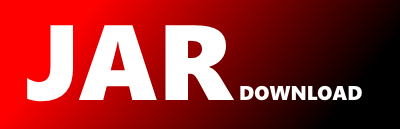
com.antwerkz.sofia.SofiaJava Maven / Gradle / Ivy
package com.antwerkz.sofia;
import java.util.*;
import java.text.*;
import org.slf4j.*;
public class SofiaJava {
private static final Logger logger = LoggerFactory.getLogger("com.antwerkz.sofia.SofiaJava");
private static final Map IMPLS = new HashMap<>();
static {
IMPLS.put("", new LocalizedImpl());
IMPLS.put("de", new LocalizedImpl_de());
IMPLS.put("en", new LocalizedImpl_en());
IMPLS.put("en_GB", new LocalizedImpl_en_GB());
}
private static Localized get(Locale... locale) {
if(locale.length == 0) {
return IMPLS.get("");
}
String name = locale[0].toString();
while (!name.isEmpty()) {
Localized localized = IMPLS.get(name);
if(localized != null) {
return localized;
} else {
name = name.contains("_") ? name.substring(0, name.lastIndexOf('_')) : "";
}
}
return IMPLS.get("");
}
public static String another(Locale... locale) {
return get(locale).another();
}
public static void logAnother(Locale... locale) {
if(logger.isErrorEnabled()) {
logger.error(another(locale));
}
}
public static String dateProperty(Date arg0, Number arg1, Locale... locale) {
return get(locale).dateProperty(arg0, arg1);
}
public static void logDateProperty(Date arg0, Number arg1, Locale... locale) {
if(logger.isErrorEnabled()) {
logger.error(dateProperty(arg0, arg1, locale));
}
}
public static String dateProperty2(Object arg0, Object arg1, Locale... locale) {
return get(locale).dateProperty2(arg0, arg1);
}
public static void logDateProperty2(Object arg0, Object arg1, Locale... locale) {
if(logger.isErrorEnabled()) {
logger.error(dateProperty2(arg0, arg1, locale));
}
}
public static String me(Locale... locale) {
return get(locale).me();
}
public static void logMe(Locale... locale) {
if(logger.isWarnEnabled()) {
logger.warn(me(locale));
}
}
public static String dateProperty(Locale... locale) {
return get(locale).dateProperty();
}
public static String lonely(Locale... locale) {
return get(locale).lonely();
}
public static String newProperty(Locale... locale) {
return get(locale).newProperty();
}
public static String parameterizedPropertyLongName(Object arg0, Object arg1, Locale... locale) {
return get(locale).parameterizedPropertyLongName(arg0, arg1);
}
public static String testProperty(Locale... locale) {
return get(locale).testProperty();
}
interface Localized {
/**
* Generated from @error.another
*/
default String another(Locale... locale) {
return "I'm an error";
}
/**
* Generated from @error.another
*/
default void logAnother(Locale... locale) {
if(SofiaJava.logger.isErrorEnabled()) {
SofiaJava.logger.error(another(locale));
}
}
/**
* Generated from @error.date.property
*/
default String dateProperty(Date arg0, Number arg1, Locale... locale) {
return MessageFormat.format("Today''s date {0,date,full} and now a number {1,number}", arg0, arg1);
}
/**
* Generated from @error.date.property
*/
default void logDateProperty(Date arg0, Number arg1, Locale... locale) {
if(SofiaJava.logger.isErrorEnabled()) {
SofiaJava.logger.error(dateProperty(arg0, arg1, locale));
}
}
/**
* Generated from @error.date.property2
*/
default String dateProperty2(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("Today''s date {0} and now a number {1}", arg0, arg1);
}
/**
* Generated from @error.date.property2
*/
default void logDateProperty2(Object arg0, Object arg1, Locale... locale) {
if(SofiaJava.logger.isErrorEnabled()) {
SofiaJava.logger.error(dateProperty2(arg0, arg1, locale));
}
}
/**
* Generated from @warn.me
*/
default String me(Locale... locale) {
return "I'm just a warning, though.";
}
/**
* Generated from @warn.me
*/
default void logMe(Locale... locale) {
if(SofiaJava.logger.isWarnEnabled()) {
SofiaJava.logger.warn(me(locale));
}
}
/**
* Generated from date.property
*/
default String dateProperty(Locale... locale) {
return "Today's date is {0,date,full} and now a number {1,number}";
}
/**
* Generated from lonely
*/
default String lonely(Locale... locale) {
return "I'm only in the default bundle.";
}
/**
* Generated from new.property
*/
default String newProperty(Locale... locale) {
return "New Property";
}
/**
* Generated from parameterized.property.long.name
*/
default String parameterizedPropertyLongName(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("I need parameters {0} and {1}", arg0, arg1);
}
/**
* Generated from test.property
*/
default String testProperty(Locale... locale) {
return "I'm the first test property";
}
}
private static class LocalizedImpl implements Localized {
}
private static class LocalizedImpl_de extends LocalizedImpl {
public String another(Locale... locale) {
return "Ich bin ein Fehler";
}
public String dateProperty(Date arg0, Number arg1, Locale... locale) {
return MessageFormat.format("Today''s date {0,date,full} and now a number {1,number}", arg0, arg1);
}
public String dateProperty2(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("Die heutigen Datum {0} und nun eine Reihe {1}", arg0, arg1);
}
public String me(Locale... locale) {
return "Ich bin nur eine Warnung, wenn.";
}
public String dateProperty(Locale... locale) {
return "Today's date is {0,date,full} and now a number {1,number}";
}
public String newProperty(Locale... locale) {
return "Neue Propertische";
}
public String parameterizedPropertyLongName(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("I need zwei parameters {0} and {1}", arg0, arg1);
}
public String testProperty(Locale... locale) {
return "I'm zee first test property";
}
}
private static class LocalizedImpl_en extends LocalizedImpl {
public String dateProperty(Date arg0, Number arg1, Locale... locale) {
return MessageFormat.format("I only want a date {0,date,full} and this number {1,number}", arg0, arg1);
}
public String dateProperty2(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("date {0} number {1}", arg0, arg1);
}
}
private static class LocalizedImpl_en_GB extends LocalizedImpl_en {
public String another(Locale... locale) {
return "I'm an error";
}
public String me(Locale... locale) {
return "I'm just a warning, though.";
}
public String dateProperty(Locale... locale) {
return "Today's date is {0,date,full} and now a number {1,number}";
}
public String newProperty(Locale... locale) {
return "New Guy";
}
public String parameterizedPropertyLongName(Object arg0, Object arg1, Locale... locale) {
return MessageFormat.format("I need two parameters {0} and {1}", arg0, arg1);
}
public String testProperty(Locale... locale) {
return "I'm the first test property, bloke";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy