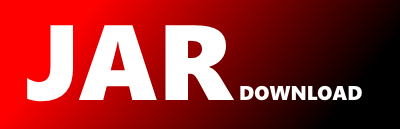
com.aoindustries.aoserv.client.BackupReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2003-2013, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.CompressedDataInputStream;
import com.aoindustries.io.CompressedDataOutputStream;
import java.io.IOException;
import java.sql.Date;
import java.sql.ResultSet;
import java.sql.SQLException;
/**
* A BackupReport
is generated once per day per package and per server. This information
* is averaged through a month and used for account billing. The reports are processed at or near 2:00am
* and basically represent the report for the previous day.
*
* @author AO Industries, Inc.
*/
final public class BackupReport extends AOServObject implements SingleTableObject {
static final int COLUMN_PKEY=0;
static final String COLUMN_DATE_name = "date";
static final String COLUMN_SERVER_name = "server";
static final String COLUMN_PACKAGE_name = "package";
/**
* The hour of the day (in master server time zone) that backup reports will be created.
*/
public static final int BACKUP_REPORT_HOUR=2;
/**
* The minute (in master server time zone) that backup reports will be created.
*/
public static final int BACKUP_REPORT_MINUTE=15;
/**
* The maximum number of days that reports will be maintained. This is roughly 5 years.
*/
public static final int MAX_REPORT_AGE=2*366+3*365; // Assumes worst-case of two leap years in 5-year span.
private int pkey;
int server;
int packageNum;
private long date;
private int file_count;
private long disk_size;
AOServTable table;
@Override
boolean equalsImpl(Object O) {
return
O instanceof BackupReport
&& ((BackupReport)O).pkey==pkey
;
}
@Override
Object getColumnImpl(int i) {
switch(i) {
case COLUMN_PKEY: return pkey;
case 1: return server;
case 2: return packageNum;
case 3: return getDate();
case 4: return file_count;
case 5: return disk_size;
default: throw new IllegalArgumentException("Invalid index: "+i);
}
}
public int getPkey() {
return pkey;
}
public Server getServer() throws SQLException, IOException {
Server se=table.connector.getServers().get(server);
if(se==null) throw new SQLException("Unable to find Server: "+server);
return se;
}
public Package getPackage() throws IOException, SQLException {
Package pk=table.connector.getPackages().get(packageNum);
if(pk==null) throw new SQLException("Unable to find Package: "+packageNum);
return pk;
}
public Date getDate() {
return new Date(date);
}
public int getFileCount() {
return file_count;
}
public long getDiskSize() {
return disk_size;
}
@Override
public Integer getKey() {
return pkey;
}
@Override
public AOServTable getTable() {
return table;
}
@Override
public SchemaTable.TableID getTableID() {
return SchemaTable.TableID.BACKUP_REPORTS;
}
@Override
int hashCodeImpl() {
return pkey;
}
@Override
public void init(ResultSet result) throws SQLException {
pkey=result.getInt(1);
server=result.getInt(2);
packageNum=result.getInt(3);
date=result.getDate(4).getTime();
file_count=result.getInt(5);
disk_size=result.getLong(6);
}
@Override
public void read(CompressedDataInputStream in) throws IOException {
pkey=in.readCompressedInt();
server=in.readCompressedInt();
packageNum=in.readCompressedInt();
date=in.readLong();
file_count=in.readInt();
disk_size=in.readLong();
}
@Override
public void setTable(AOServTable table) {
if(this.table!=null) throw new IllegalStateException("table already set");
this.table=table;
}
@Override
public void write(CompressedDataOutputStream out, AOServProtocol.Version version) throws IOException {
out.writeCompressedInt(pkey);
out.writeCompressedInt(server);
out.writeCompressedInt(packageNum);
out.writeLong(date);
out.writeInt(file_count);
if(version.compareTo(AOServProtocol.Version.VERSION_1_30)<=0) {
out.writeLong(0); // uncompressed_size
out.writeLong(0); // compressed_size
}
out.writeLong(disk_size);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy