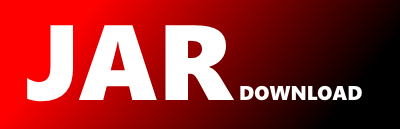
com.aoindustries.aoserv.client.LinuxServerAccountTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aoserv-client Show documentation
Show all versions of aoserv-client Show documentation
Java client for the AOServ Platform.
/*
* aoserv-client - Java client for the AOServ platform.
* Copyright (C) 2001-2013, 2015, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of aoserv-client.
*
* aoserv-client is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* aoserv-client is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with aoserv-client. If not, see .
*/
package com.aoindustries.aoserv.client;
import com.aoindustries.aoserv.client.validator.DomainName;
import com.aoindustries.io.TerminalWriter;
import com.aoindustries.security.AccountDisabledException;
import com.aoindustries.security.BadPasswordException;
import com.aoindustries.security.LoginException;
import com.aoindustries.sql.SQLUtility;
import java.io.IOException;
import java.io.Reader;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @see LinuxServerAccount
*
* @author AO Industries, Inc.
*/
final public class LinuxServerAccountTable extends CachedTableIntegerKey {
LinuxServerAccountTable(AOServConnector connector) {
super(connector, LinuxServerAccount.class);
}
private static final OrderBy[] defaultOrderBy = {
new OrderBy(LinuxServerAccount.COLUMN_USERNAME_name, ASCENDING),
new OrderBy(LinuxServerAccount.COLUMN_AO_SERVER_name+'.'+AOServer.COLUMN_HOSTNAME_name, ASCENDING)
};
@Override
OrderBy[] getDefaultOrderBy() {
return defaultOrderBy;
}
int addLinuxServerAccount(LinuxAccount linuxAccount, AOServer aoServer, String home) throws IOException, SQLException {
int pkey=connector.requestIntQueryIL(
true,
AOServProtocol.CommandID.ADD,
SchemaTable.TableID.LINUX_SERVER_ACCOUNTS,
linuxAccount.pkey,
aoServer.pkey,
home
);
return pkey;
}
@Override
public void clearCache() {
super.clearCache();
synchronized(uidHash) {
uidHashBuilt=false;
}
synchronized(nameHash) {
nameHashBuilt=false;
}
}
@Override
public LinuxServerAccount get(int pkey) throws IOException, SQLException {
return getUniqueRow(LinuxServerAccount.COLUMN_PKEY, pkey);
}
List getAlternateLinuxServerAccounts(LinuxServerGroup group) throws SQLException, IOException {
int aoServer=group.getAOServer().pkey;
String groupName = group.getLinuxGroup().pkey;
List cached = getRows();
int cachedLen = cached.size();
List matches=new ArrayList<>(cachedLen);
for (int c = 0; c < cachedLen; c++) {
LinuxServerAccount linuxServerAccount = cached.get(c);
if(linuxServerAccount.ao_server==aoServer) {
String username = linuxServerAccount.username;
// Must also have a non-primary entry in the LinuxGroupAccounts that is also a group on this server
LinuxGroupAccount linuxGroupAccount = connector.getLinuxGroupAccounts().getLinuxGroupAccount(groupName, username);
if (linuxGroupAccount != null && !linuxGroupAccount.is_primary) matches.add(linuxServerAccount);
}
}
return matches;
}
private boolean nameHashBuilt=false;
private final Map> nameHash=new HashMap<>();
LinuxServerAccount getLinuxServerAccount(AOServer aoServer, String username) throws IOException, SQLException {
synchronized(nameHash) {
if(!nameHashBuilt) {
nameHash.clear();
List list=getRows();
int len=list.size();
for(int c=0; c serverHash=nameHash.get(I);
if(serverHash==null) nameHash.put(I, serverHash=new HashMap<>());
if(serverHash.put(lsa.username, lsa)!=null) throw new SQLException("LinuxServerAccount username exists more than once on server: "+lsa.username+" on "+I);
}
nameHashBuilt=true;
}
Map serverHash=nameHash.get(aoServer.pkey);
if(serverHash==null) return null;
return serverHash.get(username);
}
}
/**
* Finds a LinuxServerAccount by a username and password combination. It tries to return an account that is not disabled and
* matches both username and password.
*
* @return the LinuxServerAccount
found if a non-disabled username and password are found, or null
if no match found
*
* @exception LoginException if a possible account match is found but the account is disabled or has a different password
*/
public LinuxServerAccount getLinuxServerAccountFromUsernamePassword(String username, String password, boolean emailOnly) throws LoginException, IOException, SQLException {
List list = getRows();
LinuxServerAccount badPasswordLSA=null;
LinuxServerAccount disabledLSA=null;
int len = list.size();
for (int c = 0; c < len; c++) {
LinuxServerAccount account=list.get(c);
if(
account.username.equals(username)
&& (!emailOnly || account.getLinuxAccount().getType().isEmail())
) {
if(account.disable_log!=-1) {
if(disabledLSA==null) disabledLSA=account;
} else {
if(account.passwordMatches(password)) return account;
else {
if(badPasswordLSA==null) badPasswordLSA=account;
}
}
}
}
if(badPasswordLSA!=null) throw new BadPasswordException("The password does not match the password for the \""+badPasswordLSA.getLinuxAccount().getUsername().getUsername()+"\" account on the \""+badPasswordLSA.getAOServer().getHostname()+"\" server.");
if(disabledLSA!=null) {
DisableLog dl=disabledLSA.getDisableLog();
String reason=dl==null?null:dl.getDisableReason();
if(reason==null) throw new AccountDisabledException("The \""+disabledLSA.getLinuxAccount().getUsername().getUsername()+"\" account on the \""+disabledLSA.getAOServer().getHostname()+"\" server has been disabled for an unspecified reason.");
else throw new AccountDisabledException("The \""+disabledLSA.getLinuxAccount().getUsername().getUsername()+"\" account on the \""+disabledLSA.getAOServer().getHostname()+"\" server has been disabled for the following reason: "+reason);
}
return null;
}
/**
* Finds a LinuxServerAccount by an email address and password combination. It tries to return an account that is not disabled and
* matches both email address and password.
*
* @return the LinuxServerAccount
found if a non-disabled email address and password are found, or null
if no match found
*
* @exception LoginException if a possible account match is found but the account is disabled or has a different password
*/
public LinuxServerAccount getLinuxServerAccountFromEmailAddress(String address, DomainName domain, String password) throws LoginException, IOException, SQLException {
LinuxServerAccount badPasswordLSA=null;
LinuxServerAccount disabledLSA=null;
List domains=connector.getEmailDomains().getRows();
int domainsLen=domains.size();
for(int c=0;c lsas=ea.getLinuxServerAccounts();
int lsasLen=lsas.size();
for(int d=0;d> uidHash=new HashMap<>();
LinuxServerAccount getLinuxServerAccount(AOServer aoServer, int uid) throws IOException, SQLException {
synchronized(uidHash) {
if(!uidHashBuilt) {
uidHash.clear();
List list = getRows();
int len = list.size();
for (int c = 0; c < len; c++) {
LinuxServerAccount lsa=list.get(c);
int lsaUID=lsa.getUid().getID();
// Only hash the root user for uid of 0
if(lsaUID!=LinuxServerAccount.ROOT_UID || lsa.username.equals(LinuxAccount.ROOT)) {
Integer aoI=lsa.getAOServer().pkey;
Map serverHash=uidHash.get(aoI);
if(serverHash==null) uidHash.put(aoI, serverHash=new HashMap<>());
Integer I=lsaUID;
if(!serverHash.containsKey(I)) serverHash.put(I, lsa);
}
}
uidHashBuilt=true;
}
Map serverHash=uidHash.get(aoServer.pkey);
if(serverHash==null) return null;
return serverHash.get(uid);
}
}
List getLinuxServerAccounts(LinuxAccount linuxAccount) throws IOException, SQLException {
return getIndexedRows(LinuxServerAccount.COLUMN_USERNAME, linuxAccount.pkey);
}
List getLinuxServerAccounts(AOServer aoServer) throws IOException, SQLException {
return getIndexedRows(LinuxServerAccount.COLUMN_AO_SERVER, aoServer.pkey);
}
public List getMailAccounts() throws IOException, SQLException {
List cached = getRows();
int len = cached.size();
List matches=new ArrayList<>(len);
for (int c = 0; c < len; c++) {
LinuxServerAccount lsa=cached.get(c);
if(lsa.getLinuxAccount().getType().isEmail()) matches.add(lsa);
}
return matches;
}
@Override
public SchemaTable.TableID getTableID() {
return SchemaTable.TableID.LINUX_SERVER_ACCOUNTS;
}
@Override
boolean handleCommand(String[] args, Reader in, TerminalWriter out, TerminalWriter err, boolean isInteractive) throws IllegalArgumentException, IOException, SQLException {
String command=args[0];
if(command.equalsIgnoreCase(AOSHCommand.ADD_LINUX_SERVER_ACCOUNT)) {
if(AOSH.checkParamCount(AOSHCommand.ADD_LINUX_SERVER_ACCOUNT, args, 3, err)) {
int pkey=connector.getSimpleAOClient().addLinuxServerAccount(
args[1],
args[2],
args[3]
);
out.println(pkey);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.COMPARE_LINUX_SERVER_ACCOUNT_PASSWORD)) {
if(AOSH.checkParamCount(AOSHCommand.COMPARE_LINUX_SERVER_ACCOUNT_PASSWORD, args, 3, err)) {
boolean result=connector.getSimpleAOClient().compareLinuxServerAccountPassword(
args[1],
args[2],
args[3]
);
out.println(result);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.COPY_HOME_DIRECTORY)) {
if(AOSH.checkParamCount(AOSHCommand.COPY_HOME_DIRECTORY, args, 3, err)) {
long byteCount=connector.getSimpleAOClient().copyHomeDirectory(
args[1],
args[2],
args[3]
);
if(isInteractive) {
out.print(byteCount);
out.println(byteCount==1?" byte":" bytes");
} else out.println(byteCount);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.COPY_LINUX_SERVER_ACCOUNT_PASSWORD)) {
if(AOSH.checkParamCount(AOSHCommand.COPY_LINUX_SERVER_ACCOUNT_PASSWORD, args, 4, err)) {
connector.getSimpleAOClient().copyLinuxServerAccountPassword(
args[1],
args[2],
args[3],
args[4]
);
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.DISABLE_LINUX_SERVER_ACCOUNT)) {
if(AOSH.checkParamCount(AOSHCommand.DISABLE_LINUX_SERVER_ACCOUNT, args, 3, err)) {
out.println(
connector.getSimpleAOClient().disableLinuxServerAccount(
args[1],
args[2],
args[3]
)
);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.ENABLE_LINUX_SERVER_ACCOUNT)) {
if(AOSH.checkParamCount(AOSHCommand.ENABLE_LINUX_SERVER_ACCOUNT, args, 2, err)) {
connector.getSimpleAOClient().enableLinuxServerAccount(args[1], args[2]);
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.GET_CRON_TABLE)) {
if(AOSH.checkParamCount(AOSHCommand.GET_CRON_TABLE, args, 2, err)) {
out.print(
connector.getSimpleAOClient().getCronTable(
args[1],
args[2]
)
);
out.flush();
}
return true;
} else if(command.equalsIgnoreCase(AOSHCommand.GET_IMAP_FOLDER_SIZES)) {
if(AOSH.checkMinParamCount(AOSHCommand.GET_IMAP_FOLDER_SIZES, args, 3, err)) {
String[] folderNames=new String[args.length-3];
System.arraycopy(args, 3, folderNames, 0, folderNames.length);
long[] sizes=connector.getSimpleAOClient().getImapFolderSizes(args[1], args[2], folderNames);
for(int c=0;c cached=getRows();
int size=cached.size();
for(int c=0;c
© 2015 - 2025 Weber Informatics LLC | Privacy Policy