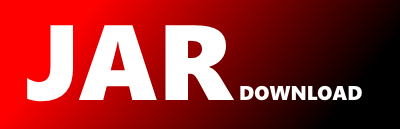
com.antiaction.raptor.sql.mssql.MSSql_View Maven / Gradle / Ivy
/*
* Created on 25/05/2010
*
* TODO To change the template for this generated file go to
* Window - Preferences - Java - Code Style - Code Templates
*/
package com.antiaction.raptor.sql.mssql;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.antiaction.raptor.dao.ViewBase;
public class MSSql_View {
private static Logger logger = Logger.getLogger( MSSql_View.class.getName() );
public static List getViews(Connection conn, ClassLoader classLoader) {
List views = new ArrayList();
ViewBase view;
String sql;
PreparedStatement stm = null;
ResultSet rs = null;
String class_namespace;
String class_name;
Class> cls;
try {
sql = "SELECT id, name, class_namespace, class_name, root_id, root_type_id, seq, default_viewpane, icon16, icon24 FROM view_views ";
sql += "ORDER BY seq";
//sql += "WHERE tree_id = ? ";
stm = conn.prepareStatement( sql );
stm.clearParameters();
//stm.setInt( 1, tree_id );
rs = stm.executeQuery();
while ( rs.next() ) {
class_namespace = rs.getString( 3 );
class_name = rs.getString( 4 );
try {
cls = classLoader.loadClass( class_namespace + "." + class_name );
Object obj = cls.newInstance();
view = (ViewBase)obj;
if ( view != null ) {
view.id = rs.getInt( 1 );
view.name = rs.getString( 2 );
view.class_namespace = rs.getString( 3 );
view.class_name = rs.getString( 4 );
view.root_id = rs.getInt( 5 );
view.root_type_id = rs.getInt( 6 );
view.seq = rs.getInt( 7 );
view.default_viewpane = rs.getString( 8 );
view.icon_16 = rs.getString( 9 );
view.icon_24 = rs.getString( 10 );
views.add( view );
}
}
catch(ClassNotFoundException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
catch(InstantiationException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
catch(IllegalAccessException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
}
rs.close();
rs = null;
stm.close();
stm = null;
}
catch (SQLException e) {
views = null;
logger.log( Level.SEVERE, e.toString(), e );
}
finally {
if ( rs != null ) {
try {
rs.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
rs = null;
}
if ( stm != null ) {
try {
stm.close();
}
catch (SQLException e) {
logger.log( Level.SEVERE, e.toString(), e );
}
stm = null;
}
}
return views;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy