ?) ";
sql += "SELECT id FROM security_tree_permission WHERE id = @@identity ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, permission.user_tree_id );
stm.setInt( 2, permission.user_id );
stm.setInt( 3, permission.on_tree_id );
stm.setInt( 4, permission.on_id );
rs = stm.executeQuery();
if ( rs.next() ) {
permission.id = rs.getInt( 1 );
}
rs.close();
rs = null;
stm.close();
stm = null;
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( rs != null ) {
try {
rs.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
rs = null;
}
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
return permission.id;
}
public static SecurityPermission getById(Connection conn, int id) {
SecurityPermission permission = null;
String sql;
PreparedStatement stm = null;
ResultSet rs = null;
try {
sql = "SELECT id, user_tree_id, user_id, on_tree_id, on_id FROM security_tree_permission ";
sql += "WHERE id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, id );
rs = stm.executeQuery();
if ( rs.next() ) {
permission = new SecurityPermission();
permission.id = rs.getInt( 1 );
permission.user_tree_id = rs.getInt( 2 );
permission.user_id = rs.getInt( 3 );
permission.on_tree_id = rs.getInt( 4 );
permission.on_id = rs.getInt( 5 );
}
rs.close();
rs = null;
stm.close();
stm = null;
}
catch (SQLException e) {
permission = null;
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( rs != null ) {
try {
rs.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
rs = null;
}
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
return permission;
}
public static void update(Connection conn, SecurityPermission permission) {
String sql;
PreparedStatement stm = null;
try {
sql = "UPDATE security_tree_permission SET ";
sql += "user_tree_id = ?, ";
sql += "user_id = ?, ";
sql += "on_tree_id = ?, ";
sql += "on_id = ? ";
sql += "WHERE id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, permission.user_tree_id );
stm.setInt( 2, permission.user_id );
stm.setInt( 3, permission.on_tree_id );
stm.setInt( 4, permission.on_id );
stm.setInt( 5, permission.id );
stm.executeUpdate();
stm.close();
stm = null;
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
}
public static void deleteByObj(Connection conn, SecurityPermission permission) {
if ( permission != null && permission.id > 0 ) {
deleteById( conn, permission.id );
}
}
public static void deleteById(Connection conn, int id) {
String sql;
PreparedStatement stm = null;
try {
sql = "DELETE security_tree_permission ";
sql += "WHERE id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
stm.setInt( 1, id );
stm.executeUpdate();
stm.close();
stm = null;
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy