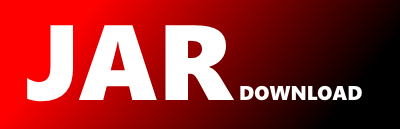
com.antwerkz.sofia.LocalizerGenerator Maven / Gradle / Ivy
package com.antwerkz.sofia;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StringWriter;
import java.io.Writer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import java.util.TreeMap;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
class LocalizerGenerator {
private List methods = new ArrayList();
private String pkgName;
private File baseFile;
private String baseName;
private String ext;
private Map messages;
private Set keySet;
private String bundleName;
public LocalizerGenerator(String pkgName, File file) throws IOException {
this.pkgName = pkgName;
baseFile = file;
bundleName = file.getName();
if(bundleName.contains(".")) {
bundleName = bundleName.substring(0, bundleName.indexOf("."));
}
final String fileName = file.getName();
baseName = fileName.substring(0, fileName.lastIndexOf('.'));
ext = fileName.contains(".") ? fileName.substring(fileName.lastIndexOf('.') + 1) : "";
messages = new HashMap();
Map map = loadPropertiesFile(file);
keySet = map.keySet();
for (Entry entry : map.entrySet()) {
methods.add(buildMethod(entry.getKey(), entry.getValue()));
}
// record(null, map);
// record(extractLocale(file), map);
// loadOtherLocales(map, file);
}
/*
private void record(String locale, Map map) {
for (Entry entry : map.entrySet()) {
messages.put(String.format("%s%s", locale == null ? "" : locale + ".", entry.getKey()), entry.getValue());
}
}
private void loadOtherLocales(Map map, File baseFile) throws IOException {
final File root = baseFile.getParentFile();
final String fileName = baseFile.getName();
final File[] files = root.listFiles(new FilenameFilter() {
public boolean accept(File dir, String name) {
return !name.equals(fileName) && name.startsWith(baseName) && name.endsWith(ext);
}
});
for (File file : files) {
record(extractLocale(file), loadPropertiesFile(file));
}
}
*/
private Map loadPropertiesFile(File file) throws IOException {
Properties props = new Properties();
Map map = new TreeMap();
props.load(new FileInputStream(file));
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy