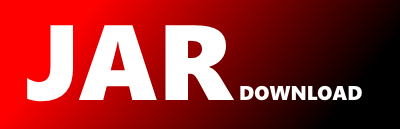
com.antwerkz.sofia.SofiaKotlin.kt Maven / Gradle / Ivy
The newest version!
package com.antwerkz.sofia
import java.text.MessageFormat
import java.util.Date
import java.util.Locale
import kotlin.Any
import kotlin.Number
import kotlin.String
import kotlin.Unit
import kotlin.collections.MutableMap
import kotlin.collections.MutableSet
import org.slf4j.Logger
import org.slf4j.LoggerFactory
public object SofiaKotlin {
private val logger: Logger = LoggerFactory.getLogger("com.antwerkz.sofia.SofiaKotlin")
private val loggedMessages: MutableSet = mutableSetOf()
private val IMPLS: MutableMap = mutableMapOf()
init {
IMPLS.put("", LocalizedImpl())
IMPLS.put("de", LocalizedImpl_de())
IMPLS.put("en", LocalizedImpl_en())
IMPLS.put("en_GB", LocalizedImpl_en_GB())
}
private fun `get`(locale: Locale?): Localized {
if (locale == null) {
return IMPLS.get("")!!
}
var name = locale.toString()
while (!name.isEmpty()) {
val localized = IMPLS.get(name)
if(localized != null) {
return localized
} else {
name = if (name.contains("_")) name.substring(0, name.lastIndexOf('_')) else ""
}
}
return IMPLS.get("")!!
}
public fun logDateProperty(
arg0: Date,
arg1: Number,
locale: Locale? = null,
): Unit {
if(logger.isErrorEnabled()) {
val message = dateProperty(arg0, arg1, locale)
logger.error(message)
}
}
/**
* Generated from @error.date.property
*/
public fun dateProperty(
arg0: Date,
arg1: Number,
locale: Locale? = null,
): String = get(locale).dateProperty(arg0, arg1)
public fun logDateProperty2(
arg0: Any,
arg1: Any,
locale: Locale? = null,
): Unit {
if(logger.isErrorEnabled()) {
val message = dateProperty2(arg0, arg1, locale)
logger.error(message)
}
}
/**
* Generated from @error.date.property2
*/
public fun dateProperty2(
arg0: Any,
arg1: Any,
locale: Locale? = null,
): String = get(locale).dateProperty2(arg0, arg1)
public fun logAnother(locale: Locale? = null): Unit {
if(logger.isErrorEnabled()) {
val message = another(locale)
if(loggedMessages.add(message)) {
logger.error(message)
}
}
}
/**
* Generated from @error[once].another
*/
public fun another(locale: Locale? = null): String = get(locale).another()
public fun logMe(locale: Locale? = null): Unit {
if(logger.isWarnEnabled()) {
val message = me(locale)
logger.warn(message)
}
}
/**
* Generated from @warn.me
*/
public fun me(locale: Locale? = null): String = get(locale).me()
/**
* Generated from date.property
*/
public fun dateProperty(locale: Locale? = null): String = get(locale).dateProperty()
/**
* Generated from lonely
*/
public fun lonely(locale: Locale? = null): String = get(locale).lonely()
/**
* Generated from new.property
*/
public fun newProperty(locale: Locale? = null): String = get(locale).newProperty()
/**
* Generated from parameterized.property.long.name
*/
public fun parameterizedPropertyLongName(
arg0: Any,
arg1: Any,
locale: Locale? = null,
): String = get(locale).parameterizedPropertyLongName(arg0, arg1)
/**
* Generated from quoted
*/
public fun quoted(locale: Locale? = null): String = get(locale).quoted()
/**
* Generated from quoted2
*/
public fun quoted2(arg0: Any, locale: Locale? = null): String = get(locale).quoted2(arg0)
/**
* Generated from test.property
*/
public fun testProperty(locale: Locale? = null): String = get(locale).testProperty()
private interface Localized {
public fun dateProperty(arg0: Date, arg1: Number): String =
MessageFormat.format("Today''s date {0,date,full} and now a number {1,number}", arg0, arg1)
public fun dateProperty2(arg0: Any, arg1: Any): String =
MessageFormat.format("Today''s date {0} and now a number {1}", arg0, arg1)
public fun another(): String = "I'm an error"
public fun me(): String = "I'm just a warning, though."
public fun dateProperty(): String = "Today's date is {0,date,full} and now a number {1,number}"
public fun lonely(): String = "I'm only in the default bundle."
public fun newProperty(): String = "New Property"
public fun parameterizedPropertyLongName(arg0: Any, arg1: Any): String =
MessageFormat.format("I need parameters {0} and {1}", arg0, arg1)
public fun quoted(): String =
"\"To be or not to be. That is the question,\" said an overly wrought Hamlet."
public fun quoted2(arg0: Any): String =
MessageFormat.format("But sometimes one needs to \"quote\" {0} in the middle, too.", arg0)
public fun testProperty(): String = "I'm the first test property"
}
private open class LocalizedImpl : Localized
private open class LocalizedImpl_de : LocalizedImpl() {
public override fun another(): String = "Ich bin ein Fehler"
public override fun dateProperty(arg0: Date, arg1: Number): String =
MessageFormat.format("Today''s date {0,date,full} and now a number {1,number}", arg0, arg1)
public override fun dateProperty2(arg0: Any, arg1: Any): String =
MessageFormat.format("Die heutigen Datum {0} und nun eine Reihe {1}", arg0, arg1)
public override fun me(): String = "Ich bin nur eine Warnung, wenn."
public override fun dateProperty(): String =
"Today's date is {0,date,full} and now a number {1,number}"
public override fun newProperty(): String = "Neue Propertische"
public override fun parameterizedPropertyLongName(arg0: Any, arg1: Any): String =
MessageFormat.format("I need zwei parameters {0} and {1}", arg0, arg1)
public override fun testProperty(): String = "I'm zee first test property"
}
private open class LocalizedImpl_en : LocalizedImpl() {
public override fun dateProperty(arg0: Date, arg1: Number): String =
MessageFormat.format("I only want a date {0,date,full} and this number {1,number}", arg0,
arg1)
public override fun dateProperty2(arg0: Any, arg1: Any): String =
MessageFormat.format("date {0} number {1}", arg0, arg1)
}
private open class LocalizedImpl_en_GB : LocalizedImpl_en() {
public override fun another(): String = "I'm an error"
public override fun me(): String = "I'm just a warning, though."
public override fun dateProperty(): String =
"Today's date is {0,date,full} and now a number {1,number}"
public override fun newProperty(): String = "New Guy"
public override fun parameterizedPropertyLongName(arg0: Any, arg1: Any): String =
MessageFormat.format("I need two parameters {0} and {1}", arg0, arg1)
public override fun testProperty(): String = "I'm the first test property, bloke"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy