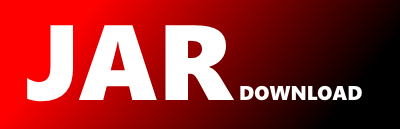
com.antwerkz.sofia.SofiaConfig Maven / Gradle / Ivy
package com.antwerkz.sofia;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.nio.charset.Charset;
import java.util.Locale;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.TreeMap;
public class SofiaConfig {
private String bundleName = "sofia";
private String className;
private String packageName = "com.antwerkz.sofia";
private File outputDirectory;
private LoggingType type = LoggingType.NONE;
private boolean useControl = false;
private Map properties;
private boolean generateJavascript;
private File javascriptOutputFile;
// This is the default charset used when reading property files from an InputStream
private Charset charset = Charset.forName("ISO-8859-1");
private Map> bundles;
public String getBundleName() {
return bundleName;
}
public String getClassName() {
return className;
}
public File getOutputDirectory() {
return outputDirectory;
}
public String getPackageName() {
return packageName;
}
public Map getProperties() {
return properties;
}
public LoggingType getType() {
return type;
}
public boolean isUseControl() {
return useControl;
}
public SofiaConfig setBundleName(String bundleName) {
this.bundleName = bundleName;
return this;
}
public SofiaConfig setClassName(String className) {
this.className = className;
return this;
}
public SofiaConfig setOutputDirectory(File outputDirectory) {
this.outputDirectory = outputDirectory;
return this;
}
public SofiaConfig setOutputDirectory(String outputDirectory) {
this.outputDirectory = new File(outputDirectory);
return this;
}
public SofiaConfig setPackageName(String packageName) {
this.packageName = packageName;
return this;
}
public SofiaConfig setProperties(File propertiesFile) {
try {
this.properties = loadProperties(new FileInputStream(propertiesFile));
discoverBundles(propertiesFile);
} catch (FileNotFoundException e) {
throw new RuntimeException(e.getMessage(), e);
}
return this;
}
private void discoverBundles(final File propertiesFile) throws FileNotFoundException {
String name = propertiesFile.getName();
String rootDir = propertiesFile.getParent();
bundles = new TreeMap<>();
int last = name.lastIndexOf('.');
if(!name.matches(".*_[a-zA-Z]+[_a-zA-Z]*.properties")) {
Map value = loadProperties(new FileInputStream(propertiesFile));
bundles.put("", value);
bundles.put(Locale.getDefault().toString(), value);
}
for (Locale locale : Locale.getAvailableLocales()) {
File file = new File(rootDir, String.format("%s_%s%s", name.substring(0, last), locale.toString(), name.substring(last)));
if(file.exists()) {
bundles.put(locale.toString(), loadProperties(new FileInputStream(file)));
}
}
}
public SofiaConfig setProperties(Map properties) {
this.properties = properties;
return this;
}
public SofiaConfig setProperties(InputStream stream) {
this.properties = loadProperties(stream);
return this;
}
public SofiaConfig setUseControl(boolean useControl) {
this.useControl = useControl;
return this;
}
public SofiaConfig setType(LoggingType type) {
this.type = type;
return this;
}
private Map loadProperties(InputStream inputStream) {
Map map = new TreeMap<>();
try (
InputStream stream = inputStream;
Reader reader = new InputStreamReader(stream, charset)
) {
Properties props = new Properties();
props.load(reader);
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy