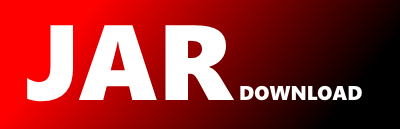
com.aoindustries.aoserv.client.EmailForwardingTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2013, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.io.TerminalWriter;
import java.io.IOException;
import java.io.Reader;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* An Architecture
wraps all the data for a single supported
* computer architecture.
*
* @author AO Industries, Inc.
*/
final public class EmailForwardingTable extends CachedTableIntegerKey {
EmailForwardingTable(AOServConnector connector) {
super(connector, EmailForwarding.class);
}
private static final OrderBy[] defaultOrderBy = {
new OrderBy(EmailForwarding.COLUMN_EMAIL_ADDRESS_name+'.'+EmailAddress.COLUMN_DOMAIN_name+'.'+EmailDomain.COLUMN_DOMAIN_name, ASCENDING),
new OrderBy(EmailForwarding.COLUMN_EMAIL_ADDRESS_name+'.'+EmailAddress.COLUMN_DOMAIN_name+'.'+EmailDomain.COLUMN_AO_SERVER_name+'.'+AOServer.COLUMN_HOSTNAME_name, ASCENDING),
new OrderBy(EmailForwarding.COLUMN_EMAIL_ADDRESS_name+'.'+EmailAddress.COLUMN_ADDRESS_name, ASCENDING),
new OrderBy(EmailForwarding.COLUMN_DESTINATION_name, ASCENDING)
};
@Override
OrderBy[] getDefaultOrderBy() {
return defaultOrderBy;
}
int addEmailForwarding(EmailAddress emailAddressObject, String destination) throws IOException, SQLException {
if (!EmailAddress.isValidEmailAddress(destination)) throw new SQLException("Invalid destination: " + destination);
return connector.requestIntQueryIL(
true,
AOServProtocol.CommandID.ADD,
SchemaTable.TableID.EMAIL_FORWARDING,
emailAddressObject.pkey,
destination
);
}
@Override
public EmailForwarding get(int pkey) throws SQLException, IOException {
return getUniqueRow(EmailForwarding.COLUMN_PKEY, pkey);
}
List getEmailForwarding(Business business) throws SQLException, IOException {
List cached = getRows();
int len = cached.size();
List matches=new ArrayList<>(len);
for (int c = 0; c < len; c++) {
EmailForwarding forward = cached.get(c);
if (forward
.getEmailAddress()
.getDomain()
.getPackage()
.getBusiness()
.equals(business)
) matches.add(forward);
}
return matches;
}
List getEmailForwardings(EmailAddress ea) throws IOException, SQLException {
return getIndexedRows(EmailForwarding.COLUMN_EMAIL_ADDRESS, ea.pkey);
}
List getEnabledEmailForwardings(EmailAddress ea) throws SQLException, IOException {
if(ea.getDomain().getPackage().disable_log==-1) return getEmailForwardings(ea);
else return Collections.emptyList();
}
EmailForwarding getEmailForwarding(EmailAddress ea, String destination) throws IOException, SQLException {
// Use index first
List cached=getEmailForwardings(ea);
int len=cached.size();
for (int c=0;c getEmailForwarding(AOServer ao) throws SQLException, IOException {
int aoPKey=ao.pkey;
List cached = getRows();
int len = cached.size();
List matches=new ArrayList<>(len);
for (int c = 0; c < len; c++) {
EmailForwarding forward = cached.get(c);
if (forward.getEmailAddress().getDomain().ao_server==aoPKey) matches.add(forward);
}
return matches;
}
@Override
public SchemaTable.TableID getTableID() {
return SchemaTable.TableID.EMAIL_FORWARDING;
}
@Override
boolean handleCommand(String[] args, Reader in, TerminalWriter out, TerminalWriter err, boolean isInteractive) throws IllegalArgumentException, IOException, SQLException {
String command=args[0];
if(command.equalsIgnoreCase(AOSHCommand.ADD_EMAIL_FORWARDING)) {
if(AOSH.checkMinParamCount(AOSHCommand.ADD_EMAIL_FORWARDING, args, 3, err)) {
if((args.length%3)!=1) {
err.println("aosh: "+AOSHCommand.ADD_EMAIL_FORWARDING+": must have multiple of three number of parameters");
err.flush();
} else {
for(int c=1;c3) {
out.print(addr);
out.print(": ");
}
out.print("invalid email address: ");
out.println(addr);
} else {
try {
SimpleAOClient.checkEmailForwarding(
addr.substring(0, pos),
addr.substring(pos+1),
args[c+1]
);
if(args.length>3) {
out.print(addr);
out.print(": ");
}
out.println("true");
} catch(IllegalArgumentException ia) {
if(args.length>3) {
out.print(addr);
out.print(": ");
}
out.println(ia.getMessage());
}
}
out.flush();
}
}
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.REMOVE_EMAIL_FORWARDING)) {
if(AOSH.checkParamCount(AOSHCommand.REMOVE_EMAIL_FORWARDING, args, 3, err)) {
String addr=args[1];
int pos=addr.indexOf('@');
if(pos==-1) {
err.print("aosh: "+AOSHCommand.REMOVE_EMAIL_FORWARDING+": invalid email address: ");
err.println(addr);
err.flush();
} else {
connector.getSimpleAOClient().removeEmailForwarding(
addr.substring(0, pos),
AOSH.parseDomainName(addr.substring(pos+1), "domain"),
args[2],
args[3]
);
}
}
return true;
} else return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy