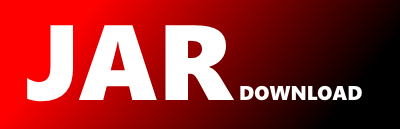
com.aoindustries.aoserv.client.CachedTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2009, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import java.io.IOException;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.BitSet;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* A CachedTable
stores all of the
* available CachedObject
s and performs
* all subsequent data access locally. The server
* notifies the client when a table is updated, and
* the caches are then invalidated. Once invalidated,
* the data is reloaded upon next use.
*
* @author AO Industries, Inc.
*/
public abstract class CachedTable> extends AOServTable {
/**
* The last time that the data was loaded, or
* -1
if not yet loaded.
*/
private long lastLoaded=-1;
/**
* The internal objects are stored in HashMaps
* based on unique columns.
*/
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy